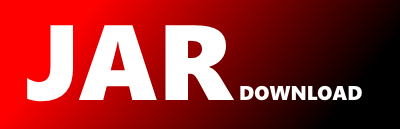
com.tencentcloudapi.teo.v20220901.models.DescribeTopL7CacheDataRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.teo.v20220901.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class DescribeTopL7CacheDataRequest extends AbstractModel {
/**
* The start time.
*/
@SerializedName("StartTime")
@Expose
private String StartTime;
/**
* The end time.
*/
@SerializedName("EndTime")
@Expose
private String EndTime;
/**
* The query metric. Values:
`l7Cache_outFlux_domain`: Host/Domain name;
`l7Cache_outFlux_url`: URL address;
`l7Cache_outFlux_resourceType`: Resource type;
`l7Cache_outFlux_statusCode`: Status code.
*/
@SerializedName("MetricName")
@Expose
private String MetricName;
/**
* ZoneId set. This parameter is required.
*/
@SerializedName("ZoneIds")
@Expose
private String [] ZoneIds;
/**
* The number of data entries to be queried. The maximum value is 1000. If it is not specified, the value 10 is used by default, indicating that the top 10 data entries.
*/
@SerializedName("Limit")
@Expose
private Long Limit;
/**
* Filter conditions. See below for details:
`domain`
Filter by the sub-domain name, such as `test.example.com`
Type: String
Required: No
`url`
Filter by the URL, such as `/content`. The query period cannot exceed 30 days.
Type: String
Required: No
`resourceType`
Filter by the resource file type, such as `jpg`, `png`. The query period cannot exceed 30 days.
Type: String
Required: No
cacheType
Filter by the cache hit result.
Type: String
Required: No
Values:
`hit`: Cache hit;
`dynamic`: Resource non-cacheable;
`miss`: Cache miss
`statusCode`
Filter by the status code. The query period cannot exceed 30 days.
Type: String
Required: No
Values:
`1XX`: All 1xx status codes;
`100`: 100 status code;
`101`: 101 status code;
`102`: 102 status code;
`2XX`: All 2xx status codes;
`200`: 200 status code;
`201`: 201 status code;
`202`: 202 status code;
`203`: 203 status code;
`204`: 204 status code;
`205`: 205 status code;
`206`: 206 status code;
`207`: 207 status code;
`3XX`: All 3xx status codes;
`300`: 300 status code;
`301`: 301 status code;
`302`: 302 status code;
`303`: 303 status code;
`304`: 304 status code;
`305`: 305 status code;
`307`: 307 status code;
`4XX`: All 4xx status codes;
`400`: 400 status code;
`401`: 401 status code;
`402`: 402 status code;
`403`: 403 status code;
`404`: 404 status code;
`405`: 405 status code;
`406`: 406 status code;
`407`: 407 status code;
`408`: 408 status code;
`409`: 409 status code;
`410`: 410 status code;
`411`: 411 status code;
`412`: 412 status code;
`412`: 413 status code;
`414`: 414 status code;
`415`: 415 status code;
`416`: 416 status code;
`417`: 417 status code;
`422`: 422 status code;
`423`: 423 status code;
`424`: 424 status code;
`426`: 426 status code;
`451`: 451 status code;
`5XX`: All 5xx status codes;
`500`: 500 status code;
`501`: 501 status code;
`502`: 502 status code;
`503`: 503 status code;
`504`: 504 status code;
`505`: 505 status code;
`506`: 506 status code;
`507`: 507 status code;
`510`: 510 status code;
`514`: 514 status code;
`544`: 544 status code.
`tagKey`:
Filter by the tag key
Type: String
Required: No
`tagValue`
Filter by the tag value
Type: String
Required: No
*/
@SerializedName("Filters")
@Expose
private QueryCondition [] Filters;
/**
* The query time granularity. Values:
`min`: 1 minute;
`5min`: 5 minutes;
`hour`: 1 hour;
`day`: 1 day. If this field is not specified, the granularity will be determined based on the interval between the start time and end time as follows: 1-minute granularity applies for a 1-hour interval, 5-minute granularity for a 2-day interval, 1-hour granularity for a 7-day interval, and 1-day granularity for an interval of over 7 days.
*/
@SerializedName("Interval")
@Expose
private String Interval;
/**
* Geolocation scope. Values:
`overseas`: Regions outside the Chinese mainland
`mainland`: Chinese mainland
`global`: Global If this field is not specified, the default value `global` is used.
*/
@SerializedName("Area")
@Expose
private String Area;
/**
* Get The start time.
* @return StartTime The start time.
*/
public String getStartTime() {
return this.StartTime;
}
/**
* Set The start time.
* @param StartTime The start time.
*/
public void setStartTime(String StartTime) {
this.StartTime = StartTime;
}
/**
* Get The end time.
* @return EndTime The end time.
*/
public String getEndTime() {
return this.EndTime;
}
/**
* Set The end time.
* @param EndTime The end time.
*/
public void setEndTime(String EndTime) {
this.EndTime = EndTime;
}
/**
* Get The query metric. Values:
`l7Cache_outFlux_domain`: Host/Domain name;
`l7Cache_outFlux_url`: URL address;
`l7Cache_outFlux_resourceType`: Resource type;
`l7Cache_outFlux_statusCode`: Status code.
* @return MetricName The query metric. Values:
`l7Cache_outFlux_domain`: Host/Domain name;
`l7Cache_outFlux_url`: URL address;
`l7Cache_outFlux_resourceType`: Resource type;
`l7Cache_outFlux_statusCode`: Status code.
*/
public String getMetricName() {
return this.MetricName;
}
/**
* Set The query metric. Values:
`l7Cache_outFlux_domain`: Host/Domain name;
`l7Cache_outFlux_url`: URL address;
`l7Cache_outFlux_resourceType`: Resource type;
`l7Cache_outFlux_statusCode`: Status code.
* @param MetricName The query metric. Values:
`l7Cache_outFlux_domain`: Host/Domain name;
`l7Cache_outFlux_url`: URL address;
`l7Cache_outFlux_resourceType`: Resource type;
`l7Cache_outFlux_statusCode`: Status code.
*/
public void setMetricName(String MetricName) {
this.MetricName = MetricName;
}
/**
* Get ZoneId set. This parameter is required.
* @return ZoneIds ZoneId set. This parameter is required.
*/
public String [] getZoneIds() {
return this.ZoneIds;
}
/**
* Set ZoneId set. This parameter is required.
* @param ZoneIds ZoneId set. This parameter is required.
*/
public void setZoneIds(String [] ZoneIds) {
this.ZoneIds = ZoneIds;
}
/**
* Get The number of data entries to be queried. The maximum value is 1000. If it is not specified, the value 10 is used by default, indicating that the top 10 data entries.
* @return Limit The number of data entries to be queried. The maximum value is 1000. If it is not specified, the value 10 is used by default, indicating that the top 10 data entries.
*/
public Long getLimit() {
return this.Limit;
}
/**
* Set The number of data entries to be queried. The maximum value is 1000. If it is not specified, the value 10 is used by default, indicating that the top 10 data entries.
* @param Limit The number of data entries to be queried. The maximum value is 1000. If it is not specified, the value 10 is used by default, indicating that the top 10 data entries.
*/
public void setLimit(Long Limit) {
this.Limit = Limit;
}
/**
* Get Filter conditions. See below for details:
`domain`
Filter by the sub-domain name, such as `test.example.com`
Type: String
Required: No
`url`
Filter by the URL, such as `/content`. The query period cannot exceed 30 days.
Type: String
Required: No
`resourceType`
Filter by the resource file type, such as `jpg`, `png`. The query period cannot exceed 30 days.
Type: String
Required: No
cacheType
Filter by the cache hit result.
Type: String
Required: No
Values:
`hit`: Cache hit;
`dynamic`: Resource non-cacheable;
`miss`: Cache miss
`statusCode`
Filter by the status code. The query period cannot exceed 30 days.
Type: String
Required: No
Values:
`1XX`: All 1xx status codes;
`100`: 100 status code;
`101`: 101 status code;
`102`: 102 status code;
`2XX`: All 2xx status codes;
`200`: 200 status code;
`201`: 201 status code;
`202`: 202 status code;
`203`: 203 status code;
`204`: 204 status code;
`205`: 205 status code;
`206`: 206 status code;
`207`: 207 status code;
`3XX`: All 3xx status codes;
`300`: 300 status code;
`301`: 301 status code;
`302`: 302 status code;
`303`: 303 status code;
`304`: 304 status code;
`305`: 305 status code;
`307`: 307 status code;
`4XX`: All 4xx status codes;
`400`: 400 status code;
`401`: 401 status code;
`402`: 402 status code;
`403`: 403 status code;
`404`: 404 status code;
`405`: 405 status code;
`406`: 406 status code;
`407`: 407 status code;
`408`: 408 status code;
`409`: 409 status code;
`410`: 410 status code;
`411`: 411 status code;
`412`: 412 status code;
`412`: 413 status code;
`414`: 414 status code;
`415`: 415 status code;
`416`: 416 status code;
`417`: 417 status code;
`422`: 422 status code;
`423`: 423 status code;
`424`: 424 status code;
`426`: 426 status code;
`451`: 451 status code;
`5XX`: All 5xx status codes;
`500`: 500 status code;
`501`: 501 status code;
`502`: 502 status code;
`503`: 503 status code;
`504`: 504 status code;
`505`: 505 status code;
`506`: 506 status code;
`507`: 507 status code;
`510`: 510 status code;
`514`: 514 status code;
`544`: 544 status code.
`tagKey`:
Filter by the tag key
Type: String
Required: No
`tagValue`
Filter by the tag value
Type: String
Required: No
* @return Filters Filter conditions. See below for details:
`domain`
Filter by the sub-domain name, such as `test.example.com`
Type: String
Required: No
`url`
Filter by the URL, such as `/content`. The query period cannot exceed 30 days.
Type: String
Required: No
`resourceType`
Filter by the resource file type, such as `jpg`, `png`. The query period cannot exceed 30 days.
Type: String
Required: No
cacheType
Filter by the cache hit result.
Type: String
Required: No
Values:
`hit`: Cache hit;
`dynamic`: Resource non-cacheable;
`miss`: Cache miss
`statusCode`
Filter by the status code. The query period cannot exceed 30 days.
Type: String
Required: No
Values:
`1XX`: All 1xx status codes;
`100`: 100 status code;
`101`: 101 status code;
`102`: 102 status code;
`2XX`: All 2xx status codes;
`200`: 200 status code;
`201`: 201 status code;
`202`: 202 status code;
`203`: 203 status code;
`204`: 204 status code;
`205`: 205 status code;
`206`: 206 status code;
`207`: 207 status code;
`3XX`: All 3xx status codes;
`300`: 300 status code;
`301`: 301 status code;
`302`: 302 status code;
`303`: 303 status code;
`304`: 304 status code;
`305`: 305 status code;
`307`: 307 status code;
`4XX`: All 4xx status codes;
`400`: 400 status code;
`401`: 401 status code;
`402`: 402 status code;
`403`: 403 status code;
`404`: 404 status code;
`405`: 405 status code;
`406`: 406 status code;
`407`: 407 status code;
`408`: 408 status code;
`409`: 409 status code;
`410`: 410 status code;
`411`: 411 status code;
`412`: 412 status code;
`412`: 413 status code;
`414`: 414 status code;
`415`: 415 status code;
`416`: 416 status code;
`417`: 417 status code;
`422`: 422 status code;
`423`: 423 status code;
`424`: 424 status code;
`426`: 426 status code;
`451`: 451 status code;
`5XX`: All 5xx status codes;
`500`: 500 status code;
`501`: 501 status code;
`502`: 502 status code;
`503`: 503 status code;
`504`: 504 status code;
`505`: 505 status code;
`506`: 506 status code;
`507`: 507 status code;
`510`: 510 status code;
`514`: 514 status code;
`544`: 544 status code.
`tagKey`:
Filter by the tag key
Type: String
Required: No
`tagValue`
Filter by the tag value
Type: String
Required: No
*/
public QueryCondition [] getFilters() {
return this.Filters;
}
/**
* Set Filter conditions. See below for details:
`domain`
Filter by the sub-domain name, such as `test.example.com`
Type: String
Required: No
`url`
Filter by the URL, such as `/content`. The query period cannot exceed 30 days.
Type: String
Required: No
`resourceType`
Filter by the resource file type, such as `jpg`, `png`. The query period cannot exceed 30 days.
Type: String
Required: No
cacheType
Filter by the cache hit result.
Type: String
Required: No
Values:
`hit`: Cache hit;
`dynamic`: Resource non-cacheable;
`miss`: Cache miss
`statusCode`
Filter by the status code. The query period cannot exceed 30 days.
Type: String
Required: No
Values:
`1XX`: All 1xx status codes;
`100`: 100 status code;
`101`: 101 status code;
`102`: 102 status code;
`2XX`: All 2xx status codes;
`200`: 200 status code;
`201`: 201 status code;
`202`: 202 status code;
`203`: 203 status code;
`204`: 204 status code;
`205`: 205 status code;
`206`: 206 status code;
`207`: 207 status code;
`3XX`: All 3xx status codes;
`300`: 300 status code;
`301`: 301 status code;
`302`: 302 status code;
`303`: 303 status code;
`304`: 304 status code;
`305`: 305 status code;
`307`: 307 status code;
`4XX`: All 4xx status codes;
`400`: 400 status code;
`401`: 401 status code;
`402`: 402 status code;
`403`: 403 status code;
`404`: 404 status code;
`405`: 405 status code;
`406`: 406 status code;
`407`: 407 status code;
`408`: 408 status code;
`409`: 409 status code;
`410`: 410 status code;
`411`: 411 status code;
`412`: 412 status code;
`412`: 413 status code;
`414`: 414 status code;
`415`: 415 status code;
`416`: 416 status code;
`417`: 417 status code;
`422`: 422 status code;
`423`: 423 status code;
`424`: 424 status code;
`426`: 426 status code;
`451`: 451 status code;
`5XX`: All 5xx status codes;
`500`: 500 status code;
`501`: 501 status code;
`502`: 502 status code;
`503`: 503 status code;
`504`: 504 status code;
`505`: 505 status code;
`506`: 506 status code;
`507`: 507 status code;
`510`: 510 status code;
`514`: 514 status code;
`544`: 544 status code.
`tagKey`:
Filter by the tag key
Type: String
Required: No
`tagValue`
Filter by the tag value
Type: String
Required: No
* @param Filters Filter conditions. See below for details:
`domain`
Filter by the sub-domain name, such as `test.example.com`
Type: String
Required: No
`url`
Filter by the URL, such as `/content`. The query period cannot exceed 30 days.
Type: String
Required: No
`resourceType`
Filter by the resource file type, such as `jpg`, `png`. The query period cannot exceed 30 days.
Type: String
Required: No
cacheType
Filter by the cache hit result.
Type: String
Required: No
Values:
`hit`: Cache hit;
`dynamic`: Resource non-cacheable;
`miss`: Cache miss
`statusCode`
Filter by the status code. The query period cannot exceed 30 days.
Type: String
Required: No
Values:
`1XX`: All 1xx status codes;
`100`: 100 status code;
`101`: 101 status code;
`102`: 102 status code;
`2XX`: All 2xx status codes;
`200`: 200 status code;
`201`: 201 status code;
`202`: 202 status code;
`203`: 203 status code;
`204`: 204 status code;
`205`: 205 status code;
`206`: 206 status code;
`207`: 207 status code;
`3XX`: All 3xx status codes;
`300`: 300 status code;
`301`: 301 status code;
`302`: 302 status code;
`303`: 303 status code;
`304`: 304 status code;
`305`: 305 status code;
`307`: 307 status code;
`4XX`: All 4xx status codes;
`400`: 400 status code;
`401`: 401 status code;
`402`: 402 status code;
`403`: 403 status code;
`404`: 404 status code;
`405`: 405 status code;
`406`: 406 status code;
`407`: 407 status code;
`408`: 408 status code;
`409`: 409 status code;
`410`: 410 status code;
`411`: 411 status code;
`412`: 412 status code;
`412`: 413 status code;
`414`: 414 status code;
`415`: 415 status code;
`416`: 416 status code;
`417`: 417 status code;
`422`: 422 status code;
`423`: 423 status code;
`424`: 424 status code;
`426`: 426 status code;
`451`: 451 status code;
`5XX`: All 5xx status codes;
`500`: 500 status code;
`501`: 501 status code;
`502`: 502 status code;
`503`: 503 status code;
`504`: 504 status code;
`505`: 505 status code;
`506`: 506 status code;
`507`: 507 status code;
`510`: 510 status code;
`514`: 514 status code;
`544`: 544 status code.
`tagKey`:
Filter by the tag key
Type: String
Required: No
`tagValue`
Filter by the tag value
Type: String
Required: No
*/
public void setFilters(QueryCondition [] Filters) {
this.Filters = Filters;
}
/**
* Get The query time granularity. Values:
`min`: 1 minute;
`5min`: 5 minutes;
`hour`: 1 hour;
`day`: 1 day. If this field is not specified, the granularity will be determined based on the interval between the start time and end time as follows: 1-minute granularity applies for a 1-hour interval, 5-minute granularity for a 2-day interval, 1-hour granularity for a 7-day interval, and 1-day granularity for an interval of over 7 days.
* @return Interval The query time granularity. Values:
`min`: 1 minute;
`5min`: 5 minutes;
`hour`: 1 hour;
`day`: 1 day. If this field is not specified, the granularity will be determined based on the interval between the start time and end time as follows: 1-minute granularity applies for a 1-hour interval, 5-minute granularity for a 2-day interval, 1-hour granularity for a 7-day interval, and 1-day granularity for an interval of over 7 days.
*/
public String getInterval() {
return this.Interval;
}
/**
* Set The query time granularity. Values:
`min`: 1 minute;
`5min`: 5 minutes;
`hour`: 1 hour;
`day`: 1 day. If this field is not specified, the granularity will be determined based on the interval between the start time and end time as follows: 1-minute granularity applies for a 1-hour interval, 5-minute granularity for a 2-day interval, 1-hour granularity for a 7-day interval, and 1-day granularity for an interval of over 7 days.
* @param Interval The query time granularity. Values:
`min`: 1 minute;
`5min`: 5 minutes;
`hour`: 1 hour;
`day`: 1 day. If this field is not specified, the granularity will be determined based on the interval between the start time and end time as follows: 1-minute granularity applies for a 1-hour interval, 5-minute granularity for a 2-day interval, 1-hour granularity for a 7-day interval, and 1-day granularity for an interval of over 7 days.
*/
public void setInterval(String Interval) {
this.Interval = Interval;
}
/**
* Get Geolocation scope. Values:
`overseas`: Regions outside the Chinese mainland
`mainland`: Chinese mainland
`global`: Global If this field is not specified, the default value `global` is used.
* @return Area Geolocation scope. Values:
`overseas`: Regions outside the Chinese mainland
`mainland`: Chinese mainland
`global`: Global If this field is not specified, the default value `global` is used.
*/
public String getArea() {
return this.Area;
}
/**
* Set Geolocation scope. Values:
`overseas`: Regions outside the Chinese mainland
`mainland`: Chinese mainland
`global`: Global If this field is not specified, the default value `global` is used.
* @param Area Geolocation scope. Values:
`overseas`: Regions outside the Chinese mainland
`mainland`: Chinese mainland
`global`: Global If this field is not specified, the default value `global` is used.
*/
public void setArea(String Area) {
this.Area = Area;
}
public DescribeTopL7CacheDataRequest() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public DescribeTopL7CacheDataRequest(DescribeTopL7CacheDataRequest source) {
if (source.StartTime != null) {
this.StartTime = new String(source.StartTime);
}
if (source.EndTime != null) {
this.EndTime = new String(source.EndTime);
}
if (source.MetricName != null) {
this.MetricName = new String(source.MetricName);
}
if (source.ZoneIds != null) {
this.ZoneIds = new String[source.ZoneIds.length];
for (int i = 0; i < source.ZoneIds.length; i++) {
this.ZoneIds[i] = new String(source.ZoneIds[i]);
}
}
if (source.Limit != null) {
this.Limit = new Long(source.Limit);
}
if (source.Filters != null) {
this.Filters = new QueryCondition[source.Filters.length];
for (int i = 0; i < source.Filters.length; i++) {
this.Filters[i] = new QueryCondition(source.Filters[i]);
}
}
if (source.Interval != null) {
this.Interval = new String(source.Interval);
}
if (source.Area != null) {
this.Area = new String(source.Area);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "StartTime", this.StartTime);
this.setParamSimple(map, prefix + "EndTime", this.EndTime);
this.setParamSimple(map, prefix + "MetricName", this.MetricName);
this.setParamArraySimple(map, prefix + "ZoneIds.", this.ZoneIds);
this.setParamSimple(map, prefix + "Limit", this.Limit);
this.setParamArrayObj(map, prefix + "Filters.", this.Filters);
this.setParamSimple(map, prefix + "Interval", this.Interval);
this.setParamSimple(map, prefix + "Area", this.Area);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy