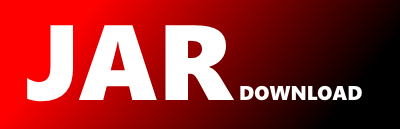
com.tencentcloudapi.antiddos.v20200309.models.IPAlarmThresholdRelation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.antiddos.v20200309.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class IPAlarmThresholdRelation extends AbstractModel {
/**
* Alarm threshold type. Valid values:
`1`: alarm threshold for inbound traffic
`2`: alarm threshold for cleansing attack traffic
]
*/
@SerializedName("AlarmType")
@Expose
private Long AlarmType;
/**
* Alarm threshold (Mbps). The value should be greater than or equal to 0. Note that the alarm threshold configuration will be removed if you pass the parameter for input and set it to 0.
*/
@SerializedName("AlarmThreshold")
@Expose
private Long AlarmThreshold;
/**
* Anti-DDoS instance configured
*/
@SerializedName("InstanceDetailList")
@Expose
private InstanceRelation [] InstanceDetailList;
/**
* Get Alarm threshold type. Valid values:
`1`: alarm threshold for inbound traffic
`2`: alarm threshold for cleansing attack traffic
]
* @return AlarmType Alarm threshold type. Valid values:
`1`: alarm threshold for inbound traffic
`2`: alarm threshold for cleansing attack traffic
]
*/
public Long getAlarmType() {
return this.AlarmType;
}
/**
* Set Alarm threshold type. Valid values:
`1`: alarm threshold for inbound traffic
`2`: alarm threshold for cleansing attack traffic
]
* @param AlarmType Alarm threshold type. Valid values:
`1`: alarm threshold for inbound traffic
`2`: alarm threshold for cleansing attack traffic
]
*/
public void setAlarmType(Long AlarmType) {
this.AlarmType = AlarmType;
}
/**
* Get Alarm threshold (Mbps). The value should be greater than or equal to 0. Note that the alarm threshold configuration will be removed if you pass the parameter for input and set it to 0.
* @return AlarmThreshold Alarm threshold (Mbps). The value should be greater than or equal to 0. Note that the alarm threshold configuration will be removed if you pass the parameter for input and set it to 0.
*/
public Long getAlarmThreshold() {
return this.AlarmThreshold;
}
/**
* Set Alarm threshold (Mbps). The value should be greater than or equal to 0. Note that the alarm threshold configuration will be removed if you pass the parameter for input and set it to 0.
* @param AlarmThreshold Alarm threshold (Mbps). The value should be greater than or equal to 0. Note that the alarm threshold configuration will be removed if you pass the parameter for input and set it to 0.
*/
public void setAlarmThreshold(Long AlarmThreshold) {
this.AlarmThreshold = AlarmThreshold;
}
/**
* Get Anti-DDoS instance configured
* @return InstanceDetailList Anti-DDoS instance configured
*/
public InstanceRelation [] getInstanceDetailList() {
return this.InstanceDetailList;
}
/**
* Set Anti-DDoS instance configured
* @param InstanceDetailList Anti-DDoS instance configured
*/
public void setInstanceDetailList(InstanceRelation [] InstanceDetailList) {
this.InstanceDetailList = InstanceDetailList;
}
public IPAlarmThresholdRelation() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public IPAlarmThresholdRelation(IPAlarmThresholdRelation source) {
if (source.AlarmType != null) {
this.AlarmType = new Long(source.AlarmType);
}
if (source.AlarmThreshold != null) {
this.AlarmThreshold = new Long(source.AlarmThreshold);
}
if (source.InstanceDetailList != null) {
this.InstanceDetailList = new InstanceRelation[source.InstanceDetailList.length];
for (int i = 0; i < source.InstanceDetailList.length; i++) {
this.InstanceDetailList[i] = new InstanceRelation(source.InstanceDetailList[i]);
}
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "AlarmType", this.AlarmType);
this.setParamSimple(map, prefix + "AlarmThreshold", this.AlarmThreshold);
this.setParamArrayObj(map, prefix + "InstanceDetailList.", this.InstanceDetailList);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy