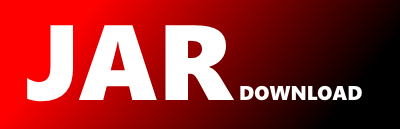
com.tencent.cloud.CosStsClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cos-sts-java Show documentation
Show all versions of cos-sts-java Show documentation
sts java sdk for qcloud cos
package com.tencent.cloud;
import com.tencent.cloud.cos.util.Request;
import org.json.JSONArray;
import org.json.JSONObject;
import java.io.IOException;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
public class CosStsClient {
private static final int DEFAULT_DURATION_SECONDS = 1800;
public static JSONObject getCredential(TreeMap config) throws IOException {
TreeMap params = new TreeMap();
Parameters parameters = new Parameters();
parameters.parse(config);
String policy = parameters.policy;
if (policy != null) {
params.put("Policy", policy);
} else {
params.put("Policy", getPolicy(parameters).toString());
}
params.put("DurationSeconds", parameters.duration);
params.put("Name", "cos-sts-java");
params.put("Action", "GetFederationToken");
params.put("Version", "2018-08-13");
String region = RegionCodeFilter.convert(parameters.region);
params.put("Region", region);
if(parameters.secretType != null) {
params.put("SecretType", parameters.secretType);
}
String host = "sts.tencentcloudapi.com";
String path = "/";
String result = null;
JSONObject jsonResult = null;
try {
result = Request.send(params, (String) parameters.secretId,
parameters.secretKey,
"POST", host, path);
jsonResult = new JSONObject(result);
JSONObject data = jsonResult.optJSONObject("Response");
if (data == null) {
data = jsonResult;
}
long expiredTime = data.getLong("ExpiredTime");
data.put("startTime", expiredTime - parameters.duration);
return downCompat(data);
} catch (Exception e) {
if (jsonResult != null) {
JSONObject response = jsonResult.optJSONObject("Response");
if (response != null) {
JSONObject error = response.optJSONObject("Error");
if (error != null) {
String message = error.optString("Message");
String code = error.optString("Code");
if ("InvalidParameterValue".equals(code) && message != null && message.contains("Region")) {
// Region is not recognized
if (RegionCodeFilter.block(region)) {
return getCredential(config);
}
}
}
}
}
throw new IOException("result = " + result, e);
}
}
public static String getPolicy(List scopes) {
if(scopes == null || scopes.size() == 0)return null;
STSPolicy stsPolicy = new STSPolicy();
stsPolicy.addScope(scopes);
return stsPolicy.toString();
}
// v2接口的key首字母小写,v3改成大写,此处做了向下兼容
private static JSONObject downCompat(JSONObject resultJson) {
JSONObject dcJson = new JSONObject();
for (String key : resultJson.keySet()) {
Object value = resultJson.get(key);
if (value instanceof JSONObject) {
dcJson.put(headerToLowerCase(key), downCompat((JSONObject) value));
} else {
String newKey = "Token".equals(key) ? "sessionToken" : headerToLowerCase(key);
dcJson.put(newKey, resultJson.get(key));
}
}
return dcJson;
}
private static String headerToLowerCase(String source) {
return Character.toLowerCase(source.charAt(0)) + source.substring(1);
}
private static JSONObject getPolicy(Parameters parameters) {
if(parameters.bucket == null) {
throw new NullPointerException("bucket == null");
}
if(parameters.allowPrefix == null) {
throw new NullPointerException("allowPrefix == null");
}
String bucket = parameters.bucket;
String region = parameters.region;
String allowPrefix = parameters.allowPrefix;
if(!allowPrefix.startsWith("/")) {
allowPrefix = "/" + allowPrefix;
}
String[] allowActions = parameters.allowActions;
JSONObject policy = new JSONObject();
policy.put("version", "2.0");
JSONObject statement = new JSONObject();
statement.put("effect", "allow");
JSONArray actions = new JSONArray();
for (String action : allowActions) {
actions.put(action);
}
statement.put("action", actions);
int lastSplit = bucket.lastIndexOf("-");
String appId = bucket.substring(lastSplit + 1);
String resource = String.format("qcs::cos:%s:uid/%s:%s%s",
region, appId, bucket, allowPrefix);
statement.put("resource", resource);
policy.put("statement", statement);
return policy;
}
private static class Parameters{
String secretId;
String secretKey;
int duration = DEFAULT_DURATION_SECONDS;
String bucket;
String region;
String allowPrefix;
String[] allowActions;
String policy;
Integer secretType; // option argument, only can choose 0 or 1, 0 for long certificate, 1 for short certificate
public void parse(Map config) {
if(config == null) throw new NullPointerException("config == null");
for(Map.Entry entry : config.entrySet()) {
String key = entry.getKey();
if("SecretId".equalsIgnoreCase(key)) {
secretId = (String) entry.getValue();
}else if("SecretKey".equalsIgnoreCase(key)) {
secretKey = (String) entry.getValue();
}else if("durationSeconds".equalsIgnoreCase(key)) {
duration = (Integer) entry.getValue();
}else if("bucket".equalsIgnoreCase(key)) {
bucket = (String) entry.getValue();
}else if("region".equalsIgnoreCase(key)) {
region = (String) entry.getValue();
}else if("allowPrefix".equalsIgnoreCase(key)) {
allowPrefix = (String) entry.getValue();
}else if("policy".equalsIgnoreCase(key)) {
policy = (String) entry.getValue();
}else if("allowActions".equalsIgnoreCase(key)) {
allowActions = (String[]) entry.getValue();
}else if("secretType".equalsIgnoreCase(key)) {
secretType = (Integer)entry.getValue();
}
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy