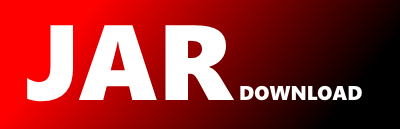
com.tencentcloudapi.cwp.v20180228.models.ModifyMalwareWhiteListRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.cwp.v20180228.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class ModifyMalwareWhiteListRequest extends AbstractModel {
/**
* Allowlist mode. 0: MD5 allowlist; 1: custom.
*/
@SerializedName("Mode")
@Expose
private Long Mode;
/**
* Unique rule ID
*/
@SerializedName("Id")
@Expose
private Long Id;
/**
* QUUID list
*/
@SerializedName("QuuidList")
@Expose
private String [] QuuidList;
/**
* Whether the allowlist applies to all hosts. 0: no; 1: yes.
*/
@SerializedName("IsGlobal")
@Expose
private Long IsGlobal;
/**
* Matching pattern; 0 for exact match, 1 for fuzzy match (deprecated)
*/
@SerializedName("MatchType")
@Expose
private Long MatchType;
/**
* File Name (regular expression); up to 200 characters in length
*/
@SerializedName("FileName")
@Expose
private String [] FileName;
/**
* File Directory (regular expression); up to 200 characters in length, content base64 encoded.
*/
@SerializedName("FileDirectory")
@Expose
private String [] FileDirectory;
/**
* File Suffix; Up to 200 characters in length, content base64 escaped (deprecated).
*/
@SerializedName("FileExtension")
@Expose
private String [] FileExtension;
/**
* MD5 list
*/
@SerializedName("Md5List")
@Expose
private String [] Md5List;
/**
* Get Allowlist mode. 0: MD5 allowlist; 1: custom.
* @return Mode Allowlist mode. 0: MD5 allowlist; 1: custom.
*/
public Long getMode() {
return this.Mode;
}
/**
* Set Allowlist mode. 0: MD5 allowlist; 1: custom.
* @param Mode Allowlist mode. 0: MD5 allowlist; 1: custom.
*/
public void setMode(Long Mode) {
this.Mode = Mode;
}
/**
* Get Unique rule ID
* @return Id Unique rule ID
*/
public Long getId() {
return this.Id;
}
/**
* Set Unique rule ID
* @param Id Unique rule ID
*/
public void setId(Long Id) {
this.Id = Id;
}
/**
* Get QUUID list
* @return QuuidList QUUID list
*/
public String [] getQuuidList() {
return this.QuuidList;
}
/**
* Set QUUID list
* @param QuuidList QUUID list
*/
public void setQuuidList(String [] QuuidList) {
this.QuuidList = QuuidList;
}
/**
* Get Whether the allowlist applies to all hosts. 0: no; 1: yes.
* @return IsGlobal Whether the allowlist applies to all hosts. 0: no; 1: yes.
*/
public Long getIsGlobal() {
return this.IsGlobal;
}
/**
* Set Whether the allowlist applies to all hosts. 0: no; 1: yes.
* @param IsGlobal Whether the allowlist applies to all hosts. 0: no; 1: yes.
*/
public void setIsGlobal(Long IsGlobal) {
this.IsGlobal = IsGlobal;
}
/**
* Get Matching pattern; 0 for exact match, 1 for fuzzy match (deprecated)
* @return MatchType Matching pattern; 0 for exact match, 1 for fuzzy match (deprecated)
*/
public Long getMatchType() {
return this.MatchType;
}
/**
* Set Matching pattern; 0 for exact match, 1 for fuzzy match (deprecated)
* @param MatchType Matching pattern; 0 for exact match, 1 for fuzzy match (deprecated)
*/
public void setMatchType(Long MatchType) {
this.MatchType = MatchType;
}
/**
* Get File Name (regular expression); up to 200 characters in length
* @return FileName File Name (regular expression); up to 200 characters in length
*/
public String [] getFileName() {
return this.FileName;
}
/**
* Set File Name (regular expression); up to 200 characters in length
* @param FileName File Name (regular expression); up to 200 characters in length
*/
public void setFileName(String [] FileName) {
this.FileName = FileName;
}
/**
* Get File Directory (regular expression); up to 200 characters in length, content base64 encoded.
* @return FileDirectory File Directory (regular expression); up to 200 characters in length, content base64 encoded.
*/
public String [] getFileDirectory() {
return this.FileDirectory;
}
/**
* Set File Directory (regular expression); up to 200 characters in length, content base64 encoded.
* @param FileDirectory File Directory (regular expression); up to 200 characters in length, content base64 encoded.
*/
public void setFileDirectory(String [] FileDirectory) {
this.FileDirectory = FileDirectory;
}
/**
* Get File Suffix; Up to 200 characters in length, content base64 escaped (deprecated).
* @return FileExtension File Suffix; Up to 200 characters in length, content base64 escaped (deprecated).
*/
public String [] getFileExtension() {
return this.FileExtension;
}
/**
* Set File Suffix; Up to 200 characters in length, content base64 escaped (deprecated).
* @param FileExtension File Suffix; Up to 200 characters in length, content base64 escaped (deprecated).
*/
public void setFileExtension(String [] FileExtension) {
this.FileExtension = FileExtension;
}
/**
* Get MD5 list
* @return Md5List MD5 list
*/
public String [] getMd5List() {
return this.Md5List;
}
/**
* Set MD5 list
* @param Md5List MD5 list
*/
public void setMd5List(String [] Md5List) {
this.Md5List = Md5List;
}
public ModifyMalwareWhiteListRequest() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public ModifyMalwareWhiteListRequest(ModifyMalwareWhiteListRequest source) {
if (source.Mode != null) {
this.Mode = new Long(source.Mode);
}
if (source.Id != null) {
this.Id = new Long(source.Id);
}
if (source.QuuidList != null) {
this.QuuidList = new String[source.QuuidList.length];
for (int i = 0; i < source.QuuidList.length; i++) {
this.QuuidList[i] = new String(source.QuuidList[i]);
}
}
if (source.IsGlobal != null) {
this.IsGlobal = new Long(source.IsGlobal);
}
if (source.MatchType != null) {
this.MatchType = new Long(source.MatchType);
}
if (source.FileName != null) {
this.FileName = new String[source.FileName.length];
for (int i = 0; i < source.FileName.length; i++) {
this.FileName[i] = new String(source.FileName[i]);
}
}
if (source.FileDirectory != null) {
this.FileDirectory = new String[source.FileDirectory.length];
for (int i = 0; i < source.FileDirectory.length; i++) {
this.FileDirectory[i] = new String(source.FileDirectory[i]);
}
}
if (source.FileExtension != null) {
this.FileExtension = new String[source.FileExtension.length];
for (int i = 0; i < source.FileExtension.length; i++) {
this.FileExtension[i] = new String(source.FileExtension[i]);
}
}
if (source.Md5List != null) {
this.Md5List = new String[source.Md5List.length];
for (int i = 0; i < source.Md5List.length; i++) {
this.Md5List[i] = new String(source.Md5List[i]);
}
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "Mode", this.Mode);
this.setParamSimple(map, prefix + "Id", this.Id);
this.setParamArraySimple(map, prefix + "QuuidList.", this.QuuidList);
this.setParamSimple(map, prefix + "IsGlobal", this.IsGlobal);
this.setParamSimple(map, prefix + "MatchType", this.MatchType);
this.setParamArraySimple(map, prefix + "FileName.", this.FileName);
this.setParamArraySimple(map, prefix + "FileDirectory.", this.FileDirectory);
this.setParamArraySimple(map, prefix + "FileExtension.", this.FileExtension);
this.setParamArraySimple(map, prefix + "Md5List.", this.Md5List);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy