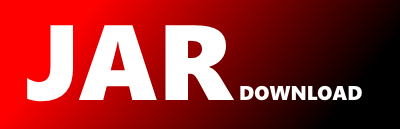
shade.polaris.io.grpc.lookup.v1.HttpKeyBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of polaris-all Show documentation
Show all versions of polaris-all Show documentation
All in one project for polaris-java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: grpc/lookup/v1/rls_config.proto
// Protobuf Java Version: 3.25.1
package io.grpc.lookup.v1;
/**
*
* An HttpKeyBuilder applies to a given HTTP URL and headers.
*
* Path and host patterns use the matching syntax from gRPC transcoding to
* extract named key/value pairs from the path and host components of the URL:
* https://github.com/googleapis/googleapis/blob/master/google/api/http.proto
*
* It is invalid to specify the same key name in multiple places in a pattern.
*
* For a service where the project id can be expressed either as a subdomain or
* in the path, separate HttpKeyBuilders must be used:
* host_pattern: 'example.com' path_pattern: '/{id}/{object}/**'
* host_pattern: '{id}.example.com' path_pattern: '/{object}/**'
* If the host is exactly 'example.com', the first path segment will be used as
* the id and the second segment as the object. If the host has a subdomain, the
* subdomain will be used as the id and the first segment as the object. If
* neither pattern matches, no keys will be extracted.
*
*
* Protobuf type {@code grpc.lookup.v1.HttpKeyBuilder}
*/
public final class HttpKeyBuilder extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:grpc.lookup.v1.HttpKeyBuilder)
HttpKeyBuilderOrBuilder {
private static final long serialVersionUID = 0L;
// Use HttpKeyBuilder.newBuilder() to construct.
private HttpKeyBuilder(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HttpKeyBuilder() {
hostPatterns_ =
com.google.protobuf.LazyStringArrayList.emptyList();
pathPatterns_ =
com.google.protobuf.LazyStringArrayList.emptyList();
queryParameters_ = java.util.Collections.emptyList();
headers_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HttpKeyBuilder();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.lookup.v1.RlsConfigProto.internal_static_grpc_lookup_v1_HttpKeyBuilder_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 5:
return internalGetConstantKeys();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.lookup.v1.RlsConfigProto.internal_static_grpc_lookup_v1_HttpKeyBuilder_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.lookup.v1.HttpKeyBuilder.class, io.grpc.lookup.v1.HttpKeyBuilder.Builder.class);
}
public static final int HOST_PATTERNS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList hostPatterns_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @return A list containing the hostPatterns.
*/
public com.google.protobuf.ProtocolStringList
getHostPatternsList() {
return hostPatterns_;
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @return The count of hostPatterns.
*/
public int getHostPatternsCount() {
return hostPatterns_.size();
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @param index The index of the element to return.
* @return The hostPatterns at the given index.
*/
public java.lang.String getHostPatterns(int index) {
return hostPatterns_.get(index);
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @param index The index of the value to return.
* @return The bytes of the hostPatterns at the given index.
*/
public com.google.protobuf.ByteString
getHostPatternsBytes(int index) {
return hostPatterns_.getByteString(index);
}
public static final int PATH_PATTERNS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList pathPatterns_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @return A list containing the pathPatterns.
*/
public com.google.protobuf.ProtocolStringList
getPathPatternsList() {
return pathPatterns_;
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @return The count of pathPatterns.
*/
public int getPathPatternsCount() {
return pathPatterns_.size();
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @param index The index of the element to return.
* @return The pathPatterns at the given index.
*/
public java.lang.String getPathPatterns(int index) {
return pathPatterns_.get(index);
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @param index The index of the value to return.
* @return The bytes of the pathPatterns at the given index.
*/
public com.google.protobuf.ByteString
getPathPatternsBytes(int index) {
return pathPatterns_.getByteString(index);
}
public static final int QUERY_PARAMETERS_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List queryParameters_;
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
@java.lang.Override
public java.util.List getQueryParametersList() {
return queryParameters_;
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
@java.lang.Override
public java.util.List extends io.grpc.lookup.v1.NameMatcherOrBuilder>
getQueryParametersOrBuilderList() {
return queryParameters_;
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
@java.lang.Override
public int getQueryParametersCount() {
return queryParameters_.size();
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
@java.lang.Override
public io.grpc.lookup.v1.NameMatcher getQueryParameters(int index) {
return queryParameters_.get(index);
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
@java.lang.Override
public io.grpc.lookup.v1.NameMatcherOrBuilder getQueryParametersOrBuilder(
int index) {
return queryParameters_.get(index);
}
public static final int HEADERS_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private java.util.List headers_;
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
@java.lang.Override
public java.util.List getHeadersList() {
return headers_;
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
@java.lang.Override
public java.util.List extends io.grpc.lookup.v1.NameMatcherOrBuilder>
getHeadersOrBuilderList() {
return headers_;
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
@java.lang.Override
public int getHeadersCount() {
return headers_.size();
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
@java.lang.Override
public io.grpc.lookup.v1.NameMatcher getHeaders(int index) {
return headers_.get(index);
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
@java.lang.Override
public io.grpc.lookup.v1.NameMatcherOrBuilder getHeadersOrBuilder(
int index) {
return headers_.get(index);
}
public static final int CONSTANT_KEYS_FIELD_NUMBER = 5;
private static final class ConstantKeysDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
io.grpc.lookup.v1.RlsConfigProto.internal_static_grpc_lookup_v1_HttpKeyBuilder_ConstantKeysEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> constantKeys_;
private com.google.protobuf.MapField
internalGetConstantKeys() {
if (constantKeys_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ConstantKeysDefaultEntryHolder.defaultEntry);
}
return constantKeys_;
}
public int getConstantKeysCount() {
return internalGetConstantKeys().getMap().size();
}
/**
*
* You can optionally set one or more specific key/value pairs to be added to
* the key_map. This can be useful to identify which builder built the key,
* for example if you are suppressing a lot of information from the URL, but
* need to separately cache and request URLs with that content.
*
*
* map<string, string> constant_keys = 5;
*/
@java.lang.Override
public boolean containsConstantKeys(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetConstantKeys().getMap().containsKey(key);
}
/**
* Use {@link #getConstantKeysMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getConstantKeys() {
return getConstantKeysMap();
}
/**
*
* You can optionally set one or more specific key/value pairs to be added to
* the key_map. This can be useful to identify which builder built the key,
* for example if you are suppressing a lot of information from the URL, but
* need to separately cache and request URLs with that content.
*
*
* map<string, string> constant_keys = 5;
*/
@java.lang.Override
public java.util.Map getConstantKeysMap() {
return internalGetConstantKeys().getMap();
}
/**
*
* You can optionally set one or more specific key/value pairs to be added to
* the key_map. This can be useful to identify which builder built the key,
* for example if you are suppressing a lot of information from the URL, but
* need to separately cache and request URLs with that content.
*
*
* map<string, string> constant_keys = 5;
*/
@java.lang.Override
public /* nullable */
java.lang.String getConstantKeysOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetConstantKeys().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* You can optionally set one or more specific key/value pairs to be added to
* the key_map. This can be useful to identify which builder built the key,
* for example if you are suppressing a lot of information from the URL, but
* need to separately cache and request URLs with that content.
*
*
* map<string, string> constant_keys = 5;
*/
@java.lang.Override
public java.lang.String getConstantKeysOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetConstantKeys().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < hostPatterns_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, hostPatterns_.getRaw(i));
}
for (int i = 0; i < pathPatterns_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, pathPatterns_.getRaw(i));
}
for (int i = 0; i < queryParameters_.size(); i++) {
output.writeMessage(3, queryParameters_.get(i));
}
for (int i = 0; i < headers_.size(); i++) {
output.writeMessage(4, headers_.get(i));
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetConstantKeys(),
ConstantKeysDefaultEntryHolder.defaultEntry,
5);
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < hostPatterns_.size(); i++) {
dataSize += computeStringSizeNoTag(hostPatterns_.getRaw(i));
}
size += dataSize;
size += 1 * getHostPatternsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < pathPatterns_.size(); i++) {
dataSize += computeStringSizeNoTag(pathPatterns_.getRaw(i));
}
size += dataSize;
size += 1 * getPathPatternsList().size();
}
for (int i = 0; i < queryParameters_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, queryParameters_.get(i));
}
for (int i = 0; i < headers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, headers_.get(i));
}
for (java.util.Map.Entry entry
: internalGetConstantKeys().getMap().entrySet()) {
com.google.protobuf.MapEntry
constantKeys__ = ConstantKeysDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, constantKeys__);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.grpc.lookup.v1.HttpKeyBuilder)) {
return super.equals(obj);
}
io.grpc.lookup.v1.HttpKeyBuilder other = (io.grpc.lookup.v1.HttpKeyBuilder) obj;
if (!getHostPatternsList()
.equals(other.getHostPatternsList())) return false;
if (!getPathPatternsList()
.equals(other.getPathPatternsList())) return false;
if (!getQueryParametersList()
.equals(other.getQueryParametersList())) return false;
if (!getHeadersList()
.equals(other.getHeadersList())) return false;
if (!internalGetConstantKeys().equals(
other.internalGetConstantKeys())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getHostPatternsCount() > 0) {
hash = (37 * hash) + HOST_PATTERNS_FIELD_NUMBER;
hash = (53 * hash) + getHostPatternsList().hashCode();
}
if (getPathPatternsCount() > 0) {
hash = (37 * hash) + PATH_PATTERNS_FIELD_NUMBER;
hash = (53 * hash) + getPathPatternsList().hashCode();
}
if (getQueryParametersCount() > 0) {
hash = (37 * hash) + QUERY_PARAMETERS_FIELD_NUMBER;
hash = (53 * hash) + getQueryParametersList().hashCode();
}
if (getHeadersCount() > 0) {
hash = (37 * hash) + HEADERS_FIELD_NUMBER;
hash = (53 * hash) + getHeadersList().hashCode();
}
if (!internalGetConstantKeys().getMap().isEmpty()) {
hash = (37 * hash) + CONSTANT_KEYS_FIELD_NUMBER;
hash = (53 * hash) + internalGetConstantKeys().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.lookup.v1.HttpKeyBuilder parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.grpc.lookup.v1.HttpKeyBuilder prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An HttpKeyBuilder applies to a given HTTP URL and headers.
*
* Path and host patterns use the matching syntax from gRPC transcoding to
* extract named key/value pairs from the path and host components of the URL:
* https://github.com/googleapis/googleapis/blob/master/google/api/http.proto
*
* It is invalid to specify the same key name in multiple places in a pattern.
*
* For a service where the project id can be expressed either as a subdomain or
* in the path, separate HttpKeyBuilders must be used:
* host_pattern: 'example.com' path_pattern: '/{id}/{object}/**'
* host_pattern: '{id}.example.com' path_pattern: '/{object}/**'
* If the host is exactly 'example.com', the first path segment will be used as
* the id and the second segment as the object. If the host has a subdomain, the
* subdomain will be used as the id and the first segment as the object. If
* neither pattern matches, no keys will be extracted.
*
*
* Protobuf type {@code grpc.lookup.v1.HttpKeyBuilder}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:grpc.lookup.v1.HttpKeyBuilder)
io.grpc.lookup.v1.HttpKeyBuilderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.lookup.v1.RlsConfigProto.internal_static_grpc_lookup_v1_HttpKeyBuilder_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 5:
return internalGetConstantKeys();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 5:
return internalGetMutableConstantKeys();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.lookup.v1.RlsConfigProto.internal_static_grpc_lookup_v1_HttpKeyBuilder_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.lookup.v1.HttpKeyBuilder.class, io.grpc.lookup.v1.HttpKeyBuilder.Builder.class);
}
// Construct using io.grpc.lookup.v1.HttpKeyBuilder.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
hostPatterns_ =
com.google.protobuf.LazyStringArrayList.emptyList();
pathPatterns_ =
com.google.protobuf.LazyStringArrayList.emptyList();
if (queryParametersBuilder_ == null) {
queryParameters_ = java.util.Collections.emptyList();
} else {
queryParameters_ = null;
queryParametersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (headersBuilder_ == null) {
headers_ = java.util.Collections.emptyList();
} else {
headers_ = null;
headersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
internalGetMutableConstantKeys().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.grpc.lookup.v1.RlsConfigProto.internal_static_grpc_lookup_v1_HttpKeyBuilder_descriptor;
}
@java.lang.Override
public io.grpc.lookup.v1.HttpKeyBuilder getDefaultInstanceForType() {
return io.grpc.lookup.v1.HttpKeyBuilder.getDefaultInstance();
}
@java.lang.Override
public io.grpc.lookup.v1.HttpKeyBuilder build() {
io.grpc.lookup.v1.HttpKeyBuilder result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.grpc.lookup.v1.HttpKeyBuilder buildPartial() {
io.grpc.lookup.v1.HttpKeyBuilder result = new io.grpc.lookup.v1.HttpKeyBuilder(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(io.grpc.lookup.v1.HttpKeyBuilder result) {
if (queryParametersBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
queryParameters_ = java.util.Collections.unmodifiableList(queryParameters_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.queryParameters_ = queryParameters_;
} else {
result.queryParameters_ = queryParametersBuilder_.build();
}
if (headersBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
headers_ = java.util.Collections.unmodifiableList(headers_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.headers_ = headers_;
} else {
result.headers_ = headersBuilder_.build();
}
}
private void buildPartial0(io.grpc.lookup.v1.HttpKeyBuilder result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
hostPatterns_.makeImmutable();
result.hostPatterns_ = hostPatterns_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
pathPatterns_.makeImmutable();
result.pathPatterns_ = pathPatterns_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.constantKeys_ = internalGetConstantKeys();
result.constantKeys_.makeImmutable();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.grpc.lookup.v1.HttpKeyBuilder) {
return mergeFrom((io.grpc.lookup.v1.HttpKeyBuilder)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.grpc.lookup.v1.HttpKeyBuilder other) {
if (other == io.grpc.lookup.v1.HttpKeyBuilder.getDefaultInstance()) return this;
if (!other.hostPatterns_.isEmpty()) {
if (hostPatterns_.isEmpty()) {
hostPatterns_ = other.hostPatterns_;
bitField0_ |= 0x00000001;
} else {
ensureHostPatternsIsMutable();
hostPatterns_.addAll(other.hostPatterns_);
}
onChanged();
}
if (!other.pathPatterns_.isEmpty()) {
if (pathPatterns_.isEmpty()) {
pathPatterns_ = other.pathPatterns_;
bitField0_ |= 0x00000002;
} else {
ensurePathPatternsIsMutable();
pathPatterns_.addAll(other.pathPatterns_);
}
onChanged();
}
if (queryParametersBuilder_ == null) {
if (!other.queryParameters_.isEmpty()) {
if (queryParameters_.isEmpty()) {
queryParameters_ = other.queryParameters_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureQueryParametersIsMutable();
queryParameters_.addAll(other.queryParameters_);
}
onChanged();
}
} else {
if (!other.queryParameters_.isEmpty()) {
if (queryParametersBuilder_.isEmpty()) {
queryParametersBuilder_.dispose();
queryParametersBuilder_ = null;
queryParameters_ = other.queryParameters_;
bitField0_ = (bitField0_ & ~0x00000004);
queryParametersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getQueryParametersFieldBuilder() : null;
} else {
queryParametersBuilder_.addAllMessages(other.queryParameters_);
}
}
}
if (headersBuilder_ == null) {
if (!other.headers_.isEmpty()) {
if (headers_.isEmpty()) {
headers_ = other.headers_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureHeadersIsMutable();
headers_.addAll(other.headers_);
}
onChanged();
}
} else {
if (!other.headers_.isEmpty()) {
if (headersBuilder_.isEmpty()) {
headersBuilder_.dispose();
headersBuilder_ = null;
headers_ = other.headers_;
bitField0_ = (bitField0_ & ~0x00000008);
headersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getHeadersFieldBuilder() : null;
} else {
headersBuilder_.addAllMessages(other.headers_);
}
}
}
internalGetMutableConstantKeys().mergeFrom(
other.internalGetConstantKeys());
bitField0_ |= 0x00000010;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
ensureHostPatternsIsMutable();
hostPatterns_.add(s);
break;
} // case 10
case 18: {
java.lang.String s = input.readStringRequireUtf8();
ensurePathPatternsIsMutable();
pathPatterns_.add(s);
break;
} // case 18
case 26: {
io.grpc.lookup.v1.NameMatcher m =
input.readMessage(
io.grpc.lookup.v1.NameMatcher.parser(),
extensionRegistry);
if (queryParametersBuilder_ == null) {
ensureQueryParametersIsMutable();
queryParameters_.add(m);
} else {
queryParametersBuilder_.addMessage(m);
}
break;
} // case 26
case 34: {
io.grpc.lookup.v1.NameMatcher m =
input.readMessage(
io.grpc.lookup.v1.NameMatcher.parser(),
extensionRegistry);
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
headers_.add(m);
} else {
headersBuilder_.addMessage(m);
}
break;
} // case 34
case 42: {
com.google.protobuf.MapEntry
constantKeys__ = input.readMessage(
ConstantKeysDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableConstantKeys().getMutableMap().put(
constantKeys__.getKey(), constantKeys__.getValue());
bitField0_ |= 0x00000010;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList hostPatterns_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureHostPatternsIsMutable() {
if (!hostPatterns_.isModifiable()) {
hostPatterns_ = new com.google.protobuf.LazyStringArrayList(hostPatterns_);
}
bitField0_ |= 0x00000001;
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @return A list containing the hostPatterns.
*/
public com.google.protobuf.ProtocolStringList
getHostPatternsList() {
hostPatterns_.makeImmutable();
return hostPatterns_;
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @return The count of hostPatterns.
*/
public int getHostPatternsCount() {
return hostPatterns_.size();
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @param index The index of the element to return.
* @return The hostPatterns at the given index.
*/
public java.lang.String getHostPatterns(int index) {
return hostPatterns_.get(index);
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @param index The index of the value to return.
* @return The bytes of the hostPatterns at the given index.
*/
public com.google.protobuf.ByteString
getHostPatternsBytes(int index) {
return hostPatterns_.getByteString(index);
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @param index The index to set the value at.
* @param value The hostPatterns to set.
* @return This builder for chaining.
*/
public Builder setHostPatterns(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureHostPatternsIsMutable();
hostPatterns_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @param value The hostPatterns to add.
* @return This builder for chaining.
*/
public Builder addHostPatterns(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureHostPatternsIsMutable();
hostPatterns_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @param values The hostPatterns to add.
* @return This builder for chaining.
*/
public Builder addAllHostPatterns(
java.lang.Iterable values) {
ensureHostPatternsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, hostPatterns_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @return This builder for chaining.
*/
public Builder clearHostPatterns() {
hostPatterns_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);;
onChanged();
return this;
}
/**
*
* host_pattern is an ordered list of host template patterns for the desired
* value. If any host_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A host
* consists of labels separated by dots. Each label is matched against the
* label in the pattern as follows:
* - "*": Matches any single label.
* - "**": Matches zero or more labels (first or last part of host only).
* - "{<name>=...}": One or more label capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single label capture. Identical to {<name>=*}.
*
* Examples:
* - "example.com": Only applies to the exact host example.com.
* - "*.example.com": Matches subdomains of example.com.
* - "**.example.com": matches example.com, and all levels of subdomains.
* - "{project}.example.com": Extracts the third level subdomain.
* - "{project=**}.example.com": Extracts the third level+ subdomains.
* - "{project=**}": Extracts the entire host.
*
*
* repeated string host_patterns = 1;
* @param value The bytes of the hostPatterns to add.
* @return This builder for chaining.
*/
public Builder addHostPatternsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureHostPatternsIsMutable();
hostPatterns_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList pathPatterns_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensurePathPatternsIsMutable() {
if (!pathPatterns_.isModifiable()) {
pathPatterns_ = new com.google.protobuf.LazyStringArrayList(pathPatterns_);
}
bitField0_ |= 0x00000002;
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @return A list containing the pathPatterns.
*/
public com.google.protobuf.ProtocolStringList
getPathPatternsList() {
pathPatterns_.makeImmutable();
return pathPatterns_;
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @return The count of pathPatterns.
*/
public int getPathPatternsCount() {
return pathPatterns_.size();
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @param index The index of the element to return.
* @return The pathPatterns at the given index.
*/
public java.lang.String getPathPatterns(int index) {
return pathPatterns_.get(index);
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @param index The index of the value to return.
* @return The bytes of the pathPatterns at the given index.
*/
public com.google.protobuf.ByteString
getPathPatternsBytes(int index) {
return pathPatterns_.getByteString(index);
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @param index The index to set the value at.
* @param value The pathPatterns to set.
* @return This builder for chaining.
*/
public Builder setPathPatterns(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensurePathPatternsIsMutable();
pathPatterns_.set(index, value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @param value The pathPatterns to add.
* @return This builder for chaining.
*/
public Builder addPathPatterns(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensurePathPatternsIsMutable();
pathPatterns_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @param values The pathPatterns to add.
* @return This builder for chaining.
*/
public Builder addAllPathPatterns(
java.lang.Iterable values) {
ensurePathPatternsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, pathPatterns_);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @return This builder for chaining.
*/
public Builder clearPathPatterns() {
pathPatterns_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);;
onChanged();
return this;
}
/**
*
* path_pattern is an ordered list of path template patterns for the desired
* value. If any path_pattern values are specified, then at least one must
* match, and the last one wins and sets any specified variables. A path
* consists of segments separated by slashes. Each segment is matched against
* the segment in the pattern as follows:
* - "*": Matches any single segment.
* - "**": Matches zero or more segments (first or last part of path only).
* - "{<name>=...}": One or more segment capture, where "..." can be any
* template that does not include a capture.
* - "{<name>}": A single segment capture. Identical to {<name>=*}.
* A custom method may also be specified by appending ":" and the custom
* method name or "*" to indicate any custom method (including no custom
* method). For example, "/*/projects/{project_id}/**:*" extracts
* `{project_id}` for any version, resource and custom method that includes
* it. By default, any custom method will be matched.
*
* Examples:
* - "/v1/{name=messages/*}": extracts a name like "messages/12345".
* - "/v1/messages/{message_id}": extracts a message_id like "12345".
* - "/v1/users/{user_id}/messages/{message_id}": extracts two key values.
*
*
* repeated string path_patterns = 2;
* @param value The bytes of the pathPatterns to add.
* @return This builder for chaining.
*/
public Builder addPathPatternsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensurePathPatternsIsMutable();
pathPatterns_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.util.List queryParameters_ =
java.util.Collections.emptyList();
private void ensureQueryParametersIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
queryParameters_ = new java.util.ArrayList(queryParameters_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.lookup.v1.NameMatcher, io.grpc.lookup.v1.NameMatcher.Builder, io.grpc.lookup.v1.NameMatcherOrBuilder> queryParametersBuilder_;
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public java.util.List getQueryParametersList() {
if (queryParametersBuilder_ == null) {
return java.util.Collections.unmodifiableList(queryParameters_);
} else {
return queryParametersBuilder_.getMessageList();
}
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public int getQueryParametersCount() {
if (queryParametersBuilder_ == null) {
return queryParameters_.size();
} else {
return queryParametersBuilder_.getCount();
}
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public io.grpc.lookup.v1.NameMatcher getQueryParameters(int index) {
if (queryParametersBuilder_ == null) {
return queryParameters_.get(index);
} else {
return queryParametersBuilder_.getMessage(index);
}
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public Builder setQueryParameters(
int index, io.grpc.lookup.v1.NameMatcher value) {
if (queryParametersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryParametersIsMutable();
queryParameters_.set(index, value);
onChanged();
} else {
queryParametersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public Builder setQueryParameters(
int index, io.grpc.lookup.v1.NameMatcher.Builder builderForValue) {
if (queryParametersBuilder_ == null) {
ensureQueryParametersIsMutable();
queryParameters_.set(index, builderForValue.build());
onChanged();
} else {
queryParametersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public Builder addQueryParameters(io.grpc.lookup.v1.NameMatcher value) {
if (queryParametersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryParametersIsMutable();
queryParameters_.add(value);
onChanged();
} else {
queryParametersBuilder_.addMessage(value);
}
return this;
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public Builder addQueryParameters(
int index, io.grpc.lookup.v1.NameMatcher value) {
if (queryParametersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryParametersIsMutable();
queryParameters_.add(index, value);
onChanged();
} else {
queryParametersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public Builder addQueryParameters(
io.grpc.lookup.v1.NameMatcher.Builder builderForValue) {
if (queryParametersBuilder_ == null) {
ensureQueryParametersIsMutable();
queryParameters_.add(builderForValue.build());
onChanged();
} else {
queryParametersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public Builder addQueryParameters(
int index, io.grpc.lookup.v1.NameMatcher.Builder builderForValue) {
if (queryParametersBuilder_ == null) {
ensureQueryParametersIsMutable();
queryParameters_.add(index, builderForValue.build());
onChanged();
} else {
queryParametersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public Builder addAllQueryParameters(
java.lang.Iterable extends io.grpc.lookup.v1.NameMatcher> values) {
if (queryParametersBuilder_ == null) {
ensureQueryParametersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, queryParameters_);
onChanged();
} else {
queryParametersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public Builder clearQueryParameters() {
if (queryParametersBuilder_ == null) {
queryParameters_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
queryParametersBuilder_.clear();
}
return this;
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public Builder removeQueryParameters(int index) {
if (queryParametersBuilder_ == null) {
ensureQueryParametersIsMutable();
queryParameters_.remove(index);
onChanged();
} else {
queryParametersBuilder_.remove(index);
}
return this;
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public io.grpc.lookup.v1.NameMatcher.Builder getQueryParametersBuilder(
int index) {
return getQueryParametersFieldBuilder().getBuilder(index);
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public io.grpc.lookup.v1.NameMatcherOrBuilder getQueryParametersOrBuilder(
int index) {
if (queryParametersBuilder_ == null) {
return queryParameters_.get(index); } else {
return queryParametersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public java.util.List extends io.grpc.lookup.v1.NameMatcherOrBuilder>
getQueryParametersOrBuilderList() {
if (queryParametersBuilder_ != null) {
return queryParametersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(queryParameters_);
}
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public io.grpc.lookup.v1.NameMatcher.Builder addQueryParametersBuilder() {
return getQueryParametersFieldBuilder().addBuilder(
io.grpc.lookup.v1.NameMatcher.getDefaultInstance());
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public io.grpc.lookup.v1.NameMatcher.Builder addQueryParametersBuilder(
int index) {
return getQueryParametersFieldBuilder().addBuilder(
index, io.grpc.lookup.v1.NameMatcher.getDefaultInstance());
}
/**
*
* List of query parameter names to try to match.
* For example: ["parent", "name", "resource.name"]
* We extract all the specified query_parameters (case-sensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given parameter appears multiple times (?foo=a&foo=b) we
* will report it as a comma-separated string (foo=a,b).
*
*
* repeated .grpc.lookup.v1.NameMatcher query_parameters = 3;
*/
public java.util.List
getQueryParametersBuilderList() {
return getQueryParametersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.lookup.v1.NameMatcher, io.grpc.lookup.v1.NameMatcher.Builder, io.grpc.lookup.v1.NameMatcherOrBuilder>
getQueryParametersFieldBuilder() {
if (queryParametersBuilder_ == null) {
queryParametersBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.lookup.v1.NameMatcher, io.grpc.lookup.v1.NameMatcher.Builder, io.grpc.lookup.v1.NameMatcherOrBuilder>(
queryParameters_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
queryParameters_ = null;
}
return queryParametersBuilder_;
}
private java.util.List headers_ =
java.util.Collections.emptyList();
private void ensureHeadersIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
headers_ = new java.util.ArrayList(headers_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.lookup.v1.NameMatcher, io.grpc.lookup.v1.NameMatcher.Builder, io.grpc.lookup.v1.NameMatcherOrBuilder> headersBuilder_;
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public java.util.List getHeadersList() {
if (headersBuilder_ == null) {
return java.util.Collections.unmodifiableList(headers_);
} else {
return headersBuilder_.getMessageList();
}
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public int getHeadersCount() {
if (headersBuilder_ == null) {
return headers_.size();
} else {
return headersBuilder_.getCount();
}
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public io.grpc.lookup.v1.NameMatcher getHeaders(int index) {
if (headersBuilder_ == null) {
return headers_.get(index);
} else {
return headersBuilder_.getMessage(index);
}
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public Builder setHeaders(
int index, io.grpc.lookup.v1.NameMatcher value) {
if (headersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeadersIsMutable();
headers_.set(index, value);
onChanged();
} else {
headersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public Builder setHeaders(
int index, io.grpc.lookup.v1.NameMatcher.Builder builderForValue) {
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
headers_.set(index, builderForValue.build());
onChanged();
} else {
headersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public Builder addHeaders(io.grpc.lookup.v1.NameMatcher value) {
if (headersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeadersIsMutable();
headers_.add(value);
onChanged();
} else {
headersBuilder_.addMessage(value);
}
return this;
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public Builder addHeaders(
int index, io.grpc.lookup.v1.NameMatcher value) {
if (headersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeadersIsMutable();
headers_.add(index, value);
onChanged();
} else {
headersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public Builder addHeaders(
io.grpc.lookup.v1.NameMatcher.Builder builderForValue) {
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
headers_.add(builderForValue.build());
onChanged();
} else {
headersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public Builder addHeaders(
int index, io.grpc.lookup.v1.NameMatcher.Builder builderForValue) {
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
headers_.add(index, builderForValue.build());
onChanged();
} else {
headersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public Builder addAllHeaders(
java.lang.Iterable extends io.grpc.lookup.v1.NameMatcher> values) {
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, headers_);
onChanged();
} else {
headersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public Builder clearHeaders() {
if (headersBuilder_ == null) {
headers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
headersBuilder_.clear();
}
return this;
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public Builder removeHeaders(int index) {
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
headers_.remove(index);
onChanged();
} else {
headersBuilder_.remove(index);
}
return this;
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public io.grpc.lookup.v1.NameMatcher.Builder getHeadersBuilder(
int index) {
return getHeadersFieldBuilder().getBuilder(index);
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public io.grpc.lookup.v1.NameMatcherOrBuilder getHeadersOrBuilder(
int index) {
if (headersBuilder_ == null) {
return headers_.get(index); } else {
return headersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public java.util.List extends io.grpc.lookup.v1.NameMatcherOrBuilder>
getHeadersOrBuilderList() {
if (headersBuilder_ != null) {
return headersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(headers_);
}
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public io.grpc.lookup.v1.NameMatcher.Builder addHeadersBuilder() {
return getHeadersFieldBuilder().addBuilder(
io.grpc.lookup.v1.NameMatcher.getDefaultInstance());
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public io.grpc.lookup.v1.NameMatcher.Builder addHeadersBuilder(
int index) {
return getHeadersFieldBuilder().addBuilder(
index, io.grpc.lookup.v1.NameMatcher.getDefaultInstance());
}
/**
*
* List of headers to try to match.
* We extract all the specified header values (case-insensitively). If any
* are marked as "required_match" and are not present, this keybuilder fails
* to match. If a given header appears multiple times in the request we will
* report it as a comma-separated string, in standard HTTP fashion.
*
*
* repeated .grpc.lookup.v1.NameMatcher headers = 4;
*/
public java.util.List
getHeadersBuilderList() {
return getHeadersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.lookup.v1.NameMatcher, io.grpc.lookup.v1.NameMatcher.Builder, io.grpc.lookup.v1.NameMatcherOrBuilder>
getHeadersFieldBuilder() {
if (headersBuilder_ == null) {
headersBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.lookup.v1.NameMatcher, io.grpc.lookup.v1.NameMatcher.Builder, io.grpc.lookup.v1.NameMatcherOrBuilder>(
headers_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
headers_ = null;
}
return headersBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> constantKeys_;
private com.google.protobuf.MapField
internalGetConstantKeys() {
if (constantKeys_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ConstantKeysDefaultEntryHolder.defaultEntry);
}
return constantKeys_;
}
private com.google.protobuf.MapField
internalGetMutableConstantKeys() {
if (constantKeys_ == null) {
constantKeys_ = com.google.protobuf.MapField.newMapField(
ConstantKeysDefaultEntryHolder.defaultEntry);
}
if (!constantKeys_.isMutable()) {
constantKeys_ = constantKeys_.copy();
}
bitField0_ |= 0x00000010;
onChanged();
return constantKeys_;
}
public int getConstantKeysCount() {
return internalGetConstantKeys().getMap().size();
}
/**
*
* You can optionally set one or more specific key/value pairs to be added to
* the key_map. This can be useful to identify which builder built the key,
* for example if you are suppressing a lot of information from the URL, but
* need to separately cache and request URLs with that content.
*
*
* map<string, string> constant_keys = 5;
*/
@java.lang.Override
public boolean containsConstantKeys(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetConstantKeys().getMap().containsKey(key);
}
/**
* Use {@link #getConstantKeysMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getConstantKeys() {
return getConstantKeysMap();
}
/**
*
* You can optionally set one or more specific key/value pairs to be added to
* the key_map. This can be useful to identify which builder built the key,
* for example if you are suppressing a lot of information from the URL, but
* need to separately cache and request URLs with that content.
*
*
* map<string, string> constant_keys = 5;
*/
@java.lang.Override
public java.util.Map getConstantKeysMap() {
return internalGetConstantKeys().getMap();
}
/**
*
* You can optionally set one or more specific key/value pairs to be added to
* the key_map. This can be useful to identify which builder built the key,
* for example if you are suppressing a lot of information from the URL, but
* need to separately cache and request URLs with that content.
*
*
* map<string, string> constant_keys = 5;
*/
@java.lang.Override
public /* nullable */
java.lang.String getConstantKeysOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetConstantKeys().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* You can optionally set one or more specific key/value pairs to be added to
* the key_map. This can be useful to identify which builder built the key,
* for example if you are suppressing a lot of information from the URL, but
* need to separately cache and request URLs with that content.
*
*
* map<string, string> constant_keys = 5;
*/
@java.lang.Override
public java.lang.String getConstantKeysOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetConstantKeys().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearConstantKeys() {
bitField0_ = (bitField0_ & ~0x00000010);
internalGetMutableConstantKeys().getMutableMap()
.clear();
return this;
}
/**
*
* You can optionally set one or more specific key/value pairs to be added to
* the key_map. This can be useful to identify which builder built the key,
* for example if you are suppressing a lot of information from the URL, but
* need to separately cache and request URLs with that content.
*
*
* map<string, string> constant_keys = 5;
*/
public Builder removeConstantKeys(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableConstantKeys().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableConstantKeys() {
bitField0_ |= 0x00000010;
return internalGetMutableConstantKeys().getMutableMap();
}
/**
*
* You can optionally set one or more specific key/value pairs to be added to
* the key_map. This can be useful to identify which builder built the key,
* for example if you are suppressing a lot of information from the URL, but
* need to separately cache and request URLs with that content.
*
*
* map<string, string> constant_keys = 5;
*/
public Builder putConstantKeys(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableConstantKeys().getMutableMap()
.put(key, value);
bitField0_ |= 0x00000010;
return this;
}
/**
*
* You can optionally set one or more specific key/value pairs to be added to
* the key_map. This can be useful to identify which builder built the key,
* for example if you are suppressing a lot of information from the URL, but
* need to separately cache and request URLs with that content.
*
*
* map<string, string> constant_keys = 5;
*/
public Builder putAllConstantKeys(
java.util.Map values) {
internalGetMutableConstantKeys().getMutableMap()
.putAll(values);
bitField0_ |= 0x00000010;
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:grpc.lookup.v1.HttpKeyBuilder)
}
// @@protoc_insertion_point(class_scope:grpc.lookup.v1.HttpKeyBuilder)
private static final io.grpc.lookup.v1.HttpKeyBuilder DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.grpc.lookup.v1.HttpKeyBuilder();
}
public static io.grpc.lookup.v1.HttpKeyBuilder getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HttpKeyBuilder parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.grpc.lookup.v1.HttpKeyBuilder getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy