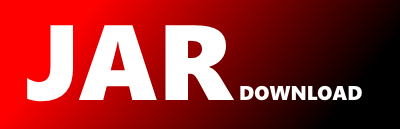
com.tencentcloudapi.cwp.v20180228.models.CreateScanMalwareSettingRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.cwp.v20180228.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class CreateScanMalwareSettingRequest extends AbstractModel {
/**
* Scan mode: 0 - full disk scan; 1 - rapid scan
*/
@SerializedName("ScanPattern")
@Expose
private Long ScanPattern;
/**
* Server classification: 1: Professional Edition servers; 2: self-selected servers
*/
@SerializedName("HostType")
@Expose
private Long HostType;
/**
* Effective for selected servers; a string array of host QUUIDs
*/
@SerializedName("QuuidList")
@Expose
private String [] QuuidList;
/**
* Timeout unit: seconds, which is 3,600 seconds by default.
*/
@SerializedName("TimeoutPeriod")
@Expose
private Long TimeoutPeriod;
/**
* 1 - standard mode (only critical and high-risk are reported.); 2 - enhanced mode (critical, high-risk, and medium-risk are reported.); 3 - strict mode (critical, high-risk, medium-risk, low-risk, and prompt are reported.)
*/
@SerializedName("EngineType")
@Expose
private Long EngineType;
/**
* Whether to enable malicious process killing [0: Not Enabled; 1: Enabled]
*/
@SerializedName("EnableMemShellScan")
@Expose
private Long EnableMemShellScan;
/**
* Get Scan mode: 0 - full disk scan; 1 - rapid scan
* @return ScanPattern Scan mode: 0 - full disk scan; 1 - rapid scan
*/
public Long getScanPattern() {
return this.ScanPattern;
}
/**
* Set Scan mode: 0 - full disk scan; 1 - rapid scan
* @param ScanPattern Scan mode: 0 - full disk scan; 1 - rapid scan
*/
public void setScanPattern(Long ScanPattern) {
this.ScanPattern = ScanPattern;
}
/**
* Get Server classification: 1: Professional Edition servers; 2: self-selected servers
* @return HostType Server classification: 1: Professional Edition servers; 2: self-selected servers
*/
public Long getHostType() {
return this.HostType;
}
/**
* Set Server classification: 1: Professional Edition servers; 2: self-selected servers
* @param HostType Server classification: 1: Professional Edition servers; 2: self-selected servers
*/
public void setHostType(Long HostType) {
this.HostType = HostType;
}
/**
* Get Effective for selected servers; a string array of host QUUIDs
* @return QuuidList Effective for selected servers; a string array of host QUUIDs
*/
public String [] getQuuidList() {
return this.QuuidList;
}
/**
* Set Effective for selected servers; a string array of host QUUIDs
* @param QuuidList Effective for selected servers; a string array of host QUUIDs
*/
public void setQuuidList(String [] QuuidList) {
this.QuuidList = QuuidList;
}
/**
* Get Timeout unit: seconds, which is 3,600 seconds by default.
* @return TimeoutPeriod Timeout unit: seconds, which is 3,600 seconds by default.
*/
public Long getTimeoutPeriod() {
return this.TimeoutPeriod;
}
/**
* Set Timeout unit: seconds, which is 3,600 seconds by default.
* @param TimeoutPeriod Timeout unit: seconds, which is 3,600 seconds by default.
*/
public void setTimeoutPeriod(Long TimeoutPeriod) {
this.TimeoutPeriod = TimeoutPeriod;
}
/**
* Get 1 - standard mode (only critical and high-risk are reported.); 2 - enhanced mode (critical, high-risk, and medium-risk are reported.); 3 - strict mode (critical, high-risk, medium-risk, low-risk, and prompt are reported.)
* @return EngineType 1 - standard mode (only critical and high-risk are reported.); 2 - enhanced mode (critical, high-risk, and medium-risk are reported.); 3 - strict mode (critical, high-risk, medium-risk, low-risk, and prompt are reported.)
*/
public Long getEngineType() {
return this.EngineType;
}
/**
* Set 1 - standard mode (only critical and high-risk are reported.); 2 - enhanced mode (critical, high-risk, and medium-risk are reported.); 3 - strict mode (critical, high-risk, medium-risk, low-risk, and prompt are reported.)
* @param EngineType 1 - standard mode (only critical and high-risk are reported.); 2 - enhanced mode (critical, high-risk, and medium-risk are reported.); 3 - strict mode (critical, high-risk, medium-risk, low-risk, and prompt are reported.)
*/
public void setEngineType(Long EngineType) {
this.EngineType = EngineType;
}
/**
* Get Whether to enable malicious process killing [0: Not Enabled; 1: Enabled]
* @return EnableMemShellScan Whether to enable malicious process killing [0: Not Enabled; 1: Enabled]
*/
public Long getEnableMemShellScan() {
return this.EnableMemShellScan;
}
/**
* Set Whether to enable malicious process killing [0: Not Enabled; 1: Enabled]
* @param EnableMemShellScan Whether to enable malicious process killing [0: Not Enabled; 1: Enabled]
*/
public void setEnableMemShellScan(Long EnableMemShellScan) {
this.EnableMemShellScan = EnableMemShellScan;
}
public CreateScanMalwareSettingRequest() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public CreateScanMalwareSettingRequest(CreateScanMalwareSettingRequest source) {
if (source.ScanPattern != null) {
this.ScanPattern = new Long(source.ScanPattern);
}
if (source.HostType != null) {
this.HostType = new Long(source.HostType);
}
if (source.QuuidList != null) {
this.QuuidList = new String[source.QuuidList.length];
for (int i = 0; i < source.QuuidList.length; i++) {
this.QuuidList[i] = new String(source.QuuidList[i]);
}
}
if (source.TimeoutPeriod != null) {
this.TimeoutPeriod = new Long(source.TimeoutPeriod);
}
if (source.EngineType != null) {
this.EngineType = new Long(source.EngineType);
}
if (source.EnableMemShellScan != null) {
this.EnableMemShellScan = new Long(source.EnableMemShellScan);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "ScanPattern", this.ScanPattern);
this.setParamSimple(map, prefix + "HostType", this.HostType);
this.setParamArraySimple(map, prefix + "QuuidList.", this.QuuidList);
this.setParamSimple(map, prefix + "TimeoutPeriod", this.TimeoutPeriod);
this.setParamSimple(map, prefix + "EngineType", this.EngineType);
this.setParamSimple(map, prefix + "EnableMemShellScan", this.EnableMemShellScan);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy