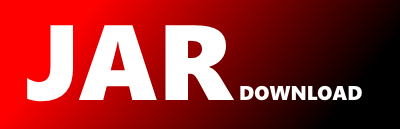
com.tencentcloudapi.vod.v20180717.models.ModifyMediaInfoRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.vod.v20180717.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class ModifyMediaInfoRequest extends AbstractModel {
/**
* Unique media file ID.
*/
@SerializedName("FileId")
@Expose
private String FileId;
/**
* The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
*/
@SerializedName("SubAppId")
@Expose
private Long SubAppId;
/**
* Media filename, which can contain up to 64 characters.
*/
@SerializedName("Name")
@Expose
private String Name;
/**
* Media file description, which can contain up to 128 characters.
*/
@SerializedName("Description")
@Expose
private String Description;
/**
* Media file category ID.
*/
@SerializedName("ClassId")
@Expose
private Long ClassId;
/**
* Media file expiration time in [ISO date format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I). The value `9999-12-31T23:59:59Z` indicates that the media file never expires. After the expiration, the media file and its related resources (such as transcoding results and image sprites) will be permanently deleted.
*/
@SerializedName("ExpireTime")
@Expose
private String ExpireTime;
/**
* String generated by [Base64-encoding](https://tools.ietf.org/html/rfc4648) the video cover image file (such as .jpeg or .png file). Only .gif, .jpeg, and .png image formats are supported.
*/
@SerializedName("CoverData")
@Expose
private String CoverData;
/**
* Set of video timestamps to be added. If a timestamp already exists at an offset time point, it will be overwritten. Up to 100 timestamps can be added to one media file. In the same request, the time offset parameters of `AddKeyFrameDescs` must be different from those of `DeleteKeyFrameDescs`.
*/
@SerializedName("AddKeyFrameDescs")
@Expose
private MediaKeyFrameDescItem [] AddKeyFrameDescs;
/**
* Time offset of the set of video timestamps to be deleted in seconds. In the same request, the time offset parameters of `AddKeyFrameDescs` must be different from those of `DeleteKeyFrameDescs`.
*/
@SerializedName("DeleteKeyFrameDescs")
@Expose
private Float [] DeleteKeyFrameDescs;
/**
* The value `1` indicates to delete all timestamps in the video. Other values are meaningless.
In the same request, `ClearKeyFrameDescs` and `AddKeyFrameDescs` cannot be present at the same time.
*/
@SerializedName("ClearKeyFrameDescs")
@Expose
private Long ClearKeyFrameDescs;
/**
* The tags to add. Each file can have up to 16 tags. A tag can contain at most 32 characters. You cannot include the same tag in `AddTags` and `DeleteTags` at the same time.
*/
@SerializedName("AddTags")
@Expose
private String [] AddTags;
/**
* Set of tags to be deleted. In the same request, the parameters of `AddTags` must be different from those of `DeleteTags`.
*/
@SerializedName("DeleteTags")
@Expose
private String [] DeleteTags;
/**
* The value `1` indicates to delete all tags of the media file. Other values are meaningless.
In the same request, `ClearTags` and `AddTags` cannot be present at the same time.
*/
@SerializedName("ClearTags")
@Expose
private Long ClearTags;
/**
* Information of multiple subtitles to be added. A single media file can have up to 16 subtitles. In the same request, the subtitle IDs specified in `AddSubtitles` must be different from those in `DeleteSubtitleIds`.
*/
@SerializedName("AddSubtitles")
@Expose
private MediaSubtitleInput [] AddSubtitles;
/**
* Unique IDs of the subtitles to be deleted. In the same request, the subtitle IDs specified in `AddSubtitles` must be different from those in `DeleteSubtitleIds`.
*/
@SerializedName("DeleteSubtitleIds")
@Expose
private String [] DeleteSubtitleIds;
/**
* The value `1` indicates to delete all subtitle information of the media file. Other values are meaningless.
`ClearSubtitles` and `AddSubtitles` cannot co-exist in the same request.
*/
@SerializedName("ClearSubtitles")
@Expose
private Long ClearSubtitles;
/**
* Get Unique media file ID.
* @return FileId Unique media file ID.
*/
public String getFileId() {
return this.FileId;
}
/**
* Set Unique media file ID.
* @param FileId Unique media file ID.
*/
public void setFileId(String FileId) {
this.FileId = FileId;
}
/**
* Get The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
* @return SubAppId The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
*/
public Long getSubAppId() {
return this.SubAppId;
}
/**
* Set The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
* @param SubAppId The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
*/
public void setSubAppId(Long SubAppId) {
this.SubAppId = SubAppId;
}
/**
* Get Media filename, which can contain up to 64 characters.
* @return Name Media filename, which can contain up to 64 characters.
*/
public String getName() {
return this.Name;
}
/**
* Set Media filename, which can contain up to 64 characters.
* @param Name Media filename, which can contain up to 64 characters.
*/
public void setName(String Name) {
this.Name = Name;
}
/**
* Get Media file description, which can contain up to 128 characters.
* @return Description Media file description, which can contain up to 128 characters.
*/
public String getDescription() {
return this.Description;
}
/**
* Set Media file description, which can contain up to 128 characters.
* @param Description Media file description, which can contain up to 128 characters.
*/
public void setDescription(String Description) {
this.Description = Description;
}
/**
* Get Media file category ID.
* @return ClassId Media file category ID.
*/
public Long getClassId() {
return this.ClassId;
}
/**
* Set Media file category ID.
* @param ClassId Media file category ID.
*/
public void setClassId(Long ClassId) {
this.ClassId = ClassId;
}
/**
* Get Media file expiration time in [ISO date format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I). The value `9999-12-31T23:59:59Z` indicates that the media file never expires. After the expiration, the media file and its related resources (such as transcoding results and image sprites) will be permanently deleted.
* @return ExpireTime Media file expiration time in [ISO date format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I). The value `9999-12-31T23:59:59Z` indicates that the media file never expires. After the expiration, the media file and its related resources (such as transcoding results and image sprites) will be permanently deleted.
*/
public String getExpireTime() {
return this.ExpireTime;
}
/**
* Set Media file expiration time in [ISO date format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I). The value `9999-12-31T23:59:59Z` indicates that the media file never expires. After the expiration, the media file and its related resources (such as transcoding results and image sprites) will be permanently deleted.
* @param ExpireTime Media file expiration time in [ISO date format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I). The value `9999-12-31T23:59:59Z` indicates that the media file never expires. After the expiration, the media file and its related resources (such as transcoding results and image sprites) will be permanently deleted.
*/
public void setExpireTime(String ExpireTime) {
this.ExpireTime = ExpireTime;
}
/**
* Get String generated by [Base64-encoding](https://tools.ietf.org/html/rfc4648) the video cover image file (such as .jpeg or .png file). Only .gif, .jpeg, and .png image formats are supported.
* @return CoverData String generated by [Base64-encoding](https://tools.ietf.org/html/rfc4648) the video cover image file (such as .jpeg or .png file). Only .gif, .jpeg, and .png image formats are supported.
*/
public String getCoverData() {
return this.CoverData;
}
/**
* Set String generated by [Base64-encoding](https://tools.ietf.org/html/rfc4648) the video cover image file (such as .jpeg or .png file). Only .gif, .jpeg, and .png image formats are supported.
* @param CoverData String generated by [Base64-encoding](https://tools.ietf.org/html/rfc4648) the video cover image file (such as .jpeg or .png file). Only .gif, .jpeg, and .png image formats are supported.
*/
public void setCoverData(String CoverData) {
this.CoverData = CoverData;
}
/**
* Get Set of video timestamps to be added. If a timestamp already exists at an offset time point, it will be overwritten. Up to 100 timestamps can be added to one media file. In the same request, the time offset parameters of `AddKeyFrameDescs` must be different from those of `DeleteKeyFrameDescs`.
* @return AddKeyFrameDescs Set of video timestamps to be added. If a timestamp already exists at an offset time point, it will be overwritten. Up to 100 timestamps can be added to one media file. In the same request, the time offset parameters of `AddKeyFrameDescs` must be different from those of `DeleteKeyFrameDescs`.
*/
public MediaKeyFrameDescItem [] getAddKeyFrameDescs() {
return this.AddKeyFrameDescs;
}
/**
* Set Set of video timestamps to be added. If a timestamp already exists at an offset time point, it will be overwritten. Up to 100 timestamps can be added to one media file. In the same request, the time offset parameters of `AddKeyFrameDescs` must be different from those of `DeleteKeyFrameDescs`.
* @param AddKeyFrameDescs Set of video timestamps to be added. If a timestamp already exists at an offset time point, it will be overwritten. Up to 100 timestamps can be added to one media file. In the same request, the time offset parameters of `AddKeyFrameDescs` must be different from those of `DeleteKeyFrameDescs`.
*/
public void setAddKeyFrameDescs(MediaKeyFrameDescItem [] AddKeyFrameDescs) {
this.AddKeyFrameDescs = AddKeyFrameDescs;
}
/**
* Get Time offset of the set of video timestamps to be deleted in seconds. In the same request, the time offset parameters of `AddKeyFrameDescs` must be different from those of `DeleteKeyFrameDescs`.
* @return DeleteKeyFrameDescs Time offset of the set of video timestamps to be deleted in seconds. In the same request, the time offset parameters of `AddKeyFrameDescs` must be different from those of `DeleteKeyFrameDescs`.
*/
public Float [] getDeleteKeyFrameDescs() {
return this.DeleteKeyFrameDescs;
}
/**
* Set Time offset of the set of video timestamps to be deleted in seconds. In the same request, the time offset parameters of `AddKeyFrameDescs` must be different from those of `DeleteKeyFrameDescs`.
* @param DeleteKeyFrameDescs Time offset of the set of video timestamps to be deleted in seconds. In the same request, the time offset parameters of `AddKeyFrameDescs` must be different from those of `DeleteKeyFrameDescs`.
*/
public void setDeleteKeyFrameDescs(Float [] DeleteKeyFrameDescs) {
this.DeleteKeyFrameDescs = DeleteKeyFrameDescs;
}
/**
* Get The value `1` indicates to delete all timestamps in the video. Other values are meaningless.
In the same request, `ClearKeyFrameDescs` and `AddKeyFrameDescs` cannot be present at the same time.
* @return ClearKeyFrameDescs The value `1` indicates to delete all timestamps in the video. Other values are meaningless.
In the same request, `ClearKeyFrameDescs` and `AddKeyFrameDescs` cannot be present at the same time.
*/
public Long getClearKeyFrameDescs() {
return this.ClearKeyFrameDescs;
}
/**
* Set The value `1` indicates to delete all timestamps in the video. Other values are meaningless.
In the same request, `ClearKeyFrameDescs` and `AddKeyFrameDescs` cannot be present at the same time.
* @param ClearKeyFrameDescs The value `1` indicates to delete all timestamps in the video. Other values are meaningless.
In the same request, `ClearKeyFrameDescs` and `AddKeyFrameDescs` cannot be present at the same time.
*/
public void setClearKeyFrameDescs(Long ClearKeyFrameDescs) {
this.ClearKeyFrameDescs = ClearKeyFrameDescs;
}
/**
* Get The tags to add. Each file can have up to 16 tags. A tag can contain at most 32 characters. You cannot include the same tag in `AddTags` and `DeleteTags` at the same time.
* @return AddTags The tags to add. Each file can have up to 16 tags. A tag can contain at most 32 characters. You cannot include the same tag in `AddTags` and `DeleteTags` at the same time.
*/
public String [] getAddTags() {
return this.AddTags;
}
/**
* Set The tags to add. Each file can have up to 16 tags. A tag can contain at most 32 characters. You cannot include the same tag in `AddTags` and `DeleteTags` at the same time.
* @param AddTags The tags to add. Each file can have up to 16 tags. A tag can contain at most 32 characters. You cannot include the same tag in `AddTags` and `DeleteTags` at the same time.
*/
public void setAddTags(String [] AddTags) {
this.AddTags = AddTags;
}
/**
* Get Set of tags to be deleted. In the same request, the parameters of `AddTags` must be different from those of `DeleteTags`.
* @return DeleteTags Set of tags to be deleted. In the same request, the parameters of `AddTags` must be different from those of `DeleteTags`.
*/
public String [] getDeleteTags() {
return this.DeleteTags;
}
/**
* Set Set of tags to be deleted. In the same request, the parameters of `AddTags` must be different from those of `DeleteTags`.
* @param DeleteTags Set of tags to be deleted. In the same request, the parameters of `AddTags` must be different from those of `DeleteTags`.
*/
public void setDeleteTags(String [] DeleteTags) {
this.DeleteTags = DeleteTags;
}
/**
* Get The value `1` indicates to delete all tags of the media file. Other values are meaningless.
In the same request, `ClearTags` and `AddTags` cannot be present at the same time.
* @return ClearTags The value `1` indicates to delete all tags of the media file. Other values are meaningless.
In the same request, `ClearTags` and `AddTags` cannot be present at the same time.
*/
public Long getClearTags() {
return this.ClearTags;
}
/**
* Set The value `1` indicates to delete all tags of the media file. Other values are meaningless.
In the same request, `ClearTags` and `AddTags` cannot be present at the same time.
* @param ClearTags The value `1` indicates to delete all tags of the media file. Other values are meaningless.
In the same request, `ClearTags` and `AddTags` cannot be present at the same time.
*/
public void setClearTags(Long ClearTags) {
this.ClearTags = ClearTags;
}
/**
* Get Information of multiple subtitles to be added. A single media file can have up to 16 subtitles. In the same request, the subtitle IDs specified in `AddSubtitles` must be different from those in `DeleteSubtitleIds`.
* @return AddSubtitles Information of multiple subtitles to be added. A single media file can have up to 16 subtitles. In the same request, the subtitle IDs specified in `AddSubtitles` must be different from those in `DeleteSubtitleIds`.
*/
public MediaSubtitleInput [] getAddSubtitles() {
return this.AddSubtitles;
}
/**
* Set Information of multiple subtitles to be added. A single media file can have up to 16 subtitles. In the same request, the subtitle IDs specified in `AddSubtitles` must be different from those in `DeleteSubtitleIds`.
* @param AddSubtitles Information of multiple subtitles to be added. A single media file can have up to 16 subtitles. In the same request, the subtitle IDs specified in `AddSubtitles` must be different from those in `DeleteSubtitleIds`.
*/
public void setAddSubtitles(MediaSubtitleInput [] AddSubtitles) {
this.AddSubtitles = AddSubtitles;
}
/**
* Get Unique IDs of the subtitles to be deleted. In the same request, the subtitle IDs specified in `AddSubtitles` must be different from those in `DeleteSubtitleIds`.
* @return DeleteSubtitleIds Unique IDs of the subtitles to be deleted. In the same request, the subtitle IDs specified in `AddSubtitles` must be different from those in `DeleteSubtitleIds`.
*/
public String [] getDeleteSubtitleIds() {
return this.DeleteSubtitleIds;
}
/**
* Set Unique IDs of the subtitles to be deleted. In the same request, the subtitle IDs specified in `AddSubtitles` must be different from those in `DeleteSubtitleIds`.
* @param DeleteSubtitleIds Unique IDs of the subtitles to be deleted. In the same request, the subtitle IDs specified in `AddSubtitles` must be different from those in `DeleteSubtitleIds`.
*/
public void setDeleteSubtitleIds(String [] DeleteSubtitleIds) {
this.DeleteSubtitleIds = DeleteSubtitleIds;
}
/**
* Get The value `1` indicates to delete all subtitle information of the media file. Other values are meaningless.
`ClearSubtitles` and `AddSubtitles` cannot co-exist in the same request.
* @return ClearSubtitles The value `1` indicates to delete all subtitle information of the media file. Other values are meaningless.
`ClearSubtitles` and `AddSubtitles` cannot co-exist in the same request.
*/
public Long getClearSubtitles() {
return this.ClearSubtitles;
}
/**
* Set The value `1` indicates to delete all subtitle information of the media file. Other values are meaningless.
`ClearSubtitles` and `AddSubtitles` cannot co-exist in the same request.
* @param ClearSubtitles The value `1` indicates to delete all subtitle information of the media file. Other values are meaningless.
`ClearSubtitles` and `AddSubtitles` cannot co-exist in the same request.
*/
public void setClearSubtitles(Long ClearSubtitles) {
this.ClearSubtitles = ClearSubtitles;
}
public ModifyMediaInfoRequest() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public ModifyMediaInfoRequest(ModifyMediaInfoRequest source) {
if (source.FileId != null) {
this.FileId = new String(source.FileId);
}
if (source.SubAppId != null) {
this.SubAppId = new Long(source.SubAppId);
}
if (source.Name != null) {
this.Name = new String(source.Name);
}
if (source.Description != null) {
this.Description = new String(source.Description);
}
if (source.ClassId != null) {
this.ClassId = new Long(source.ClassId);
}
if (source.ExpireTime != null) {
this.ExpireTime = new String(source.ExpireTime);
}
if (source.CoverData != null) {
this.CoverData = new String(source.CoverData);
}
if (source.AddKeyFrameDescs != null) {
this.AddKeyFrameDescs = new MediaKeyFrameDescItem[source.AddKeyFrameDescs.length];
for (int i = 0; i < source.AddKeyFrameDescs.length; i++) {
this.AddKeyFrameDescs[i] = new MediaKeyFrameDescItem(source.AddKeyFrameDescs[i]);
}
}
if (source.DeleteKeyFrameDescs != null) {
this.DeleteKeyFrameDescs = new Float[source.DeleteKeyFrameDescs.length];
for (int i = 0; i < source.DeleteKeyFrameDescs.length; i++) {
this.DeleteKeyFrameDescs[i] = new Float(source.DeleteKeyFrameDescs[i]);
}
}
if (source.ClearKeyFrameDescs != null) {
this.ClearKeyFrameDescs = new Long(source.ClearKeyFrameDescs);
}
if (source.AddTags != null) {
this.AddTags = new String[source.AddTags.length];
for (int i = 0; i < source.AddTags.length; i++) {
this.AddTags[i] = new String(source.AddTags[i]);
}
}
if (source.DeleteTags != null) {
this.DeleteTags = new String[source.DeleteTags.length];
for (int i = 0; i < source.DeleteTags.length; i++) {
this.DeleteTags[i] = new String(source.DeleteTags[i]);
}
}
if (source.ClearTags != null) {
this.ClearTags = new Long(source.ClearTags);
}
if (source.AddSubtitles != null) {
this.AddSubtitles = new MediaSubtitleInput[source.AddSubtitles.length];
for (int i = 0; i < source.AddSubtitles.length; i++) {
this.AddSubtitles[i] = new MediaSubtitleInput(source.AddSubtitles[i]);
}
}
if (source.DeleteSubtitleIds != null) {
this.DeleteSubtitleIds = new String[source.DeleteSubtitleIds.length];
for (int i = 0; i < source.DeleteSubtitleIds.length; i++) {
this.DeleteSubtitleIds[i] = new String(source.DeleteSubtitleIds[i]);
}
}
if (source.ClearSubtitles != null) {
this.ClearSubtitles = new Long(source.ClearSubtitles);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "FileId", this.FileId);
this.setParamSimple(map, prefix + "SubAppId", this.SubAppId);
this.setParamSimple(map, prefix + "Name", this.Name);
this.setParamSimple(map, prefix + "Description", this.Description);
this.setParamSimple(map, prefix + "ClassId", this.ClassId);
this.setParamSimple(map, prefix + "ExpireTime", this.ExpireTime);
this.setParamSimple(map, prefix + "CoverData", this.CoverData);
this.setParamArrayObj(map, prefix + "AddKeyFrameDescs.", this.AddKeyFrameDescs);
this.setParamArraySimple(map, prefix + "DeleteKeyFrameDescs.", this.DeleteKeyFrameDescs);
this.setParamSimple(map, prefix + "ClearKeyFrameDescs", this.ClearKeyFrameDescs);
this.setParamArraySimple(map, prefix + "AddTags.", this.AddTags);
this.setParamArraySimple(map, prefix + "DeleteTags.", this.DeleteTags);
this.setParamSimple(map, prefix + "ClearTags", this.ClearTags);
this.setParamArrayObj(map, prefix + "AddSubtitles.", this.AddSubtitles);
this.setParamArraySimple(map, prefix + "DeleteSubtitleIds.", this.DeleteSubtitleIds);
this.setParamSimple(map, prefix + "ClearSubtitles", this.ClearSubtitles);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy