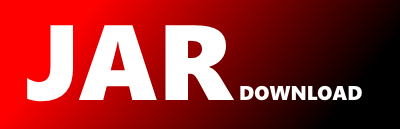
com.tencent.trpc.codegen.protoc.ProtocInstruction Maven / Gradle / Ivy
The newest version!
/*
* Tencent is pleased to support the open source community by making tRPC available.
*
* Copyright (C) 2023 THL A29 Limited, a Tencent company.
* All rights reserved.
*
* If you have downloaded a copy of the tRPC source code from Tencent,
* please note that tRPC source code is licensed under the Apache 2.0 License,
* A copy of the Apache 2.0 License can be found in the LICENSE file.
*/
package com.tencent.trpc.codegen.protoc;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
/**
* Describe the instruction of a protoc execution
*/
public class ProtocInstruction {
/**
* Directory contains .proto files
*/
private final Path sourceDirectory;
/**
* Name of the .proto files, relative to sourceDirectory
*/
private final Collection sourceFiles;
/**
* Directories of imported external .proto files
*/
private final List importPaths;
/**
* Code language
*/
private final Language language;
/**
* Path of the output descriptor set file
*/
private final Path output;
/**
* Protoc plugin instructions
*/
private final List pluginInstructions;
private ProtocInstruction(Path sourceDirectory,
Collection sourceFiles,
List importPaths,
Language language,
Path output,
List pluginInstructions) {
this.sourceDirectory = sourceDirectory;
this.sourceFiles = sourceFiles;
this.importPaths = importPaths;
this.language = language;
this.output = output;
this.pluginInstructions = pluginInstructions;
}
public static ProtocInstructionBuilder builder() {
return new ProtocInstructionBuilder();
}
public Path getSourceDirectory() {
return sourceDirectory;
}
public Collection getSourceFiles() {
return sourceFiles;
}
public List getImportPaths() {
return importPaths;
}
public Language getLanguage() {
return language;
}
public Path getOutput() {
return output;
}
public List getPluginInstructions() {
return pluginInstructions;
}
public static final class ProtocInstructionBuilder {
private Path sourceDirectory;
private Collection sourceFiles;
private List importPaths;
private Language language;
private Path output;
private final List pluginInstructions = new ArrayList<>();
private ProtocInstructionBuilder() {
}
/**
* Set proto source Directory
*/
public ProtocInstructionBuilder sourceDirectory(Path sourceDirectory) {
this.sourceDirectory = sourceDirectory;
return this;
}
/**
* Set proto source filenames, relative to sourceDirectory
*/
public ProtocInstructionBuilder sourceFiles(Collection sourceFiles) {
this.sourceFiles = sourceFiles;
return this;
}
/**
* Set directories of imported external .proto files
*/
public ProtocInstructionBuilder importPaths(List importPaths) {
this.importPaths = importPaths;
return this;
}
/**
* Set code language
*/
public ProtocInstructionBuilder language(Language language) {
this.language = language;
return this;
}
/**
* Set code output path
*/
public ProtocInstructionBuilder output(Path output) {
this.output = output;
return this;
}
/**
* Set plugin instruction
*/
public ProtocInstructionBuilder pluginInstruction(ProtocPluginInstruction pluginInstruction) {
this.pluginInstructions.add(pluginInstruction);
return this;
}
public ProtocInstruction build() {
return new ProtocInstruction(sourceDirectory, sourceFiles, importPaths, language,
output, pluginInstructions);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy