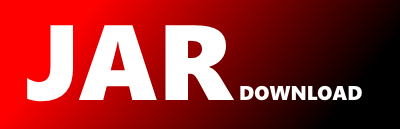
com.tencentcloudapi.organization.v20210331.models.DeleteRoleAssignmentRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.organization.v20210331.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class DeleteRoleAssignmentRequest extends AbstractModel {
/**
* Space ID.
*/
@SerializedName("ZoneId")
@Expose
private String ZoneId;
/**
* Permission configuration ID.
*/
@SerializedName("RoleConfigurationId")
@Expose
private String RoleConfigurationId;
/**
* Type of the synchronized target account of the Tencent Cloud Organization. ManagerUin: admin account; MemberUin: member account.
*/
@SerializedName("TargetType")
@Expose
private String TargetType;
/**
* UIN of the target account of the Tencent Cloud Organization.
*/
@SerializedName("TargetUin")
@Expose
private Long TargetUin;
/**
* Identity type for the CAM user synchronization. Valid values: User: indicates that the identity for the CAM user synchronization is a CIC user; Group: indicates that the identity for the CAM user synchronization is a CIC user group.
*/
@SerializedName("PrincipalType")
@Expose
private String PrincipalType;
/**
* Identity ID for the CAM user synchronization. Valid values: When the PrincipalType value is Group, it is the CIC user group ID (g-********). When the PrincipalType value is User, it is the CIC user ID (u-********).
*/
@SerializedName("PrincipalId")
@Expose
private String PrincipalId;
/**
* Whether to undeploy an access configuration synchronously when you remove the last authorization for using this access configuration on the target account of the Tencent Cloud Organization. Valid values: DeprovisionForLastRoleAssignmentOnAccount: Undeploy the access configuration; None (default): Do not undeploy access configuration.
*/
@SerializedName("DeprovisionStrategy")
@Expose
private String DeprovisionStrategy;
/**
* Get Space ID.
* @return ZoneId Space ID.
*/
public String getZoneId() {
return this.ZoneId;
}
/**
* Set Space ID.
* @param ZoneId Space ID.
*/
public void setZoneId(String ZoneId) {
this.ZoneId = ZoneId;
}
/**
* Get Permission configuration ID.
* @return RoleConfigurationId Permission configuration ID.
*/
public String getRoleConfigurationId() {
return this.RoleConfigurationId;
}
/**
* Set Permission configuration ID.
* @param RoleConfigurationId Permission configuration ID.
*/
public void setRoleConfigurationId(String RoleConfigurationId) {
this.RoleConfigurationId = RoleConfigurationId;
}
/**
* Get Type of the synchronized target account of the Tencent Cloud Organization. ManagerUin: admin account; MemberUin: member account.
* @return TargetType Type of the synchronized target account of the Tencent Cloud Organization. ManagerUin: admin account; MemberUin: member account.
*/
public String getTargetType() {
return this.TargetType;
}
/**
* Set Type of the synchronized target account of the Tencent Cloud Organization. ManagerUin: admin account; MemberUin: member account.
* @param TargetType Type of the synchronized target account of the Tencent Cloud Organization. ManagerUin: admin account; MemberUin: member account.
*/
public void setTargetType(String TargetType) {
this.TargetType = TargetType;
}
/**
* Get UIN of the target account of the Tencent Cloud Organization.
* @return TargetUin UIN of the target account of the Tencent Cloud Organization.
*/
public Long getTargetUin() {
return this.TargetUin;
}
/**
* Set UIN of the target account of the Tencent Cloud Organization.
* @param TargetUin UIN of the target account of the Tencent Cloud Organization.
*/
public void setTargetUin(Long TargetUin) {
this.TargetUin = TargetUin;
}
/**
* Get Identity type for the CAM user synchronization. Valid values: User: indicates that the identity for the CAM user synchronization is a CIC user; Group: indicates that the identity for the CAM user synchronization is a CIC user group.
* @return PrincipalType Identity type for the CAM user synchronization. Valid values: User: indicates that the identity for the CAM user synchronization is a CIC user; Group: indicates that the identity for the CAM user synchronization is a CIC user group.
*/
public String getPrincipalType() {
return this.PrincipalType;
}
/**
* Set Identity type for the CAM user synchronization. Valid values: User: indicates that the identity for the CAM user synchronization is a CIC user; Group: indicates that the identity for the CAM user synchronization is a CIC user group.
* @param PrincipalType Identity type for the CAM user synchronization. Valid values: User: indicates that the identity for the CAM user synchronization is a CIC user; Group: indicates that the identity for the CAM user synchronization is a CIC user group.
*/
public void setPrincipalType(String PrincipalType) {
this.PrincipalType = PrincipalType;
}
/**
* Get Identity ID for the CAM user synchronization. Valid values: When the PrincipalType value is Group, it is the CIC user group ID (g-********). When the PrincipalType value is User, it is the CIC user ID (u-********).
* @return PrincipalId Identity ID for the CAM user synchronization. Valid values: When the PrincipalType value is Group, it is the CIC user group ID (g-********). When the PrincipalType value is User, it is the CIC user ID (u-********).
*/
public String getPrincipalId() {
return this.PrincipalId;
}
/**
* Set Identity ID for the CAM user synchronization. Valid values: When the PrincipalType value is Group, it is the CIC user group ID (g-********). When the PrincipalType value is User, it is the CIC user ID (u-********).
* @param PrincipalId Identity ID for the CAM user synchronization. Valid values: When the PrincipalType value is Group, it is the CIC user group ID (g-********). When the PrincipalType value is User, it is the CIC user ID (u-********).
*/
public void setPrincipalId(String PrincipalId) {
this.PrincipalId = PrincipalId;
}
/**
* Get Whether to undeploy an access configuration synchronously when you remove the last authorization for using this access configuration on the target account of the Tencent Cloud Organization. Valid values: DeprovisionForLastRoleAssignmentOnAccount: Undeploy the access configuration; None (default): Do not undeploy access configuration.
* @return DeprovisionStrategy Whether to undeploy an access configuration synchronously when you remove the last authorization for using this access configuration on the target account of the Tencent Cloud Organization. Valid values: DeprovisionForLastRoleAssignmentOnAccount: Undeploy the access configuration; None (default): Do not undeploy access configuration.
*/
public String getDeprovisionStrategy() {
return this.DeprovisionStrategy;
}
/**
* Set Whether to undeploy an access configuration synchronously when you remove the last authorization for using this access configuration on the target account of the Tencent Cloud Organization. Valid values: DeprovisionForLastRoleAssignmentOnAccount: Undeploy the access configuration; None (default): Do not undeploy access configuration.
* @param DeprovisionStrategy Whether to undeploy an access configuration synchronously when you remove the last authorization for using this access configuration on the target account of the Tencent Cloud Organization. Valid values: DeprovisionForLastRoleAssignmentOnAccount: Undeploy the access configuration; None (default): Do not undeploy access configuration.
*/
public void setDeprovisionStrategy(String DeprovisionStrategy) {
this.DeprovisionStrategy = DeprovisionStrategy;
}
public DeleteRoleAssignmentRequest() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public DeleteRoleAssignmentRequest(DeleteRoleAssignmentRequest source) {
if (source.ZoneId != null) {
this.ZoneId = new String(source.ZoneId);
}
if (source.RoleConfigurationId != null) {
this.RoleConfigurationId = new String(source.RoleConfigurationId);
}
if (source.TargetType != null) {
this.TargetType = new String(source.TargetType);
}
if (source.TargetUin != null) {
this.TargetUin = new Long(source.TargetUin);
}
if (source.PrincipalType != null) {
this.PrincipalType = new String(source.PrincipalType);
}
if (source.PrincipalId != null) {
this.PrincipalId = new String(source.PrincipalId);
}
if (source.DeprovisionStrategy != null) {
this.DeprovisionStrategy = new String(source.DeprovisionStrategy);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "ZoneId", this.ZoneId);
this.setParamSimple(map, prefix + "RoleConfigurationId", this.RoleConfigurationId);
this.setParamSimple(map, prefix + "TargetType", this.TargetType);
this.setParamSimple(map, prefix + "TargetUin", this.TargetUin);
this.setParamSimple(map, prefix + "PrincipalType", this.PrincipalType);
this.setParamSimple(map, prefix + "PrincipalId", this.PrincipalId);
this.setParamSimple(map, prefix + "DeprovisionStrategy", this.DeprovisionStrategy);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy