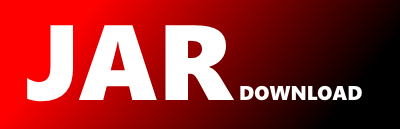
com.tencentcloudapi.cwp.v20180228.models.ModifyMalwareTimingScanSettingsRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.cwp.v20180228.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class ModifyMalwareTimingScanSettingsRequest extends AbstractModel {
/**
* Detection mode. 0: full disk detection; 1: rapid detection
*/
@SerializedName("CheckPattern")
@Expose
private Long CheckPattern;
/**
* Detection interval start time, such as 02:00:00
*/
@SerializedName("StartTime")
@Expose
private String StartTime;
/**
* Detection interval timeout end time, such as 04:00:00
*/
@SerializedName("EndTime")
@Expose
private String EndTime;
/**
* Whether all servers. 1: all; 2: specified
*/
@SerializedName("IsGlobal")
@Expose
private Long IsGlobal;
/**
* Regular detection switch. 0: off; 1: on
*/
@SerializedName("EnableScan")
@Expose
private Long EnableScan;
/**
* Monitoring mode. 0: standard; 1: deep
*/
@SerializedName("MonitoringPattern")
@Expose
private Long MonitoringPattern;
/**
* Scan interval: defaults to 1 per day
*/
@SerializedName("Cycle")
@Expose
private Long Cycle;
/**
* Real-time monitoring. 0: off; 1: on
*/
@SerializedName("RealTimeMonitoring")
@Expose
private Long RealTimeMonitoring;
/**
* Required for specified servers, a string array of host QUUIDs
*/
@SerializedName("QuuidList")
@Expose
private String [] QuuidList;
/**
* Whether to apply automatic isolation. 1: yes; 0: no
*/
@SerializedName("AutoIsolation")
@Expose
private Long AutoIsolation;
/**
* Whether to terminate the process. 1: terminate; 0: not terminate
*/
@SerializedName("KillProcess")
@Expose
private Long KillProcess;
/**
* 1: clean up; 0: not clean up
This operation will fix tampered system commands, scheduled tasks, and other system files. Please ensure that yum/apt is available during the operation.
*/
@SerializedName("DoClean")
@Expose
private Long DoClean;
/**
* 1: standard mode (only critical and high-risk are reported.); 2: enhanced mode (critical, high-risk, and medium-risk are reported.); 3: strict mode (critical, high-risk, medium-risk, low-risk, and prompt are reported.)
*/
@SerializedName("EngineType")
@Expose
private Long EngineType;
/**
* Heuristic Engine Switch: 0 OFF, 1 ON.
*/
@SerializedName("EnableInspiredEngine")
@Expose
private Long EnableInspiredEngine;
/**
* Whether to enable malicious process killing [0: Not Enabled; 1: Enabled]
*/
@SerializedName("EnableMemShellScan")
@Expose
private Long EnableMemShellScan;
/**
* Protection mode: 0: standard; 1: important period guarantee
*/
@SerializedName("ProtectMode")
@Expose
private Long ProtectMode;
/**
* Scope of detection: 0: malicious files other than scripts; 1: all malicious files
*/
@SerializedName("ProtectFileScope")
@Expose
private Long ProtectFileScope;
/**
* Get Detection mode. 0: full disk detection; 1: rapid detection
* @return CheckPattern Detection mode. 0: full disk detection; 1: rapid detection
*/
public Long getCheckPattern() {
return this.CheckPattern;
}
/**
* Set Detection mode. 0: full disk detection; 1: rapid detection
* @param CheckPattern Detection mode. 0: full disk detection; 1: rapid detection
*/
public void setCheckPattern(Long CheckPattern) {
this.CheckPattern = CheckPattern;
}
/**
* Get Detection interval start time, such as 02:00:00
* @return StartTime Detection interval start time, such as 02:00:00
*/
public String getStartTime() {
return this.StartTime;
}
/**
* Set Detection interval start time, such as 02:00:00
* @param StartTime Detection interval start time, such as 02:00:00
*/
public void setStartTime(String StartTime) {
this.StartTime = StartTime;
}
/**
* Get Detection interval timeout end time, such as 04:00:00
* @return EndTime Detection interval timeout end time, such as 04:00:00
*/
public String getEndTime() {
return this.EndTime;
}
/**
* Set Detection interval timeout end time, such as 04:00:00
* @param EndTime Detection interval timeout end time, such as 04:00:00
*/
public void setEndTime(String EndTime) {
this.EndTime = EndTime;
}
/**
* Get Whether all servers. 1: all; 2: specified
* @return IsGlobal Whether all servers. 1: all; 2: specified
*/
public Long getIsGlobal() {
return this.IsGlobal;
}
/**
* Set Whether all servers. 1: all; 2: specified
* @param IsGlobal Whether all servers. 1: all; 2: specified
*/
public void setIsGlobal(Long IsGlobal) {
this.IsGlobal = IsGlobal;
}
/**
* Get Regular detection switch. 0: off; 1: on
* @return EnableScan Regular detection switch. 0: off; 1: on
*/
public Long getEnableScan() {
return this.EnableScan;
}
/**
* Set Regular detection switch. 0: off; 1: on
* @param EnableScan Regular detection switch. 0: off; 1: on
*/
public void setEnableScan(Long EnableScan) {
this.EnableScan = EnableScan;
}
/**
* Get Monitoring mode. 0: standard; 1: deep
* @return MonitoringPattern Monitoring mode. 0: standard; 1: deep
*/
public Long getMonitoringPattern() {
return this.MonitoringPattern;
}
/**
* Set Monitoring mode. 0: standard; 1: deep
* @param MonitoringPattern Monitoring mode. 0: standard; 1: deep
*/
public void setMonitoringPattern(Long MonitoringPattern) {
this.MonitoringPattern = MonitoringPattern;
}
/**
* Get Scan interval: defaults to 1 per day
* @return Cycle Scan interval: defaults to 1 per day
*/
public Long getCycle() {
return this.Cycle;
}
/**
* Set Scan interval: defaults to 1 per day
* @param Cycle Scan interval: defaults to 1 per day
*/
public void setCycle(Long Cycle) {
this.Cycle = Cycle;
}
/**
* Get Real-time monitoring. 0: off; 1: on
* @return RealTimeMonitoring Real-time monitoring. 0: off; 1: on
*/
public Long getRealTimeMonitoring() {
return this.RealTimeMonitoring;
}
/**
* Set Real-time monitoring. 0: off; 1: on
* @param RealTimeMonitoring Real-time monitoring. 0: off; 1: on
*/
public void setRealTimeMonitoring(Long RealTimeMonitoring) {
this.RealTimeMonitoring = RealTimeMonitoring;
}
/**
* Get Required for specified servers, a string array of host QUUIDs
* @return QuuidList Required for specified servers, a string array of host QUUIDs
*/
public String [] getQuuidList() {
return this.QuuidList;
}
/**
* Set Required for specified servers, a string array of host QUUIDs
* @param QuuidList Required for specified servers, a string array of host QUUIDs
*/
public void setQuuidList(String [] QuuidList) {
this.QuuidList = QuuidList;
}
/**
* Get Whether to apply automatic isolation. 1: yes; 0: no
* @return AutoIsolation Whether to apply automatic isolation. 1: yes; 0: no
*/
public Long getAutoIsolation() {
return this.AutoIsolation;
}
/**
* Set Whether to apply automatic isolation. 1: yes; 0: no
* @param AutoIsolation Whether to apply automatic isolation. 1: yes; 0: no
*/
public void setAutoIsolation(Long AutoIsolation) {
this.AutoIsolation = AutoIsolation;
}
/**
* Get Whether to terminate the process. 1: terminate; 0: not terminate
* @return KillProcess Whether to terminate the process. 1: terminate; 0: not terminate
*/
public Long getKillProcess() {
return this.KillProcess;
}
/**
* Set Whether to terminate the process. 1: terminate; 0: not terminate
* @param KillProcess Whether to terminate the process. 1: terminate; 0: not terminate
*/
public void setKillProcess(Long KillProcess) {
this.KillProcess = KillProcess;
}
/**
* Get 1: clean up; 0: not clean up
This operation will fix tampered system commands, scheduled tasks, and other system files. Please ensure that yum/apt is available during the operation.
* @return DoClean 1: clean up; 0: not clean up
This operation will fix tampered system commands, scheduled tasks, and other system files. Please ensure that yum/apt is available during the operation.
*/
public Long getDoClean() {
return this.DoClean;
}
/**
* Set 1: clean up; 0: not clean up
This operation will fix tampered system commands, scheduled tasks, and other system files. Please ensure that yum/apt is available during the operation.
* @param DoClean 1: clean up; 0: not clean up
This operation will fix tampered system commands, scheduled tasks, and other system files. Please ensure that yum/apt is available during the operation.
*/
public void setDoClean(Long DoClean) {
this.DoClean = DoClean;
}
/**
* Get 1: standard mode (only critical and high-risk are reported.); 2: enhanced mode (critical, high-risk, and medium-risk are reported.); 3: strict mode (critical, high-risk, medium-risk, low-risk, and prompt are reported.)
* @return EngineType 1: standard mode (only critical and high-risk are reported.); 2: enhanced mode (critical, high-risk, and medium-risk are reported.); 3: strict mode (critical, high-risk, medium-risk, low-risk, and prompt are reported.)
*/
public Long getEngineType() {
return this.EngineType;
}
/**
* Set 1: standard mode (only critical and high-risk are reported.); 2: enhanced mode (critical, high-risk, and medium-risk are reported.); 3: strict mode (critical, high-risk, medium-risk, low-risk, and prompt are reported.)
* @param EngineType 1: standard mode (only critical and high-risk are reported.); 2: enhanced mode (critical, high-risk, and medium-risk are reported.); 3: strict mode (critical, high-risk, medium-risk, low-risk, and prompt are reported.)
*/
public void setEngineType(Long EngineType) {
this.EngineType = EngineType;
}
/**
* Get Heuristic Engine Switch: 0 OFF, 1 ON.
* @return EnableInspiredEngine Heuristic Engine Switch: 0 OFF, 1 ON.
*/
public Long getEnableInspiredEngine() {
return this.EnableInspiredEngine;
}
/**
* Set Heuristic Engine Switch: 0 OFF, 1 ON.
* @param EnableInspiredEngine Heuristic Engine Switch: 0 OFF, 1 ON.
*/
public void setEnableInspiredEngine(Long EnableInspiredEngine) {
this.EnableInspiredEngine = EnableInspiredEngine;
}
/**
* Get Whether to enable malicious process killing [0: Not Enabled; 1: Enabled]
* @return EnableMemShellScan Whether to enable malicious process killing [0: Not Enabled; 1: Enabled]
*/
public Long getEnableMemShellScan() {
return this.EnableMemShellScan;
}
/**
* Set Whether to enable malicious process killing [0: Not Enabled; 1: Enabled]
* @param EnableMemShellScan Whether to enable malicious process killing [0: Not Enabled; 1: Enabled]
*/
public void setEnableMemShellScan(Long EnableMemShellScan) {
this.EnableMemShellScan = EnableMemShellScan;
}
/**
* Get Protection mode: 0: standard; 1: important period guarantee
* @return ProtectMode Protection mode: 0: standard; 1: important period guarantee
*/
public Long getProtectMode() {
return this.ProtectMode;
}
/**
* Set Protection mode: 0: standard; 1: important period guarantee
* @param ProtectMode Protection mode: 0: standard; 1: important period guarantee
*/
public void setProtectMode(Long ProtectMode) {
this.ProtectMode = ProtectMode;
}
/**
* Get Scope of detection: 0: malicious files other than scripts; 1: all malicious files
* @return ProtectFileScope Scope of detection: 0: malicious files other than scripts; 1: all malicious files
*/
public Long getProtectFileScope() {
return this.ProtectFileScope;
}
/**
* Set Scope of detection: 0: malicious files other than scripts; 1: all malicious files
* @param ProtectFileScope Scope of detection: 0: malicious files other than scripts; 1: all malicious files
*/
public void setProtectFileScope(Long ProtectFileScope) {
this.ProtectFileScope = ProtectFileScope;
}
public ModifyMalwareTimingScanSettingsRequest() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public ModifyMalwareTimingScanSettingsRequest(ModifyMalwareTimingScanSettingsRequest source) {
if (source.CheckPattern != null) {
this.CheckPattern = new Long(source.CheckPattern);
}
if (source.StartTime != null) {
this.StartTime = new String(source.StartTime);
}
if (source.EndTime != null) {
this.EndTime = new String(source.EndTime);
}
if (source.IsGlobal != null) {
this.IsGlobal = new Long(source.IsGlobal);
}
if (source.EnableScan != null) {
this.EnableScan = new Long(source.EnableScan);
}
if (source.MonitoringPattern != null) {
this.MonitoringPattern = new Long(source.MonitoringPattern);
}
if (source.Cycle != null) {
this.Cycle = new Long(source.Cycle);
}
if (source.RealTimeMonitoring != null) {
this.RealTimeMonitoring = new Long(source.RealTimeMonitoring);
}
if (source.QuuidList != null) {
this.QuuidList = new String[source.QuuidList.length];
for (int i = 0; i < source.QuuidList.length; i++) {
this.QuuidList[i] = new String(source.QuuidList[i]);
}
}
if (source.AutoIsolation != null) {
this.AutoIsolation = new Long(source.AutoIsolation);
}
if (source.KillProcess != null) {
this.KillProcess = new Long(source.KillProcess);
}
if (source.DoClean != null) {
this.DoClean = new Long(source.DoClean);
}
if (source.EngineType != null) {
this.EngineType = new Long(source.EngineType);
}
if (source.EnableInspiredEngine != null) {
this.EnableInspiredEngine = new Long(source.EnableInspiredEngine);
}
if (source.EnableMemShellScan != null) {
this.EnableMemShellScan = new Long(source.EnableMemShellScan);
}
if (source.ProtectMode != null) {
this.ProtectMode = new Long(source.ProtectMode);
}
if (source.ProtectFileScope != null) {
this.ProtectFileScope = new Long(source.ProtectFileScope);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "CheckPattern", this.CheckPattern);
this.setParamSimple(map, prefix + "StartTime", this.StartTime);
this.setParamSimple(map, prefix + "EndTime", this.EndTime);
this.setParamSimple(map, prefix + "IsGlobal", this.IsGlobal);
this.setParamSimple(map, prefix + "EnableScan", this.EnableScan);
this.setParamSimple(map, prefix + "MonitoringPattern", this.MonitoringPattern);
this.setParamSimple(map, prefix + "Cycle", this.Cycle);
this.setParamSimple(map, prefix + "RealTimeMonitoring", this.RealTimeMonitoring);
this.setParamArraySimple(map, prefix + "QuuidList.", this.QuuidList);
this.setParamSimple(map, prefix + "AutoIsolation", this.AutoIsolation);
this.setParamSimple(map, prefix + "KillProcess", this.KillProcess);
this.setParamSimple(map, prefix + "DoClean", this.DoClean);
this.setParamSimple(map, prefix + "EngineType", this.EngineType);
this.setParamSimple(map, prefix + "EnableInspiredEngine", this.EnableInspiredEngine);
this.setParamSimple(map, prefix + "EnableMemShellScan", this.EnableMemShellScan);
this.setParamSimple(map, prefix + "ProtectMode", this.ProtectMode);
this.setParamSimple(map, prefix + "ProtectFileScope", this.ProtectFileScope);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy