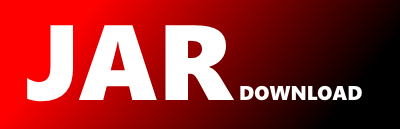
com.tectonica.jonix.onix3.SupplyDetail Maven / Gradle / Ivy
/*
* Copyright (C) 2012-2024 Zach Melamed
*
* Latest version available online at https://github.com/zach-m/jonix
* Contact me at [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tectonica.jonix.onix3;
import com.tectonica.jonix.common.JPU;
import com.tectonica.jonix.common.ListOfOnixComposite;
import com.tectonica.jonix.common.ListOfOnixDataCompositeWithKey;
import com.tectonica.jonix.common.ListOfOnixElement;
import com.tectonica.jonix.common.OnixComposite.OnixSuperComposite;
import com.tectonica.jonix.common.codelist.RecordSourceTypes;
import com.tectonica.jonix.common.codelist.ReturnsConditionsCodeTypes;
import com.tectonica.jonix.common.codelist.SupplierOwnCodeTypes;
import com.tectonica.jonix.common.codelist.SupplyDateRoles;
import com.tectonica.jonix.common.struct.JonixReturnsConditions;
import com.tectonica.jonix.common.struct.JonixSupplierOwnCoding;
import com.tectonica.jonix.common.struct.JonixSupplyDate;
import java.io.Serializable;
import java.util.function.Consumer;
/*
* NOTE: THIS IS AN AUTO-GENERATED FILE, DO NOT EDIT MANUALLY
*/
/**
* Supply detail composite
*
* A group of data elements which together give details of a supply source, and price and availability from that source.
* Mandatory in each occurrence of the <ProductSupply> block and repeatable to give details of multiple supply
* sources.
*
*
*
* Reference name
* <SupplyDetail>
*
*
* Short tag
* <supplydetail>
*
*
* Cardinality
* 1…n
*
*
*
* This tag may be included in the following composites:
*
* - <{@link ProductSupply}>
*
*
* Possible placements within ONIX message:
*
* - {@link Product} ⯈ {@link ProductSupply} ⯈ {@link SupplyDetail}
*
*/
public class SupplyDetail implements OnixSuperComposite, Serializable {
private static final long serialVersionUID = 1L;
public static final String refname = "SupplyDetail";
public static final String shortname = "supplydetail";
/////////////////////////////////////////////////////////////////////////////////
// ATTRIBUTES
/////////////////////////////////////////////////////////////////////////////////
/**
* (type: dt.DateOrDateTime)
*/
public String datestamp;
/**
* (type: dt.NonEmptyString)
*/
public String sourcename;
public RecordSourceTypes sourcetype;
/////////////////////////////////////////////////////////////////////////////////
// CONSTRUCTION
/////////////////////////////////////////////////////////////////////////////////
private boolean initialized;
private final boolean exists;
private final org.w3c.dom.Element element;
public static final SupplyDetail EMPTY = new SupplyDetail();
public SupplyDetail() {
exists = false;
element = null;
initialized = true; // so that no further processing will be done on this intentionally-empty object
}
public SupplyDetail(org.w3c.dom.Element element) {
exists = true;
initialized = false;
this.element = element;
datestamp = JPU.getAttribute(element, "datestamp");
sourcename = JPU.getAttribute(element, "sourcename");
sourcetype = RecordSourceTypes.byCode(JPU.getAttribute(element, "sourcetype"));
}
@Override
public void _initialize() {
if (initialized) {
return;
}
initialized = true;
JPU.forElementsOf(element, e -> {
final String name = e.getNodeName();
switch (name) {
case Supplier.refname:
case Supplier.shortname:
supplier = new Supplier(e);
break;
case ProductAvailability.refname:
case ProductAvailability.shortname:
productAvailability = new ProductAvailability(e);
break;
case OrderTime.refname:
case OrderTime.shortname:
orderTime = new OrderTime(e);
break;
case NewSupplier.refname:
case NewSupplier.shortname:
newSupplier = new NewSupplier(e);
break;
case PackQuantity.refname:
case PackQuantity.shortname:
packQuantity = new PackQuantity(e);
break;
case PalletQuantity.refname:
case PalletQuantity.shortname:
palletQuantity = new PalletQuantity(e);
break;
case OrderQuantityMultiple.refname:
case OrderQuantityMultiple.shortname:
orderQuantityMultiple = new OrderQuantityMultiple(e);
break;
case UnpricedItemType.refname:
case UnpricedItemType.shortname:
unpricedItemType = new UnpricedItemType(e);
break;
case Reissue.refname:
case Reissue.shortname:
reissue = new Reissue(e);
break;
case SupplyContact.refname:
case SupplyContact.shortname:
supplyContacts = JPU.addToList(supplyContacts, new SupplyContact(e));
break;
case SupplierOwnCoding.refname:
case SupplierOwnCoding.shortname:
supplierOwnCodings = JPU.addToList(supplierOwnCodings, new SupplierOwnCoding(e));
break;
case ReturnsConditions.refname:
case ReturnsConditions.shortname:
returnsConditionss = JPU.addToList(returnsConditionss, new ReturnsConditions(e));
break;
case SupplyDate.refname:
case SupplyDate.shortname:
supplyDates = JPU.addToList(supplyDates, new SupplyDate(e));
break;
case Stock.refname:
case Stock.shortname:
stocks = JPU.addToList(stocks, new Stock(e));
break;
case OrderQuantityMinimum.refname:
case OrderQuantityMinimum.shortname:
orderQuantityMinimums = JPU.addToList(orderQuantityMinimums, new OrderQuantityMinimum(e));
break;
case Price.refname:
case Price.shortname:
prices = JPU.addToList(prices, new Price(e));
break;
default:
break;
}
});
}
/**
* @return whether this tag (<SupplyDetail> or <supplydetail>) is explicitly provided in the ONIX XML
*/
@Override
public boolean exists() {
return exists;
}
public void ifExists(Consumer action) {
if (exists) {
action.accept(this);
}
}
@Override
public org.w3c.dom.Element getXmlElement() {
return element;
}
/////////////////////////////////////////////////////////////////////////////////
// MEMBERS
/////////////////////////////////////////////////////////////////////////////////
private Supplier supplier = Supplier.EMPTY;
/**
*
* A group of data elements which together identify a specific supplier. Mandatory in each occurrence of the
* <SupplyDetail> composite, and not repeatable.
*
* Jonix-Comment: this field is required
*/
public Supplier supplier() {
_initialize();
return supplier;
}
private ProductAvailability productAvailability = ProductAvailability.EMPTY;
/**
*
* An ONIX code indicating the availability of a product from a supplier. Mandatory in each occurrence of the
* <SupplyDetail> composite, and non-repeating.
*
* Jonix-Comment: this field is required
*/
public ProductAvailability productAvailability() {
_initialize();
return productAvailability;
}
private OrderTime orderTime = OrderTime.EMPTY;
/**
*
* The expected average number of business days from receipt of order to despatch (for items ‘manufactured on
* demand’ or ‘only to order’). Optional and non-repeating.
*
* Jonix-Comment: this field is optional
*/
public OrderTime orderTime() {
_initialize();
return orderTime;
}
private NewSupplier newSupplier = NewSupplier.EMPTY;
/**
*
* An optional group of data elements which together specify a new supply source to which orders are referred. Use
* only when the code in <ProductAvailability> indicates ‘no longer available from us, refer to new supplier’.
* Only one occurrence of the composite is permitted in this context.
*
* Jonix-Comment: this field is optional
*/
public NewSupplier newSupplier() {
_initialize();
return newSupplier;
}
private PackQuantity packQuantity = PackQuantity.EMPTY;
/**
*
* The quantity in each carton or binder’s pack in stock currently held by the supplier. Optional and non-repeating.
*
*
* Note that orders do not have to be aligned with multiples of the pack quantity, but such orders may be
* more convenient to handle.
*
* Jonix-Comment: this field is optional
*/
public PackQuantity packQuantity() {
_initialize();
return packQuantity;
}
private PalletQuantity palletQuantity = PalletQuantity.EMPTY;
/**
*
* The quantity (number of copies) on each complete pallet in stock currently held by the supplier. Optional and
* non-repeating. Of course, orders do not have to be aligned to the pallet quantity, but for bulk orders, it may be
* useful to know how many pallets will be required for a delivery.
*
* Jonix-Comment: this field is optional
*/
public PalletQuantity palletQuantity() {
_initialize();
return palletQuantity;
}
private OrderQuantityMultiple orderQuantityMultiple = OrderQuantityMultiple.EMPTY;
/**
*
* The order quantity multiple that must be used in any order for the product placed with the supplier. Optional,
* but where supplied, must be preceded by at least one <OrderQuantityMinimum> element. For example with a
* minimum order quantity of 6 and a multiple of 4, orders for 6, 10 or 14 copies are acceptable, but orders for
* fewer than 6, or for 7, 8, 9 or 11 copies are not. If omitted, the minimum or any larger quantity may be ordered.
*
* Jonix-Comment: this field is optional
*/
public OrderQuantityMultiple orderQuantityMultiple() {
_initialize();
return orderQuantityMultiple;
}
private UnpricedItemType unpricedItemType = UnpricedItemType.EMPTY;
/**
*
* An ONIX code which specifies the product is free of charge, or a reason why a price is not sent. If code
* value 02 is used to send advance information without giving a price, the price must be confirmed as soon as
* possible. Optional and non-repeating, but required if the <SupplyDetail> composite does not carry a
* price.
*
*
* Use here in preference to P.26.70b when the product is available only under free of charge or unpriced
* terms from the supplier.
*
* Jonix-Comment: this field is optional
*/
public UnpricedItemType unpricedItemType() {
_initialize();
return unpricedItemType;
}
private Reissue reissue = Reissue.EMPTY;
/**
*
* An optional and non-repeating group of data elements which together specify that a product is to be reissued
* within the market to which the <SupplyDetail> composite applies.
*
*
* The entire <Reissue> composite is deprecated. Suppliers should supply information about planned reissues in
* other parts of the Product record – the date of a planned reissue in <PublishingDate> and/or in
* <MarketDate>, and new collateral material alongside old collateral in
* Block 2 where collateral items may be associated with
* appropriate end and start dates using <ContentDate>.
*
*
* The <Reissue> composite was (prior to deprecation) used only when the publisher intended to re-launch the
* product under the same ISBN. There are two possible cases:
*
*
* - When the product is unavailable during the period immediately before reissue. In this case,
* <ProductAvailability> should carry the value 33 for ‘unavailable, awaiting reissue’, and the ONIX record
* can be updated to describe the reissued product as soon as details can be made available;
* - When the product is still available during the period up to the reissue date. In this case, the ONIX record
* should continue to describe the existing product and the <ProductAvailability> value should continue to
* record the product as ‘available’ (eg code 21) right up to the reissue date. At that date, the record
* should be updated to describe the reissued product, with the <ProductAvailability> value usually remaining
* unchanged.
*
*
* After reissue, the <Reissue> composite can be retained as a permanent element of the ONIX record, carrying
* only the <ReissueDate> element, which will then indicate ‘date last reissued’.
*
* Jonix-Comment: this field is optional
*/
public Reissue reissue() {
_initialize();
return reissue;
}
private ListOfOnixComposite supplyContacts = JPU.emptyListOfOnixComposite(SupplyContact.class);
/**
*
* An optional group of data elements which together specify an organization (which may or may not be the supplier)
* responsible for dealing with enquiries related to the product. Repeatable in order to specify multiple contacts.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixComposite supplyContacts() {
_initialize();
return supplyContacts;
}
private ListOfOnixDataCompositeWithKey supplierOwnCodings = JPU.emptyListOfOnixDataCompositeWithKey(SupplierOwnCoding.class);
/**
*
* An optional and repeatable group of data elements which together allow a supplier to send coded data of a
* specified type, using its own coding schemes.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixDataCompositeWithKey
supplierOwnCodings() {
_initialize();
return supplierOwnCodings;
}
private ListOfOnixDataCompositeWithKey returnsConditionss =
JPU.emptyListOfOnixDataCompositeWithKey(ReturnsConditions.class);
/**
*
* An optional and repeatable group of data elements which together allow the supplier’s returns conditions to be
* specified in coded form.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixDataCompositeWithKey
returnsConditionss() {
_initialize();
return returnsConditionss;
}
private ListOfOnixDataCompositeWithKey supplyDates =
JPU.emptyListOfOnixDataCompositeWithKey(SupplyDate.class);
/**
*
* An optional group of data elements which together specify a date associated with the supply status of the
* product, eg expected ship date. Repeatable in order to specify multiple dates.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixDataCompositeWithKey supplyDates() {
_initialize();
return supplyDates;
}
private ListOfOnixComposite stocks = JPU.emptyListOfOnixComposite(Stock.class);
/**
*
* An optional group of data elements which together specify a quantity of stock, repeatable where a supplier has
* more than one warehouse or supplier location.
*
*
* Within a single instance of the <Stock> composite, the location name and identifier are both optional. If
* <Stock> is repeated, at least one identifier or a location name must be included in each instance.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixComposite stocks() {
_initialize();
return stocks;
}
private ListOfOnixElement orderQuantityMinimums = ListOfOnixElement.empty();
/**
*
* The minimum quantity of the product that may be ordered in a single order placed with the supplier. Optional. If
* omitted, any number may be ordered.
*
*
* If supplied without a succeeding Minimum initial order quantity, the Minimum order quantity applies to all orders
* for the product. If followed by a Minimum initial order quantity, the Minimum order quantity applies to the
* second and subsequent orders for the product.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixElement orderQuantityMinimums() {
_initialize();
return orderQuantityMinimums;
}
private ListOfOnixComposite prices = JPU.emptyListOfOnixComposite(Price.class);
/**
*
* An optional group of data elements which together specify a unit price, repeatable in order to specify multiple
* prices. Each <SupplyDetail> composite must include either one or more prices, or a single
* <UnpricedItemType> element (see P.26.42).
*
*
* Where multiple prices are specified for a product, each price option should specify a distinct combination of its
* terms of trade and the group of end customers it is applicable to, any relevant price conditions, periods of
* time, currency and territory etc, so that the data recipient can clearly select the correct pricing
* option. If, under a particular combination, the product is free of charge or its price is not yet set, an
* <UnpricedItemType> element (P.26.70b) must be used in place of a <PriceAmount>. Each pricing option
* may optionally be given an identifier for use in subsequent revenue reporting or for other internal purposes.
*
* Jonix-Comment: this list may be empty
*/
public ListOfOnixComposite prices() {
_initialize();
return prices;
}
}