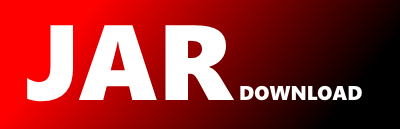
com.tencentcloudapi.teo.v20220901.models.OriginDetail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.teo.v20220901.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class OriginDetail extends AbstractModel {
/**
* Origin server type. Valid values:
IP_DOMAIN: IPv4, IPv6, or domain name type origin server;
COS: Tencent Cloud COS origin server;
AWS_S3: AWS S3 COS origin server;
ORIGIN_GROUP: origin server group;
VOD: Video on Demand;
SPACE: origin server uninstallation, currently only available to the allowlist;
LB: load balancing. Currently only available to the allowlist.
*/
@SerializedName("OriginType")
@Expose
private String OriginType;
/**
* Origin server address, which varies with the value of OriginType:
When OriginType = IP_DOMAIN, this parameter is an IPv4 address, an IPv6 address, or a domain name.
When OriginType = COS, this parameter is the access domain name of the COS bucket.
When OriginType = AWS_S3, this parameter is the access domain name of the S3 bucket.
When OriginType = ORIGIN_GROUP, this parameter is the origin server group ID.
When OriginType = VOD, this parameter is the VOD application ID.
*/
@SerializedName("Origin")
@Expose
private String Origin;
/**
* Secondary origin group ID. This parameter is valid only when OriginType is ORIGIN_GROUP and a secondary origin group is configured.
*/
@SerializedName("BackupOrigin")
@Expose
private String BackupOrigin;
/**
* Primary origin group name. This parameter returns a value when OriginType is ORIGIN_GROUP.
*/
@SerializedName("OriginGroupName")
@Expose
private String OriginGroupName;
/**
* Secondary origin group name. This parameter is valid only when OriginType is ORIGIN_GROUP and a secondary origin group is configured.
*/
@SerializedName("BackOriginGroupName")
@Expose
private String BackOriginGroupName;
/**
* Whether access to the private object storage origin server is allowed. This parameter is valid only when the origin server type OriginType is COS or AWS_S3. Valid values:
on: Enable private authentication;
off: Disable private authentication.
If this field is not specified, the default value 'off' will be used.
*/
@SerializedName("PrivateAccess")
@Expose
private String PrivateAccess;
/**
* Private authentication parameter. This parameter is valid only when PrivateAccess is on.
Note: This field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("PrivateParameters")
@Expose
private PrivateParameter [] PrivateParameters;
/**
* MO sub-application ID
*/
@SerializedName("VodeoSubAppId")
@Expose
private Long VodeoSubAppId;
/**
* MO distribution range. Valid values: All: all Bucket: bucket
*/
@SerializedName("VodeoDistributionRange")
@Expose
private String VodeoDistributionRange;
/**
* MO bucket ID, required when the distribution range (DistributionRange) is bucket (Bucket)
*/
@SerializedName("VodeoBucketId")
@Expose
private String VodeoBucketId;
/**
* Get Origin server type. Valid values:
IP_DOMAIN: IPv4, IPv6, or domain name type origin server;
COS: Tencent Cloud COS origin server;
AWS_S3: AWS S3 COS origin server;
ORIGIN_GROUP: origin server group;
VOD: Video on Demand;
SPACE: origin server uninstallation, currently only available to the allowlist;
LB: load balancing. Currently only available to the allowlist.
* @return OriginType Origin server type. Valid values:
IP_DOMAIN: IPv4, IPv6, or domain name type origin server;
COS: Tencent Cloud COS origin server;
AWS_S3: AWS S3 COS origin server;
ORIGIN_GROUP: origin server group;
VOD: Video on Demand;
SPACE: origin server uninstallation, currently only available to the allowlist;
LB: load balancing. Currently only available to the allowlist.
*/
public String getOriginType() {
return this.OriginType;
}
/**
* Set Origin server type. Valid values:
IP_DOMAIN: IPv4, IPv6, or domain name type origin server;
COS: Tencent Cloud COS origin server;
AWS_S3: AWS S3 COS origin server;
ORIGIN_GROUP: origin server group;
VOD: Video on Demand;
SPACE: origin server uninstallation, currently only available to the allowlist;
LB: load balancing. Currently only available to the allowlist.
* @param OriginType Origin server type. Valid values:
IP_DOMAIN: IPv4, IPv6, or domain name type origin server;
COS: Tencent Cloud COS origin server;
AWS_S3: AWS S3 COS origin server;
ORIGIN_GROUP: origin server group;
VOD: Video on Demand;
SPACE: origin server uninstallation, currently only available to the allowlist;
LB: load balancing. Currently only available to the allowlist.
*/
public void setOriginType(String OriginType) {
this.OriginType = OriginType;
}
/**
* Get Origin server address, which varies with the value of OriginType:
When OriginType = IP_DOMAIN, this parameter is an IPv4 address, an IPv6 address, or a domain name.
When OriginType = COS, this parameter is the access domain name of the COS bucket.
When OriginType = AWS_S3, this parameter is the access domain name of the S3 bucket.
When OriginType = ORIGIN_GROUP, this parameter is the origin server group ID.
When OriginType = VOD, this parameter is the VOD application ID.
* @return Origin Origin server address, which varies with the value of OriginType:
When OriginType = IP_DOMAIN, this parameter is an IPv4 address, an IPv6 address, or a domain name.
When OriginType = COS, this parameter is the access domain name of the COS bucket.
When OriginType = AWS_S3, this parameter is the access domain name of the S3 bucket.
When OriginType = ORIGIN_GROUP, this parameter is the origin server group ID.
When OriginType = VOD, this parameter is the VOD application ID.
*/
public String getOrigin() {
return this.Origin;
}
/**
* Set Origin server address, which varies with the value of OriginType:
When OriginType = IP_DOMAIN, this parameter is an IPv4 address, an IPv6 address, or a domain name.
When OriginType = COS, this parameter is the access domain name of the COS bucket.
When OriginType = AWS_S3, this parameter is the access domain name of the S3 bucket.
When OriginType = ORIGIN_GROUP, this parameter is the origin server group ID.
When OriginType = VOD, this parameter is the VOD application ID.
* @param Origin Origin server address, which varies with the value of OriginType:
When OriginType = IP_DOMAIN, this parameter is an IPv4 address, an IPv6 address, or a domain name.
When OriginType = COS, this parameter is the access domain name of the COS bucket.
When OriginType = AWS_S3, this parameter is the access domain name of the S3 bucket.
When OriginType = ORIGIN_GROUP, this parameter is the origin server group ID.
When OriginType = VOD, this parameter is the VOD application ID.
*/
public void setOrigin(String Origin) {
this.Origin = Origin;
}
/**
* Get Secondary origin group ID. This parameter is valid only when OriginType is ORIGIN_GROUP and a secondary origin group is configured.
* @return BackupOrigin Secondary origin group ID. This parameter is valid only when OriginType is ORIGIN_GROUP and a secondary origin group is configured.
*/
public String getBackupOrigin() {
return this.BackupOrigin;
}
/**
* Set Secondary origin group ID. This parameter is valid only when OriginType is ORIGIN_GROUP and a secondary origin group is configured.
* @param BackupOrigin Secondary origin group ID. This parameter is valid only when OriginType is ORIGIN_GROUP and a secondary origin group is configured.
*/
public void setBackupOrigin(String BackupOrigin) {
this.BackupOrigin = BackupOrigin;
}
/**
* Get Primary origin group name. This parameter returns a value when OriginType is ORIGIN_GROUP.
* @return OriginGroupName Primary origin group name. This parameter returns a value when OriginType is ORIGIN_GROUP.
*/
public String getOriginGroupName() {
return this.OriginGroupName;
}
/**
* Set Primary origin group name. This parameter returns a value when OriginType is ORIGIN_GROUP.
* @param OriginGroupName Primary origin group name. This parameter returns a value when OriginType is ORIGIN_GROUP.
*/
public void setOriginGroupName(String OriginGroupName) {
this.OriginGroupName = OriginGroupName;
}
/**
* Get Secondary origin group name. This parameter is valid only when OriginType is ORIGIN_GROUP and a secondary origin group is configured.
* @return BackOriginGroupName Secondary origin group name. This parameter is valid only when OriginType is ORIGIN_GROUP and a secondary origin group is configured.
*/
public String getBackOriginGroupName() {
return this.BackOriginGroupName;
}
/**
* Set Secondary origin group name. This parameter is valid only when OriginType is ORIGIN_GROUP and a secondary origin group is configured.
* @param BackOriginGroupName Secondary origin group name. This parameter is valid only when OriginType is ORIGIN_GROUP and a secondary origin group is configured.
*/
public void setBackOriginGroupName(String BackOriginGroupName) {
this.BackOriginGroupName = BackOriginGroupName;
}
/**
* Get Whether access to the private object storage origin server is allowed. This parameter is valid only when the origin server type OriginType is COS or AWS_S3. Valid values:
on: Enable private authentication;
off: Disable private authentication.
If this field is not specified, the default value 'off' will be used.
* @return PrivateAccess Whether access to the private object storage origin server is allowed. This parameter is valid only when the origin server type OriginType is COS or AWS_S3. Valid values:
on: Enable private authentication;
off: Disable private authentication.
If this field is not specified, the default value 'off' will be used.
*/
public String getPrivateAccess() {
return this.PrivateAccess;
}
/**
* Set Whether access to the private object storage origin server is allowed. This parameter is valid only when the origin server type OriginType is COS or AWS_S3. Valid values:
on: Enable private authentication;
off: Disable private authentication.
If this field is not specified, the default value 'off' will be used.
* @param PrivateAccess Whether access to the private object storage origin server is allowed. This parameter is valid only when the origin server type OriginType is COS or AWS_S3. Valid values:
on: Enable private authentication;
off: Disable private authentication.
If this field is not specified, the default value 'off' will be used.
*/
public void setPrivateAccess(String PrivateAccess) {
this.PrivateAccess = PrivateAccess;
}
/**
* Get Private authentication parameter. This parameter is valid only when PrivateAccess is on.
Note: This field may return null, indicating that no valid values can be obtained.
* @return PrivateParameters Private authentication parameter. This parameter is valid only when PrivateAccess is on.
Note: This field may return null, indicating that no valid values can be obtained.
*/
public PrivateParameter [] getPrivateParameters() {
return this.PrivateParameters;
}
/**
* Set Private authentication parameter. This parameter is valid only when PrivateAccess is on.
Note: This field may return null, indicating that no valid values can be obtained.
* @param PrivateParameters Private authentication parameter. This parameter is valid only when PrivateAccess is on.
Note: This field may return null, indicating that no valid values can be obtained.
*/
public void setPrivateParameters(PrivateParameter [] PrivateParameters) {
this.PrivateParameters = PrivateParameters;
}
/**
* Get MO sub-application ID
* @return VodeoSubAppId MO sub-application ID
* @deprecated
*/
@Deprecated
public Long getVodeoSubAppId() {
return this.VodeoSubAppId;
}
/**
* Set MO sub-application ID
* @param VodeoSubAppId MO sub-application ID
* @deprecated
*/
@Deprecated
public void setVodeoSubAppId(Long VodeoSubAppId) {
this.VodeoSubAppId = VodeoSubAppId;
}
/**
* Get MO distribution range. Valid values: All: all Bucket: bucket
* @return VodeoDistributionRange MO distribution range. Valid values: All: all Bucket: bucket
* @deprecated
*/
@Deprecated
public String getVodeoDistributionRange() {
return this.VodeoDistributionRange;
}
/**
* Set MO distribution range. Valid values: All: all Bucket: bucket
* @param VodeoDistributionRange MO distribution range. Valid values: All: all Bucket: bucket
* @deprecated
*/
@Deprecated
public void setVodeoDistributionRange(String VodeoDistributionRange) {
this.VodeoDistributionRange = VodeoDistributionRange;
}
/**
* Get MO bucket ID, required when the distribution range (DistributionRange) is bucket (Bucket)
* @return VodeoBucketId MO bucket ID, required when the distribution range (DistributionRange) is bucket (Bucket)
* @deprecated
*/
@Deprecated
public String getVodeoBucketId() {
return this.VodeoBucketId;
}
/**
* Set MO bucket ID, required when the distribution range (DistributionRange) is bucket (Bucket)
* @param VodeoBucketId MO bucket ID, required when the distribution range (DistributionRange) is bucket (Bucket)
* @deprecated
*/
@Deprecated
public void setVodeoBucketId(String VodeoBucketId) {
this.VodeoBucketId = VodeoBucketId;
}
public OriginDetail() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public OriginDetail(OriginDetail source) {
if (source.OriginType != null) {
this.OriginType = new String(source.OriginType);
}
if (source.Origin != null) {
this.Origin = new String(source.Origin);
}
if (source.BackupOrigin != null) {
this.BackupOrigin = new String(source.BackupOrigin);
}
if (source.OriginGroupName != null) {
this.OriginGroupName = new String(source.OriginGroupName);
}
if (source.BackOriginGroupName != null) {
this.BackOriginGroupName = new String(source.BackOriginGroupName);
}
if (source.PrivateAccess != null) {
this.PrivateAccess = new String(source.PrivateAccess);
}
if (source.PrivateParameters != null) {
this.PrivateParameters = new PrivateParameter[source.PrivateParameters.length];
for (int i = 0; i < source.PrivateParameters.length; i++) {
this.PrivateParameters[i] = new PrivateParameter(source.PrivateParameters[i]);
}
}
if (source.VodeoSubAppId != null) {
this.VodeoSubAppId = new Long(source.VodeoSubAppId);
}
if (source.VodeoDistributionRange != null) {
this.VodeoDistributionRange = new String(source.VodeoDistributionRange);
}
if (source.VodeoBucketId != null) {
this.VodeoBucketId = new String(source.VodeoBucketId);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "OriginType", this.OriginType);
this.setParamSimple(map, prefix + "Origin", this.Origin);
this.setParamSimple(map, prefix + "BackupOrigin", this.BackupOrigin);
this.setParamSimple(map, prefix + "OriginGroupName", this.OriginGroupName);
this.setParamSimple(map, prefix + "BackOriginGroupName", this.BackOriginGroupName);
this.setParamSimple(map, prefix + "PrivateAccess", this.PrivateAccess);
this.setParamArrayObj(map, prefix + "PrivateParameters.", this.PrivateParameters);
this.setParamSimple(map, prefix + "VodeoSubAppId", this.VodeoSubAppId);
this.setParamSimple(map, prefix + "VodeoDistributionRange", this.VodeoDistributionRange);
this.setParamSimple(map, prefix + "VodeoBucketId", this.VodeoBucketId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy