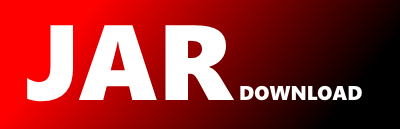
com.tencentcloudapi.cwp.v20180228.models.DescribeLicenseGeneralResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.cwp.v20180228.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class DescribeLicenseGeneralResponse extends AbstractModel {
/**
* Total number of authorizations (including those that are isolated, expired, and in other states)
*/
@SerializedName("LicenseCnt")
@Expose
private Long LicenseCnt;
/**
* Number of available authorizations
*/
@SerializedName("AvailableLicenseCnt")
@Expose
private Long AvailableLicenseCnt;
/**
* Number of available Professional Edition authorizations (including those in postpaid mode)
*/
@SerializedName("AvailableProVersionLicenseCnt")
@Expose
private Long AvailableProVersionLicenseCnt;
/**
* Number of available Ultimate Edition authorizations
*/
@SerializedName("AvailableFlagshipVersionLicenseCnt")
@Expose
private Long AvailableFlagshipVersionLicenseCnt;
/**
* Number of authorizations about to expire (no more than 15 days left)
*/
@SerializedName("NearExpiryLicenseCnt")
@Expose
private Long NearExpiryLicenseCnt;
/**
* Number of expired authorizations (excluding deleted ones)
*/
@SerializedName("ExpireLicenseCnt")
@Expose
private Long ExpireLicenseCnt;
/**
* Automatic upgrade enabling status. True: enabled; false: disabled. Default value: false.
*/
@SerializedName("AutoOpenStatus")
@Expose
private Boolean AutoOpenStatus;
/**
* PROVERSION_POSTPAY: Pro Edition - postpaid; PROVERSION_PREPAY: Pro edition - prepaid; FLAGSHIP_PREPAY: Ultimate Edition - prepaid.
*/
@SerializedName("ProtectType")
@Expose
private String ProtectType;
/**
* Whether automatic upgrade has been enabled before
*/
@SerializedName("IsOpenStatusHistory")
@Expose
private Boolean IsOpenStatusHistory;
/**
* Number of used authorizations
*/
@SerializedName("UsedLicenseCnt")
@Expose
private Long UsedLicenseCnt;
/**
* Number of authorizations that have not expired
*/
@SerializedName("NotExpiredLicenseCnt")
@Expose
private Long NotExpiredLicenseCnt;
/**
* Total number of Ultimate Edition authorizations (valid orders)
*/
@SerializedName("FlagshipVersionLicenseCnt")
@Expose
private Long FlagshipVersionLicenseCnt;
/**
* Total number of Pro Edition authorizations (valid orders)
*/
@SerializedName("ProVersionLicenseCnt")
@Expose
private Long ProVersionLicenseCnt;
/**
* Total number of Inclusive Edition authorizations (those of valid orders)
*/
@SerializedName("CwpVersionLicenseCnt")
@Expose
private Long CwpVersionLicenseCnt;
/**
* Number of available Inclusive Edition authorizations
*/
@SerializedName("AvailableLHLicenseCnt")
@Expose
private Long AvailableLHLicenseCnt;
/**
* Auto-purchase switch, true for ON, false for OFF
*/
@SerializedName("AutoRepurchaseSwitch")
@Expose
private Boolean AutoRepurchaseSwitch;
/**
* Is auto-renewal required for auto-purchase orders, true for ON, false for OFF
*/
@SerializedName("AutoRepurchaseRenewSwitch")
@Expose
private Boolean AutoRepurchaseRenewSwitch;
/**
* Number of terminated orders
*/
@SerializedName("DestroyOrderNum")
@Expose
private Long DestroyOrderNum;
/**
* Whether automatic renewal is enabled. True: enabled; false: disabled.
*/
@SerializedName("RepurchaseRenewSwitch")
@Expose
private Boolean RepurchaseRenewSwitch;
/**
* The unique request ID, generated by the server, will be returned for every request (if the request fails to reach the server for other reasons, the request will not obtain a RequestId). RequestId is required for locating a problem.
*/
@SerializedName("RequestId")
@Expose
private String RequestId;
/**
* Get Total number of authorizations (including those that are isolated, expired, and in other states)
* @return LicenseCnt Total number of authorizations (including those that are isolated, expired, and in other states)
*/
public Long getLicenseCnt() {
return this.LicenseCnt;
}
/**
* Set Total number of authorizations (including those that are isolated, expired, and in other states)
* @param LicenseCnt Total number of authorizations (including those that are isolated, expired, and in other states)
*/
public void setLicenseCnt(Long LicenseCnt) {
this.LicenseCnt = LicenseCnt;
}
/**
* Get Number of available authorizations
* @return AvailableLicenseCnt Number of available authorizations
*/
public Long getAvailableLicenseCnt() {
return this.AvailableLicenseCnt;
}
/**
* Set Number of available authorizations
* @param AvailableLicenseCnt Number of available authorizations
*/
public void setAvailableLicenseCnt(Long AvailableLicenseCnt) {
this.AvailableLicenseCnt = AvailableLicenseCnt;
}
/**
* Get Number of available Professional Edition authorizations (including those in postpaid mode)
* @return AvailableProVersionLicenseCnt Number of available Professional Edition authorizations (including those in postpaid mode)
*/
public Long getAvailableProVersionLicenseCnt() {
return this.AvailableProVersionLicenseCnt;
}
/**
* Set Number of available Professional Edition authorizations (including those in postpaid mode)
* @param AvailableProVersionLicenseCnt Number of available Professional Edition authorizations (including those in postpaid mode)
*/
public void setAvailableProVersionLicenseCnt(Long AvailableProVersionLicenseCnt) {
this.AvailableProVersionLicenseCnt = AvailableProVersionLicenseCnt;
}
/**
* Get Number of available Ultimate Edition authorizations
* @return AvailableFlagshipVersionLicenseCnt Number of available Ultimate Edition authorizations
*/
public Long getAvailableFlagshipVersionLicenseCnt() {
return this.AvailableFlagshipVersionLicenseCnt;
}
/**
* Set Number of available Ultimate Edition authorizations
* @param AvailableFlagshipVersionLicenseCnt Number of available Ultimate Edition authorizations
*/
public void setAvailableFlagshipVersionLicenseCnt(Long AvailableFlagshipVersionLicenseCnt) {
this.AvailableFlagshipVersionLicenseCnt = AvailableFlagshipVersionLicenseCnt;
}
/**
* Get Number of authorizations about to expire (no more than 15 days left)
* @return NearExpiryLicenseCnt Number of authorizations about to expire (no more than 15 days left)
*/
public Long getNearExpiryLicenseCnt() {
return this.NearExpiryLicenseCnt;
}
/**
* Set Number of authorizations about to expire (no more than 15 days left)
* @param NearExpiryLicenseCnt Number of authorizations about to expire (no more than 15 days left)
*/
public void setNearExpiryLicenseCnt(Long NearExpiryLicenseCnt) {
this.NearExpiryLicenseCnt = NearExpiryLicenseCnt;
}
/**
* Get Number of expired authorizations (excluding deleted ones)
* @return ExpireLicenseCnt Number of expired authorizations (excluding deleted ones)
*/
public Long getExpireLicenseCnt() {
return this.ExpireLicenseCnt;
}
/**
* Set Number of expired authorizations (excluding deleted ones)
* @param ExpireLicenseCnt Number of expired authorizations (excluding deleted ones)
*/
public void setExpireLicenseCnt(Long ExpireLicenseCnt) {
this.ExpireLicenseCnt = ExpireLicenseCnt;
}
/**
* Get Automatic upgrade enabling status. True: enabled; false: disabled. Default value: false.
* @return AutoOpenStatus Automatic upgrade enabling status. True: enabled; false: disabled. Default value: false.
*/
public Boolean getAutoOpenStatus() {
return this.AutoOpenStatus;
}
/**
* Set Automatic upgrade enabling status. True: enabled; false: disabled. Default value: false.
* @param AutoOpenStatus Automatic upgrade enabling status. True: enabled; false: disabled. Default value: false.
*/
public void setAutoOpenStatus(Boolean AutoOpenStatus) {
this.AutoOpenStatus = AutoOpenStatus;
}
/**
* Get PROVERSION_POSTPAY: Pro Edition - postpaid; PROVERSION_PREPAY: Pro edition - prepaid; FLAGSHIP_PREPAY: Ultimate Edition - prepaid.
* @return ProtectType PROVERSION_POSTPAY: Pro Edition - postpaid; PROVERSION_PREPAY: Pro edition - prepaid; FLAGSHIP_PREPAY: Ultimate Edition - prepaid.
*/
public String getProtectType() {
return this.ProtectType;
}
/**
* Set PROVERSION_POSTPAY: Pro Edition - postpaid; PROVERSION_PREPAY: Pro edition - prepaid; FLAGSHIP_PREPAY: Ultimate Edition - prepaid.
* @param ProtectType PROVERSION_POSTPAY: Pro Edition - postpaid; PROVERSION_PREPAY: Pro edition - prepaid; FLAGSHIP_PREPAY: Ultimate Edition - prepaid.
*/
public void setProtectType(String ProtectType) {
this.ProtectType = ProtectType;
}
/**
* Get Whether automatic upgrade has been enabled before
* @return IsOpenStatusHistory Whether automatic upgrade has been enabled before
*/
public Boolean getIsOpenStatusHistory() {
return this.IsOpenStatusHistory;
}
/**
* Set Whether automatic upgrade has been enabled before
* @param IsOpenStatusHistory Whether automatic upgrade has been enabled before
*/
public void setIsOpenStatusHistory(Boolean IsOpenStatusHistory) {
this.IsOpenStatusHistory = IsOpenStatusHistory;
}
/**
* Get Number of used authorizations
* @return UsedLicenseCnt Number of used authorizations
*/
public Long getUsedLicenseCnt() {
return this.UsedLicenseCnt;
}
/**
* Set Number of used authorizations
* @param UsedLicenseCnt Number of used authorizations
*/
public void setUsedLicenseCnt(Long UsedLicenseCnt) {
this.UsedLicenseCnt = UsedLicenseCnt;
}
/**
* Get Number of authorizations that have not expired
* @return NotExpiredLicenseCnt Number of authorizations that have not expired
*/
public Long getNotExpiredLicenseCnt() {
return this.NotExpiredLicenseCnt;
}
/**
* Set Number of authorizations that have not expired
* @param NotExpiredLicenseCnt Number of authorizations that have not expired
*/
public void setNotExpiredLicenseCnt(Long NotExpiredLicenseCnt) {
this.NotExpiredLicenseCnt = NotExpiredLicenseCnt;
}
/**
* Get Total number of Ultimate Edition authorizations (valid orders)
* @return FlagshipVersionLicenseCnt Total number of Ultimate Edition authorizations (valid orders)
*/
public Long getFlagshipVersionLicenseCnt() {
return this.FlagshipVersionLicenseCnt;
}
/**
* Set Total number of Ultimate Edition authorizations (valid orders)
* @param FlagshipVersionLicenseCnt Total number of Ultimate Edition authorizations (valid orders)
*/
public void setFlagshipVersionLicenseCnt(Long FlagshipVersionLicenseCnt) {
this.FlagshipVersionLicenseCnt = FlagshipVersionLicenseCnt;
}
/**
* Get Total number of Pro Edition authorizations (valid orders)
* @return ProVersionLicenseCnt Total number of Pro Edition authorizations (valid orders)
*/
public Long getProVersionLicenseCnt() {
return this.ProVersionLicenseCnt;
}
/**
* Set Total number of Pro Edition authorizations (valid orders)
* @param ProVersionLicenseCnt Total number of Pro Edition authorizations (valid orders)
*/
public void setProVersionLicenseCnt(Long ProVersionLicenseCnt) {
this.ProVersionLicenseCnt = ProVersionLicenseCnt;
}
/**
* Get Total number of Inclusive Edition authorizations (those of valid orders)
* @return CwpVersionLicenseCnt Total number of Inclusive Edition authorizations (those of valid orders)
*/
public Long getCwpVersionLicenseCnt() {
return this.CwpVersionLicenseCnt;
}
/**
* Set Total number of Inclusive Edition authorizations (those of valid orders)
* @param CwpVersionLicenseCnt Total number of Inclusive Edition authorizations (those of valid orders)
*/
public void setCwpVersionLicenseCnt(Long CwpVersionLicenseCnt) {
this.CwpVersionLicenseCnt = CwpVersionLicenseCnt;
}
/**
* Get Number of available Inclusive Edition authorizations
* @return AvailableLHLicenseCnt Number of available Inclusive Edition authorizations
*/
public Long getAvailableLHLicenseCnt() {
return this.AvailableLHLicenseCnt;
}
/**
* Set Number of available Inclusive Edition authorizations
* @param AvailableLHLicenseCnt Number of available Inclusive Edition authorizations
*/
public void setAvailableLHLicenseCnt(Long AvailableLHLicenseCnt) {
this.AvailableLHLicenseCnt = AvailableLHLicenseCnt;
}
/**
* Get Auto-purchase switch, true for ON, false for OFF
* @return AutoRepurchaseSwitch Auto-purchase switch, true for ON, false for OFF
*/
public Boolean getAutoRepurchaseSwitch() {
return this.AutoRepurchaseSwitch;
}
/**
* Set Auto-purchase switch, true for ON, false for OFF
* @param AutoRepurchaseSwitch Auto-purchase switch, true for ON, false for OFF
*/
public void setAutoRepurchaseSwitch(Boolean AutoRepurchaseSwitch) {
this.AutoRepurchaseSwitch = AutoRepurchaseSwitch;
}
/**
* Get Is auto-renewal required for auto-purchase orders, true for ON, false for OFF
* @return AutoRepurchaseRenewSwitch Is auto-renewal required for auto-purchase orders, true for ON, false for OFF
*/
public Boolean getAutoRepurchaseRenewSwitch() {
return this.AutoRepurchaseRenewSwitch;
}
/**
* Set Is auto-renewal required for auto-purchase orders, true for ON, false for OFF
* @param AutoRepurchaseRenewSwitch Is auto-renewal required for auto-purchase orders, true for ON, false for OFF
*/
public void setAutoRepurchaseRenewSwitch(Boolean AutoRepurchaseRenewSwitch) {
this.AutoRepurchaseRenewSwitch = AutoRepurchaseRenewSwitch;
}
/**
* Get Number of terminated orders
* @return DestroyOrderNum Number of terminated orders
*/
public Long getDestroyOrderNum() {
return this.DestroyOrderNum;
}
/**
* Set Number of terminated orders
* @param DestroyOrderNum Number of terminated orders
*/
public void setDestroyOrderNum(Long DestroyOrderNum) {
this.DestroyOrderNum = DestroyOrderNum;
}
/**
* Get Whether automatic renewal is enabled. True: enabled; false: disabled.
* @return RepurchaseRenewSwitch Whether automatic renewal is enabled. True: enabled; false: disabled.
*/
public Boolean getRepurchaseRenewSwitch() {
return this.RepurchaseRenewSwitch;
}
/**
* Set Whether automatic renewal is enabled. True: enabled; false: disabled.
* @param RepurchaseRenewSwitch Whether automatic renewal is enabled. True: enabled; false: disabled.
*/
public void setRepurchaseRenewSwitch(Boolean RepurchaseRenewSwitch) {
this.RepurchaseRenewSwitch = RepurchaseRenewSwitch;
}
/**
* Get The unique request ID, generated by the server, will be returned for every request (if the request fails to reach the server for other reasons, the request will not obtain a RequestId). RequestId is required for locating a problem.
* @return RequestId The unique request ID, generated by the server, will be returned for every request (if the request fails to reach the server for other reasons, the request will not obtain a RequestId). RequestId is required for locating a problem.
*/
public String getRequestId() {
return this.RequestId;
}
/**
* Set The unique request ID, generated by the server, will be returned for every request (if the request fails to reach the server for other reasons, the request will not obtain a RequestId). RequestId is required for locating a problem.
* @param RequestId The unique request ID, generated by the server, will be returned for every request (if the request fails to reach the server for other reasons, the request will not obtain a RequestId). RequestId is required for locating a problem.
*/
public void setRequestId(String RequestId) {
this.RequestId = RequestId;
}
public DescribeLicenseGeneralResponse() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public DescribeLicenseGeneralResponse(DescribeLicenseGeneralResponse source) {
if (source.LicenseCnt != null) {
this.LicenseCnt = new Long(source.LicenseCnt);
}
if (source.AvailableLicenseCnt != null) {
this.AvailableLicenseCnt = new Long(source.AvailableLicenseCnt);
}
if (source.AvailableProVersionLicenseCnt != null) {
this.AvailableProVersionLicenseCnt = new Long(source.AvailableProVersionLicenseCnt);
}
if (source.AvailableFlagshipVersionLicenseCnt != null) {
this.AvailableFlagshipVersionLicenseCnt = new Long(source.AvailableFlagshipVersionLicenseCnt);
}
if (source.NearExpiryLicenseCnt != null) {
this.NearExpiryLicenseCnt = new Long(source.NearExpiryLicenseCnt);
}
if (source.ExpireLicenseCnt != null) {
this.ExpireLicenseCnt = new Long(source.ExpireLicenseCnt);
}
if (source.AutoOpenStatus != null) {
this.AutoOpenStatus = new Boolean(source.AutoOpenStatus);
}
if (source.ProtectType != null) {
this.ProtectType = new String(source.ProtectType);
}
if (source.IsOpenStatusHistory != null) {
this.IsOpenStatusHistory = new Boolean(source.IsOpenStatusHistory);
}
if (source.UsedLicenseCnt != null) {
this.UsedLicenseCnt = new Long(source.UsedLicenseCnt);
}
if (source.NotExpiredLicenseCnt != null) {
this.NotExpiredLicenseCnt = new Long(source.NotExpiredLicenseCnt);
}
if (source.FlagshipVersionLicenseCnt != null) {
this.FlagshipVersionLicenseCnt = new Long(source.FlagshipVersionLicenseCnt);
}
if (source.ProVersionLicenseCnt != null) {
this.ProVersionLicenseCnt = new Long(source.ProVersionLicenseCnt);
}
if (source.CwpVersionLicenseCnt != null) {
this.CwpVersionLicenseCnt = new Long(source.CwpVersionLicenseCnt);
}
if (source.AvailableLHLicenseCnt != null) {
this.AvailableLHLicenseCnt = new Long(source.AvailableLHLicenseCnt);
}
if (source.AutoRepurchaseSwitch != null) {
this.AutoRepurchaseSwitch = new Boolean(source.AutoRepurchaseSwitch);
}
if (source.AutoRepurchaseRenewSwitch != null) {
this.AutoRepurchaseRenewSwitch = new Boolean(source.AutoRepurchaseRenewSwitch);
}
if (source.DestroyOrderNum != null) {
this.DestroyOrderNum = new Long(source.DestroyOrderNum);
}
if (source.RepurchaseRenewSwitch != null) {
this.RepurchaseRenewSwitch = new Boolean(source.RepurchaseRenewSwitch);
}
if (source.RequestId != null) {
this.RequestId = new String(source.RequestId);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "LicenseCnt", this.LicenseCnt);
this.setParamSimple(map, prefix + "AvailableLicenseCnt", this.AvailableLicenseCnt);
this.setParamSimple(map, prefix + "AvailableProVersionLicenseCnt", this.AvailableProVersionLicenseCnt);
this.setParamSimple(map, prefix + "AvailableFlagshipVersionLicenseCnt", this.AvailableFlagshipVersionLicenseCnt);
this.setParamSimple(map, prefix + "NearExpiryLicenseCnt", this.NearExpiryLicenseCnt);
this.setParamSimple(map, prefix + "ExpireLicenseCnt", this.ExpireLicenseCnt);
this.setParamSimple(map, prefix + "AutoOpenStatus", this.AutoOpenStatus);
this.setParamSimple(map, prefix + "ProtectType", this.ProtectType);
this.setParamSimple(map, prefix + "IsOpenStatusHistory", this.IsOpenStatusHistory);
this.setParamSimple(map, prefix + "UsedLicenseCnt", this.UsedLicenseCnt);
this.setParamSimple(map, prefix + "NotExpiredLicenseCnt", this.NotExpiredLicenseCnt);
this.setParamSimple(map, prefix + "FlagshipVersionLicenseCnt", this.FlagshipVersionLicenseCnt);
this.setParamSimple(map, prefix + "ProVersionLicenseCnt", this.ProVersionLicenseCnt);
this.setParamSimple(map, prefix + "CwpVersionLicenseCnt", this.CwpVersionLicenseCnt);
this.setParamSimple(map, prefix + "AvailableLHLicenseCnt", this.AvailableLHLicenseCnt);
this.setParamSimple(map, prefix + "AutoRepurchaseSwitch", this.AutoRepurchaseSwitch);
this.setParamSimple(map, prefix + "AutoRepurchaseRenewSwitch", this.AutoRepurchaseRenewSwitch);
this.setParamSimple(map, prefix + "DestroyOrderNum", this.DestroyOrderNum);
this.setParamSimple(map, prefix + "RepurchaseRenewSwitch", this.RepurchaseRenewSwitch);
this.setParamSimple(map, prefix + "RequestId", this.RequestId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy