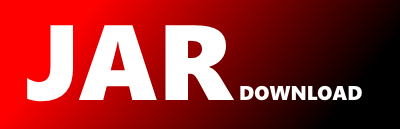
com.tencentcloudapi.cwp.v20180228.models.SyncBaselineDetectSummaryResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.cwp.v20180228.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class SyncBaselineDetectSummaryResponse extends AbstractModel {
/**
* Processing progress
*/
@SerializedName("ProgressRate")
@Expose
private Long ProgressRate;
/**
* Total number of failed policies
*/
@SerializedName("NotPassPolicyCount")
@Expose
private Long NotPassPolicyCount;
/**
* Total number of hosts
*/
@SerializedName("HostCount")
@Expose
private Long HostCount;
/**
* Start time
*/
@SerializedName("StartTime")
@Expose
private String StartTime;
/**
* End time
*/
@SerializedName("EndTime")
@Expose
private String EndTime;
/**
* 1: first scan about to start; 0: already scanned.
*/
@SerializedName("WillFirstScan")
@Expose
private Long WillFirstScan;
/**
* ID of ongoing detection task
*/
@SerializedName("DetectingTaskIds")
@Expose
private Long [] DetectingTaskIds;
/**
* Remaining scanning time (minutes)
*/
@SerializedName("LeftMins")
@Expose
private Long LeftMins;
/**
* The unique request ID, generated by the server, will be returned for every request (if the request fails to reach the server for other reasons, the request will not obtain a RequestId). RequestId is required for locating a problem.
*/
@SerializedName("RequestId")
@Expose
private String RequestId;
/**
* Get Processing progress
* @return ProgressRate Processing progress
*/
public Long getProgressRate() {
return this.ProgressRate;
}
/**
* Set Processing progress
* @param ProgressRate Processing progress
*/
public void setProgressRate(Long ProgressRate) {
this.ProgressRate = ProgressRate;
}
/**
* Get Total number of failed policies
* @return NotPassPolicyCount Total number of failed policies
*/
public Long getNotPassPolicyCount() {
return this.NotPassPolicyCount;
}
/**
* Set Total number of failed policies
* @param NotPassPolicyCount Total number of failed policies
*/
public void setNotPassPolicyCount(Long NotPassPolicyCount) {
this.NotPassPolicyCount = NotPassPolicyCount;
}
/**
* Get Total number of hosts
* @return HostCount Total number of hosts
*/
public Long getHostCount() {
return this.HostCount;
}
/**
* Set Total number of hosts
* @param HostCount Total number of hosts
*/
public void setHostCount(Long HostCount) {
this.HostCount = HostCount;
}
/**
* Get Start time
* @return StartTime Start time
*/
public String getStartTime() {
return this.StartTime;
}
/**
* Set Start time
* @param StartTime Start time
*/
public void setStartTime(String StartTime) {
this.StartTime = StartTime;
}
/**
* Get End time
* @return EndTime End time
*/
public String getEndTime() {
return this.EndTime;
}
/**
* Set End time
* @param EndTime End time
*/
public void setEndTime(String EndTime) {
this.EndTime = EndTime;
}
/**
* Get 1: first scan about to start; 0: already scanned.
* @return WillFirstScan 1: first scan about to start; 0: already scanned.
*/
public Long getWillFirstScan() {
return this.WillFirstScan;
}
/**
* Set 1: first scan about to start; 0: already scanned.
* @param WillFirstScan 1: first scan about to start; 0: already scanned.
*/
public void setWillFirstScan(Long WillFirstScan) {
this.WillFirstScan = WillFirstScan;
}
/**
* Get ID of ongoing detection task
* @return DetectingTaskIds ID of ongoing detection task
*/
public Long [] getDetectingTaskIds() {
return this.DetectingTaskIds;
}
/**
* Set ID of ongoing detection task
* @param DetectingTaskIds ID of ongoing detection task
*/
public void setDetectingTaskIds(Long [] DetectingTaskIds) {
this.DetectingTaskIds = DetectingTaskIds;
}
/**
* Get Remaining scanning time (minutes)
* @return LeftMins Remaining scanning time (minutes)
*/
public Long getLeftMins() {
return this.LeftMins;
}
/**
* Set Remaining scanning time (minutes)
* @param LeftMins Remaining scanning time (minutes)
*/
public void setLeftMins(Long LeftMins) {
this.LeftMins = LeftMins;
}
/**
* Get The unique request ID, generated by the server, will be returned for every request (if the request fails to reach the server for other reasons, the request will not obtain a RequestId). RequestId is required for locating a problem.
* @return RequestId The unique request ID, generated by the server, will be returned for every request (if the request fails to reach the server for other reasons, the request will not obtain a RequestId). RequestId is required for locating a problem.
*/
public String getRequestId() {
return this.RequestId;
}
/**
* Set The unique request ID, generated by the server, will be returned for every request (if the request fails to reach the server for other reasons, the request will not obtain a RequestId). RequestId is required for locating a problem.
* @param RequestId The unique request ID, generated by the server, will be returned for every request (if the request fails to reach the server for other reasons, the request will not obtain a RequestId). RequestId is required for locating a problem.
*/
public void setRequestId(String RequestId) {
this.RequestId = RequestId;
}
public SyncBaselineDetectSummaryResponse() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public SyncBaselineDetectSummaryResponse(SyncBaselineDetectSummaryResponse source) {
if (source.ProgressRate != null) {
this.ProgressRate = new Long(source.ProgressRate);
}
if (source.NotPassPolicyCount != null) {
this.NotPassPolicyCount = new Long(source.NotPassPolicyCount);
}
if (source.HostCount != null) {
this.HostCount = new Long(source.HostCount);
}
if (source.StartTime != null) {
this.StartTime = new String(source.StartTime);
}
if (source.EndTime != null) {
this.EndTime = new String(source.EndTime);
}
if (source.WillFirstScan != null) {
this.WillFirstScan = new Long(source.WillFirstScan);
}
if (source.DetectingTaskIds != null) {
this.DetectingTaskIds = new Long[source.DetectingTaskIds.length];
for (int i = 0; i < source.DetectingTaskIds.length; i++) {
this.DetectingTaskIds[i] = new Long(source.DetectingTaskIds[i]);
}
}
if (source.LeftMins != null) {
this.LeftMins = new Long(source.LeftMins);
}
if (source.RequestId != null) {
this.RequestId = new String(source.RequestId);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "ProgressRate", this.ProgressRate);
this.setParamSimple(map, prefix + "NotPassPolicyCount", this.NotPassPolicyCount);
this.setParamSimple(map, prefix + "HostCount", this.HostCount);
this.setParamSimple(map, prefix + "StartTime", this.StartTime);
this.setParamSimple(map, prefix + "EndTime", this.EndTime);
this.setParamSimple(map, prefix + "WillFirstScan", this.WillFirstScan);
this.setParamArraySimple(map, prefix + "DetectingTaskIds.", this.DetectingTaskIds);
this.setParamSimple(map, prefix + "LeftMins", this.LeftMins);
this.setParamSimple(map, prefix + "RequestId", this.RequestId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy