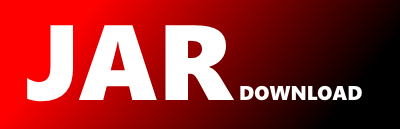
org.slf4j.TrpcMDCAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of trpc-core Show documentation
Show all versions of trpc-core Show documentation
The core module of tencent trpc Java project
/*
* Tencent is pleased to support the open source community by making tRPC available.
*
* Copyright (C) 2023 THL A29 Limited, a Tencent company.
* All rights reserved.
*
* If you have downloaded a copy of the tRPC source code from Tencent,
* please note that tRPC source code is licensed under the Apache 2.0 License,
* A copy of the Apache 2.0 License can be found in the LICENSE file.
*/
package org.slf4j;
import com.alibaba.ttl.TransmittableThreadLocal;
import com.tencent.trpc.core.utils.StringUtils;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.concurrent.ConcurrentHashMap;
import org.slf4j.spi.MDCAdapter;
/**
* TrpcMDCAdapter
*
* trpc internal cross-thread context tracking.
*
* Due to the implementation of the MDC class, the MDC.mdcAdapter does not provide read and write interfaces, so
* the package is org.slf4j.
*
* @link https://github.com/alibaba/transmittable-thread-local
*/
public class TrpcMDCAdapter implements MDCAdapter {
private static final int WRITE_OPERATION = 1;
private static final int READ_OPERATION = 2;
private static final TrpcMDCAdapter mtcMDCAdapter;
private final ThreadLocal
© 2015 - 2024 Weber Informatics LLC | Privacy Policy