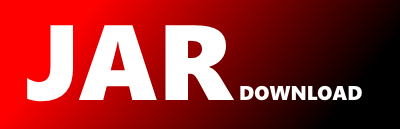
shade.polaris.io.grpc.channelz.v1.SocketData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of polaris-all Show documentation
Show all versions of polaris-all Show documentation
All in one project for polaris-java
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: grpc/channelz/v1/channelz.proto
package io.grpc.channelz.v1;
/**
*
* SocketData is data associated for a specific Socket. The fields present
* are specific to the implementation, so there may be minor differences in
* the semantics. (e.g. flow control windows)
*
*
* Protobuf type {@code grpc.channelz.v1.SocketData}
*/
public final class SocketData extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:grpc.channelz.v1.SocketData)
SocketDataOrBuilder {
private static final long serialVersionUID = 0L;
// Use SocketData.newBuilder() to construct.
private SocketData(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SocketData() {
option_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SocketData();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.channelz.v1.ChannelzProto.internal_static_grpc_channelz_v1_SocketData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.channelz.v1.ChannelzProto.internal_static_grpc_channelz_v1_SocketData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.channelz.v1.SocketData.class, io.grpc.channelz.v1.SocketData.Builder.class);
}
private int bitField0_;
public static final int STREAMS_STARTED_FIELD_NUMBER = 1;
private long streamsStarted_ = 0L;
/**
*
* The number of streams that have been started.
*
*
* int64 streams_started = 1;
* @return The streamsStarted.
*/
@java.lang.Override
public long getStreamsStarted() {
return streamsStarted_;
}
public static final int STREAMS_SUCCEEDED_FIELD_NUMBER = 2;
private long streamsSucceeded_ = 0L;
/**
*
* The number of streams that have ended successfully:
* On client side, received frame with eos bit set;
* On server side, sent frame with eos bit set.
*
*
* int64 streams_succeeded = 2;
* @return The streamsSucceeded.
*/
@java.lang.Override
public long getStreamsSucceeded() {
return streamsSucceeded_;
}
public static final int STREAMS_FAILED_FIELD_NUMBER = 3;
private long streamsFailed_ = 0L;
/**
*
* The number of streams that have ended unsuccessfully:
* On client side, ended without receiving frame with eos bit set;
* On server side, ended without sending frame with eos bit set.
*
*
* int64 streams_failed = 3;
* @return The streamsFailed.
*/
@java.lang.Override
public long getStreamsFailed() {
return streamsFailed_;
}
public static final int MESSAGES_SENT_FIELD_NUMBER = 4;
private long messagesSent_ = 0L;
/**
*
* The number of grpc messages successfully sent on this socket.
*
*
* int64 messages_sent = 4;
* @return The messagesSent.
*/
@java.lang.Override
public long getMessagesSent() {
return messagesSent_;
}
public static final int MESSAGES_RECEIVED_FIELD_NUMBER = 5;
private long messagesReceived_ = 0L;
/**
*
* The number of grpc messages received on this socket.
*
*
* int64 messages_received = 5;
* @return The messagesReceived.
*/
@java.lang.Override
public long getMessagesReceived() {
return messagesReceived_;
}
public static final int KEEP_ALIVES_SENT_FIELD_NUMBER = 6;
private long keepAlivesSent_ = 0L;
/**
*
* The number of keep alives sent. This is typically implemented with HTTP/2
* ping messages.
*
*
* int64 keep_alives_sent = 6;
* @return The keepAlivesSent.
*/
@java.lang.Override
public long getKeepAlivesSent() {
return keepAlivesSent_;
}
public static final int LAST_LOCAL_STREAM_CREATED_TIMESTAMP_FIELD_NUMBER = 7;
private com.google.protobuf.Timestamp lastLocalStreamCreatedTimestamp_;
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
* @return Whether the lastLocalStreamCreatedTimestamp field is set.
*/
@java.lang.Override
public boolean hasLastLocalStreamCreatedTimestamp() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
* @return The lastLocalStreamCreatedTimestamp.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getLastLocalStreamCreatedTimestamp() {
return lastLocalStreamCreatedTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastLocalStreamCreatedTimestamp_;
}
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getLastLocalStreamCreatedTimestampOrBuilder() {
return lastLocalStreamCreatedTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastLocalStreamCreatedTimestamp_;
}
public static final int LAST_REMOTE_STREAM_CREATED_TIMESTAMP_FIELD_NUMBER = 8;
private com.google.protobuf.Timestamp lastRemoteStreamCreatedTimestamp_;
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
* @return Whether the lastRemoteStreamCreatedTimestamp field is set.
*/
@java.lang.Override
public boolean hasLastRemoteStreamCreatedTimestamp() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
* @return The lastRemoteStreamCreatedTimestamp.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getLastRemoteStreamCreatedTimestamp() {
return lastRemoteStreamCreatedTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastRemoteStreamCreatedTimestamp_;
}
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getLastRemoteStreamCreatedTimestampOrBuilder() {
return lastRemoteStreamCreatedTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastRemoteStreamCreatedTimestamp_;
}
public static final int LAST_MESSAGE_SENT_TIMESTAMP_FIELD_NUMBER = 9;
private com.google.protobuf.Timestamp lastMessageSentTimestamp_;
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
* @return Whether the lastMessageSentTimestamp field is set.
*/
@java.lang.Override
public boolean hasLastMessageSentTimestamp() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
* @return The lastMessageSentTimestamp.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getLastMessageSentTimestamp() {
return lastMessageSentTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastMessageSentTimestamp_;
}
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getLastMessageSentTimestampOrBuilder() {
return lastMessageSentTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastMessageSentTimestamp_;
}
public static final int LAST_MESSAGE_RECEIVED_TIMESTAMP_FIELD_NUMBER = 10;
private com.google.protobuf.Timestamp lastMessageReceivedTimestamp_;
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
* @return Whether the lastMessageReceivedTimestamp field is set.
*/
@java.lang.Override
public boolean hasLastMessageReceivedTimestamp() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
* @return The lastMessageReceivedTimestamp.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getLastMessageReceivedTimestamp() {
return lastMessageReceivedTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastMessageReceivedTimestamp_;
}
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getLastMessageReceivedTimestampOrBuilder() {
return lastMessageReceivedTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastMessageReceivedTimestamp_;
}
public static final int LOCAL_FLOW_CONTROL_WINDOW_FIELD_NUMBER = 11;
private com.google.protobuf.Int64Value localFlowControlWindow_;
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
* @return Whether the localFlowControlWindow field is set.
*/
@java.lang.Override
public boolean hasLocalFlowControlWindow() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
* @return The localFlowControlWindow.
*/
@java.lang.Override
public com.google.protobuf.Int64Value getLocalFlowControlWindow() {
return localFlowControlWindow_ == null ? com.google.protobuf.Int64Value.getDefaultInstance() : localFlowControlWindow_;
}
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
*/
@java.lang.Override
public com.google.protobuf.Int64ValueOrBuilder getLocalFlowControlWindowOrBuilder() {
return localFlowControlWindow_ == null ? com.google.protobuf.Int64Value.getDefaultInstance() : localFlowControlWindow_;
}
public static final int REMOTE_FLOW_CONTROL_WINDOW_FIELD_NUMBER = 12;
private com.google.protobuf.Int64Value remoteFlowControlWindow_;
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
* @return Whether the remoteFlowControlWindow field is set.
*/
@java.lang.Override
public boolean hasRemoteFlowControlWindow() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
* @return The remoteFlowControlWindow.
*/
@java.lang.Override
public com.google.protobuf.Int64Value getRemoteFlowControlWindow() {
return remoteFlowControlWindow_ == null ? com.google.protobuf.Int64Value.getDefaultInstance() : remoteFlowControlWindow_;
}
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
*/
@java.lang.Override
public com.google.protobuf.Int64ValueOrBuilder getRemoteFlowControlWindowOrBuilder() {
return remoteFlowControlWindow_ == null ? com.google.protobuf.Int64Value.getDefaultInstance() : remoteFlowControlWindow_;
}
public static final int OPTION_FIELD_NUMBER = 13;
@SuppressWarnings("serial")
private java.util.List option_;
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
@java.lang.Override
public java.util.List getOptionList() {
return option_;
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
@java.lang.Override
public java.util.List extends io.grpc.channelz.v1.SocketOptionOrBuilder>
getOptionOrBuilderList() {
return option_;
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
@java.lang.Override
public int getOptionCount() {
return option_.size();
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
@java.lang.Override
public io.grpc.channelz.v1.SocketOption getOption(int index) {
return option_.get(index);
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
@java.lang.Override
public io.grpc.channelz.v1.SocketOptionOrBuilder getOptionOrBuilder(
int index) {
return option_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (streamsStarted_ != 0L) {
output.writeInt64(1, streamsStarted_);
}
if (streamsSucceeded_ != 0L) {
output.writeInt64(2, streamsSucceeded_);
}
if (streamsFailed_ != 0L) {
output.writeInt64(3, streamsFailed_);
}
if (messagesSent_ != 0L) {
output.writeInt64(4, messagesSent_);
}
if (messagesReceived_ != 0L) {
output.writeInt64(5, messagesReceived_);
}
if (keepAlivesSent_ != 0L) {
output.writeInt64(6, keepAlivesSent_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(7, getLastLocalStreamCreatedTimestamp());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(8, getLastRemoteStreamCreatedTimestamp());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(9, getLastMessageSentTimestamp());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(10, getLastMessageReceivedTimestamp());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(11, getLocalFlowControlWindow());
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(12, getRemoteFlowControlWindow());
}
for (int i = 0; i < option_.size(); i++) {
output.writeMessage(13, option_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (streamsStarted_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, streamsStarted_);
}
if (streamsSucceeded_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, streamsSucceeded_);
}
if (streamsFailed_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, streamsFailed_);
}
if (messagesSent_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, messagesSent_);
}
if (messagesReceived_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, messagesReceived_);
}
if (keepAlivesSent_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, keepAlivesSent_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getLastLocalStreamCreatedTimestamp());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getLastRemoteStreamCreatedTimestamp());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getLastMessageSentTimestamp());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getLastMessageReceivedTimestamp());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getLocalFlowControlWindow());
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getRemoteFlowControlWindow());
}
for (int i = 0; i < option_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, option_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.grpc.channelz.v1.SocketData)) {
return super.equals(obj);
}
io.grpc.channelz.v1.SocketData other = (io.grpc.channelz.v1.SocketData) obj;
if (getStreamsStarted()
!= other.getStreamsStarted()) return false;
if (getStreamsSucceeded()
!= other.getStreamsSucceeded()) return false;
if (getStreamsFailed()
!= other.getStreamsFailed()) return false;
if (getMessagesSent()
!= other.getMessagesSent()) return false;
if (getMessagesReceived()
!= other.getMessagesReceived()) return false;
if (getKeepAlivesSent()
!= other.getKeepAlivesSent()) return false;
if (hasLastLocalStreamCreatedTimestamp() != other.hasLastLocalStreamCreatedTimestamp()) return false;
if (hasLastLocalStreamCreatedTimestamp()) {
if (!getLastLocalStreamCreatedTimestamp()
.equals(other.getLastLocalStreamCreatedTimestamp())) return false;
}
if (hasLastRemoteStreamCreatedTimestamp() != other.hasLastRemoteStreamCreatedTimestamp()) return false;
if (hasLastRemoteStreamCreatedTimestamp()) {
if (!getLastRemoteStreamCreatedTimestamp()
.equals(other.getLastRemoteStreamCreatedTimestamp())) return false;
}
if (hasLastMessageSentTimestamp() != other.hasLastMessageSentTimestamp()) return false;
if (hasLastMessageSentTimestamp()) {
if (!getLastMessageSentTimestamp()
.equals(other.getLastMessageSentTimestamp())) return false;
}
if (hasLastMessageReceivedTimestamp() != other.hasLastMessageReceivedTimestamp()) return false;
if (hasLastMessageReceivedTimestamp()) {
if (!getLastMessageReceivedTimestamp()
.equals(other.getLastMessageReceivedTimestamp())) return false;
}
if (hasLocalFlowControlWindow() != other.hasLocalFlowControlWindow()) return false;
if (hasLocalFlowControlWindow()) {
if (!getLocalFlowControlWindow()
.equals(other.getLocalFlowControlWindow())) return false;
}
if (hasRemoteFlowControlWindow() != other.hasRemoteFlowControlWindow()) return false;
if (hasRemoteFlowControlWindow()) {
if (!getRemoteFlowControlWindow()
.equals(other.getRemoteFlowControlWindow())) return false;
}
if (!getOptionList()
.equals(other.getOptionList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STREAMS_STARTED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStreamsStarted());
hash = (37 * hash) + STREAMS_SUCCEEDED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStreamsSucceeded());
hash = (37 * hash) + STREAMS_FAILED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStreamsFailed());
hash = (37 * hash) + MESSAGES_SENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMessagesSent());
hash = (37 * hash) + MESSAGES_RECEIVED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMessagesReceived());
hash = (37 * hash) + KEEP_ALIVES_SENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getKeepAlivesSent());
if (hasLastLocalStreamCreatedTimestamp()) {
hash = (37 * hash) + LAST_LOCAL_STREAM_CREATED_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getLastLocalStreamCreatedTimestamp().hashCode();
}
if (hasLastRemoteStreamCreatedTimestamp()) {
hash = (37 * hash) + LAST_REMOTE_STREAM_CREATED_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getLastRemoteStreamCreatedTimestamp().hashCode();
}
if (hasLastMessageSentTimestamp()) {
hash = (37 * hash) + LAST_MESSAGE_SENT_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getLastMessageSentTimestamp().hashCode();
}
if (hasLastMessageReceivedTimestamp()) {
hash = (37 * hash) + LAST_MESSAGE_RECEIVED_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getLastMessageReceivedTimestamp().hashCode();
}
if (hasLocalFlowControlWindow()) {
hash = (37 * hash) + LOCAL_FLOW_CONTROL_WINDOW_FIELD_NUMBER;
hash = (53 * hash) + getLocalFlowControlWindow().hashCode();
}
if (hasRemoteFlowControlWindow()) {
hash = (37 * hash) + REMOTE_FLOW_CONTROL_WINDOW_FIELD_NUMBER;
hash = (53 * hash) + getRemoteFlowControlWindow().hashCode();
}
if (getOptionCount() > 0) {
hash = (37 * hash) + OPTION_FIELD_NUMBER;
hash = (53 * hash) + getOptionList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.grpc.channelz.v1.SocketData parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.channelz.v1.SocketData parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.channelz.v1.SocketData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.channelz.v1.SocketData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.channelz.v1.SocketData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.channelz.v1.SocketData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.channelz.v1.SocketData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.channelz.v1.SocketData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.channelz.v1.SocketData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.grpc.channelz.v1.SocketData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.channelz.v1.SocketData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.channelz.v1.SocketData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.grpc.channelz.v1.SocketData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* SocketData is data associated for a specific Socket. The fields present
* are specific to the implementation, so there may be minor differences in
* the semantics. (e.g. flow control windows)
*
*
* Protobuf type {@code grpc.channelz.v1.SocketData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:grpc.channelz.v1.SocketData)
io.grpc.channelz.v1.SocketDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.channelz.v1.ChannelzProto.internal_static_grpc_channelz_v1_SocketData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.channelz.v1.ChannelzProto.internal_static_grpc_channelz_v1_SocketData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.channelz.v1.SocketData.class, io.grpc.channelz.v1.SocketData.Builder.class);
}
// Construct using io.grpc.channelz.v1.SocketData.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getLastLocalStreamCreatedTimestampFieldBuilder();
getLastRemoteStreamCreatedTimestampFieldBuilder();
getLastMessageSentTimestampFieldBuilder();
getLastMessageReceivedTimestampFieldBuilder();
getLocalFlowControlWindowFieldBuilder();
getRemoteFlowControlWindowFieldBuilder();
getOptionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
streamsStarted_ = 0L;
streamsSucceeded_ = 0L;
streamsFailed_ = 0L;
messagesSent_ = 0L;
messagesReceived_ = 0L;
keepAlivesSent_ = 0L;
lastLocalStreamCreatedTimestamp_ = null;
if (lastLocalStreamCreatedTimestampBuilder_ != null) {
lastLocalStreamCreatedTimestampBuilder_.dispose();
lastLocalStreamCreatedTimestampBuilder_ = null;
}
lastRemoteStreamCreatedTimestamp_ = null;
if (lastRemoteStreamCreatedTimestampBuilder_ != null) {
lastRemoteStreamCreatedTimestampBuilder_.dispose();
lastRemoteStreamCreatedTimestampBuilder_ = null;
}
lastMessageSentTimestamp_ = null;
if (lastMessageSentTimestampBuilder_ != null) {
lastMessageSentTimestampBuilder_.dispose();
lastMessageSentTimestampBuilder_ = null;
}
lastMessageReceivedTimestamp_ = null;
if (lastMessageReceivedTimestampBuilder_ != null) {
lastMessageReceivedTimestampBuilder_.dispose();
lastMessageReceivedTimestampBuilder_ = null;
}
localFlowControlWindow_ = null;
if (localFlowControlWindowBuilder_ != null) {
localFlowControlWindowBuilder_.dispose();
localFlowControlWindowBuilder_ = null;
}
remoteFlowControlWindow_ = null;
if (remoteFlowControlWindowBuilder_ != null) {
remoteFlowControlWindowBuilder_.dispose();
remoteFlowControlWindowBuilder_ = null;
}
if (optionBuilder_ == null) {
option_ = java.util.Collections.emptyList();
} else {
option_ = null;
optionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00001000);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.grpc.channelz.v1.ChannelzProto.internal_static_grpc_channelz_v1_SocketData_descriptor;
}
@java.lang.Override
public io.grpc.channelz.v1.SocketData getDefaultInstanceForType() {
return io.grpc.channelz.v1.SocketData.getDefaultInstance();
}
@java.lang.Override
public io.grpc.channelz.v1.SocketData build() {
io.grpc.channelz.v1.SocketData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.grpc.channelz.v1.SocketData buildPartial() {
io.grpc.channelz.v1.SocketData result = new io.grpc.channelz.v1.SocketData(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(io.grpc.channelz.v1.SocketData result) {
if (optionBuilder_ == null) {
if (((bitField0_ & 0x00001000) != 0)) {
option_ = java.util.Collections.unmodifiableList(option_);
bitField0_ = (bitField0_ & ~0x00001000);
}
result.option_ = option_;
} else {
result.option_ = optionBuilder_.build();
}
}
private void buildPartial0(io.grpc.channelz.v1.SocketData result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.streamsStarted_ = streamsStarted_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.streamsSucceeded_ = streamsSucceeded_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.streamsFailed_ = streamsFailed_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.messagesSent_ = messagesSent_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.messagesReceived_ = messagesReceived_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.keepAlivesSent_ = keepAlivesSent_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000040) != 0)) {
result.lastLocalStreamCreatedTimestamp_ = lastLocalStreamCreatedTimestampBuilder_ == null
? lastLocalStreamCreatedTimestamp_
: lastLocalStreamCreatedTimestampBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.lastRemoteStreamCreatedTimestamp_ = lastRemoteStreamCreatedTimestampBuilder_ == null
? lastRemoteStreamCreatedTimestamp_
: lastRemoteStreamCreatedTimestampBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.lastMessageSentTimestamp_ = lastMessageSentTimestampBuilder_ == null
? lastMessageSentTimestamp_
: lastMessageSentTimestampBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.lastMessageReceivedTimestamp_ = lastMessageReceivedTimestampBuilder_ == null
? lastMessageReceivedTimestamp_
: lastMessageReceivedTimestampBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.localFlowControlWindow_ = localFlowControlWindowBuilder_ == null
? localFlowControlWindow_
: localFlowControlWindowBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.remoteFlowControlWindow_ = remoteFlowControlWindowBuilder_ == null
? remoteFlowControlWindow_
: remoteFlowControlWindowBuilder_.build();
to_bitField0_ |= 0x00000020;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.grpc.channelz.v1.SocketData) {
return mergeFrom((io.grpc.channelz.v1.SocketData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.grpc.channelz.v1.SocketData other) {
if (other == io.grpc.channelz.v1.SocketData.getDefaultInstance()) return this;
if (other.getStreamsStarted() != 0L) {
setStreamsStarted(other.getStreamsStarted());
}
if (other.getStreamsSucceeded() != 0L) {
setStreamsSucceeded(other.getStreamsSucceeded());
}
if (other.getStreamsFailed() != 0L) {
setStreamsFailed(other.getStreamsFailed());
}
if (other.getMessagesSent() != 0L) {
setMessagesSent(other.getMessagesSent());
}
if (other.getMessagesReceived() != 0L) {
setMessagesReceived(other.getMessagesReceived());
}
if (other.getKeepAlivesSent() != 0L) {
setKeepAlivesSent(other.getKeepAlivesSent());
}
if (other.hasLastLocalStreamCreatedTimestamp()) {
mergeLastLocalStreamCreatedTimestamp(other.getLastLocalStreamCreatedTimestamp());
}
if (other.hasLastRemoteStreamCreatedTimestamp()) {
mergeLastRemoteStreamCreatedTimestamp(other.getLastRemoteStreamCreatedTimestamp());
}
if (other.hasLastMessageSentTimestamp()) {
mergeLastMessageSentTimestamp(other.getLastMessageSentTimestamp());
}
if (other.hasLastMessageReceivedTimestamp()) {
mergeLastMessageReceivedTimestamp(other.getLastMessageReceivedTimestamp());
}
if (other.hasLocalFlowControlWindow()) {
mergeLocalFlowControlWindow(other.getLocalFlowControlWindow());
}
if (other.hasRemoteFlowControlWindow()) {
mergeRemoteFlowControlWindow(other.getRemoteFlowControlWindow());
}
if (optionBuilder_ == null) {
if (!other.option_.isEmpty()) {
if (option_.isEmpty()) {
option_ = other.option_;
bitField0_ = (bitField0_ & ~0x00001000);
} else {
ensureOptionIsMutable();
option_.addAll(other.option_);
}
onChanged();
}
} else {
if (!other.option_.isEmpty()) {
if (optionBuilder_.isEmpty()) {
optionBuilder_.dispose();
optionBuilder_ = null;
option_ = other.option_;
bitField0_ = (bitField0_ & ~0x00001000);
optionBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOptionFieldBuilder() : null;
} else {
optionBuilder_.addAllMessages(other.option_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
streamsStarted_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
streamsSucceeded_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
streamsFailed_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
messagesSent_ = input.readInt64();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
messagesReceived_ = input.readInt64();
bitField0_ |= 0x00000010;
break;
} // case 40
case 48: {
keepAlivesSent_ = input.readInt64();
bitField0_ |= 0x00000020;
break;
} // case 48
case 58: {
input.readMessage(
getLastLocalStreamCreatedTimestampFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 58
case 66: {
input.readMessage(
getLastRemoteStreamCreatedTimestampFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 66
case 74: {
input.readMessage(
getLastMessageSentTimestampFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 74
case 82: {
input.readMessage(
getLastMessageReceivedTimestampFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000200;
break;
} // case 82
case 90: {
input.readMessage(
getLocalFlowControlWindowFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000400;
break;
} // case 90
case 98: {
input.readMessage(
getRemoteFlowControlWindowFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000800;
break;
} // case 98
case 106: {
io.grpc.channelz.v1.SocketOption m =
input.readMessage(
io.grpc.channelz.v1.SocketOption.parser(),
extensionRegistry);
if (optionBuilder_ == null) {
ensureOptionIsMutable();
option_.add(m);
} else {
optionBuilder_.addMessage(m);
}
break;
} // case 106
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long streamsStarted_ ;
/**
*
* The number of streams that have been started.
*
*
* int64 streams_started = 1;
* @return The streamsStarted.
*/
@java.lang.Override
public long getStreamsStarted() {
return streamsStarted_;
}
/**
*
* The number of streams that have been started.
*
*
* int64 streams_started = 1;
* @param value The streamsStarted to set.
* @return This builder for chaining.
*/
public Builder setStreamsStarted(long value) {
streamsStarted_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The number of streams that have been started.
*
*
* int64 streams_started = 1;
* @return This builder for chaining.
*/
public Builder clearStreamsStarted() {
bitField0_ = (bitField0_ & ~0x00000001);
streamsStarted_ = 0L;
onChanged();
return this;
}
private long streamsSucceeded_ ;
/**
*
* The number of streams that have ended successfully:
* On client side, received frame with eos bit set;
* On server side, sent frame with eos bit set.
*
*
* int64 streams_succeeded = 2;
* @return The streamsSucceeded.
*/
@java.lang.Override
public long getStreamsSucceeded() {
return streamsSucceeded_;
}
/**
*
* The number of streams that have ended successfully:
* On client side, received frame with eos bit set;
* On server side, sent frame with eos bit set.
*
*
* int64 streams_succeeded = 2;
* @param value The streamsSucceeded to set.
* @return This builder for chaining.
*/
public Builder setStreamsSucceeded(long value) {
streamsSucceeded_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The number of streams that have ended successfully:
* On client side, received frame with eos bit set;
* On server side, sent frame with eos bit set.
*
*
* int64 streams_succeeded = 2;
* @return This builder for chaining.
*/
public Builder clearStreamsSucceeded() {
bitField0_ = (bitField0_ & ~0x00000002);
streamsSucceeded_ = 0L;
onChanged();
return this;
}
private long streamsFailed_ ;
/**
*
* The number of streams that have ended unsuccessfully:
* On client side, ended without receiving frame with eos bit set;
* On server side, ended without sending frame with eos bit set.
*
*
* int64 streams_failed = 3;
* @return The streamsFailed.
*/
@java.lang.Override
public long getStreamsFailed() {
return streamsFailed_;
}
/**
*
* The number of streams that have ended unsuccessfully:
* On client side, ended without receiving frame with eos bit set;
* On server side, ended without sending frame with eos bit set.
*
*
* int64 streams_failed = 3;
* @param value The streamsFailed to set.
* @return This builder for chaining.
*/
public Builder setStreamsFailed(long value) {
streamsFailed_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The number of streams that have ended unsuccessfully:
* On client side, ended without receiving frame with eos bit set;
* On server side, ended without sending frame with eos bit set.
*
*
* int64 streams_failed = 3;
* @return This builder for chaining.
*/
public Builder clearStreamsFailed() {
bitField0_ = (bitField0_ & ~0x00000004);
streamsFailed_ = 0L;
onChanged();
return this;
}
private long messagesSent_ ;
/**
*
* The number of grpc messages successfully sent on this socket.
*
*
* int64 messages_sent = 4;
* @return The messagesSent.
*/
@java.lang.Override
public long getMessagesSent() {
return messagesSent_;
}
/**
*
* The number of grpc messages successfully sent on this socket.
*
*
* int64 messages_sent = 4;
* @param value The messagesSent to set.
* @return This builder for chaining.
*/
public Builder setMessagesSent(long value) {
messagesSent_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The number of grpc messages successfully sent on this socket.
*
*
* int64 messages_sent = 4;
* @return This builder for chaining.
*/
public Builder clearMessagesSent() {
bitField0_ = (bitField0_ & ~0x00000008);
messagesSent_ = 0L;
onChanged();
return this;
}
private long messagesReceived_ ;
/**
*
* The number of grpc messages received on this socket.
*
*
* int64 messages_received = 5;
* @return The messagesReceived.
*/
@java.lang.Override
public long getMessagesReceived() {
return messagesReceived_;
}
/**
*
* The number of grpc messages received on this socket.
*
*
* int64 messages_received = 5;
* @param value The messagesReceived to set.
* @return This builder for chaining.
*/
public Builder setMessagesReceived(long value) {
messagesReceived_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The number of grpc messages received on this socket.
*
*
* int64 messages_received = 5;
* @return This builder for chaining.
*/
public Builder clearMessagesReceived() {
bitField0_ = (bitField0_ & ~0x00000010);
messagesReceived_ = 0L;
onChanged();
return this;
}
private long keepAlivesSent_ ;
/**
*
* The number of keep alives sent. This is typically implemented with HTTP/2
* ping messages.
*
*
* int64 keep_alives_sent = 6;
* @return The keepAlivesSent.
*/
@java.lang.Override
public long getKeepAlivesSent() {
return keepAlivesSent_;
}
/**
*
* The number of keep alives sent. This is typically implemented with HTTP/2
* ping messages.
*
*
* int64 keep_alives_sent = 6;
* @param value The keepAlivesSent to set.
* @return This builder for chaining.
*/
public Builder setKeepAlivesSent(long value) {
keepAlivesSent_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* The number of keep alives sent. This is typically implemented with HTTP/2
* ping messages.
*
*
* int64 keep_alives_sent = 6;
* @return This builder for chaining.
*/
public Builder clearKeepAlivesSent() {
bitField0_ = (bitField0_ & ~0x00000020);
keepAlivesSent_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.Timestamp lastLocalStreamCreatedTimestamp_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> lastLocalStreamCreatedTimestampBuilder_;
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
* @return Whether the lastLocalStreamCreatedTimestamp field is set.
*/
public boolean hasLastLocalStreamCreatedTimestamp() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
* @return The lastLocalStreamCreatedTimestamp.
*/
public com.google.protobuf.Timestamp getLastLocalStreamCreatedTimestamp() {
if (lastLocalStreamCreatedTimestampBuilder_ == null) {
return lastLocalStreamCreatedTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastLocalStreamCreatedTimestamp_;
} else {
return lastLocalStreamCreatedTimestampBuilder_.getMessage();
}
}
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
*/
public Builder setLastLocalStreamCreatedTimestamp(com.google.protobuf.Timestamp value) {
if (lastLocalStreamCreatedTimestampBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lastLocalStreamCreatedTimestamp_ = value;
} else {
lastLocalStreamCreatedTimestampBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
*/
public Builder setLastLocalStreamCreatedTimestamp(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (lastLocalStreamCreatedTimestampBuilder_ == null) {
lastLocalStreamCreatedTimestamp_ = builderForValue.build();
} else {
lastLocalStreamCreatedTimestampBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
*/
public Builder mergeLastLocalStreamCreatedTimestamp(com.google.protobuf.Timestamp value) {
if (lastLocalStreamCreatedTimestampBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0) &&
lastLocalStreamCreatedTimestamp_ != null &&
lastLocalStreamCreatedTimestamp_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getLastLocalStreamCreatedTimestampBuilder().mergeFrom(value);
} else {
lastLocalStreamCreatedTimestamp_ = value;
}
} else {
lastLocalStreamCreatedTimestampBuilder_.mergeFrom(value);
}
if (lastLocalStreamCreatedTimestamp_ != null) {
bitField0_ |= 0x00000040;
onChanged();
}
return this;
}
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
*/
public Builder clearLastLocalStreamCreatedTimestamp() {
bitField0_ = (bitField0_ & ~0x00000040);
lastLocalStreamCreatedTimestamp_ = null;
if (lastLocalStreamCreatedTimestampBuilder_ != null) {
lastLocalStreamCreatedTimestampBuilder_.dispose();
lastLocalStreamCreatedTimestampBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
*/
public com.google.protobuf.Timestamp.Builder getLastLocalStreamCreatedTimestampBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getLastLocalStreamCreatedTimestampFieldBuilder().getBuilder();
}
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
*/
public com.google.protobuf.TimestampOrBuilder getLastLocalStreamCreatedTimestampOrBuilder() {
if (lastLocalStreamCreatedTimestampBuilder_ != null) {
return lastLocalStreamCreatedTimestampBuilder_.getMessageOrBuilder();
} else {
return lastLocalStreamCreatedTimestamp_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : lastLocalStreamCreatedTimestamp_;
}
}
/**
*
* The last time a stream was created by this endpoint. Usually unset for
* servers.
*
*
* .google.protobuf.Timestamp last_local_stream_created_timestamp = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getLastLocalStreamCreatedTimestampFieldBuilder() {
if (lastLocalStreamCreatedTimestampBuilder_ == null) {
lastLocalStreamCreatedTimestampBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getLastLocalStreamCreatedTimestamp(),
getParentForChildren(),
isClean());
lastLocalStreamCreatedTimestamp_ = null;
}
return lastLocalStreamCreatedTimestampBuilder_;
}
private com.google.protobuf.Timestamp lastRemoteStreamCreatedTimestamp_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> lastRemoteStreamCreatedTimestampBuilder_;
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
* @return Whether the lastRemoteStreamCreatedTimestamp field is set.
*/
public boolean hasLastRemoteStreamCreatedTimestamp() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
* @return The lastRemoteStreamCreatedTimestamp.
*/
public com.google.protobuf.Timestamp getLastRemoteStreamCreatedTimestamp() {
if (lastRemoteStreamCreatedTimestampBuilder_ == null) {
return lastRemoteStreamCreatedTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastRemoteStreamCreatedTimestamp_;
} else {
return lastRemoteStreamCreatedTimestampBuilder_.getMessage();
}
}
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
*/
public Builder setLastRemoteStreamCreatedTimestamp(com.google.protobuf.Timestamp value) {
if (lastRemoteStreamCreatedTimestampBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lastRemoteStreamCreatedTimestamp_ = value;
} else {
lastRemoteStreamCreatedTimestampBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
*/
public Builder setLastRemoteStreamCreatedTimestamp(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (lastRemoteStreamCreatedTimestampBuilder_ == null) {
lastRemoteStreamCreatedTimestamp_ = builderForValue.build();
} else {
lastRemoteStreamCreatedTimestampBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
*/
public Builder mergeLastRemoteStreamCreatedTimestamp(com.google.protobuf.Timestamp value) {
if (lastRemoteStreamCreatedTimestampBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0) &&
lastRemoteStreamCreatedTimestamp_ != null &&
lastRemoteStreamCreatedTimestamp_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getLastRemoteStreamCreatedTimestampBuilder().mergeFrom(value);
} else {
lastRemoteStreamCreatedTimestamp_ = value;
}
} else {
lastRemoteStreamCreatedTimestampBuilder_.mergeFrom(value);
}
if (lastRemoteStreamCreatedTimestamp_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
*/
public Builder clearLastRemoteStreamCreatedTimestamp() {
bitField0_ = (bitField0_ & ~0x00000080);
lastRemoteStreamCreatedTimestamp_ = null;
if (lastRemoteStreamCreatedTimestampBuilder_ != null) {
lastRemoteStreamCreatedTimestampBuilder_.dispose();
lastRemoteStreamCreatedTimestampBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
*/
public com.google.protobuf.Timestamp.Builder getLastRemoteStreamCreatedTimestampBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getLastRemoteStreamCreatedTimestampFieldBuilder().getBuilder();
}
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
*/
public com.google.protobuf.TimestampOrBuilder getLastRemoteStreamCreatedTimestampOrBuilder() {
if (lastRemoteStreamCreatedTimestampBuilder_ != null) {
return lastRemoteStreamCreatedTimestampBuilder_.getMessageOrBuilder();
} else {
return lastRemoteStreamCreatedTimestamp_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : lastRemoteStreamCreatedTimestamp_;
}
}
/**
*
* The last time a stream was created by the remote endpoint. Usually unset
* for clients.
*
*
* .google.protobuf.Timestamp last_remote_stream_created_timestamp = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getLastRemoteStreamCreatedTimestampFieldBuilder() {
if (lastRemoteStreamCreatedTimestampBuilder_ == null) {
lastRemoteStreamCreatedTimestampBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getLastRemoteStreamCreatedTimestamp(),
getParentForChildren(),
isClean());
lastRemoteStreamCreatedTimestamp_ = null;
}
return lastRemoteStreamCreatedTimestampBuilder_;
}
private com.google.protobuf.Timestamp lastMessageSentTimestamp_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> lastMessageSentTimestampBuilder_;
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
* @return Whether the lastMessageSentTimestamp field is set.
*/
public boolean hasLastMessageSentTimestamp() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
* @return The lastMessageSentTimestamp.
*/
public com.google.protobuf.Timestamp getLastMessageSentTimestamp() {
if (lastMessageSentTimestampBuilder_ == null) {
return lastMessageSentTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastMessageSentTimestamp_;
} else {
return lastMessageSentTimestampBuilder_.getMessage();
}
}
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
*/
public Builder setLastMessageSentTimestamp(com.google.protobuf.Timestamp value) {
if (lastMessageSentTimestampBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lastMessageSentTimestamp_ = value;
} else {
lastMessageSentTimestampBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
*/
public Builder setLastMessageSentTimestamp(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (lastMessageSentTimestampBuilder_ == null) {
lastMessageSentTimestamp_ = builderForValue.build();
} else {
lastMessageSentTimestampBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
*/
public Builder mergeLastMessageSentTimestamp(com.google.protobuf.Timestamp value) {
if (lastMessageSentTimestampBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0) &&
lastMessageSentTimestamp_ != null &&
lastMessageSentTimestamp_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getLastMessageSentTimestampBuilder().mergeFrom(value);
} else {
lastMessageSentTimestamp_ = value;
}
} else {
lastMessageSentTimestampBuilder_.mergeFrom(value);
}
if (lastMessageSentTimestamp_ != null) {
bitField0_ |= 0x00000100;
onChanged();
}
return this;
}
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
*/
public Builder clearLastMessageSentTimestamp() {
bitField0_ = (bitField0_ & ~0x00000100);
lastMessageSentTimestamp_ = null;
if (lastMessageSentTimestampBuilder_ != null) {
lastMessageSentTimestampBuilder_.dispose();
lastMessageSentTimestampBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
*/
public com.google.protobuf.Timestamp.Builder getLastMessageSentTimestampBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getLastMessageSentTimestampFieldBuilder().getBuilder();
}
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
*/
public com.google.protobuf.TimestampOrBuilder getLastMessageSentTimestampOrBuilder() {
if (lastMessageSentTimestampBuilder_ != null) {
return lastMessageSentTimestampBuilder_.getMessageOrBuilder();
} else {
return lastMessageSentTimestamp_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : lastMessageSentTimestamp_;
}
}
/**
*
* The last time a message was sent by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_sent_timestamp = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getLastMessageSentTimestampFieldBuilder() {
if (lastMessageSentTimestampBuilder_ == null) {
lastMessageSentTimestampBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getLastMessageSentTimestamp(),
getParentForChildren(),
isClean());
lastMessageSentTimestamp_ = null;
}
return lastMessageSentTimestampBuilder_;
}
private com.google.protobuf.Timestamp lastMessageReceivedTimestamp_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> lastMessageReceivedTimestampBuilder_;
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
* @return Whether the lastMessageReceivedTimestamp field is set.
*/
public boolean hasLastMessageReceivedTimestamp() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
* @return The lastMessageReceivedTimestamp.
*/
public com.google.protobuf.Timestamp getLastMessageReceivedTimestamp() {
if (lastMessageReceivedTimestampBuilder_ == null) {
return lastMessageReceivedTimestamp_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastMessageReceivedTimestamp_;
} else {
return lastMessageReceivedTimestampBuilder_.getMessage();
}
}
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
*/
public Builder setLastMessageReceivedTimestamp(com.google.protobuf.Timestamp value) {
if (lastMessageReceivedTimestampBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lastMessageReceivedTimestamp_ = value;
} else {
lastMessageReceivedTimestampBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
*/
public Builder setLastMessageReceivedTimestamp(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (lastMessageReceivedTimestampBuilder_ == null) {
lastMessageReceivedTimestamp_ = builderForValue.build();
} else {
lastMessageReceivedTimestampBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
*/
public Builder mergeLastMessageReceivedTimestamp(com.google.protobuf.Timestamp value) {
if (lastMessageReceivedTimestampBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0) &&
lastMessageReceivedTimestamp_ != null &&
lastMessageReceivedTimestamp_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getLastMessageReceivedTimestampBuilder().mergeFrom(value);
} else {
lastMessageReceivedTimestamp_ = value;
}
} else {
lastMessageReceivedTimestampBuilder_.mergeFrom(value);
}
if (lastMessageReceivedTimestamp_ != null) {
bitField0_ |= 0x00000200;
onChanged();
}
return this;
}
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
*/
public Builder clearLastMessageReceivedTimestamp() {
bitField0_ = (bitField0_ & ~0x00000200);
lastMessageReceivedTimestamp_ = null;
if (lastMessageReceivedTimestampBuilder_ != null) {
lastMessageReceivedTimestampBuilder_.dispose();
lastMessageReceivedTimestampBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
*/
public com.google.protobuf.Timestamp.Builder getLastMessageReceivedTimestampBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getLastMessageReceivedTimestampFieldBuilder().getBuilder();
}
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
*/
public com.google.protobuf.TimestampOrBuilder getLastMessageReceivedTimestampOrBuilder() {
if (lastMessageReceivedTimestampBuilder_ != null) {
return lastMessageReceivedTimestampBuilder_.getMessageOrBuilder();
} else {
return lastMessageReceivedTimestamp_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : lastMessageReceivedTimestamp_;
}
}
/**
*
* The last time a message was received by this endpoint.
*
*
* .google.protobuf.Timestamp last_message_received_timestamp = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getLastMessageReceivedTimestampFieldBuilder() {
if (lastMessageReceivedTimestampBuilder_ == null) {
lastMessageReceivedTimestampBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getLastMessageReceivedTimestamp(),
getParentForChildren(),
isClean());
lastMessageReceivedTimestamp_ = null;
}
return lastMessageReceivedTimestampBuilder_;
}
private com.google.protobuf.Int64Value localFlowControlWindow_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int64Value, com.google.protobuf.Int64Value.Builder, com.google.protobuf.Int64ValueOrBuilder> localFlowControlWindowBuilder_;
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
* @return Whether the localFlowControlWindow field is set.
*/
public boolean hasLocalFlowControlWindow() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
* @return The localFlowControlWindow.
*/
public com.google.protobuf.Int64Value getLocalFlowControlWindow() {
if (localFlowControlWindowBuilder_ == null) {
return localFlowControlWindow_ == null ? com.google.protobuf.Int64Value.getDefaultInstance() : localFlowControlWindow_;
} else {
return localFlowControlWindowBuilder_.getMessage();
}
}
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
*/
public Builder setLocalFlowControlWindow(com.google.protobuf.Int64Value value) {
if (localFlowControlWindowBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
localFlowControlWindow_ = value;
} else {
localFlowControlWindowBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
*/
public Builder setLocalFlowControlWindow(
com.google.protobuf.Int64Value.Builder builderForValue) {
if (localFlowControlWindowBuilder_ == null) {
localFlowControlWindow_ = builderForValue.build();
} else {
localFlowControlWindowBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
*/
public Builder mergeLocalFlowControlWindow(com.google.protobuf.Int64Value value) {
if (localFlowControlWindowBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0) &&
localFlowControlWindow_ != null &&
localFlowControlWindow_ != com.google.protobuf.Int64Value.getDefaultInstance()) {
getLocalFlowControlWindowBuilder().mergeFrom(value);
} else {
localFlowControlWindow_ = value;
}
} else {
localFlowControlWindowBuilder_.mergeFrom(value);
}
if (localFlowControlWindow_ != null) {
bitField0_ |= 0x00000400;
onChanged();
}
return this;
}
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
*/
public Builder clearLocalFlowControlWindow() {
bitField0_ = (bitField0_ & ~0x00000400);
localFlowControlWindow_ = null;
if (localFlowControlWindowBuilder_ != null) {
localFlowControlWindowBuilder_.dispose();
localFlowControlWindowBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
*/
public com.google.protobuf.Int64Value.Builder getLocalFlowControlWindowBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getLocalFlowControlWindowFieldBuilder().getBuilder();
}
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
*/
public com.google.protobuf.Int64ValueOrBuilder getLocalFlowControlWindowOrBuilder() {
if (localFlowControlWindowBuilder_ != null) {
return localFlowControlWindowBuilder_.getMessageOrBuilder();
} else {
return localFlowControlWindow_ == null ?
com.google.protobuf.Int64Value.getDefaultInstance() : localFlowControlWindow_;
}
}
/**
*
* The amount of window, granted to the local endpoint by the remote endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value local_flow_control_window = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int64Value, com.google.protobuf.Int64Value.Builder, com.google.protobuf.Int64ValueOrBuilder>
getLocalFlowControlWindowFieldBuilder() {
if (localFlowControlWindowBuilder_ == null) {
localFlowControlWindowBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int64Value, com.google.protobuf.Int64Value.Builder, com.google.protobuf.Int64ValueOrBuilder>(
getLocalFlowControlWindow(),
getParentForChildren(),
isClean());
localFlowControlWindow_ = null;
}
return localFlowControlWindowBuilder_;
}
private com.google.protobuf.Int64Value remoteFlowControlWindow_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int64Value, com.google.protobuf.Int64Value.Builder, com.google.protobuf.Int64ValueOrBuilder> remoteFlowControlWindowBuilder_;
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
* @return Whether the remoteFlowControlWindow field is set.
*/
public boolean hasRemoteFlowControlWindow() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
* @return The remoteFlowControlWindow.
*/
public com.google.protobuf.Int64Value getRemoteFlowControlWindow() {
if (remoteFlowControlWindowBuilder_ == null) {
return remoteFlowControlWindow_ == null ? com.google.protobuf.Int64Value.getDefaultInstance() : remoteFlowControlWindow_;
} else {
return remoteFlowControlWindowBuilder_.getMessage();
}
}
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
*/
public Builder setRemoteFlowControlWindow(com.google.protobuf.Int64Value value) {
if (remoteFlowControlWindowBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
remoteFlowControlWindow_ = value;
} else {
remoteFlowControlWindowBuilder_.setMessage(value);
}
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
*/
public Builder setRemoteFlowControlWindow(
com.google.protobuf.Int64Value.Builder builderForValue) {
if (remoteFlowControlWindowBuilder_ == null) {
remoteFlowControlWindow_ = builderForValue.build();
} else {
remoteFlowControlWindowBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
*/
public Builder mergeRemoteFlowControlWindow(com.google.protobuf.Int64Value value) {
if (remoteFlowControlWindowBuilder_ == null) {
if (((bitField0_ & 0x00000800) != 0) &&
remoteFlowControlWindow_ != null &&
remoteFlowControlWindow_ != com.google.protobuf.Int64Value.getDefaultInstance()) {
getRemoteFlowControlWindowBuilder().mergeFrom(value);
} else {
remoteFlowControlWindow_ = value;
}
} else {
remoteFlowControlWindowBuilder_.mergeFrom(value);
}
if (remoteFlowControlWindow_ != null) {
bitField0_ |= 0x00000800;
onChanged();
}
return this;
}
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
*/
public Builder clearRemoteFlowControlWindow() {
bitField0_ = (bitField0_ & ~0x00000800);
remoteFlowControlWindow_ = null;
if (remoteFlowControlWindowBuilder_ != null) {
remoteFlowControlWindowBuilder_.dispose();
remoteFlowControlWindowBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
*/
public com.google.protobuf.Int64Value.Builder getRemoteFlowControlWindowBuilder() {
bitField0_ |= 0x00000800;
onChanged();
return getRemoteFlowControlWindowFieldBuilder().getBuilder();
}
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
*/
public com.google.protobuf.Int64ValueOrBuilder getRemoteFlowControlWindowOrBuilder() {
if (remoteFlowControlWindowBuilder_ != null) {
return remoteFlowControlWindowBuilder_.getMessageOrBuilder();
} else {
return remoteFlowControlWindow_ == null ?
com.google.protobuf.Int64Value.getDefaultInstance() : remoteFlowControlWindow_;
}
}
/**
*
* The amount of window, granted to the remote endpoint by the local endpoint.
* This may be slightly out of date due to network latency. This does NOT
* include stream level or TCP level flow control info.
*
*
* .google.protobuf.Int64Value remote_flow_control_window = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int64Value, com.google.protobuf.Int64Value.Builder, com.google.protobuf.Int64ValueOrBuilder>
getRemoteFlowControlWindowFieldBuilder() {
if (remoteFlowControlWindowBuilder_ == null) {
remoteFlowControlWindowBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int64Value, com.google.protobuf.Int64Value.Builder, com.google.protobuf.Int64ValueOrBuilder>(
getRemoteFlowControlWindow(),
getParentForChildren(),
isClean());
remoteFlowControlWindow_ = null;
}
return remoteFlowControlWindowBuilder_;
}
private java.util.List option_ =
java.util.Collections.emptyList();
private void ensureOptionIsMutable() {
if (!((bitField0_ & 0x00001000) != 0)) {
option_ = new java.util.ArrayList(option_);
bitField0_ |= 0x00001000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.channelz.v1.SocketOption, io.grpc.channelz.v1.SocketOption.Builder, io.grpc.channelz.v1.SocketOptionOrBuilder> optionBuilder_;
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public java.util.List getOptionList() {
if (optionBuilder_ == null) {
return java.util.Collections.unmodifiableList(option_);
} else {
return optionBuilder_.getMessageList();
}
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public int getOptionCount() {
if (optionBuilder_ == null) {
return option_.size();
} else {
return optionBuilder_.getCount();
}
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public io.grpc.channelz.v1.SocketOption getOption(int index) {
if (optionBuilder_ == null) {
return option_.get(index);
} else {
return optionBuilder_.getMessage(index);
}
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public Builder setOption(
int index, io.grpc.channelz.v1.SocketOption value) {
if (optionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionIsMutable();
option_.set(index, value);
onChanged();
} else {
optionBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public Builder setOption(
int index, io.grpc.channelz.v1.SocketOption.Builder builderForValue) {
if (optionBuilder_ == null) {
ensureOptionIsMutable();
option_.set(index, builderForValue.build());
onChanged();
} else {
optionBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public Builder addOption(io.grpc.channelz.v1.SocketOption value) {
if (optionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionIsMutable();
option_.add(value);
onChanged();
} else {
optionBuilder_.addMessage(value);
}
return this;
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public Builder addOption(
int index, io.grpc.channelz.v1.SocketOption value) {
if (optionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionIsMutable();
option_.add(index, value);
onChanged();
} else {
optionBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public Builder addOption(
io.grpc.channelz.v1.SocketOption.Builder builderForValue) {
if (optionBuilder_ == null) {
ensureOptionIsMutable();
option_.add(builderForValue.build());
onChanged();
} else {
optionBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public Builder addOption(
int index, io.grpc.channelz.v1.SocketOption.Builder builderForValue) {
if (optionBuilder_ == null) {
ensureOptionIsMutable();
option_.add(index, builderForValue.build());
onChanged();
} else {
optionBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public Builder addAllOption(
java.lang.Iterable extends io.grpc.channelz.v1.SocketOption> values) {
if (optionBuilder_ == null) {
ensureOptionIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, option_);
onChanged();
} else {
optionBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public Builder clearOption() {
if (optionBuilder_ == null) {
option_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
} else {
optionBuilder_.clear();
}
return this;
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public Builder removeOption(int index) {
if (optionBuilder_ == null) {
ensureOptionIsMutable();
option_.remove(index);
onChanged();
} else {
optionBuilder_.remove(index);
}
return this;
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public io.grpc.channelz.v1.SocketOption.Builder getOptionBuilder(
int index) {
return getOptionFieldBuilder().getBuilder(index);
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public io.grpc.channelz.v1.SocketOptionOrBuilder getOptionOrBuilder(
int index) {
if (optionBuilder_ == null) {
return option_.get(index); } else {
return optionBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public java.util.List extends io.grpc.channelz.v1.SocketOptionOrBuilder>
getOptionOrBuilderList() {
if (optionBuilder_ != null) {
return optionBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(option_);
}
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public io.grpc.channelz.v1.SocketOption.Builder addOptionBuilder() {
return getOptionFieldBuilder().addBuilder(
io.grpc.channelz.v1.SocketOption.getDefaultInstance());
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public io.grpc.channelz.v1.SocketOption.Builder addOptionBuilder(
int index) {
return getOptionFieldBuilder().addBuilder(
index, io.grpc.channelz.v1.SocketOption.getDefaultInstance());
}
/**
*
* Socket options set on this socket. May be absent if 'summary' is set
* on GetSocketRequest.
*
*
* repeated .grpc.channelz.v1.SocketOption option = 13;
*/
public java.util.List
getOptionBuilderList() {
return getOptionFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.channelz.v1.SocketOption, io.grpc.channelz.v1.SocketOption.Builder, io.grpc.channelz.v1.SocketOptionOrBuilder>
getOptionFieldBuilder() {
if (optionBuilder_ == null) {
optionBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.channelz.v1.SocketOption, io.grpc.channelz.v1.SocketOption.Builder, io.grpc.channelz.v1.SocketOptionOrBuilder>(
option_,
((bitField0_ & 0x00001000) != 0),
getParentForChildren(),
isClean());
option_ = null;
}
return optionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:grpc.channelz.v1.SocketData)
}
// @@protoc_insertion_point(class_scope:grpc.channelz.v1.SocketData)
private static final io.grpc.channelz.v1.SocketData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.grpc.channelz.v1.SocketData();
}
public static io.grpc.channelz.v1.SocketData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SocketData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.grpc.channelz.v1.SocketData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy