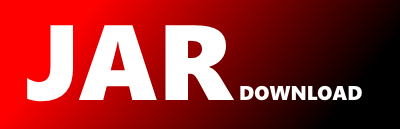
com.tencent.trpc.spring.context.configuration.schema.server.ServerServiceSchema Maven / Gradle / Ivy
/*
* Tencent is pleased to support the open source community by making tRPC available.
*
* Copyright (C) 2023 THL A29 Limited, a Tencent company.
* All rights reserved.
*
* If you have downloaded a copy of the tRPC source code from Tencent,
* please note that tRPC source code is licensed under the Apache 2.0 License,
* A copy of the Apache 2.0 License can be found in the LICENSE file.
*/
package com.tencent.trpc.spring.context.configuration.schema.server;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.tencent.trpc.spring.context.configuration.schema.AbstractProtocolSchema;
import java.util.List;
import java.util.Map;
/**
* Configurations for tRPC server service
*
* @see com.tencent.trpc.core.common.config.ServiceConfig
*/
public class ServerServiceSchema extends AbstractProtocolSchema {
/**
* Service name
*/
private String name;
/**
* Service version
*/
private String version;
/**
* Service group
*/
private String group;
/**
* Service implementation class names
*
* @see ServiceProviderSchema
*/
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy