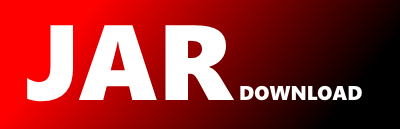
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: service.proto
package com.tencent.polaris.specification.api.v1.service.manage;
public final class ServiceProto {
private ServiceProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code v1.AliasType}
*/
public enum AliasType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* DEFAULT = 0;
*/
DEFAULT(0),
/**
* CL5SID = 1;
*/
CL5SID(1),
UNRECOGNIZED(-1),
;
/**
* DEFAULT = 0;
*/
public static final int DEFAULT_VALUE = 0;
/**
* CL5SID = 1;
*/
public static final int CL5SID_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static AliasType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static AliasType forNumber(int value) {
switch (value) {
case 0: return DEFAULT;
case 1: return CL5SID;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
AliasType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public AliasType findValueByNumber(int number) {
return AliasType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.getDescriptor().getEnumTypes().get(0);
}
private static final AliasType[] VALUES = values();
public static AliasType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private AliasType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:v1.AliasType)
}
public interface ServiceOrBuilder extends
// @@protoc_insertion_point(interface_extends:v1.Service)
com.google.protobuf.MessageOrBuilder {
/**
* .google.protobuf.StringValue name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* .google.protobuf.StringValue name = 1;
* @return The name.
*/
com.google.protobuf.StringValue getName();
/**
* .google.protobuf.StringValue name = 1;
*/
com.google.protobuf.StringValueOrBuilder getNameOrBuilder();
/**
* .google.protobuf.StringValue namespace = 2;
* @return Whether the namespace field is set.
*/
boolean hasNamespace();
/**
* .google.protobuf.StringValue namespace = 2;
* @return The namespace.
*/
com.google.protobuf.StringValue getNamespace();
/**
* .google.protobuf.StringValue namespace = 2;
*/
com.google.protobuf.StringValueOrBuilder getNamespaceOrBuilder();
/**
* map<string, string> metadata = 3;
*/
int getMetadataCount();
/**
* map<string, string> metadata = 3;
*/
boolean containsMetadata(
java.lang.String key);
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getMetadata();
/**
* map<string, string> metadata = 3;
*/
java.util.Map
getMetadataMap();
/**
* map<string, string> metadata = 3;
*/
/* nullable */
java.lang.String getMetadataOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> metadata = 3;
*/
java.lang.String getMetadataOrThrow(
java.lang.String key);
/**
* .google.protobuf.StringValue ports = 4;
* @return Whether the ports field is set.
*/
boolean hasPorts();
/**
* .google.protobuf.StringValue ports = 4;
* @return The ports.
*/
com.google.protobuf.StringValue getPorts();
/**
* .google.protobuf.StringValue ports = 4;
*/
com.google.protobuf.StringValueOrBuilder getPortsOrBuilder();
/**
* .google.protobuf.StringValue business = 5;
* @return Whether the business field is set.
*/
boolean hasBusiness();
/**
* .google.protobuf.StringValue business = 5;
* @return The business.
*/
com.google.protobuf.StringValue getBusiness();
/**
* .google.protobuf.StringValue business = 5;
*/
com.google.protobuf.StringValueOrBuilder getBusinessOrBuilder();
/**
* .google.protobuf.StringValue department = 6;
* @return Whether the department field is set.
*/
boolean hasDepartment();
/**
* .google.protobuf.StringValue department = 6;
* @return The department.
*/
com.google.protobuf.StringValue getDepartment();
/**
* .google.protobuf.StringValue department = 6;
*/
com.google.protobuf.StringValueOrBuilder getDepartmentOrBuilder();
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
* @return Whether the cmdbMod1 field is set.
*/
boolean hasCmdbMod1();
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
* @return The cmdbMod1.
*/
com.google.protobuf.StringValue getCmdbMod1();
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
*/
com.google.protobuf.StringValueOrBuilder getCmdbMod1OrBuilder();
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
* @return Whether the cmdbMod2 field is set.
*/
boolean hasCmdbMod2();
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
* @return The cmdbMod2.
*/
com.google.protobuf.StringValue getCmdbMod2();
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
*/
com.google.protobuf.StringValueOrBuilder getCmdbMod2OrBuilder();
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
* @return Whether the cmdbMod3 field is set.
*/
boolean hasCmdbMod3();
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
* @return The cmdbMod3.
*/
com.google.protobuf.StringValue getCmdbMod3();
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
*/
com.google.protobuf.StringValueOrBuilder getCmdbMod3OrBuilder();
/**
* .google.protobuf.StringValue comment = 10;
* @return Whether the comment field is set.
*/
boolean hasComment();
/**
* .google.protobuf.StringValue comment = 10;
* @return The comment.
*/
com.google.protobuf.StringValue getComment();
/**
* .google.protobuf.StringValue comment = 10;
*/
com.google.protobuf.StringValueOrBuilder getCommentOrBuilder();
/**
* .google.protobuf.StringValue owners = 11;
* @return Whether the owners field is set.
*/
boolean hasOwners();
/**
* .google.protobuf.StringValue owners = 11;
* @return The owners.
*/
com.google.protobuf.StringValue getOwners();
/**
* .google.protobuf.StringValue owners = 11;
*/
com.google.protobuf.StringValueOrBuilder getOwnersOrBuilder();
/**
* .google.protobuf.StringValue token = 12;
* @return Whether the token field is set.
*/
boolean hasToken();
/**
* .google.protobuf.StringValue token = 12;
* @return The token.
*/
com.google.protobuf.StringValue getToken();
/**
* .google.protobuf.StringValue token = 12;
*/
com.google.protobuf.StringValueOrBuilder getTokenOrBuilder();
/**
* .google.protobuf.StringValue ctime = 13;
* @return Whether the ctime field is set.
*/
boolean hasCtime();
/**
* .google.protobuf.StringValue ctime = 13;
* @return The ctime.
*/
com.google.protobuf.StringValue getCtime();
/**
* .google.protobuf.StringValue ctime = 13;
*/
com.google.protobuf.StringValueOrBuilder getCtimeOrBuilder();
/**
* .google.protobuf.StringValue mtime = 14;
* @return Whether the mtime field is set.
*/
boolean hasMtime();
/**
* .google.protobuf.StringValue mtime = 14;
* @return The mtime.
*/
com.google.protobuf.StringValue getMtime();
/**
* .google.protobuf.StringValue mtime = 14;
*/
com.google.protobuf.StringValueOrBuilder getMtimeOrBuilder();
/**
* .google.protobuf.StringValue revision = 15;
* @return Whether the revision field is set.
*/
boolean hasRevision();
/**
* .google.protobuf.StringValue revision = 15;
* @return The revision.
*/
com.google.protobuf.StringValue getRevision();
/**
* .google.protobuf.StringValue revision = 15;
*/
com.google.protobuf.StringValueOrBuilder getRevisionOrBuilder();
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
* @return Whether the platformId field is set.
*/
boolean hasPlatformId();
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
* @return The platformId.
*/
com.google.protobuf.StringValue getPlatformId();
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
*/
com.google.protobuf.StringValueOrBuilder getPlatformIdOrBuilder();
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
* @return Whether the totalInstanceCount field is set.
*/
boolean hasTotalInstanceCount();
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
* @return The totalInstanceCount.
*/
com.google.protobuf.UInt32Value getTotalInstanceCount();
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
*/
com.google.protobuf.UInt32ValueOrBuilder getTotalInstanceCountOrBuilder();
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
* @return Whether the healthyInstanceCount field is set.
*/
boolean hasHealthyInstanceCount();
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
* @return The healthyInstanceCount.
*/
com.google.protobuf.UInt32Value getHealthyInstanceCount();
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
*/
com.google.protobuf.UInt32ValueOrBuilder getHealthyInstanceCountOrBuilder();
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
java.util.List
getUserIdsList();
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
com.google.protobuf.StringValue getUserIds(int index);
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
int getUserIdsCount();
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
java.util.List extends com.google.protobuf.StringValueOrBuilder>
getUserIdsOrBuilderList();
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
com.google.protobuf.StringValueOrBuilder getUserIdsOrBuilder(
int index);
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
java.util.List
getGroupIdsList();
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
com.google.protobuf.StringValue getGroupIds(int index);
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
int getGroupIdsCount();
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
java.util.List extends com.google.protobuf.StringValueOrBuilder>
getGroupIdsOrBuilderList();
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
com.google.protobuf.StringValueOrBuilder getGroupIdsOrBuilder(
int index);
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
java.util.List
getRemoveUserIdsList();
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
com.google.protobuf.StringValue getRemoveUserIds(int index);
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
int getRemoveUserIdsCount();
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
java.util.List extends com.google.protobuf.StringValueOrBuilder>
getRemoveUserIdsOrBuilderList();
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
com.google.protobuf.StringValueOrBuilder getRemoveUserIdsOrBuilder(
int index);
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
java.util.List
getRemoveGroupIdsList();
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
com.google.protobuf.StringValue getRemoveGroupIds(int index);
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
int getRemoveGroupIdsCount();
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
java.util.List extends com.google.protobuf.StringValueOrBuilder>
getRemoveGroupIdsOrBuilderList();
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
com.google.protobuf.StringValueOrBuilder getRemoveGroupIdsOrBuilder(
int index);
/**
* .google.protobuf.StringValue id = 21;
* @return Whether the id field is set.
*/
boolean hasId();
/**
* .google.protobuf.StringValue id = 21;
* @return The id.
*/
com.google.protobuf.StringValue getId();
/**
* .google.protobuf.StringValue id = 21;
*/
com.google.protobuf.StringValueOrBuilder getIdOrBuilder();
/**
* .google.protobuf.BoolValue editable = 24;
* @return Whether the editable field is set.
*/
boolean hasEditable();
/**
* .google.protobuf.BoolValue editable = 24;
* @return The editable.
*/
com.google.protobuf.BoolValue getEditable();
/**
* .google.protobuf.BoolValue editable = 24;
*/
com.google.protobuf.BoolValueOrBuilder getEditableOrBuilder();
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
java.util.List
getExportToList();
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
com.google.protobuf.StringValue getExportTo(int index);
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
int getExportToCount();
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
java.util.List extends com.google.protobuf.StringValueOrBuilder>
getExportToOrBuilderList();
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
com.google.protobuf.StringValueOrBuilder getExportToOrBuilder(
int index);
}
/**
* Protobuf type {@code v1.Service}
*/
public static final class Service extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:v1.Service)
ServiceOrBuilder {
private static final long serialVersionUID = 0L;
// Use Service.newBuilder() to construct.
private Service(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Service() {
userIds_ = java.util.Collections.emptyList();
groupIds_ = java.util.Collections.emptyList();
removeUserIds_ = java.util.Collections.emptyList();
removeGroupIds_ = java.util.Collections.emptyList();
exportTo_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Service();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Service_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 3:
return internalGetMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Service_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service.class, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private com.google.protobuf.StringValue name_;
/**
* .google.protobuf.StringValue name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return name_ != null;
}
/**
* .google.protobuf.StringValue name = 1;
* @return The name.
*/
@java.lang.Override
public com.google.protobuf.StringValue getName() {
return name_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : name_;
}
/**
* .google.protobuf.StringValue name = 1;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getNameOrBuilder() {
return getName();
}
public static final int NAMESPACE_FIELD_NUMBER = 2;
private com.google.protobuf.StringValue namespace_;
/**
* .google.protobuf.StringValue namespace = 2;
* @return Whether the namespace field is set.
*/
@java.lang.Override
public boolean hasNamespace() {
return namespace_ != null;
}
/**
* .google.protobuf.StringValue namespace = 2;
* @return The namespace.
*/
@java.lang.Override
public com.google.protobuf.StringValue getNamespace() {
return namespace_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : namespace_;
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getNamespaceOrBuilder() {
return getNamespace();
}
public static final int METADATA_FIELD_NUMBER = 3;
private static final class MetadataDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Service_MetadataEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> metadata_;
private com.google.protobuf.MapField
internalGetMetadata() {
if (metadata_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
return metadata_;
}
public int getMetadataCount() {
return internalGetMetadata().getMap().size();
}
/**
* map<string, string> metadata = 3;
*/
@java.lang.Override
public boolean containsMetadata(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetMetadata().getMap().containsKey(key);
}
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getMetadata() {
return getMetadataMap();
}
/**
* map<string, string> metadata = 3;
*/
@java.lang.Override
public java.util.Map getMetadataMap() {
return internalGetMetadata().getMap();
}
/**
* map<string, string> metadata = 3;
*/
@java.lang.Override
public java.lang.String getMetadataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetMetadata().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> metadata = 3;
*/
@java.lang.Override
public java.lang.String getMetadataOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetMetadata().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int PORTS_FIELD_NUMBER = 4;
private com.google.protobuf.StringValue ports_;
/**
* .google.protobuf.StringValue ports = 4;
* @return Whether the ports field is set.
*/
@java.lang.Override
public boolean hasPorts() {
return ports_ != null;
}
/**
* .google.protobuf.StringValue ports = 4;
* @return The ports.
*/
@java.lang.Override
public com.google.protobuf.StringValue getPorts() {
return ports_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : ports_;
}
/**
* .google.protobuf.StringValue ports = 4;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getPortsOrBuilder() {
return getPorts();
}
public static final int BUSINESS_FIELD_NUMBER = 5;
private com.google.protobuf.StringValue business_;
/**
* .google.protobuf.StringValue business = 5;
* @return Whether the business field is set.
*/
@java.lang.Override
public boolean hasBusiness() {
return business_ != null;
}
/**
* .google.protobuf.StringValue business = 5;
* @return The business.
*/
@java.lang.Override
public com.google.protobuf.StringValue getBusiness() {
return business_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : business_;
}
/**
* .google.protobuf.StringValue business = 5;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getBusinessOrBuilder() {
return getBusiness();
}
public static final int DEPARTMENT_FIELD_NUMBER = 6;
private com.google.protobuf.StringValue department_;
/**
* .google.protobuf.StringValue department = 6;
* @return Whether the department field is set.
*/
@java.lang.Override
public boolean hasDepartment() {
return department_ != null;
}
/**
* .google.protobuf.StringValue department = 6;
* @return The department.
*/
@java.lang.Override
public com.google.protobuf.StringValue getDepartment() {
return department_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : department_;
}
/**
* .google.protobuf.StringValue department = 6;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getDepartmentOrBuilder() {
return getDepartment();
}
public static final int CMDB_MOD1_FIELD_NUMBER = 7;
private com.google.protobuf.StringValue cmdbMod1_;
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
* @return Whether the cmdbMod1 field is set.
*/
@java.lang.Override
public boolean hasCmdbMod1() {
return cmdbMod1_ != null;
}
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
* @return The cmdbMod1.
*/
@java.lang.Override
public com.google.protobuf.StringValue getCmdbMod1() {
return cmdbMod1_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : cmdbMod1_;
}
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getCmdbMod1OrBuilder() {
return getCmdbMod1();
}
public static final int CMDB_MOD2_FIELD_NUMBER = 8;
private com.google.protobuf.StringValue cmdbMod2_;
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
* @return Whether the cmdbMod2 field is set.
*/
@java.lang.Override
public boolean hasCmdbMod2() {
return cmdbMod2_ != null;
}
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
* @return The cmdbMod2.
*/
@java.lang.Override
public com.google.protobuf.StringValue getCmdbMod2() {
return cmdbMod2_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : cmdbMod2_;
}
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getCmdbMod2OrBuilder() {
return getCmdbMod2();
}
public static final int CMDB_MOD3_FIELD_NUMBER = 9;
private com.google.protobuf.StringValue cmdbMod3_;
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
* @return Whether the cmdbMod3 field is set.
*/
@java.lang.Override
public boolean hasCmdbMod3() {
return cmdbMod3_ != null;
}
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
* @return The cmdbMod3.
*/
@java.lang.Override
public com.google.protobuf.StringValue getCmdbMod3() {
return cmdbMod3_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : cmdbMod3_;
}
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getCmdbMod3OrBuilder() {
return getCmdbMod3();
}
public static final int COMMENT_FIELD_NUMBER = 10;
private com.google.protobuf.StringValue comment_;
/**
* .google.protobuf.StringValue comment = 10;
* @return Whether the comment field is set.
*/
@java.lang.Override
public boolean hasComment() {
return comment_ != null;
}
/**
* .google.protobuf.StringValue comment = 10;
* @return The comment.
*/
@java.lang.Override
public com.google.protobuf.StringValue getComment() {
return comment_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : comment_;
}
/**
* .google.protobuf.StringValue comment = 10;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getCommentOrBuilder() {
return getComment();
}
public static final int OWNERS_FIELD_NUMBER = 11;
private com.google.protobuf.StringValue owners_;
/**
* .google.protobuf.StringValue owners = 11;
* @return Whether the owners field is set.
*/
@java.lang.Override
public boolean hasOwners() {
return owners_ != null;
}
/**
* .google.protobuf.StringValue owners = 11;
* @return The owners.
*/
@java.lang.Override
public com.google.protobuf.StringValue getOwners() {
return owners_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : owners_;
}
/**
* .google.protobuf.StringValue owners = 11;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getOwnersOrBuilder() {
return getOwners();
}
public static final int TOKEN_FIELD_NUMBER = 12;
private com.google.protobuf.StringValue token_;
/**
* .google.protobuf.StringValue token = 12;
* @return Whether the token field is set.
*/
@java.lang.Override
public boolean hasToken() {
return token_ != null;
}
/**
* .google.protobuf.StringValue token = 12;
* @return The token.
*/
@java.lang.Override
public com.google.protobuf.StringValue getToken() {
return token_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : token_;
}
/**
* .google.protobuf.StringValue token = 12;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getTokenOrBuilder() {
return getToken();
}
public static final int CTIME_FIELD_NUMBER = 13;
private com.google.protobuf.StringValue ctime_;
/**
* .google.protobuf.StringValue ctime = 13;
* @return Whether the ctime field is set.
*/
@java.lang.Override
public boolean hasCtime() {
return ctime_ != null;
}
/**
* .google.protobuf.StringValue ctime = 13;
* @return The ctime.
*/
@java.lang.Override
public com.google.protobuf.StringValue getCtime() {
return ctime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : ctime_;
}
/**
* .google.protobuf.StringValue ctime = 13;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getCtimeOrBuilder() {
return getCtime();
}
public static final int MTIME_FIELD_NUMBER = 14;
private com.google.protobuf.StringValue mtime_;
/**
* .google.protobuf.StringValue mtime = 14;
* @return Whether the mtime field is set.
*/
@java.lang.Override
public boolean hasMtime() {
return mtime_ != null;
}
/**
* .google.protobuf.StringValue mtime = 14;
* @return The mtime.
*/
@java.lang.Override
public com.google.protobuf.StringValue getMtime() {
return mtime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : mtime_;
}
/**
* .google.protobuf.StringValue mtime = 14;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getMtimeOrBuilder() {
return getMtime();
}
public static final int REVISION_FIELD_NUMBER = 15;
private com.google.protobuf.StringValue revision_;
/**
* .google.protobuf.StringValue revision = 15;
* @return Whether the revision field is set.
*/
@java.lang.Override
public boolean hasRevision() {
return revision_ != null;
}
/**
* .google.protobuf.StringValue revision = 15;
* @return The revision.
*/
@java.lang.Override
public com.google.protobuf.StringValue getRevision() {
return revision_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : revision_;
}
/**
* .google.protobuf.StringValue revision = 15;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getRevisionOrBuilder() {
return getRevision();
}
public static final int PLATFORM_ID_FIELD_NUMBER = 16;
private com.google.protobuf.StringValue platformId_;
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
* @return Whether the platformId field is set.
*/
@java.lang.Override
public boolean hasPlatformId() {
return platformId_ != null;
}
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
* @return The platformId.
*/
@java.lang.Override
public com.google.protobuf.StringValue getPlatformId() {
return platformId_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : platformId_;
}
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getPlatformIdOrBuilder() {
return getPlatformId();
}
public static final int TOTAL_INSTANCE_COUNT_FIELD_NUMBER = 17;
private com.google.protobuf.UInt32Value totalInstanceCount_;
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
* @return Whether the totalInstanceCount field is set.
*/
@java.lang.Override
public boolean hasTotalInstanceCount() {
return totalInstanceCount_ != null;
}
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
* @return The totalInstanceCount.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getTotalInstanceCount() {
return totalInstanceCount_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : totalInstanceCount_;
}
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getTotalInstanceCountOrBuilder() {
return getTotalInstanceCount();
}
public static final int HEALTHY_INSTANCE_COUNT_FIELD_NUMBER = 18;
private com.google.protobuf.UInt32Value healthyInstanceCount_;
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
* @return Whether the healthyInstanceCount field is set.
*/
@java.lang.Override
public boolean hasHealthyInstanceCount() {
return healthyInstanceCount_ != null;
}
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
* @return The healthyInstanceCount.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getHealthyInstanceCount() {
return healthyInstanceCount_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : healthyInstanceCount_;
}
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getHealthyInstanceCountOrBuilder() {
return getHealthyInstanceCount();
}
public static final int USER_IDS_FIELD_NUMBER = 19;
private java.util.List userIds_;
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
@java.lang.Override
public java.util.List getUserIdsList() {
return userIds_;
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
@java.lang.Override
public java.util.List extends com.google.protobuf.StringValueOrBuilder>
getUserIdsOrBuilderList() {
return userIds_;
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
@java.lang.Override
public int getUserIdsCount() {
return userIds_.size();
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
@java.lang.Override
public com.google.protobuf.StringValue getUserIds(int index) {
return userIds_.get(index);
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getUserIdsOrBuilder(
int index) {
return userIds_.get(index);
}
public static final int GROUP_IDS_FIELD_NUMBER = 20;
private java.util.List groupIds_;
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
@java.lang.Override
public java.util.List getGroupIdsList() {
return groupIds_;
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
@java.lang.Override
public java.util.List extends com.google.protobuf.StringValueOrBuilder>
getGroupIdsOrBuilderList() {
return groupIds_;
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
@java.lang.Override
public int getGroupIdsCount() {
return groupIds_.size();
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
@java.lang.Override
public com.google.protobuf.StringValue getGroupIds(int index) {
return groupIds_.get(index);
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getGroupIdsOrBuilder(
int index) {
return groupIds_.get(index);
}
public static final int REMOVE_USER_IDS_FIELD_NUMBER = 22;
private java.util.List removeUserIds_;
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
@java.lang.Override
public java.util.List getRemoveUserIdsList() {
return removeUserIds_;
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
@java.lang.Override
public java.util.List extends com.google.protobuf.StringValueOrBuilder>
getRemoveUserIdsOrBuilderList() {
return removeUserIds_;
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
@java.lang.Override
public int getRemoveUserIdsCount() {
return removeUserIds_.size();
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
@java.lang.Override
public com.google.protobuf.StringValue getRemoveUserIds(int index) {
return removeUserIds_.get(index);
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getRemoveUserIdsOrBuilder(
int index) {
return removeUserIds_.get(index);
}
public static final int REMOVE_GROUP_IDS_FIELD_NUMBER = 23;
private java.util.List removeGroupIds_;
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
@java.lang.Override
public java.util.List getRemoveGroupIdsList() {
return removeGroupIds_;
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
@java.lang.Override
public java.util.List extends com.google.protobuf.StringValueOrBuilder>
getRemoveGroupIdsOrBuilderList() {
return removeGroupIds_;
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
@java.lang.Override
public int getRemoveGroupIdsCount() {
return removeGroupIds_.size();
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
@java.lang.Override
public com.google.protobuf.StringValue getRemoveGroupIds(int index) {
return removeGroupIds_.get(index);
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getRemoveGroupIdsOrBuilder(
int index) {
return removeGroupIds_.get(index);
}
public static final int ID_FIELD_NUMBER = 21;
private com.google.protobuf.StringValue id_;
/**
* .google.protobuf.StringValue id = 21;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return id_ != null;
}
/**
* .google.protobuf.StringValue id = 21;
* @return The id.
*/
@java.lang.Override
public com.google.protobuf.StringValue getId() {
return id_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : id_;
}
/**
* .google.protobuf.StringValue id = 21;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getIdOrBuilder() {
return getId();
}
public static final int EDITABLE_FIELD_NUMBER = 24;
private com.google.protobuf.BoolValue editable_;
/**
* .google.protobuf.BoolValue editable = 24;
* @return Whether the editable field is set.
*/
@java.lang.Override
public boolean hasEditable() {
return editable_ != null;
}
/**
* .google.protobuf.BoolValue editable = 24;
* @return The editable.
*/
@java.lang.Override
public com.google.protobuf.BoolValue getEditable() {
return editable_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : editable_;
}
/**
* .google.protobuf.BoolValue editable = 24;
*/
@java.lang.Override
public com.google.protobuf.BoolValueOrBuilder getEditableOrBuilder() {
return getEditable();
}
public static final int EXPORT_TO_FIELD_NUMBER = 25;
private java.util.List exportTo_;
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
@java.lang.Override
public java.util.List getExportToList() {
return exportTo_;
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
@java.lang.Override
public java.util.List extends com.google.protobuf.StringValueOrBuilder>
getExportToOrBuilderList() {
return exportTo_;
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
@java.lang.Override
public int getExportToCount() {
return exportTo_.size();
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
@java.lang.Override
public com.google.protobuf.StringValue getExportTo(int index) {
return exportTo_.get(index);
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getExportToOrBuilder(
int index) {
return exportTo_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (name_ != null) {
output.writeMessage(1, getName());
}
if (namespace_ != null) {
output.writeMessage(2, getNamespace());
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetMetadata(),
MetadataDefaultEntryHolder.defaultEntry,
3);
if (ports_ != null) {
output.writeMessage(4, getPorts());
}
if (business_ != null) {
output.writeMessage(5, getBusiness());
}
if (department_ != null) {
output.writeMessage(6, getDepartment());
}
if (cmdbMod1_ != null) {
output.writeMessage(7, getCmdbMod1());
}
if (cmdbMod2_ != null) {
output.writeMessage(8, getCmdbMod2());
}
if (cmdbMod3_ != null) {
output.writeMessage(9, getCmdbMod3());
}
if (comment_ != null) {
output.writeMessage(10, getComment());
}
if (owners_ != null) {
output.writeMessage(11, getOwners());
}
if (token_ != null) {
output.writeMessage(12, getToken());
}
if (ctime_ != null) {
output.writeMessage(13, getCtime());
}
if (mtime_ != null) {
output.writeMessage(14, getMtime());
}
if (revision_ != null) {
output.writeMessage(15, getRevision());
}
if (platformId_ != null) {
output.writeMessage(16, getPlatformId());
}
if (totalInstanceCount_ != null) {
output.writeMessage(17, getTotalInstanceCount());
}
if (healthyInstanceCount_ != null) {
output.writeMessage(18, getHealthyInstanceCount());
}
for (int i = 0; i < userIds_.size(); i++) {
output.writeMessage(19, userIds_.get(i));
}
for (int i = 0; i < groupIds_.size(); i++) {
output.writeMessage(20, groupIds_.get(i));
}
if (id_ != null) {
output.writeMessage(21, getId());
}
for (int i = 0; i < removeUserIds_.size(); i++) {
output.writeMessage(22, removeUserIds_.get(i));
}
for (int i = 0; i < removeGroupIds_.size(); i++) {
output.writeMessage(23, removeGroupIds_.get(i));
}
if (editable_ != null) {
output.writeMessage(24, getEditable());
}
for (int i = 0; i < exportTo_.size(); i++) {
output.writeMessage(25, exportTo_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (name_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getName());
}
if (namespace_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getNamespace());
}
for (java.util.Map.Entry entry
: internalGetMetadata().getMap().entrySet()) {
com.google.protobuf.MapEntry
metadata__ = MetadataDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, metadata__);
}
if (ports_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getPorts());
}
if (business_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getBusiness());
}
if (department_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getDepartment());
}
if (cmdbMod1_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getCmdbMod1());
}
if (cmdbMod2_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getCmdbMod2());
}
if (cmdbMod3_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getCmdbMod3());
}
if (comment_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getComment());
}
if (owners_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getOwners());
}
if (token_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getToken());
}
if (ctime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getCtime());
}
if (mtime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getMtime());
}
if (revision_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, getRevision());
}
if (platformId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, getPlatformId());
}
if (totalInstanceCount_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(17, getTotalInstanceCount());
}
if (healthyInstanceCount_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, getHealthyInstanceCount());
}
for (int i = 0; i < userIds_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(19, userIds_.get(i));
}
for (int i = 0; i < groupIds_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, groupIds_.get(i));
}
if (id_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, getId());
}
for (int i = 0; i < removeUserIds_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(22, removeUserIds_.get(i));
}
for (int i = 0; i < removeGroupIds_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(23, removeGroupIds_.get(i));
}
if (editable_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(24, getEditable());
}
for (int i = 0; i < exportTo_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(25, exportTo_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service)) {
return super.equals(obj);
}
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service other = (com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasNamespace() != other.hasNamespace()) return false;
if (hasNamespace()) {
if (!getNamespace()
.equals(other.getNamespace())) return false;
}
if (!internalGetMetadata().equals(
other.internalGetMetadata())) return false;
if (hasPorts() != other.hasPorts()) return false;
if (hasPorts()) {
if (!getPorts()
.equals(other.getPorts())) return false;
}
if (hasBusiness() != other.hasBusiness()) return false;
if (hasBusiness()) {
if (!getBusiness()
.equals(other.getBusiness())) return false;
}
if (hasDepartment() != other.hasDepartment()) return false;
if (hasDepartment()) {
if (!getDepartment()
.equals(other.getDepartment())) return false;
}
if (hasCmdbMod1() != other.hasCmdbMod1()) return false;
if (hasCmdbMod1()) {
if (!getCmdbMod1()
.equals(other.getCmdbMod1())) return false;
}
if (hasCmdbMod2() != other.hasCmdbMod2()) return false;
if (hasCmdbMod2()) {
if (!getCmdbMod2()
.equals(other.getCmdbMod2())) return false;
}
if (hasCmdbMod3() != other.hasCmdbMod3()) return false;
if (hasCmdbMod3()) {
if (!getCmdbMod3()
.equals(other.getCmdbMod3())) return false;
}
if (hasComment() != other.hasComment()) return false;
if (hasComment()) {
if (!getComment()
.equals(other.getComment())) return false;
}
if (hasOwners() != other.hasOwners()) return false;
if (hasOwners()) {
if (!getOwners()
.equals(other.getOwners())) return false;
}
if (hasToken() != other.hasToken()) return false;
if (hasToken()) {
if (!getToken()
.equals(other.getToken())) return false;
}
if (hasCtime() != other.hasCtime()) return false;
if (hasCtime()) {
if (!getCtime()
.equals(other.getCtime())) return false;
}
if (hasMtime() != other.hasMtime()) return false;
if (hasMtime()) {
if (!getMtime()
.equals(other.getMtime())) return false;
}
if (hasRevision() != other.hasRevision()) return false;
if (hasRevision()) {
if (!getRevision()
.equals(other.getRevision())) return false;
}
if (hasPlatformId() != other.hasPlatformId()) return false;
if (hasPlatformId()) {
if (!getPlatformId()
.equals(other.getPlatformId())) return false;
}
if (hasTotalInstanceCount() != other.hasTotalInstanceCount()) return false;
if (hasTotalInstanceCount()) {
if (!getTotalInstanceCount()
.equals(other.getTotalInstanceCount())) return false;
}
if (hasHealthyInstanceCount() != other.hasHealthyInstanceCount()) return false;
if (hasHealthyInstanceCount()) {
if (!getHealthyInstanceCount()
.equals(other.getHealthyInstanceCount())) return false;
}
if (!getUserIdsList()
.equals(other.getUserIdsList())) return false;
if (!getGroupIdsList()
.equals(other.getGroupIdsList())) return false;
if (!getRemoveUserIdsList()
.equals(other.getRemoveUserIdsList())) return false;
if (!getRemoveGroupIdsList()
.equals(other.getRemoveGroupIdsList())) return false;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (!getId()
.equals(other.getId())) return false;
}
if (hasEditable() != other.hasEditable()) return false;
if (hasEditable()) {
if (!getEditable()
.equals(other.getEditable())) return false;
}
if (!getExportToList()
.equals(other.getExportToList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasNamespace()) {
hash = (37 * hash) + NAMESPACE_FIELD_NUMBER;
hash = (53 * hash) + getNamespace().hashCode();
}
if (!internalGetMetadata().getMap().isEmpty()) {
hash = (37 * hash) + METADATA_FIELD_NUMBER;
hash = (53 * hash) + internalGetMetadata().hashCode();
}
if (hasPorts()) {
hash = (37 * hash) + PORTS_FIELD_NUMBER;
hash = (53 * hash) + getPorts().hashCode();
}
if (hasBusiness()) {
hash = (37 * hash) + BUSINESS_FIELD_NUMBER;
hash = (53 * hash) + getBusiness().hashCode();
}
if (hasDepartment()) {
hash = (37 * hash) + DEPARTMENT_FIELD_NUMBER;
hash = (53 * hash) + getDepartment().hashCode();
}
if (hasCmdbMod1()) {
hash = (37 * hash) + CMDB_MOD1_FIELD_NUMBER;
hash = (53 * hash) + getCmdbMod1().hashCode();
}
if (hasCmdbMod2()) {
hash = (37 * hash) + CMDB_MOD2_FIELD_NUMBER;
hash = (53 * hash) + getCmdbMod2().hashCode();
}
if (hasCmdbMod3()) {
hash = (37 * hash) + CMDB_MOD3_FIELD_NUMBER;
hash = (53 * hash) + getCmdbMod3().hashCode();
}
if (hasComment()) {
hash = (37 * hash) + COMMENT_FIELD_NUMBER;
hash = (53 * hash) + getComment().hashCode();
}
if (hasOwners()) {
hash = (37 * hash) + OWNERS_FIELD_NUMBER;
hash = (53 * hash) + getOwners().hashCode();
}
if (hasToken()) {
hash = (37 * hash) + TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getToken().hashCode();
}
if (hasCtime()) {
hash = (37 * hash) + CTIME_FIELD_NUMBER;
hash = (53 * hash) + getCtime().hashCode();
}
if (hasMtime()) {
hash = (37 * hash) + MTIME_FIELD_NUMBER;
hash = (53 * hash) + getMtime().hashCode();
}
if (hasRevision()) {
hash = (37 * hash) + REVISION_FIELD_NUMBER;
hash = (53 * hash) + getRevision().hashCode();
}
if (hasPlatformId()) {
hash = (37 * hash) + PLATFORM_ID_FIELD_NUMBER;
hash = (53 * hash) + getPlatformId().hashCode();
}
if (hasTotalInstanceCount()) {
hash = (37 * hash) + TOTAL_INSTANCE_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getTotalInstanceCount().hashCode();
}
if (hasHealthyInstanceCount()) {
hash = (37 * hash) + HEALTHY_INSTANCE_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getHealthyInstanceCount().hashCode();
}
if (getUserIdsCount() > 0) {
hash = (37 * hash) + USER_IDS_FIELD_NUMBER;
hash = (53 * hash) + getUserIdsList().hashCode();
}
if (getGroupIdsCount() > 0) {
hash = (37 * hash) + GROUP_IDS_FIELD_NUMBER;
hash = (53 * hash) + getGroupIdsList().hashCode();
}
if (getRemoveUserIdsCount() > 0) {
hash = (37 * hash) + REMOVE_USER_IDS_FIELD_NUMBER;
hash = (53 * hash) + getRemoveUserIdsList().hashCode();
}
if (getRemoveGroupIdsCount() > 0) {
hash = (37 * hash) + REMOVE_GROUP_IDS_FIELD_NUMBER;
hash = (53 * hash) + getRemoveGroupIdsList().hashCode();
}
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasEditable()) {
hash = (37 * hash) + EDITABLE_FIELD_NUMBER;
hash = (53 * hash) + getEditable().hashCode();
}
if (getExportToCount() > 0) {
hash = (37 * hash) + EXPORT_TO_FIELD_NUMBER;
hash = (53 * hash) + getExportToList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code v1.Service}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:v1.Service)
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Service_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 3:
return internalGetMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 3:
return internalGetMutableMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Service_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service.class, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service.Builder.class);
}
// Construct using com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
if (nameBuilder_ == null) {
name_ = null;
} else {
name_ = null;
nameBuilder_ = null;
}
if (namespaceBuilder_ == null) {
namespace_ = null;
} else {
namespace_ = null;
namespaceBuilder_ = null;
}
internalGetMutableMetadata().clear();
if (portsBuilder_ == null) {
ports_ = null;
} else {
ports_ = null;
portsBuilder_ = null;
}
if (businessBuilder_ == null) {
business_ = null;
} else {
business_ = null;
businessBuilder_ = null;
}
if (departmentBuilder_ == null) {
department_ = null;
} else {
department_ = null;
departmentBuilder_ = null;
}
if (cmdbMod1Builder_ == null) {
cmdbMod1_ = null;
} else {
cmdbMod1_ = null;
cmdbMod1Builder_ = null;
}
if (cmdbMod2Builder_ == null) {
cmdbMod2_ = null;
} else {
cmdbMod2_ = null;
cmdbMod2Builder_ = null;
}
if (cmdbMod3Builder_ == null) {
cmdbMod3_ = null;
} else {
cmdbMod3_ = null;
cmdbMod3Builder_ = null;
}
if (commentBuilder_ == null) {
comment_ = null;
} else {
comment_ = null;
commentBuilder_ = null;
}
if (ownersBuilder_ == null) {
owners_ = null;
} else {
owners_ = null;
ownersBuilder_ = null;
}
if (tokenBuilder_ == null) {
token_ = null;
} else {
token_ = null;
tokenBuilder_ = null;
}
if (ctimeBuilder_ == null) {
ctime_ = null;
} else {
ctime_ = null;
ctimeBuilder_ = null;
}
if (mtimeBuilder_ == null) {
mtime_ = null;
} else {
mtime_ = null;
mtimeBuilder_ = null;
}
if (revisionBuilder_ == null) {
revision_ = null;
} else {
revision_ = null;
revisionBuilder_ = null;
}
if (platformIdBuilder_ == null) {
platformId_ = null;
} else {
platformId_ = null;
platformIdBuilder_ = null;
}
if (totalInstanceCountBuilder_ == null) {
totalInstanceCount_ = null;
} else {
totalInstanceCount_ = null;
totalInstanceCountBuilder_ = null;
}
if (healthyInstanceCountBuilder_ == null) {
healthyInstanceCount_ = null;
} else {
healthyInstanceCount_ = null;
healthyInstanceCountBuilder_ = null;
}
if (userIdsBuilder_ == null) {
userIds_ = java.util.Collections.emptyList();
} else {
userIds_ = null;
userIdsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (groupIdsBuilder_ == null) {
groupIds_ = java.util.Collections.emptyList();
} else {
groupIds_ = null;
groupIdsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (removeUserIdsBuilder_ == null) {
removeUserIds_ = java.util.Collections.emptyList();
} else {
removeUserIds_ = null;
removeUserIdsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (removeGroupIdsBuilder_ == null) {
removeGroupIds_ = java.util.Collections.emptyList();
} else {
removeGroupIds_ = null;
removeGroupIdsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (idBuilder_ == null) {
id_ = null;
} else {
id_ = null;
idBuilder_ = null;
}
if (editableBuilder_ == null) {
editable_ = null;
} else {
editable_ = null;
editableBuilder_ = null;
}
if (exportToBuilder_ == null) {
exportTo_ = java.util.Collections.emptyList();
} else {
exportTo_ = null;
exportToBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Service_descriptor;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service getDefaultInstanceForType() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service build() {
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service buildPartial() {
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service result = new com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service(this);
int from_bitField0_ = bitField0_;
if (nameBuilder_ == null) {
result.name_ = name_;
} else {
result.name_ = nameBuilder_.build();
}
if (namespaceBuilder_ == null) {
result.namespace_ = namespace_;
} else {
result.namespace_ = namespaceBuilder_.build();
}
result.metadata_ = internalGetMetadata();
result.metadata_.makeImmutable();
if (portsBuilder_ == null) {
result.ports_ = ports_;
} else {
result.ports_ = portsBuilder_.build();
}
if (businessBuilder_ == null) {
result.business_ = business_;
} else {
result.business_ = businessBuilder_.build();
}
if (departmentBuilder_ == null) {
result.department_ = department_;
} else {
result.department_ = departmentBuilder_.build();
}
if (cmdbMod1Builder_ == null) {
result.cmdbMod1_ = cmdbMod1_;
} else {
result.cmdbMod1_ = cmdbMod1Builder_.build();
}
if (cmdbMod2Builder_ == null) {
result.cmdbMod2_ = cmdbMod2_;
} else {
result.cmdbMod2_ = cmdbMod2Builder_.build();
}
if (cmdbMod3Builder_ == null) {
result.cmdbMod3_ = cmdbMod3_;
} else {
result.cmdbMod3_ = cmdbMod3Builder_.build();
}
if (commentBuilder_ == null) {
result.comment_ = comment_;
} else {
result.comment_ = commentBuilder_.build();
}
if (ownersBuilder_ == null) {
result.owners_ = owners_;
} else {
result.owners_ = ownersBuilder_.build();
}
if (tokenBuilder_ == null) {
result.token_ = token_;
} else {
result.token_ = tokenBuilder_.build();
}
if (ctimeBuilder_ == null) {
result.ctime_ = ctime_;
} else {
result.ctime_ = ctimeBuilder_.build();
}
if (mtimeBuilder_ == null) {
result.mtime_ = mtime_;
} else {
result.mtime_ = mtimeBuilder_.build();
}
if (revisionBuilder_ == null) {
result.revision_ = revision_;
} else {
result.revision_ = revisionBuilder_.build();
}
if (platformIdBuilder_ == null) {
result.platformId_ = platformId_;
} else {
result.platformId_ = platformIdBuilder_.build();
}
if (totalInstanceCountBuilder_ == null) {
result.totalInstanceCount_ = totalInstanceCount_;
} else {
result.totalInstanceCount_ = totalInstanceCountBuilder_.build();
}
if (healthyInstanceCountBuilder_ == null) {
result.healthyInstanceCount_ = healthyInstanceCount_;
} else {
result.healthyInstanceCount_ = healthyInstanceCountBuilder_.build();
}
if (userIdsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
userIds_ = java.util.Collections.unmodifiableList(userIds_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.userIds_ = userIds_;
} else {
result.userIds_ = userIdsBuilder_.build();
}
if (groupIdsBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
groupIds_ = java.util.Collections.unmodifiableList(groupIds_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.groupIds_ = groupIds_;
} else {
result.groupIds_ = groupIdsBuilder_.build();
}
if (removeUserIdsBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
removeUserIds_ = java.util.Collections.unmodifiableList(removeUserIds_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.removeUserIds_ = removeUserIds_;
} else {
result.removeUserIds_ = removeUserIdsBuilder_.build();
}
if (removeGroupIdsBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
removeGroupIds_ = java.util.Collections.unmodifiableList(removeGroupIds_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.removeGroupIds_ = removeGroupIds_;
} else {
result.removeGroupIds_ = removeGroupIdsBuilder_.build();
}
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (editableBuilder_ == null) {
result.editable_ = editable_;
} else {
result.editable_ = editableBuilder_.build();
}
if (exportToBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
exportTo_ = java.util.Collections.unmodifiableList(exportTo_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.exportTo_ = exportTo_;
} else {
result.exportTo_ = exportToBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service) {
return mergeFrom((com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service other) {
if (other == com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service.getDefaultInstance()) return this;
if (other.hasName()) {
mergeName(other.getName());
}
if (other.hasNamespace()) {
mergeNamespace(other.getNamespace());
}
internalGetMutableMetadata().mergeFrom(
other.internalGetMetadata());
if (other.hasPorts()) {
mergePorts(other.getPorts());
}
if (other.hasBusiness()) {
mergeBusiness(other.getBusiness());
}
if (other.hasDepartment()) {
mergeDepartment(other.getDepartment());
}
if (other.hasCmdbMod1()) {
mergeCmdbMod1(other.getCmdbMod1());
}
if (other.hasCmdbMod2()) {
mergeCmdbMod2(other.getCmdbMod2());
}
if (other.hasCmdbMod3()) {
mergeCmdbMod3(other.getCmdbMod3());
}
if (other.hasComment()) {
mergeComment(other.getComment());
}
if (other.hasOwners()) {
mergeOwners(other.getOwners());
}
if (other.hasToken()) {
mergeToken(other.getToken());
}
if (other.hasCtime()) {
mergeCtime(other.getCtime());
}
if (other.hasMtime()) {
mergeMtime(other.getMtime());
}
if (other.hasRevision()) {
mergeRevision(other.getRevision());
}
if (other.hasPlatformId()) {
mergePlatformId(other.getPlatformId());
}
if (other.hasTotalInstanceCount()) {
mergeTotalInstanceCount(other.getTotalInstanceCount());
}
if (other.hasHealthyInstanceCount()) {
mergeHealthyInstanceCount(other.getHealthyInstanceCount());
}
if (userIdsBuilder_ == null) {
if (!other.userIds_.isEmpty()) {
if (userIds_.isEmpty()) {
userIds_ = other.userIds_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureUserIdsIsMutable();
userIds_.addAll(other.userIds_);
}
onChanged();
}
} else {
if (!other.userIds_.isEmpty()) {
if (userIdsBuilder_.isEmpty()) {
userIdsBuilder_.dispose();
userIdsBuilder_ = null;
userIds_ = other.userIds_;
bitField0_ = (bitField0_ & ~0x00000002);
userIdsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getUserIdsFieldBuilder() : null;
} else {
userIdsBuilder_.addAllMessages(other.userIds_);
}
}
}
if (groupIdsBuilder_ == null) {
if (!other.groupIds_.isEmpty()) {
if (groupIds_.isEmpty()) {
groupIds_ = other.groupIds_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureGroupIdsIsMutable();
groupIds_.addAll(other.groupIds_);
}
onChanged();
}
} else {
if (!other.groupIds_.isEmpty()) {
if (groupIdsBuilder_.isEmpty()) {
groupIdsBuilder_.dispose();
groupIdsBuilder_ = null;
groupIds_ = other.groupIds_;
bitField0_ = (bitField0_ & ~0x00000004);
groupIdsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getGroupIdsFieldBuilder() : null;
} else {
groupIdsBuilder_.addAllMessages(other.groupIds_);
}
}
}
if (removeUserIdsBuilder_ == null) {
if (!other.removeUserIds_.isEmpty()) {
if (removeUserIds_.isEmpty()) {
removeUserIds_ = other.removeUserIds_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureRemoveUserIdsIsMutable();
removeUserIds_.addAll(other.removeUserIds_);
}
onChanged();
}
} else {
if (!other.removeUserIds_.isEmpty()) {
if (removeUserIdsBuilder_.isEmpty()) {
removeUserIdsBuilder_.dispose();
removeUserIdsBuilder_ = null;
removeUserIds_ = other.removeUserIds_;
bitField0_ = (bitField0_ & ~0x00000008);
removeUserIdsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRemoveUserIdsFieldBuilder() : null;
} else {
removeUserIdsBuilder_.addAllMessages(other.removeUserIds_);
}
}
}
if (removeGroupIdsBuilder_ == null) {
if (!other.removeGroupIds_.isEmpty()) {
if (removeGroupIds_.isEmpty()) {
removeGroupIds_ = other.removeGroupIds_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureRemoveGroupIdsIsMutable();
removeGroupIds_.addAll(other.removeGroupIds_);
}
onChanged();
}
} else {
if (!other.removeGroupIds_.isEmpty()) {
if (removeGroupIdsBuilder_.isEmpty()) {
removeGroupIdsBuilder_.dispose();
removeGroupIdsBuilder_ = null;
removeGroupIds_ = other.removeGroupIds_;
bitField0_ = (bitField0_ & ~0x00000010);
removeGroupIdsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRemoveGroupIdsFieldBuilder() : null;
} else {
removeGroupIdsBuilder_.addAllMessages(other.removeGroupIds_);
}
}
}
if (other.hasId()) {
mergeId(other.getId());
}
if (other.hasEditable()) {
mergeEditable(other.getEditable());
}
if (exportToBuilder_ == null) {
if (!other.exportTo_.isEmpty()) {
if (exportTo_.isEmpty()) {
exportTo_ = other.exportTo_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureExportToIsMutable();
exportTo_.addAll(other.exportTo_);
}
onChanged();
}
} else {
if (!other.exportTo_.isEmpty()) {
if (exportToBuilder_.isEmpty()) {
exportToBuilder_.dispose();
exportToBuilder_ = null;
exportTo_ = other.exportTo_;
bitField0_ = (bitField0_ & ~0x00000020);
exportToBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getExportToFieldBuilder() : null;
} else {
exportToBuilder_.addAllMessages(other.exportTo_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getNameFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 10
case 18: {
input.readMessage(
getNamespaceFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 18
case 26: {
com.google.protobuf.MapEntry
metadata__ = input.readMessage(
MetadataDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableMetadata().getMutableMap().put(
metadata__.getKey(), metadata__.getValue());
break;
} // case 26
case 34: {
input.readMessage(
getPortsFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 34
case 42: {
input.readMessage(
getBusinessFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 42
case 50: {
input.readMessage(
getDepartmentFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 50
case 58: {
input.readMessage(
getCmdbMod1FieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 58
case 66: {
input.readMessage(
getCmdbMod2FieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 66
case 74: {
input.readMessage(
getCmdbMod3FieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 74
case 82: {
input.readMessage(
getCommentFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 82
case 90: {
input.readMessage(
getOwnersFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 90
case 98: {
input.readMessage(
getTokenFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 98
case 106: {
input.readMessage(
getCtimeFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 106
case 114: {
input.readMessage(
getMtimeFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 114
case 122: {
input.readMessage(
getRevisionFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 122
case 130: {
input.readMessage(
getPlatformIdFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 130
case 138: {
input.readMessage(
getTotalInstanceCountFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 138
case 146: {
input.readMessage(
getHealthyInstanceCountFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 146
case 154: {
com.google.protobuf.StringValue m =
input.readMessage(
com.google.protobuf.StringValue.parser(),
extensionRegistry);
if (userIdsBuilder_ == null) {
ensureUserIdsIsMutable();
userIds_.add(m);
} else {
userIdsBuilder_.addMessage(m);
}
break;
} // case 154
case 162: {
com.google.protobuf.StringValue m =
input.readMessage(
com.google.protobuf.StringValue.parser(),
extensionRegistry);
if (groupIdsBuilder_ == null) {
ensureGroupIdsIsMutable();
groupIds_.add(m);
} else {
groupIdsBuilder_.addMessage(m);
}
break;
} // case 162
case 170: {
input.readMessage(
getIdFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 170
case 178: {
com.google.protobuf.StringValue m =
input.readMessage(
com.google.protobuf.StringValue.parser(),
extensionRegistry);
if (removeUserIdsBuilder_ == null) {
ensureRemoveUserIdsIsMutable();
removeUserIds_.add(m);
} else {
removeUserIdsBuilder_.addMessage(m);
}
break;
} // case 178
case 186: {
com.google.protobuf.StringValue m =
input.readMessage(
com.google.protobuf.StringValue.parser(),
extensionRegistry);
if (removeGroupIdsBuilder_ == null) {
ensureRemoveGroupIdsIsMutable();
removeGroupIds_.add(m);
} else {
removeGroupIdsBuilder_.addMessage(m);
}
break;
} // case 186
case 194: {
input.readMessage(
getEditableFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 194
case 202: {
com.google.protobuf.StringValue m =
input.readMessage(
com.google.protobuf.StringValue.parser(),
extensionRegistry);
if (exportToBuilder_ == null) {
ensureExportToIsMutable();
exportTo_.add(m);
} else {
exportToBuilder_.addMessage(m);
}
break;
} // case 202
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.StringValue name_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> nameBuilder_;
/**
* .google.protobuf.StringValue name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return nameBuilder_ != null || name_ != null;
}
/**
* .google.protobuf.StringValue name = 1;
* @return The name.
*/
public com.google.protobuf.StringValue getName() {
if (nameBuilder_ == null) {
return name_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : name_;
} else {
return nameBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue name = 1;
*/
public Builder setName(com.google.protobuf.StringValue value) {
if (nameBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
} else {
nameBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue name = 1;
*/
public Builder setName(
com.google.protobuf.StringValue.Builder builderForValue) {
if (nameBuilder_ == null) {
name_ = builderForValue.build();
onChanged();
} else {
nameBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue name = 1;
*/
public Builder mergeName(com.google.protobuf.StringValue value) {
if (nameBuilder_ == null) {
if (name_ != null) {
name_ =
com.google.protobuf.StringValue.newBuilder(name_).mergeFrom(value).buildPartial();
} else {
name_ = value;
}
onChanged();
} else {
nameBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue name = 1;
*/
public Builder clearName() {
if (nameBuilder_ == null) {
name_ = null;
onChanged();
} else {
name_ = null;
nameBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue name = 1;
*/
public com.google.protobuf.StringValue.Builder getNameBuilder() {
onChanged();
return getNameFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue name = 1;
*/
public com.google.protobuf.StringValueOrBuilder getNameOrBuilder() {
if (nameBuilder_ != null) {
return nameBuilder_.getMessageOrBuilder();
} else {
return name_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : name_;
}
}
/**
* .google.protobuf.StringValue name = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getNameFieldBuilder() {
if (nameBuilder_ == null) {
nameBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getName(),
getParentForChildren(),
isClean());
name_ = null;
}
return nameBuilder_;
}
private com.google.protobuf.StringValue namespace_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> namespaceBuilder_;
/**
* .google.protobuf.StringValue namespace = 2;
* @return Whether the namespace field is set.
*/
public boolean hasNamespace() {
return namespaceBuilder_ != null || namespace_ != null;
}
/**
* .google.protobuf.StringValue namespace = 2;
* @return The namespace.
*/
public com.google.protobuf.StringValue getNamespace() {
if (namespaceBuilder_ == null) {
return namespace_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : namespace_;
} else {
return namespaceBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public Builder setNamespace(com.google.protobuf.StringValue value) {
if (namespaceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
namespace_ = value;
onChanged();
} else {
namespaceBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public Builder setNamespace(
com.google.protobuf.StringValue.Builder builderForValue) {
if (namespaceBuilder_ == null) {
namespace_ = builderForValue.build();
onChanged();
} else {
namespaceBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public Builder mergeNamespace(com.google.protobuf.StringValue value) {
if (namespaceBuilder_ == null) {
if (namespace_ != null) {
namespace_ =
com.google.protobuf.StringValue.newBuilder(namespace_).mergeFrom(value).buildPartial();
} else {
namespace_ = value;
}
onChanged();
} else {
namespaceBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public Builder clearNamespace() {
if (namespaceBuilder_ == null) {
namespace_ = null;
onChanged();
} else {
namespace_ = null;
namespaceBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public com.google.protobuf.StringValue.Builder getNamespaceBuilder() {
onChanged();
return getNamespaceFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public com.google.protobuf.StringValueOrBuilder getNamespaceOrBuilder() {
if (namespaceBuilder_ != null) {
return namespaceBuilder_.getMessageOrBuilder();
} else {
return namespace_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : namespace_;
}
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getNamespaceFieldBuilder() {
if (namespaceBuilder_ == null) {
namespaceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getNamespace(),
getParentForChildren(),
isClean());
namespace_ = null;
}
return namespaceBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> metadata_;
private com.google.protobuf.MapField
internalGetMetadata() {
if (metadata_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
return metadata_;
}
private com.google.protobuf.MapField
internalGetMutableMetadata() {
onChanged();;
if (metadata_ == null) {
metadata_ = com.google.protobuf.MapField.newMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
if (!metadata_.isMutable()) {
metadata_ = metadata_.copy();
}
return metadata_;
}
public int getMetadataCount() {
return internalGetMetadata().getMap().size();
}
/**
* map<string, string> metadata = 3;
*/
@java.lang.Override
public boolean containsMetadata(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetMetadata().getMap().containsKey(key);
}
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getMetadata() {
return getMetadataMap();
}
/**
* map<string, string> metadata = 3;
*/
@java.lang.Override
public java.util.Map getMetadataMap() {
return internalGetMetadata().getMap();
}
/**
* map<string, string> metadata = 3;
*/
@java.lang.Override
public java.lang.String getMetadataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetMetadata().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> metadata = 3;
*/
@java.lang.Override
public java.lang.String getMetadataOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetMetadata().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearMetadata() {
internalGetMutableMetadata().getMutableMap()
.clear();
return this;
}
/**
* map<string, string> metadata = 3;
*/
public Builder removeMetadata(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableMetadata().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableMetadata() {
return internalGetMutableMetadata().getMutableMap();
}
/**
* map<string, string> metadata = 3;
*/
public Builder putMetadata(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableMetadata().getMutableMap()
.put(key, value);
return this;
}
/**
* map<string, string> metadata = 3;
*/
public Builder putAllMetadata(
java.util.Map values) {
internalGetMutableMetadata().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.StringValue ports_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> portsBuilder_;
/**
* .google.protobuf.StringValue ports = 4;
* @return Whether the ports field is set.
*/
public boolean hasPorts() {
return portsBuilder_ != null || ports_ != null;
}
/**
* .google.protobuf.StringValue ports = 4;
* @return The ports.
*/
public com.google.protobuf.StringValue getPorts() {
if (portsBuilder_ == null) {
return ports_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : ports_;
} else {
return portsBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue ports = 4;
*/
public Builder setPorts(com.google.protobuf.StringValue value) {
if (portsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ports_ = value;
onChanged();
} else {
portsBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue ports = 4;
*/
public Builder setPorts(
com.google.protobuf.StringValue.Builder builderForValue) {
if (portsBuilder_ == null) {
ports_ = builderForValue.build();
onChanged();
} else {
portsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue ports = 4;
*/
public Builder mergePorts(com.google.protobuf.StringValue value) {
if (portsBuilder_ == null) {
if (ports_ != null) {
ports_ =
com.google.protobuf.StringValue.newBuilder(ports_).mergeFrom(value).buildPartial();
} else {
ports_ = value;
}
onChanged();
} else {
portsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue ports = 4;
*/
public Builder clearPorts() {
if (portsBuilder_ == null) {
ports_ = null;
onChanged();
} else {
ports_ = null;
portsBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue ports = 4;
*/
public com.google.protobuf.StringValue.Builder getPortsBuilder() {
onChanged();
return getPortsFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue ports = 4;
*/
public com.google.protobuf.StringValueOrBuilder getPortsOrBuilder() {
if (portsBuilder_ != null) {
return portsBuilder_.getMessageOrBuilder();
} else {
return ports_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : ports_;
}
}
/**
* .google.protobuf.StringValue ports = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getPortsFieldBuilder() {
if (portsBuilder_ == null) {
portsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getPorts(),
getParentForChildren(),
isClean());
ports_ = null;
}
return portsBuilder_;
}
private com.google.protobuf.StringValue business_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> businessBuilder_;
/**
* .google.protobuf.StringValue business = 5;
* @return Whether the business field is set.
*/
public boolean hasBusiness() {
return businessBuilder_ != null || business_ != null;
}
/**
* .google.protobuf.StringValue business = 5;
* @return The business.
*/
public com.google.protobuf.StringValue getBusiness() {
if (businessBuilder_ == null) {
return business_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : business_;
} else {
return businessBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue business = 5;
*/
public Builder setBusiness(com.google.protobuf.StringValue value) {
if (businessBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
business_ = value;
onChanged();
} else {
businessBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue business = 5;
*/
public Builder setBusiness(
com.google.protobuf.StringValue.Builder builderForValue) {
if (businessBuilder_ == null) {
business_ = builderForValue.build();
onChanged();
} else {
businessBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue business = 5;
*/
public Builder mergeBusiness(com.google.protobuf.StringValue value) {
if (businessBuilder_ == null) {
if (business_ != null) {
business_ =
com.google.protobuf.StringValue.newBuilder(business_).mergeFrom(value).buildPartial();
} else {
business_ = value;
}
onChanged();
} else {
businessBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue business = 5;
*/
public Builder clearBusiness() {
if (businessBuilder_ == null) {
business_ = null;
onChanged();
} else {
business_ = null;
businessBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue business = 5;
*/
public com.google.protobuf.StringValue.Builder getBusinessBuilder() {
onChanged();
return getBusinessFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue business = 5;
*/
public com.google.protobuf.StringValueOrBuilder getBusinessOrBuilder() {
if (businessBuilder_ != null) {
return businessBuilder_.getMessageOrBuilder();
} else {
return business_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : business_;
}
}
/**
* .google.protobuf.StringValue business = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getBusinessFieldBuilder() {
if (businessBuilder_ == null) {
businessBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getBusiness(),
getParentForChildren(),
isClean());
business_ = null;
}
return businessBuilder_;
}
private com.google.protobuf.StringValue department_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> departmentBuilder_;
/**
* .google.protobuf.StringValue department = 6;
* @return Whether the department field is set.
*/
public boolean hasDepartment() {
return departmentBuilder_ != null || department_ != null;
}
/**
* .google.protobuf.StringValue department = 6;
* @return The department.
*/
public com.google.protobuf.StringValue getDepartment() {
if (departmentBuilder_ == null) {
return department_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : department_;
} else {
return departmentBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue department = 6;
*/
public Builder setDepartment(com.google.protobuf.StringValue value) {
if (departmentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
department_ = value;
onChanged();
} else {
departmentBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue department = 6;
*/
public Builder setDepartment(
com.google.protobuf.StringValue.Builder builderForValue) {
if (departmentBuilder_ == null) {
department_ = builderForValue.build();
onChanged();
} else {
departmentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue department = 6;
*/
public Builder mergeDepartment(com.google.protobuf.StringValue value) {
if (departmentBuilder_ == null) {
if (department_ != null) {
department_ =
com.google.protobuf.StringValue.newBuilder(department_).mergeFrom(value).buildPartial();
} else {
department_ = value;
}
onChanged();
} else {
departmentBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue department = 6;
*/
public Builder clearDepartment() {
if (departmentBuilder_ == null) {
department_ = null;
onChanged();
} else {
department_ = null;
departmentBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue department = 6;
*/
public com.google.protobuf.StringValue.Builder getDepartmentBuilder() {
onChanged();
return getDepartmentFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue department = 6;
*/
public com.google.protobuf.StringValueOrBuilder getDepartmentOrBuilder() {
if (departmentBuilder_ != null) {
return departmentBuilder_.getMessageOrBuilder();
} else {
return department_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : department_;
}
}
/**
* .google.protobuf.StringValue department = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getDepartmentFieldBuilder() {
if (departmentBuilder_ == null) {
departmentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getDepartment(),
getParentForChildren(),
isClean());
department_ = null;
}
return departmentBuilder_;
}
private com.google.protobuf.StringValue cmdbMod1_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> cmdbMod1Builder_;
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
* @return Whether the cmdbMod1 field is set.
*/
public boolean hasCmdbMod1() {
return cmdbMod1Builder_ != null || cmdbMod1_ != null;
}
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
* @return The cmdbMod1.
*/
public com.google.protobuf.StringValue getCmdbMod1() {
if (cmdbMod1Builder_ == null) {
return cmdbMod1_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : cmdbMod1_;
} else {
return cmdbMod1Builder_.getMessage();
}
}
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
*/
public Builder setCmdbMod1(com.google.protobuf.StringValue value) {
if (cmdbMod1Builder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cmdbMod1_ = value;
onChanged();
} else {
cmdbMod1Builder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
*/
public Builder setCmdbMod1(
com.google.protobuf.StringValue.Builder builderForValue) {
if (cmdbMod1Builder_ == null) {
cmdbMod1_ = builderForValue.build();
onChanged();
} else {
cmdbMod1Builder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
*/
public Builder mergeCmdbMod1(com.google.protobuf.StringValue value) {
if (cmdbMod1Builder_ == null) {
if (cmdbMod1_ != null) {
cmdbMod1_ =
com.google.protobuf.StringValue.newBuilder(cmdbMod1_).mergeFrom(value).buildPartial();
} else {
cmdbMod1_ = value;
}
onChanged();
} else {
cmdbMod1Builder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
*/
public Builder clearCmdbMod1() {
if (cmdbMod1Builder_ == null) {
cmdbMod1_ = null;
onChanged();
} else {
cmdbMod1_ = null;
cmdbMod1Builder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
*/
public com.google.protobuf.StringValue.Builder getCmdbMod1Builder() {
onChanged();
return getCmdbMod1FieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
*/
public com.google.protobuf.StringValueOrBuilder getCmdbMod1OrBuilder() {
if (cmdbMod1Builder_ != null) {
return cmdbMod1Builder_.getMessageOrBuilder();
} else {
return cmdbMod1_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : cmdbMod1_;
}
}
/**
* .google.protobuf.StringValue cmdb_mod1 = 7 [json_name = "cmdb_mod1"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getCmdbMod1FieldBuilder() {
if (cmdbMod1Builder_ == null) {
cmdbMod1Builder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getCmdbMod1(),
getParentForChildren(),
isClean());
cmdbMod1_ = null;
}
return cmdbMod1Builder_;
}
private com.google.protobuf.StringValue cmdbMod2_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> cmdbMod2Builder_;
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
* @return Whether the cmdbMod2 field is set.
*/
public boolean hasCmdbMod2() {
return cmdbMod2Builder_ != null || cmdbMod2_ != null;
}
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
* @return The cmdbMod2.
*/
public com.google.protobuf.StringValue getCmdbMod2() {
if (cmdbMod2Builder_ == null) {
return cmdbMod2_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : cmdbMod2_;
} else {
return cmdbMod2Builder_.getMessage();
}
}
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
*/
public Builder setCmdbMod2(com.google.protobuf.StringValue value) {
if (cmdbMod2Builder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cmdbMod2_ = value;
onChanged();
} else {
cmdbMod2Builder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
*/
public Builder setCmdbMod2(
com.google.protobuf.StringValue.Builder builderForValue) {
if (cmdbMod2Builder_ == null) {
cmdbMod2_ = builderForValue.build();
onChanged();
} else {
cmdbMod2Builder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
*/
public Builder mergeCmdbMod2(com.google.protobuf.StringValue value) {
if (cmdbMod2Builder_ == null) {
if (cmdbMod2_ != null) {
cmdbMod2_ =
com.google.protobuf.StringValue.newBuilder(cmdbMod2_).mergeFrom(value).buildPartial();
} else {
cmdbMod2_ = value;
}
onChanged();
} else {
cmdbMod2Builder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
*/
public Builder clearCmdbMod2() {
if (cmdbMod2Builder_ == null) {
cmdbMod2_ = null;
onChanged();
} else {
cmdbMod2_ = null;
cmdbMod2Builder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
*/
public com.google.protobuf.StringValue.Builder getCmdbMod2Builder() {
onChanged();
return getCmdbMod2FieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
*/
public com.google.protobuf.StringValueOrBuilder getCmdbMod2OrBuilder() {
if (cmdbMod2Builder_ != null) {
return cmdbMod2Builder_.getMessageOrBuilder();
} else {
return cmdbMod2_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : cmdbMod2_;
}
}
/**
* .google.protobuf.StringValue cmdb_mod2 = 8 [json_name = "cmdb_mod2"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getCmdbMod2FieldBuilder() {
if (cmdbMod2Builder_ == null) {
cmdbMod2Builder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getCmdbMod2(),
getParentForChildren(),
isClean());
cmdbMod2_ = null;
}
return cmdbMod2Builder_;
}
private com.google.protobuf.StringValue cmdbMod3_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> cmdbMod3Builder_;
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
* @return Whether the cmdbMod3 field is set.
*/
public boolean hasCmdbMod3() {
return cmdbMod3Builder_ != null || cmdbMod3_ != null;
}
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
* @return The cmdbMod3.
*/
public com.google.protobuf.StringValue getCmdbMod3() {
if (cmdbMod3Builder_ == null) {
return cmdbMod3_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : cmdbMod3_;
} else {
return cmdbMod3Builder_.getMessage();
}
}
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
*/
public Builder setCmdbMod3(com.google.protobuf.StringValue value) {
if (cmdbMod3Builder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cmdbMod3_ = value;
onChanged();
} else {
cmdbMod3Builder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
*/
public Builder setCmdbMod3(
com.google.protobuf.StringValue.Builder builderForValue) {
if (cmdbMod3Builder_ == null) {
cmdbMod3_ = builderForValue.build();
onChanged();
} else {
cmdbMod3Builder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
*/
public Builder mergeCmdbMod3(com.google.protobuf.StringValue value) {
if (cmdbMod3Builder_ == null) {
if (cmdbMod3_ != null) {
cmdbMod3_ =
com.google.protobuf.StringValue.newBuilder(cmdbMod3_).mergeFrom(value).buildPartial();
} else {
cmdbMod3_ = value;
}
onChanged();
} else {
cmdbMod3Builder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
*/
public Builder clearCmdbMod3() {
if (cmdbMod3Builder_ == null) {
cmdbMod3_ = null;
onChanged();
} else {
cmdbMod3_ = null;
cmdbMod3Builder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
*/
public com.google.protobuf.StringValue.Builder getCmdbMod3Builder() {
onChanged();
return getCmdbMod3FieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
*/
public com.google.protobuf.StringValueOrBuilder getCmdbMod3OrBuilder() {
if (cmdbMod3Builder_ != null) {
return cmdbMod3Builder_.getMessageOrBuilder();
} else {
return cmdbMod3_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : cmdbMod3_;
}
}
/**
* .google.protobuf.StringValue cmdb_mod3 = 9 [json_name = "cmdb_mod3"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getCmdbMod3FieldBuilder() {
if (cmdbMod3Builder_ == null) {
cmdbMod3Builder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getCmdbMod3(),
getParentForChildren(),
isClean());
cmdbMod3_ = null;
}
return cmdbMod3Builder_;
}
private com.google.protobuf.StringValue comment_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> commentBuilder_;
/**
* .google.protobuf.StringValue comment = 10;
* @return Whether the comment field is set.
*/
public boolean hasComment() {
return commentBuilder_ != null || comment_ != null;
}
/**
* .google.protobuf.StringValue comment = 10;
* @return The comment.
*/
public com.google.protobuf.StringValue getComment() {
if (commentBuilder_ == null) {
return comment_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : comment_;
} else {
return commentBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue comment = 10;
*/
public Builder setComment(com.google.protobuf.StringValue value) {
if (commentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
comment_ = value;
onChanged();
} else {
commentBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue comment = 10;
*/
public Builder setComment(
com.google.protobuf.StringValue.Builder builderForValue) {
if (commentBuilder_ == null) {
comment_ = builderForValue.build();
onChanged();
} else {
commentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue comment = 10;
*/
public Builder mergeComment(com.google.protobuf.StringValue value) {
if (commentBuilder_ == null) {
if (comment_ != null) {
comment_ =
com.google.protobuf.StringValue.newBuilder(comment_).mergeFrom(value).buildPartial();
} else {
comment_ = value;
}
onChanged();
} else {
commentBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue comment = 10;
*/
public Builder clearComment() {
if (commentBuilder_ == null) {
comment_ = null;
onChanged();
} else {
comment_ = null;
commentBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue comment = 10;
*/
public com.google.protobuf.StringValue.Builder getCommentBuilder() {
onChanged();
return getCommentFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue comment = 10;
*/
public com.google.protobuf.StringValueOrBuilder getCommentOrBuilder() {
if (commentBuilder_ != null) {
return commentBuilder_.getMessageOrBuilder();
} else {
return comment_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : comment_;
}
}
/**
* .google.protobuf.StringValue comment = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getCommentFieldBuilder() {
if (commentBuilder_ == null) {
commentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getComment(),
getParentForChildren(),
isClean());
comment_ = null;
}
return commentBuilder_;
}
private com.google.protobuf.StringValue owners_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> ownersBuilder_;
/**
* .google.protobuf.StringValue owners = 11;
* @return Whether the owners field is set.
*/
public boolean hasOwners() {
return ownersBuilder_ != null || owners_ != null;
}
/**
* .google.protobuf.StringValue owners = 11;
* @return The owners.
*/
public com.google.protobuf.StringValue getOwners() {
if (ownersBuilder_ == null) {
return owners_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : owners_;
} else {
return ownersBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue owners = 11;
*/
public Builder setOwners(com.google.protobuf.StringValue value) {
if (ownersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
owners_ = value;
onChanged();
} else {
ownersBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue owners = 11;
*/
public Builder setOwners(
com.google.protobuf.StringValue.Builder builderForValue) {
if (ownersBuilder_ == null) {
owners_ = builderForValue.build();
onChanged();
} else {
ownersBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue owners = 11;
*/
public Builder mergeOwners(com.google.protobuf.StringValue value) {
if (ownersBuilder_ == null) {
if (owners_ != null) {
owners_ =
com.google.protobuf.StringValue.newBuilder(owners_).mergeFrom(value).buildPartial();
} else {
owners_ = value;
}
onChanged();
} else {
ownersBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue owners = 11;
*/
public Builder clearOwners() {
if (ownersBuilder_ == null) {
owners_ = null;
onChanged();
} else {
owners_ = null;
ownersBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue owners = 11;
*/
public com.google.protobuf.StringValue.Builder getOwnersBuilder() {
onChanged();
return getOwnersFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue owners = 11;
*/
public com.google.protobuf.StringValueOrBuilder getOwnersOrBuilder() {
if (ownersBuilder_ != null) {
return ownersBuilder_.getMessageOrBuilder();
} else {
return owners_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : owners_;
}
}
/**
* .google.protobuf.StringValue owners = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getOwnersFieldBuilder() {
if (ownersBuilder_ == null) {
ownersBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getOwners(),
getParentForChildren(),
isClean());
owners_ = null;
}
return ownersBuilder_;
}
private com.google.protobuf.StringValue token_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> tokenBuilder_;
/**
* .google.protobuf.StringValue token = 12;
* @return Whether the token field is set.
*/
public boolean hasToken() {
return tokenBuilder_ != null || token_ != null;
}
/**
* .google.protobuf.StringValue token = 12;
* @return The token.
*/
public com.google.protobuf.StringValue getToken() {
if (tokenBuilder_ == null) {
return token_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : token_;
} else {
return tokenBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue token = 12;
*/
public Builder setToken(com.google.protobuf.StringValue value) {
if (tokenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
token_ = value;
onChanged();
} else {
tokenBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue token = 12;
*/
public Builder setToken(
com.google.protobuf.StringValue.Builder builderForValue) {
if (tokenBuilder_ == null) {
token_ = builderForValue.build();
onChanged();
} else {
tokenBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue token = 12;
*/
public Builder mergeToken(com.google.protobuf.StringValue value) {
if (tokenBuilder_ == null) {
if (token_ != null) {
token_ =
com.google.protobuf.StringValue.newBuilder(token_).mergeFrom(value).buildPartial();
} else {
token_ = value;
}
onChanged();
} else {
tokenBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue token = 12;
*/
public Builder clearToken() {
if (tokenBuilder_ == null) {
token_ = null;
onChanged();
} else {
token_ = null;
tokenBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue token = 12;
*/
public com.google.protobuf.StringValue.Builder getTokenBuilder() {
onChanged();
return getTokenFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue token = 12;
*/
public com.google.protobuf.StringValueOrBuilder getTokenOrBuilder() {
if (tokenBuilder_ != null) {
return tokenBuilder_.getMessageOrBuilder();
} else {
return token_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : token_;
}
}
/**
* .google.protobuf.StringValue token = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getTokenFieldBuilder() {
if (tokenBuilder_ == null) {
tokenBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getToken(),
getParentForChildren(),
isClean());
token_ = null;
}
return tokenBuilder_;
}
private com.google.protobuf.StringValue ctime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> ctimeBuilder_;
/**
* .google.protobuf.StringValue ctime = 13;
* @return Whether the ctime field is set.
*/
public boolean hasCtime() {
return ctimeBuilder_ != null || ctime_ != null;
}
/**
* .google.protobuf.StringValue ctime = 13;
* @return The ctime.
*/
public com.google.protobuf.StringValue getCtime() {
if (ctimeBuilder_ == null) {
return ctime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : ctime_;
} else {
return ctimeBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue ctime = 13;
*/
public Builder setCtime(com.google.protobuf.StringValue value) {
if (ctimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ctime_ = value;
onChanged();
} else {
ctimeBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 13;
*/
public Builder setCtime(
com.google.protobuf.StringValue.Builder builderForValue) {
if (ctimeBuilder_ == null) {
ctime_ = builderForValue.build();
onChanged();
} else {
ctimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 13;
*/
public Builder mergeCtime(com.google.protobuf.StringValue value) {
if (ctimeBuilder_ == null) {
if (ctime_ != null) {
ctime_ =
com.google.protobuf.StringValue.newBuilder(ctime_).mergeFrom(value).buildPartial();
} else {
ctime_ = value;
}
onChanged();
} else {
ctimeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 13;
*/
public Builder clearCtime() {
if (ctimeBuilder_ == null) {
ctime_ = null;
onChanged();
} else {
ctime_ = null;
ctimeBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 13;
*/
public com.google.protobuf.StringValue.Builder getCtimeBuilder() {
onChanged();
return getCtimeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue ctime = 13;
*/
public com.google.protobuf.StringValueOrBuilder getCtimeOrBuilder() {
if (ctimeBuilder_ != null) {
return ctimeBuilder_.getMessageOrBuilder();
} else {
return ctime_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : ctime_;
}
}
/**
* .google.protobuf.StringValue ctime = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getCtimeFieldBuilder() {
if (ctimeBuilder_ == null) {
ctimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getCtime(),
getParentForChildren(),
isClean());
ctime_ = null;
}
return ctimeBuilder_;
}
private com.google.protobuf.StringValue mtime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> mtimeBuilder_;
/**
* .google.protobuf.StringValue mtime = 14;
* @return Whether the mtime field is set.
*/
public boolean hasMtime() {
return mtimeBuilder_ != null || mtime_ != null;
}
/**
* .google.protobuf.StringValue mtime = 14;
* @return The mtime.
*/
public com.google.protobuf.StringValue getMtime() {
if (mtimeBuilder_ == null) {
return mtime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : mtime_;
} else {
return mtimeBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue mtime = 14;
*/
public Builder setMtime(com.google.protobuf.StringValue value) {
if (mtimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
mtime_ = value;
onChanged();
} else {
mtimeBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 14;
*/
public Builder setMtime(
com.google.protobuf.StringValue.Builder builderForValue) {
if (mtimeBuilder_ == null) {
mtime_ = builderForValue.build();
onChanged();
} else {
mtimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 14;
*/
public Builder mergeMtime(com.google.protobuf.StringValue value) {
if (mtimeBuilder_ == null) {
if (mtime_ != null) {
mtime_ =
com.google.protobuf.StringValue.newBuilder(mtime_).mergeFrom(value).buildPartial();
} else {
mtime_ = value;
}
onChanged();
} else {
mtimeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 14;
*/
public Builder clearMtime() {
if (mtimeBuilder_ == null) {
mtime_ = null;
onChanged();
} else {
mtime_ = null;
mtimeBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 14;
*/
public com.google.protobuf.StringValue.Builder getMtimeBuilder() {
onChanged();
return getMtimeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue mtime = 14;
*/
public com.google.protobuf.StringValueOrBuilder getMtimeOrBuilder() {
if (mtimeBuilder_ != null) {
return mtimeBuilder_.getMessageOrBuilder();
} else {
return mtime_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : mtime_;
}
}
/**
* .google.protobuf.StringValue mtime = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getMtimeFieldBuilder() {
if (mtimeBuilder_ == null) {
mtimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getMtime(),
getParentForChildren(),
isClean());
mtime_ = null;
}
return mtimeBuilder_;
}
private com.google.protobuf.StringValue revision_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> revisionBuilder_;
/**
* .google.protobuf.StringValue revision = 15;
* @return Whether the revision field is set.
*/
public boolean hasRevision() {
return revisionBuilder_ != null || revision_ != null;
}
/**
* .google.protobuf.StringValue revision = 15;
* @return The revision.
*/
public com.google.protobuf.StringValue getRevision() {
if (revisionBuilder_ == null) {
return revision_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : revision_;
} else {
return revisionBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue revision = 15;
*/
public Builder setRevision(com.google.protobuf.StringValue value) {
if (revisionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
revision_ = value;
onChanged();
} else {
revisionBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue revision = 15;
*/
public Builder setRevision(
com.google.protobuf.StringValue.Builder builderForValue) {
if (revisionBuilder_ == null) {
revision_ = builderForValue.build();
onChanged();
} else {
revisionBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue revision = 15;
*/
public Builder mergeRevision(com.google.protobuf.StringValue value) {
if (revisionBuilder_ == null) {
if (revision_ != null) {
revision_ =
com.google.protobuf.StringValue.newBuilder(revision_).mergeFrom(value).buildPartial();
} else {
revision_ = value;
}
onChanged();
} else {
revisionBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue revision = 15;
*/
public Builder clearRevision() {
if (revisionBuilder_ == null) {
revision_ = null;
onChanged();
} else {
revision_ = null;
revisionBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue revision = 15;
*/
public com.google.protobuf.StringValue.Builder getRevisionBuilder() {
onChanged();
return getRevisionFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue revision = 15;
*/
public com.google.protobuf.StringValueOrBuilder getRevisionOrBuilder() {
if (revisionBuilder_ != null) {
return revisionBuilder_.getMessageOrBuilder();
} else {
return revision_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : revision_;
}
}
/**
* .google.protobuf.StringValue revision = 15;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getRevisionFieldBuilder() {
if (revisionBuilder_ == null) {
revisionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getRevision(),
getParentForChildren(),
isClean());
revision_ = null;
}
return revisionBuilder_;
}
private com.google.protobuf.StringValue platformId_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> platformIdBuilder_;
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
* @return Whether the platformId field is set.
*/
public boolean hasPlatformId() {
return platformIdBuilder_ != null || platformId_ != null;
}
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
* @return The platformId.
*/
public com.google.protobuf.StringValue getPlatformId() {
if (platformIdBuilder_ == null) {
return platformId_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : platformId_;
} else {
return platformIdBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
*/
public Builder setPlatformId(com.google.protobuf.StringValue value) {
if (platformIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
platformId_ = value;
onChanged();
} else {
platformIdBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
*/
public Builder setPlatformId(
com.google.protobuf.StringValue.Builder builderForValue) {
if (platformIdBuilder_ == null) {
platformId_ = builderForValue.build();
onChanged();
} else {
platformIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
*/
public Builder mergePlatformId(com.google.protobuf.StringValue value) {
if (platformIdBuilder_ == null) {
if (platformId_ != null) {
platformId_ =
com.google.protobuf.StringValue.newBuilder(platformId_).mergeFrom(value).buildPartial();
} else {
platformId_ = value;
}
onChanged();
} else {
platformIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
*/
public Builder clearPlatformId() {
if (platformIdBuilder_ == null) {
platformId_ = null;
onChanged();
} else {
platformId_ = null;
platformIdBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
*/
public com.google.protobuf.StringValue.Builder getPlatformIdBuilder() {
onChanged();
return getPlatformIdFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
*/
public com.google.protobuf.StringValueOrBuilder getPlatformIdOrBuilder() {
if (platformIdBuilder_ != null) {
return platformIdBuilder_.getMessageOrBuilder();
} else {
return platformId_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : platformId_;
}
}
/**
* .google.protobuf.StringValue platform_id = 16 [json_name = "platform_id"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getPlatformIdFieldBuilder() {
if (platformIdBuilder_ == null) {
platformIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getPlatformId(),
getParentForChildren(),
isClean());
platformId_ = null;
}
return platformIdBuilder_;
}
private com.google.protobuf.UInt32Value totalInstanceCount_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> totalInstanceCountBuilder_;
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
* @return Whether the totalInstanceCount field is set.
*/
public boolean hasTotalInstanceCount() {
return totalInstanceCountBuilder_ != null || totalInstanceCount_ != null;
}
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
* @return The totalInstanceCount.
*/
public com.google.protobuf.UInt32Value getTotalInstanceCount() {
if (totalInstanceCountBuilder_ == null) {
return totalInstanceCount_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : totalInstanceCount_;
} else {
return totalInstanceCountBuilder_.getMessage();
}
}
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
*/
public Builder setTotalInstanceCount(com.google.protobuf.UInt32Value value) {
if (totalInstanceCountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
totalInstanceCount_ = value;
onChanged();
} else {
totalInstanceCountBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
*/
public Builder setTotalInstanceCount(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (totalInstanceCountBuilder_ == null) {
totalInstanceCount_ = builderForValue.build();
onChanged();
} else {
totalInstanceCountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
*/
public Builder mergeTotalInstanceCount(com.google.protobuf.UInt32Value value) {
if (totalInstanceCountBuilder_ == null) {
if (totalInstanceCount_ != null) {
totalInstanceCount_ =
com.google.protobuf.UInt32Value.newBuilder(totalInstanceCount_).mergeFrom(value).buildPartial();
} else {
totalInstanceCount_ = value;
}
onChanged();
} else {
totalInstanceCountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
*/
public Builder clearTotalInstanceCount() {
if (totalInstanceCountBuilder_ == null) {
totalInstanceCount_ = null;
onChanged();
} else {
totalInstanceCount_ = null;
totalInstanceCountBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
*/
public com.google.protobuf.UInt32Value.Builder getTotalInstanceCountBuilder() {
onChanged();
return getTotalInstanceCountFieldBuilder().getBuilder();
}
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
*/
public com.google.protobuf.UInt32ValueOrBuilder getTotalInstanceCountOrBuilder() {
if (totalInstanceCountBuilder_ != null) {
return totalInstanceCountBuilder_.getMessageOrBuilder();
} else {
return totalInstanceCount_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : totalInstanceCount_;
}
}
/**
* .google.protobuf.UInt32Value total_instance_count = 17 [json_name = "total_instance_count"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getTotalInstanceCountFieldBuilder() {
if (totalInstanceCountBuilder_ == null) {
totalInstanceCountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getTotalInstanceCount(),
getParentForChildren(),
isClean());
totalInstanceCount_ = null;
}
return totalInstanceCountBuilder_;
}
private com.google.protobuf.UInt32Value healthyInstanceCount_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> healthyInstanceCountBuilder_;
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
* @return Whether the healthyInstanceCount field is set.
*/
public boolean hasHealthyInstanceCount() {
return healthyInstanceCountBuilder_ != null || healthyInstanceCount_ != null;
}
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
* @return The healthyInstanceCount.
*/
public com.google.protobuf.UInt32Value getHealthyInstanceCount() {
if (healthyInstanceCountBuilder_ == null) {
return healthyInstanceCount_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : healthyInstanceCount_;
} else {
return healthyInstanceCountBuilder_.getMessage();
}
}
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
*/
public Builder setHealthyInstanceCount(com.google.protobuf.UInt32Value value) {
if (healthyInstanceCountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
healthyInstanceCount_ = value;
onChanged();
} else {
healthyInstanceCountBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
*/
public Builder setHealthyInstanceCount(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (healthyInstanceCountBuilder_ == null) {
healthyInstanceCount_ = builderForValue.build();
onChanged();
} else {
healthyInstanceCountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
*/
public Builder mergeHealthyInstanceCount(com.google.protobuf.UInt32Value value) {
if (healthyInstanceCountBuilder_ == null) {
if (healthyInstanceCount_ != null) {
healthyInstanceCount_ =
com.google.protobuf.UInt32Value.newBuilder(healthyInstanceCount_).mergeFrom(value).buildPartial();
} else {
healthyInstanceCount_ = value;
}
onChanged();
} else {
healthyInstanceCountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
*/
public Builder clearHealthyInstanceCount() {
if (healthyInstanceCountBuilder_ == null) {
healthyInstanceCount_ = null;
onChanged();
} else {
healthyInstanceCount_ = null;
healthyInstanceCountBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
*/
public com.google.protobuf.UInt32Value.Builder getHealthyInstanceCountBuilder() {
onChanged();
return getHealthyInstanceCountFieldBuilder().getBuilder();
}
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
*/
public com.google.protobuf.UInt32ValueOrBuilder getHealthyInstanceCountOrBuilder() {
if (healthyInstanceCountBuilder_ != null) {
return healthyInstanceCountBuilder_.getMessageOrBuilder();
} else {
return healthyInstanceCount_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : healthyInstanceCount_;
}
}
/**
* .google.protobuf.UInt32Value healthy_instance_count = 18 [json_name = "healthy_instance_count"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getHealthyInstanceCountFieldBuilder() {
if (healthyInstanceCountBuilder_ == null) {
healthyInstanceCountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getHealthyInstanceCount(),
getParentForChildren(),
isClean());
healthyInstanceCount_ = null;
}
return healthyInstanceCountBuilder_;
}
private java.util.List userIds_ =
java.util.Collections.emptyList();
private void ensureUserIdsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
userIds_ = new java.util.ArrayList(userIds_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> userIdsBuilder_;
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public java.util.List getUserIdsList() {
if (userIdsBuilder_ == null) {
return java.util.Collections.unmodifiableList(userIds_);
} else {
return userIdsBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public int getUserIdsCount() {
if (userIdsBuilder_ == null) {
return userIds_.size();
} else {
return userIdsBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public com.google.protobuf.StringValue getUserIds(int index) {
if (userIdsBuilder_ == null) {
return userIds_.get(index);
} else {
return userIdsBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public Builder setUserIds(
int index, com.google.protobuf.StringValue value) {
if (userIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserIdsIsMutable();
userIds_.set(index, value);
onChanged();
} else {
userIdsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public Builder setUserIds(
int index, com.google.protobuf.StringValue.Builder builderForValue) {
if (userIdsBuilder_ == null) {
ensureUserIdsIsMutable();
userIds_.set(index, builderForValue.build());
onChanged();
} else {
userIdsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public Builder addUserIds(com.google.protobuf.StringValue value) {
if (userIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserIdsIsMutable();
userIds_.add(value);
onChanged();
} else {
userIdsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public Builder addUserIds(
int index, com.google.protobuf.StringValue value) {
if (userIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUserIdsIsMutable();
userIds_.add(index, value);
onChanged();
} else {
userIdsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public Builder addUserIds(
com.google.protobuf.StringValue.Builder builderForValue) {
if (userIdsBuilder_ == null) {
ensureUserIdsIsMutable();
userIds_.add(builderForValue.build());
onChanged();
} else {
userIdsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public Builder addUserIds(
int index, com.google.protobuf.StringValue.Builder builderForValue) {
if (userIdsBuilder_ == null) {
ensureUserIdsIsMutable();
userIds_.add(index, builderForValue.build());
onChanged();
} else {
userIdsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public Builder addAllUserIds(
java.lang.Iterable extends com.google.protobuf.StringValue> values) {
if (userIdsBuilder_ == null) {
ensureUserIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, userIds_);
onChanged();
} else {
userIdsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public Builder clearUserIds() {
if (userIdsBuilder_ == null) {
userIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
userIdsBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public Builder removeUserIds(int index) {
if (userIdsBuilder_ == null) {
ensureUserIdsIsMutable();
userIds_.remove(index);
onChanged();
} else {
userIdsBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public com.google.protobuf.StringValue.Builder getUserIdsBuilder(
int index) {
return getUserIdsFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public com.google.protobuf.StringValueOrBuilder getUserIdsOrBuilder(
int index) {
if (userIdsBuilder_ == null) {
return userIds_.get(index); } else {
return userIdsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public java.util.List extends com.google.protobuf.StringValueOrBuilder>
getUserIdsOrBuilderList() {
if (userIdsBuilder_ != null) {
return userIdsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(userIds_);
}
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public com.google.protobuf.StringValue.Builder addUserIdsBuilder() {
return getUserIdsFieldBuilder().addBuilder(
com.google.protobuf.StringValue.getDefaultInstance());
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public com.google.protobuf.StringValue.Builder addUserIdsBuilder(
int index) {
return getUserIdsFieldBuilder().addBuilder(
index, com.google.protobuf.StringValue.getDefaultInstance());
}
/**
* repeated .google.protobuf.StringValue user_ids = 19 [json_name = "user_ids"];
*/
public java.util.List
getUserIdsBuilderList() {
return getUserIdsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getUserIdsFieldBuilder() {
if (userIdsBuilder_ == null) {
userIdsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
userIds_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
userIds_ = null;
}
return userIdsBuilder_;
}
private java.util.List groupIds_ =
java.util.Collections.emptyList();
private void ensureGroupIdsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
groupIds_ = new java.util.ArrayList(groupIds_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> groupIdsBuilder_;
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public java.util.List getGroupIdsList() {
if (groupIdsBuilder_ == null) {
return java.util.Collections.unmodifiableList(groupIds_);
} else {
return groupIdsBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public int getGroupIdsCount() {
if (groupIdsBuilder_ == null) {
return groupIds_.size();
} else {
return groupIdsBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public com.google.protobuf.StringValue getGroupIds(int index) {
if (groupIdsBuilder_ == null) {
return groupIds_.get(index);
} else {
return groupIdsBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public Builder setGroupIds(
int index, com.google.protobuf.StringValue value) {
if (groupIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupIdsIsMutable();
groupIds_.set(index, value);
onChanged();
} else {
groupIdsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public Builder setGroupIds(
int index, com.google.protobuf.StringValue.Builder builderForValue) {
if (groupIdsBuilder_ == null) {
ensureGroupIdsIsMutable();
groupIds_.set(index, builderForValue.build());
onChanged();
} else {
groupIdsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public Builder addGroupIds(com.google.protobuf.StringValue value) {
if (groupIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupIdsIsMutable();
groupIds_.add(value);
onChanged();
} else {
groupIdsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public Builder addGroupIds(
int index, com.google.protobuf.StringValue value) {
if (groupIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGroupIdsIsMutable();
groupIds_.add(index, value);
onChanged();
} else {
groupIdsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public Builder addGroupIds(
com.google.protobuf.StringValue.Builder builderForValue) {
if (groupIdsBuilder_ == null) {
ensureGroupIdsIsMutable();
groupIds_.add(builderForValue.build());
onChanged();
} else {
groupIdsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public Builder addGroupIds(
int index, com.google.protobuf.StringValue.Builder builderForValue) {
if (groupIdsBuilder_ == null) {
ensureGroupIdsIsMutable();
groupIds_.add(index, builderForValue.build());
onChanged();
} else {
groupIdsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public Builder addAllGroupIds(
java.lang.Iterable extends com.google.protobuf.StringValue> values) {
if (groupIdsBuilder_ == null) {
ensureGroupIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, groupIds_);
onChanged();
} else {
groupIdsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public Builder clearGroupIds() {
if (groupIdsBuilder_ == null) {
groupIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
groupIdsBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public Builder removeGroupIds(int index) {
if (groupIdsBuilder_ == null) {
ensureGroupIdsIsMutable();
groupIds_.remove(index);
onChanged();
} else {
groupIdsBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public com.google.protobuf.StringValue.Builder getGroupIdsBuilder(
int index) {
return getGroupIdsFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public com.google.protobuf.StringValueOrBuilder getGroupIdsOrBuilder(
int index) {
if (groupIdsBuilder_ == null) {
return groupIds_.get(index); } else {
return groupIdsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public java.util.List extends com.google.protobuf.StringValueOrBuilder>
getGroupIdsOrBuilderList() {
if (groupIdsBuilder_ != null) {
return groupIdsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(groupIds_);
}
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public com.google.protobuf.StringValue.Builder addGroupIdsBuilder() {
return getGroupIdsFieldBuilder().addBuilder(
com.google.protobuf.StringValue.getDefaultInstance());
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public com.google.protobuf.StringValue.Builder addGroupIdsBuilder(
int index) {
return getGroupIdsFieldBuilder().addBuilder(
index, com.google.protobuf.StringValue.getDefaultInstance());
}
/**
* repeated .google.protobuf.StringValue group_ids = 20 [json_name = "group_ids"];
*/
public java.util.List
getGroupIdsBuilderList() {
return getGroupIdsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getGroupIdsFieldBuilder() {
if (groupIdsBuilder_ == null) {
groupIdsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
groupIds_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
groupIds_ = null;
}
return groupIdsBuilder_;
}
private java.util.List removeUserIds_ =
java.util.Collections.emptyList();
private void ensureRemoveUserIdsIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
removeUserIds_ = new java.util.ArrayList(removeUserIds_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> removeUserIdsBuilder_;
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public java.util.List getRemoveUserIdsList() {
if (removeUserIdsBuilder_ == null) {
return java.util.Collections.unmodifiableList(removeUserIds_);
} else {
return removeUserIdsBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public int getRemoveUserIdsCount() {
if (removeUserIdsBuilder_ == null) {
return removeUserIds_.size();
} else {
return removeUserIdsBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public com.google.protobuf.StringValue getRemoveUserIds(int index) {
if (removeUserIdsBuilder_ == null) {
return removeUserIds_.get(index);
} else {
return removeUserIdsBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public Builder setRemoveUserIds(
int index, com.google.protobuf.StringValue value) {
if (removeUserIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRemoveUserIdsIsMutable();
removeUserIds_.set(index, value);
onChanged();
} else {
removeUserIdsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public Builder setRemoveUserIds(
int index, com.google.protobuf.StringValue.Builder builderForValue) {
if (removeUserIdsBuilder_ == null) {
ensureRemoveUserIdsIsMutable();
removeUserIds_.set(index, builderForValue.build());
onChanged();
} else {
removeUserIdsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public Builder addRemoveUserIds(com.google.protobuf.StringValue value) {
if (removeUserIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRemoveUserIdsIsMutable();
removeUserIds_.add(value);
onChanged();
} else {
removeUserIdsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public Builder addRemoveUserIds(
int index, com.google.protobuf.StringValue value) {
if (removeUserIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRemoveUserIdsIsMutable();
removeUserIds_.add(index, value);
onChanged();
} else {
removeUserIdsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public Builder addRemoveUserIds(
com.google.protobuf.StringValue.Builder builderForValue) {
if (removeUserIdsBuilder_ == null) {
ensureRemoveUserIdsIsMutable();
removeUserIds_.add(builderForValue.build());
onChanged();
} else {
removeUserIdsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public Builder addRemoveUserIds(
int index, com.google.protobuf.StringValue.Builder builderForValue) {
if (removeUserIdsBuilder_ == null) {
ensureRemoveUserIdsIsMutable();
removeUserIds_.add(index, builderForValue.build());
onChanged();
} else {
removeUserIdsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public Builder addAllRemoveUserIds(
java.lang.Iterable extends com.google.protobuf.StringValue> values) {
if (removeUserIdsBuilder_ == null) {
ensureRemoveUserIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, removeUserIds_);
onChanged();
} else {
removeUserIdsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public Builder clearRemoveUserIds() {
if (removeUserIdsBuilder_ == null) {
removeUserIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
removeUserIdsBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public Builder removeRemoveUserIds(int index) {
if (removeUserIdsBuilder_ == null) {
ensureRemoveUserIdsIsMutable();
removeUserIds_.remove(index);
onChanged();
} else {
removeUserIdsBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public com.google.protobuf.StringValue.Builder getRemoveUserIdsBuilder(
int index) {
return getRemoveUserIdsFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public com.google.protobuf.StringValueOrBuilder getRemoveUserIdsOrBuilder(
int index) {
if (removeUserIdsBuilder_ == null) {
return removeUserIds_.get(index); } else {
return removeUserIdsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public java.util.List extends com.google.protobuf.StringValueOrBuilder>
getRemoveUserIdsOrBuilderList() {
if (removeUserIdsBuilder_ != null) {
return removeUserIdsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(removeUserIds_);
}
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public com.google.protobuf.StringValue.Builder addRemoveUserIdsBuilder() {
return getRemoveUserIdsFieldBuilder().addBuilder(
com.google.protobuf.StringValue.getDefaultInstance());
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public com.google.protobuf.StringValue.Builder addRemoveUserIdsBuilder(
int index) {
return getRemoveUserIdsFieldBuilder().addBuilder(
index, com.google.protobuf.StringValue.getDefaultInstance());
}
/**
* repeated .google.protobuf.StringValue remove_user_ids = 22 [json_name = "remove_user_ids"];
*/
public java.util.List
getRemoveUserIdsBuilderList() {
return getRemoveUserIdsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getRemoveUserIdsFieldBuilder() {
if (removeUserIdsBuilder_ == null) {
removeUserIdsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
removeUserIds_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
removeUserIds_ = null;
}
return removeUserIdsBuilder_;
}
private java.util.List removeGroupIds_ =
java.util.Collections.emptyList();
private void ensureRemoveGroupIdsIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
removeGroupIds_ = new java.util.ArrayList(removeGroupIds_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> removeGroupIdsBuilder_;
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public java.util.List getRemoveGroupIdsList() {
if (removeGroupIdsBuilder_ == null) {
return java.util.Collections.unmodifiableList(removeGroupIds_);
} else {
return removeGroupIdsBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public int getRemoveGroupIdsCount() {
if (removeGroupIdsBuilder_ == null) {
return removeGroupIds_.size();
} else {
return removeGroupIdsBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public com.google.protobuf.StringValue getRemoveGroupIds(int index) {
if (removeGroupIdsBuilder_ == null) {
return removeGroupIds_.get(index);
} else {
return removeGroupIdsBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public Builder setRemoveGroupIds(
int index, com.google.protobuf.StringValue value) {
if (removeGroupIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRemoveGroupIdsIsMutable();
removeGroupIds_.set(index, value);
onChanged();
} else {
removeGroupIdsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public Builder setRemoveGroupIds(
int index, com.google.protobuf.StringValue.Builder builderForValue) {
if (removeGroupIdsBuilder_ == null) {
ensureRemoveGroupIdsIsMutable();
removeGroupIds_.set(index, builderForValue.build());
onChanged();
} else {
removeGroupIdsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public Builder addRemoveGroupIds(com.google.protobuf.StringValue value) {
if (removeGroupIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRemoveGroupIdsIsMutable();
removeGroupIds_.add(value);
onChanged();
} else {
removeGroupIdsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public Builder addRemoveGroupIds(
int index, com.google.protobuf.StringValue value) {
if (removeGroupIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRemoveGroupIdsIsMutable();
removeGroupIds_.add(index, value);
onChanged();
} else {
removeGroupIdsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public Builder addRemoveGroupIds(
com.google.protobuf.StringValue.Builder builderForValue) {
if (removeGroupIdsBuilder_ == null) {
ensureRemoveGroupIdsIsMutable();
removeGroupIds_.add(builderForValue.build());
onChanged();
} else {
removeGroupIdsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public Builder addRemoveGroupIds(
int index, com.google.protobuf.StringValue.Builder builderForValue) {
if (removeGroupIdsBuilder_ == null) {
ensureRemoveGroupIdsIsMutable();
removeGroupIds_.add(index, builderForValue.build());
onChanged();
} else {
removeGroupIdsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public Builder addAllRemoveGroupIds(
java.lang.Iterable extends com.google.protobuf.StringValue> values) {
if (removeGroupIdsBuilder_ == null) {
ensureRemoveGroupIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, removeGroupIds_);
onChanged();
} else {
removeGroupIdsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public Builder clearRemoveGroupIds() {
if (removeGroupIdsBuilder_ == null) {
removeGroupIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
removeGroupIdsBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public Builder removeRemoveGroupIds(int index) {
if (removeGroupIdsBuilder_ == null) {
ensureRemoveGroupIdsIsMutable();
removeGroupIds_.remove(index);
onChanged();
} else {
removeGroupIdsBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public com.google.protobuf.StringValue.Builder getRemoveGroupIdsBuilder(
int index) {
return getRemoveGroupIdsFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public com.google.protobuf.StringValueOrBuilder getRemoveGroupIdsOrBuilder(
int index) {
if (removeGroupIdsBuilder_ == null) {
return removeGroupIds_.get(index); } else {
return removeGroupIdsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public java.util.List extends com.google.protobuf.StringValueOrBuilder>
getRemoveGroupIdsOrBuilderList() {
if (removeGroupIdsBuilder_ != null) {
return removeGroupIdsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(removeGroupIds_);
}
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public com.google.protobuf.StringValue.Builder addRemoveGroupIdsBuilder() {
return getRemoveGroupIdsFieldBuilder().addBuilder(
com.google.protobuf.StringValue.getDefaultInstance());
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public com.google.protobuf.StringValue.Builder addRemoveGroupIdsBuilder(
int index) {
return getRemoveGroupIdsFieldBuilder().addBuilder(
index, com.google.protobuf.StringValue.getDefaultInstance());
}
/**
* repeated .google.protobuf.StringValue remove_group_ids = 23 [json_name = "remove_group_ids"];
*/
public java.util.List
getRemoveGroupIdsBuilderList() {
return getRemoveGroupIdsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getRemoveGroupIdsFieldBuilder() {
if (removeGroupIdsBuilder_ == null) {
removeGroupIdsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
removeGroupIds_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
removeGroupIds_ = null;
}
return removeGroupIdsBuilder_;
}
private com.google.protobuf.StringValue id_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> idBuilder_;
/**
* .google.protobuf.StringValue id = 21;
* @return Whether the id field is set.
*/
public boolean hasId() {
return idBuilder_ != null || id_ != null;
}
/**
* .google.protobuf.StringValue id = 21;
* @return The id.
*/
public com.google.protobuf.StringValue getId() {
if (idBuilder_ == null) {
return id_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue id = 21;
*/
public Builder setId(com.google.protobuf.StringValue value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue id = 21;
*/
public Builder setId(
com.google.protobuf.StringValue.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue id = 21;
*/
public Builder mergeId(com.google.protobuf.StringValue value) {
if (idBuilder_ == null) {
if (id_ != null) {
id_ =
com.google.protobuf.StringValue.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue id = 21;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = null;
onChanged();
} else {
id_ = null;
idBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue id = 21;
*/
public com.google.protobuf.StringValue.Builder getIdBuilder() {
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue id = 21;
*/
public com.google.protobuf.StringValueOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : id_;
}
}
/**
* .google.protobuf.StringValue id = 21;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getId(),
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
private com.google.protobuf.BoolValue editable_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder> editableBuilder_;
/**
* .google.protobuf.BoolValue editable = 24;
* @return Whether the editable field is set.
*/
public boolean hasEditable() {
return editableBuilder_ != null || editable_ != null;
}
/**
* .google.protobuf.BoolValue editable = 24;
* @return The editable.
*/
public com.google.protobuf.BoolValue getEditable() {
if (editableBuilder_ == null) {
return editable_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : editable_;
} else {
return editableBuilder_.getMessage();
}
}
/**
* .google.protobuf.BoolValue editable = 24;
*/
public Builder setEditable(com.google.protobuf.BoolValue value) {
if (editableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
editable_ = value;
onChanged();
} else {
editableBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.BoolValue editable = 24;
*/
public Builder setEditable(
com.google.protobuf.BoolValue.Builder builderForValue) {
if (editableBuilder_ == null) {
editable_ = builderForValue.build();
onChanged();
} else {
editableBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.BoolValue editable = 24;
*/
public Builder mergeEditable(com.google.protobuf.BoolValue value) {
if (editableBuilder_ == null) {
if (editable_ != null) {
editable_ =
com.google.protobuf.BoolValue.newBuilder(editable_).mergeFrom(value).buildPartial();
} else {
editable_ = value;
}
onChanged();
} else {
editableBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.BoolValue editable = 24;
*/
public Builder clearEditable() {
if (editableBuilder_ == null) {
editable_ = null;
onChanged();
} else {
editable_ = null;
editableBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.BoolValue editable = 24;
*/
public com.google.protobuf.BoolValue.Builder getEditableBuilder() {
onChanged();
return getEditableFieldBuilder().getBuilder();
}
/**
* .google.protobuf.BoolValue editable = 24;
*/
public com.google.protobuf.BoolValueOrBuilder getEditableOrBuilder() {
if (editableBuilder_ != null) {
return editableBuilder_.getMessageOrBuilder();
} else {
return editable_ == null ?
com.google.protobuf.BoolValue.getDefaultInstance() : editable_;
}
}
/**
* .google.protobuf.BoolValue editable = 24;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>
getEditableFieldBuilder() {
if (editableBuilder_ == null) {
editableBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>(
getEditable(),
getParentForChildren(),
isClean());
editable_ = null;
}
return editableBuilder_;
}
private java.util.List exportTo_ =
java.util.Collections.emptyList();
private void ensureExportToIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
exportTo_ = new java.util.ArrayList(exportTo_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> exportToBuilder_;
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public java.util.List getExportToList() {
if (exportToBuilder_ == null) {
return java.util.Collections.unmodifiableList(exportTo_);
} else {
return exportToBuilder_.getMessageList();
}
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public int getExportToCount() {
if (exportToBuilder_ == null) {
return exportTo_.size();
} else {
return exportToBuilder_.getCount();
}
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public com.google.protobuf.StringValue getExportTo(int index) {
if (exportToBuilder_ == null) {
return exportTo_.get(index);
} else {
return exportToBuilder_.getMessage(index);
}
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public Builder setExportTo(
int index, com.google.protobuf.StringValue value) {
if (exportToBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExportToIsMutable();
exportTo_.set(index, value);
onChanged();
} else {
exportToBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public Builder setExportTo(
int index, com.google.protobuf.StringValue.Builder builderForValue) {
if (exportToBuilder_ == null) {
ensureExportToIsMutable();
exportTo_.set(index, builderForValue.build());
onChanged();
} else {
exportToBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public Builder addExportTo(com.google.protobuf.StringValue value) {
if (exportToBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExportToIsMutable();
exportTo_.add(value);
onChanged();
} else {
exportToBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public Builder addExportTo(
int index, com.google.protobuf.StringValue value) {
if (exportToBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExportToIsMutable();
exportTo_.add(index, value);
onChanged();
} else {
exportToBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public Builder addExportTo(
com.google.protobuf.StringValue.Builder builderForValue) {
if (exportToBuilder_ == null) {
ensureExportToIsMutable();
exportTo_.add(builderForValue.build());
onChanged();
} else {
exportToBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public Builder addExportTo(
int index, com.google.protobuf.StringValue.Builder builderForValue) {
if (exportToBuilder_ == null) {
ensureExportToIsMutable();
exportTo_.add(index, builderForValue.build());
onChanged();
} else {
exportToBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public Builder addAllExportTo(
java.lang.Iterable extends com.google.protobuf.StringValue> values) {
if (exportToBuilder_ == null) {
ensureExportToIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, exportTo_);
onChanged();
} else {
exportToBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public Builder clearExportTo() {
if (exportToBuilder_ == null) {
exportTo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
exportToBuilder_.clear();
}
return this;
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public Builder removeExportTo(int index) {
if (exportToBuilder_ == null) {
ensureExportToIsMutable();
exportTo_.remove(index);
onChanged();
} else {
exportToBuilder_.remove(index);
}
return this;
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public com.google.protobuf.StringValue.Builder getExportToBuilder(
int index) {
return getExportToFieldBuilder().getBuilder(index);
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public com.google.protobuf.StringValueOrBuilder getExportToOrBuilder(
int index) {
if (exportToBuilder_ == null) {
return exportTo_.get(index); } else {
return exportToBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public java.util.List extends com.google.protobuf.StringValueOrBuilder>
getExportToOrBuilderList() {
if (exportToBuilder_ != null) {
return exportToBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(exportTo_);
}
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public com.google.protobuf.StringValue.Builder addExportToBuilder() {
return getExportToFieldBuilder().addBuilder(
com.google.protobuf.StringValue.getDefaultInstance());
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public com.google.protobuf.StringValue.Builder addExportToBuilder(
int index) {
return getExportToFieldBuilder().addBuilder(
index, com.google.protobuf.StringValue.getDefaultInstance());
}
/**
* repeated .google.protobuf.StringValue export_to = 25 [json_name = "export_to"];
*/
public java.util.List
getExportToBuilderList() {
return getExportToFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getExportToFieldBuilder() {
if (exportToBuilder_ == null) {
exportToBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
exportTo_,
((bitField0_ & 0x00000020) != 0),
getParentForChildren(),
isClean());
exportTo_ = null;
}
return exportToBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:v1.Service)
}
// @@protoc_insertion_point(class_scope:v1.Service)
private static final com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service();
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Service parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Service getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ServiceAliasOrBuilder extends
// @@protoc_insertion_point(interface_extends:v1.ServiceAlias)
com.google.protobuf.MessageOrBuilder {
/**
* .google.protobuf.StringValue service = 1;
* @return Whether the service field is set.
*/
boolean hasService();
/**
* .google.protobuf.StringValue service = 1;
* @return The service.
*/
com.google.protobuf.StringValue getService();
/**
* .google.protobuf.StringValue service = 1;
*/
com.google.protobuf.StringValueOrBuilder getServiceOrBuilder();
/**
* .google.protobuf.StringValue namespace = 2;
* @return Whether the namespace field is set.
*/
boolean hasNamespace();
/**
* .google.protobuf.StringValue namespace = 2;
* @return The namespace.
*/
com.google.protobuf.StringValue getNamespace();
/**
* .google.protobuf.StringValue namespace = 2;
*/
com.google.protobuf.StringValueOrBuilder getNamespaceOrBuilder();
/**
* .google.protobuf.StringValue alias = 3;
* @return Whether the alias field is set.
*/
boolean hasAlias();
/**
* .google.protobuf.StringValue alias = 3;
* @return The alias.
*/
com.google.protobuf.StringValue getAlias();
/**
* .google.protobuf.StringValue alias = 3;
*/
com.google.protobuf.StringValueOrBuilder getAliasOrBuilder();
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
* @return Whether the aliasNamespace field is set.
*/
boolean hasAliasNamespace();
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
* @return The aliasNamespace.
*/
com.google.protobuf.StringValue getAliasNamespace();
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
*/
com.google.protobuf.StringValueOrBuilder getAliasNamespaceOrBuilder();
/**
* .v1.AliasType type = 5;
* @return The enum numeric value on the wire for type.
*/
int getTypeValue();
/**
* .v1.AliasType type = 5;
* @return The type.
*/
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType getType();
/**
* .google.protobuf.StringValue owners = 6;
* @return Whether the owners field is set.
*/
boolean hasOwners();
/**
* .google.protobuf.StringValue owners = 6;
* @return The owners.
*/
com.google.protobuf.StringValue getOwners();
/**
* .google.protobuf.StringValue owners = 6;
*/
com.google.protobuf.StringValueOrBuilder getOwnersOrBuilder();
/**
* .google.protobuf.StringValue comment = 7;
* @return Whether the comment field is set.
*/
boolean hasComment();
/**
* .google.protobuf.StringValue comment = 7;
* @return The comment.
*/
com.google.protobuf.StringValue getComment();
/**
* .google.protobuf.StringValue comment = 7;
*/
com.google.protobuf.StringValueOrBuilder getCommentOrBuilder();
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
* @return Whether the serviceToken field is set.
*/
boolean hasServiceToken();
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
* @return The serviceToken.
*/
com.google.protobuf.StringValue getServiceToken();
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
*/
com.google.protobuf.StringValueOrBuilder getServiceTokenOrBuilder();
/**
* .google.protobuf.StringValue ctime = 9;
* @return Whether the ctime field is set.
*/
boolean hasCtime();
/**
* .google.protobuf.StringValue ctime = 9;
* @return The ctime.
*/
com.google.protobuf.StringValue getCtime();
/**
* .google.protobuf.StringValue ctime = 9;
*/
com.google.protobuf.StringValueOrBuilder getCtimeOrBuilder();
/**
* .google.protobuf.StringValue mtime = 10;
* @return Whether the mtime field is set.
*/
boolean hasMtime();
/**
* .google.protobuf.StringValue mtime = 10;
* @return The mtime.
*/
com.google.protobuf.StringValue getMtime();
/**
* .google.protobuf.StringValue mtime = 10;
*/
com.google.protobuf.StringValueOrBuilder getMtimeOrBuilder();
/**
* .google.protobuf.StringValue id = 11;
* @return Whether the id field is set.
*/
boolean hasId();
/**
* .google.protobuf.StringValue id = 11;
* @return The id.
*/
com.google.protobuf.StringValue getId();
/**
* .google.protobuf.StringValue id = 11;
*/
com.google.protobuf.StringValueOrBuilder getIdOrBuilder();
/**
* .google.protobuf.BoolValue editable = 12;
* @return Whether the editable field is set.
*/
boolean hasEditable();
/**
* .google.protobuf.BoolValue editable = 12;
* @return The editable.
*/
com.google.protobuf.BoolValue getEditable();
/**
* .google.protobuf.BoolValue editable = 12;
*/
com.google.protobuf.BoolValueOrBuilder getEditableOrBuilder();
}
/**
* Protobuf type {@code v1.ServiceAlias}
*/
public static final class ServiceAlias extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:v1.ServiceAlias)
ServiceAliasOrBuilder {
private static final long serialVersionUID = 0L;
// Use ServiceAlias.newBuilder() to construct.
private ServiceAlias(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ServiceAlias() {
type_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ServiceAlias();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_ServiceAlias_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_ServiceAlias_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias.class, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias.Builder.class);
}
public static final int SERVICE_FIELD_NUMBER = 1;
private com.google.protobuf.StringValue service_;
/**
* .google.protobuf.StringValue service = 1;
* @return Whether the service field is set.
*/
@java.lang.Override
public boolean hasService() {
return service_ != null;
}
/**
* .google.protobuf.StringValue service = 1;
* @return The service.
*/
@java.lang.Override
public com.google.protobuf.StringValue getService() {
return service_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : service_;
}
/**
* .google.protobuf.StringValue service = 1;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getServiceOrBuilder() {
return getService();
}
public static final int NAMESPACE_FIELD_NUMBER = 2;
private com.google.protobuf.StringValue namespace_;
/**
* .google.protobuf.StringValue namespace = 2;
* @return Whether the namespace field is set.
*/
@java.lang.Override
public boolean hasNamespace() {
return namespace_ != null;
}
/**
* .google.protobuf.StringValue namespace = 2;
* @return The namespace.
*/
@java.lang.Override
public com.google.protobuf.StringValue getNamespace() {
return namespace_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : namespace_;
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getNamespaceOrBuilder() {
return getNamespace();
}
public static final int ALIAS_FIELD_NUMBER = 3;
private com.google.protobuf.StringValue alias_;
/**
* .google.protobuf.StringValue alias = 3;
* @return Whether the alias field is set.
*/
@java.lang.Override
public boolean hasAlias() {
return alias_ != null;
}
/**
* .google.protobuf.StringValue alias = 3;
* @return The alias.
*/
@java.lang.Override
public com.google.protobuf.StringValue getAlias() {
return alias_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : alias_;
}
/**
* .google.protobuf.StringValue alias = 3;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getAliasOrBuilder() {
return getAlias();
}
public static final int ALIAS_NAMESPACE_FIELD_NUMBER = 4;
private com.google.protobuf.StringValue aliasNamespace_;
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
* @return Whether the aliasNamespace field is set.
*/
@java.lang.Override
public boolean hasAliasNamespace() {
return aliasNamespace_ != null;
}
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
* @return The aliasNamespace.
*/
@java.lang.Override
public com.google.protobuf.StringValue getAliasNamespace() {
return aliasNamespace_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : aliasNamespace_;
}
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getAliasNamespaceOrBuilder() {
return getAliasNamespace();
}
public static final int TYPE_FIELD_NUMBER = 5;
private int type_;
/**
* .v1.AliasType type = 5;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .v1.AliasType type = 5;
* @return The type.
*/
@java.lang.Override public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType getType() {
@SuppressWarnings("deprecation")
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType result = com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType.valueOf(type_);
return result == null ? com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType.UNRECOGNIZED : result;
}
public static final int OWNERS_FIELD_NUMBER = 6;
private com.google.protobuf.StringValue owners_;
/**
* .google.protobuf.StringValue owners = 6;
* @return Whether the owners field is set.
*/
@java.lang.Override
public boolean hasOwners() {
return owners_ != null;
}
/**
* .google.protobuf.StringValue owners = 6;
* @return The owners.
*/
@java.lang.Override
public com.google.protobuf.StringValue getOwners() {
return owners_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : owners_;
}
/**
* .google.protobuf.StringValue owners = 6;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getOwnersOrBuilder() {
return getOwners();
}
public static final int COMMENT_FIELD_NUMBER = 7;
private com.google.protobuf.StringValue comment_;
/**
* .google.protobuf.StringValue comment = 7;
* @return Whether the comment field is set.
*/
@java.lang.Override
public boolean hasComment() {
return comment_ != null;
}
/**
* .google.protobuf.StringValue comment = 7;
* @return The comment.
*/
@java.lang.Override
public com.google.protobuf.StringValue getComment() {
return comment_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : comment_;
}
/**
* .google.protobuf.StringValue comment = 7;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getCommentOrBuilder() {
return getComment();
}
public static final int SERVICE_TOKEN_FIELD_NUMBER = 8;
private com.google.protobuf.StringValue serviceToken_;
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
* @return Whether the serviceToken field is set.
*/
@java.lang.Override
public boolean hasServiceToken() {
return serviceToken_ != null;
}
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
* @return The serviceToken.
*/
@java.lang.Override
public com.google.protobuf.StringValue getServiceToken() {
return serviceToken_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : serviceToken_;
}
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getServiceTokenOrBuilder() {
return getServiceToken();
}
public static final int CTIME_FIELD_NUMBER = 9;
private com.google.protobuf.StringValue ctime_;
/**
* .google.protobuf.StringValue ctime = 9;
* @return Whether the ctime field is set.
*/
@java.lang.Override
public boolean hasCtime() {
return ctime_ != null;
}
/**
* .google.protobuf.StringValue ctime = 9;
* @return The ctime.
*/
@java.lang.Override
public com.google.protobuf.StringValue getCtime() {
return ctime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : ctime_;
}
/**
* .google.protobuf.StringValue ctime = 9;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getCtimeOrBuilder() {
return getCtime();
}
public static final int MTIME_FIELD_NUMBER = 10;
private com.google.protobuf.StringValue mtime_;
/**
* .google.protobuf.StringValue mtime = 10;
* @return Whether the mtime field is set.
*/
@java.lang.Override
public boolean hasMtime() {
return mtime_ != null;
}
/**
* .google.protobuf.StringValue mtime = 10;
* @return The mtime.
*/
@java.lang.Override
public com.google.protobuf.StringValue getMtime() {
return mtime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : mtime_;
}
/**
* .google.protobuf.StringValue mtime = 10;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getMtimeOrBuilder() {
return getMtime();
}
public static final int ID_FIELD_NUMBER = 11;
private com.google.protobuf.StringValue id_;
/**
* .google.protobuf.StringValue id = 11;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return id_ != null;
}
/**
* .google.protobuf.StringValue id = 11;
* @return The id.
*/
@java.lang.Override
public com.google.protobuf.StringValue getId() {
return id_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : id_;
}
/**
* .google.protobuf.StringValue id = 11;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getIdOrBuilder() {
return getId();
}
public static final int EDITABLE_FIELD_NUMBER = 12;
private com.google.protobuf.BoolValue editable_;
/**
* .google.protobuf.BoolValue editable = 12;
* @return Whether the editable field is set.
*/
@java.lang.Override
public boolean hasEditable() {
return editable_ != null;
}
/**
* .google.protobuf.BoolValue editable = 12;
* @return The editable.
*/
@java.lang.Override
public com.google.protobuf.BoolValue getEditable() {
return editable_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : editable_;
}
/**
* .google.protobuf.BoolValue editable = 12;
*/
@java.lang.Override
public com.google.protobuf.BoolValueOrBuilder getEditableOrBuilder() {
return getEditable();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (service_ != null) {
output.writeMessage(1, getService());
}
if (namespace_ != null) {
output.writeMessage(2, getNamespace());
}
if (alias_ != null) {
output.writeMessage(3, getAlias());
}
if (aliasNamespace_ != null) {
output.writeMessage(4, getAliasNamespace());
}
if (type_ != com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType.DEFAULT.getNumber()) {
output.writeEnum(5, type_);
}
if (owners_ != null) {
output.writeMessage(6, getOwners());
}
if (comment_ != null) {
output.writeMessage(7, getComment());
}
if (serviceToken_ != null) {
output.writeMessage(8, getServiceToken());
}
if (ctime_ != null) {
output.writeMessage(9, getCtime());
}
if (mtime_ != null) {
output.writeMessage(10, getMtime());
}
if (id_ != null) {
output.writeMessage(11, getId());
}
if (editable_ != null) {
output.writeMessage(12, getEditable());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (service_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getService());
}
if (namespace_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getNamespace());
}
if (alias_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getAlias());
}
if (aliasNamespace_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getAliasNamespace());
}
if (type_ != com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType.DEFAULT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, type_);
}
if (owners_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getOwners());
}
if (comment_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getComment());
}
if (serviceToken_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getServiceToken());
}
if (ctime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getCtime());
}
if (mtime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getMtime());
}
if (id_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getId());
}
if (editable_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getEditable());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias)) {
return super.equals(obj);
}
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias other = (com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias) obj;
if (hasService() != other.hasService()) return false;
if (hasService()) {
if (!getService()
.equals(other.getService())) return false;
}
if (hasNamespace() != other.hasNamespace()) return false;
if (hasNamespace()) {
if (!getNamespace()
.equals(other.getNamespace())) return false;
}
if (hasAlias() != other.hasAlias()) return false;
if (hasAlias()) {
if (!getAlias()
.equals(other.getAlias())) return false;
}
if (hasAliasNamespace() != other.hasAliasNamespace()) return false;
if (hasAliasNamespace()) {
if (!getAliasNamespace()
.equals(other.getAliasNamespace())) return false;
}
if (type_ != other.type_) return false;
if (hasOwners() != other.hasOwners()) return false;
if (hasOwners()) {
if (!getOwners()
.equals(other.getOwners())) return false;
}
if (hasComment() != other.hasComment()) return false;
if (hasComment()) {
if (!getComment()
.equals(other.getComment())) return false;
}
if (hasServiceToken() != other.hasServiceToken()) return false;
if (hasServiceToken()) {
if (!getServiceToken()
.equals(other.getServiceToken())) return false;
}
if (hasCtime() != other.hasCtime()) return false;
if (hasCtime()) {
if (!getCtime()
.equals(other.getCtime())) return false;
}
if (hasMtime() != other.hasMtime()) return false;
if (hasMtime()) {
if (!getMtime()
.equals(other.getMtime())) return false;
}
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (!getId()
.equals(other.getId())) return false;
}
if (hasEditable() != other.hasEditable()) return false;
if (hasEditable()) {
if (!getEditable()
.equals(other.getEditable())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasService()) {
hash = (37 * hash) + SERVICE_FIELD_NUMBER;
hash = (53 * hash) + getService().hashCode();
}
if (hasNamespace()) {
hash = (37 * hash) + NAMESPACE_FIELD_NUMBER;
hash = (53 * hash) + getNamespace().hashCode();
}
if (hasAlias()) {
hash = (37 * hash) + ALIAS_FIELD_NUMBER;
hash = (53 * hash) + getAlias().hashCode();
}
if (hasAliasNamespace()) {
hash = (37 * hash) + ALIAS_NAMESPACE_FIELD_NUMBER;
hash = (53 * hash) + getAliasNamespace().hashCode();
}
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
if (hasOwners()) {
hash = (37 * hash) + OWNERS_FIELD_NUMBER;
hash = (53 * hash) + getOwners().hashCode();
}
if (hasComment()) {
hash = (37 * hash) + COMMENT_FIELD_NUMBER;
hash = (53 * hash) + getComment().hashCode();
}
if (hasServiceToken()) {
hash = (37 * hash) + SERVICE_TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getServiceToken().hashCode();
}
if (hasCtime()) {
hash = (37 * hash) + CTIME_FIELD_NUMBER;
hash = (53 * hash) + getCtime().hashCode();
}
if (hasMtime()) {
hash = (37 * hash) + MTIME_FIELD_NUMBER;
hash = (53 * hash) + getMtime().hashCode();
}
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasEditable()) {
hash = (37 * hash) + EDITABLE_FIELD_NUMBER;
hash = (53 * hash) + getEditable().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code v1.ServiceAlias}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:v1.ServiceAlias)
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAliasOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_ServiceAlias_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_ServiceAlias_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias.class, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias.Builder.class);
}
// Construct using com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
if (serviceBuilder_ == null) {
service_ = null;
} else {
service_ = null;
serviceBuilder_ = null;
}
if (namespaceBuilder_ == null) {
namespace_ = null;
} else {
namespace_ = null;
namespaceBuilder_ = null;
}
if (aliasBuilder_ == null) {
alias_ = null;
} else {
alias_ = null;
aliasBuilder_ = null;
}
if (aliasNamespaceBuilder_ == null) {
aliasNamespace_ = null;
} else {
aliasNamespace_ = null;
aliasNamespaceBuilder_ = null;
}
type_ = 0;
if (ownersBuilder_ == null) {
owners_ = null;
} else {
owners_ = null;
ownersBuilder_ = null;
}
if (commentBuilder_ == null) {
comment_ = null;
} else {
comment_ = null;
commentBuilder_ = null;
}
if (serviceTokenBuilder_ == null) {
serviceToken_ = null;
} else {
serviceToken_ = null;
serviceTokenBuilder_ = null;
}
if (ctimeBuilder_ == null) {
ctime_ = null;
} else {
ctime_ = null;
ctimeBuilder_ = null;
}
if (mtimeBuilder_ == null) {
mtime_ = null;
} else {
mtime_ = null;
mtimeBuilder_ = null;
}
if (idBuilder_ == null) {
id_ = null;
} else {
id_ = null;
idBuilder_ = null;
}
if (editableBuilder_ == null) {
editable_ = null;
} else {
editable_ = null;
editableBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_ServiceAlias_descriptor;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias getDefaultInstanceForType() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias build() {
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias buildPartial() {
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias result = new com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias(this);
if (serviceBuilder_ == null) {
result.service_ = service_;
} else {
result.service_ = serviceBuilder_.build();
}
if (namespaceBuilder_ == null) {
result.namespace_ = namespace_;
} else {
result.namespace_ = namespaceBuilder_.build();
}
if (aliasBuilder_ == null) {
result.alias_ = alias_;
} else {
result.alias_ = aliasBuilder_.build();
}
if (aliasNamespaceBuilder_ == null) {
result.aliasNamespace_ = aliasNamespace_;
} else {
result.aliasNamespace_ = aliasNamespaceBuilder_.build();
}
result.type_ = type_;
if (ownersBuilder_ == null) {
result.owners_ = owners_;
} else {
result.owners_ = ownersBuilder_.build();
}
if (commentBuilder_ == null) {
result.comment_ = comment_;
} else {
result.comment_ = commentBuilder_.build();
}
if (serviceTokenBuilder_ == null) {
result.serviceToken_ = serviceToken_;
} else {
result.serviceToken_ = serviceTokenBuilder_.build();
}
if (ctimeBuilder_ == null) {
result.ctime_ = ctime_;
} else {
result.ctime_ = ctimeBuilder_.build();
}
if (mtimeBuilder_ == null) {
result.mtime_ = mtime_;
} else {
result.mtime_ = mtimeBuilder_.build();
}
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (editableBuilder_ == null) {
result.editable_ = editable_;
} else {
result.editable_ = editableBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias) {
return mergeFrom((com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias other) {
if (other == com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias.getDefaultInstance()) return this;
if (other.hasService()) {
mergeService(other.getService());
}
if (other.hasNamespace()) {
mergeNamespace(other.getNamespace());
}
if (other.hasAlias()) {
mergeAlias(other.getAlias());
}
if (other.hasAliasNamespace()) {
mergeAliasNamespace(other.getAliasNamespace());
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.hasOwners()) {
mergeOwners(other.getOwners());
}
if (other.hasComment()) {
mergeComment(other.getComment());
}
if (other.hasServiceToken()) {
mergeServiceToken(other.getServiceToken());
}
if (other.hasCtime()) {
mergeCtime(other.getCtime());
}
if (other.hasMtime()) {
mergeMtime(other.getMtime());
}
if (other.hasId()) {
mergeId(other.getId());
}
if (other.hasEditable()) {
mergeEditable(other.getEditable());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getServiceFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 10
case 18: {
input.readMessage(
getNamespaceFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 18
case 26: {
input.readMessage(
getAliasFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 26
case 34: {
input.readMessage(
getAliasNamespaceFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 34
case 40: {
type_ = input.readEnum();
break;
} // case 40
case 50: {
input.readMessage(
getOwnersFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 50
case 58: {
input.readMessage(
getCommentFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 58
case 66: {
input.readMessage(
getServiceTokenFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 66
case 74: {
input.readMessage(
getCtimeFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 74
case 82: {
input.readMessage(
getMtimeFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 82
case 90: {
input.readMessage(
getIdFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 90
case 98: {
input.readMessage(
getEditableFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 98
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private com.google.protobuf.StringValue service_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> serviceBuilder_;
/**
* .google.protobuf.StringValue service = 1;
* @return Whether the service field is set.
*/
public boolean hasService() {
return serviceBuilder_ != null || service_ != null;
}
/**
* .google.protobuf.StringValue service = 1;
* @return The service.
*/
public com.google.protobuf.StringValue getService() {
if (serviceBuilder_ == null) {
return service_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : service_;
} else {
return serviceBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue service = 1;
*/
public Builder setService(com.google.protobuf.StringValue value) {
if (serviceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
service_ = value;
onChanged();
} else {
serviceBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue service = 1;
*/
public Builder setService(
com.google.protobuf.StringValue.Builder builderForValue) {
if (serviceBuilder_ == null) {
service_ = builderForValue.build();
onChanged();
} else {
serviceBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue service = 1;
*/
public Builder mergeService(com.google.protobuf.StringValue value) {
if (serviceBuilder_ == null) {
if (service_ != null) {
service_ =
com.google.protobuf.StringValue.newBuilder(service_).mergeFrom(value).buildPartial();
} else {
service_ = value;
}
onChanged();
} else {
serviceBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue service = 1;
*/
public Builder clearService() {
if (serviceBuilder_ == null) {
service_ = null;
onChanged();
} else {
service_ = null;
serviceBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue service = 1;
*/
public com.google.protobuf.StringValue.Builder getServiceBuilder() {
onChanged();
return getServiceFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue service = 1;
*/
public com.google.protobuf.StringValueOrBuilder getServiceOrBuilder() {
if (serviceBuilder_ != null) {
return serviceBuilder_.getMessageOrBuilder();
} else {
return service_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : service_;
}
}
/**
* .google.protobuf.StringValue service = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getServiceFieldBuilder() {
if (serviceBuilder_ == null) {
serviceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getService(),
getParentForChildren(),
isClean());
service_ = null;
}
return serviceBuilder_;
}
private com.google.protobuf.StringValue namespace_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> namespaceBuilder_;
/**
* .google.protobuf.StringValue namespace = 2;
* @return Whether the namespace field is set.
*/
public boolean hasNamespace() {
return namespaceBuilder_ != null || namespace_ != null;
}
/**
* .google.protobuf.StringValue namespace = 2;
* @return The namespace.
*/
public com.google.protobuf.StringValue getNamespace() {
if (namespaceBuilder_ == null) {
return namespace_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : namespace_;
} else {
return namespaceBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public Builder setNamespace(com.google.protobuf.StringValue value) {
if (namespaceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
namespace_ = value;
onChanged();
} else {
namespaceBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public Builder setNamespace(
com.google.protobuf.StringValue.Builder builderForValue) {
if (namespaceBuilder_ == null) {
namespace_ = builderForValue.build();
onChanged();
} else {
namespaceBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public Builder mergeNamespace(com.google.protobuf.StringValue value) {
if (namespaceBuilder_ == null) {
if (namespace_ != null) {
namespace_ =
com.google.protobuf.StringValue.newBuilder(namespace_).mergeFrom(value).buildPartial();
} else {
namespace_ = value;
}
onChanged();
} else {
namespaceBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public Builder clearNamespace() {
if (namespaceBuilder_ == null) {
namespace_ = null;
onChanged();
} else {
namespace_ = null;
namespaceBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public com.google.protobuf.StringValue.Builder getNamespaceBuilder() {
onChanged();
return getNamespaceFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
public com.google.protobuf.StringValueOrBuilder getNamespaceOrBuilder() {
if (namespaceBuilder_ != null) {
return namespaceBuilder_.getMessageOrBuilder();
} else {
return namespace_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : namespace_;
}
}
/**
* .google.protobuf.StringValue namespace = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getNamespaceFieldBuilder() {
if (namespaceBuilder_ == null) {
namespaceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getNamespace(),
getParentForChildren(),
isClean());
namespace_ = null;
}
return namespaceBuilder_;
}
private com.google.protobuf.StringValue alias_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> aliasBuilder_;
/**
* .google.protobuf.StringValue alias = 3;
* @return Whether the alias field is set.
*/
public boolean hasAlias() {
return aliasBuilder_ != null || alias_ != null;
}
/**
* .google.protobuf.StringValue alias = 3;
* @return The alias.
*/
public com.google.protobuf.StringValue getAlias() {
if (aliasBuilder_ == null) {
return alias_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : alias_;
} else {
return aliasBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue alias = 3;
*/
public Builder setAlias(com.google.protobuf.StringValue value) {
if (aliasBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
alias_ = value;
onChanged();
} else {
aliasBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue alias = 3;
*/
public Builder setAlias(
com.google.protobuf.StringValue.Builder builderForValue) {
if (aliasBuilder_ == null) {
alias_ = builderForValue.build();
onChanged();
} else {
aliasBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue alias = 3;
*/
public Builder mergeAlias(com.google.protobuf.StringValue value) {
if (aliasBuilder_ == null) {
if (alias_ != null) {
alias_ =
com.google.protobuf.StringValue.newBuilder(alias_).mergeFrom(value).buildPartial();
} else {
alias_ = value;
}
onChanged();
} else {
aliasBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue alias = 3;
*/
public Builder clearAlias() {
if (aliasBuilder_ == null) {
alias_ = null;
onChanged();
} else {
alias_ = null;
aliasBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue alias = 3;
*/
public com.google.protobuf.StringValue.Builder getAliasBuilder() {
onChanged();
return getAliasFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue alias = 3;
*/
public com.google.protobuf.StringValueOrBuilder getAliasOrBuilder() {
if (aliasBuilder_ != null) {
return aliasBuilder_.getMessageOrBuilder();
} else {
return alias_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : alias_;
}
}
/**
* .google.protobuf.StringValue alias = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getAliasFieldBuilder() {
if (aliasBuilder_ == null) {
aliasBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getAlias(),
getParentForChildren(),
isClean());
alias_ = null;
}
return aliasBuilder_;
}
private com.google.protobuf.StringValue aliasNamespace_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> aliasNamespaceBuilder_;
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
* @return Whether the aliasNamespace field is set.
*/
public boolean hasAliasNamespace() {
return aliasNamespaceBuilder_ != null || aliasNamespace_ != null;
}
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
* @return The aliasNamespace.
*/
public com.google.protobuf.StringValue getAliasNamespace() {
if (aliasNamespaceBuilder_ == null) {
return aliasNamespace_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : aliasNamespace_;
} else {
return aliasNamespaceBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
*/
public Builder setAliasNamespace(com.google.protobuf.StringValue value) {
if (aliasNamespaceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
aliasNamespace_ = value;
onChanged();
} else {
aliasNamespaceBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
*/
public Builder setAliasNamespace(
com.google.protobuf.StringValue.Builder builderForValue) {
if (aliasNamespaceBuilder_ == null) {
aliasNamespace_ = builderForValue.build();
onChanged();
} else {
aliasNamespaceBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
*/
public Builder mergeAliasNamespace(com.google.protobuf.StringValue value) {
if (aliasNamespaceBuilder_ == null) {
if (aliasNamespace_ != null) {
aliasNamespace_ =
com.google.protobuf.StringValue.newBuilder(aliasNamespace_).mergeFrom(value).buildPartial();
} else {
aliasNamespace_ = value;
}
onChanged();
} else {
aliasNamespaceBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
*/
public Builder clearAliasNamespace() {
if (aliasNamespaceBuilder_ == null) {
aliasNamespace_ = null;
onChanged();
} else {
aliasNamespace_ = null;
aliasNamespaceBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
*/
public com.google.protobuf.StringValue.Builder getAliasNamespaceBuilder() {
onChanged();
return getAliasNamespaceFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
*/
public com.google.protobuf.StringValueOrBuilder getAliasNamespaceOrBuilder() {
if (aliasNamespaceBuilder_ != null) {
return aliasNamespaceBuilder_.getMessageOrBuilder();
} else {
return aliasNamespace_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : aliasNamespace_;
}
}
/**
* .google.protobuf.StringValue alias_namespace = 4 [json_name = "alias_namespace"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getAliasNamespaceFieldBuilder() {
if (aliasNamespaceBuilder_ == null) {
aliasNamespaceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getAliasNamespace(),
getParentForChildren(),
isClean());
aliasNamespace_ = null;
}
return aliasNamespaceBuilder_;
}
private int type_ = 0;
/**
* .v1.AliasType type = 5;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .v1.AliasType type = 5;
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* .v1.AliasType type = 5;
* @return The type.
*/
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType getType() {
@SuppressWarnings("deprecation")
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType result = com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType.valueOf(type_);
return result == null ? com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType.UNRECOGNIZED : result;
}
/**
* .v1.AliasType type = 5;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.AliasType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* .v1.AliasType type = 5;
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private com.google.protobuf.StringValue owners_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> ownersBuilder_;
/**
* .google.protobuf.StringValue owners = 6;
* @return Whether the owners field is set.
*/
public boolean hasOwners() {
return ownersBuilder_ != null || owners_ != null;
}
/**
* .google.protobuf.StringValue owners = 6;
* @return The owners.
*/
public com.google.protobuf.StringValue getOwners() {
if (ownersBuilder_ == null) {
return owners_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : owners_;
} else {
return ownersBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue owners = 6;
*/
public Builder setOwners(com.google.protobuf.StringValue value) {
if (ownersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
owners_ = value;
onChanged();
} else {
ownersBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue owners = 6;
*/
public Builder setOwners(
com.google.protobuf.StringValue.Builder builderForValue) {
if (ownersBuilder_ == null) {
owners_ = builderForValue.build();
onChanged();
} else {
ownersBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue owners = 6;
*/
public Builder mergeOwners(com.google.protobuf.StringValue value) {
if (ownersBuilder_ == null) {
if (owners_ != null) {
owners_ =
com.google.protobuf.StringValue.newBuilder(owners_).mergeFrom(value).buildPartial();
} else {
owners_ = value;
}
onChanged();
} else {
ownersBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue owners = 6;
*/
public Builder clearOwners() {
if (ownersBuilder_ == null) {
owners_ = null;
onChanged();
} else {
owners_ = null;
ownersBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue owners = 6;
*/
public com.google.protobuf.StringValue.Builder getOwnersBuilder() {
onChanged();
return getOwnersFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue owners = 6;
*/
public com.google.protobuf.StringValueOrBuilder getOwnersOrBuilder() {
if (ownersBuilder_ != null) {
return ownersBuilder_.getMessageOrBuilder();
} else {
return owners_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : owners_;
}
}
/**
* .google.protobuf.StringValue owners = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getOwnersFieldBuilder() {
if (ownersBuilder_ == null) {
ownersBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getOwners(),
getParentForChildren(),
isClean());
owners_ = null;
}
return ownersBuilder_;
}
private com.google.protobuf.StringValue comment_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> commentBuilder_;
/**
* .google.protobuf.StringValue comment = 7;
* @return Whether the comment field is set.
*/
public boolean hasComment() {
return commentBuilder_ != null || comment_ != null;
}
/**
* .google.protobuf.StringValue comment = 7;
* @return The comment.
*/
public com.google.protobuf.StringValue getComment() {
if (commentBuilder_ == null) {
return comment_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : comment_;
} else {
return commentBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue comment = 7;
*/
public Builder setComment(com.google.protobuf.StringValue value) {
if (commentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
comment_ = value;
onChanged();
} else {
commentBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue comment = 7;
*/
public Builder setComment(
com.google.protobuf.StringValue.Builder builderForValue) {
if (commentBuilder_ == null) {
comment_ = builderForValue.build();
onChanged();
} else {
commentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue comment = 7;
*/
public Builder mergeComment(com.google.protobuf.StringValue value) {
if (commentBuilder_ == null) {
if (comment_ != null) {
comment_ =
com.google.protobuf.StringValue.newBuilder(comment_).mergeFrom(value).buildPartial();
} else {
comment_ = value;
}
onChanged();
} else {
commentBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue comment = 7;
*/
public Builder clearComment() {
if (commentBuilder_ == null) {
comment_ = null;
onChanged();
} else {
comment_ = null;
commentBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue comment = 7;
*/
public com.google.protobuf.StringValue.Builder getCommentBuilder() {
onChanged();
return getCommentFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue comment = 7;
*/
public com.google.protobuf.StringValueOrBuilder getCommentOrBuilder() {
if (commentBuilder_ != null) {
return commentBuilder_.getMessageOrBuilder();
} else {
return comment_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : comment_;
}
}
/**
* .google.protobuf.StringValue comment = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getCommentFieldBuilder() {
if (commentBuilder_ == null) {
commentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getComment(),
getParentForChildren(),
isClean());
comment_ = null;
}
return commentBuilder_;
}
private com.google.protobuf.StringValue serviceToken_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> serviceTokenBuilder_;
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
* @return Whether the serviceToken field is set.
*/
public boolean hasServiceToken() {
return serviceTokenBuilder_ != null || serviceToken_ != null;
}
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
* @return The serviceToken.
*/
public com.google.protobuf.StringValue getServiceToken() {
if (serviceTokenBuilder_ == null) {
return serviceToken_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : serviceToken_;
} else {
return serviceTokenBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
*/
public Builder setServiceToken(com.google.protobuf.StringValue value) {
if (serviceTokenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
serviceToken_ = value;
onChanged();
} else {
serviceTokenBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
*/
public Builder setServiceToken(
com.google.protobuf.StringValue.Builder builderForValue) {
if (serviceTokenBuilder_ == null) {
serviceToken_ = builderForValue.build();
onChanged();
} else {
serviceTokenBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
*/
public Builder mergeServiceToken(com.google.protobuf.StringValue value) {
if (serviceTokenBuilder_ == null) {
if (serviceToken_ != null) {
serviceToken_ =
com.google.protobuf.StringValue.newBuilder(serviceToken_).mergeFrom(value).buildPartial();
} else {
serviceToken_ = value;
}
onChanged();
} else {
serviceTokenBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
*/
public Builder clearServiceToken() {
if (serviceTokenBuilder_ == null) {
serviceToken_ = null;
onChanged();
} else {
serviceToken_ = null;
serviceTokenBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
*/
public com.google.protobuf.StringValue.Builder getServiceTokenBuilder() {
onChanged();
return getServiceTokenFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
*/
public com.google.protobuf.StringValueOrBuilder getServiceTokenOrBuilder() {
if (serviceTokenBuilder_ != null) {
return serviceTokenBuilder_.getMessageOrBuilder();
} else {
return serviceToken_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : serviceToken_;
}
}
/**
* .google.protobuf.StringValue service_token = 8 [json_name = "service_token"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getServiceTokenFieldBuilder() {
if (serviceTokenBuilder_ == null) {
serviceTokenBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getServiceToken(),
getParentForChildren(),
isClean());
serviceToken_ = null;
}
return serviceTokenBuilder_;
}
private com.google.protobuf.StringValue ctime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> ctimeBuilder_;
/**
* .google.protobuf.StringValue ctime = 9;
* @return Whether the ctime field is set.
*/
public boolean hasCtime() {
return ctimeBuilder_ != null || ctime_ != null;
}
/**
* .google.protobuf.StringValue ctime = 9;
* @return The ctime.
*/
public com.google.protobuf.StringValue getCtime() {
if (ctimeBuilder_ == null) {
return ctime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : ctime_;
} else {
return ctimeBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue ctime = 9;
*/
public Builder setCtime(com.google.protobuf.StringValue value) {
if (ctimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ctime_ = value;
onChanged();
} else {
ctimeBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 9;
*/
public Builder setCtime(
com.google.protobuf.StringValue.Builder builderForValue) {
if (ctimeBuilder_ == null) {
ctime_ = builderForValue.build();
onChanged();
} else {
ctimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 9;
*/
public Builder mergeCtime(com.google.protobuf.StringValue value) {
if (ctimeBuilder_ == null) {
if (ctime_ != null) {
ctime_ =
com.google.protobuf.StringValue.newBuilder(ctime_).mergeFrom(value).buildPartial();
} else {
ctime_ = value;
}
onChanged();
} else {
ctimeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 9;
*/
public Builder clearCtime() {
if (ctimeBuilder_ == null) {
ctime_ = null;
onChanged();
} else {
ctime_ = null;
ctimeBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 9;
*/
public com.google.protobuf.StringValue.Builder getCtimeBuilder() {
onChanged();
return getCtimeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue ctime = 9;
*/
public com.google.protobuf.StringValueOrBuilder getCtimeOrBuilder() {
if (ctimeBuilder_ != null) {
return ctimeBuilder_.getMessageOrBuilder();
} else {
return ctime_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : ctime_;
}
}
/**
* .google.protobuf.StringValue ctime = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getCtimeFieldBuilder() {
if (ctimeBuilder_ == null) {
ctimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getCtime(),
getParentForChildren(),
isClean());
ctime_ = null;
}
return ctimeBuilder_;
}
private com.google.protobuf.StringValue mtime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> mtimeBuilder_;
/**
* .google.protobuf.StringValue mtime = 10;
* @return Whether the mtime field is set.
*/
public boolean hasMtime() {
return mtimeBuilder_ != null || mtime_ != null;
}
/**
* .google.protobuf.StringValue mtime = 10;
* @return The mtime.
*/
public com.google.protobuf.StringValue getMtime() {
if (mtimeBuilder_ == null) {
return mtime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : mtime_;
} else {
return mtimeBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue mtime = 10;
*/
public Builder setMtime(com.google.protobuf.StringValue value) {
if (mtimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
mtime_ = value;
onChanged();
} else {
mtimeBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 10;
*/
public Builder setMtime(
com.google.protobuf.StringValue.Builder builderForValue) {
if (mtimeBuilder_ == null) {
mtime_ = builderForValue.build();
onChanged();
} else {
mtimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 10;
*/
public Builder mergeMtime(com.google.protobuf.StringValue value) {
if (mtimeBuilder_ == null) {
if (mtime_ != null) {
mtime_ =
com.google.protobuf.StringValue.newBuilder(mtime_).mergeFrom(value).buildPartial();
} else {
mtime_ = value;
}
onChanged();
} else {
mtimeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 10;
*/
public Builder clearMtime() {
if (mtimeBuilder_ == null) {
mtime_ = null;
onChanged();
} else {
mtime_ = null;
mtimeBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 10;
*/
public com.google.protobuf.StringValue.Builder getMtimeBuilder() {
onChanged();
return getMtimeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue mtime = 10;
*/
public com.google.protobuf.StringValueOrBuilder getMtimeOrBuilder() {
if (mtimeBuilder_ != null) {
return mtimeBuilder_.getMessageOrBuilder();
} else {
return mtime_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : mtime_;
}
}
/**
* .google.protobuf.StringValue mtime = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getMtimeFieldBuilder() {
if (mtimeBuilder_ == null) {
mtimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getMtime(),
getParentForChildren(),
isClean());
mtime_ = null;
}
return mtimeBuilder_;
}
private com.google.protobuf.StringValue id_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> idBuilder_;
/**
* .google.protobuf.StringValue id = 11;
* @return Whether the id field is set.
*/
public boolean hasId() {
return idBuilder_ != null || id_ != null;
}
/**
* .google.protobuf.StringValue id = 11;
* @return The id.
*/
public com.google.protobuf.StringValue getId() {
if (idBuilder_ == null) {
return id_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue id = 11;
*/
public Builder setId(com.google.protobuf.StringValue value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue id = 11;
*/
public Builder setId(
com.google.protobuf.StringValue.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue id = 11;
*/
public Builder mergeId(com.google.protobuf.StringValue value) {
if (idBuilder_ == null) {
if (id_ != null) {
id_ =
com.google.protobuf.StringValue.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue id = 11;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = null;
onChanged();
} else {
id_ = null;
idBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue id = 11;
*/
public com.google.protobuf.StringValue.Builder getIdBuilder() {
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue id = 11;
*/
public com.google.protobuf.StringValueOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : id_;
}
}
/**
* .google.protobuf.StringValue id = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getId(),
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
private com.google.protobuf.BoolValue editable_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder> editableBuilder_;
/**
* .google.protobuf.BoolValue editable = 12;
* @return Whether the editable field is set.
*/
public boolean hasEditable() {
return editableBuilder_ != null || editable_ != null;
}
/**
* .google.protobuf.BoolValue editable = 12;
* @return The editable.
*/
public com.google.protobuf.BoolValue getEditable() {
if (editableBuilder_ == null) {
return editable_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : editable_;
} else {
return editableBuilder_.getMessage();
}
}
/**
* .google.protobuf.BoolValue editable = 12;
*/
public Builder setEditable(com.google.protobuf.BoolValue value) {
if (editableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
editable_ = value;
onChanged();
} else {
editableBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.BoolValue editable = 12;
*/
public Builder setEditable(
com.google.protobuf.BoolValue.Builder builderForValue) {
if (editableBuilder_ == null) {
editable_ = builderForValue.build();
onChanged();
} else {
editableBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.BoolValue editable = 12;
*/
public Builder mergeEditable(com.google.protobuf.BoolValue value) {
if (editableBuilder_ == null) {
if (editable_ != null) {
editable_ =
com.google.protobuf.BoolValue.newBuilder(editable_).mergeFrom(value).buildPartial();
} else {
editable_ = value;
}
onChanged();
} else {
editableBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.BoolValue editable = 12;
*/
public Builder clearEditable() {
if (editableBuilder_ == null) {
editable_ = null;
onChanged();
} else {
editable_ = null;
editableBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.BoolValue editable = 12;
*/
public com.google.protobuf.BoolValue.Builder getEditableBuilder() {
onChanged();
return getEditableFieldBuilder().getBuilder();
}
/**
* .google.protobuf.BoolValue editable = 12;
*/
public com.google.protobuf.BoolValueOrBuilder getEditableOrBuilder() {
if (editableBuilder_ != null) {
return editableBuilder_.getMessageOrBuilder();
} else {
return editable_ == null ?
com.google.protobuf.BoolValue.getDefaultInstance() : editable_;
}
}
/**
* .google.protobuf.BoolValue editable = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>
getEditableFieldBuilder() {
if (editableBuilder_ == null) {
editableBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>(
getEditable(),
getParentForChildren(),
isClean());
editable_ = null;
}
return editableBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:v1.ServiceAlias)
}
// @@protoc_insertion_point(class_scope:v1.ServiceAlias)
private static final com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias();
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ServiceAlias parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.ServiceAlias getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InstanceOrBuilder extends
// @@protoc_insertion_point(interface_extends:v1.Instance)
com.google.protobuf.MessageOrBuilder {
/**
* .google.protobuf.StringValue id = 1;
* @return Whether the id field is set.
*/
boolean hasId();
/**
* .google.protobuf.StringValue id = 1;
* @return The id.
*/
com.google.protobuf.StringValue getId();
/**
* .google.protobuf.StringValue id = 1;
*/
com.google.protobuf.StringValueOrBuilder getIdOrBuilder();
/**
* .google.protobuf.StringValue service = 2;
* @return Whether the service field is set.
*/
boolean hasService();
/**
* .google.protobuf.StringValue service = 2;
* @return The service.
*/
com.google.protobuf.StringValue getService();
/**
* .google.protobuf.StringValue service = 2;
*/
com.google.protobuf.StringValueOrBuilder getServiceOrBuilder();
/**
* .google.protobuf.StringValue namespace = 3;
* @return Whether the namespace field is set.
*/
boolean hasNamespace();
/**
* .google.protobuf.StringValue namespace = 3;
* @return The namespace.
*/
com.google.protobuf.StringValue getNamespace();
/**
* .google.protobuf.StringValue namespace = 3;
*/
com.google.protobuf.StringValueOrBuilder getNamespaceOrBuilder();
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
* @return Whether the vpcId field is set.
*/
boolean hasVpcId();
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
* @return The vpcId.
*/
com.google.protobuf.StringValue getVpcId();
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
*/
com.google.protobuf.StringValueOrBuilder getVpcIdOrBuilder();
/**
* .google.protobuf.StringValue host = 4;
* @return Whether the host field is set.
*/
boolean hasHost();
/**
* .google.protobuf.StringValue host = 4;
* @return The host.
*/
com.google.protobuf.StringValue getHost();
/**
* .google.protobuf.StringValue host = 4;
*/
com.google.protobuf.StringValueOrBuilder getHostOrBuilder();
/**
* .google.protobuf.UInt32Value port = 5;
* @return Whether the port field is set.
*/
boolean hasPort();
/**
* .google.protobuf.UInt32Value port = 5;
* @return The port.
*/
com.google.protobuf.UInt32Value getPort();
/**
* .google.protobuf.UInt32Value port = 5;
*/
com.google.protobuf.UInt32ValueOrBuilder getPortOrBuilder();
/**
* .google.protobuf.StringValue protocol = 6;
* @return Whether the protocol field is set.
*/
boolean hasProtocol();
/**
* .google.protobuf.StringValue protocol = 6;
* @return The protocol.
*/
com.google.protobuf.StringValue getProtocol();
/**
* .google.protobuf.StringValue protocol = 6;
*/
com.google.protobuf.StringValueOrBuilder getProtocolOrBuilder();
/**
* .google.protobuf.StringValue version = 7;
* @return Whether the version field is set.
*/
boolean hasVersion();
/**
* .google.protobuf.StringValue version = 7;
* @return The version.
*/
com.google.protobuf.StringValue getVersion();
/**
* .google.protobuf.StringValue version = 7;
*/
com.google.protobuf.StringValueOrBuilder getVersionOrBuilder();
/**
* .google.protobuf.UInt32Value priority = 8;
* @return Whether the priority field is set.
*/
boolean hasPriority();
/**
* .google.protobuf.UInt32Value priority = 8;
* @return The priority.
*/
com.google.protobuf.UInt32Value getPriority();
/**
* .google.protobuf.UInt32Value priority = 8;
*/
com.google.protobuf.UInt32ValueOrBuilder getPriorityOrBuilder();
/**
* .google.protobuf.UInt32Value weight = 9;
* @return Whether the weight field is set.
*/
boolean hasWeight();
/**
* .google.protobuf.UInt32Value weight = 9;
* @return The weight.
*/
com.google.protobuf.UInt32Value getWeight();
/**
* .google.protobuf.UInt32Value weight = 9;
*/
com.google.protobuf.UInt32ValueOrBuilder getWeightOrBuilder();
/**
* .google.protobuf.BoolValue enable_health_check = 20;
* @return Whether the enableHealthCheck field is set.
*/
boolean hasEnableHealthCheck();
/**
* .google.protobuf.BoolValue enable_health_check = 20;
* @return The enableHealthCheck.
*/
com.google.protobuf.BoolValue getEnableHealthCheck();
/**
* .google.protobuf.BoolValue enable_health_check = 20;
*/
com.google.protobuf.BoolValueOrBuilder getEnableHealthCheckOrBuilder();
/**
* .v1.HealthCheck health_check = 10;
* @return Whether the healthCheck field is set.
*/
boolean hasHealthCheck();
/**
* .v1.HealthCheck health_check = 10;
* @return The healthCheck.
*/
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck getHealthCheck();
/**
* .v1.HealthCheck health_check = 10;
*/
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheckOrBuilder getHealthCheckOrBuilder();
/**
* .google.protobuf.BoolValue healthy = 11;
* @return Whether the healthy field is set.
*/
boolean hasHealthy();
/**
* .google.protobuf.BoolValue healthy = 11;
* @return The healthy.
*/
com.google.protobuf.BoolValue getHealthy();
/**
* .google.protobuf.BoolValue healthy = 11;
*/
com.google.protobuf.BoolValueOrBuilder getHealthyOrBuilder();
/**
* .google.protobuf.BoolValue isolate = 12;
* @return Whether the isolate field is set.
*/
boolean hasIsolate();
/**
* .google.protobuf.BoolValue isolate = 12;
* @return The isolate.
*/
com.google.protobuf.BoolValue getIsolate();
/**
* .google.protobuf.BoolValue isolate = 12;
*/
com.google.protobuf.BoolValueOrBuilder getIsolateOrBuilder();
/**
* .v1.Location location = 13;
* @return Whether the location field is set.
*/
boolean hasLocation();
/**
* .v1.Location location = 13;
* @return The location.
*/
com.tencent.polaris.specification.api.v1.model.ModelProto.Location getLocation();
/**
* .v1.Location location = 13;
*/
com.tencent.polaris.specification.api.v1.model.ModelProto.LocationOrBuilder getLocationOrBuilder();
/**
* map<string, string> metadata = 14;
*/
int getMetadataCount();
/**
* map<string, string> metadata = 14;
*/
boolean containsMetadata(
java.lang.String key);
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getMetadata();
/**
* map<string, string> metadata = 14;
*/
java.util.Map
getMetadataMap();
/**
* map<string, string> metadata = 14;
*/
/* nullable */
java.lang.String getMetadataOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
* map<string, string> metadata = 14;
*/
java.lang.String getMetadataOrThrow(
java.lang.String key);
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
* @return Whether the logicSet field is set.
*/
boolean hasLogicSet();
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
* @return The logicSet.
*/
com.google.protobuf.StringValue getLogicSet();
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
*/
com.google.protobuf.StringValueOrBuilder getLogicSetOrBuilder();
/**
* .google.protobuf.StringValue ctime = 16;
* @return Whether the ctime field is set.
*/
boolean hasCtime();
/**
* .google.protobuf.StringValue ctime = 16;
* @return The ctime.
*/
com.google.protobuf.StringValue getCtime();
/**
* .google.protobuf.StringValue ctime = 16;
*/
com.google.protobuf.StringValueOrBuilder getCtimeOrBuilder();
/**
* .google.protobuf.StringValue mtime = 17;
* @return Whether the mtime field is set.
*/
boolean hasMtime();
/**
* .google.protobuf.StringValue mtime = 17;
* @return The mtime.
*/
com.google.protobuf.StringValue getMtime();
/**
* .google.protobuf.StringValue mtime = 17;
*/
com.google.protobuf.StringValueOrBuilder getMtimeOrBuilder();
/**
* .google.protobuf.StringValue revision = 18;
* @return Whether the revision field is set.
*/
boolean hasRevision();
/**
* .google.protobuf.StringValue revision = 18;
* @return The revision.
*/
com.google.protobuf.StringValue getRevision();
/**
* .google.protobuf.StringValue revision = 18;
*/
com.google.protobuf.StringValueOrBuilder getRevisionOrBuilder();
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
* @return Whether the serviceToken field is set.
*/
boolean hasServiceToken();
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
* @return The serviceToken.
*/
com.google.protobuf.StringValue getServiceToken();
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
*/
com.google.protobuf.StringValueOrBuilder getServiceTokenOrBuilder();
}
/**
* Protobuf type {@code v1.Instance}
*/
public static final class Instance extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:v1.Instance)
InstanceOrBuilder {
private static final long serialVersionUID = 0L;
// Use Instance.newBuilder() to construct.
private Instance(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Instance() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Instance();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Instance_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 14:
return internalGetMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Instance_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance.class, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private com.google.protobuf.StringValue id_;
/**
* .google.protobuf.StringValue id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return id_ != null;
}
/**
* .google.protobuf.StringValue id = 1;
* @return The id.
*/
@java.lang.Override
public com.google.protobuf.StringValue getId() {
return id_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : id_;
}
/**
* .google.protobuf.StringValue id = 1;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getIdOrBuilder() {
return getId();
}
public static final int SERVICE_FIELD_NUMBER = 2;
private com.google.protobuf.StringValue service_;
/**
* .google.protobuf.StringValue service = 2;
* @return Whether the service field is set.
*/
@java.lang.Override
public boolean hasService() {
return service_ != null;
}
/**
* .google.protobuf.StringValue service = 2;
* @return The service.
*/
@java.lang.Override
public com.google.protobuf.StringValue getService() {
return service_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : service_;
}
/**
* .google.protobuf.StringValue service = 2;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getServiceOrBuilder() {
return getService();
}
public static final int NAMESPACE_FIELD_NUMBER = 3;
private com.google.protobuf.StringValue namespace_;
/**
* .google.protobuf.StringValue namespace = 3;
* @return Whether the namespace field is set.
*/
@java.lang.Override
public boolean hasNamespace() {
return namespace_ != null;
}
/**
* .google.protobuf.StringValue namespace = 3;
* @return The namespace.
*/
@java.lang.Override
public com.google.protobuf.StringValue getNamespace() {
return namespace_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : namespace_;
}
/**
* .google.protobuf.StringValue namespace = 3;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getNamespaceOrBuilder() {
return getNamespace();
}
public static final int VPC_ID_FIELD_NUMBER = 21;
private com.google.protobuf.StringValue vpcId_;
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
* @return Whether the vpcId field is set.
*/
@java.lang.Override
public boolean hasVpcId() {
return vpcId_ != null;
}
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
* @return The vpcId.
*/
@java.lang.Override
public com.google.protobuf.StringValue getVpcId() {
return vpcId_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : vpcId_;
}
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getVpcIdOrBuilder() {
return getVpcId();
}
public static final int HOST_FIELD_NUMBER = 4;
private com.google.protobuf.StringValue host_;
/**
* .google.protobuf.StringValue host = 4;
* @return Whether the host field is set.
*/
@java.lang.Override
public boolean hasHost() {
return host_ != null;
}
/**
* .google.protobuf.StringValue host = 4;
* @return The host.
*/
@java.lang.Override
public com.google.protobuf.StringValue getHost() {
return host_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : host_;
}
/**
* .google.protobuf.StringValue host = 4;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getHostOrBuilder() {
return getHost();
}
public static final int PORT_FIELD_NUMBER = 5;
private com.google.protobuf.UInt32Value port_;
/**
* .google.protobuf.UInt32Value port = 5;
* @return Whether the port field is set.
*/
@java.lang.Override
public boolean hasPort() {
return port_ != null;
}
/**
* .google.protobuf.UInt32Value port = 5;
* @return The port.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getPort() {
return port_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : port_;
}
/**
* .google.protobuf.UInt32Value port = 5;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getPortOrBuilder() {
return getPort();
}
public static final int PROTOCOL_FIELD_NUMBER = 6;
private com.google.protobuf.StringValue protocol_;
/**
* .google.protobuf.StringValue protocol = 6;
* @return Whether the protocol field is set.
*/
@java.lang.Override
public boolean hasProtocol() {
return protocol_ != null;
}
/**
* .google.protobuf.StringValue protocol = 6;
* @return The protocol.
*/
@java.lang.Override
public com.google.protobuf.StringValue getProtocol() {
return protocol_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : protocol_;
}
/**
* .google.protobuf.StringValue protocol = 6;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getProtocolOrBuilder() {
return getProtocol();
}
public static final int VERSION_FIELD_NUMBER = 7;
private com.google.protobuf.StringValue version_;
/**
* .google.protobuf.StringValue version = 7;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return version_ != null;
}
/**
* .google.protobuf.StringValue version = 7;
* @return The version.
*/
@java.lang.Override
public com.google.protobuf.StringValue getVersion() {
return version_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : version_;
}
/**
* .google.protobuf.StringValue version = 7;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getVersionOrBuilder() {
return getVersion();
}
public static final int PRIORITY_FIELD_NUMBER = 8;
private com.google.protobuf.UInt32Value priority_;
/**
* .google.protobuf.UInt32Value priority = 8;
* @return Whether the priority field is set.
*/
@java.lang.Override
public boolean hasPriority() {
return priority_ != null;
}
/**
* .google.protobuf.UInt32Value priority = 8;
* @return The priority.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getPriority() {
return priority_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : priority_;
}
/**
* .google.protobuf.UInt32Value priority = 8;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getPriorityOrBuilder() {
return getPriority();
}
public static final int WEIGHT_FIELD_NUMBER = 9;
private com.google.protobuf.UInt32Value weight_;
/**
* .google.protobuf.UInt32Value weight = 9;
* @return Whether the weight field is set.
*/
@java.lang.Override
public boolean hasWeight() {
return weight_ != null;
}
/**
* .google.protobuf.UInt32Value weight = 9;
* @return The weight.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getWeight() {
return weight_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : weight_;
}
/**
* .google.protobuf.UInt32Value weight = 9;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getWeightOrBuilder() {
return getWeight();
}
public static final int ENABLE_HEALTH_CHECK_FIELD_NUMBER = 20;
private com.google.protobuf.BoolValue enableHealthCheck_;
/**
* .google.protobuf.BoolValue enable_health_check = 20;
* @return Whether the enableHealthCheck field is set.
*/
@java.lang.Override
public boolean hasEnableHealthCheck() {
return enableHealthCheck_ != null;
}
/**
* .google.protobuf.BoolValue enable_health_check = 20;
* @return The enableHealthCheck.
*/
@java.lang.Override
public com.google.protobuf.BoolValue getEnableHealthCheck() {
return enableHealthCheck_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : enableHealthCheck_;
}
/**
* .google.protobuf.BoolValue enable_health_check = 20;
*/
@java.lang.Override
public com.google.protobuf.BoolValueOrBuilder getEnableHealthCheckOrBuilder() {
return getEnableHealthCheck();
}
public static final int HEALTH_CHECK_FIELD_NUMBER = 10;
private com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck healthCheck_;
/**
* .v1.HealthCheck health_check = 10;
* @return Whether the healthCheck field is set.
*/
@java.lang.Override
public boolean hasHealthCheck() {
return healthCheck_ != null;
}
/**
* .v1.HealthCheck health_check = 10;
* @return The healthCheck.
*/
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck getHealthCheck() {
return healthCheck_ == null ? com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.getDefaultInstance() : healthCheck_;
}
/**
* .v1.HealthCheck health_check = 10;
*/
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheckOrBuilder getHealthCheckOrBuilder() {
return getHealthCheck();
}
public static final int HEALTHY_FIELD_NUMBER = 11;
private com.google.protobuf.BoolValue healthy_;
/**
* .google.protobuf.BoolValue healthy = 11;
* @return Whether the healthy field is set.
*/
@java.lang.Override
public boolean hasHealthy() {
return healthy_ != null;
}
/**
* .google.protobuf.BoolValue healthy = 11;
* @return The healthy.
*/
@java.lang.Override
public com.google.protobuf.BoolValue getHealthy() {
return healthy_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : healthy_;
}
/**
* .google.protobuf.BoolValue healthy = 11;
*/
@java.lang.Override
public com.google.protobuf.BoolValueOrBuilder getHealthyOrBuilder() {
return getHealthy();
}
public static final int ISOLATE_FIELD_NUMBER = 12;
private com.google.protobuf.BoolValue isolate_;
/**
* .google.protobuf.BoolValue isolate = 12;
* @return Whether the isolate field is set.
*/
@java.lang.Override
public boolean hasIsolate() {
return isolate_ != null;
}
/**
* .google.protobuf.BoolValue isolate = 12;
* @return The isolate.
*/
@java.lang.Override
public com.google.protobuf.BoolValue getIsolate() {
return isolate_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : isolate_;
}
/**
* .google.protobuf.BoolValue isolate = 12;
*/
@java.lang.Override
public com.google.protobuf.BoolValueOrBuilder getIsolateOrBuilder() {
return getIsolate();
}
public static final int LOCATION_FIELD_NUMBER = 13;
private com.tencent.polaris.specification.api.v1.model.ModelProto.Location location_;
/**
* .v1.Location location = 13;
* @return Whether the location field is set.
*/
@java.lang.Override
public boolean hasLocation() {
return location_ != null;
}
/**
* .v1.Location location = 13;
* @return The location.
*/
@java.lang.Override
public com.tencent.polaris.specification.api.v1.model.ModelProto.Location getLocation() {
return location_ == null ? com.tencent.polaris.specification.api.v1.model.ModelProto.Location.getDefaultInstance() : location_;
}
/**
* .v1.Location location = 13;
*/
@java.lang.Override
public com.tencent.polaris.specification.api.v1.model.ModelProto.LocationOrBuilder getLocationOrBuilder() {
return getLocation();
}
public static final int METADATA_FIELD_NUMBER = 14;
private static final class MetadataDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Instance_MetadataEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> metadata_;
private com.google.protobuf.MapField
internalGetMetadata() {
if (metadata_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
return metadata_;
}
public int getMetadataCount() {
return internalGetMetadata().getMap().size();
}
/**
* map<string, string> metadata = 14;
*/
@java.lang.Override
public boolean containsMetadata(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetMetadata().getMap().containsKey(key);
}
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getMetadata() {
return getMetadataMap();
}
/**
* map<string, string> metadata = 14;
*/
@java.lang.Override
public java.util.Map getMetadataMap() {
return internalGetMetadata().getMap();
}
/**
* map<string, string> metadata = 14;
*/
@java.lang.Override
public java.lang.String getMetadataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetMetadata().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> metadata = 14;
*/
@java.lang.Override
public java.lang.String getMetadataOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetMetadata().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int LOGIC_SET_FIELD_NUMBER = 15;
private com.google.protobuf.StringValue logicSet_;
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
* @return Whether the logicSet field is set.
*/
@java.lang.Override
public boolean hasLogicSet() {
return logicSet_ != null;
}
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
* @return The logicSet.
*/
@java.lang.Override
public com.google.protobuf.StringValue getLogicSet() {
return logicSet_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : logicSet_;
}
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getLogicSetOrBuilder() {
return getLogicSet();
}
public static final int CTIME_FIELD_NUMBER = 16;
private com.google.protobuf.StringValue ctime_;
/**
* .google.protobuf.StringValue ctime = 16;
* @return Whether the ctime field is set.
*/
@java.lang.Override
public boolean hasCtime() {
return ctime_ != null;
}
/**
* .google.protobuf.StringValue ctime = 16;
* @return The ctime.
*/
@java.lang.Override
public com.google.protobuf.StringValue getCtime() {
return ctime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : ctime_;
}
/**
* .google.protobuf.StringValue ctime = 16;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getCtimeOrBuilder() {
return getCtime();
}
public static final int MTIME_FIELD_NUMBER = 17;
private com.google.protobuf.StringValue mtime_;
/**
* .google.protobuf.StringValue mtime = 17;
* @return Whether the mtime field is set.
*/
@java.lang.Override
public boolean hasMtime() {
return mtime_ != null;
}
/**
* .google.protobuf.StringValue mtime = 17;
* @return The mtime.
*/
@java.lang.Override
public com.google.protobuf.StringValue getMtime() {
return mtime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : mtime_;
}
/**
* .google.protobuf.StringValue mtime = 17;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getMtimeOrBuilder() {
return getMtime();
}
public static final int REVISION_FIELD_NUMBER = 18;
private com.google.protobuf.StringValue revision_;
/**
* .google.protobuf.StringValue revision = 18;
* @return Whether the revision field is set.
*/
@java.lang.Override
public boolean hasRevision() {
return revision_ != null;
}
/**
* .google.protobuf.StringValue revision = 18;
* @return The revision.
*/
@java.lang.Override
public com.google.protobuf.StringValue getRevision() {
return revision_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : revision_;
}
/**
* .google.protobuf.StringValue revision = 18;
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getRevisionOrBuilder() {
return getRevision();
}
public static final int SERVICE_TOKEN_FIELD_NUMBER = 19;
private com.google.protobuf.StringValue serviceToken_;
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
* @return Whether the serviceToken field is set.
*/
@java.lang.Override
public boolean hasServiceToken() {
return serviceToken_ != null;
}
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
* @return The serviceToken.
*/
@java.lang.Override
public com.google.protobuf.StringValue getServiceToken() {
return serviceToken_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : serviceToken_;
}
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
*/
@java.lang.Override
public com.google.protobuf.StringValueOrBuilder getServiceTokenOrBuilder() {
return getServiceToken();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (id_ != null) {
output.writeMessage(1, getId());
}
if (service_ != null) {
output.writeMessage(2, getService());
}
if (namespace_ != null) {
output.writeMessage(3, getNamespace());
}
if (host_ != null) {
output.writeMessage(4, getHost());
}
if (port_ != null) {
output.writeMessage(5, getPort());
}
if (protocol_ != null) {
output.writeMessage(6, getProtocol());
}
if (version_ != null) {
output.writeMessage(7, getVersion());
}
if (priority_ != null) {
output.writeMessage(8, getPriority());
}
if (weight_ != null) {
output.writeMessage(9, getWeight());
}
if (healthCheck_ != null) {
output.writeMessage(10, getHealthCheck());
}
if (healthy_ != null) {
output.writeMessage(11, getHealthy());
}
if (isolate_ != null) {
output.writeMessage(12, getIsolate());
}
if (location_ != null) {
output.writeMessage(13, getLocation());
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetMetadata(),
MetadataDefaultEntryHolder.defaultEntry,
14);
if (logicSet_ != null) {
output.writeMessage(15, getLogicSet());
}
if (ctime_ != null) {
output.writeMessage(16, getCtime());
}
if (mtime_ != null) {
output.writeMessage(17, getMtime());
}
if (revision_ != null) {
output.writeMessage(18, getRevision());
}
if (serviceToken_ != null) {
output.writeMessage(19, getServiceToken());
}
if (enableHealthCheck_ != null) {
output.writeMessage(20, getEnableHealthCheck());
}
if (vpcId_ != null) {
output.writeMessage(21, getVpcId());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (id_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getId());
}
if (service_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getService());
}
if (namespace_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getNamespace());
}
if (host_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getHost());
}
if (port_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getPort());
}
if (protocol_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getProtocol());
}
if (version_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getVersion());
}
if (priority_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getPriority());
}
if (weight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getWeight());
}
if (healthCheck_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getHealthCheck());
}
if (healthy_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getHealthy());
}
if (isolate_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getIsolate());
}
if (location_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getLocation());
}
for (java.util.Map.Entry entry
: internalGetMetadata().getMap().entrySet()) {
com.google.protobuf.MapEntry
metadata__ = MetadataDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, metadata__);
}
if (logicSet_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, getLogicSet());
}
if (ctime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, getCtime());
}
if (mtime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(17, getMtime());
}
if (revision_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, getRevision());
}
if (serviceToken_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(19, getServiceToken());
}
if (enableHealthCheck_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, getEnableHealthCheck());
}
if (vpcId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, getVpcId());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance)) {
return super.equals(obj);
}
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance other = (com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance) obj;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (!getId()
.equals(other.getId())) return false;
}
if (hasService() != other.hasService()) return false;
if (hasService()) {
if (!getService()
.equals(other.getService())) return false;
}
if (hasNamespace() != other.hasNamespace()) return false;
if (hasNamespace()) {
if (!getNamespace()
.equals(other.getNamespace())) return false;
}
if (hasVpcId() != other.hasVpcId()) return false;
if (hasVpcId()) {
if (!getVpcId()
.equals(other.getVpcId())) return false;
}
if (hasHost() != other.hasHost()) return false;
if (hasHost()) {
if (!getHost()
.equals(other.getHost())) return false;
}
if (hasPort() != other.hasPort()) return false;
if (hasPort()) {
if (!getPort()
.equals(other.getPort())) return false;
}
if (hasProtocol() != other.hasProtocol()) return false;
if (hasProtocol()) {
if (!getProtocol()
.equals(other.getProtocol())) return false;
}
if (hasVersion() != other.hasVersion()) return false;
if (hasVersion()) {
if (!getVersion()
.equals(other.getVersion())) return false;
}
if (hasPriority() != other.hasPriority()) return false;
if (hasPriority()) {
if (!getPriority()
.equals(other.getPriority())) return false;
}
if (hasWeight() != other.hasWeight()) return false;
if (hasWeight()) {
if (!getWeight()
.equals(other.getWeight())) return false;
}
if (hasEnableHealthCheck() != other.hasEnableHealthCheck()) return false;
if (hasEnableHealthCheck()) {
if (!getEnableHealthCheck()
.equals(other.getEnableHealthCheck())) return false;
}
if (hasHealthCheck() != other.hasHealthCheck()) return false;
if (hasHealthCheck()) {
if (!getHealthCheck()
.equals(other.getHealthCheck())) return false;
}
if (hasHealthy() != other.hasHealthy()) return false;
if (hasHealthy()) {
if (!getHealthy()
.equals(other.getHealthy())) return false;
}
if (hasIsolate() != other.hasIsolate()) return false;
if (hasIsolate()) {
if (!getIsolate()
.equals(other.getIsolate())) return false;
}
if (hasLocation() != other.hasLocation()) return false;
if (hasLocation()) {
if (!getLocation()
.equals(other.getLocation())) return false;
}
if (!internalGetMetadata().equals(
other.internalGetMetadata())) return false;
if (hasLogicSet() != other.hasLogicSet()) return false;
if (hasLogicSet()) {
if (!getLogicSet()
.equals(other.getLogicSet())) return false;
}
if (hasCtime() != other.hasCtime()) return false;
if (hasCtime()) {
if (!getCtime()
.equals(other.getCtime())) return false;
}
if (hasMtime() != other.hasMtime()) return false;
if (hasMtime()) {
if (!getMtime()
.equals(other.getMtime())) return false;
}
if (hasRevision() != other.hasRevision()) return false;
if (hasRevision()) {
if (!getRevision()
.equals(other.getRevision())) return false;
}
if (hasServiceToken() != other.hasServiceToken()) return false;
if (hasServiceToken()) {
if (!getServiceToken()
.equals(other.getServiceToken())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasService()) {
hash = (37 * hash) + SERVICE_FIELD_NUMBER;
hash = (53 * hash) + getService().hashCode();
}
if (hasNamespace()) {
hash = (37 * hash) + NAMESPACE_FIELD_NUMBER;
hash = (53 * hash) + getNamespace().hashCode();
}
if (hasVpcId()) {
hash = (37 * hash) + VPC_ID_FIELD_NUMBER;
hash = (53 * hash) + getVpcId().hashCode();
}
if (hasHost()) {
hash = (37 * hash) + HOST_FIELD_NUMBER;
hash = (53 * hash) + getHost().hashCode();
}
if (hasPort()) {
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort().hashCode();
}
if (hasProtocol()) {
hash = (37 * hash) + PROTOCOL_FIELD_NUMBER;
hash = (53 * hash) + getProtocol().hashCode();
}
if (hasVersion()) {
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion().hashCode();
}
if (hasPriority()) {
hash = (37 * hash) + PRIORITY_FIELD_NUMBER;
hash = (53 * hash) + getPriority().hashCode();
}
if (hasWeight()) {
hash = (37 * hash) + WEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getWeight().hashCode();
}
if (hasEnableHealthCheck()) {
hash = (37 * hash) + ENABLE_HEALTH_CHECK_FIELD_NUMBER;
hash = (53 * hash) + getEnableHealthCheck().hashCode();
}
if (hasHealthCheck()) {
hash = (37 * hash) + HEALTH_CHECK_FIELD_NUMBER;
hash = (53 * hash) + getHealthCheck().hashCode();
}
if (hasHealthy()) {
hash = (37 * hash) + HEALTHY_FIELD_NUMBER;
hash = (53 * hash) + getHealthy().hashCode();
}
if (hasIsolate()) {
hash = (37 * hash) + ISOLATE_FIELD_NUMBER;
hash = (53 * hash) + getIsolate().hashCode();
}
if (hasLocation()) {
hash = (37 * hash) + LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getLocation().hashCode();
}
if (!internalGetMetadata().getMap().isEmpty()) {
hash = (37 * hash) + METADATA_FIELD_NUMBER;
hash = (53 * hash) + internalGetMetadata().hashCode();
}
if (hasLogicSet()) {
hash = (37 * hash) + LOGIC_SET_FIELD_NUMBER;
hash = (53 * hash) + getLogicSet().hashCode();
}
if (hasCtime()) {
hash = (37 * hash) + CTIME_FIELD_NUMBER;
hash = (53 * hash) + getCtime().hashCode();
}
if (hasMtime()) {
hash = (37 * hash) + MTIME_FIELD_NUMBER;
hash = (53 * hash) + getMtime().hashCode();
}
if (hasRevision()) {
hash = (37 * hash) + REVISION_FIELD_NUMBER;
hash = (53 * hash) + getRevision().hashCode();
}
if (hasServiceToken()) {
hash = (37 * hash) + SERVICE_TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getServiceToken().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code v1.Instance}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:v1.Instance)
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.InstanceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Instance_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 14:
return internalGetMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 14:
return internalGetMutableMetadata();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Instance_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance.class, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance.Builder.class);
}
// Construct using com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
if (idBuilder_ == null) {
id_ = null;
} else {
id_ = null;
idBuilder_ = null;
}
if (serviceBuilder_ == null) {
service_ = null;
} else {
service_ = null;
serviceBuilder_ = null;
}
if (namespaceBuilder_ == null) {
namespace_ = null;
} else {
namespace_ = null;
namespaceBuilder_ = null;
}
if (vpcIdBuilder_ == null) {
vpcId_ = null;
} else {
vpcId_ = null;
vpcIdBuilder_ = null;
}
if (hostBuilder_ == null) {
host_ = null;
} else {
host_ = null;
hostBuilder_ = null;
}
if (portBuilder_ == null) {
port_ = null;
} else {
port_ = null;
portBuilder_ = null;
}
if (protocolBuilder_ == null) {
protocol_ = null;
} else {
protocol_ = null;
protocolBuilder_ = null;
}
if (versionBuilder_ == null) {
version_ = null;
} else {
version_ = null;
versionBuilder_ = null;
}
if (priorityBuilder_ == null) {
priority_ = null;
} else {
priority_ = null;
priorityBuilder_ = null;
}
if (weightBuilder_ == null) {
weight_ = null;
} else {
weight_ = null;
weightBuilder_ = null;
}
if (enableHealthCheckBuilder_ == null) {
enableHealthCheck_ = null;
} else {
enableHealthCheck_ = null;
enableHealthCheckBuilder_ = null;
}
if (healthCheckBuilder_ == null) {
healthCheck_ = null;
} else {
healthCheck_ = null;
healthCheckBuilder_ = null;
}
if (healthyBuilder_ == null) {
healthy_ = null;
} else {
healthy_ = null;
healthyBuilder_ = null;
}
if (isolateBuilder_ == null) {
isolate_ = null;
} else {
isolate_ = null;
isolateBuilder_ = null;
}
if (locationBuilder_ == null) {
location_ = null;
} else {
location_ = null;
locationBuilder_ = null;
}
internalGetMutableMetadata().clear();
if (logicSetBuilder_ == null) {
logicSet_ = null;
} else {
logicSet_ = null;
logicSetBuilder_ = null;
}
if (ctimeBuilder_ == null) {
ctime_ = null;
} else {
ctime_ = null;
ctimeBuilder_ = null;
}
if (mtimeBuilder_ == null) {
mtime_ = null;
} else {
mtime_ = null;
mtimeBuilder_ = null;
}
if (revisionBuilder_ == null) {
revision_ = null;
} else {
revision_ = null;
revisionBuilder_ = null;
}
if (serviceTokenBuilder_ == null) {
serviceToken_ = null;
} else {
serviceToken_ = null;
serviceTokenBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_Instance_descriptor;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance getDefaultInstanceForType() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance build() {
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance buildPartial() {
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance result = new com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance(this);
int from_bitField0_ = bitField0_;
if (idBuilder_ == null) {
result.id_ = id_;
} else {
result.id_ = idBuilder_.build();
}
if (serviceBuilder_ == null) {
result.service_ = service_;
} else {
result.service_ = serviceBuilder_.build();
}
if (namespaceBuilder_ == null) {
result.namespace_ = namespace_;
} else {
result.namespace_ = namespaceBuilder_.build();
}
if (vpcIdBuilder_ == null) {
result.vpcId_ = vpcId_;
} else {
result.vpcId_ = vpcIdBuilder_.build();
}
if (hostBuilder_ == null) {
result.host_ = host_;
} else {
result.host_ = hostBuilder_.build();
}
if (portBuilder_ == null) {
result.port_ = port_;
} else {
result.port_ = portBuilder_.build();
}
if (protocolBuilder_ == null) {
result.protocol_ = protocol_;
} else {
result.protocol_ = protocolBuilder_.build();
}
if (versionBuilder_ == null) {
result.version_ = version_;
} else {
result.version_ = versionBuilder_.build();
}
if (priorityBuilder_ == null) {
result.priority_ = priority_;
} else {
result.priority_ = priorityBuilder_.build();
}
if (weightBuilder_ == null) {
result.weight_ = weight_;
} else {
result.weight_ = weightBuilder_.build();
}
if (enableHealthCheckBuilder_ == null) {
result.enableHealthCheck_ = enableHealthCheck_;
} else {
result.enableHealthCheck_ = enableHealthCheckBuilder_.build();
}
if (healthCheckBuilder_ == null) {
result.healthCheck_ = healthCheck_;
} else {
result.healthCheck_ = healthCheckBuilder_.build();
}
if (healthyBuilder_ == null) {
result.healthy_ = healthy_;
} else {
result.healthy_ = healthyBuilder_.build();
}
if (isolateBuilder_ == null) {
result.isolate_ = isolate_;
} else {
result.isolate_ = isolateBuilder_.build();
}
if (locationBuilder_ == null) {
result.location_ = location_;
} else {
result.location_ = locationBuilder_.build();
}
result.metadata_ = internalGetMetadata();
result.metadata_.makeImmutable();
if (logicSetBuilder_ == null) {
result.logicSet_ = logicSet_;
} else {
result.logicSet_ = logicSetBuilder_.build();
}
if (ctimeBuilder_ == null) {
result.ctime_ = ctime_;
} else {
result.ctime_ = ctimeBuilder_.build();
}
if (mtimeBuilder_ == null) {
result.mtime_ = mtime_;
} else {
result.mtime_ = mtimeBuilder_.build();
}
if (revisionBuilder_ == null) {
result.revision_ = revision_;
} else {
result.revision_ = revisionBuilder_.build();
}
if (serviceTokenBuilder_ == null) {
result.serviceToken_ = serviceToken_;
} else {
result.serviceToken_ = serviceTokenBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance) {
return mergeFrom((com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance other) {
if (other == com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance.getDefaultInstance()) return this;
if (other.hasId()) {
mergeId(other.getId());
}
if (other.hasService()) {
mergeService(other.getService());
}
if (other.hasNamespace()) {
mergeNamespace(other.getNamespace());
}
if (other.hasVpcId()) {
mergeVpcId(other.getVpcId());
}
if (other.hasHost()) {
mergeHost(other.getHost());
}
if (other.hasPort()) {
mergePort(other.getPort());
}
if (other.hasProtocol()) {
mergeProtocol(other.getProtocol());
}
if (other.hasVersion()) {
mergeVersion(other.getVersion());
}
if (other.hasPriority()) {
mergePriority(other.getPriority());
}
if (other.hasWeight()) {
mergeWeight(other.getWeight());
}
if (other.hasEnableHealthCheck()) {
mergeEnableHealthCheck(other.getEnableHealthCheck());
}
if (other.hasHealthCheck()) {
mergeHealthCheck(other.getHealthCheck());
}
if (other.hasHealthy()) {
mergeHealthy(other.getHealthy());
}
if (other.hasIsolate()) {
mergeIsolate(other.getIsolate());
}
if (other.hasLocation()) {
mergeLocation(other.getLocation());
}
internalGetMutableMetadata().mergeFrom(
other.internalGetMetadata());
if (other.hasLogicSet()) {
mergeLogicSet(other.getLogicSet());
}
if (other.hasCtime()) {
mergeCtime(other.getCtime());
}
if (other.hasMtime()) {
mergeMtime(other.getMtime());
}
if (other.hasRevision()) {
mergeRevision(other.getRevision());
}
if (other.hasServiceToken()) {
mergeServiceToken(other.getServiceToken());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getIdFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 10
case 18: {
input.readMessage(
getServiceFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 18
case 26: {
input.readMessage(
getNamespaceFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 26
case 34: {
input.readMessage(
getHostFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 34
case 42: {
input.readMessage(
getPortFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 42
case 50: {
input.readMessage(
getProtocolFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 50
case 58: {
input.readMessage(
getVersionFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 58
case 66: {
input.readMessage(
getPriorityFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 66
case 74: {
input.readMessage(
getWeightFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 74
case 82: {
input.readMessage(
getHealthCheckFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 82
case 90: {
input.readMessage(
getHealthyFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 90
case 98: {
input.readMessage(
getIsolateFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 98
case 106: {
input.readMessage(
getLocationFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 106
case 114: {
com.google.protobuf.MapEntry
metadata__ = input.readMessage(
MetadataDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableMetadata().getMutableMap().put(
metadata__.getKey(), metadata__.getValue());
break;
} // case 114
case 122: {
input.readMessage(
getLogicSetFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 122
case 130: {
input.readMessage(
getCtimeFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 130
case 138: {
input.readMessage(
getMtimeFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 138
case 146: {
input.readMessage(
getRevisionFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 146
case 154: {
input.readMessage(
getServiceTokenFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 154
case 162: {
input.readMessage(
getEnableHealthCheckFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 162
case 170: {
input.readMessage(
getVpcIdFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 170
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.StringValue id_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> idBuilder_;
/**
* .google.protobuf.StringValue id = 1;
* @return Whether the id field is set.
*/
public boolean hasId() {
return idBuilder_ != null || id_ != null;
}
/**
* .google.protobuf.StringValue id = 1;
* @return The id.
*/
public com.google.protobuf.StringValue getId() {
if (idBuilder_ == null) {
return id_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : id_;
} else {
return idBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue id = 1;
*/
public Builder setId(com.google.protobuf.StringValue value) {
if (idBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
} else {
idBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue id = 1;
*/
public Builder setId(
com.google.protobuf.StringValue.Builder builderForValue) {
if (idBuilder_ == null) {
id_ = builderForValue.build();
onChanged();
} else {
idBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue id = 1;
*/
public Builder mergeId(com.google.protobuf.StringValue value) {
if (idBuilder_ == null) {
if (id_ != null) {
id_ =
com.google.protobuf.StringValue.newBuilder(id_).mergeFrom(value).buildPartial();
} else {
id_ = value;
}
onChanged();
} else {
idBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue id = 1;
*/
public Builder clearId() {
if (idBuilder_ == null) {
id_ = null;
onChanged();
} else {
id_ = null;
idBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue id = 1;
*/
public com.google.protobuf.StringValue.Builder getIdBuilder() {
onChanged();
return getIdFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue id = 1;
*/
public com.google.protobuf.StringValueOrBuilder getIdOrBuilder() {
if (idBuilder_ != null) {
return idBuilder_.getMessageOrBuilder();
} else {
return id_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : id_;
}
}
/**
* .google.protobuf.StringValue id = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getIdFieldBuilder() {
if (idBuilder_ == null) {
idBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getId(),
getParentForChildren(),
isClean());
id_ = null;
}
return idBuilder_;
}
private com.google.protobuf.StringValue service_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> serviceBuilder_;
/**
* .google.protobuf.StringValue service = 2;
* @return Whether the service field is set.
*/
public boolean hasService() {
return serviceBuilder_ != null || service_ != null;
}
/**
* .google.protobuf.StringValue service = 2;
* @return The service.
*/
public com.google.protobuf.StringValue getService() {
if (serviceBuilder_ == null) {
return service_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : service_;
} else {
return serviceBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue service = 2;
*/
public Builder setService(com.google.protobuf.StringValue value) {
if (serviceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
service_ = value;
onChanged();
} else {
serviceBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue service = 2;
*/
public Builder setService(
com.google.protobuf.StringValue.Builder builderForValue) {
if (serviceBuilder_ == null) {
service_ = builderForValue.build();
onChanged();
} else {
serviceBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue service = 2;
*/
public Builder mergeService(com.google.protobuf.StringValue value) {
if (serviceBuilder_ == null) {
if (service_ != null) {
service_ =
com.google.protobuf.StringValue.newBuilder(service_).mergeFrom(value).buildPartial();
} else {
service_ = value;
}
onChanged();
} else {
serviceBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue service = 2;
*/
public Builder clearService() {
if (serviceBuilder_ == null) {
service_ = null;
onChanged();
} else {
service_ = null;
serviceBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue service = 2;
*/
public com.google.protobuf.StringValue.Builder getServiceBuilder() {
onChanged();
return getServiceFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue service = 2;
*/
public com.google.protobuf.StringValueOrBuilder getServiceOrBuilder() {
if (serviceBuilder_ != null) {
return serviceBuilder_.getMessageOrBuilder();
} else {
return service_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : service_;
}
}
/**
* .google.protobuf.StringValue service = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getServiceFieldBuilder() {
if (serviceBuilder_ == null) {
serviceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getService(),
getParentForChildren(),
isClean());
service_ = null;
}
return serviceBuilder_;
}
private com.google.protobuf.StringValue namespace_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> namespaceBuilder_;
/**
* .google.protobuf.StringValue namespace = 3;
* @return Whether the namespace field is set.
*/
public boolean hasNamespace() {
return namespaceBuilder_ != null || namespace_ != null;
}
/**
* .google.protobuf.StringValue namespace = 3;
* @return The namespace.
*/
public com.google.protobuf.StringValue getNamespace() {
if (namespaceBuilder_ == null) {
return namespace_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : namespace_;
} else {
return namespaceBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue namespace = 3;
*/
public Builder setNamespace(com.google.protobuf.StringValue value) {
if (namespaceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
namespace_ = value;
onChanged();
} else {
namespaceBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 3;
*/
public Builder setNamespace(
com.google.protobuf.StringValue.Builder builderForValue) {
if (namespaceBuilder_ == null) {
namespace_ = builderForValue.build();
onChanged();
} else {
namespaceBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 3;
*/
public Builder mergeNamespace(com.google.protobuf.StringValue value) {
if (namespaceBuilder_ == null) {
if (namespace_ != null) {
namespace_ =
com.google.protobuf.StringValue.newBuilder(namespace_).mergeFrom(value).buildPartial();
} else {
namespace_ = value;
}
onChanged();
} else {
namespaceBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 3;
*/
public Builder clearNamespace() {
if (namespaceBuilder_ == null) {
namespace_ = null;
onChanged();
} else {
namespace_ = null;
namespaceBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue namespace = 3;
*/
public com.google.protobuf.StringValue.Builder getNamespaceBuilder() {
onChanged();
return getNamespaceFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue namespace = 3;
*/
public com.google.protobuf.StringValueOrBuilder getNamespaceOrBuilder() {
if (namespaceBuilder_ != null) {
return namespaceBuilder_.getMessageOrBuilder();
} else {
return namespace_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : namespace_;
}
}
/**
* .google.protobuf.StringValue namespace = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getNamespaceFieldBuilder() {
if (namespaceBuilder_ == null) {
namespaceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getNamespace(),
getParentForChildren(),
isClean());
namespace_ = null;
}
return namespaceBuilder_;
}
private com.google.protobuf.StringValue vpcId_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> vpcIdBuilder_;
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
* @return Whether the vpcId field is set.
*/
public boolean hasVpcId() {
return vpcIdBuilder_ != null || vpcId_ != null;
}
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
* @return The vpcId.
*/
public com.google.protobuf.StringValue getVpcId() {
if (vpcIdBuilder_ == null) {
return vpcId_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : vpcId_;
} else {
return vpcIdBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
*/
public Builder setVpcId(com.google.protobuf.StringValue value) {
if (vpcIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
vpcId_ = value;
onChanged();
} else {
vpcIdBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
*/
public Builder setVpcId(
com.google.protobuf.StringValue.Builder builderForValue) {
if (vpcIdBuilder_ == null) {
vpcId_ = builderForValue.build();
onChanged();
} else {
vpcIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
*/
public Builder mergeVpcId(com.google.protobuf.StringValue value) {
if (vpcIdBuilder_ == null) {
if (vpcId_ != null) {
vpcId_ =
com.google.protobuf.StringValue.newBuilder(vpcId_).mergeFrom(value).buildPartial();
} else {
vpcId_ = value;
}
onChanged();
} else {
vpcIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
*/
public Builder clearVpcId() {
if (vpcIdBuilder_ == null) {
vpcId_ = null;
onChanged();
} else {
vpcId_ = null;
vpcIdBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
*/
public com.google.protobuf.StringValue.Builder getVpcIdBuilder() {
onChanged();
return getVpcIdFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
*/
public com.google.protobuf.StringValueOrBuilder getVpcIdOrBuilder() {
if (vpcIdBuilder_ != null) {
return vpcIdBuilder_.getMessageOrBuilder();
} else {
return vpcId_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : vpcId_;
}
}
/**
* .google.protobuf.StringValue vpc_id = 21 [json_name = "vpc_id"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getVpcIdFieldBuilder() {
if (vpcIdBuilder_ == null) {
vpcIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getVpcId(),
getParentForChildren(),
isClean());
vpcId_ = null;
}
return vpcIdBuilder_;
}
private com.google.protobuf.StringValue host_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> hostBuilder_;
/**
* .google.protobuf.StringValue host = 4;
* @return Whether the host field is set.
*/
public boolean hasHost() {
return hostBuilder_ != null || host_ != null;
}
/**
* .google.protobuf.StringValue host = 4;
* @return The host.
*/
public com.google.protobuf.StringValue getHost() {
if (hostBuilder_ == null) {
return host_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : host_;
} else {
return hostBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue host = 4;
*/
public Builder setHost(com.google.protobuf.StringValue value) {
if (hostBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
host_ = value;
onChanged();
} else {
hostBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue host = 4;
*/
public Builder setHost(
com.google.protobuf.StringValue.Builder builderForValue) {
if (hostBuilder_ == null) {
host_ = builderForValue.build();
onChanged();
} else {
hostBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue host = 4;
*/
public Builder mergeHost(com.google.protobuf.StringValue value) {
if (hostBuilder_ == null) {
if (host_ != null) {
host_ =
com.google.protobuf.StringValue.newBuilder(host_).mergeFrom(value).buildPartial();
} else {
host_ = value;
}
onChanged();
} else {
hostBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue host = 4;
*/
public Builder clearHost() {
if (hostBuilder_ == null) {
host_ = null;
onChanged();
} else {
host_ = null;
hostBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue host = 4;
*/
public com.google.protobuf.StringValue.Builder getHostBuilder() {
onChanged();
return getHostFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue host = 4;
*/
public com.google.protobuf.StringValueOrBuilder getHostOrBuilder() {
if (hostBuilder_ != null) {
return hostBuilder_.getMessageOrBuilder();
} else {
return host_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : host_;
}
}
/**
* .google.protobuf.StringValue host = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getHostFieldBuilder() {
if (hostBuilder_ == null) {
hostBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getHost(),
getParentForChildren(),
isClean());
host_ = null;
}
return hostBuilder_;
}
private com.google.protobuf.UInt32Value port_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> portBuilder_;
/**
* .google.protobuf.UInt32Value port = 5;
* @return Whether the port field is set.
*/
public boolean hasPort() {
return portBuilder_ != null || port_ != null;
}
/**
* .google.protobuf.UInt32Value port = 5;
* @return The port.
*/
public com.google.protobuf.UInt32Value getPort() {
if (portBuilder_ == null) {
return port_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : port_;
} else {
return portBuilder_.getMessage();
}
}
/**
* .google.protobuf.UInt32Value port = 5;
*/
public Builder setPort(com.google.protobuf.UInt32Value value) {
if (portBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
port_ = value;
onChanged();
} else {
portBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value port = 5;
*/
public Builder setPort(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (portBuilder_ == null) {
port_ = builderForValue.build();
onChanged();
} else {
portBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.UInt32Value port = 5;
*/
public Builder mergePort(com.google.protobuf.UInt32Value value) {
if (portBuilder_ == null) {
if (port_ != null) {
port_ =
com.google.protobuf.UInt32Value.newBuilder(port_).mergeFrom(value).buildPartial();
} else {
port_ = value;
}
onChanged();
} else {
portBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value port = 5;
*/
public Builder clearPort() {
if (portBuilder_ == null) {
port_ = null;
onChanged();
} else {
port_ = null;
portBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.UInt32Value port = 5;
*/
public com.google.protobuf.UInt32Value.Builder getPortBuilder() {
onChanged();
return getPortFieldBuilder().getBuilder();
}
/**
* .google.protobuf.UInt32Value port = 5;
*/
public com.google.protobuf.UInt32ValueOrBuilder getPortOrBuilder() {
if (portBuilder_ != null) {
return portBuilder_.getMessageOrBuilder();
} else {
return port_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : port_;
}
}
/**
* .google.protobuf.UInt32Value port = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getPortFieldBuilder() {
if (portBuilder_ == null) {
portBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getPort(),
getParentForChildren(),
isClean());
port_ = null;
}
return portBuilder_;
}
private com.google.protobuf.StringValue protocol_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> protocolBuilder_;
/**
* .google.protobuf.StringValue protocol = 6;
* @return Whether the protocol field is set.
*/
public boolean hasProtocol() {
return protocolBuilder_ != null || protocol_ != null;
}
/**
* .google.protobuf.StringValue protocol = 6;
* @return The protocol.
*/
public com.google.protobuf.StringValue getProtocol() {
if (protocolBuilder_ == null) {
return protocol_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : protocol_;
} else {
return protocolBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue protocol = 6;
*/
public Builder setProtocol(com.google.protobuf.StringValue value) {
if (protocolBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
protocol_ = value;
onChanged();
} else {
protocolBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue protocol = 6;
*/
public Builder setProtocol(
com.google.protobuf.StringValue.Builder builderForValue) {
if (protocolBuilder_ == null) {
protocol_ = builderForValue.build();
onChanged();
} else {
protocolBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue protocol = 6;
*/
public Builder mergeProtocol(com.google.protobuf.StringValue value) {
if (protocolBuilder_ == null) {
if (protocol_ != null) {
protocol_ =
com.google.protobuf.StringValue.newBuilder(protocol_).mergeFrom(value).buildPartial();
} else {
protocol_ = value;
}
onChanged();
} else {
protocolBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue protocol = 6;
*/
public Builder clearProtocol() {
if (protocolBuilder_ == null) {
protocol_ = null;
onChanged();
} else {
protocol_ = null;
protocolBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue protocol = 6;
*/
public com.google.protobuf.StringValue.Builder getProtocolBuilder() {
onChanged();
return getProtocolFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue protocol = 6;
*/
public com.google.protobuf.StringValueOrBuilder getProtocolOrBuilder() {
if (protocolBuilder_ != null) {
return protocolBuilder_.getMessageOrBuilder();
} else {
return protocol_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : protocol_;
}
}
/**
* .google.protobuf.StringValue protocol = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getProtocolFieldBuilder() {
if (protocolBuilder_ == null) {
protocolBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getProtocol(),
getParentForChildren(),
isClean());
protocol_ = null;
}
return protocolBuilder_;
}
private com.google.protobuf.StringValue version_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> versionBuilder_;
/**
* .google.protobuf.StringValue version = 7;
* @return Whether the version field is set.
*/
public boolean hasVersion() {
return versionBuilder_ != null || version_ != null;
}
/**
* .google.protobuf.StringValue version = 7;
* @return The version.
*/
public com.google.protobuf.StringValue getVersion() {
if (versionBuilder_ == null) {
return version_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : version_;
} else {
return versionBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue version = 7;
*/
public Builder setVersion(com.google.protobuf.StringValue value) {
if (versionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
version_ = value;
onChanged();
} else {
versionBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue version = 7;
*/
public Builder setVersion(
com.google.protobuf.StringValue.Builder builderForValue) {
if (versionBuilder_ == null) {
version_ = builderForValue.build();
onChanged();
} else {
versionBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue version = 7;
*/
public Builder mergeVersion(com.google.protobuf.StringValue value) {
if (versionBuilder_ == null) {
if (version_ != null) {
version_ =
com.google.protobuf.StringValue.newBuilder(version_).mergeFrom(value).buildPartial();
} else {
version_ = value;
}
onChanged();
} else {
versionBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue version = 7;
*/
public Builder clearVersion() {
if (versionBuilder_ == null) {
version_ = null;
onChanged();
} else {
version_ = null;
versionBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue version = 7;
*/
public com.google.protobuf.StringValue.Builder getVersionBuilder() {
onChanged();
return getVersionFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue version = 7;
*/
public com.google.protobuf.StringValueOrBuilder getVersionOrBuilder() {
if (versionBuilder_ != null) {
return versionBuilder_.getMessageOrBuilder();
} else {
return version_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : version_;
}
}
/**
* .google.protobuf.StringValue version = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getVersionFieldBuilder() {
if (versionBuilder_ == null) {
versionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getVersion(),
getParentForChildren(),
isClean());
version_ = null;
}
return versionBuilder_;
}
private com.google.protobuf.UInt32Value priority_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> priorityBuilder_;
/**
* .google.protobuf.UInt32Value priority = 8;
* @return Whether the priority field is set.
*/
public boolean hasPriority() {
return priorityBuilder_ != null || priority_ != null;
}
/**
* .google.protobuf.UInt32Value priority = 8;
* @return The priority.
*/
public com.google.protobuf.UInt32Value getPriority() {
if (priorityBuilder_ == null) {
return priority_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : priority_;
} else {
return priorityBuilder_.getMessage();
}
}
/**
* .google.protobuf.UInt32Value priority = 8;
*/
public Builder setPriority(com.google.protobuf.UInt32Value value) {
if (priorityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
priority_ = value;
onChanged();
} else {
priorityBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value priority = 8;
*/
public Builder setPriority(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (priorityBuilder_ == null) {
priority_ = builderForValue.build();
onChanged();
} else {
priorityBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.UInt32Value priority = 8;
*/
public Builder mergePriority(com.google.protobuf.UInt32Value value) {
if (priorityBuilder_ == null) {
if (priority_ != null) {
priority_ =
com.google.protobuf.UInt32Value.newBuilder(priority_).mergeFrom(value).buildPartial();
} else {
priority_ = value;
}
onChanged();
} else {
priorityBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value priority = 8;
*/
public Builder clearPriority() {
if (priorityBuilder_ == null) {
priority_ = null;
onChanged();
} else {
priority_ = null;
priorityBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.UInt32Value priority = 8;
*/
public com.google.protobuf.UInt32Value.Builder getPriorityBuilder() {
onChanged();
return getPriorityFieldBuilder().getBuilder();
}
/**
* .google.protobuf.UInt32Value priority = 8;
*/
public com.google.protobuf.UInt32ValueOrBuilder getPriorityOrBuilder() {
if (priorityBuilder_ != null) {
return priorityBuilder_.getMessageOrBuilder();
} else {
return priority_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : priority_;
}
}
/**
* .google.protobuf.UInt32Value priority = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getPriorityFieldBuilder() {
if (priorityBuilder_ == null) {
priorityBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getPriority(),
getParentForChildren(),
isClean());
priority_ = null;
}
return priorityBuilder_;
}
private com.google.protobuf.UInt32Value weight_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> weightBuilder_;
/**
* .google.protobuf.UInt32Value weight = 9;
* @return Whether the weight field is set.
*/
public boolean hasWeight() {
return weightBuilder_ != null || weight_ != null;
}
/**
* .google.protobuf.UInt32Value weight = 9;
* @return The weight.
*/
public com.google.protobuf.UInt32Value getWeight() {
if (weightBuilder_ == null) {
return weight_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : weight_;
} else {
return weightBuilder_.getMessage();
}
}
/**
* .google.protobuf.UInt32Value weight = 9;
*/
public Builder setWeight(com.google.protobuf.UInt32Value value) {
if (weightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
weight_ = value;
onChanged();
} else {
weightBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value weight = 9;
*/
public Builder setWeight(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (weightBuilder_ == null) {
weight_ = builderForValue.build();
onChanged();
} else {
weightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.UInt32Value weight = 9;
*/
public Builder mergeWeight(com.google.protobuf.UInt32Value value) {
if (weightBuilder_ == null) {
if (weight_ != null) {
weight_ =
com.google.protobuf.UInt32Value.newBuilder(weight_).mergeFrom(value).buildPartial();
} else {
weight_ = value;
}
onChanged();
} else {
weightBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value weight = 9;
*/
public Builder clearWeight() {
if (weightBuilder_ == null) {
weight_ = null;
onChanged();
} else {
weight_ = null;
weightBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.UInt32Value weight = 9;
*/
public com.google.protobuf.UInt32Value.Builder getWeightBuilder() {
onChanged();
return getWeightFieldBuilder().getBuilder();
}
/**
* .google.protobuf.UInt32Value weight = 9;
*/
public com.google.protobuf.UInt32ValueOrBuilder getWeightOrBuilder() {
if (weightBuilder_ != null) {
return weightBuilder_.getMessageOrBuilder();
} else {
return weight_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : weight_;
}
}
/**
* .google.protobuf.UInt32Value weight = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getWeightFieldBuilder() {
if (weightBuilder_ == null) {
weightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getWeight(),
getParentForChildren(),
isClean());
weight_ = null;
}
return weightBuilder_;
}
private com.google.protobuf.BoolValue enableHealthCheck_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder> enableHealthCheckBuilder_;
/**
* .google.protobuf.BoolValue enable_health_check = 20;
* @return Whether the enableHealthCheck field is set.
*/
public boolean hasEnableHealthCheck() {
return enableHealthCheckBuilder_ != null || enableHealthCheck_ != null;
}
/**
* .google.protobuf.BoolValue enable_health_check = 20;
* @return The enableHealthCheck.
*/
public com.google.protobuf.BoolValue getEnableHealthCheck() {
if (enableHealthCheckBuilder_ == null) {
return enableHealthCheck_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : enableHealthCheck_;
} else {
return enableHealthCheckBuilder_.getMessage();
}
}
/**
* .google.protobuf.BoolValue enable_health_check = 20;
*/
public Builder setEnableHealthCheck(com.google.protobuf.BoolValue value) {
if (enableHealthCheckBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
enableHealthCheck_ = value;
onChanged();
} else {
enableHealthCheckBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.BoolValue enable_health_check = 20;
*/
public Builder setEnableHealthCheck(
com.google.protobuf.BoolValue.Builder builderForValue) {
if (enableHealthCheckBuilder_ == null) {
enableHealthCheck_ = builderForValue.build();
onChanged();
} else {
enableHealthCheckBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.BoolValue enable_health_check = 20;
*/
public Builder mergeEnableHealthCheck(com.google.protobuf.BoolValue value) {
if (enableHealthCheckBuilder_ == null) {
if (enableHealthCheck_ != null) {
enableHealthCheck_ =
com.google.protobuf.BoolValue.newBuilder(enableHealthCheck_).mergeFrom(value).buildPartial();
} else {
enableHealthCheck_ = value;
}
onChanged();
} else {
enableHealthCheckBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.BoolValue enable_health_check = 20;
*/
public Builder clearEnableHealthCheck() {
if (enableHealthCheckBuilder_ == null) {
enableHealthCheck_ = null;
onChanged();
} else {
enableHealthCheck_ = null;
enableHealthCheckBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.BoolValue enable_health_check = 20;
*/
public com.google.protobuf.BoolValue.Builder getEnableHealthCheckBuilder() {
onChanged();
return getEnableHealthCheckFieldBuilder().getBuilder();
}
/**
* .google.protobuf.BoolValue enable_health_check = 20;
*/
public com.google.protobuf.BoolValueOrBuilder getEnableHealthCheckOrBuilder() {
if (enableHealthCheckBuilder_ != null) {
return enableHealthCheckBuilder_.getMessageOrBuilder();
} else {
return enableHealthCheck_ == null ?
com.google.protobuf.BoolValue.getDefaultInstance() : enableHealthCheck_;
}
}
/**
* .google.protobuf.BoolValue enable_health_check = 20;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>
getEnableHealthCheckFieldBuilder() {
if (enableHealthCheckBuilder_ == null) {
enableHealthCheckBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>(
getEnableHealthCheck(),
getParentForChildren(),
isClean());
enableHealthCheck_ = null;
}
return enableHealthCheckBuilder_;
}
private com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck healthCheck_;
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.Builder, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheckOrBuilder> healthCheckBuilder_;
/**
* .v1.HealthCheck health_check = 10;
* @return Whether the healthCheck field is set.
*/
public boolean hasHealthCheck() {
return healthCheckBuilder_ != null || healthCheck_ != null;
}
/**
* .v1.HealthCheck health_check = 10;
* @return The healthCheck.
*/
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck getHealthCheck() {
if (healthCheckBuilder_ == null) {
return healthCheck_ == null ? com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.getDefaultInstance() : healthCheck_;
} else {
return healthCheckBuilder_.getMessage();
}
}
/**
* .v1.HealthCheck health_check = 10;
*/
public Builder setHealthCheck(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck value) {
if (healthCheckBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
healthCheck_ = value;
onChanged();
} else {
healthCheckBuilder_.setMessage(value);
}
return this;
}
/**
* .v1.HealthCheck health_check = 10;
*/
public Builder setHealthCheck(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.Builder builderForValue) {
if (healthCheckBuilder_ == null) {
healthCheck_ = builderForValue.build();
onChanged();
} else {
healthCheckBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .v1.HealthCheck health_check = 10;
*/
public Builder mergeHealthCheck(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck value) {
if (healthCheckBuilder_ == null) {
if (healthCheck_ != null) {
healthCheck_ =
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.newBuilder(healthCheck_).mergeFrom(value).buildPartial();
} else {
healthCheck_ = value;
}
onChanged();
} else {
healthCheckBuilder_.mergeFrom(value);
}
return this;
}
/**
* .v1.HealthCheck health_check = 10;
*/
public Builder clearHealthCheck() {
if (healthCheckBuilder_ == null) {
healthCheck_ = null;
onChanged();
} else {
healthCheck_ = null;
healthCheckBuilder_ = null;
}
return this;
}
/**
* .v1.HealthCheck health_check = 10;
*/
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.Builder getHealthCheckBuilder() {
onChanged();
return getHealthCheckFieldBuilder().getBuilder();
}
/**
* .v1.HealthCheck health_check = 10;
*/
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheckOrBuilder getHealthCheckOrBuilder() {
if (healthCheckBuilder_ != null) {
return healthCheckBuilder_.getMessageOrBuilder();
} else {
return healthCheck_ == null ?
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.getDefaultInstance() : healthCheck_;
}
}
/**
* .v1.HealthCheck health_check = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.Builder, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheckOrBuilder>
getHealthCheckFieldBuilder() {
if (healthCheckBuilder_ == null) {
healthCheckBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.Builder, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheckOrBuilder>(
getHealthCheck(),
getParentForChildren(),
isClean());
healthCheck_ = null;
}
return healthCheckBuilder_;
}
private com.google.protobuf.BoolValue healthy_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder> healthyBuilder_;
/**
* .google.protobuf.BoolValue healthy = 11;
* @return Whether the healthy field is set.
*/
public boolean hasHealthy() {
return healthyBuilder_ != null || healthy_ != null;
}
/**
* .google.protobuf.BoolValue healthy = 11;
* @return The healthy.
*/
public com.google.protobuf.BoolValue getHealthy() {
if (healthyBuilder_ == null) {
return healthy_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : healthy_;
} else {
return healthyBuilder_.getMessage();
}
}
/**
* .google.protobuf.BoolValue healthy = 11;
*/
public Builder setHealthy(com.google.protobuf.BoolValue value) {
if (healthyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
healthy_ = value;
onChanged();
} else {
healthyBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.BoolValue healthy = 11;
*/
public Builder setHealthy(
com.google.protobuf.BoolValue.Builder builderForValue) {
if (healthyBuilder_ == null) {
healthy_ = builderForValue.build();
onChanged();
} else {
healthyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.BoolValue healthy = 11;
*/
public Builder mergeHealthy(com.google.protobuf.BoolValue value) {
if (healthyBuilder_ == null) {
if (healthy_ != null) {
healthy_ =
com.google.protobuf.BoolValue.newBuilder(healthy_).mergeFrom(value).buildPartial();
} else {
healthy_ = value;
}
onChanged();
} else {
healthyBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.BoolValue healthy = 11;
*/
public Builder clearHealthy() {
if (healthyBuilder_ == null) {
healthy_ = null;
onChanged();
} else {
healthy_ = null;
healthyBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.BoolValue healthy = 11;
*/
public com.google.protobuf.BoolValue.Builder getHealthyBuilder() {
onChanged();
return getHealthyFieldBuilder().getBuilder();
}
/**
* .google.protobuf.BoolValue healthy = 11;
*/
public com.google.protobuf.BoolValueOrBuilder getHealthyOrBuilder() {
if (healthyBuilder_ != null) {
return healthyBuilder_.getMessageOrBuilder();
} else {
return healthy_ == null ?
com.google.protobuf.BoolValue.getDefaultInstance() : healthy_;
}
}
/**
* .google.protobuf.BoolValue healthy = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>
getHealthyFieldBuilder() {
if (healthyBuilder_ == null) {
healthyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>(
getHealthy(),
getParentForChildren(),
isClean());
healthy_ = null;
}
return healthyBuilder_;
}
private com.google.protobuf.BoolValue isolate_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder> isolateBuilder_;
/**
* .google.protobuf.BoolValue isolate = 12;
* @return Whether the isolate field is set.
*/
public boolean hasIsolate() {
return isolateBuilder_ != null || isolate_ != null;
}
/**
* .google.protobuf.BoolValue isolate = 12;
* @return The isolate.
*/
public com.google.protobuf.BoolValue getIsolate() {
if (isolateBuilder_ == null) {
return isolate_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : isolate_;
} else {
return isolateBuilder_.getMessage();
}
}
/**
* .google.protobuf.BoolValue isolate = 12;
*/
public Builder setIsolate(com.google.protobuf.BoolValue value) {
if (isolateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
isolate_ = value;
onChanged();
} else {
isolateBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.BoolValue isolate = 12;
*/
public Builder setIsolate(
com.google.protobuf.BoolValue.Builder builderForValue) {
if (isolateBuilder_ == null) {
isolate_ = builderForValue.build();
onChanged();
} else {
isolateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.BoolValue isolate = 12;
*/
public Builder mergeIsolate(com.google.protobuf.BoolValue value) {
if (isolateBuilder_ == null) {
if (isolate_ != null) {
isolate_ =
com.google.protobuf.BoolValue.newBuilder(isolate_).mergeFrom(value).buildPartial();
} else {
isolate_ = value;
}
onChanged();
} else {
isolateBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.BoolValue isolate = 12;
*/
public Builder clearIsolate() {
if (isolateBuilder_ == null) {
isolate_ = null;
onChanged();
} else {
isolate_ = null;
isolateBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.BoolValue isolate = 12;
*/
public com.google.protobuf.BoolValue.Builder getIsolateBuilder() {
onChanged();
return getIsolateFieldBuilder().getBuilder();
}
/**
* .google.protobuf.BoolValue isolate = 12;
*/
public com.google.protobuf.BoolValueOrBuilder getIsolateOrBuilder() {
if (isolateBuilder_ != null) {
return isolateBuilder_.getMessageOrBuilder();
} else {
return isolate_ == null ?
com.google.protobuf.BoolValue.getDefaultInstance() : isolate_;
}
}
/**
* .google.protobuf.BoolValue isolate = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>
getIsolateFieldBuilder() {
if (isolateBuilder_ == null) {
isolateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>(
getIsolate(),
getParentForChildren(),
isClean());
isolate_ = null;
}
return isolateBuilder_;
}
private com.tencent.polaris.specification.api.v1.model.ModelProto.Location location_;
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.specification.api.v1.model.ModelProto.Location, com.tencent.polaris.specification.api.v1.model.ModelProto.Location.Builder, com.tencent.polaris.specification.api.v1.model.ModelProto.LocationOrBuilder> locationBuilder_;
/**
* .v1.Location location = 13;
* @return Whether the location field is set.
*/
public boolean hasLocation() {
return locationBuilder_ != null || location_ != null;
}
/**
* .v1.Location location = 13;
* @return The location.
*/
public com.tencent.polaris.specification.api.v1.model.ModelProto.Location getLocation() {
if (locationBuilder_ == null) {
return location_ == null ? com.tencent.polaris.specification.api.v1.model.ModelProto.Location.getDefaultInstance() : location_;
} else {
return locationBuilder_.getMessage();
}
}
/**
* .v1.Location location = 13;
*/
public Builder setLocation(com.tencent.polaris.specification.api.v1.model.ModelProto.Location value) {
if (locationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
location_ = value;
onChanged();
} else {
locationBuilder_.setMessage(value);
}
return this;
}
/**
* .v1.Location location = 13;
*/
public Builder setLocation(
com.tencent.polaris.specification.api.v1.model.ModelProto.Location.Builder builderForValue) {
if (locationBuilder_ == null) {
location_ = builderForValue.build();
onChanged();
} else {
locationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .v1.Location location = 13;
*/
public Builder mergeLocation(com.tencent.polaris.specification.api.v1.model.ModelProto.Location value) {
if (locationBuilder_ == null) {
if (location_ != null) {
location_ =
com.tencent.polaris.specification.api.v1.model.ModelProto.Location.newBuilder(location_).mergeFrom(value).buildPartial();
} else {
location_ = value;
}
onChanged();
} else {
locationBuilder_.mergeFrom(value);
}
return this;
}
/**
* .v1.Location location = 13;
*/
public Builder clearLocation() {
if (locationBuilder_ == null) {
location_ = null;
onChanged();
} else {
location_ = null;
locationBuilder_ = null;
}
return this;
}
/**
* .v1.Location location = 13;
*/
public com.tencent.polaris.specification.api.v1.model.ModelProto.Location.Builder getLocationBuilder() {
onChanged();
return getLocationFieldBuilder().getBuilder();
}
/**
* .v1.Location location = 13;
*/
public com.tencent.polaris.specification.api.v1.model.ModelProto.LocationOrBuilder getLocationOrBuilder() {
if (locationBuilder_ != null) {
return locationBuilder_.getMessageOrBuilder();
} else {
return location_ == null ?
com.tencent.polaris.specification.api.v1.model.ModelProto.Location.getDefaultInstance() : location_;
}
}
/**
* .v1.Location location = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.specification.api.v1.model.ModelProto.Location, com.tencent.polaris.specification.api.v1.model.ModelProto.Location.Builder, com.tencent.polaris.specification.api.v1.model.ModelProto.LocationOrBuilder>
getLocationFieldBuilder() {
if (locationBuilder_ == null) {
locationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.specification.api.v1.model.ModelProto.Location, com.tencent.polaris.specification.api.v1.model.ModelProto.Location.Builder, com.tencent.polaris.specification.api.v1.model.ModelProto.LocationOrBuilder>(
getLocation(),
getParentForChildren(),
isClean());
location_ = null;
}
return locationBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> metadata_;
private com.google.protobuf.MapField
internalGetMetadata() {
if (metadata_ == null) {
return com.google.protobuf.MapField.emptyMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
return metadata_;
}
private com.google.protobuf.MapField
internalGetMutableMetadata() {
onChanged();;
if (metadata_ == null) {
metadata_ = com.google.protobuf.MapField.newMapField(
MetadataDefaultEntryHolder.defaultEntry);
}
if (!metadata_.isMutable()) {
metadata_ = metadata_.copy();
}
return metadata_;
}
public int getMetadataCount() {
return internalGetMetadata().getMap().size();
}
/**
* map<string, string> metadata = 14;
*/
@java.lang.Override
public boolean containsMetadata(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetMetadata().getMap().containsKey(key);
}
/**
* Use {@link #getMetadataMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getMetadata() {
return getMetadataMap();
}
/**
* map<string, string> metadata = 14;
*/
@java.lang.Override
public java.util.Map getMetadataMap() {
return internalGetMetadata().getMap();
}
/**
* map<string, string> metadata = 14;
*/
@java.lang.Override
public java.lang.String getMetadataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetMetadata().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> metadata = 14;
*/
@java.lang.Override
public java.lang.String getMetadataOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetMetadata().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearMetadata() {
internalGetMutableMetadata().getMutableMap()
.clear();
return this;
}
/**
* map<string, string> metadata = 14;
*/
public Builder removeMetadata(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableMetadata().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableMetadata() {
return internalGetMutableMetadata().getMutableMap();
}
/**
* map<string, string> metadata = 14;
*/
public Builder putMetadata(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableMetadata().getMutableMap()
.put(key, value);
return this;
}
/**
* map<string, string> metadata = 14;
*/
public Builder putAllMetadata(
java.util.Map values) {
internalGetMutableMetadata().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.StringValue logicSet_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> logicSetBuilder_;
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
* @return Whether the logicSet field is set.
*/
public boolean hasLogicSet() {
return logicSetBuilder_ != null || logicSet_ != null;
}
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
* @return The logicSet.
*/
public com.google.protobuf.StringValue getLogicSet() {
if (logicSetBuilder_ == null) {
return logicSet_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : logicSet_;
} else {
return logicSetBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
*/
public Builder setLogicSet(com.google.protobuf.StringValue value) {
if (logicSetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
logicSet_ = value;
onChanged();
} else {
logicSetBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
*/
public Builder setLogicSet(
com.google.protobuf.StringValue.Builder builderForValue) {
if (logicSetBuilder_ == null) {
logicSet_ = builderForValue.build();
onChanged();
} else {
logicSetBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
*/
public Builder mergeLogicSet(com.google.protobuf.StringValue value) {
if (logicSetBuilder_ == null) {
if (logicSet_ != null) {
logicSet_ =
com.google.protobuf.StringValue.newBuilder(logicSet_).mergeFrom(value).buildPartial();
} else {
logicSet_ = value;
}
onChanged();
} else {
logicSetBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
*/
public Builder clearLogicSet() {
if (logicSetBuilder_ == null) {
logicSet_ = null;
onChanged();
} else {
logicSet_ = null;
logicSetBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
*/
public com.google.protobuf.StringValue.Builder getLogicSetBuilder() {
onChanged();
return getLogicSetFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
*/
public com.google.protobuf.StringValueOrBuilder getLogicSetOrBuilder() {
if (logicSetBuilder_ != null) {
return logicSetBuilder_.getMessageOrBuilder();
} else {
return logicSet_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : logicSet_;
}
}
/**
* .google.protobuf.StringValue logic_set = 15 [json_name = "logic_set"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getLogicSetFieldBuilder() {
if (logicSetBuilder_ == null) {
logicSetBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getLogicSet(),
getParentForChildren(),
isClean());
logicSet_ = null;
}
return logicSetBuilder_;
}
private com.google.protobuf.StringValue ctime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> ctimeBuilder_;
/**
* .google.protobuf.StringValue ctime = 16;
* @return Whether the ctime field is set.
*/
public boolean hasCtime() {
return ctimeBuilder_ != null || ctime_ != null;
}
/**
* .google.protobuf.StringValue ctime = 16;
* @return The ctime.
*/
public com.google.protobuf.StringValue getCtime() {
if (ctimeBuilder_ == null) {
return ctime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : ctime_;
} else {
return ctimeBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue ctime = 16;
*/
public Builder setCtime(com.google.protobuf.StringValue value) {
if (ctimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ctime_ = value;
onChanged();
} else {
ctimeBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 16;
*/
public Builder setCtime(
com.google.protobuf.StringValue.Builder builderForValue) {
if (ctimeBuilder_ == null) {
ctime_ = builderForValue.build();
onChanged();
} else {
ctimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 16;
*/
public Builder mergeCtime(com.google.protobuf.StringValue value) {
if (ctimeBuilder_ == null) {
if (ctime_ != null) {
ctime_ =
com.google.protobuf.StringValue.newBuilder(ctime_).mergeFrom(value).buildPartial();
} else {
ctime_ = value;
}
onChanged();
} else {
ctimeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 16;
*/
public Builder clearCtime() {
if (ctimeBuilder_ == null) {
ctime_ = null;
onChanged();
} else {
ctime_ = null;
ctimeBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue ctime = 16;
*/
public com.google.protobuf.StringValue.Builder getCtimeBuilder() {
onChanged();
return getCtimeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue ctime = 16;
*/
public com.google.protobuf.StringValueOrBuilder getCtimeOrBuilder() {
if (ctimeBuilder_ != null) {
return ctimeBuilder_.getMessageOrBuilder();
} else {
return ctime_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : ctime_;
}
}
/**
* .google.protobuf.StringValue ctime = 16;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getCtimeFieldBuilder() {
if (ctimeBuilder_ == null) {
ctimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getCtime(),
getParentForChildren(),
isClean());
ctime_ = null;
}
return ctimeBuilder_;
}
private com.google.protobuf.StringValue mtime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> mtimeBuilder_;
/**
* .google.protobuf.StringValue mtime = 17;
* @return Whether the mtime field is set.
*/
public boolean hasMtime() {
return mtimeBuilder_ != null || mtime_ != null;
}
/**
* .google.protobuf.StringValue mtime = 17;
* @return The mtime.
*/
public com.google.protobuf.StringValue getMtime() {
if (mtimeBuilder_ == null) {
return mtime_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : mtime_;
} else {
return mtimeBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue mtime = 17;
*/
public Builder setMtime(com.google.protobuf.StringValue value) {
if (mtimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
mtime_ = value;
onChanged();
} else {
mtimeBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 17;
*/
public Builder setMtime(
com.google.protobuf.StringValue.Builder builderForValue) {
if (mtimeBuilder_ == null) {
mtime_ = builderForValue.build();
onChanged();
} else {
mtimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 17;
*/
public Builder mergeMtime(com.google.protobuf.StringValue value) {
if (mtimeBuilder_ == null) {
if (mtime_ != null) {
mtime_ =
com.google.protobuf.StringValue.newBuilder(mtime_).mergeFrom(value).buildPartial();
} else {
mtime_ = value;
}
onChanged();
} else {
mtimeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 17;
*/
public Builder clearMtime() {
if (mtimeBuilder_ == null) {
mtime_ = null;
onChanged();
} else {
mtime_ = null;
mtimeBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue mtime = 17;
*/
public com.google.protobuf.StringValue.Builder getMtimeBuilder() {
onChanged();
return getMtimeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue mtime = 17;
*/
public com.google.protobuf.StringValueOrBuilder getMtimeOrBuilder() {
if (mtimeBuilder_ != null) {
return mtimeBuilder_.getMessageOrBuilder();
} else {
return mtime_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : mtime_;
}
}
/**
* .google.protobuf.StringValue mtime = 17;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getMtimeFieldBuilder() {
if (mtimeBuilder_ == null) {
mtimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getMtime(),
getParentForChildren(),
isClean());
mtime_ = null;
}
return mtimeBuilder_;
}
private com.google.protobuf.StringValue revision_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> revisionBuilder_;
/**
* .google.protobuf.StringValue revision = 18;
* @return Whether the revision field is set.
*/
public boolean hasRevision() {
return revisionBuilder_ != null || revision_ != null;
}
/**
* .google.protobuf.StringValue revision = 18;
* @return The revision.
*/
public com.google.protobuf.StringValue getRevision() {
if (revisionBuilder_ == null) {
return revision_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : revision_;
} else {
return revisionBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue revision = 18;
*/
public Builder setRevision(com.google.protobuf.StringValue value) {
if (revisionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
revision_ = value;
onChanged();
} else {
revisionBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue revision = 18;
*/
public Builder setRevision(
com.google.protobuf.StringValue.Builder builderForValue) {
if (revisionBuilder_ == null) {
revision_ = builderForValue.build();
onChanged();
} else {
revisionBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue revision = 18;
*/
public Builder mergeRevision(com.google.protobuf.StringValue value) {
if (revisionBuilder_ == null) {
if (revision_ != null) {
revision_ =
com.google.protobuf.StringValue.newBuilder(revision_).mergeFrom(value).buildPartial();
} else {
revision_ = value;
}
onChanged();
} else {
revisionBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue revision = 18;
*/
public Builder clearRevision() {
if (revisionBuilder_ == null) {
revision_ = null;
onChanged();
} else {
revision_ = null;
revisionBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue revision = 18;
*/
public com.google.protobuf.StringValue.Builder getRevisionBuilder() {
onChanged();
return getRevisionFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue revision = 18;
*/
public com.google.protobuf.StringValueOrBuilder getRevisionOrBuilder() {
if (revisionBuilder_ != null) {
return revisionBuilder_.getMessageOrBuilder();
} else {
return revision_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : revision_;
}
}
/**
* .google.protobuf.StringValue revision = 18;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getRevisionFieldBuilder() {
if (revisionBuilder_ == null) {
revisionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getRevision(),
getParentForChildren(),
isClean());
revision_ = null;
}
return revisionBuilder_;
}
private com.google.protobuf.StringValue serviceToken_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder> serviceTokenBuilder_;
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
* @return Whether the serviceToken field is set.
*/
public boolean hasServiceToken() {
return serviceTokenBuilder_ != null || serviceToken_ != null;
}
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
* @return The serviceToken.
*/
public com.google.protobuf.StringValue getServiceToken() {
if (serviceTokenBuilder_ == null) {
return serviceToken_ == null ? com.google.protobuf.StringValue.getDefaultInstance() : serviceToken_;
} else {
return serviceTokenBuilder_.getMessage();
}
}
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
*/
public Builder setServiceToken(com.google.protobuf.StringValue value) {
if (serviceTokenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
serviceToken_ = value;
onChanged();
} else {
serviceTokenBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
*/
public Builder setServiceToken(
com.google.protobuf.StringValue.Builder builderForValue) {
if (serviceTokenBuilder_ == null) {
serviceToken_ = builderForValue.build();
onChanged();
} else {
serviceTokenBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
*/
public Builder mergeServiceToken(com.google.protobuf.StringValue value) {
if (serviceTokenBuilder_ == null) {
if (serviceToken_ != null) {
serviceToken_ =
com.google.protobuf.StringValue.newBuilder(serviceToken_).mergeFrom(value).buildPartial();
} else {
serviceToken_ = value;
}
onChanged();
} else {
serviceTokenBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
*/
public Builder clearServiceToken() {
if (serviceTokenBuilder_ == null) {
serviceToken_ = null;
onChanged();
} else {
serviceToken_ = null;
serviceTokenBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
*/
public com.google.protobuf.StringValue.Builder getServiceTokenBuilder() {
onChanged();
return getServiceTokenFieldBuilder().getBuilder();
}
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
*/
public com.google.protobuf.StringValueOrBuilder getServiceTokenOrBuilder() {
if (serviceTokenBuilder_ != null) {
return serviceTokenBuilder_.getMessageOrBuilder();
} else {
return serviceToken_ == null ?
com.google.protobuf.StringValue.getDefaultInstance() : serviceToken_;
}
}
/**
* .google.protobuf.StringValue service_token = 19 [json_name = "service_token"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>
getServiceTokenFieldBuilder() {
if (serviceTokenBuilder_ == null) {
serviceTokenBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.StringValue, com.google.protobuf.StringValue.Builder, com.google.protobuf.StringValueOrBuilder>(
getServiceToken(),
getParentForChildren(),
isClean());
serviceToken_ = null;
}
return serviceTokenBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:v1.Instance)
}
// @@protoc_insertion_point(class_scope:v1.Instance)
private static final com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance();
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Instance parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.Instance getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HealthCheckOrBuilder extends
// @@protoc_insertion_point(interface_extends:v1.HealthCheck)
com.google.protobuf.MessageOrBuilder {
/**
* .v1.HealthCheck.HealthCheckType type = 1;
* @return The enum numeric value on the wire for type.
*/
int getTypeValue();
/**
* .v1.HealthCheck.HealthCheckType type = 1;
* @return The type.
*/
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType getType();
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
* @return Whether the heartbeat field is set.
*/
boolean hasHeartbeat();
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
* @return The heartbeat.
*/
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck getHeartbeat();
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
*/
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheckOrBuilder getHeartbeatOrBuilder();
}
/**
* Protobuf type {@code v1.HealthCheck}
*/
public static final class HealthCheck extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:v1.HealthCheck)
HealthCheckOrBuilder {
private static final long serialVersionUID = 0L;
// Use HealthCheck.newBuilder() to construct.
private HealthCheck(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HealthCheck() {
type_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HealthCheck();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_HealthCheck_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_HealthCheck_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.class, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.Builder.class);
}
/**
* Protobuf enum {@code v1.HealthCheck.HealthCheckType}
*/
public enum HealthCheckType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
* HEARTBEAT = 1;
*/
HEARTBEAT(1),
UNRECOGNIZED(-1),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
* HEARTBEAT = 1;
*/
public static final int HEARTBEAT_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static HealthCheckType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static HealthCheckType forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return HEARTBEAT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
HealthCheckType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public HealthCheckType findValueByNumber(int number) {
return HealthCheckType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.getDescriptor().getEnumTypes().get(0);
}
private static final HealthCheckType[] VALUES = values();
public static HealthCheckType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private HealthCheckType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:v1.HealthCheck.HealthCheckType)
}
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
* .v1.HealthCheck.HealthCheckType type = 1;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .v1.HealthCheck.HealthCheckType type = 1;
* @return The type.
*/
@java.lang.Override public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType getType() {
@SuppressWarnings("deprecation")
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType result = com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType.valueOf(type_);
return result == null ? com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType.UNRECOGNIZED : result;
}
public static final int HEARTBEAT_FIELD_NUMBER = 2;
private com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck heartbeat_;
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
* @return Whether the heartbeat field is set.
*/
@java.lang.Override
public boolean hasHeartbeat() {
return heartbeat_ != null;
}
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
* @return The heartbeat.
*/
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck getHeartbeat() {
return heartbeat_ == null ? com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.getDefaultInstance() : heartbeat_;
}
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
*/
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheckOrBuilder getHeartbeatOrBuilder() {
return getHeartbeat();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType.UNKNOWN.getNumber()) {
output.writeEnum(1, type_);
}
if (heartbeat_ != null) {
output.writeMessage(2, getHeartbeat());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType.UNKNOWN.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (heartbeat_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getHeartbeat());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck)) {
return super.equals(obj);
}
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck other = (com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck) obj;
if (type_ != other.type_) return false;
if (hasHeartbeat() != other.hasHeartbeat()) return false;
if (hasHeartbeat()) {
if (!getHeartbeat()
.equals(other.getHeartbeat())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
if (hasHeartbeat()) {
hash = (37 * hash) + HEARTBEAT_FIELD_NUMBER;
hash = (53 * hash) + getHeartbeat().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code v1.HealthCheck}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:v1.HealthCheck)
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheckOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_HealthCheck_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_HealthCheck_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.class, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.Builder.class);
}
// Construct using com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 0;
if (heartbeatBuilder_ == null) {
heartbeat_ = null;
} else {
heartbeat_ = null;
heartbeatBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_HealthCheck_descriptor;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck getDefaultInstanceForType() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck build() {
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck buildPartial() {
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck result = new com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck(this);
result.type_ = type_;
if (heartbeatBuilder_ == null) {
result.heartbeat_ = heartbeat_;
} else {
result.heartbeat_ = heartbeatBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck) {
return mergeFrom((com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck other) {
if (other == com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.getDefaultInstance()) return this;
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.hasHeartbeat()) {
mergeHeartbeat(other.getHeartbeat());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
type_ = input.readEnum();
break;
} // case 8
case 18: {
input.readMessage(
getHeartbeatFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int type_ = 0;
/**
* .v1.HealthCheck.HealthCheckType type = 1;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .v1.HealthCheck.HealthCheckType type = 1;
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* .v1.HealthCheck.HealthCheckType type = 1;
* @return The type.
*/
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType getType() {
@SuppressWarnings("deprecation")
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType result = com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType.valueOf(type_);
return result == null ? com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType.UNRECOGNIZED : result;
}
/**
* .v1.HealthCheck.HealthCheckType type = 1;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck.HealthCheckType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* .v1.HealthCheck.HealthCheckType type = 1;
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck heartbeat_;
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.Builder, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheckOrBuilder> heartbeatBuilder_;
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
* @return Whether the heartbeat field is set.
*/
public boolean hasHeartbeat() {
return heartbeatBuilder_ != null || heartbeat_ != null;
}
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
* @return The heartbeat.
*/
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck getHeartbeat() {
if (heartbeatBuilder_ == null) {
return heartbeat_ == null ? com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.getDefaultInstance() : heartbeat_;
} else {
return heartbeatBuilder_.getMessage();
}
}
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
*/
public Builder setHeartbeat(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck value) {
if (heartbeatBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
heartbeat_ = value;
onChanged();
} else {
heartbeatBuilder_.setMessage(value);
}
return this;
}
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
*/
public Builder setHeartbeat(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.Builder builderForValue) {
if (heartbeatBuilder_ == null) {
heartbeat_ = builderForValue.build();
onChanged();
} else {
heartbeatBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
*/
public Builder mergeHeartbeat(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck value) {
if (heartbeatBuilder_ == null) {
if (heartbeat_ != null) {
heartbeat_ =
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.newBuilder(heartbeat_).mergeFrom(value).buildPartial();
} else {
heartbeat_ = value;
}
onChanged();
} else {
heartbeatBuilder_.mergeFrom(value);
}
return this;
}
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
*/
public Builder clearHeartbeat() {
if (heartbeatBuilder_ == null) {
heartbeat_ = null;
onChanged();
} else {
heartbeat_ = null;
heartbeatBuilder_ = null;
}
return this;
}
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
*/
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.Builder getHeartbeatBuilder() {
onChanged();
return getHeartbeatFieldBuilder().getBuilder();
}
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
*/
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheckOrBuilder getHeartbeatOrBuilder() {
if (heartbeatBuilder_ != null) {
return heartbeatBuilder_.getMessageOrBuilder();
} else {
return heartbeat_ == null ?
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.getDefaultInstance() : heartbeat_;
}
}
/**
* .v1.HeartbeatHealthCheck heartbeat = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.Builder, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheckOrBuilder>
getHeartbeatFieldBuilder() {
if (heartbeatBuilder_ == null) {
heartbeatBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.Builder, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheckOrBuilder>(
getHeartbeat(),
getParentForChildren(),
isClean());
heartbeat_ = null;
}
return heartbeatBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:v1.HealthCheck)
}
// @@protoc_insertion_point(class_scope:v1.HealthCheck)
private static final com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck();
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HealthCheck parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HealthCheck getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HeartbeatHealthCheckOrBuilder extends
// @@protoc_insertion_point(interface_extends:v1.HeartbeatHealthCheck)
com.google.protobuf.MessageOrBuilder {
/**
* .google.protobuf.UInt32Value ttl = 1;
* @return Whether the ttl field is set.
*/
boolean hasTtl();
/**
* .google.protobuf.UInt32Value ttl = 1;
* @return The ttl.
*/
com.google.protobuf.UInt32Value getTtl();
/**
* .google.protobuf.UInt32Value ttl = 1;
*/
com.google.protobuf.UInt32ValueOrBuilder getTtlOrBuilder();
}
/**
* Protobuf type {@code v1.HeartbeatHealthCheck}
*/
public static final class HeartbeatHealthCheck extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:v1.HeartbeatHealthCheck)
HeartbeatHealthCheckOrBuilder {
private static final long serialVersionUID = 0L;
// Use HeartbeatHealthCheck.newBuilder() to construct.
private HeartbeatHealthCheck(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HeartbeatHealthCheck() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HeartbeatHealthCheck();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_HeartbeatHealthCheck_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_HeartbeatHealthCheck_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.class, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.Builder.class);
}
public static final int TTL_FIELD_NUMBER = 1;
private com.google.protobuf.UInt32Value ttl_;
/**
* .google.protobuf.UInt32Value ttl = 1;
* @return Whether the ttl field is set.
*/
@java.lang.Override
public boolean hasTtl() {
return ttl_ != null;
}
/**
* .google.protobuf.UInt32Value ttl = 1;
* @return The ttl.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getTtl() {
return ttl_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : ttl_;
}
/**
* .google.protobuf.UInt32Value ttl = 1;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getTtlOrBuilder() {
return getTtl();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (ttl_ != null) {
output.writeMessage(1, getTtl());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (ttl_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTtl());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck)) {
return super.equals(obj);
}
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck other = (com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck) obj;
if (hasTtl() != other.hasTtl()) return false;
if (hasTtl()) {
if (!getTtl()
.equals(other.getTtl())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTtl()) {
hash = (37 * hash) + TTL_FIELD_NUMBER;
hash = (53 * hash) + getTtl().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code v1.HeartbeatHealthCheck}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:v1.HeartbeatHealthCheck)
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheckOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_HeartbeatHealthCheck_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_HeartbeatHealthCheck_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.class, com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.Builder.class);
}
// Construct using com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
if (ttlBuilder_ == null) {
ttl_ = null;
} else {
ttl_ = null;
ttlBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.internal_static_v1_HeartbeatHealthCheck_descriptor;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck getDefaultInstanceForType() {
return com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.getDefaultInstance();
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck build() {
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck buildPartial() {
com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck result = new com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck(this);
if (ttlBuilder_ == null) {
result.ttl_ = ttl_;
} else {
result.ttl_ = ttlBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck) {
return mergeFrom((com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck other) {
if (other == com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck.getDefaultInstance()) return this;
if (other.hasTtl()) {
mergeTtl(other.getTtl());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getTtlFieldBuilder().getBuilder(),
extensionRegistry);
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private com.google.protobuf.UInt32Value ttl_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> ttlBuilder_;
/**
* .google.protobuf.UInt32Value ttl = 1;
* @return Whether the ttl field is set.
*/
public boolean hasTtl() {
return ttlBuilder_ != null || ttl_ != null;
}
/**
* .google.protobuf.UInt32Value ttl = 1;
* @return The ttl.
*/
public com.google.protobuf.UInt32Value getTtl() {
if (ttlBuilder_ == null) {
return ttl_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : ttl_;
} else {
return ttlBuilder_.getMessage();
}
}
/**
* .google.protobuf.UInt32Value ttl = 1;
*/
public Builder setTtl(com.google.protobuf.UInt32Value value) {
if (ttlBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ttl_ = value;
onChanged();
} else {
ttlBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value ttl = 1;
*/
public Builder setTtl(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (ttlBuilder_ == null) {
ttl_ = builderForValue.build();
onChanged();
} else {
ttlBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.UInt32Value ttl = 1;
*/
public Builder mergeTtl(com.google.protobuf.UInt32Value value) {
if (ttlBuilder_ == null) {
if (ttl_ != null) {
ttl_ =
com.google.protobuf.UInt32Value.newBuilder(ttl_).mergeFrom(value).buildPartial();
} else {
ttl_ = value;
}
onChanged();
} else {
ttlBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.UInt32Value ttl = 1;
*/
public Builder clearTtl() {
if (ttlBuilder_ == null) {
ttl_ = null;
onChanged();
} else {
ttl_ = null;
ttlBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.UInt32Value ttl = 1;
*/
public com.google.protobuf.UInt32Value.Builder getTtlBuilder() {
onChanged();
return getTtlFieldBuilder().getBuilder();
}
/**
* .google.protobuf.UInt32Value ttl = 1;
*/
public com.google.protobuf.UInt32ValueOrBuilder getTtlOrBuilder() {
if (ttlBuilder_ != null) {
return ttlBuilder_.getMessageOrBuilder();
} else {
return ttl_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : ttl_;
}
}
/**
* .google.protobuf.UInt32Value ttl = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getTtlFieldBuilder() {
if (ttlBuilder_ == null) {
ttlBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getTtl(),
getParentForChildren(),
isClean());
ttl_ = null;
}
return ttlBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:v1.HeartbeatHealthCheck)
}
// @@protoc_insertion_point(class_scope:v1.HeartbeatHealthCheck)
private static final com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck();
}
public static com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HeartbeatHealthCheck parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.tencent.polaris.specification.api.v1.service.manage.ServiceProto.HeartbeatHealthCheck getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_v1_Service_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_v1_Service_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_v1_Service_MetadataEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_v1_Service_MetadataEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_v1_ServiceAlias_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_v1_ServiceAlias_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_v1_Instance_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_v1_Instance_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_v1_Instance_MetadataEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_v1_Instance_MetadataEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_v1_HealthCheck_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_v1_HealthCheck_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_v1_HeartbeatHealthCheck_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_v1_HeartbeatHealthCheck_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\rservice.proto\022\002v1\032\036google/protobuf/wra" +
"ppers.proto\032\013model.proto\"\237\013\n\007Service\022*\n\004" +
"name\030\001 \001(\0132\034.google.protobuf.StringValue" +
"\022/\n\tnamespace\030\002 \001(\0132\034.google.protobuf.St" +
"ringValue\022+\n\010metadata\030\003 \003(\0132\031.v1.Service" +
".MetadataEntry\022+\n\005ports\030\004 \001(\0132\034.google.p" +
"rotobuf.StringValue\022.\n\010business\030\005 \001(\0132\034." +
"google.protobuf.StringValue\0220\n\ndepartmen" +
"t\030\006 \001(\0132\034.google.protobuf.StringValue\022:\n" +
"\tcmdb_mod1\030\007 \001(\0132\034.google.protobuf.Strin" +
"gValueR\tcmdb_mod1\022:\n\tcmdb_mod2\030\010 \001(\0132\034.g" +
"oogle.protobuf.StringValueR\tcmdb_mod2\022:\n" +
"\tcmdb_mod3\030\t \001(\0132\034.google.protobuf.Strin" +
"gValueR\tcmdb_mod3\022-\n\007comment\030\n \001(\0132\034.goo" +
"gle.protobuf.StringValue\022,\n\006owners\030\013 \001(\013" +
"2\034.google.protobuf.StringValue\022+\n\005token\030" +
"\014 \001(\0132\034.google.protobuf.StringValue\022+\n\005c" +
"time\030\r \001(\0132\034.google.protobuf.StringValue" +
"\022+\n\005mtime\030\016 \001(\0132\034.google.protobuf.String" +
"Value\022.\n\010revision\030\017 \001(\0132\034.google.protobu" +
"f.StringValue\022>\n\013platform_id\030\020 \001(\0132\034.goo" +
"gle.protobuf.StringValueR\013platform_id\022P\n" +
"\024total_instance_count\030\021 \001(\0132\034.google.pro" +
"tobuf.UInt32ValueR\024total_instance_count\022" +
"T\n\026healthy_instance_count\030\022 \001(\0132\034.google" +
".protobuf.UInt32ValueR\026healthy_instance_" +
"count\0228\n\010user_ids\030\023 \003(\0132\034.google.protobu" +
"f.StringValueR\010user_ids\022:\n\tgroup_ids\030\024 \003" +
"(\0132\034.google.protobuf.StringValueR\tgroup_" +
"ids\022F\n\017remove_user_ids\030\026 \003(\0132\034.google.pr" +
"otobuf.StringValueR\017remove_user_ids\022H\n\020r" +
"emove_group_ids\030\027 \003(\0132\034.google.protobuf." +
"StringValueR\020remove_group_ids\022(\n\002id\030\025 \001(" +
"\0132\034.google.protobuf.StringValue\022,\n\010edita" +
"ble\030\030 \001(\0132\032.google.protobuf.BoolValue\022:\n" +
"\texport_to\030\031 \003(\0132\034.google.protobuf.Strin" +
"gValueR\texport_to\032/\n\rMetadataEntry\022\013\n\003ke" +
"y\030\001 \001(\t\022\r\n\005value\030\002 \001(\t:\0028\001\"\323\004\n\014ServiceAl" +
"ias\022-\n\007service\030\001 \001(\0132\034.google.protobuf.S" +
"tringValue\022/\n\tnamespace\030\002 \001(\0132\034.google.p" +
"rotobuf.StringValue\022+\n\005alias\030\003 \001(\0132\034.goo" +
"gle.protobuf.StringValue\022F\n\017alias_namesp" +
"ace\030\004 \001(\0132\034.google.protobuf.StringValueR" +
"\017alias_namespace\022\033\n\004type\030\005 \001(\0162\r.v1.Alia" +
"sType\022,\n\006owners\030\006 \001(\0132\034.google.protobuf." +
"StringValue\022-\n\007comment\030\007 \001(\0132\034.google.pr" +
"otobuf.StringValue\022B\n\rservice_token\030\010 \001(" +
"\0132\034.google.protobuf.StringValueR\rservice" +
"_token\022+\n\005ctime\030\t \001(\0132\034.google.protobuf." +
"StringValue\022+\n\005mtime\030\n \001(\0132\034.google.prot" +
"obuf.StringValue\022(\n\002id\030\013 \001(\0132\034.google.pr" +
"otobuf.StringValue\022,\n\010editable\030\014 \001(\0132\032.g" +
"oogle.protobuf.BoolValue\"\242\010\n\010Instance\022(\n" +
"\002id\030\001 \001(\0132\034.google.protobuf.StringValue\022" +
"-\n\007service\030\002 \001(\0132\034.google.protobuf.Strin" +
"gValue\022/\n\tnamespace\030\003 \001(\0132\034.google.proto" +
"buf.StringValue\0224\n\006vpc_id\030\025 \001(\0132\034.google" +
".protobuf.StringValueR\006vpc_id\022*\n\004host\030\004 " +
"\001(\0132\034.google.protobuf.StringValue\022*\n\004por" +
"t\030\005 \001(\0132\034.google.protobuf.UInt32Value\022.\n" +
"\010protocol\030\006 \001(\0132\034.google.protobuf.String" +
"Value\022-\n\007version\030\007 \001(\0132\034.google.protobuf" +
".StringValue\022.\n\010priority\030\010 \001(\0132\034.google." +
"protobuf.UInt32Value\022,\n\006weight\030\t \001(\0132\034.g" +
"oogle.protobuf.UInt32Value\0227\n\023enable_hea" +
"lth_check\030\024 \001(\0132\032.google.protobuf.BoolVa" +
"lue\022%\n\014health_check\030\n \001(\0132\017.v1.HealthChe" +
"ck\022+\n\007healthy\030\013 \001(\0132\032.google.protobuf.Bo" +
"olValue\022+\n\007isolate\030\014 \001(\0132\032.google.protob" +
"uf.BoolValue\022\036\n\010location\030\r \001(\0132\014.v1.Loca" +
"tion\022,\n\010metadata\030\016 \003(\0132\032.v1.Instance.Met" +
"adataEntry\022:\n\tlogic_set\030\017 \001(\0132\034.google.p" +
"rotobuf.StringValueR\tlogic_set\022+\n\005ctime\030" +
"\020 \001(\0132\034.google.protobuf.StringValue\022+\n\005m" +
"time\030\021 \001(\0132\034.google.protobuf.StringValue" +
"\022.\n\010revision\030\022 \001(\0132\034.google.protobuf.Str" +
"ingValue\022B\n\rservice_token\030\023 \001(\0132\034.google" +
".protobuf.StringValueR\rservice_token\032/\n\r" +
"MetadataEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(" +
"\t:\0028\001\"\230\001\n\013HealthCheck\022-\n\004type\030\001 \001(\0162\037.v1" +
".HealthCheck.HealthCheckType\022+\n\theartbea" +
"t\030\002 \001(\0132\030.v1.HeartbeatHealthCheck\"-\n\017Hea" +
"lthCheckType\022\013\n\007UNKNOWN\020\000\022\r\n\tHEARTBEAT\020\001" +
"\"A\n\024HeartbeatHealthCheck\022)\n\003ttl\030\001 \001(\0132\034." +
"google.protobuf.UInt32Value*$\n\tAliasType" +
"\022\013\n\007DEFAULT\020\000\022\n\n\006CL5SID\020\001B\215\001\n7com.tencen" +
"t.polaris.specification.api.v1.service.m" +
"anageB\014ServiceProtoZDgithub.com/polarism" +
"esh/specification/source/go/api/v1/servi" +
"ce_manageb\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.WrappersProto.getDescriptor(),
com.tencent.polaris.specification.api.v1.model.ModelProto.getDescriptor(),
});
internal_static_v1_Service_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_v1_Service_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_v1_Service_descriptor,
new java.lang.String[] { "Name", "Namespace", "Metadata", "Ports", "Business", "Department", "CmdbMod1", "CmdbMod2", "CmdbMod3", "Comment", "Owners", "Token", "Ctime", "Mtime", "Revision", "PlatformId", "TotalInstanceCount", "HealthyInstanceCount", "UserIds", "GroupIds", "RemoveUserIds", "RemoveGroupIds", "Id", "Editable", "ExportTo", });
internal_static_v1_Service_MetadataEntry_descriptor =
internal_static_v1_Service_descriptor.getNestedTypes().get(0);
internal_static_v1_Service_MetadataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_v1_Service_MetadataEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_v1_ServiceAlias_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_v1_ServiceAlias_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_v1_ServiceAlias_descriptor,
new java.lang.String[] { "Service", "Namespace", "Alias", "AliasNamespace", "Type", "Owners", "Comment", "ServiceToken", "Ctime", "Mtime", "Id", "Editable", });
internal_static_v1_Instance_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_v1_Instance_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_v1_Instance_descriptor,
new java.lang.String[] { "Id", "Service", "Namespace", "VpcId", "Host", "Port", "Protocol", "Version", "Priority", "Weight", "EnableHealthCheck", "HealthCheck", "Healthy", "Isolate", "Location", "Metadata", "LogicSet", "Ctime", "Mtime", "Revision", "ServiceToken", });
internal_static_v1_Instance_MetadataEntry_descriptor =
internal_static_v1_Instance_descriptor.getNestedTypes().get(0);
internal_static_v1_Instance_MetadataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_v1_Instance_MetadataEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_v1_HealthCheck_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_v1_HealthCheck_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_v1_HealthCheck_descriptor,
new java.lang.String[] { "Type", "Heartbeat", });
internal_static_v1_HeartbeatHealthCheck_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_v1_HeartbeatHealthCheck_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_v1_HeartbeatHealthCheck_descriptor,
new java.lang.String[] { "Ttl", });
com.google.protobuf.WrappersProto.getDescriptor();
com.tencent.polaris.specification.api.v1.model.ModelProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy