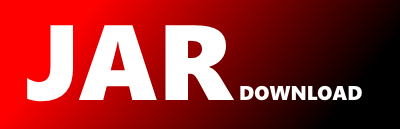
shade.polaris.io.prometheus.client.Info Maven / Gradle / Ivy
Show all versions of polaris-all Show documentation
package io.prometheus.client;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
/**
* Info metric, key-value pairs.
*
* Examples of Info include build information, version information, and potential target metadata.
* The string "_info" will be appended to the sample name.
*
*
* Example Info:
*
* {@code
* class YourClass {
* static final Info buildInfo = Info.build()
* .name("your_build").help("Build information.")
* .register();
*
* void func() {
* // Your code here.
* buildInfo.info("branch", "HEAD", "version", "1.2.3", "revision", "e0704b");
* }
* }
* }
*
*
* @since 0.10.0
*/
public class Info extends SimpleCollector implements Counter.Describable {
Info(Builder b) {
super(b);
}
public static class Builder extends SimpleCollector.Builder {
@Override
public Info create() {
if (!unit.isEmpty()) {
throw new IllegalStateException("Info metrics cannot have a unit.");
}
return new Info(this);
}
}
/**
* Return a Builder to allow configuration of a new Info. Ensures required fields are provided.
*
* @param name The name of the metric
* @param help The help string of the metric
*/
public static Builder build(String name, String help) {
return new Builder().name(name).help(help);
}
/**
* Return a Builder to allow configuration of a new Info.
*/
public static Builder build() {
return new Builder();
}
@Override
protected Child newChild() {
return new Child(labelNames);
}
/**
* The value of a single Info.
*
* Warning: References to a Child become invalid after using
* {@link SimpleCollector#remove} or {@link SimpleCollector#clear}.
*/
public static class Child {
private Map value = Collections.emptyMap();
private List labelNames;
private Child(List labelNames) {
this.labelNames = labelNames;
}
/**
* Set the info.
*/
public void info(Map v) {
for (String key : v.keySet()) {
checkMetricLabelName(key);
}
for (String label : labelNames) {
if (v.containsKey(label)) {
throw new IllegalArgumentException("Info and its value cannot have the same label name.");
}
}
this.value = v;
}
/**
* Set the info.
*
* @param v labels as pairs of key values
*/
public void info(String... v) {
if (v.length % 2 != 0) {
throw new IllegalArgumentException("An even number of arguments must be passed");
}
Map m = new TreeMap();
for (int i = 0; i < v.length; i+=2) {
m.put(v[i], v[i+1]);
}
info(m);
}
/**
* Get the info.
*/
public Map get() {
return value;
}
}
// Convenience methods.
/**
* Set the info on the info with no labels.
*/
public void info(String... v) {
noLabelsChild.info(v);
}
/**
* Set the info on the info with no labels.
*
* @param v labels as pairs of key values
*/
public void info(Map v) {
noLabelsChild.info(v);
}
/**
* Get the the info.
*/
public Map get() {
return noLabelsChild.get();
}
@Override
public List collect() {
List samples = new ArrayList();
for(Map.Entry, Child> c: children.entrySet()) {
Map v = c.getValue().get();
List names = new ArrayList(labelNames);
List values = new ArrayList(c.getKey());
for(Map.Entry l: v.entrySet()) {
names.add(l.getKey());
values.add(l.getValue());
}
samples.add(new MetricFamilySamples.Sample(fullname + "_info", names, values, 1.0));
}
return familySamplesList(Type.INFO, samples);
}
@Override
public List describe() {
return Collections.singletonList(
new MetricFamilySamples(fullname, Type.INFO, help, Collections.emptyList()));
}
}