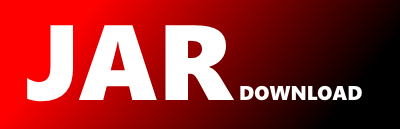
com.tencent.polaris.ratelimit.client.pb.RatelimitV2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of polaris-all Show documentation
Show all versions of polaris-all Show documentation
All in one project for polaris-java
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ratelimit_v2.proto
package com.tencent.polaris.ratelimit.client.pb;
public final class RatelimitV2 {
private RatelimitV2() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
*命令字
*
*
* Protobuf enum {@code polaris.metric.v2.RateLimitCmd}
*/
public enum RateLimitCmd
implements com.google.protobuf.ProtocolMessageEnum {
/**
* INIT = 0;
*/
INIT(0),
/**
* ACQUIRE = 1;
*/
ACQUIRE(1),
UNRECOGNIZED(-1),
;
/**
* INIT = 0;
*/
public static final int INIT_VALUE = 0;
/**
* ACQUIRE = 1;
*/
public static final int ACQUIRE_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static RateLimitCmd valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static RateLimitCmd forNumber(int value) {
switch (value) {
case 0: return INIT;
case 1: return ACQUIRE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
RateLimitCmd> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public RateLimitCmd findValueByNumber(int number) {
return RateLimitCmd.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.getDescriptor().getEnumTypes().get(0);
}
private static final RateLimitCmd[] VALUES = values();
public static RateLimitCmd valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private RateLimitCmd(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:polaris.metric.v2.RateLimitCmd)
}
/**
*
*限频模式
*
*
* Protobuf enum {@code polaris.metric.v2.Mode}
*/
public enum Mode
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*自适应模式,根据历史流量自动调整
*
*
* ADAPTIVE = 0;
*/
ADAPTIVE(0),
/**
*
*批量抢占模式,客户端进行拉取,Server返回全量剩余配额
*
*
* BATCH_OCCUPY = 1;
*/
BATCH_OCCUPY(1),
/**
*
*批量分摊模式,客户端进行拉取,Server按比例进行分摊
*
*
* BATCH_SHARE = 2;
*/
BATCH_SHARE(2),
UNRECOGNIZED(-1),
;
/**
*
*自适应模式,根据历史流量自动调整
*
*
* ADAPTIVE = 0;
*/
public static final int ADAPTIVE_VALUE = 0;
/**
*
*批量抢占模式,客户端进行拉取,Server返回全量剩余配额
*
*
* BATCH_OCCUPY = 1;
*/
public static final int BATCH_OCCUPY_VALUE = 1;
/**
*
*批量分摊模式,客户端进行拉取,Server按比例进行分摊
*
*
* BATCH_SHARE = 2;
*/
public static final int BATCH_SHARE_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Mode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Mode forNumber(int value) {
switch (value) {
case 0: return ADAPTIVE;
case 1: return BATCH_OCCUPY;
case 2: return BATCH_SHARE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Mode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Mode findValueByNumber(int number) {
return Mode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.getDescriptor().getEnumTypes().get(1);
}
private static final Mode[] VALUES = values();
public static Mode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Mode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:polaris.metric.v2.Mode)
}
/**
*
*阈值模式
*
*
* Protobuf enum {@code polaris.metric.v2.QuotaMode}
*/
public enum QuotaMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*整体阈值
*
*
* WHOLE = 0;
*/
WHOLE(0),
/**
*
*单机均分阈值
*
*
* DIVIDE = 1;
*/
DIVIDE(1),
UNRECOGNIZED(-1),
;
/**
*
*整体阈值
*
*
* WHOLE = 0;
*/
public static final int WHOLE_VALUE = 0;
/**
*
*单机均分阈值
*
*
* DIVIDE = 1;
*/
public static final int DIVIDE_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static QuotaMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static QuotaMode forNumber(int value) {
switch (value) {
case 0: return WHOLE;
case 1: return DIVIDE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
QuotaMode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public QuotaMode findValueByNumber(int number) {
return QuotaMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.getDescriptor().getEnumTypes().get(2);
}
private static final QuotaMode[] VALUES = values();
public static QuotaMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private QuotaMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:polaris.metric.v2.QuotaMode)
}
public interface RateLimitRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.RateLimitRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The enum numeric value on the wire for cmd.
*/
int getCmdValue();
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The cmd.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd getCmd();
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
* @return Whether the rateLimitInitRequest field is set.
*/
boolean hasRateLimitInitRequest();
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
* @return The rateLimitInitRequest.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest getRateLimitInitRequest();
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequestOrBuilder getRateLimitInitRequestOrBuilder();
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
* @return Whether the rateLimitReportRequest field is set.
*/
boolean hasRateLimitReportRequest();
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
* @return The rateLimitReportRequest.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest getRateLimitReportRequest();
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequestOrBuilder getRateLimitReportRequestOrBuilder();
}
/**
*
*限流请求
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitRequest}
*/
public static final class RateLimitRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.RateLimitRequest)
RateLimitRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use RateLimitRequest.newBuilder() to construct.
private RateLimitRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RateLimitRequest() {
cmd_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RateLimitRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest.Builder.class);
}
public static final int CMD_FIELD_NUMBER = 1;
private int cmd_;
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The enum numeric value on the wire for cmd.
*/
@java.lang.Override public int getCmdValue() {
return cmd_;
}
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The cmd.
*/
@java.lang.Override public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd getCmd() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.valueOf(cmd_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.UNRECOGNIZED : result;
}
public static final int RATELIMITINITREQUEST_FIELD_NUMBER = 2;
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest rateLimitInitRequest_;
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
* @return Whether the rateLimitInitRequest field is set.
*/
@java.lang.Override
public boolean hasRateLimitInitRequest() {
return rateLimitInitRequest_ != null;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
* @return The rateLimitInitRequest.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest getRateLimitInitRequest() {
return rateLimitInitRequest_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.getDefaultInstance() : rateLimitInitRequest_;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequestOrBuilder getRateLimitInitRequestOrBuilder() {
return getRateLimitInitRequest();
}
public static final int RATELIMITREPORTREQUEST_FIELD_NUMBER = 3;
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest rateLimitReportRequest_;
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
* @return Whether the rateLimitReportRequest field is set.
*/
@java.lang.Override
public boolean hasRateLimitReportRequest() {
return rateLimitReportRequest_ != null;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
* @return The rateLimitReportRequest.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest getRateLimitReportRequest() {
return rateLimitReportRequest_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.getDefaultInstance() : rateLimitReportRequest_;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequestOrBuilder getRateLimitReportRequestOrBuilder() {
return getRateLimitReportRequest();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (cmd_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.INIT.getNumber()) {
output.writeEnum(1, cmd_);
}
if (rateLimitInitRequest_ != null) {
output.writeMessage(2, getRateLimitInitRequest());
}
if (rateLimitReportRequest_ != null) {
output.writeMessage(3, getRateLimitReportRequest());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (cmd_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.INIT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, cmd_);
}
if (rateLimitInitRequest_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRateLimitInitRequest());
}
if (rateLimitReportRequest_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getRateLimitReportRequest());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest) obj;
if (cmd_ != other.cmd_) return false;
if (hasRateLimitInitRequest() != other.hasRateLimitInitRequest()) return false;
if (hasRateLimitInitRequest()) {
if (!getRateLimitInitRequest()
.equals(other.getRateLimitInitRequest())) return false;
}
if (hasRateLimitReportRequest() != other.hasRateLimitReportRequest()) return false;
if (hasRateLimitReportRequest()) {
if (!getRateLimitReportRequest()
.equals(other.getRateLimitReportRequest())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CMD_FIELD_NUMBER;
hash = (53 * hash) + cmd_;
if (hasRateLimitInitRequest()) {
hash = (37 * hash) + RATELIMITINITREQUEST_FIELD_NUMBER;
hash = (53 * hash) + getRateLimitInitRequest().hashCode();
}
if (hasRateLimitReportRequest()) {
hash = (37 * hash) + RATELIMITREPORTREQUEST_FIELD_NUMBER;
hash = (53 * hash) + getRateLimitReportRequest().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*限流请求
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.RateLimitRequest)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
cmd_ = 0;
if (rateLimitInitRequestBuilder_ == null) {
rateLimitInitRequest_ = null;
} else {
rateLimitInitRequest_ = null;
rateLimitInitRequestBuilder_ = null;
}
if (rateLimitReportRequestBuilder_ == null) {
rateLimitReportRequest_ = null;
} else {
rateLimitReportRequest_ = null;
rateLimitReportRequestBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitRequest_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest(this);
result.cmd_ = cmd_;
if (rateLimitInitRequestBuilder_ == null) {
result.rateLimitInitRequest_ = rateLimitInitRequest_;
} else {
result.rateLimitInitRequest_ = rateLimitInitRequestBuilder_.build();
}
if (rateLimitReportRequestBuilder_ == null) {
result.rateLimitReportRequest_ = rateLimitReportRequest_;
} else {
result.rateLimitReportRequest_ = rateLimitReportRequestBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest.getDefaultInstance()) return this;
if (other.cmd_ != 0) {
setCmdValue(other.getCmdValue());
}
if (other.hasRateLimitInitRequest()) {
mergeRateLimitInitRequest(other.getRateLimitInitRequest());
}
if (other.hasRateLimitReportRequest()) {
mergeRateLimitReportRequest(other.getRateLimitReportRequest());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
cmd_ = input.readEnum();
break;
} // case 8
case 18: {
input.readMessage(
getRateLimitInitRequestFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 18
case 26: {
input.readMessage(
getRateLimitReportRequestFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int cmd_ = 0;
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The enum numeric value on the wire for cmd.
*/
@java.lang.Override public int getCmdValue() {
return cmd_;
}
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @param value The enum numeric value on the wire for cmd to set.
* @return This builder for chaining.
*/
public Builder setCmdValue(int value) {
cmd_ = value;
onChanged();
return this;
}
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The cmd.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd getCmd() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.valueOf(cmd_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.UNRECOGNIZED : result;
}
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @param value The cmd to set.
* @return This builder for chaining.
*/
public Builder setCmd(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd value) {
if (value == null) {
throw new NullPointerException();
}
cmd_ = value.getNumber();
onChanged();
return this;
}
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return This builder for chaining.
*/
public Builder clearCmd() {
cmd_ = 0;
onChanged();
return this;
}
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest rateLimitInitRequest_;
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequestOrBuilder> rateLimitInitRequestBuilder_;
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
* @return Whether the rateLimitInitRequest field is set.
*/
public boolean hasRateLimitInitRequest() {
return rateLimitInitRequestBuilder_ != null || rateLimitInitRequest_ != null;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
* @return The rateLimitInitRequest.
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest getRateLimitInitRequest() {
if (rateLimitInitRequestBuilder_ == null) {
return rateLimitInitRequest_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.getDefaultInstance() : rateLimitInitRequest_;
} else {
return rateLimitInitRequestBuilder_.getMessage();
}
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
*/
public Builder setRateLimitInitRequest(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest value) {
if (rateLimitInitRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rateLimitInitRequest_ = value;
onChanged();
} else {
rateLimitInitRequestBuilder_.setMessage(value);
}
return this;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
*/
public Builder setRateLimitInitRequest(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.Builder builderForValue) {
if (rateLimitInitRequestBuilder_ == null) {
rateLimitInitRequest_ = builderForValue.build();
onChanged();
} else {
rateLimitInitRequestBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
*/
public Builder mergeRateLimitInitRequest(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest value) {
if (rateLimitInitRequestBuilder_ == null) {
if (rateLimitInitRequest_ != null) {
rateLimitInitRequest_ =
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.newBuilder(rateLimitInitRequest_).mergeFrom(value).buildPartial();
} else {
rateLimitInitRequest_ = value;
}
onChanged();
} else {
rateLimitInitRequestBuilder_.mergeFrom(value);
}
return this;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
*/
public Builder clearRateLimitInitRequest() {
if (rateLimitInitRequestBuilder_ == null) {
rateLimitInitRequest_ = null;
onChanged();
} else {
rateLimitInitRequest_ = null;
rateLimitInitRequestBuilder_ = null;
}
return this;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.Builder getRateLimitInitRequestBuilder() {
onChanged();
return getRateLimitInitRequestFieldBuilder().getBuilder();
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequestOrBuilder getRateLimitInitRequestOrBuilder() {
if (rateLimitInitRequestBuilder_ != null) {
return rateLimitInitRequestBuilder_.getMessageOrBuilder();
} else {
return rateLimitInitRequest_ == null ?
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.getDefaultInstance() : rateLimitInitRequest_;
}
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitRequest rateLimitInitRequest = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequestOrBuilder>
getRateLimitInitRequestFieldBuilder() {
if (rateLimitInitRequestBuilder_ == null) {
rateLimitInitRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequestOrBuilder>(
getRateLimitInitRequest(),
getParentForChildren(),
isClean());
rateLimitInitRequest_ = null;
}
return rateLimitInitRequestBuilder_;
}
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest rateLimitReportRequest_;
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequestOrBuilder> rateLimitReportRequestBuilder_;
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
* @return Whether the rateLimitReportRequest field is set.
*/
public boolean hasRateLimitReportRequest() {
return rateLimitReportRequestBuilder_ != null || rateLimitReportRequest_ != null;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
* @return The rateLimitReportRequest.
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest getRateLimitReportRequest() {
if (rateLimitReportRequestBuilder_ == null) {
return rateLimitReportRequest_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.getDefaultInstance() : rateLimitReportRequest_;
} else {
return rateLimitReportRequestBuilder_.getMessage();
}
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
*/
public Builder setRateLimitReportRequest(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest value) {
if (rateLimitReportRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rateLimitReportRequest_ = value;
onChanged();
} else {
rateLimitReportRequestBuilder_.setMessage(value);
}
return this;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
*/
public Builder setRateLimitReportRequest(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.Builder builderForValue) {
if (rateLimitReportRequestBuilder_ == null) {
rateLimitReportRequest_ = builderForValue.build();
onChanged();
} else {
rateLimitReportRequestBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
*/
public Builder mergeRateLimitReportRequest(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest value) {
if (rateLimitReportRequestBuilder_ == null) {
if (rateLimitReportRequest_ != null) {
rateLimitReportRequest_ =
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.newBuilder(rateLimitReportRequest_).mergeFrom(value).buildPartial();
} else {
rateLimitReportRequest_ = value;
}
onChanged();
} else {
rateLimitReportRequestBuilder_.mergeFrom(value);
}
return this;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
*/
public Builder clearRateLimitReportRequest() {
if (rateLimitReportRequestBuilder_ == null) {
rateLimitReportRequest_ = null;
onChanged();
} else {
rateLimitReportRequest_ = null;
rateLimitReportRequestBuilder_ = null;
}
return this;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.Builder getRateLimitReportRequestBuilder() {
onChanged();
return getRateLimitReportRequestFieldBuilder().getBuilder();
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequestOrBuilder getRateLimitReportRequestOrBuilder() {
if (rateLimitReportRequestBuilder_ != null) {
return rateLimitReportRequestBuilder_.getMessageOrBuilder();
} else {
return rateLimitReportRequest_ == null ?
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.getDefaultInstance() : rateLimitReportRequest_;
}
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportRequest rateLimitReportRequest = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequestOrBuilder>
getRateLimitReportRequestFieldBuilder() {
if (rateLimitReportRequestBuilder_ == null) {
rateLimitReportRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequestOrBuilder>(
getRateLimitReportRequest(),
getParentForChildren(),
isClean());
rateLimitReportRequest_ = null;
}
return rateLimitReportRequestBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.RateLimitRequest)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.RateLimitRequest)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RateLimitRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RateLimitResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.RateLimitResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The enum numeric value on the wire for cmd.
*/
int getCmdValue();
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The cmd.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd getCmd();
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
* @return Whether the rateLimitInitResponse field is set.
*/
boolean hasRateLimitInitResponse();
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
* @return The rateLimitInitResponse.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse getRateLimitInitResponse();
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponseOrBuilder getRateLimitInitResponseOrBuilder();
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
* @return Whether the rateLimitReportResponse field is set.
*/
boolean hasRateLimitReportResponse();
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
* @return The rateLimitReportResponse.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse getRateLimitReportResponse();
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponseOrBuilder getRateLimitReportResponseOrBuilder();
}
/**
*
*限流应答
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitResponse}
*/
public static final class RateLimitResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.RateLimitResponse)
RateLimitResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RateLimitResponse.newBuilder() to construct.
private RateLimitResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RateLimitResponse() {
cmd_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RateLimitResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse.Builder.class);
}
public static final int CMD_FIELD_NUMBER = 1;
private int cmd_;
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The enum numeric value on the wire for cmd.
*/
@java.lang.Override public int getCmdValue() {
return cmd_;
}
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The cmd.
*/
@java.lang.Override public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd getCmd() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.valueOf(cmd_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.UNRECOGNIZED : result;
}
public static final int RATELIMITINITRESPONSE_FIELD_NUMBER = 2;
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse rateLimitInitResponse_;
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
* @return Whether the rateLimitInitResponse field is set.
*/
@java.lang.Override
public boolean hasRateLimitInitResponse() {
return rateLimitInitResponse_ != null;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
* @return The rateLimitInitResponse.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse getRateLimitInitResponse() {
return rateLimitInitResponse_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.getDefaultInstance() : rateLimitInitResponse_;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponseOrBuilder getRateLimitInitResponseOrBuilder() {
return getRateLimitInitResponse();
}
public static final int RATELIMITREPORTRESPONSE_FIELD_NUMBER = 3;
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse rateLimitReportResponse_;
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
* @return Whether the rateLimitReportResponse field is set.
*/
@java.lang.Override
public boolean hasRateLimitReportResponse() {
return rateLimitReportResponse_ != null;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
* @return The rateLimitReportResponse.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse getRateLimitReportResponse() {
return rateLimitReportResponse_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.getDefaultInstance() : rateLimitReportResponse_;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponseOrBuilder getRateLimitReportResponseOrBuilder() {
return getRateLimitReportResponse();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (cmd_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.INIT.getNumber()) {
output.writeEnum(1, cmd_);
}
if (rateLimitInitResponse_ != null) {
output.writeMessage(2, getRateLimitInitResponse());
}
if (rateLimitReportResponse_ != null) {
output.writeMessage(3, getRateLimitReportResponse());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (cmd_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.INIT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, cmd_);
}
if (rateLimitInitResponse_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRateLimitInitResponse());
}
if (rateLimitReportResponse_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getRateLimitReportResponse());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse) obj;
if (cmd_ != other.cmd_) return false;
if (hasRateLimitInitResponse() != other.hasRateLimitInitResponse()) return false;
if (hasRateLimitInitResponse()) {
if (!getRateLimitInitResponse()
.equals(other.getRateLimitInitResponse())) return false;
}
if (hasRateLimitReportResponse() != other.hasRateLimitReportResponse()) return false;
if (hasRateLimitReportResponse()) {
if (!getRateLimitReportResponse()
.equals(other.getRateLimitReportResponse())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CMD_FIELD_NUMBER;
hash = (53 * hash) + cmd_;
if (hasRateLimitInitResponse()) {
hash = (37 * hash) + RATELIMITINITRESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getRateLimitInitResponse().hashCode();
}
if (hasRateLimitReportResponse()) {
hash = (37 * hash) + RATELIMITREPORTRESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getRateLimitReportResponse().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*限流应答
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.RateLimitResponse)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
cmd_ = 0;
if (rateLimitInitResponseBuilder_ == null) {
rateLimitInitResponse_ = null;
} else {
rateLimitInitResponse_ = null;
rateLimitInitResponseBuilder_ = null;
}
if (rateLimitReportResponseBuilder_ == null) {
rateLimitReportResponse_ = null;
} else {
rateLimitReportResponse_ = null;
rateLimitReportResponseBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitResponse_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse(this);
result.cmd_ = cmd_;
if (rateLimitInitResponseBuilder_ == null) {
result.rateLimitInitResponse_ = rateLimitInitResponse_;
} else {
result.rateLimitInitResponse_ = rateLimitInitResponseBuilder_.build();
}
if (rateLimitReportResponseBuilder_ == null) {
result.rateLimitReportResponse_ = rateLimitReportResponse_;
} else {
result.rateLimitReportResponse_ = rateLimitReportResponseBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse.getDefaultInstance()) return this;
if (other.cmd_ != 0) {
setCmdValue(other.getCmdValue());
}
if (other.hasRateLimitInitResponse()) {
mergeRateLimitInitResponse(other.getRateLimitInitResponse());
}
if (other.hasRateLimitReportResponse()) {
mergeRateLimitReportResponse(other.getRateLimitReportResponse());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
cmd_ = input.readEnum();
break;
} // case 8
case 18: {
input.readMessage(
getRateLimitInitResponseFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 18
case 26: {
input.readMessage(
getRateLimitReportResponseFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int cmd_ = 0;
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The enum numeric value on the wire for cmd.
*/
@java.lang.Override public int getCmdValue() {
return cmd_;
}
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @param value The enum numeric value on the wire for cmd to set.
* @return This builder for chaining.
*/
public Builder setCmdValue(int value) {
cmd_ = value;
onChanged();
return this;
}
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return The cmd.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd getCmd() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.valueOf(cmd_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd.UNRECOGNIZED : result;
}
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @param value The cmd to set.
* @return This builder for chaining.
*/
public Builder setCmd(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitCmd value) {
if (value == null) {
throw new NullPointerException();
}
cmd_ = value.getNumber();
onChanged();
return this;
}
/**
*
*命令字
*
*
* .polaris.metric.v2.RateLimitCmd cmd = 1;
* @return This builder for chaining.
*/
public Builder clearCmd() {
cmd_ = 0;
onChanged();
return this;
}
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse rateLimitInitResponse_;
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponseOrBuilder> rateLimitInitResponseBuilder_;
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
* @return Whether the rateLimitInitResponse field is set.
*/
public boolean hasRateLimitInitResponse() {
return rateLimitInitResponseBuilder_ != null || rateLimitInitResponse_ != null;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
* @return The rateLimitInitResponse.
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse getRateLimitInitResponse() {
if (rateLimitInitResponseBuilder_ == null) {
return rateLimitInitResponse_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.getDefaultInstance() : rateLimitInitResponse_;
} else {
return rateLimitInitResponseBuilder_.getMessage();
}
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
*/
public Builder setRateLimitInitResponse(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse value) {
if (rateLimitInitResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rateLimitInitResponse_ = value;
onChanged();
} else {
rateLimitInitResponseBuilder_.setMessage(value);
}
return this;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
*/
public Builder setRateLimitInitResponse(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.Builder builderForValue) {
if (rateLimitInitResponseBuilder_ == null) {
rateLimitInitResponse_ = builderForValue.build();
onChanged();
} else {
rateLimitInitResponseBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
*/
public Builder mergeRateLimitInitResponse(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse value) {
if (rateLimitInitResponseBuilder_ == null) {
if (rateLimitInitResponse_ != null) {
rateLimitInitResponse_ =
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.newBuilder(rateLimitInitResponse_).mergeFrom(value).buildPartial();
} else {
rateLimitInitResponse_ = value;
}
onChanged();
} else {
rateLimitInitResponseBuilder_.mergeFrom(value);
}
return this;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
*/
public Builder clearRateLimitInitResponse() {
if (rateLimitInitResponseBuilder_ == null) {
rateLimitInitResponse_ = null;
onChanged();
} else {
rateLimitInitResponse_ = null;
rateLimitInitResponseBuilder_ = null;
}
return this;
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.Builder getRateLimitInitResponseBuilder() {
onChanged();
return getRateLimitInitResponseFieldBuilder().getBuilder();
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponseOrBuilder getRateLimitInitResponseOrBuilder() {
if (rateLimitInitResponseBuilder_ != null) {
return rateLimitInitResponseBuilder_.getMessageOrBuilder();
} else {
return rateLimitInitResponse_ == null ?
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.getDefaultInstance() : rateLimitInitResponse_;
}
}
/**
*
*初始化请求
*
*
* .polaris.metric.v2.RateLimitInitResponse rateLimitInitResponse = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponseOrBuilder>
getRateLimitInitResponseFieldBuilder() {
if (rateLimitInitResponseBuilder_ == null) {
rateLimitInitResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponseOrBuilder>(
getRateLimitInitResponse(),
getParentForChildren(),
isClean());
rateLimitInitResponse_ = null;
}
return rateLimitInitResponseBuilder_;
}
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse rateLimitReportResponse_;
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponseOrBuilder> rateLimitReportResponseBuilder_;
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
* @return Whether the rateLimitReportResponse field is set.
*/
public boolean hasRateLimitReportResponse() {
return rateLimitReportResponseBuilder_ != null || rateLimitReportResponse_ != null;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
* @return The rateLimitReportResponse.
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse getRateLimitReportResponse() {
if (rateLimitReportResponseBuilder_ == null) {
return rateLimitReportResponse_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.getDefaultInstance() : rateLimitReportResponse_;
} else {
return rateLimitReportResponseBuilder_.getMessage();
}
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
*/
public Builder setRateLimitReportResponse(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse value) {
if (rateLimitReportResponseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rateLimitReportResponse_ = value;
onChanged();
} else {
rateLimitReportResponseBuilder_.setMessage(value);
}
return this;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
*/
public Builder setRateLimitReportResponse(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.Builder builderForValue) {
if (rateLimitReportResponseBuilder_ == null) {
rateLimitReportResponse_ = builderForValue.build();
onChanged();
} else {
rateLimitReportResponseBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
*/
public Builder mergeRateLimitReportResponse(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse value) {
if (rateLimitReportResponseBuilder_ == null) {
if (rateLimitReportResponse_ != null) {
rateLimitReportResponse_ =
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.newBuilder(rateLimitReportResponse_).mergeFrom(value).buildPartial();
} else {
rateLimitReportResponse_ = value;
}
onChanged();
} else {
rateLimitReportResponseBuilder_.mergeFrom(value);
}
return this;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
*/
public Builder clearRateLimitReportResponse() {
if (rateLimitReportResponseBuilder_ == null) {
rateLimitReportResponse_ = null;
onChanged();
} else {
rateLimitReportResponse_ = null;
rateLimitReportResponseBuilder_ = null;
}
return this;
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.Builder getRateLimitReportResponseBuilder() {
onChanged();
return getRateLimitReportResponseFieldBuilder().getBuilder();
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponseOrBuilder getRateLimitReportResponseOrBuilder() {
if (rateLimitReportResponseBuilder_ != null) {
return rateLimitReportResponseBuilder_.getMessageOrBuilder();
} else {
return rateLimitReportResponse_ == null ?
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.getDefaultInstance() : rateLimitReportResponse_;
}
}
/**
*
*上报请求
*
*
* .polaris.metric.v2.RateLimitReportResponse rateLimitReportResponse = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponseOrBuilder>
getRateLimitReportResponseFieldBuilder() {
if (rateLimitReportResponseBuilder_ == null) {
rateLimitReportResponseBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponseOrBuilder>(
getRateLimitReportResponse(),
getParentForChildren(),
isClean());
rateLimitReportResponse_ = null;
}
return rateLimitReportResponseBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.RateLimitResponse)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.RateLimitResponse)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RateLimitResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RateLimitInitRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.RateLimitInitRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
* @return Whether the target field is set.
*/
boolean hasTarget();
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
* @return The target.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget getTarget();
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder getTargetOrBuilder();
/**
*
*客户端唯一标识
*
*
* string clientId = 2;
* @return The clientId.
*/
java.lang.String getClientId();
/**
*
*客户端唯一标识
*
*
* string clientId = 2;
* @return The bytes for clientId.
*/
com.google.protobuf.ByteString
getClientIdBytes();
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
java.util.List
getTotalsList();
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal getTotals(int index);
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
int getTotalsCount();
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotalOrBuilder>
getTotalsOrBuilderList();
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotalOrBuilder getTotalsOrBuilder(
int index);
/**
*
*客户端可指定滑窗数,不指定用默认值
*
*
* uint32 slideCount = 4;
* @return The slideCount.
*/
int getSlideCount();
/**
*
*限流模式
*
*
* .polaris.metric.v2.Mode mode = 5;
* @return The enum numeric value on the wire for mode.
*/
int getModeValue();
/**
*
*限流模式
*
*
* .polaris.metric.v2.Mode mode = 5;
* @return The mode.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode getMode();
}
/**
*
*初始化请求
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitInitRequest}
*/
public static final class RateLimitInitRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.RateLimitInitRequest)
RateLimitInitRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use RateLimitInitRequest.newBuilder() to construct.
private RateLimitInitRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RateLimitInitRequest() {
clientId_ = "";
totals_ = java.util.Collections.emptyList();
mode_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RateLimitInitRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitInitRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitInitRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.Builder.class);
}
public static final int TARGET_FIELD_NUMBER = 1;
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget target_;
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
* @return Whether the target field is set.
*/
@java.lang.Override
public boolean hasTarget() {
return target_ != null;
}
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
* @return The target.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget getTarget() {
return target_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.getDefaultInstance() : target_;
}
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder getTargetOrBuilder() {
return getTarget();
}
public static final int CLIENTID_FIELD_NUMBER = 2;
private volatile java.lang.Object clientId_;
/**
*
*客户端唯一标识
*
*
* string clientId = 2;
* @return The clientId.
*/
@java.lang.Override
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
}
}
/**
*
*客户端唯一标识
*
*
* string clientId = 2;
* @return The bytes for clientId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TOTALS_FIELD_NUMBER = 3;
private java.util.List totals_;
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
@java.lang.Override
public java.util.List getTotalsList() {
return totals_;
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
@java.lang.Override
public java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotalOrBuilder>
getTotalsOrBuilderList() {
return totals_;
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
@java.lang.Override
public int getTotalsCount() {
return totals_.size();
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal getTotals(int index) {
return totals_.get(index);
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotalOrBuilder getTotalsOrBuilder(
int index) {
return totals_.get(index);
}
public static final int SLIDECOUNT_FIELD_NUMBER = 4;
private int slideCount_;
/**
*
*客户端可指定滑窗数,不指定用默认值
*
*
* uint32 slideCount = 4;
* @return The slideCount.
*/
@java.lang.Override
public int getSlideCount() {
return slideCount_;
}
public static final int MODE_FIELD_NUMBER = 5;
private int mode_;
/**
*
*限流模式
*
*
* .polaris.metric.v2.Mode mode = 5;
* @return The enum numeric value on the wire for mode.
*/
@java.lang.Override public int getModeValue() {
return mode_;
}
/**
*
*限流模式
*
*
* .polaris.metric.v2.Mode mode = 5;
* @return The mode.
*/
@java.lang.Override public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode getMode() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.valueOf(mode_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (target_ != null) {
output.writeMessage(1, getTarget());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(clientId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, clientId_);
}
for (int i = 0; i < totals_.size(); i++) {
output.writeMessage(3, totals_.get(i));
}
if (slideCount_ != 0) {
output.writeUInt32(4, slideCount_);
}
if (mode_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.ADAPTIVE.getNumber()) {
output.writeEnum(5, mode_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (target_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTarget());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(clientId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, clientId_);
}
for (int i = 0; i < totals_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, totals_.get(i));
}
if (slideCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, slideCount_);
}
if (mode_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.ADAPTIVE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, mode_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest) obj;
if (hasTarget() != other.hasTarget()) return false;
if (hasTarget()) {
if (!getTarget()
.equals(other.getTarget())) return false;
}
if (!getClientId()
.equals(other.getClientId())) return false;
if (!getTotalsList()
.equals(other.getTotalsList())) return false;
if (getSlideCount()
!= other.getSlideCount()) return false;
if (mode_ != other.mode_) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTarget()) {
hash = (37 * hash) + TARGET_FIELD_NUMBER;
hash = (53 * hash) + getTarget().hashCode();
}
hash = (37 * hash) + CLIENTID_FIELD_NUMBER;
hash = (53 * hash) + getClientId().hashCode();
if (getTotalsCount() > 0) {
hash = (37 * hash) + TOTALS_FIELD_NUMBER;
hash = (53 * hash) + getTotalsList().hashCode();
}
hash = (37 * hash) + SLIDECOUNT_FIELD_NUMBER;
hash = (53 * hash) + getSlideCount();
hash = (37 * hash) + MODE_FIELD_NUMBER;
hash = (53 * hash) + mode_;
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*初始化请求
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitInitRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.RateLimitInitRequest)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitInitRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitInitRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
if (targetBuilder_ == null) {
target_ = null;
} else {
target_ = null;
targetBuilder_ = null;
}
clientId_ = "";
if (totalsBuilder_ == null) {
totals_ = java.util.Collections.emptyList();
} else {
totals_ = null;
totalsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
slideCount_ = 0;
mode_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitInitRequest_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest(this);
int from_bitField0_ = bitField0_;
if (targetBuilder_ == null) {
result.target_ = target_;
} else {
result.target_ = targetBuilder_.build();
}
result.clientId_ = clientId_;
if (totalsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
totals_ = java.util.Collections.unmodifiableList(totals_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.totals_ = totals_;
} else {
result.totals_ = totalsBuilder_.build();
}
result.slideCount_ = slideCount_;
result.mode_ = mode_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest.getDefaultInstance()) return this;
if (other.hasTarget()) {
mergeTarget(other.getTarget());
}
if (!other.getClientId().isEmpty()) {
clientId_ = other.clientId_;
onChanged();
}
if (totalsBuilder_ == null) {
if (!other.totals_.isEmpty()) {
if (totals_.isEmpty()) {
totals_ = other.totals_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTotalsIsMutable();
totals_.addAll(other.totals_);
}
onChanged();
}
} else {
if (!other.totals_.isEmpty()) {
if (totalsBuilder_.isEmpty()) {
totalsBuilder_.dispose();
totalsBuilder_ = null;
totals_ = other.totals_;
bitField0_ = (bitField0_ & ~0x00000001);
totalsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTotalsFieldBuilder() : null;
} else {
totalsBuilder_.addAllMessages(other.totals_);
}
}
}
if (other.getSlideCount() != 0) {
setSlideCount(other.getSlideCount());
}
if (other.mode_ != 0) {
setModeValue(other.getModeValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getTargetFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 10
case 18: {
clientId_ = input.readStringRequireUtf8();
break;
} // case 18
case 26: {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal m =
input.readMessage(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.parser(),
extensionRegistry);
if (totalsBuilder_ == null) {
ensureTotalsIsMutable();
totals_.add(m);
} else {
totalsBuilder_.addMessage(m);
}
break;
} // case 26
case 32: {
slideCount_ = input.readUInt32();
break;
} // case 32
case 40: {
mode_ = input.readEnum();
break;
} // case 40
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget target_;
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder> targetBuilder_;
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
* @return Whether the target field is set.
*/
public boolean hasTarget() {
return targetBuilder_ != null || target_ != null;
}
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
* @return The target.
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget getTarget() {
if (targetBuilder_ == null) {
return target_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.getDefaultInstance() : target_;
} else {
return targetBuilder_.getMessage();
}
}
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
*/
public Builder setTarget(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget value) {
if (targetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
target_ = value;
onChanged();
} else {
targetBuilder_.setMessage(value);
}
return this;
}
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
*/
public Builder setTarget(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder builderForValue) {
if (targetBuilder_ == null) {
target_ = builderForValue.build();
onChanged();
} else {
targetBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
*/
public Builder mergeTarget(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget value) {
if (targetBuilder_ == null) {
if (target_ != null) {
target_ =
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.newBuilder(target_).mergeFrom(value).buildPartial();
} else {
target_ = value;
}
onChanged();
} else {
targetBuilder_.mergeFrom(value);
}
return this;
}
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
*/
public Builder clearTarget() {
if (targetBuilder_ == null) {
target_ = null;
onChanged();
} else {
target_ = null;
targetBuilder_ = null;
}
return this;
}
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder getTargetBuilder() {
onChanged();
return getTargetFieldBuilder().getBuilder();
}
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder getTargetOrBuilder() {
if (targetBuilder_ != null) {
return targetBuilder_.getMessageOrBuilder();
} else {
return target_ == null ?
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.getDefaultInstance() : target_;
}
}
/**
*
*限流目标对象数据
*
*
* .polaris.metric.v2.LimitTarget target = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder>
getTargetFieldBuilder() {
if (targetBuilder_ == null) {
targetBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder>(
getTarget(),
getParentForChildren(),
isClean());
target_ = null;
}
return targetBuilder_;
}
private java.lang.Object clientId_ = "";
/**
*
*客户端唯一标识
*
*
* string clientId = 2;
* @return The clientId.
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*客户端唯一标识
*
*
* string clientId = 2;
* @return The bytes for clientId.
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*客户端唯一标识
*
*
* string clientId = 2;
* @param value The clientId to set.
* @return This builder for chaining.
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
clientId_ = value;
onChanged();
return this;
}
/**
*
*客户端唯一标识
*
*
* string clientId = 2;
* @return This builder for chaining.
*/
public Builder clearClientId() {
clientId_ = getDefaultInstance().getClientId();
onChanged();
return this;
}
/**
*
*客户端唯一标识
*
*
* string clientId = 2;
* @param value The bytes for clientId to set.
* @return This builder for chaining.
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
clientId_ = value;
onChanged();
return this;
}
private java.util.List totals_ =
java.util.Collections.emptyList();
private void ensureTotalsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
totals_ = new java.util.ArrayList(totals_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotalOrBuilder> totalsBuilder_;
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public java.util.List getTotalsList() {
if (totalsBuilder_ == null) {
return java.util.Collections.unmodifiableList(totals_);
} else {
return totalsBuilder_.getMessageList();
}
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public int getTotalsCount() {
if (totalsBuilder_ == null) {
return totals_.size();
} else {
return totalsBuilder_.getCount();
}
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal getTotals(int index) {
if (totalsBuilder_ == null) {
return totals_.get(index);
} else {
return totalsBuilder_.getMessage(index);
}
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public Builder setTotals(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal value) {
if (totalsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTotalsIsMutable();
totals_.set(index, value);
onChanged();
} else {
totalsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public Builder setTotals(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.Builder builderForValue) {
if (totalsBuilder_ == null) {
ensureTotalsIsMutable();
totals_.set(index, builderForValue.build());
onChanged();
} else {
totalsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public Builder addTotals(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal value) {
if (totalsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTotalsIsMutable();
totals_.add(value);
onChanged();
} else {
totalsBuilder_.addMessage(value);
}
return this;
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public Builder addTotals(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal value) {
if (totalsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTotalsIsMutable();
totals_.add(index, value);
onChanged();
} else {
totalsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public Builder addTotals(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.Builder builderForValue) {
if (totalsBuilder_ == null) {
ensureTotalsIsMutable();
totals_.add(builderForValue.build());
onChanged();
} else {
totalsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public Builder addTotals(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.Builder builderForValue) {
if (totalsBuilder_ == null) {
ensureTotalsIsMutable();
totals_.add(index, builderForValue.build());
onChanged();
} else {
totalsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public Builder addAllTotals(
java.lang.Iterable extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal> values) {
if (totalsBuilder_ == null) {
ensureTotalsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, totals_);
onChanged();
} else {
totalsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public Builder clearTotals() {
if (totalsBuilder_ == null) {
totals_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
totalsBuilder_.clear();
}
return this;
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public Builder removeTotals(int index) {
if (totalsBuilder_ == null) {
ensureTotalsIsMutable();
totals_.remove(index);
onChanged();
} else {
totalsBuilder_.remove(index);
}
return this;
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.Builder getTotalsBuilder(
int index) {
return getTotalsFieldBuilder().getBuilder(index);
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotalOrBuilder getTotalsOrBuilder(
int index) {
if (totalsBuilder_ == null) {
return totals_.get(index); } else {
return totalsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotalOrBuilder>
getTotalsOrBuilderList() {
if (totalsBuilder_ != null) {
return totalsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(totals_);
}
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.Builder addTotalsBuilder() {
return getTotalsFieldBuilder().addBuilder(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.getDefaultInstance());
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.Builder addTotalsBuilder(
int index) {
return getTotalsFieldBuilder().addBuilder(
index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.getDefaultInstance());
}
/**
*
*限流规则信息
*
*
* repeated .polaris.metric.v2.QuotaTotal totals = 3;
*/
public java.util.List
getTotalsBuilderList() {
return getTotalsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotalOrBuilder>
getTotalsFieldBuilder() {
if (totalsBuilder_ == null) {
totalsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotalOrBuilder>(
totals_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
totals_ = null;
}
return totalsBuilder_;
}
private int slideCount_ ;
/**
*
*客户端可指定滑窗数,不指定用默认值
*
*
* uint32 slideCount = 4;
* @return The slideCount.
*/
@java.lang.Override
public int getSlideCount() {
return slideCount_;
}
/**
*
*客户端可指定滑窗数,不指定用默认值
*
*
* uint32 slideCount = 4;
* @param value The slideCount to set.
* @return This builder for chaining.
*/
public Builder setSlideCount(int value) {
slideCount_ = value;
onChanged();
return this;
}
/**
*
*客户端可指定滑窗数,不指定用默认值
*
*
* uint32 slideCount = 4;
* @return This builder for chaining.
*/
public Builder clearSlideCount() {
slideCount_ = 0;
onChanged();
return this;
}
private int mode_ = 0;
/**
*
*限流模式
*
*
* .polaris.metric.v2.Mode mode = 5;
* @return The enum numeric value on the wire for mode.
*/
@java.lang.Override public int getModeValue() {
return mode_;
}
/**
*
*限流模式
*
*
* .polaris.metric.v2.Mode mode = 5;
* @param value The enum numeric value on the wire for mode to set.
* @return This builder for chaining.
*/
public Builder setModeValue(int value) {
mode_ = value;
onChanged();
return this;
}
/**
*
*限流模式
*
*
* .polaris.metric.v2.Mode mode = 5;
* @return The mode.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode getMode() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.valueOf(mode_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.UNRECOGNIZED : result;
}
/**
*
*限流模式
*
*
* .polaris.metric.v2.Mode mode = 5;
* @param value The mode to set.
* @return This builder for chaining.
*/
public Builder setMode(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode value) {
if (value == null) {
throw new NullPointerException();
}
mode_ = value.getNumber();
onChanged();
return this;
}
/**
*
*限流模式
*
*
* .polaris.metric.v2.Mode mode = 5;
* @return This builder for chaining.
*/
public Builder clearMode() {
mode_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.RateLimitInitRequest)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.RateLimitInitRequest)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RateLimitInitRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RateLimitInitResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.RateLimitInitResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
*应答错误码
*
*
* uint32 code = 1;
* @return The code.
*/
int getCode();
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
* @return Whether the target field is set.
*/
boolean hasTarget();
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
* @return The target.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget getTarget();
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder getTargetOrBuilder();
/**
*
*客户端的标识,与clientId对应,一个server全局唯一,上报时候带入
*
*
* uint32 clientKey = 3;
* @return The clientKey.
*/
int getClientKey();
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
java.util.List
getCountersList();
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter getCounters(int index);
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
int getCountersCount();
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounterOrBuilder>
getCountersOrBuilderList();
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounterOrBuilder getCountersOrBuilder(
int index);
/**
*
*实际滑窗个数
*
*
* uint32 slideCount = 6;
* @return The slideCount.
*/
int getSlideCount();
/**
*
*限流server绝对时间,单位ms
*
*
* int64 timestamp = 7;
* @return The timestamp.
*/
long getTimestamp();
}
/**
*
*初始化应答
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitInitResponse}
*/
public static final class RateLimitInitResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.RateLimitInitResponse)
RateLimitInitResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RateLimitInitResponse.newBuilder() to construct.
private RateLimitInitResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RateLimitInitResponse() {
counters_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RateLimitInitResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitInitResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitInitResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.Builder.class);
}
public static final int CODE_FIELD_NUMBER = 1;
private int code_;
/**
*
*应答错误码
*
*
* uint32 code = 1;
* @return The code.
*/
@java.lang.Override
public int getCode() {
return code_;
}
public static final int TARGET_FIELD_NUMBER = 2;
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget target_;
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
* @return Whether the target field is set.
*/
@java.lang.Override
public boolean hasTarget() {
return target_ != null;
}
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
* @return The target.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget getTarget() {
return target_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.getDefaultInstance() : target_;
}
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder getTargetOrBuilder() {
return getTarget();
}
public static final int CLIENTKEY_FIELD_NUMBER = 3;
private int clientKey_;
/**
*
*客户端的标识,与clientId对应,一个server全局唯一,上报时候带入
*
*
* uint32 clientKey = 3;
* @return The clientKey.
*/
@java.lang.Override
public int getClientKey() {
return clientKey_;
}
public static final int COUNTERS_FIELD_NUMBER = 5;
private java.util.List counters_;
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
@java.lang.Override
public java.util.List getCountersList() {
return counters_;
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
@java.lang.Override
public java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounterOrBuilder>
getCountersOrBuilderList() {
return counters_;
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
@java.lang.Override
public int getCountersCount() {
return counters_.size();
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter getCounters(int index) {
return counters_.get(index);
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounterOrBuilder getCountersOrBuilder(
int index) {
return counters_.get(index);
}
public static final int SLIDECOUNT_FIELD_NUMBER = 6;
private int slideCount_;
/**
*
*实际滑窗个数
*
*
* uint32 slideCount = 6;
* @return The slideCount.
*/
@java.lang.Override
public int getSlideCount() {
return slideCount_;
}
public static final int TIMESTAMP_FIELD_NUMBER = 7;
private long timestamp_;
/**
*
*限流server绝对时间,单位ms
*
*
* int64 timestamp = 7;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (code_ != 0) {
output.writeUInt32(1, code_);
}
if (target_ != null) {
output.writeMessage(2, getTarget());
}
if (clientKey_ != 0) {
output.writeUInt32(3, clientKey_);
}
for (int i = 0; i < counters_.size(); i++) {
output.writeMessage(5, counters_.get(i));
}
if (slideCount_ != 0) {
output.writeUInt32(6, slideCount_);
}
if (timestamp_ != 0L) {
output.writeInt64(7, timestamp_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (code_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, code_);
}
if (target_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getTarget());
}
if (clientKey_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, clientKey_);
}
for (int i = 0; i < counters_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, counters_.get(i));
}
if (slideCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(6, slideCount_);
}
if (timestamp_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(7, timestamp_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse) obj;
if (getCode()
!= other.getCode()) return false;
if (hasTarget() != other.hasTarget()) return false;
if (hasTarget()) {
if (!getTarget()
.equals(other.getTarget())) return false;
}
if (getClientKey()
!= other.getClientKey()) return false;
if (!getCountersList()
.equals(other.getCountersList())) return false;
if (getSlideCount()
!= other.getSlideCount()) return false;
if (getTimestamp()
!= other.getTimestamp()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CODE_FIELD_NUMBER;
hash = (53 * hash) + getCode();
if (hasTarget()) {
hash = (37 * hash) + TARGET_FIELD_NUMBER;
hash = (53 * hash) + getTarget().hashCode();
}
hash = (37 * hash) + CLIENTKEY_FIELD_NUMBER;
hash = (53 * hash) + getClientKey();
if (getCountersCount() > 0) {
hash = (37 * hash) + COUNTERS_FIELD_NUMBER;
hash = (53 * hash) + getCountersList().hashCode();
}
hash = (37 * hash) + SLIDECOUNT_FIELD_NUMBER;
hash = (53 * hash) + getSlideCount();
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*初始化应答
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitInitResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.RateLimitInitResponse)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitInitResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitInitResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
code_ = 0;
if (targetBuilder_ == null) {
target_ = null;
} else {
target_ = null;
targetBuilder_ = null;
}
clientKey_ = 0;
if (countersBuilder_ == null) {
counters_ = java.util.Collections.emptyList();
} else {
counters_ = null;
countersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
slideCount_ = 0;
timestamp_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitInitResponse_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse(this);
int from_bitField0_ = bitField0_;
result.code_ = code_;
if (targetBuilder_ == null) {
result.target_ = target_;
} else {
result.target_ = targetBuilder_.build();
}
result.clientKey_ = clientKey_;
if (countersBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
counters_ = java.util.Collections.unmodifiableList(counters_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.counters_ = counters_;
} else {
result.counters_ = countersBuilder_.build();
}
result.slideCount_ = slideCount_;
result.timestamp_ = timestamp_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse.getDefaultInstance()) return this;
if (other.getCode() != 0) {
setCode(other.getCode());
}
if (other.hasTarget()) {
mergeTarget(other.getTarget());
}
if (other.getClientKey() != 0) {
setClientKey(other.getClientKey());
}
if (countersBuilder_ == null) {
if (!other.counters_.isEmpty()) {
if (counters_.isEmpty()) {
counters_ = other.counters_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCountersIsMutable();
counters_.addAll(other.counters_);
}
onChanged();
}
} else {
if (!other.counters_.isEmpty()) {
if (countersBuilder_.isEmpty()) {
countersBuilder_.dispose();
countersBuilder_ = null;
counters_ = other.counters_;
bitField0_ = (bitField0_ & ~0x00000001);
countersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCountersFieldBuilder() : null;
} else {
countersBuilder_.addAllMessages(other.counters_);
}
}
}
if (other.getSlideCount() != 0) {
setSlideCount(other.getSlideCount());
}
if (other.getTimestamp() != 0L) {
setTimestamp(other.getTimestamp());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
code_ = input.readUInt32();
break;
} // case 8
case 18: {
input.readMessage(
getTargetFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 18
case 24: {
clientKey_ = input.readUInt32();
break;
} // case 24
case 42: {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter m =
input.readMessage(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.parser(),
extensionRegistry);
if (countersBuilder_ == null) {
ensureCountersIsMutable();
counters_.add(m);
} else {
countersBuilder_.addMessage(m);
}
break;
} // case 42
case 48: {
slideCount_ = input.readUInt32();
break;
} // case 48
case 56: {
timestamp_ = input.readInt64();
break;
} // case 56
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int code_ ;
/**
*
*应答错误码
*
*
* uint32 code = 1;
* @return The code.
*/
@java.lang.Override
public int getCode() {
return code_;
}
/**
*
*应答错误码
*
*
* uint32 code = 1;
* @param value The code to set.
* @return This builder for chaining.
*/
public Builder setCode(int value) {
code_ = value;
onChanged();
return this;
}
/**
*
*应答错误码
*
*
* uint32 code = 1;
* @return This builder for chaining.
*/
public Builder clearCode() {
code_ = 0;
onChanged();
return this;
}
private com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget target_;
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder> targetBuilder_;
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
* @return Whether the target field is set.
*/
public boolean hasTarget() {
return targetBuilder_ != null || target_ != null;
}
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
* @return The target.
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget getTarget() {
if (targetBuilder_ == null) {
return target_ == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.getDefaultInstance() : target_;
} else {
return targetBuilder_.getMessage();
}
}
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
*/
public Builder setTarget(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget value) {
if (targetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
target_ = value;
onChanged();
} else {
targetBuilder_.setMessage(value);
}
return this;
}
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
*/
public Builder setTarget(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder builderForValue) {
if (targetBuilder_ == null) {
target_ = builderForValue.build();
onChanged();
} else {
targetBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
*/
public Builder mergeTarget(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget value) {
if (targetBuilder_ == null) {
if (target_ != null) {
target_ =
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.newBuilder(target_).mergeFrom(value).buildPartial();
} else {
target_ = value;
}
onChanged();
} else {
targetBuilder_.mergeFrom(value);
}
return this;
}
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
*/
public Builder clearTarget() {
if (targetBuilder_ == null) {
target_ = null;
onChanged();
} else {
target_ = null;
targetBuilder_ = null;
}
return this;
}
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder getTargetBuilder() {
onChanged();
return getTargetFieldBuilder().getBuilder();
}
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder getTargetOrBuilder() {
if (targetBuilder_ != null) {
return targetBuilder_.getMessageOrBuilder();
} else {
return target_ == null ?
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.getDefaultInstance() : target_;
}
}
/**
*
*限流目标对象,回传给客户端
*
*
* .polaris.metric.v2.LimitTarget target = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder>
getTargetFieldBuilder() {
if (targetBuilder_ == null) {
targetBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder>(
getTarget(),
getParentForChildren(),
isClean());
target_ = null;
}
return targetBuilder_;
}
private int clientKey_ ;
/**
*
*客户端的标识,与clientId对应,一个server全局唯一,上报时候带入
*
*
* uint32 clientKey = 3;
* @return The clientKey.
*/
@java.lang.Override
public int getClientKey() {
return clientKey_;
}
/**
*
*客户端的标识,与clientId对应,一个server全局唯一,上报时候带入
*
*
* uint32 clientKey = 3;
* @param value The clientKey to set.
* @return This builder for chaining.
*/
public Builder setClientKey(int value) {
clientKey_ = value;
onChanged();
return this;
}
/**
*
*客户端的标识,与clientId对应,一个server全局唯一,上报时候带入
*
*
* uint32 clientKey = 3;
* @return This builder for chaining.
*/
public Builder clearClientKey() {
clientKey_ = 0;
onChanged();
return this;
}
private java.util.List counters_ =
java.util.Collections.emptyList();
private void ensureCountersIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
counters_ = new java.util.ArrayList(counters_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounterOrBuilder> countersBuilder_;
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public java.util.List getCountersList() {
if (countersBuilder_ == null) {
return java.util.Collections.unmodifiableList(counters_);
} else {
return countersBuilder_.getMessageList();
}
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public int getCountersCount() {
if (countersBuilder_ == null) {
return counters_.size();
} else {
return countersBuilder_.getCount();
}
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter getCounters(int index) {
if (countersBuilder_ == null) {
return counters_.get(index);
} else {
return countersBuilder_.getMessage(index);
}
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public Builder setCounters(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter value) {
if (countersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCountersIsMutable();
counters_.set(index, value);
onChanged();
} else {
countersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public Builder setCounters(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.Builder builderForValue) {
if (countersBuilder_ == null) {
ensureCountersIsMutable();
counters_.set(index, builderForValue.build());
onChanged();
} else {
countersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public Builder addCounters(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter value) {
if (countersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCountersIsMutable();
counters_.add(value);
onChanged();
} else {
countersBuilder_.addMessage(value);
}
return this;
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public Builder addCounters(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter value) {
if (countersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCountersIsMutable();
counters_.add(index, value);
onChanged();
} else {
countersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public Builder addCounters(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.Builder builderForValue) {
if (countersBuilder_ == null) {
ensureCountersIsMutable();
counters_.add(builderForValue.build());
onChanged();
} else {
countersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public Builder addCounters(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.Builder builderForValue) {
if (countersBuilder_ == null) {
ensureCountersIsMutable();
counters_.add(index, builderForValue.build());
onChanged();
} else {
countersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public Builder addAllCounters(
java.lang.Iterable extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter> values) {
if (countersBuilder_ == null) {
ensureCountersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, counters_);
onChanged();
} else {
countersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public Builder clearCounters() {
if (countersBuilder_ == null) {
counters_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
countersBuilder_.clear();
}
return this;
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public Builder removeCounters(int index) {
if (countersBuilder_ == null) {
ensureCountersIsMutable();
counters_.remove(index);
onChanged();
} else {
countersBuilder_.remove(index);
}
return this;
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.Builder getCountersBuilder(
int index) {
return getCountersFieldBuilder().getBuilder(index);
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounterOrBuilder getCountersOrBuilder(
int index) {
if (countersBuilder_ == null) {
return counters_.get(index); } else {
return countersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounterOrBuilder>
getCountersOrBuilderList() {
if (countersBuilder_ != null) {
return countersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(counters_);
}
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.Builder addCountersBuilder() {
return getCountersFieldBuilder().addBuilder(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.getDefaultInstance());
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.Builder addCountersBuilder(
int index) {
return getCountersFieldBuilder().addBuilder(
index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.getDefaultInstance());
}
/**
*
*计数器的标识
*
*
* repeated .polaris.metric.v2.QuotaCounter counters = 5;
*/
public java.util.List
getCountersBuilderList() {
return getCountersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounterOrBuilder>
getCountersFieldBuilder() {
if (countersBuilder_ == null) {
countersBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounterOrBuilder>(
counters_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
counters_ = null;
}
return countersBuilder_;
}
private int slideCount_ ;
/**
*
*实际滑窗个数
*
*
* uint32 slideCount = 6;
* @return The slideCount.
*/
@java.lang.Override
public int getSlideCount() {
return slideCount_;
}
/**
*
*实际滑窗个数
*
*
* uint32 slideCount = 6;
* @param value The slideCount to set.
* @return This builder for chaining.
*/
public Builder setSlideCount(int value) {
slideCount_ = value;
onChanged();
return this;
}
/**
*
*实际滑窗个数
*
*
* uint32 slideCount = 6;
* @return This builder for chaining.
*/
public Builder clearSlideCount() {
slideCount_ = 0;
onChanged();
return this;
}
private long timestamp_ ;
/**
*
*限流server绝对时间,单位ms
*
*
* int64 timestamp = 7;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
/**
*
*限流server绝对时间,单位ms
*
*
* int64 timestamp = 7;
* @param value The timestamp to set.
* @return This builder for chaining.
*/
public Builder setTimestamp(long value) {
timestamp_ = value;
onChanged();
return this;
}
/**
*
*限流server绝对时间,单位ms
*
*
* int64 timestamp = 7;
* @return This builder for chaining.
*/
public Builder clearTimestamp() {
timestamp_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.RateLimitInitResponse)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.RateLimitInitResponse)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RateLimitInitResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitInitResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RateLimitReportRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.RateLimitReportRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
*客户端标识
*
*
* uint32 clientKey = 1;
* @return The clientKey.
*/
int getClientKey();
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
java.util.List
getQuotaUsesList();
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum getQuotaUses(int index);
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
int getQuotaUsesCount();
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSumOrBuilder>
getQuotaUsesOrBuilderList();
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSumOrBuilder getQuotaUsesOrBuilder(
int index);
/**
*
*配额发生的时间,单位ms
*
*
* int64 timestamp = 3;
* @return The timestamp.
*/
long getTimestamp();
}
/**
*
*限流上报请求
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitReportRequest}
*/
public static final class RateLimitReportRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.RateLimitReportRequest)
RateLimitReportRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use RateLimitReportRequest.newBuilder() to construct.
private RateLimitReportRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RateLimitReportRequest() {
quotaUses_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RateLimitReportRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitReportRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitReportRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.Builder.class);
}
public static final int CLIENTKEY_FIELD_NUMBER = 1;
private int clientKey_;
/**
*
*客户端标识
*
*
* uint32 clientKey = 1;
* @return The clientKey.
*/
@java.lang.Override
public int getClientKey() {
return clientKey_;
}
public static final int QUOTAUSES_FIELD_NUMBER = 2;
private java.util.List quotaUses_;
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
@java.lang.Override
public java.util.List getQuotaUsesList() {
return quotaUses_;
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
@java.lang.Override
public java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSumOrBuilder>
getQuotaUsesOrBuilderList() {
return quotaUses_;
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
@java.lang.Override
public int getQuotaUsesCount() {
return quotaUses_.size();
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum getQuotaUses(int index) {
return quotaUses_.get(index);
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSumOrBuilder getQuotaUsesOrBuilder(
int index) {
return quotaUses_.get(index);
}
public static final int TIMESTAMP_FIELD_NUMBER = 3;
private long timestamp_;
/**
*
*配额发生的时间,单位ms
*
*
* int64 timestamp = 3;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (clientKey_ != 0) {
output.writeUInt32(1, clientKey_);
}
for (int i = 0; i < quotaUses_.size(); i++) {
output.writeMessage(2, quotaUses_.get(i));
}
if (timestamp_ != 0L) {
output.writeInt64(3, timestamp_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (clientKey_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, clientKey_);
}
for (int i = 0; i < quotaUses_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, quotaUses_.get(i));
}
if (timestamp_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, timestamp_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest) obj;
if (getClientKey()
!= other.getClientKey()) return false;
if (!getQuotaUsesList()
.equals(other.getQuotaUsesList())) return false;
if (getTimestamp()
!= other.getTimestamp()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CLIENTKEY_FIELD_NUMBER;
hash = (53 * hash) + getClientKey();
if (getQuotaUsesCount() > 0) {
hash = (37 * hash) + QUOTAUSES_FIELD_NUMBER;
hash = (53 * hash) + getQuotaUsesList().hashCode();
}
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*限流上报请求
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitReportRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.RateLimitReportRequest)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitReportRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitReportRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
clientKey_ = 0;
if (quotaUsesBuilder_ == null) {
quotaUses_ = java.util.Collections.emptyList();
} else {
quotaUses_ = null;
quotaUsesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
timestamp_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitReportRequest_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest(this);
int from_bitField0_ = bitField0_;
result.clientKey_ = clientKey_;
if (quotaUsesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
quotaUses_ = java.util.Collections.unmodifiableList(quotaUses_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.quotaUses_ = quotaUses_;
} else {
result.quotaUses_ = quotaUsesBuilder_.build();
}
result.timestamp_ = timestamp_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest.getDefaultInstance()) return this;
if (other.getClientKey() != 0) {
setClientKey(other.getClientKey());
}
if (quotaUsesBuilder_ == null) {
if (!other.quotaUses_.isEmpty()) {
if (quotaUses_.isEmpty()) {
quotaUses_ = other.quotaUses_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureQuotaUsesIsMutable();
quotaUses_.addAll(other.quotaUses_);
}
onChanged();
}
} else {
if (!other.quotaUses_.isEmpty()) {
if (quotaUsesBuilder_.isEmpty()) {
quotaUsesBuilder_.dispose();
quotaUsesBuilder_ = null;
quotaUses_ = other.quotaUses_;
bitField0_ = (bitField0_ & ~0x00000001);
quotaUsesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getQuotaUsesFieldBuilder() : null;
} else {
quotaUsesBuilder_.addAllMessages(other.quotaUses_);
}
}
}
if (other.getTimestamp() != 0L) {
setTimestamp(other.getTimestamp());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
clientKey_ = input.readUInt32();
break;
} // case 8
case 18: {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum m =
input.readMessage(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.parser(),
extensionRegistry);
if (quotaUsesBuilder_ == null) {
ensureQuotaUsesIsMutable();
quotaUses_.add(m);
} else {
quotaUsesBuilder_.addMessage(m);
}
break;
} // case 18
case 24: {
timestamp_ = input.readInt64();
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int clientKey_ ;
/**
*
*客户端标识
*
*
* uint32 clientKey = 1;
* @return The clientKey.
*/
@java.lang.Override
public int getClientKey() {
return clientKey_;
}
/**
*
*客户端标识
*
*
* uint32 clientKey = 1;
* @param value The clientKey to set.
* @return This builder for chaining.
*/
public Builder setClientKey(int value) {
clientKey_ = value;
onChanged();
return this;
}
/**
*
*客户端标识
*
*
* uint32 clientKey = 1;
* @return This builder for chaining.
*/
public Builder clearClientKey() {
clientKey_ = 0;
onChanged();
return this;
}
private java.util.List quotaUses_ =
java.util.Collections.emptyList();
private void ensureQuotaUsesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
quotaUses_ = new java.util.ArrayList(quotaUses_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSumOrBuilder> quotaUsesBuilder_;
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public java.util.List getQuotaUsesList() {
if (quotaUsesBuilder_ == null) {
return java.util.Collections.unmodifiableList(quotaUses_);
} else {
return quotaUsesBuilder_.getMessageList();
}
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public int getQuotaUsesCount() {
if (quotaUsesBuilder_ == null) {
return quotaUses_.size();
} else {
return quotaUsesBuilder_.getCount();
}
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum getQuotaUses(int index) {
if (quotaUsesBuilder_ == null) {
return quotaUses_.get(index);
} else {
return quotaUsesBuilder_.getMessage(index);
}
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public Builder setQuotaUses(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum value) {
if (quotaUsesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQuotaUsesIsMutable();
quotaUses_.set(index, value);
onChanged();
} else {
quotaUsesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public Builder setQuotaUses(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.Builder builderForValue) {
if (quotaUsesBuilder_ == null) {
ensureQuotaUsesIsMutable();
quotaUses_.set(index, builderForValue.build());
onChanged();
} else {
quotaUsesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public Builder addQuotaUses(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum value) {
if (quotaUsesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQuotaUsesIsMutable();
quotaUses_.add(value);
onChanged();
} else {
quotaUsesBuilder_.addMessage(value);
}
return this;
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public Builder addQuotaUses(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum value) {
if (quotaUsesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQuotaUsesIsMutable();
quotaUses_.add(index, value);
onChanged();
} else {
quotaUsesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public Builder addQuotaUses(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.Builder builderForValue) {
if (quotaUsesBuilder_ == null) {
ensureQuotaUsesIsMutable();
quotaUses_.add(builderForValue.build());
onChanged();
} else {
quotaUsesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public Builder addQuotaUses(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.Builder builderForValue) {
if (quotaUsesBuilder_ == null) {
ensureQuotaUsesIsMutable();
quotaUses_.add(index, builderForValue.build());
onChanged();
} else {
quotaUsesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public Builder addAllQuotaUses(
java.lang.Iterable extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum> values) {
if (quotaUsesBuilder_ == null) {
ensureQuotaUsesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, quotaUses_);
onChanged();
} else {
quotaUsesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public Builder clearQuotaUses() {
if (quotaUsesBuilder_ == null) {
quotaUses_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
quotaUsesBuilder_.clear();
}
return this;
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public Builder removeQuotaUses(int index) {
if (quotaUsesBuilder_ == null) {
ensureQuotaUsesIsMutable();
quotaUses_.remove(index);
onChanged();
} else {
quotaUsesBuilder_.remove(index);
}
return this;
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.Builder getQuotaUsesBuilder(
int index) {
return getQuotaUsesFieldBuilder().getBuilder(index);
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSumOrBuilder getQuotaUsesOrBuilder(
int index) {
if (quotaUsesBuilder_ == null) {
return quotaUses_.get(index); } else {
return quotaUsesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSumOrBuilder>
getQuotaUsesOrBuilderList() {
if (quotaUsesBuilder_ != null) {
return quotaUsesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(quotaUses_);
}
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.Builder addQuotaUsesBuilder() {
return getQuotaUsesFieldBuilder().addBuilder(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.getDefaultInstance());
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.Builder addQuotaUsesBuilder(
int index) {
return getQuotaUsesFieldBuilder().addBuilder(
index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.getDefaultInstance());
}
/**
*
*已使用的配额数
*
*
* repeated .polaris.metric.v2.QuotaSum quotaUses = 2;
*/
public java.util.List
getQuotaUsesBuilderList() {
return getQuotaUsesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSumOrBuilder>
getQuotaUsesFieldBuilder() {
if (quotaUsesBuilder_ == null) {
quotaUsesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSumOrBuilder>(
quotaUses_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
quotaUses_ = null;
}
return quotaUsesBuilder_;
}
private long timestamp_ ;
/**
*
*配额发生的时间,单位ms
*
*
* int64 timestamp = 3;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
/**
*
*配额发生的时间,单位ms
*
*
* int64 timestamp = 3;
* @param value The timestamp to set.
* @return This builder for chaining.
*/
public Builder setTimestamp(long value) {
timestamp_ = value;
onChanged();
return this;
}
/**
*
*配额发生的时间,单位ms
*
*
* int64 timestamp = 3;
* @return This builder for chaining.
*/
public Builder clearTimestamp() {
timestamp_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.RateLimitReportRequest)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.RateLimitReportRequest)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RateLimitReportRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RateLimitReportResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.RateLimitReportResponse)
com.google.protobuf.MessageOrBuilder {
/**
* uint32 code = 1;
* @return The code.
*/
int getCode();
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
java.util.List
getQuotaLeftsList();
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft getQuotaLefts(int index);
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
int getQuotaLeftsCount();
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeftOrBuilder>
getQuotaLeftsOrBuilderList();
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeftOrBuilder getQuotaLeftsOrBuilder(
int index);
/**
*
*限流server绝对时间,单位ms
*
*
* int64 timestamp = 3;
* @return The timestamp.
*/
long getTimestamp();
}
/**
*
*限流上报应答
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitReportResponse}
*/
public static final class RateLimitReportResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.RateLimitReportResponse)
RateLimitReportResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RateLimitReportResponse.newBuilder() to construct.
private RateLimitReportResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RateLimitReportResponse() {
quotaLefts_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RateLimitReportResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitReportResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitReportResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.Builder.class);
}
public static final int CODE_FIELD_NUMBER = 1;
private int code_;
/**
* uint32 code = 1;
* @return The code.
*/
@java.lang.Override
public int getCode() {
return code_;
}
public static final int QUOTALEFTS_FIELD_NUMBER = 2;
private java.util.List quotaLefts_;
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
@java.lang.Override
public java.util.List getQuotaLeftsList() {
return quotaLefts_;
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
@java.lang.Override
public java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeftOrBuilder>
getQuotaLeftsOrBuilderList() {
return quotaLefts_;
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
@java.lang.Override
public int getQuotaLeftsCount() {
return quotaLefts_.size();
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft getQuotaLefts(int index) {
return quotaLefts_.get(index);
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeftOrBuilder getQuotaLeftsOrBuilder(
int index) {
return quotaLefts_.get(index);
}
public static final int TIMESTAMP_FIELD_NUMBER = 3;
private long timestamp_;
/**
*
*限流server绝对时间,单位ms
*
*
* int64 timestamp = 3;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (code_ != 0) {
output.writeUInt32(1, code_);
}
for (int i = 0; i < quotaLefts_.size(); i++) {
output.writeMessage(2, quotaLefts_.get(i));
}
if (timestamp_ != 0L) {
output.writeInt64(3, timestamp_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (code_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, code_);
}
for (int i = 0; i < quotaLefts_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, quotaLefts_.get(i));
}
if (timestamp_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, timestamp_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse) obj;
if (getCode()
!= other.getCode()) return false;
if (!getQuotaLeftsList()
.equals(other.getQuotaLeftsList())) return false;
if (getTimestamp()
!= other.getTimestamp()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CODE_FIELD_NUMBER;
hash = (53 * hash) + getCode();
if (getQuotaLeftsCount() > 0) {
hash = (37 * hash) + QUOTALEFTS_FIELD_NUMBER;
hash = (53 * hash) + getQuotaLeftsList().hashCode();
}
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*限流上报应答
*
*
* Protobuf type {@code polaris.metric.v2.RateLimitReportResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.RateLimitReportResponse)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitReportResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitReportResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
code_ = 0;
if (quotaLeftsBuilder_ == null) {
quotaLefts_ = java.util.Collections.emptyList();
} else {
quotaLefts_ = null;
quotaLeftsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
timestamp_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_RateLimitReportResponse_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse(this);
int from_bitField0_ = bitField0_;
result.code_ = code_;
if (quotaLeftsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
quotaLefts_ = java.util.Collections.unmodifiableList(quotaLefts_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.quotaLefts_ = quotaLefts_;
} else {
result.quotaLefts_ = quotaLeftsBuilder_.build();
}
result.timestamp_ = timestamp_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse.getDefaultInstance()) return this;
if (other.getCode() != 0) {
setCode(other.getCode());
}
if (quotaLeftsBuilder_ == null) {
if (!other.quotaLefts_.isEmpty()) {
if (quotaLefts_.isEmpty()) {
quotaLefts_ = other.quotaLefts_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureQuotaLeftsIsMutable();
quotaLefts_.addAll(other.quotaLefts_);
}
onChanged();
}
} else {
if (!other.quotaLefts_.isEmpty()) {
if (quotaLeftsBuilder_.isEmpty()) {
quotaLeftsBuilder_.dispose();
quotaLeftsBuilder_ = null;
quotaLefts_ = other.quotaLefts_;
bitField0_ = (bitField0_ & ~0x00000001);
quotaLeftsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getQuotaLeftsFieldBuilder() : null;
} else {
quotaLeftsBuilder_.addAllMessages(other.quotaLefts_);
}
}
}
if (other.getTimestamp() != 0L) {
setTimestamp(other.getTimestamp());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
code_ = input.readUInt32();
break;
} // case 8
case 18: {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft m =
input.readMessage(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.parser(),
extensionRegistry);
if (quotaLeftsBuilder_ == null) {
ensureQuotaLeftsIsMutable();
quotaLefts_.add(m);
} else {
quotaLeftsBuilder_.addMessage(m);
}
break;
} // case 18
case 24: {
timestamp_ = input.readInt64();
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int code_ ;
/**
* uint32 code = 1;
* @return The code.
*/
@java.lang.Override
public int getCode() {
return code_;
}
/**
* uint32 code = 1;
* @param value The code to set.
* @return This builder for chaining.
*/
public Builder setCode(int value) {
code_ = value;
onChanged();
return this;
}
/**
* uint32 code = 1;
* @return This builder for chaining.
*/
public Builder clearCode() {
code_ = 0;
onChanged();
return this;
}
private java.util.List quotaLefts_ =
java.util.Collections.emptyList();
private void ensureQuotaLeftsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
quotaLefts_ = new java.util.ArrayList(quotaLefts_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeftOrBuilder> quotaLeftsBuilder_;
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public java.util.List getQuotaLeftsList() {
if (quotaLeftsBuilder_ == null) {
return java.util.Collections.unmodifiableList(quotaLefts_);
} else {
return quotaLeftsBuilder_.getMessageList();
}
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public int getQuotaLeftsCount() {
if (quotaLeftsBuilder_ == null) {
return quotaLefts_.size();
} else {
return quotaLeftsBuilder_.getCount();
}
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft getQuotaLefts(int index) {
if (quotaLeftsBuilder_ == null) {
return quotaLefts_.get(index);
} else {
return quotaLeftsBuilder_.getMessage(index);
}
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public Builder setQuotaLefts(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft value) {
if (quotaLeftsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQuotaLeftsIsMutable();
quotaLefts_.set(index, value);
onChanged();
} else {
quotaLeftsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public Builder setQuotaLefts(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.Builder builderForValue) {
if (quotaLeftsBuilder_ == null) {
ensureQuotaLeftsIsMutable();
quotaLefts_.set(index, builderForValue.build());
onChanged();
} else {
quotaLeftsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public Builder addQuotaLefts(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft value) {
if (quotaLeftsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQuotaLeftsIsMutable();
quotaLefts_.add(value);
onChanged();
} else {
quotaLeftsBuilder_.addMessage(value);
}
return this;
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public Builder addQuotaLefts(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft value) {
if (quotaLeftsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQuotaLeftsIsMutable();
quotaLefts_.add(index, value);
onChanged();
} else {
quotaLeftsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public Builder addQuotaLefts(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.Builder builderForValue) {
if (quotaLeftsBuilder_ == null) {
ensureQuotaLeftsIsMutable();
quotaLefts_.add(builderForValue.build());
onChanged();
} else {
quotaLeftsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public Builder addQuotaLefts(
int index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.Builder builderForValue) {
if (quotaLeftsBuilder_ == null) {
ensureQuotaLeftsIsMutable();
quotaLefts_.add(index, builderForValue.build());
onChanged();
} else {
quotaLeftsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public Builder addAllQuotaLefts(
java.lang.Iterable extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft> values) {
if (quotaLeftsBuilder_ == null) {
ensureQuotaLeftsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, quotaLefts_);
onChanged();
} else {
quotaLeftsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public Builder clearQuotaLefts() {
if (quotaLeftsBuilder_ == null) {
quotaLefts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
quotaLeftsBuilder_.clear();
}
return this;
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public Builder removeQuotaLefts(int index) {
if (quotaLeftsBuilder_ == null) {
ensureQuotaLeftsIsMutable();
quotaLefts_.remove(index);
onChanged();
} else {
quotaLeftsBuilder_.remove(index);
}
return this;
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.Builder getQuotaLeftsBuilder(
int index) {
return getQuotaLeftsFieldBuilder().getBuilder(index);
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeftOrBuilder getQuotaLeftsOrBuilder(
int index) {
if (quotaLeftsBuilder_ == null) {
return quotaLefts_.get(index); } else {
return quotaLeftsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public java.util.List extends com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeftOrBuilder>
getQuotaLeftsOrBuilderList() {
if (quotaLeftsBuilder_ != null) {
return quotaLeftsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(quotaLefts_);
}
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.Builder addQuotaLeftsBuilder() {
return getQuotaLeftsFieldBuilder().addBuilder(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.getDefaultInstance());
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.Builder addQuotaLeftsBuilder(
int index) {
return getQuotaLeftsFieldBuilder().addBuilder(
index, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.getDefaultInstance());
}
/**
*
*剩余配额数
*
*
* repeated .polaris.metric.v2.QuotaLeft quotaLefts = 2;
*/
public java.util.List
getQuotaLeftsBuilderList() {
return getQuotaLeftsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeftOrBuilder>
getQuotaLeftsFieldBuilder() {
if (quotaLeftsBuilder_ == null) {
quotaLeftsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.Builder, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeftOrBuilder>(
quotaLefts_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
quotaLefts_ = null;
}
return quotaLeftsBuilder_;
}
private long timestamp_ ;
/**
*
*限流server绝对时间,单位ms
*
*
* int64 timestamp = 3;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
/**
*
*限流server绝对时间,单位ms
*
*
* int64 timestamp = 3;
* @param value The timestamp to set.
* @return This builder for chaining.
*/
public Builder setTimestamp(long value) {
timestamp_ = value;
onChanged();
return this;
}
/**
*
*限流server绝对时间,单位ms
*
*
* int64 timestamp = 3;
* @return This builder for chaining.
*/
public Builder clearTimestamp() {
timestamp_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.RateLimitReportResponse)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.RateLimitReportResponse)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RateLimitReportResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.RateLimitReportResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LimitTargetOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.LimitTarget)
com.google.protobuf.MessageOrBuilder {
/**
*
*命名空间
*
*
* string namespace = 1;
* @return The namespace.
*/
java.lang.String getNamespace();
/**
*
*命名空间
*
*
* string namespace = 1;
* @return The bytes for namespace.
*/
com.google.protobuf.ByteString
getNamespaceBytes();
/**
*
*服务名
*
*
* string service = 2;
* @return The service.
*/
java.lang.String getService();
/**
*
*服务名
*
*
* string service = 2;
* @return The bytes for service.
*/
com.google.protobuf.ByteString
getServiceBytes();
/**
*
*自定义标签
*
*
* string labels = 3;
* @return The labels.
*/
java.lang.String getLabels();
/**
*
*自定义标签
*
*
* string labels = 3;
* @return The bytes for labels.
*/
com.google.protobuf.ByteString
getLabelsBytes();
}
/**
*
*限流目标,针对哪部分数据进行限流
*
*
* Protobuf type {@code polaris.metric.v2.LimitTarget}
*/
public static final class LimitTarget extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.LimitTarget)
LimitTargetOrBuilder {
private static final long serialVersionUID = 0L;
// Use LimitTarget.newBuilder() to construct.
private LimitTarget(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LimitTarget() {
namespace_ = "";
service_ = "";
labels_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LimitTarget();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_LimitTarget_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_LimitTarget_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder.class);
}
public static final int NAMESPACE_FIELD_NUMBER = 1;
private volatile java.lang.Object namespace_;
/**
*
*命名空间
*
*
* string namespace = 1;
* @return The namespace.
*/
@java.lang.Override
public java.lang.String getNamespace() {
java.lang.Object ref = namespace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
namespace_ = s;
return s;
}
}
/**
*
*命名空间
*
*
* string namespace = 1;
* @return The bytes for namespace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNamespaceBytes() {
java.lang.Object ref = namespace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
namespace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERVICE_FIELD_NUMBER = 2;
private volatile java.lang.Object service_;
/**
*
*服务名
*
*
* string service = 2;
* @return The service.
*/
@java.lang.Override
public java.lang.String getService() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
service_ = s;
return s;
}
}
/**
*
*服务名
*
*
* string service = 2;
* @return The bytes for service.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LABELS_FIELD_NUMBER = 3;
private volatile java.lang.Object labels_;
/**
*
*自定义标签
*
*
* string labels = 3;
* @return The labels.
*/
@java.lang.Override
public java.lang.String getLabels() {
java.lang.Object ref = labels_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
labels_ = s;
return s;
}
}
/**
*
*自定义标签
*
*
* string labels = 3;
* @return The bytes for labels.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLabelsBytes() {
java.lang.Object ref = labels_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
labels_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(namespace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, namespace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(service_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, service_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(labels_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, labels_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(namespace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, namespace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(service_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, service_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(labels_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, labels_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget) obj;
if (!getNamespace()
.equals(other.getNamespace())) return false;
if (!getService()
.equals(other.getService())) return false;
if (!getLabels()
.equals(other.getLabels())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAMESPACE_FIELD_NUMBER;
hash = (53 * hash) + getNamespace().hashCode();
hash = (37 * hash) + SERVICE_FIELD_NUMBER;
hash = (53 * hash) + getService().hashCode();
hash = (37 * hash) + LABELS_FIELD_NUMBER;
hash = (53 * hash) + getLabels().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*限流目标,针对哪部分数据进行限流
*
*
* Protobuf type {@code polaris.metric.v2.LimitTarget}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.LimitTarget)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTargetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_LimitTarget_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_LimitTarget_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
namespace_ = "";
service_ = "";
labels_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_LimitTarget_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget(this);
result.namespace_ = namespace_;
result.service_ = service_;
result.labels_ = labels_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget.getDefaultInstance()) return this;
if (!other.getNamespace().isEmpty()) {
namespace_ = other.namespace_;
onChanged();
}
if (!other.getService().isEmpty()) {
service_ = other.service_;
onChanged();
}
if (!other.getLabels().isEmpty()) {
labels_ = other.labels_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
namespace_ = input.readStringRequireUtf8();
break;
} // case 10
case 18: {
service_ = input.readStringRequireUtf8();
break;
} // case 18
case 26: {
labels_ = input.readStringRequireUtf8();
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private java.lang.Object namespace_ = "";
/**
*
*命名空间
*
*
* string namespace = 1;
* @return The namespace.
*/
public java.lang.String getNamespace() {
java.lang.Object ref = namespace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
namespace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*命名空间
*
*
* string namespace = 1;
* @return The bytes for namespace.
*/
public com.google.protobuf.ByteString
getNamespaceBytes() {
java.lang.Object ref = namespace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
namespace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*命名空间
*
*
* string namespace = 1;
* @param value The namespace to set.
* @return This builder for chaining.
*/
public Builder setNamespace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
namespace_ = value;
onChanged();
return this;
}
/**
*
*命名空间
*
*
* string namespace = 1;
* @return This builder for chaining.
*/
public Builder clearNamespace() {
namespace_ = getDefaultInstance().getNamespace();
onChanged();
return this;
}
/**
*
*命名空间
*
*
* string namespace = 1;
* @param value The bytes for namespace to set.
* @return This builder for chaining.
*/
public Builder setNamespaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
namespace_ = value;
onChanged();
return this;
}
private java.lang.Object service_ = "";
/**
*
*服务名
*
*
* string service = 2;
* @return The service.
*/
public java.lang.String getService() {
java.lang.Object ref = service_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
service_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*服务名
*
*
* string service = 2;
* @return The bytes for service.
*/
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*服务名
*
*
* string service = 2;
* @param value The service to set.
* @return This builder for chaining.
*/
public Builder setService(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
service_ = value;
onChanged();
return this;
}
/**
*
*服务名
*
*
* string service = 2;
* @return This builder for chaining.
*/
public Builder clearService() {
service_ = getDefaultInstance().getService();
onChanged();
return this;
}
/**
*
*服务名
*
*
* string service = 2;
* @param value The bytes for service to set.
* @return This builder for chaining.
*/
public Builder setServiceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
service_ = value;
onChanged();
return this;
}
private java.lang.Object labels_ = "";
/**
*
*自定义标签
*
*
* string labels = 3;
* @return The labels.
*/
public java.lang.String getLabels() {
java.lang.Object ref = labels_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
labels_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*自定义标签
*
*
* string labels = 3;
* @return The bytes for labels.
*/
public com.google.protobuf.ByteString
getLabelsBytes() {
java.lang.Object ref = labels_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
labels_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*自定义标签
*
*
* string labels = 3;
* @param value The labels to set.
* @return This builder for chaining.
*/
public Builder setLabels(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
labels_ = value;
onChanged();
return this;
}
/**
*
*自定义标签
*
*
* string labels = 3;
* @return This builder for chaining.
*/
public Builder clearLabels() {
labels_ = getDefaultInstance().getLabels();
onChanged();
return this;
}
/**
*
*自定义标签
*
*
* string labels = 3;
* @param value The bytes for labels to set.
* @return This builder for chaining.
*/
public Builder setLabelsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
labels_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.LimitTarget)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.LimitTarget)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LimitTarget parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.LimitTarget getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QuotaTotalOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.QuotaTotal)
com.google.protobuf.MessageOrBuilder {
/**
*
*阈值模式
*
*
* .polaris.metric.v2.QuotaMode mode = 1;
* @return The enum numeric value on the wire for mode.
*/
int getModeValue();
/**
*
*阈值模式
*
*
* .polaris.metric.v2.QuotaMode mode = 1;
* @return The mode.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode getMode();
/**
*
*单位秒
*
*
* uint32 duration = 2;
* @return The duration.
*/
int getDuration();
/**
*
*限流阈值
*
*
* uint32 maxAmount = 3;
* @return The maxAmount.
*/
int getMaxAmount();
}
/**
*
*阈值配置的值
*
*
* Protobuf type {@code polaris.metric.v2.QuotaTotal}
*/
public static final class QuotaTotal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.QuotaTotal)
QuotaTotalOrBuilder {
private static final long serialVersionUID = 0L;
// Use QuotaTotal.newBuilder() to construct.
private QuotaTotal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QuotaTotal() {
mode_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new QuotaTotal();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaTotal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaTotal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.Builder.class);
}
public static final int MODE_FIELD_NUMBER = 1;
private int mode_;
/**
*
*阈值模式
*
*
* .polaris.metric.v2.QuotaMode mode = 1;
* @return The enum numeric value on the wire for mode.
*/
@java.lang.Override public int getModeValue() {
return mode_;
}
/**
*
*阈值模式
*
*
* .polaris.metric.v2.QuotaMode mode = 1;
* @return The mode.
*/
@java.lang.Override public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode getMode() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode.valueOf(mode_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode.UNRECOGNIZED : result;
}
public static final int DURATION_FIELD_NUMBER = 2;
private int duration_;
/**
*
*单位秒
*
*
* uint32 duration = 2;
* @return The duration.
*/
@java.lang.Override
public int getDuration() {
return duration_;
}
public static final int MAXAMOUNT_FIELD_NUMBER = 3;
private int maxAmount_;
/**
*
*限流阈值
*
*
* uint32 maxAmount = 3;
* @return The maxAmount.
*/
@java.lang.Override
public int getMaxAmount() {
return maxAmount_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (mode_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode.WHOLE.getNumber()) {
output.writeEnum(1, mode_);
}
if (duration_ != 0) {
output.writeUInt32(2, duration_);
}
if (maxAmount_ != 0) {
output.writeUInt32(3, maxAmount_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (mode_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode.WHOLE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, mode_);
}
if (duration_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, duration_);
}
if (maxAmount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, maxAmount_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal) obj;
if (mode_ != other.mode_) return false;
if (getDuration()
!= other.getDuration()) return false;
if (getMaxAmount()
!= other.getMaxAmount()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MODE_FIELD_NUMBER;
hash = (53 * hash) + mode_;
hash = (37 * hash) + DURATION_FIELD_NUMBER;
hash = (53 * hash) + getDuration();
hash = (37 * hash) + MAXAMOUNT_FIELD_NUMBER;
hash = (53 * hash) + getMaxAmount();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*阈值配置的值
*
*
* Protobuf type {@code polaris.metric.v2.QuotaTotal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.QuotaTotal)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaTotal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaTotal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
mode_ = 0;
duration_ = 0;
maxAmount_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaTotal_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal(this);
result.mode_ = mode_;
result.duration_ = duration_;
result.maxAmount_ = maxAmount_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal.getDefaultInstance()) return this;
if (other.mode_ != 0) {
setModeValue(other.getModeValue());
}
if (other.getDuration() != 0) {
setDuration(other.getDuration());
}
if (other.getMaxAmount() != 0) {
setMaxAmount(other.getMaxAmount());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
mode_ = input.readEnum();
break;
} // case 8
case 16: {
duration_ = input.readUInt32();
break;
} // case 16
case 24: {
maxAmount_ = input.readUInt32();
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int mode_ = 0;
/**
*
*阈值模式
*
*
* .polaris.metric.v2.QuotaMode mode = 1;
* @return The enum numeric value on the wire for mode.
*/
@java.lang.Override public int getModeValue() {
return mode_;
}
/**
*
*阈值模式
*
*
* .polaris.metric.v2.QuotaMode mode = 1;
* @param value The enum numeric value on the wire for mode to set.
* @return This builder for chaining.
*/
public Builder setModeValue(int value) {
mode_ = value;
onChanged();
return this;
}
/**
*
*阈值模式
*
*
* .polaris.metric.v2.QuotaMode mode = 1;
* @return The mode.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode getMode() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode.valueOf(mode_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode.UNRECOGNIZED : result;
}
/**
*
*阈值模式
*
*
* .polaris.metric.v2.QuotaMode mode = 1;
* @param value The mode to set.
* @return This builder for chaining.
*/
public Builder setMode(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaMode value) {
if (value == null) {
throw new NullPointerException();
}
mode_ = value.getNumber();
onChanged();
return this;
}
/**
*
*阈值模式
*
*
* .polaris.metric.v2.QuotaMode mode = 1;
* @return This builder for chaining.
*/
public Builder clearMode() {
mode_ = 0;
onChanged();
return this;
}
private int duration_ ;
/**
*
*单位秒
*
*
* uint32 duration = 2;
* @return The duration.
*/
@java.lang.Override
public int getDuration() {
return duration_;
}
/**
*
*单位秒
*
*
* uint32 duration = 2;
* @param value The duration to set.
* @return This builder for chaining.
*/
public Builder setDuration(int value) {
duration_ = value;
onChanged();
return this;
}
/**
*
*单位秒
*
*
* uint32 duration = 2;
* @return This builder for chaining.
*/
public Builder clearDuration() {
duration_ = 0;
onChanged();
return this;
}
private int maxAmount_ ;
/**
*
*限流阈值
*
*
* uint32 maxAmount = 3;
* @return The maxAmount.
*/
@java.lang.Override
public int getMaxAmount() {
return maxAmount_;
}
/**
*
*限流阈值
*
*
* uint32 maxAmount = 3;
* @param value The maxAmount to set.
* @return This builder for chaining.
*/
public Builder setMaxAmount(int value) {
maxAmount_ = value;
onChanged();
return this;
}
/**
*
*限流阈值
*
*
* uint32 maxAmount = 3;
* @return This builder for chaining.
*/
public Builder clearMaxAmount() {
maxAmount_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.QuotaTotal)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.QuotaTotal)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QuotaTotal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaTotal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QuotaCounterOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.QuotaCounter)
com.google.protobuf.MessageOrBuilder {
/**
*
*单位秒
*
*
* uint32 duration = 1;
* @return The duration.
*/
int getDuration();
/**
*
*bucket的标识,上报时候带入:namespace+service+labelStr+duration
*
*
* uint32 counterKey = 2;
* @return The counterKey.
*/
int getCounterKey();
/**
*
*剩余配额数,应答返回,允许为负数
*
*
* int64 left = 3;
* @return The left.
*/
long getLeft();
/**
*
*实际限流模式
*
*
* .polaris.metric.v2.Mode mode = 4;
* @return The enum numeric value on the wire for mode.
*/
int getModeValue();
/**
*
*实际限流模式
*
*
* .polaris.metric.v2.Mode mode = 4;
* @return The mode.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode getMode();
/**
*
*接入的客户端数量
*
*
* uint32 clientCount = 5;
* @return The clientCount.
*/
int getClientCount();
}
/**
*
*限流计数器
*
*
* Protobuf type {@code polaris.metric.v2.QuotaCounter}
*/
public static final class QuotaCounter extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.QuotaCounter)
QuotaCounterOrBuilder {
private static final long serialVersionUID = 0L;
// Use QuotaCounter.newBuilder() to construct.
private QuotaCounter(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QuotaCounter() {
mode_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new QuotaCounter();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaCounter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaCounter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.Builder.class);
}
public static final int DURATION_FIELD_NUMBER = 1;
private int duration_;
/**
*
*单位秒
*
*
* uint32 duration = 1;
* @return The duration.
*/
@java.lang.Override
public int getDuration() {
return duration_;
}
public static final int COUNTERKEY_FIELD_NUMBER = 2;
private int counterKey_;
/**
*
*bucket的标识,上报时候带入:namespace+service+labelStr+duration
*
*
* uint32 counterKey = 2;
* @return The counterKey.
*/
@java.lang.Override
public int getCounterKey() {
return counterKey_;
}
public static final int LEFT_FIELD_NUMBER = 3;
private long left_;
/**
*
*剩余配额数,应答返回,允许为负数
*
*
* int64 left = 3;
* @return The left.
*/
@java.lang.Override
public long getLeft() {
return left_;
}
public static final int MODE_FIELD_NUMBER = 4;
private int mode_;
/**
*
*实际限流模式
*
*
* .polaris.metric.v2.Mode mode = 4;
* @return The enum numeric value on the wire for mode.
*/
@java.lang.Override public int getModeValue() {
return mode_;
}
/**
*
*实际限流模式
*
*
* .polaris.metric.v2.Mode mode = 4;
* @return The mode.
*/
@java.lang.Override public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode getMode() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.valueOf(mode_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.UNRECOGNIZED : result;
}
public static final int CLIENTCOUNT_FIELD_NUMBER = 5;
private int clientCount_;
/**
*
*接入的客户端数量
*
*
* uint32 clientCount = 5;
* @return The clientCount.
*/
@java.lang.Override
public int getClientCount() {
return clientCount_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (duration_ != 0) {
output.writeUInt32(1, duration_);
}
if (counterKey_ != 0) {
output.writeUInt32(2, counterKey_);
}
if (left_ != 0L) {
output.writeInt64(3, left_);
}
if (mode_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.ADAPTIVE.getNumber()) {
output.writeEnum(4, mode_);
}
if (clientCount_ != 0) {
output.writeUInt32(5, clientCount_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (duration_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, duration_);
}
if (counterKey_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, counterKey_);
}
if (left_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, left_);
}
if (mode_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.ADAPTIVE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, mode_);
}
if (clientCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, clientCount_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter) obj;
if (getDuration()
!= other.getDuration()) return false;
if (getCounterKey()
!= other.getCounterKey()) return false;
if (getLeft()
!= other.getLeft()) return false;
if (mode_ != other.mode_) return false;
if (getClientCount()
!= other.getClientCount()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DURATION_FIELD_NUMBER;
hash = (53 * hash) + getDuration();
hash = (37 * hash) + COUNTERKEY_FIELD_NUMBER;
hash = (53 * hash) + getCounterKey();
hash = (37 * hash) + LEFT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLeft());
hash = (37 * hash) + MODE_FIELD_NUMBER;
hash = (53 * hash) + mode_;
hash = (37 * hash) + CLIENTCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getClientCount();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*限流计数器
*
*
* Protobuf type {@code polaris.metric.v2.QuotaCounter}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.QuotaCounter)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaCounter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaCounter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
duration_ = 0;
counterKey_ = 0;
left_ = 0L;
mode_ = 0;
clientCount_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaCounter_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter(this);
result.duration_ = duration_;
result.counterKey_ = counterKey_;
result.left_ = left_;
result.mode_ = mode_;
result.clientCount_ = clientCount_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter.getDefaultInstance()) return this;
if (other.getDuration() != 0) {
setDuration(other.getDuration());
}
if (other.getCounterKey() != 0) {
setCounterKey(other.getCounterKey());
}
if (other.getLeft() != 0L) {
setLeft(other.getLeft());
}
if (other.mode_ != 0) {
setModeValue(other.getModeValue());
}
if (other.getClientCount() != 0) {
setClientCount(other.getClientCount());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
duration_ = input.readUInt32();
break;
} // case 8
case 16: {
counterKey_ = input.readUInt32();
break;
} // case 16
case 24: {
left_ = input.readInt64();
break;
} // case 24
case 32: {
mode_ = input.readEnum();
break;
} // case 32
case 40: {
clientCount_ = input.readUInt32();
break;
} // case 40
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int duration_ ;
/**
*
*单位秒
*
*
* uint32 duration = 1;
* @return The duration.
*/
@java.lang.Override
public int getDuration() {
return duration_;
}
/**
*
*单位秒
*
*
* uint32 duration = 1;
* @param value The duration to set.
* @return This builder for chaining.
*/
public Builder setDuration(int value) {
duration_ = value;
onChanged();
return this;
}
/**
*
*单位秒
*
*
* uint32 duration = 1;
* @return This builder for chaining.
*/
public Builder clearDuration() {
duration_ = 0;
onChanged();
return this;
}
private int counterKey_ ;
/**
*
*bucket的标识,上报时候带入:namespace+service+labelStr+duration
*
*
* uint32 counterKey = 2;
* @return The counterKey.
*/
@java.lang.Override
public int getCounterKey() {
return counterKey_;
}
/**
*
*bucket的标识,上报时候带入:namespace+service+labelStr+duration
*
*
* uint32 counterKey = 2;
* @param value The counterKey to set.
* @return This builder for chaining.
*/
public Builder setCounterKey(int value) {
counterKey_ = value;
onChanged();
return this;
}
/**
*
*bucket的标识,上报时候带入:namespace+service+labelStr+duration
*
*
* uint32 counterKey = 2;
* @return This builder for chaining.
*/
public Builder clearCounterKey() {
counterKey_ = 0;
onChanged();
return this;
}
private long left_ ;
/**
*
*剩余配额数,应答返回,允许为负数
*
*
* int64 left = 3;
* @return The left.
*/
@java.lang.Override
public long getLeft() {
return left_;
}
/**
*
*剩余配额数,应答返回,允许为负数
*
*
* int64 left = 3;
* @param value The left to set.
* @return This builder for chaining.
*/
public Builder setLeft(long value) {
left_ = value;
onChanged();
return this;
}
/**
*
*剩余配额数,应答返回,允许为负数
*
*
* int64 left = 3;
* @return This builder for chaining.
*/
public Builder clearLeft() {
left_ = 0L;
onChanged();
return this;
}
private int mode_ = 0;
/**
*
*实际限流模式
*
*
* .polaris.metric.v2.Mode mode = 4;
* @return The enum numeric value on the wire for mode.
*/
@java.lang.Override public int getModeValue() {
return mode_;
}
/**
*
*实际限流模式
*
*
* .polaris.metric.v2.Mode mode = 4;
* @param value The enum numeric value on the wire for mode to set.
* @return This builder for chaining.
*/
public Builder setModeValue(int value) {
mode_ = value;
onChanged();
return this;
}
/**
*
*实际限流模式
*
*
* .polaris.metric.v2.Mode mode = 4;
* @return The mode.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode getMode() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.valueOf(mode_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.UNRECOGNIZED : result;
}
/**
*
*实际限流模式
*
*
* .polaris.metric.v2.Mode mode = 4;
* @param value The mode to set.
* @return This builder for chaining.
*/
public Builder setMode(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode value) {
if (value == null) {
throw new NullPointerException();
}
mode_ = value.getNumber();
onChanged();
return this;
}
/**
*
*实际限流模式
*
*
* .polaris.metric.v2.Mode mode = 4;
* @return This builder for chaining.
*/
public Builder clearMode() {
mode_ = 0;
onChanged();
return this;
}
private int clientCount_ ;
/**
*
*接入的客户端数量
*
*
* uint32 clientCount = 5;
* @return The clientCount.
*/
@java.lang.Override
public int getClientCount() {
return clientCount_;
}
/**
*
*接入的客户端数量
*
*
* uint32 clientCount = 5;
* @param value The clientCount to set.
* @return This builder for chaining.
*/
public Builder setClientCount(int value) {
clientCount_ = value;
onChanged();
return this;
}
/**
*
*接入的客户端数量
*
*
* uint32 clientCount = 5;
* @return This builder for chaining.
*/
public Builder clearClientCount() {
clientCount_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.QuotaCounter)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.QuotaCounter)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QuotaCounter parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaCounter getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QuotaSumOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.QuotaSum)
com.google.protobuf.MessageOrBuilder {
/**
*
*计数器的标识,一个server全局唯一,上报时候带入
*
*
* uint32 counterKey = 1;
* @return The counterKey.
*/
int getCounterKey();
/**
*
*已使用的配额数,上报时候带入
*
*
* uint32 used = 2;
* @return The used.
*/
int getUsed();
/**
*
*被限流数,上报时候带入
*
*
* uint32 limited = 3;
* @return The limited.
*/
int getLimited();
}
/**
*
*客户端阈值使用统计
*
*
* Protobuf type {@code polaris.metric.v2.QuotaSum}
*/
public static final class QuotaSum extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.QuotaSum)
QuotaSumOrBuilder {
private static final long serialVersionUID = 0L;
// Use QuotaSum.newBuilder() to construct.
private QuotaSum(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QuotaSum() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new QuotaSum();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaSum_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaSum_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.Builder.class);
}
public static final int COUNTERKEY_FIELD_NUMBER = 1;
private int counterKey_;
/**
*
*计数器的标识,一个server全局唯一,上报时候带入
*
*
* uint32 counterKey = 1;
* @return The counterKey.
*/
@java.lang.Override
public int getCounterKey() {
return counterKey_;
}
public static final int USED_FIELD_NUMBER = 2;
private int used_;
/**
*
*已使用的配额数,上报时候带入
*
*
* uint32 used = 2;
* @return The used.
*/
@java.lang.Override
public int getUsed() {
return used_;
}
public static final int LIMITED_FIELD_NUMBER = 3;
private int limited_;
/**
*
*被限流数,上报时候带入
*
*
* uint32 limited = 3;
* @return The limited.
*/
@java.lang.Override
public int getLimited() {
return limited_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (counterKey_ != 0) {
output.writeUInt32(1, counterKey_);
}
if (used_ != 0) {
output.writeUInt32(2, used_);
}
if (limited_ != 0) {
output.writeUInt32(3, limited_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (counterKey_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, counterKey_);
}
if (used_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, used_);
}
if (limited_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, limited_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum) obj;
if (getCounterKey()
!= other.getCounterKey()) return false;
if (getUsed()
!= other.getUsed()) return false;
if (getLimited()
!= other.getLimited()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COUNTERKEY_FIELD_NUMBER;
hash = (53 * hash) + getCounterKey();
hash = (37 * hash) + USED_FIELD_NUMBER;
hash = (53 * hash) + getUsed();
hash = (37 * hash) + LIMITED_FIELD_NUMBER;
hash = (53 * hash) + getLimited();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*客户端阈值使用统计
*
*
* Protobuf type {@code polaris.metric.v2.QuotaSum}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.QuotaSum)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSumOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaSum_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaSum_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
counterKey_ = 0;
used_ = 0;
limited_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaSum_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum(this);
result.counterKey_ = counterKey_;
result.used_ = used_;
result.limited_ = limited_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum.getDefaultInstance()) return this;
if (other.getCounterKey() != 0) {
setCounterKey(other.getCounterKey());
}
if (other.getUsed() != 0) {
setUsed(other.getUsed());
}
if (other.getLimited() != 0) {
setLimited(other.getLimited());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
counterKey_ = input.readUInt32();
break;
} // case 8
case 16: {
used_ = input.readUInt32();
break;
} // case 16
case 24: {
limited_ = input.readUInt32();
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int counterKey_ ;
/**
*
*计数器的标识,一个server全局唯一,上报时候带入
*
*
* uint32 counterKey = 1;
* @return The counterKey.
*/
@java.lang.Override
public int getCounterKey() {
return counterKey_;
}
/**
*
*计数器的标识,一个server全局唯一,上报时候带入
*
*
* uint32 counterKey = 1;
* @param value The counterKey to set.
* @return This builder for chaining.
*/
public Builder setCounterKey(int value) {
counterKey_ = value;
onChanged();
return this;
}
/**
*
*计数器的标识,一个server全局唯一,上报时候带入
*
*
* uint32 counterKey = 1;
* @return This builder for chaining.
*/
public Builder clearCounterKey() {
counterKey_ = 0;
onChanged();
return this;
}
private int used_ ;
/**
*
*已使用的配额数,上报时候带入
*
*
* uint32 used = 2;
* @return The used.
*/
@java.lang.Override
public int getUsed() {
return used_;
}
/**
*
*已使用的配额数,上报时候带入
*
*
* uint32 used = 2;
* @param value The used to set.
* @return This builder for chaining.
*/
public Builder setUsed(int value) {
used_ = value;
onChanged();
return this;
}
/**
*
*已使用的配额数,上报时候带入
*
*
* uint32 used = 2;
* @return This builder for chaining.
*/
public Builder clearUsed() {
used_ = 0;
onChanged();
return this;
}
private int limited_ ;
/**
*
*被限流数,上报时候带入
*
*
* uint32 limited = 3;
* @return The limited.
*/
@java.lang.Override
public int getLimited() {
return limited_;
}
/**
*
*被限流数,上报时候带入
*
*
* uint32 limited = 3;
* @param value The limited to set.
* @return This builder for chaining.
*/
public Builder setLimited(int value) {
limited_ = value;
onChanged();
return this;
}
/**
*
*被限流数,上报时候带入
*
*
* uint32 limited = 3;
* @return This builder for chaining.
*/
public Builder clearLimited() {
limited_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.QuotaSum)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.QuotaSum)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QuotaSum parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaSum getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QuotaLeftOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.QuotaLeft)
com.google.protobuf.MessageOrBuilder {
/**
*
*计数器的标识,一个server全局唯一,上报时候带入
*
*
* uint32 counterKey = 1;
* @return The counterKey.
*/
int getCounterKey();
/**
*
*剩余配额数,应答返回,允许为负数
*
*
* int64 left = 2;
* @return The left.
*/
long getLeft();
/**
*
*当前限流模式
*
*
* .polaris.metric.v2.Mode mode = 3;
* @return The enum numeric value on the wire for mode.
*/
int getModeValue();
/**
*
*当前限流模式
*
*
* .polaris.metric.v2.Mode mode = 3;
* @return The mode.
*/
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode getMode();
/**
*
*接入的客户端数量
*
*
* uint32 clientCount = 4;
* @return The clientCount.
*/
int getClientCount();
}
/**
*
*客户端阈值使用统计,由服务端返回
*
*
* Protobuf type {@code polaris.metric.v2.QuotaLeft}
*/
public static final class QuotaLeft extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.QuotaLeft)
QuotaLeftOrBuilder {
private static final long serialVersionUID = 0L;
// Use QuotaLeft.newBuilder() to construct.
private QuotaLeft(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QuotaLeft() {
mode_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new QuotaLeft();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaLeft_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaLeft_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.Builder.class);
}
public static final int COUNTERKEY_FIELD_NUMBER = 1;
private int counterKey_;
/**
*
*计数器的标识,一个server全局唯一,上报时候带入
*
*
* uint32 counterKey = 1;
* @return The counterKey.
*/
@java.lang.Override
public int getCounterKey() {
return counterKey_;
}
public static final int LEFT_FIELD_NUMBER = 2;
private long left_;
/**
*
*剩余配额数,应答返回,允许为负数
*
*
* int64 left = 2;
* @return The left.
*/
@java.lang.Override
public long getLeft() {
return left_;
}
public static final int MODE_FIELD_NUMBER = 3;
private int mode_;
/**
*
*当前限流模式
*
*
* .polaris.metric.v2.Mode mode = 3;
* @return The enum numeric value on the wire for mode.
*/
@java.lang.Override public int getModeValue() {
return mode_;
}
/**
*
*当前限流模式
*
*
* .polaris.metric.v2.Mode mode = 3;
* @return The mode.
*/
@java.lang.Override public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode getMode() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.valueOf(mode_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.UNRECOGNIZED : result;
}
public static final int CLIENTCOUNT_FIELD_NUMBER = 4;
private int clientCount_;
/**
*
*接入的客户端数量
*
*
* uint32 clientCount = 4;
* @return The clientCount.
*/
@java.lang.Override
public int getClientCount() {
return clientCount_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (counterKey_ != 0) {
output.writeUInt32(1, counterKey_);
}
if (left_ != 0L) {
output.writeInt64(2, left_);
}
if (mode_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.ADAPTIVE.getNumber()) {
output.writeEnum(3, mode_);
}
if (clientCount_ != 0) {
output.writeUInt32(4, clientCount_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (counterKey_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, counterKey_);
}
if (left_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, left_);
}
if (mode_ != com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.ADAPTIVE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, mode_);
}
if (clientCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, clientCount_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft) obj;
if (getCounterKey()
!= other.getCounterKey()) return false;
if (getLeft()
!= other.getLeft()) return false;
if (mode_ != other.mode_) return false;
if (getClientCount()
!= other.getClientCount()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COUNTERKEY_FIELD_NUMBER;
hash = (53 * hash) + getCounterKey();
hash = (37 * hash) + LEFT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLeft());
hash = (37 * hash) + MODE_FIELD_NUMBER;
hash = (53 * hash) + mode_;
hash = (37 * hash) + CLIENTCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getClientCount();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*客户端阈值使用统计,由服务端返回
*
*
* Protobuf type {@code polaris.metric.v2.QuotaLeft}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.QuotaLeft)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeftOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaLeft_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaLeft_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
counterKey_ = 0;
left_ = 0L;
mode_ = 0;
clientCount_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_QuotaLeft_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft(this);
result.counterKey_ = counterKey_;
result.left_ = left_;
result.mode_ = mode_;
result.clientCount_ = clientCount_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft.getDefaultInstance()) return this;
if (other.getCounterKey() != 0) {
setCounterKey(other.getCounterKey());
}
if (other.getLeft() != 0L) {
setLeft(other.getLeft());
}
if (other.mode_ != 0) {
setModeValue(other.getModeValue());
}
if (other.getClientCount() != 0) {
setClientCount(other.getClientCount());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
counterKey_ = input.readUInt32();
break;
} // case 8
case 16: {
left_ = input.readInt64();
break;
} // case 16
case 24: {
mode_ = input.readEnum();
break;
} // case 24
case 32: {
clientCount_ = input.readUInt32();
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int counterKey_ ;
/**
*
*计数器的标识,一个server全局唯一,上报时候带入
*
*
* uint32 counterKey = 1;
* @return The counterKey.
*/
@java.lang.Override
public int getCounterKey() {
return counterKey_;
}
/**
*
*计数器的标识,一个server全局唯一,上报时候带入
*
*
* uint32 counterKey = 1;
* @param value The counterKey to set.
* @return This builder for chaining.
*/
public Builder setCounterKey(int value) {
counterKey_ = value;
onChanged();
return this;
}
/**
*
*计数器的标识,一个server全局唯一,上报时候带入
*
*
* uint32 counterKey = 1;
* @return This builder for chaining.
*/
public Builder clearCounterKey() {
counterKey_ = 0;
onChanged();
return this;
}
private long left_ ;
/**
*
*剩余配额数,应答返回,允许为负数
*
*
* int64 left = 2;
* @return The left.
*/
@java.lang.Override
public long getLeft() {
return left_;
}
/**
*
*剩余配额数,应答返回,允许为负数
*
*
* int64 left = 2;
* @param value The left to set.
* @return This builder for chaining.
*/
public Builder setLeft(long value) {
left_ = value;
onChanged();
return this;
}
/**
*
*剩余配额数,应答返回,允许为负数
*
*
* int64 left = 2;
* @return This builder for chaining.
*/
public Builder clearLeft() {
left_ = 0L;
onChanged();
return this;
}
private int mode_ = 0;
/**
*
*当前限流模式
*
*
* .polaris.metric.v2.Mode mode = 3;
* @return The enum numeric value on the wire for mode.
*/
@java.lang.Override public int getModeValue() {
return mode_;
}
/**
*
*当前限流模式
*
*
* .polaris.metric.v2.Mode mode = 3;
* @param value The enum numeric value on the wire for mode to set.
* @return This builder for chaining.
*/
public Builder setModeValue(int value) {
mode_ = value;
onChanged();
return this;
}
/**
*
*当前限流模式
*
*
* .polaris.metric.v2.Mode mode = 3;
* @return The mode.
*/
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode getMode() {
@SuppressWarnings("deprecation")
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode result = com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.valueOf(mode_);
return result == null ? com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode.UNRECOGNIZED : result;
}
/**
*
*当前限流模式
*
*
* .polaris.metric.v2.Mode mode = 3;
* @param value The mode to set.
* @return This builder for chaining.
*/
public Builder setMode(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.Mode value) {
if (value == null) {
throw new NullPointerException();
}
mode_ = value.getNumber();
onChanged();
return this;
}
/**
*
*当前限流模式
*
*
* .polaris.metric.v2.Mode mode = 3;
* @return This builder for chaining.
*/
public Builder clearMode() {
mode_ = 0;
onChanged();
return this;
}
private int clientCount_ ;
/**
*
*接入的客户端数量
*
*
* uint32 clientCount = 4;
* @return The clientCount.
*/
@java.lang.Override
public int getClientCount() {
return clientCount_;
}
/**
*
*接入的客户端数量
*
*
* uint32 clientCount = 4;
* @param value The clientCount to set.
* @return This builder for chaining.
*/
public Builder setClientCount(int value) {
clientCount_ = value;
onChanged();
return this;
}
/**
*
*接入的客户端数量
*
*
* uint32 clientCount = 4;
* @return This builder for chaining.
*/
public Builder clearClientCount() {
clientCount_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.QuotaLeft)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.QuotaLeft)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QuotaLeft parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.QuotaLeft getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TimeAdjustRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.TimeAdjustRequest)
com.google.protobuf.MessageOrBuilder {
}
/**
*
*时间点对齐的请求
*
*
* Protobuf type {@code polaris.metric.v2.TimeAdjustRequest}
*/
public static final class TimeAdjustRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.TimeAdjustRequest)
TimeAdjustRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use TimeAdjustRequest.newBuilder() to construct.
private TimeAdjustRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TimeAdjustRequest() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TimeAdjustRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_TimeAdjustRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_TimeAdjustRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest) obj;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*时间点对齐的请求
*
*
* Protobuf type {@code polaris.metric.v2.TimeAdjustRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.TimeAdjustRequest)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_TimeAdjustRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_TimeAdjustRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_TimeAdjustRequest_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.TimeAdjustRequest)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.TimeAdjustRequest)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TimeAdjustRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TimeAdjustResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:polaris.metric.v2.TimeAdjustResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
*服务器时间点,毫秒
*
*
* int64 serverTimestamp = 1;
* @return The serverTimestamp.
*/
long getServerTimestamp();
}
/**
*
*时间点对齐的应答
*
*
* Protobuf type {@code polaris.metric.v2.TimeAdjustResponse}
*/
public static final class TimeAdjustResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polaris.metric.v2.TimeAdjustResponse)
TimeAdjustResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use TimeAdjustResponse.newBuilder() to construct.
private TimeAdjustResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TimeAdjustResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TimeAdjustResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_TimeAdjustResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_TimeAdjustResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse.Builder.class);
}
public static final int SERVERTIMESTAMP_FIELD_NUMBER = 1;
private long serverTimestamp_;
/**
*
*服务器时间点,毫秒
*
*
* int64 serverTimestamp = 1;
* @return The serverTimestamp.
*/
@java.lang.Override
public long getServerTimestamp() {
return serverTimestamp_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (serverTimestamp_ != 0L) {
output.writeInt64(1, serverTimestamp_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (serverTimestamp_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, serverTimestamp_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse)) {
return super.equals(obj);
}
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse other = (com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse) obj;
if (getServerTimestamp()
!= other.getServerTimestamp()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SERVERTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getServerTimestamp());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*时间点对齐的应答
*
*
* Protobuf type {@code polaris.metric.v2.TimeAdjustResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polaris.metric.v2.TimeAdjustResponse)
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_TimeAdjustResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_TimeAdjustResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse.class, com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse.Builder.class);
}
// Construct using com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
serverTimestamp_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.internal_static_polaris_metric_v2_TimeAdjustResponse_descriptor;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse getDefaultInstanceForType() {
return com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse build() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse buildPartial() {
com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse result = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse(this);
result.serverTimestamp_ = serverTimestamp_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse) {
return mergeFrom((com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse other) {
if (other == com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse.getDefaultInstance()) return this;
if (other.getServerTimestamp() != 0L) {
setServerTimestamp(other.getServerTimestamp());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
serverTimestamp_ = input.readInt64();
break;
} // case 8
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private long serverTimestamp_ ;
/**
*
*服务器时间点,毫秒
*
*
* int64 serverTimestamp = 1;
* @return The serverTimestamp.
*/
@java.lang.Override
public long getServerTimestamp() {
return serverTimestamp_;
}
/**
*
*服务器时间点,毫秒
*
*
* int64 serverTimestamp = 1;
* @param value The serverTimestamp to set.
* @return This builder for chaining.
*/
public Builder setServerTimestamp(long value) {
serverTimestamp_ = value;
onChanged();
return this;
}
/**
*
*服务器时间点,毫秒
*
*
* int64 serverTimestamp = 1;
* @return This builder for chaining.
*/
public Builder clearServerTimestamp() {
serverTimestamp_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polaris.metric.v2.TimeAdjustResponse)
}
// @@protoc_insertion_point(class_scope:polaris.metric.v2.TimeAdjustResponse)
private static final com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse();
}
public static com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TimeAdjustResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.ratelimit.client.pb.RatelimitV2.TimeAdjustResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_RateLimitRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_RateLimitRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_RateLimitResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_RateLimitResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_RateLimitInitRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_RateLimitInitRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_RateLimitInitResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_RateLimitInitResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_RateLimitReportRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_RateLimitReportRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_RateLimitReportResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_RateLimitReportResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_LimitTarget_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_LimitTarget_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_QuotaTotal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_QuotaTotal_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_QuotaCounter_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_QuotaCounter_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_QuotaSum_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_QuotaSum_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_QuotaLeft_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_QuotaLeft_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_TimeAdjustRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_TimeAdjustRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polaris_metric_v2_TimeAdjustResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polaris_metric_v2_TimeAdjustResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\022ratelimit_v2.proto\022\021polaris.metric.v2\"" +
"\322\001\n\020RateLimitRequest\022,\n\003cmd\030\001 \001(\0162\037.pola" +
"ris.metric.v2.RateLimitCmd\022E\n\024rateLimitI" +
"nitRequest\030\002 \001(\0132\'.polaris.metric.v2.Rat" +
"eLimitInitRequest\022I\n\026rateLimitReportRequ" +
"est\030\003 \001(\0132).polaris.metric.v2.RateLimitR" +
"eportRequest\"\327\001\n\021RateLimitResponse\022,\n\003cm" +
"d\030\001 \001(\0162\037.polaris.metric.v2.RateLimitCmd" +
"\022G\n\025rateLimitInitResponse\030\002 \001(\0132(.polari" +
"s.metric.v2.RateLimitInitResponse\022K\n\027rat" +
"eLimitReportResponse\030\003 \001(\0132*.polaris.met" +
"ric.v2.RateLimitReportResponse\"\302\001\n\024RateL" +
"imitInitRequest\022.\n\006target\030\001 \001(\0132\036.polari" +
"s.metric.v2.LimitTarget\022\020\n\010clientId\030\002 \001(" +
"\t\022-\n\006totals\030\003 \003(\0132\035.polaris.metric.v2.Qu" +
"otaTotal\022\022\n\nslideCount\030\004 \001(\r\022%\n\004mode\030\005 \001" +
"(\0162\027.polaris.metric.v2.Mode\"\302\001\n\025RateLimi" +
"tInitResponse\022\014\n\004code\030\001 \001(\r\022.\n\006target\030\002 " +
"\001(\0132\036.polaris.metric.v2.LimitTarget\022\021\n\tc" +
"lientKey\030\003 \001(\r\0221\n\010counters\030\005 \003(\0132\037.polar" +
"is.metric.v2.QuotaCounter\022\022\n\nslideCount\030" +
"\006 \001(\r\022\021\n\ttimestamp\030\007 \001(\003\"n\n\026RateLimitRep" +
"ortRequest\022\021\n\tclientKey\030\001 \001(\r\022.\n\tquotaUs" +
"es\030\002 \003(\0132\033.polaris.metric.v2.QuotaSum\022\021\n" +
"\ttimestamp\030\003 \001(\003\"l\n\027RateLimitReportRespo" +
"nse\022\014\n\004code\030\001 \001(\r\0220\n\nquotaLefts\030\002 \003(\0132\034." +
"polaris.metric.v2.QuotaLeft\022\021\n\ttimestamp" +
"\030\003 \001(\003\"A\n\013LimitTarget\022\021\n\tnamespace\030\001 \001(\t" +
"\022\017\n\007service\030\002 \001(\t\022\016\n\006labels\030\003 \001(\t\"]\n\nQuo" +
"taTotal\022*\n\004mode\030\001 \001(\0162\034.polaris.metric.v" +
"2.QuotaMode\022\020\n\010duration\030\002 \001(\r\022\021\n\tmaxAmou" +
"nt\030\003 \001(\r\"~\n\014QuotaCounter\022\020\n\010duration\030\001 \001" +
"(\r\022\022\n\ncounterKey\030\002 \001(\r\022\014\n\004left\030\003 \001(\003\022%\n\004" +
"mode\030\004 \001(\0162\027.polaris.metric.v2.Mode\022\023\n\013c" +
"lientCount\030\005 \001(\r\"=\n\010QuotaSum\022\022\n\ncounterK" +
"ey\030\001 \001(\r\022\014\n\004used\030\002 \001(\r\022\017\n\007limited\030\003 \001(\r\"" +
"i\n\tQuotaLeft\022\022\n\ncounterKey\030\001 \001(\r\022\014\n\004left" +
"\030\002 \001(\003\022%\n\004mode\030\003 \001(\0162\027.polaris.metric.v2" +
".Mode\022\023\n\013clientCount\030\004 \001(\r\"\023\n\021TimeAdjust" +
"Request\"-\n\022TimeAdjustResponse\022\027\n\017serverT" +
"imestamp\030\001 \001(\003*%\n\014RateLimitCmd\022\010\n\004INIT\020\000" +
"\022\013\n\007ACQUIRE\020\001*7\n\004Mode\022\014\n\010ADAPTIVE\020\000\022\020\n\014B" +
"ATCH_OCCUPY\020\001\022\017\n\013BATCH_SHARE\020\002*\"\n\tQuotaM" +
"ode\022\t\n\005WHOLE\020\000\022\n\n\006DIVIDE\020\001B-\n\'com.tencen" +
"t.polaris.ratelimit.client.pbZ\002v2b\006proto" +
"3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_polaris_metric_v2_RateLimitRequest_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_polaris_metric_v2_RateLimitRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_RateLimitRequest_descriptor,
new java.lang.String[] { "Cmd", "RateLimitInitRequest", "RateLimitReportRequest", });
internal_static_polaris_metric_v2_RateLimitResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_polaris_metric_v2_RateLimitResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_RateLimitResponse_descriptor,
new java.lang.String[] { "Cmd", "RateLimitInitResponse", "RateLimitReportResponse", });
internal_static_polaris_metric_v2_RateLimitInitRequest_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_polaris_metric_v2_RateLimitInitRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_RateLimitInitRequest_descriptor,
new java.lang.String[] { "Target", "ClientId", "Totals", "SlideCount", "Mode", });
internal_static_polaris_metric_v2_RateLimitInitResponse_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_polaris_metric_v2_RateLimitInitResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_RateLimitInitResponse_descriptor,
new java.lang.String[] { "Code", "Target", "ClientKey", "Counters", "SlideCount", "Timestamp", });
internal_static_polaris_metric_v2_RateLimitReportRequest_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_polaris_metric_v2_RateLimitReportRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_RateLimitReportRequest_descriptor,
new java.lang.String[] { "ClientKey", "QuotaUses", "Timestamp", });
internal_static_polaris_metric_v2_RateLimitReportResponse_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_polaris_metric_v2_RateLimitReportResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_RateLimitReportResponse_descriptor,
new java.lang.String[] { "Code", "QuotaLefts", "Timestamp", });
internal_static_polaris_metric_v2_LimitTarget_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_polaris_metric_v2_LimitTarget_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_LimitTarget_descriptor,
new java.lang.String[] { "Namespace", "Service", "Labels", });
internal_static_polaris_metric_v2_QuotaTotal_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_polaris_metric_v2_QuotaTotal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_QuotaTotal_descriptor,
new java.lang.String[] { "Mode", "Duration", "MaxAmount", });
internal_static_polaris_metric_v2_QuotaCounter_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_polaris_metric_v2_QuotaCounter_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_QuotaCounter_descriptor,
new java.lang.String[] { "Duration", "CounterKey", "Left", "Mode", "ClientCount", });
internal_static_polaris_metric_v2_QuotaSum_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_polaris_metric_v2_QuotaSum_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_QuotaSum_descriptor,
new java.lang.String[] { "CounterKey", "Used", "Limited", });
internal_static_polaris_metric_v2_QuotaLeft_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_polaris_metric_v2_QuotaLeft_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_QuotaLeft_descriptor,
new java.lang.String[] { "CounterKey", "Left", "Mode", "ClientCount", });
internal_static_polaris_metric_v2_TimeAdjustRequest_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_polaris_metric_v2_TimeAdjustRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_TimeAdjustRequest_descriptor,
new java.lang.String[] { });
internal_static_polaris_metric_v2_TimeAdjustResponse_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_polaris_metric_v2_TimeAdjustResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polaris_metric_v2_TimeAdjustResponse_descriptor,
new java.lang.String[] { "ServerTimestamp", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy