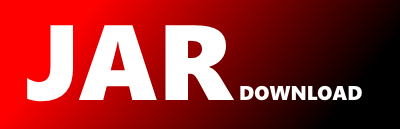
com.tencentcloudapi.vod.v20180717.models.SearchMediaRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.vod.v20180717.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class SearchMediaRequest extends AbstractModel {
/**
* The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
*/
@SerializedName("SubAppId")
@Expose
private Long SubAppId;
/**
* File ID set. Any element in the set can be matched.
Array length limit: 10.
ID length limit: 40 characters.
*/
@SerializedName("FileIds")
@Expose
private String [] FileIds;
/**
* The file names to use for fuzzy search, which are sorted by relevance in descending order.
Name length limit: 100 characters.
Array length limit: 10
*/
@SerializedName("Names")
@Expose
private String [] Names;
/**
* The file name prefixes to search.
Prefix length limit: 100 characters.
Array length limit: 10.
*/
@SerializedName("NamePrefixes")
@Expose
private String [] NamePrefixes;
/**
* File description set. Media file descriptions are fuzzily matched. The higher the match rate, the higher-ranked the result.
Length limit for a single description: 100 characters
Array length limit: 10
*/
@SerializedName("Descriptions")
@Expose
private String [] Descriptions;
/**
* Category ID set. The categories of the specified IDs and all subcategories in the set are matched.
Array length limit: 10.
*/
@SerializedName("ClassIds")
@Expose
private Long [] ClassIds;
/**
* The tags to search. A file is considered a match if it has any of the tags specified.
Tag length limit: 32 characters.
Array length limit: 16.
*/
@SerializedName("Tags")
@Expose
private String [] Tags;
/**
* File type. Any element in the set can be matched.
Video: video file
Audio: audio file
Image: image file
*/
@SerializedName("Categories")
@Expose
private String [] Categories;
/**
* Media file source set. For valid values, please see [SourceType](https://intl.cloud.tencent.com/document/product/266/31773?from_cn_redirect=1#MediaSourceData).
Array length limit: 10.
*/
@SerializedName("SourceTypes")
@Expose
private String [] SourceTypes;
/**
* The live stream code array. A media file will be returned if it matches any element in the array.
Array length limit: 10
*/
@SerializedName("StreamIds")
@Expose
private String [] StreamIds;
/**
* Matches files created within the time period.
Includes specified start and end points in time.
*/
@SerializedName("CreateTime")
@Expose
private TimeRange CreateTime;
/**
* Files whose expiration time points are within the specified time range will be returned. Expired files will not be returned.
The files whose expiration time points are on the start or end time of the specified range will also be returned.
*/
@SerializedName("ExpireTime")
@Expose
private TimeRange ExpireTime;
/**
* Regions where media files are stored, such as `ap-chongqing`. For more regions, see [Storage Regions](https://intl.cloud.tencent.com/document/product/266/9760?from_cn_redirect=1#.E5.B7.B2.E6.94.AF.E6.8C.81.E5.9C.B0.E5.9F.9F.E5.88.97.E8.A1.A8).
Length limit for a single region: 20 characters
Array length limit: 20
*/
@SerializedName("StorageRegions")
@Expose
private String [] StorageRegions;
/**
* An array of storage classes. Valid values:
STANDARD
STANDARD_IA
ARCHIVE
DEEP_ARCHIVE
*/
@SerializedName("StorageClasses")
@Expose
private String [] StorageClasses;
/**
* The file formats.
Array length limit: 10
*/
@SerializedName("MediaTypes")
@Expose
private String [] MediaTypes;
/**
* The file statuses.
`Normal`
`SystemForbidden` (blocked by VOD)
`Forbidden` (blocked by you)
*/
@SerializedName("Status")
@Expose
private String [] Status;
/**
* The types of moderation result.
`pass`
`review` (the content may be non-compliant and needs to be reviewed)
`block` (the content is non-compliant and should be blocked)
`notModerated` (the file hasn't been moderated yet)
*/
@SerializedName("ReviewResults")
@Expose
private String [] ReviewResults;
/**
* The TRTC application IDs. Any file that matches one of the application IDs will be returned.
Array length limit: 10
*/
@SerializedName("TrtcSdkAppIds")
@Expose
private Long [] TrtcSdkAppIds;
/**
* The TRTC room IDs. Any file that matches one of the room IDs will be returned.
Element length limit: 64 characters.
Array length limit: 10.
*/
@SerializedName("TrtcRoomIds")
@Expose
private String [] TrtcRoomIds;
/**
* Specifies information entry that needs to be returned for all media files. Multiple entries can be specified simultaneously. N starts from 0. If this field is left empty, all information entries will be returned by default. Valid values:
basicInfo (basic video information).
metaData (video metadata).
transcodeInfo (result information of video transcoding).
animatedGraphicsInfo (result information of animated image generating task).
imageSpriteInfo (image sprite information).
snapshotByTimeOffsetInfo (point-in-time screenshot information).
sampleSnapshotInfo (sampled screenshot information).
keyFrameDescInfo (timestamp information).
adaptiveDynamicStreamingInfo (information of adaptive bitrate streaming).
miniProgramReviewInfo (WeChat Mini Program audit information).
*/
@SerializedName("Filters")
@Expose
private String [] Filters;
/**
* Sorting order.
Valid value of `Sort.Field`: CreateTime.
If `Text`, `Names`, or `Descriptions` is not empty, the `Sort.Field` field will not take effect, and the search results will be sorted by match rate.
*/
@SerializedName("Sort")
@Expose
private SortBy Sort;
/**
* Start offset of a paged return. Default value: 0. Entries from No. "Offset" to No. "Offset + Limit - 1" will be returned.
Value range: "Offset + Limit" cannot be more than 5,000. (For more information, please see Limit on the Number of Results Returned by API)
*/
@SerializedName("Offset")
@Expose
private Long Offset;
/**
* Number of entries returned by a paged query. Default value: 10. Entries from No. "Offset" to No. "Offset + Limit - 1" will be returned.
Value range: "Offset + Limit" cannot be more than 5,000. (For more information, please see Limit on the Number of Results Returned by API)
*/
@SerializedName("Limit")
@Expose
private Long Limit;
/**
* (This is not recommended. `Names`, `NamePrefixes`, or `Descriptions` should be used instead)
Search text, which fuzzily matches the media file name or description. The more matching items and the higher the match rate, the higher-ranked the result. It can contain up to 64 characters.
*/
@SerializedName("Text")
@Expose
private String Text;
/**
* (This is not recommended. `SourceTypes` should be used instead)
Media file source. For valid values, please see [SourceType](https://intl.cloud.tencent.com/document/product/266/31773?from_cn_redirect=1#MediaSourceData).
*/
@SerializedName("SourceType")
@Expose
private String SourceType;
/**
* (Not recommended. Consider using `StreamIds` instead.)
The live stream code.
*/
@SerializedName("StreamId")
@Expose
private String StreamId;
/**
* (This is not recommended. `CreateTime` should be used instead)
Start time in the creation time range.
After or at the start time.
If `CreateTime.After` also exists, it will be used first.
In ISO 8601 format. For more information, please see [ISO Date Format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I).
*/
@SerializedName("StartTime")
@Expose
private String StartTime;
/**
* (This is not recommended. `CreateTime` should be used instead)
End time in the creation time range.
Before the end time.
If `CreateTime.Before` also exists, it will be used first.
In ISO 8601 format. For more information, please see [ISO Date Format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I).
*/
@SerializedName("EndTime")
@Expose
private String EndTime;
/**
* This parameter is invalid now.
*/
@SerializedName("Vids")
@Expose
private String [] Vids;
/**
* This parameter is invalid now.
*/
@SerializedName("Vid")
@Expose
private String Vid;
/**
* Get The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
* @return SubAppId The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
*/
public Long getSubAppId() {
return this.SubAppId;
}
/**
* Set The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
* @param SubAppId The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
*/
public void setSubAppId(Long SubAppId) {
this.SubAppId = SubAppId;
}
/**
* Get File ID set. Any element in the set can be matched.
Array length limit: 10.
ID length limit: 40 characters.
* @return FileIds File ID set. Any element in the set can be matched.
Array length limit: 10.
ID length limit: 40 characters.
*/
public String [] getFileIds() {
return this.FileIds;
}
/**
* Set File ID set. Any element in the set can be matched.
Array length limit: 10.
ID length limit: 40 characters.
* @param FileIds File ID set. Any element in the set can be matched.
Array length limit: 10.
ID length limit: 40 characters.
*/
public void setFileIds(String [] FileIds) {
this.FileIds = FileIds;
}
/**
* Get The file names to use for fuzzy search, which are sorted by relevance in descending order.
Name length limit: 100 characters.
Array length limit: 10
* @return Names The file names to use for fuzzy search, which are sorted by relevance in descending order.
Name length limit: 100 characters.
Array length limit: 10
*/
public String [] getNames() {
return this.Names;
}
/**
* Set The file names to use for fuzzy search, which are sorted by relevance in descending order.
Name length limit: 100 characters.
Array length limit: 10
* @param Names The file names to use for fuzzy search, which are sorted by relevance in descending order.
Name length limit: 100 characters.
Array length limit: 10
*/
public void setNames(String [] Names) {
this.Names = Names;
}
/**
* Get The file name prefixes to search.
Prefix length limit: 100 characters.
Array length limit: 10.
* @return NamePrefixes The file name prefixes to search.
Prefix length limit: 100 characters.
Array length limit: 10.
*/
public String [] getNamePrefixes() {
return this.NamePrefixes;
}
/**
* Set The file name prefixes to search.
Prefix length limit: 100 characters.
Array length limit: 10.
* @param NamePrefixes The file name prefixes to search.
Prefix length limit: 100 characters.
Array length limit: 10.
*/
public void setNamePrefixes(String [] NamePrefixes) {
this.NamePrefixes = NamePrefixes;
}
/**
* Get File description set. Media file descriptions are fuzzily matched. The higher the match rate, the higher-ranked the result.
Length limit for a single description: 100 characters
Array length limit: 10
* @return Descriptions File description set. Media file descriptions are fuzzily matched. The higher the match rate, the higher-ranked the result.
Length limit for a single description: 100 characters
Array length limit: 10
*/
public String [] getDescriptions() {
return this.Descriptions;
}
/**
* Set File description set. Media file descriptions are fuzzily matched. The higher the match rate, the higher-ranked the result.
Length limit for a single description: 100 characters
Array length limit: 10
* @param Descriptions File description set. Media file descriptions are fuzzily matched. The higher the match rate, the higher-ranked the result.
Length limit for a single description: 100 characters
Array length limit: 10
*/
public void setDescriptions(String [] Descriptions) {
this.Descriptions = Descriptions;
}
/**
* Get Category ID set. The categories of the specified IDs and all subcategories in the set are matched.
Array length limit: 10.
* @return ClassIds Category ID set. The categories of the specified IDs and all subcategories in the set are matched.
Array length limit: 10.
*/
public Long [] getClassIds() {
return this.ClassIds;
}
/**
* Set Category ID set. The categories of the specified IDs and all subcategories in the set are matched.
Array length limit: 10.
* @param ClassIds Category ID set. The categories of the specified IDs and all subcategories in the set are matched.
Array length limit: 10.
*/
public void setClassIds(Long [] ClassIds) {
this.ClassIds = ClassIds;
}
/**
* Get The tags to search. A file is considered a match if it has any of the tags specified.
Tag length limit: 32 characters.
Array length limit: 16.
* @return Tags The tags to search. A file is considered a match if it has any of the tags specified.
Tag length limit: 32 characters.
Array length limit: 16.
*/
public String [] getTags() {
return this.Tags;
}
/**
* Set The tags to search. A file is considered a match if it has any of the tags specified.
Tag length limit: 32 characters.
Array length limit: 16.
* @param Tags The tags to search. A file is considered a match if it has any of the tags specified.
Tag length limit: 32 characters.
Array length limit: 16.
*/
public void setTags(String [] Tags) {
this.Tags = Tags;
}
/**
* Get File type. Any element in the set can be matched.
Video: video file
Audio: audio file
Image: image file
* @return Categories File type. Any element in the set can be matched.
Video: video file
Audio: audio file
Image: image file
*/
public String [] getCategories() {
return this.Categories;
}
/**
* Set File type. Any element in the set can be matched.
Video: video file
Audio: audio file
Image: image file
* @param Categories File type. Any element in the set can be matched.
Video: video file
Audio: audio file
Image: image file
*/
public void setCategories(String [] Categories) {
this.Categories = Categories;
}
/**
* Get Media file source set. For valid values, please see [SourceType](https://intl.cloud.tencent.com/document/product/266/31773?from_cn_redirect=1#MediaSourceData).
Array length limit: 10.
* @return SourceTypes Media file source set. For valid values, please see [SourceType](https://intl.cloud.tencent.com/document/product/266/31773?from_cn_redirect=1#MediaSourceData).
Array length limit: 10.
*/
public String [] getSourceTypes() {
return this.SourceTypes;
}
/**
* Set Media file source set. For valid values, please see [SourceType](https://intl.cloud.tencent.com/document/product/266/31773?from_cn_redirect=1#MediaSourceData).
Array length limit: 10.
* @param SourceTypes Media file source set. For valid values, please see [SourceType](https://intl.cloud.tencent.com/document/product/266/31773?from_cn_redirect=1#MediaSourceData).
Array length limit: 10.
*/
public void setSourceTypes(String [] SourceTypes) {
this.SourceTypes = SourceTypes;
}
/**
* Get The live stream code array. A media file will be returned if it matches any element in the array.
Array length limit: 10
* @return StreamIds The live stream code array. A media file will be returned if it matches any element in the array.
Array length limit: 10
*/
public String [] getStreamIds() {
return this.StreamIds;
}
/**
* Set The live stream code array. A media file will be returned if it matches any element in the array.
Array length limit: 10
* @param StreamIds The live stream code array. A media file will be returned if it matches any element in the array.
Array length limit: 10
*/
public void setStreamIds(String [] StreamIds) {
this.StreamIds = StreamIds;
}
/**
* Get Matches files created within the time period.
Includes specified start and end points in time.
* @return CreateTime Matches files created within the time period.
Includes specified start and end points in time.
*/
public TimeRange getCreateTime() {
return this.CreateTime;
}
/**
* Set Matches files created within the time period.
Includes specified start and end points in time.
* @param CreateTime Matches files created within the time period.
Includes specified start and end points in time.
*/
public void setCreateTime(TimeRange CreateTime) {
this.CreateTime = CreateTime;
}
/**
* Get Files whose expiration time points are within the specified time range will be returned. Expired files will not be returned.
The files whose expiration time points are on the start or end time of the specified range will also be returned.
* @return ExpireTime Files whose expiration time points are within the specified time range will be returned. Expired files will not be returned.
The files whose expiration time points are on the start or end time of the specified range will also be returned.
*/
public TimeRange getExpireTime() {
return this.ExpireTime;
}
/**
* Set Files whose expiration time points are within the specified time range will be returned. Expired files will not be returned.
The files whose expiration time points are on the start or end time of the specified range will also be returned.
* @param ExpireTime Files whose expiration time points are within the specified time range will be returned. Expired files will not be returned.
The files whose expiration time points are on the start or end time of the specified range will also be returned.
*/
public void setExpireTime(TimeRange ExpireTime) {
this.ExpireTime = ExpireTime;
}
/**
* Get Regions where media files are stored, such as `ap-chongqing`. For more regions, see [Storage Regions](https://intl.cloud.tencent.com/document/product/266/9760?from_cn_redirect=1#.E5.B7.B2.E6.94.AF.E6.8C.81.E5.9C.B0.E5.9F.9F.E5.88.97.E8.A1.A8).
Length limit for a single region: 20 characters
Array length limit: 20
* @return StorageRegions Regions where media files are stored, such as `ap-chongqing`. For more regions, see [Storage Regions](https://intl.cloud.tencent.com/document/product/266/9760?from_cn_redirect=1#.E5.B7.B2.E6.94.AF.E6.8C.81.E5.9C.B0.E5.9F.9F.E5.88.97.E8.A1.A8).
Length limit for a single region: 20 characters
Array length limit: 20
*/
public String [] getStorageRegions() {
return this.StorageRegions;
}
/**
* Set Regions where media files are stored, such as `ap-chongqing`. For more regions, see [Storage Regions](https://intl.cloud.tencent.com/document/product/266/9760?from_cn_redirect=1#.E5.B7.B2.E6.94.AF.E6.8C.81.E5.9C.B0.E5.9F.9F.E5.88.97.E8.A1.A8).
Length limit for a single region: 20 characters
Array length limit: 20
* @param StorageRegions Regions where media files are stored, such as `ap-chongqing`. For more regions, see [Storage Regions](https://intl.cloud.tencent.com/document/product/266/9760?from_cn_redirect=1#.E5.B7.B2.E6.94.AF.E6.8C.81.E5.9C.B0.E5.9F.9F.E5.88.97.E8.A1.A8).
Length limit for a single region: 20 characters
Array length limit: 20
*/
public void setStorageRegions(String [] StorageRegions) {
this.StorageRegions = StorageRegions;
}
/**
* Get An array of storage classes. Valid values:
STANDARD
STANDARD_IA
ARCHIVE
DEEP_ARCHIVE
* @return StorageClasses An array of storage classes. Valid values:
STANDARD
STANDARD_IA
ARCHIVE
DEEP_ARCHIVE
*/
public String [] getStorageClasses() {
return this.StorageClasses;
}
/**
* Set An array of storage classes. Valid values:
STANDARD
STANDARD_IA
ARCHIVE
DEEP_ARCHIVE
* @param StorageClasses An array of storage classes. Valid values:
STANDARD
STANDARD_IA
ARCHIVE
DEEP_ARCHIVE
*/
public void setStorageClasses(String [] StorageClasses) {
this.StorageClasses = StorageClasses;
}
/**
* Get The file formats.
Array length limit: 10
* @return MediaTypes The file formats.
Array length limit: 10
*/
public String [] getMediaTypes() {
return this.MediaTypes;
}
/**
* Set The file formats.
Array length limit: 10
* @param MediaTypes The file formats.
Array length limit: 10
*/
public void setMediaTypes(String [] MediaTypes) {
this.MediaTypes = MediaTypes;
}
/**
* Get The file statuses.
`Normal`
`SystemForbidden` (blocked by VOD)
`Forbidden` (blocked by you)
* @return Status The file statuses.
`Normal`
`SystemForbidden` (blocked by VOD)
`Forbidden` (blocked by you)
*/
public String [] getStatus() {
return this.Status;
}
/**
* Set The file statuses.
`Normal`
`SystemForbidden` (blocked by VOD)
`Forbidden` (blocked by you)
* @param Status The file statuses.
`Normal`
`SystemForbidden` (blocked by VOD)
`Forbidden` (blocked by you)
*/
public void setStatus(String [] Status) {
this.Status = Status;
}
/**
* Get The types of moderation result.
`pass`
`review` (the content may be non-compliant and needs to be reviewed)
`block` (the content is non-compliant and should be blocked)
`notModerated` (the file hasn't been moderated yet)
* @return ReviewResults The types of moderation result.
`pass`
`review` (the content may be non-compliant and needs to be reviewed)
`block` (the content is non-compliant and should be blocked)
`notModerated` (the file hasn't been moderated yet)
*/
public String [] getReviewResults() {
return this.ReviewResults;
}
/**
* Set The types of moderation result.
`pass`
`review` (the content may be non-compliant and needs to be reviewed)
`block` (the content is non-compliant and should be blocked)
`notModerated` (the file hasn't been moderated yet)
* @param ReviewResults The types of moderation result.
`pass`
`review` (the content may be non-compliant and needs to be reviewed)
`block` (the content is non-compliant and should be blocked)
`notModerated` (the file hasn't been moderated yet)
*/
public void setReviewResults(String [] ReviewResults) {
this.ReviewResults = ReviewResults;
}
/**
* Get The TRTC application IDs. Any file that matches one of the application IDs will be returned.
Array length limit: 10
* @return TrtcSdkAppIds The TRTC application IDs. Any file that matches one of the application IDs will be returned.
Array length limit: 10
*/
public Long [] getTrtcSdkAppIds() {
return this.TrtcSdkAppIds;
}
/**
* Set The TRTC application IDs. Any file that matches one of the application IDs will be returned.
Array length limit: 10
* @param TrtcSdkAppIds The TRTC application IDs. Any file that matches one of the application IDs will be returned.
Array length limit: 10
*/
public void setTrtcSdkAppIds(Long [] TrtcSdkAppIds) {
this.TrtcSdkAppIds = TrtcSdkAppIds;
}
/**
* Get The TRTC room IDs. Any file that matches one of the room IDs will be returned.
Element length limit: 64 characters.
Array length limit: 10.
* @return TrtcRoomIds The TRTC room IDs. Any file that matches one of the room IDs will be returned.
Element length limit: 64 characters.
Array length limit: 10.
*/
public String [] getTrtcRoomIds() {
return this.TrtcRoomIds;
}
/**
* Set The TRTC room IDs. Any file that matches one of the room IDs will be returned.
Element length limit: 64 characters.
Array length limit: 10.
* @param TrtcRoomIds The TRTC room IDs. Any file that matches one of the room IDs will be returned.
Element length limit: 64 characters.
Array length limit: 10.
*/
public void setTrtcRoomIds(String [] TrtcRoomIds) {
this.TrtcRoomIds = TrtcRoomIds;
}
/**
* Get Specifies information entry that needs to be returned for all media files. Multiple entries can be specified simultaneously. N starts from 0. If this field is left empty, all information entries will be returned by default. Valid values:
basicInfo (basic video information).
metaData (video metadata).
transcodeInfo (result information of video transcoding).
animatedGraphicsInfo (result information of animated image generating task).
imageSpriteInfo (image sprite information).
snapshotByTimeOffsetInfo (point-in-time screenshot information).
sampleSnapshotInfo (sampled screenshot information).
keyFrameDescInfo (timestamp information).
adaptiveDynamicStreamingInfo (information of adaptive bitrate streaming).
miniProgramReviewInfo (WeChat Mini Program audit information).
* @return Filters Specifies information entry that needs to be returned for all media files. Multiple entries can be specified simultaneously. N starts from 0. If this field is left empty, all information entries will be returned by default. Valid values:
basicInfo (basic video information).
metaData (video metadata).
transcodeInfo (result information of video transcoding).
animatedGraphicsInfo (result information of animated image generating task).
imageSpriteInfo (image sprite information).
snapshotByTimeOffsetInfo (point-in-time screenshot information).
sampleSnapshotInfo (sampled screenshot information).
keyFrameDescInfo (timestamp information).
adaptiveDynamicStreamingInfo (information of adaptive bitrate streaming).
miniProgramReviewInfo (WeChat Mini Program audit information).
*/
public String [] getFilters() {
return this.Filters;
}
/**
* Set Specifies information entry that needs to be returned for all media files. Multiple entries can be specified simultaneously. N starts from 0. If this field is left empty, all information entries will be returned by default. Valid values:
basicInfo (basic video information).
metaData (video metadata).
transcodeInfo (result information of video transcoding).
animatedGraphicsInfo (result information of animated image generating task).
imageSpriteInfo (image sprite information).
snapshotByTimeOffsetInfo (point-in-time screenshot information).
sampleSnapshotInfo (sampled screenshot information).
keyFrameDescInfo (timestamp information).
adaptiveDynamicStreamingInfo (information of adaptive bitrate streaming).
miniProgramReviewInfo (WeChat Mini Program audit information).
* @param Filters Specifies information entry that needs to be returned for all media files. Multiple entries can be specified simultaneously. N starts from 0. If this field is left empty, all information entries will be returned by default. Valid values:
basicInfo (basic video information).
metaData (video metadata).
transcodeInfo (result information of video transcoding).
animatedGraphicsInfo (result information of animated image generating task).
imageSpriteInfo (image sprite information).
snapshotByTimeOffsetInfo (point-in-time screenshot information).
sampleSnapshotInfo (sampled screenshot information).
keyFrameDescInfo (timestamp information).
adaptiveDynamicStreamingInfo (information of adaptive bitrate streaming).
miniProgramReviewInfo (WeChat Mini Program audit information).
*/
public void setFilters(String [] Filters) {
this.Filters = Filters;
}
/**
* Get Sorting order.
Valid value of `Sort.Field`: CreateTime.
If `Text`, `Names`, or `Descriptions` is not empty, the `Sort.Field` field will not take effect, and the search results will be sorted by match rate.
* @return Sort Sorting order.
Valid value of `Sort.Field`: CreateTime.
If `Text`, `Names`, or `Descriptions` is not empty, the `Sort.Field` field will not take effect, and the search results will be sorted by match rate.
*/
public SortBy getSort() {
return this.Sort;
}
/**
* Set Sorting order.
Valid value of `Sort.Field`: CreateTime.
If `Text`, `Names`, or `Descriptions` is not empty, the `Sort.Field` field will not take effect, and the search results will be sorted by match rate.
* @param Sort Sorting order.
Valid value of `Sort.Field`: CreateTime.
If `Text`, `Names`, or `Descriptions` is not empty, the `Sort.Field` field will not take effect, and the search results will be sorted by match rate.
*/
public void setSort(SortBy Sort) {
this.Sort = Sort;
}
/**
* Get Start offset of a paged return. Default value: 0. Entries from No. "Offset" to No. "Offset + Limit - 1" will be returned.
Value range: "Offset + Limit" cannot be more than 5,000. (For more information, please see Limit on the Number of Results Returned by API)
* @return Offset Start offset of a paged return. Default value: 0. Entries from No. "Offset" to No. "Offset + Limit - 1" will be returned.
Value range: "Offset + Limit" cannot be more than 5,000. (For more information, please see Limit on the Number of Results Returned by API)
*/
public Long getOffset() {
return this.Offset;
}
/**
* Set Start offset of a paged return. Default value: 0. Entries from No. "Offset" to No. "Offset + Limit - 1" will be returned.
Value range: "Offset + Limit" cannot be more than 5,000. (For more information, please see Limit on the Number of Results Returned by API)
* @param Offset Start offset of a paged return. Default value: 0. Entries from No. "Offset" to No. "Offset + Limit - 1" will be returned.
Value range: "Offset + Limit" cannot be more than 5,000. (For more information, please see Limit on the Number of Results Returned by API)
*/
public void setOffset(Long Offset) {
this.Offset = Offset;
}
/**
* Get Number of entries returned by a paged query. Default value: 10. Entries from No. "Offset" to No. "Offset + Limit - 1" will be returned.
Value range: "Offset + Limit" cannot be more than 5,000. (For more information, please see Limit on the Number of Results Returned by API)
* @return Limit Number of entries returned by a paged query. Default value: 10. Entries from No. "Offset" to No. "Offset + Limit - 1" will be returned.
Value range: "Offset + Limit" cannot be more than 5,000. (For more information, please see Limit on the Number of Results Returned by API)
*/
public Long getLimit() {
return this.Limit;
}
/**
* Set Number of entries returned by a paged query. Default value: 10. Entries from No. "Offset" to No. "Offset + Limit - 1" will be returned.
Value range: "Offset + Limit" cannot be more than 5,000. (For more information, please see Limit on the Number of Results Returned by API)
* @param Limit Number of entries returned by a paged query. Default value: 10. Entries from No. "Offset" to No. "Offset + Limit - 1" will be returned.
Value range: "Offset + Limit" cannot be more than 5,000. (For more information, please see Limit on the Number of Results Returned by API)
*/
public void setLimit(Long Limit) {
this.Limit = Limit;
}
/**
* Get (This is not recommended. `Names`, `NamePrefixes`, or `Descriptions` should be used instead)
Search text, which fuzzily matches the media file name or description. The more matching items and the higher the match rate, the higher-ranked the result. It can contain up to 64 characters.
* @return Text (This is not recommended. `Names`, `NamePrefixes`, or `Descriptions` should be used instead)
Search text, which fuzzily matches the media file name or description. The more matching items and the higher the match rate, the higher-ranked the result. It can contain up to 64 characters.
*/
public String getText() {
return this.Text;
}
/**
* Set (This is not recommended. `Names`, `NamePrefixes`, or `Descriptions` should be used instead)
Search text, which fuzzily matches the media file name or description. The more matching items and the higher the match rate, the higher-ranked the result. It can contain up to 64 characters.
* @param Text (This is not recommended. `Names`, `NamePrefixes`, or `Descriptions` should be used instead)
Search text, which fuzzily matches the media file name or description. The more matching items and the higher the match rate, the higher-ranked the result. It can contain up to 64 characters.
*/
public void setText(String Text) {
this.Text = Text;
}
/**
* Get (This is not recommended. `SourceTypes` should be used instead)
Media file source. For valid values, please see [SourceType](https://intl.cloud.tencent.com/document/product/266/31773?from_cn_redirect=1#MediaSourceData).
* @return SourceType (This is not recommended. `SourceTypes` should be used instead)
Media file source. For valid values, please see [SourceType](https://intl.cloud.tencent.com/document/product/266/31773?from_cn_redirect=1#MediaSourceData).
*/
public String getSourceType() {
return this.SourceType;
}
/**
* Set (This is not recommended. `SourceTypes` should be used instead)
Media file source. For valid values, please see [SourceType](https://intl.cloud.tencent.com/document/product/266/31773?from_cn_redirect=1#MediaSourceData).
* @param SourceType (This is not recommended. `SourceTypes` should be used instead)
Media file source. For valid values, please see [SourceType](https://intl.cloud.tencent.com/document/product/266/31773?from_cn_redirect=1#MediaSourceData).
*/
public void setSourceType(String SourceType) {
this.SourceType = SourceType;
}
/**
* Get (Not recommended. Consider using `StreamIds` instead.)
The live stream code.
* @return StreamId (Not recommended. Consider using `StreamIds` instead.)
The live stream code.
*/
public String getStreamId() {
return this.StreamId;
}
/**
* Set (Not recommended. Consider using `StreamIds` instead.)
The live stream code.
* @param StreamId (Not recommended. Consider using `StreamIds` instead.)
The live stream code.
*/
public void setStreamId(String StreamId) {
this.StreamId = StreamId;
}
/**
* Get (This is not recommended. `CreateTime` should be used instead)
Start time in the creation time range.
After or at the start time.
If `CreateTime.After` also exists, it will be used first.
In ISO 8601 format. For more information, please see [ISO Date Format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I).
* @return StartTime (This is not recommended. `CreateTime` should be used instead)
Start time in the creation time range.
After or at the start time.
If `CreateTime.After` also exists, it will be used first.
In ISO 8601 format. For more information, please see [ISO Date Format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I).
*/
public String getStartTime() {
return this.StartTime;
}
/**
* Set (This is not recommended. `CreateTime` should be used instead)
Start time in the creation time range.
After or at the start time.
If `CreateTime.After` also exists, it will be used first.
In ISO 8601 format. For more information, please see [ISO Date Format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I).
* @param StartTime (This is not recommended. `CreateTime` should be used instead)
Start time in the creation time range.
After or at the start time.
If `CreateTime.After` also exists, it will be used first.
In ISO 8601 format. For more information, please see [ISO Date Format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I).
*/
public void setStartTime(String StartTime) {
this.StartTime = StartTime;
}
/**
* Get (This is not recommended. `CreateTime` should be used instead)
End time in the creation time range.
Before the end time.
If `CreateTime.Before` also exists, it will be used first.
In ISO 8601 format. For more information, please see [ISO Date Format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I).
* @return EndTime (This is not recommended. `CreateTime` should be used instead)
End time in the creation time range.
Before the end time.
If `CreateTime.Before` also exists, it will be used first.
In ISO 8601 format. For more information, please see [ISO Date Format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I).
*/
public String getEndTime() {
return this.EndTime;
}
/**
* Set (This is not recommended. `CreateTime` should be used instead)
End time in the creation time range.
Before the end time.
If `CreateTime.Before` also exists, it will be used first.
In ISO 8601 format. For more information, please see [ISO Date Format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I).
* @param EndTime (This is not recommended. `CreateTime` should be used instead)
End time in the creation time range.
Before the end time.
If `CreateTime.Before` also exists, it will be used first.
In ISO 8601 format. For more information, please see [ISO Date Format](https://intl.cloud.tencent.com/document/product/266/11732?from_cn_redirect=1#I).
*/
public void setEndTime(String EndTime) {
this.EndTime = EndTime;
}
/**
* Get This parameter is invalid now.
* @return Vids This parameter is invalid now.
*/
public String [] getVids() {
return this.Vids;
}
/**
* Set This parameter is invalid now.
* @param Vids This parameter is invalid now.
*/
public void setVids(String [] Vids) {
this.Vids = Vids;
}
/**
* Get This parameter is invalid now.
* @return Vid This parameter is invalid now.
*/
public String getVid() {
return this.Vid;
}
/**
* Set This parameter is invalid now.
* @param Vid This parameter is invalid now.
*/
public void setVid(String Vid) {
this.Vid = Vid;
}
public SearchMediaRequest() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public SearchMediaRequest(SearchMediaRequest source) {
if (source.SubAppId != null) {
this.SubAppId = new Long(source.SubAppId);
}
if (source.FileIds != null) {
this.FileIds = new String[source.FileIds.length];
for (int i = 0; i < source.FileIds.length; i++) {
this.FileIds[i] = new String(source.FileIds[i]);
}
}
if (source.Names != null) {
this.Names = new String[source.Names.length];
for (int i = 0; i < source.Names.length; i++) {
this.Names[i] = new String(source.Names[i]);
}
}
if (source.NamePrefixes != null) {
this.NamePrefixes = new String[source.NamePrefixes.length];
for (int i = 0; i < source.NamePrefixes.length; i++) {
this.NamePrefixes[i] = new String(source.NamePrefixes[i]);
}
}
if (source.Descriptions != null) {
this.Descriptions = new String[source.Descriptions.length];
for (int i = 0; i < source.Descriptions.length; i++) {
this.Descriptions[i] = new String(source.Descriptions[i]);
}
}
if (source.ClassIds != null) {
this.ClassIds = new Long[source.ClassIds.length];
for (int i = 0; i < source.ClassIds.length; i++) {
this.ClassIds[i] = new Long(source.ClassIds[i]);
}
}
if (source.Tags != null) {
this.Tags = new String[source.Tags.length];
for (int i = 0; i < source.Tags.length; i++) {
this.Tags[i] = new String(source.Tags[i]);
}
}
if (source.Categories != null) {
this.Categories = new String[source.Categories.length];
for (int i = 0; i < source.Categories.length; i++) {
this.Categories[i] = new String(source.Categories[i]);
}
}
if (source.SourceTypes != null) {
this.SourceTypes = new String[source.SourceTypes.length];
for (int i = 0; i < source.SourceTypes.length; i++) {
this.SourceTypes[i] = new String(source.SourceTypes[i]);
}
}
if (source.StreamIds != null) {
this.StreamIds = new String[source.StreamIds.length];
for (int i = 0; i < source.StreamIds.length; i++) {
this.StreamIds[i] = new String(source.StreamIds[i]);
}
}
if (source.CreateTime != null) {
this.CreateTime = new TimeRange(source.CreateTime);
}
if (source.ExpireTime != null) {
this.ExpireTime = new TimeRange(source.ExpireTime);
}
if (source.StorageRegions != null) {
this.StorageRegions = new String[source.StorageRegions.length];
for (int i = 0; i < source.StorageRegions.length; i++) {
this.StorageRegions[i] = new String(source.StorageRegions[i]);
}
}
if (source.StorageClasses != null) {
this.StorageClasses = new String[source.StorageClasses.length];
for (int i = 0; i < source.StorageClasses.length; i++) {
this.StorageClasses[i] = new String(source.StorageClasses[i]);
}
}
if (source.MediaTypes != null) {
this.MediaTypes = new String[source.MediaTypes.length];
for (int i = 0; i < source.MediaTypes.length; i++) {
this.MediaTypes[i] = new String(source.MediaTypes[i]);
}
}
if (source.Status != null) {
this.Status = new String[source.Status.length];
for (int i = 0; i < source.Status.length; i++) {
this.Status[i] = new String(source.Status[i]);
}
}
if (source.ReviewResults != null) {
this.ReviewResults = new String[source.ReviewResults.length];
for (int i = 0; i < source.ReviewResults.length; i++) {
this.ReviewResults[i] = new String(source.ReviewResults[i]);
}
}
if (source.TrtcSdkAppIds != null) {
this.TrtcSdkAppIds = new Long[source.TrtcSdkAppIds.length];
for (int i = 0; i < source.TrtcSdkAppIds.length; i++) {
this.TrtcSdkAppIds[i] = new Long(source.TrtcSdkAppIds[i]);
}
}
if (source.TrtcRoomIds != null) {
this.TrtcRoomIds = new String[source.TrtcRoomIds.length];
for (int i = 0; i < source.TrtcRoomIds.length; i++) {
this.TrtcRoomIds[i] = new String(source.TrtcRoomIds[i]);
}
}
if (source.Filters != null) {
this.Filters = new String[source.Filters.length];
for (int i = 0; i < source.Filters.length; i++) {
this.Filters[i] = new String(source.Filters[i]);
}
}
if (source.Sort != null) {
this.Sort = new SortBy(source.Sort);
}
if (source.Offset != null) {
this.Offset = new Long(source.Offset);
}
if (source.Limit != null) {
this.Limit = new Long(source.Limit);
}
if (source.Text != null) {
this.Text = new String(source.Text);
}
if (source.SourceType != null) {
this.SourceType = new String(source.SourceType);
}
if (source.StreamId != null) {
this.StreamId = new String(source.StreamId);
}
if (source.StartTime != null) {
this.StartTime = new String(source.StartTime);
}
if (source.EndTime != null) {
this.EndTime = new String(source.EndTime);
}
if (source.Vids != null) {
this.Vids = new String[source.Vids.length];
for (int i = 0; i < source.Vids.length; i++) {
this.Vids[i] = new String(source.Vids[i]);
}
}
if (source.Vid != null) {
this.Vid = new String(source.Vid);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "SubAppId", this.SubAppId);
this.setParamArraySimple(map, prefix + "FileIds.", this.FileIds);
this.setParamArraySimple(map, prefix + "Names.", this.Names);
this.setParamArraySimple(map, prefix + "NamePrefixes.", this.NamePrefixes);
this.setParamArraySimple(map, prefix + "Descriptions.", this.Descriptions);
this.setParamArraySimple(map, prefix + "ClassIds.", this.ClassIds);
this.setParamArraySimple(map, prefix + "Tags.", this.Tags);
this.setParamArraySimple(map, prefix + "Categories.", this.Categories);
this.setParamArraySimple(map, prefix + "SourceTypes.", this.SourceTypes);
this.setParamArraySimple(map, prefix + "StreamIds.", this.StreamIds);
this.setParamObj(map, prefix + "CreateTime.", this.CreateTime);
this.setParamObj(map, prefix + "ExpireTime.", this.ExpireTime);
this.setParamArraySimple(map, prefix + "StorageRegions.", this.StorageRegions);
this.setParamArraySimple(map, prefix + "StorageClasses.", this.StorageClasses);
this.setParamArraySimple(map, prefix + "MediaTypes.", this.MediaTypes);
this.setParamArraySimple(map, prefix + "Status.", this.Status);
this.setParamArraySimple(map, prefix + "ReviewResults.", this.ReviewResults);
this.setParamArraySimple(map, prefix + "TrtcSdkAppIds.", this.TrtcSdkAppIds);
this.setParamArraySimple(map, prefix + "TrtcRoomIds.", this.TrtcRoomIds);
this.setParamArraySimple(map, prefix + "Filters.", this.Filters);
this.setParamObj(map, prefix + "Sort.", this.Sort);
this.setParamSimple(map, prefix + "Offset", this.Offset);
this.setParamSimple(map, prefix + "Limit", this.Limit);
this.setParamSimple(map, prefix + "Text", this.Text);
this.setParamSimple(map, prefix + "SourceType", this.SourceType);
this.setParamSimple(map, prefix + "StreamId", this.StreamId);
this.setParamSimple(map, prefix + "StartTime", this.StartTime);
this.setParamSimple(map, prefix + "EndTime", this.EndTime);
this.setParamArraySimple(map, prefix + "Vids.", this.Vids);
this.setParamSimple(map, prefix + "Vid", this.Vid);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy