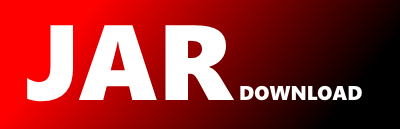
com.tencent.matrix.javalib.util.FileUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of matrix-commons Show documentation
Show all versions of matrix-commons Show documentation
Matrix is an APM (Application Performance Manage) used in Wechat to monitor, locate and analyse performance problems. It is a plugin style, non-invasive solution and is currently available on iOS, macOS and Android.
/*
* Tencent is pleased to support the open source community by making wechat-matrix available.
* Copyright (C) 2018 THL A29 Limited, a Tencent company. All rights reserved.
* Licensed under the BSD 3-Clause License (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://opensource.org/licenses/BSD-3-Clause
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencent.matrix.javalib.util;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.Closeable;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.zip.ZipEntry;
import java.util.zip.ZipException;
import java.util.zip.ZipFile;
import java.util.zip.ZipOutputStream;
/**
* Created by zhangshaowen on 17/7/4.
*/
public class FileUtil {
private static final String TAG = "Matrix.FileUtil";
public static final int BUFFER_SIZE = 16384;
public static final boolean isLegalFile(File file) {
return file != null && file.exists() && file.canRead() && file.isFile() && file.length() > 0;
}
public static final boolean isLegalFile(String filename) {
File file = new File(filename);
return isLegalFile(file);
}
/**
* get directory size
*
* @param directory
* @return
*/
public static long getFileOrDirectorySize(File directory) {
if (directory == null || !directory.exists()) {
return 0;
}
if (directory.isFile()) {
return directory.length();
}
long totalSize = 0;
File[] fileList = directory.listFiles();
if (fileList != null) {
for (File file : fileList) {
if (file.isDirectory()) {
totalSize = totalSize + getFileOrDirectorySize(file);
} else {
totalSize = totalSize + file.length();
}
}
}
return totalSize;
}
public static final boolean safeDeleteFile(File file) {
if (file == null) {
return true;
}
if (file.exists()) {
boolean deleted = file.delete();
if (!deleted) {
Log.e(TAG, "Failed to delete file, try to delete when exit. path: " + file.getPath());
file.deleteOnExit();
}
return deleted;
}
return true;
}
public static final boolean deleteDir(String dir) {
if (dir == null) {
return false;
}
return deleteDir(new File(dir));
}
public static final boolean deleteDir(File file) {
if (file == null || (!file.exists())) {
return false;
}
if (file.isFile()) {
safeDeleteFile(file);
} else if (file.isDirectory()) {
File[] files = file.listFiles();
if (files != null) {
for (File subFile : files) {
deleteDir(subFile);
}
safeDeleteFile(file);
}
}
return true;
}
/**
* Closes the given {@code Closeable}. Suppresses any IO exceptions.
*/
public static void closeQuietly(Closeable closeable) {
try {
if (closeable != null) {
closeable.close();
}
} catch (IOException e) {
Log.w(TAG, "Failed to close resource", e);
}
}
public static void closeZip(ZipFile zipFile) {
try {
if (zipFile != null) {
zipFile.close();
}
} catch (IOException e) {
Log.w(TAG, "Failed to close resource", e);
}
}
public static void ensureFileDirectory(File file) {
if (file == null) {
return;
}
File parentFile = file.getParentFile();
if (!parentFile.exists()) {
parentFile.mkdirs();
}
}
public static void copyResourceUsingStream(String name, File dest) throws IOException {
FileOutputStream os = null;
File parent = dest.getParentFile();
if (parent != null && (!parent.exists())) {
parent.mkdirs();
}
InputStream is = null;
try {
is = FileUtil.class.getResourceAsStream("/" + name);
os = new FileOutputStream(dest, false);
byte[] buffer = new byte[BUFFER_SIZE];
int length;
while ((length = is.read(buffer)) > 0) {
os.write(buffer, 0, length);
}
} finally {
closeQuietly(is);
closeQuietly(os);
}
}
public static void copyFileUsingStream(File source, File dest) throws IOException {
FileInputStream is = null;
FileOutputStream os = null;
File parent = dest.getParentFile();
if (parent != null && (!parent.exists())) {
parent.mkdirs();
}
try {
is = new FileInputStream(source);
os = new FileOutputStream(dest, false);
byte[] buffer = new byte[BUFFER_SIZE];
int length;
while ((length = is.read(buffer)) > 0) {
os.write(buffer, 0, length);
}
} finally {
closeQuietly(is);
closeQuietly(os);
}
}
public static boolean checkDirectory(String dir) {
File dirObj = new File(dir);
deleteDir(dirObj);
if (!dirObj.exists()) {
dirObj.mkdirs();
}
return true;
}
public static String readFileAsString(String filePath) {
StringBuffer fileData = new StringBuffer();
Reader fileReader = null;
InputStream inputStream = null;
try {
inputStream = new FileInputStream(filePath);
fileReader = new InputStreamReader(inputStream, "UTF-8");
char[] buf = new char[BUFFER_SIZE];
int numRead = 0;
while ((numRead = fileReader.read(buf)) != -1) {
String readData = String.valueOf(buf, 0, numRead);
fileData.append(readData);
}
} catch (Exception e) {
Log.e(TAG, "file op readFileAsString e type:%s, e msg:%s, filePath:%s",
e.getClass().getSimpleName(), e.getMessage(), filePath);
} finally {
try {
closeQuietly(fileReader);
closeQuietly(inputStream);
} catch (Exception e) {
Log.e(TAG, "file op readFileAsString close e type:%s, e msg:%s, filePath:%s",
e.getClass().getSimpleName(), e.getMessage(), filePath);
}
}
return fileData.toString();
}
public static void unzip(String filePath, String destFolder) {
ZipFile zipFile = null;
BufferedOutputStream bos = null;
BufferedInputStream bis = null;
try {
zipFile = new ZipFile(filePath);
Enumeration emu = zipFile.entries();
while (emu.hasMoreElements()) {
ZipEntry entry = (ZipEntry) emu.nextElement();
if (entry.isDirectory()) {
new File(destFolder, entry.getName()).mkdirs();
continue;
}
bis = new BufferedInputStream(zipFile.getInputStream(entry));
File file = new File(destFolder, entry.getName());
File parent = file.getParentFile();
if (parent != null && (!parent.exists())) {
parent.mkdirs();
}
byte[] data = new byte[BUFFER_SIZE];
bos = new BufferedOutputStream(new FileOutputStream(file), data.length);
int count;
while ((count = bis.read(data, 0, data.length)) != -1) {
bos.write(data, 0, count);
}
bos.flush();
}
} catch (Exception e) {
// ignore
} finally {
closeQuietly(zipFile);
closeQuietly(bis);
closeQuietly(bos);
}
}
public static void zip(String srcFolder, String destZip) {
FileOutputStream fos = null;
ZipOutputStream zos = null;
try {
File dir = new File(srcFolder);
List filesListInDir = new ArrayList<>();
populateFilesList(filesListInDir, dir);
//now zip files one by one
//create ZipOutputStream to write to the zip file
fos = new FileOutputStream(destZip);
zos = new ZipOutputStream(fos);
for (String filePath : filesListInDir) {
//for ZipEntry we need to keep only relative file path, so we used substring on absolute path
ZipEntry ze = new ZipEntry(filePath.substring(dir.getAbsolutePath().length() + 1, filePath.length()));
zos.putNextEntry(ze);
//read the file and write to ZipOutputStream
FileInputStream fis = new FileInputStream(filePath);
byte[] buffer = new byte[BUFFER_SIZE];
int len;
while ((len = fis.read(buffer)) > 0) {
zos.write(buffer, 0, len);
}
zos.closeEntry();
fis.close();
}
zos.close();
fos.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
closeQuietly(zos);
closeQuietly(fos);
}
}
/**
* This method populates all the files in a directory to a List
*
* @param dir
* @throws IOException
*/
private static void populateFilesList(List filesListInDir, File dir) throws IOException {
File[] files = dir.listFiles();
for (File file : files) {
if (file.isFile()) {
filesListInDir.add(file.getAbsolutePath());
} else {
populateFilesList(filesListInDir, file);
}
}
}
public static void addZipEntry(ZipOutputStream zipOutputStream, ZipEntry zipEntry, InputStream inputStream) throws Exception {
try {
zipOutputStream.putNextEntry(zipEntry);
byte[] buffer = new byte[BUFFER_SIZE];
int length = -1;
while ((length = inputStream.read(buffer, 0, buffer.length)) != -1) {
zipOutputStream.write(buffer, 0, length);
zipOutputStream.flush();
}
} catch (ZipException e) {
Log.e(TAG, "addZipEntry err!");
} finally {
closeQuietly(inputStream);
zipOutputStream.closeEntry();
}
}
public static boolean isClassFile(final String string) {
String regex = "^[\\S|\\s]*.class$";
boolean result = false;
if (string != null && regex != null) {
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(string);
result = matcher.find();
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy