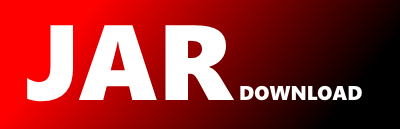
com.tencentcloudapi.cvm.v20170312.models.InternetAccessible Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.cvm.v20170312.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class InternetAccessible extends AbstractModel {
/**
* Network connection billing plan. Valid value:
TRAFFIC_POSTPAID_BY_HOUR: pay after use. You are billed for your traffic, by the hour.
*/
@SerializedName("InternetChargeType")
@Expose
private String InternetChargeType;
/**
* The maximum outbound bandwidth of the public network, in Mbps. The default value is 0 Mbps. The upper limit of bandwidth varies for different models. For more information, see [Purchase Network Bandwidth](https://intl.cloud.tencent.com/document/product/213/12523?from_cn_redirect=1).
*/
@SerializedName("InternetMaxBandwidthOut")
@Expose
private Long InternetMaxBandwidthOut;
/**
* Whether to allocate a public IP address. Valid values:
true: Allocate a public IP address. false: Do not allocate a public IP address.
When the public network bandwidth is greater than 0 Mbps, you can choose whether to enable the public IP address. The public IP address is enabled by default. When the public network bandwidth is 0, allocating the public IP address is not supported. This parameter is only used as an input parameter in the RunInstances API.
*/
@SerializedName("PublicIpAssigned")
@Expose
private Boolean PublicIpAssigned;
/**
* Bandwidth package ID. To obatin the IDs, you can call [`DescribeBandwidthPackages`](https://intl.cloud.tencent.com/document/api/215/19209?from_cn_redirect=1) and look for the `BandwidthPackageId` fields in the response.
*/
@SerializedName("BandwidthPackageId")
@Expose
private String BandwidthPackageId;
/**
* Get Network connection billing plan. Valid value:
TRAFFIC_POSTPAID_BY_HOUR: pay after use. You are billed for your traffic, by the hour.
* @return InternetChargeType Network connection billing plan. Valid value:
TRAFFIC_POSTPAID_BY_HOUR: pay after use. You are billed for your traffic, by the hour.
*/
public String getInternetChargeType() {
return this.InternetChargeType;
}
/**
* Set Network connection billing plan. Valid value:
TRAFFIC_POSTPAID_BY_HOUR: pay after use. You are billed for your traffic, by the hour.
* @param InternetChargeType Network connection billing plan. Valid value:
TRAFFIC_POSTPAID_BY_HOUR: pay after use. You are billed for your traffic, by the hour.
*/
public void setInternetChargeType(String InternetChargeType) {
this.InternetChargeType = InternetChargeType;
}
/**
* Get The maximum outbound bandwidth of the public network, in Mbps. The default value is 0 Mbps. The upper limit of bandwidth varies for different models. For more information, see [Purchase Network Bandwidth](https://intl.cloud.tencent.com/document/product/213/12523?from_cn_redirect=1).
* @return InternetMaxBandwidthOut The maximum outbound bandwidth of the public network, in Mbps. The default value is 0 Mbps. The upper limit of bandwidth varies for different models. For more information, see [Purchase Network Bandwidth](https://intl.cloud.tencent.com/document/product/213/12523?from_cn_redirect=1).
*/
public Long getInternetMaxBandwidthOut() {
return this.InternetMaxBandwidthOut;
}
/**
* Set The maximum outbound bandwidth of the public network, in Mbps. The default value is 0 Mbps. The upper limit of bandwidth varies for different models. For more information, see [Purchase Network Bandwidth](https://intl.cloud.tencent.com/document/product/213/12523?from_cn_redirect=1).
* @param InternetMaxBandwidthOut The maximum outbound bandwidth of the public network, in Mbps. The default value is 0 Mbps. The upper limit of bandwidth varies for different models. For more information, see [Purchase Network Bandwidth](https://intl.cloud.tencent.com/document/product/213/12523?from_cn_redirect=1).
*/
public void setInternetMaxBandwidthOut(Long InternetMaxBandwidthOut) {
this.InternetMaxBandwidthOut = InternetMaxBandwidthOut;
}
/**
* Get Whether to allocate a public IP address. Valid values:
true: Allocate a public IP address. false: Do not allocate a public IP address.
When the public network bandwidth is greater than 0 Mbps, you can choose whether to enable the public IP address. The public IP address is enabled by default. When the public network bandwidth is 0, allocating the public IP address is not supported. This parameter is only used as an input parameter in the RunInstances API.
* @return PublicIpAssigned Whether to allocate a public IP address. Valid values:
true: Allocate a public IP address. false: Do not allocate a public IP address.
When the public network bandwidth is greater than 0 Mbps, you can choose whether to enable the public IP address. The public IP address is enabled by default. When the public network bandwidth is 0, allocating the public IP address is not supported. This parameter is only used as an input parameter in the RunInstances API.
*/
public Boolean getPublicIpAssigned() {
return this.PublicIpAssigned;
}
/**
* Set Whether to allocate a public IP address. Valid values:
true: Allocate a public IP address. false: Do not allocate a public IP address.
When the public network bandwidth is greater than 0 Mbps, you can choose whether to enable the public IP address. The public IP address is enabled by default. When the public network bandwidth is 0, allocating the public IP address is not supported. This parameter is only used as an input parameter in the RunInstances API.
* @param PublicIpAssigned Whether to allocate a public IP address. Valid values:
true: Allocate a public IP address. false: Do not allocate a public IP address.
When the public network bandwidth is greater than 0 Mbps, you can choose whether to enable the public IP address. The public IP address is enabled by default. When the public network bandwidth is 0, allocating the public IP address is not supported. This parameter is only used as an input parameter in the RunInstances API.
*/
public void setPublicIpAssigned(Boolean PublicIpAssigned) {
this.PublicIpAssigned = PublicIpAssigned;
}
/**
* Get Bandwidth package ID. To obatin the IDs, you can call [`DescribeBandwidthPackages`](https://intl.cloud.tencent.com/document/api/215/19209?from_cn_redirect=1) and look for the `BandwidthPackageId` fields in the response.
* @return BandwidthPackageId Bandwidth package ID. To obatin the IDs, you can call [`DescribeBandwidthPackages`](https://intl.cloud.tencent.com/document/api/215/19209?from_cn_redirect=1) and look for the `BandwidthPackageId` fields in the response.
*/
public String getBandwidthPackageId() {
return this.BandwidthPackageId;
}
/**
* Set Bandwidth package ID. To obatin the IDs, you can call [`DescribeBandwidthPackages`](https://intl.cloud.tencent.com/document/api/215/19209?from_cn_redirect=1) and look for the `BandwidthPackageId` fields in the response.
* @param BandwidthPackageId Bandwidth package ID. To obatin the IDs, you can call [`DescribeBandwidthPackages`](https://intl.cloud.tencent.com/document/api/215/19209?from_cn_redirect=1) and look for the `BandwidthPackageId` fields in the response.
*/
public void setBandwidthPackageId(String BandwidthPackageId) {
this.BandwidthPackageId = BandwidthPackageId;
}
public InternetAccessible() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public InternetAccessible(InternetAccessible source) {
if (source.InternetChargeType != null) {
this.InternetChargeType = new String(source.InternetChargeType);
}
if (source.InternetMaxBandwidthOut != null) {
this.InternetMaxBandwidthOut = new Long(source.InternetMaxBandwidthOut);
}
if (source.PublicIpAssigned != null) {
this.PublicIpAssigned = new Boolean(source.PublicIpAssigned);
}
if (source.BandwidthPackageId != null) {
this.BandwidthPackageId = new String(source.BandwidthPackageId);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "InternetChargeType", this.InternetChargeType);
this.setParamSimple(map, prefix + "InternetMaxBandwidthOut", this.InternetMaxBandwidthOut);
this.setParamSimple(map, prefix + "PublicIpAssigned", this.PublicIpAssigned);
this.setParamSimple(map, prefix + "BandwidthPackageId", this.BandwidthPackageId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy