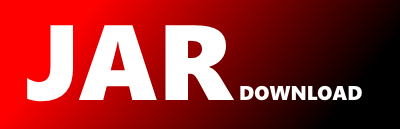
com.tencentcloudapi.teo.v20220901.models.RulesSettingAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.teo.v20220901.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class RulesSettingAction extends AbstractModel {
/**
* Feature name. Valid values:
Access URL rewrite (`AccessUrlRedirect`).
Origin-pull URL rewrite (`UpstreamUrlRedirect`).
Custom error page
(`ErrorPage`).
QUIC (`QUIC`).
WebSocket (`WebSocket`).
Video dragging (`VideoSeek`).
Token authentication (`Authentication`).
`CacheKey`: Custom cache key.
`Cache`: Node cache TTL.
`MaxAge`: Browser cache TTL.
`OfflineCache`: Offline cache.
`SmartRouting`: Smart acceleration.
`RangeOriginPull`: Range GETs.
`UpstreamHttp2`: HTTP/2 forwarding.
`HostHeader`: Host header rewrite.
`ForceRedirect`: Force HTTPS.
`OriginPullProtocol`: Origin-pull HTTPS.
`CachePrefresh`: Cache prefresh.
`Compression`: Smart compression.
`RequestHeader`: HTTP request header modification.
HTTP response header modification (`ResponseHeader`).
Status code cache TTL (`StatusCodeCache`).
`Hsts`.
`ClientIpHeader`.
`TlsVersion`.
`OcspStapling`.
*/
@SerializedName("Action")
@Expose
private String Action;
/**
* Parameter information
*/
@SerializedName("Properties")
@Expose
private RulesProperties [] Properties;
/**
* Get Feature name. Valid values:
Access URL rewrite (`AccessUrlRedirect`).
Origin-pull URL rewrite (`UpstreamUrlRedirect`).
Custom error page
(`ErrorPage`).
QUIC (`QUIC`).
WebSocket (`WebSocket`).
Video dragging (`VideoSeek`).
Token authentication (`Authentication`).
`CacheKey`: Custom cache key.
`Cache`: Node cache TTL.
`MaxAge`: Browser cache TTL.
`OfflineCache`: Offline cache.
`SmartRouting`: Smart acceleration.
`RangeOriginPull`: Range GETs.
`UpstreamHttp2`: HTTP/2 forwarding.
`HostHeader`: Host header rewrite.
`ForceRedirect`: Force HTTPS.
`OriginPullProtocol`: Origin-pull HTTPS.
`CachePrefresh`: Cache prefresh.
`Compression`: Smart compression.
`RequestHeader`: HTTP request header modification.
HTTP response header modification (`ResponseHeader`).
Status code cache TTL (`StatusCodeCache`).
`Hsts`.
`ClientIpHeader`.
`TlsVersion`.
`OcspStapling`.
* @return Action Feature name. Valid values:
Access URL rewrite (`AccessUrlRedirect`).
Origin-pull URL rewrite (`UpstreamUrlRedirect`).
Custom error page
(`ErrorPage`).
QUIC (`QUIC`).
WebSocket (`WebSocket`).
Video dragging (`VideoSeek`).
Token authentication (`Authentication`).
`CacheKey`: Custom cache key.
`Cache`: Node cache TTL.
`MaxAge`: Browser cache TTL.
`OfflineCache`: Offline cache.
`SmartRouting`: Smart acceleration.
`RangeOriginPull`: Range GETs.
`UpstreamHttp2`: HTTP/2 forwarding.
`HostHeader`: Host header rewrite.
`ForceRedirect`: Force HTTPS.
`OriginPullProtocol`: Origin-pull HTTPS.
`CachePrefresh`: Cache prefresh.
`Compression`: Smart compression.
`RequestHeader`: HTTP request header modification.
HTTP response header modification (`ResponseHeader`).
Status code cache TTL (`StatusCodeCache`).
`Hsts`.
`ClientIpHeader`.
`TlsVersion`.
`OcspStapling`.
*/
public String getAction() {
return this.Action;
}
/**
* Set Feature name. Valid values:
Access URL rewrite (`AccessUrlRedirect`).
Origin-pull URL rewrite (`UpstreamUrlRedirect`).
Custom error page
(`ErrorPage`).
QUIC (`QUIC`).
WebSocket (`WebSocket`).
Video dragging (`VideoSeek`).
Token authentication (`Authentication`).
`CacheKey`: Custom cache key.
`Cache`: Node cache TTL.
`MaxAge`: Browser cache TTL.
`OfflineCache`: Offline cache.
`SmartRouting`: Smart acceleration.
`RangeOriginPull`: Range GETs.
`UpstreamHttp2`: HTTP/2 forwarding.
`HostHeader`: Host header rewrite.
`ForceRedirect`: Force HTTPS.
`OriginPullProtocol`: Origin-pull HTTPS.
`CachePrefresh`: Cache prefresh.
`Compression`: Smart compression.
`RequestHeader`: HTTP request header modification.
HTTP response header modification (`ResponseHeader`).
Status code cache TTL (`StatusCodeCache`).
`Hsts`.
`ClientIpHeader`.
`TlsVersion`.
`OcspStapling`.
* @param Action Feature name. Valid values:
Access URL rewrite (`AccessUrlRedirect`).
Origin-pull URL rewrite (`UpstreamUrlRedirect`).
Custom error page
(`ErrorPage`).
QUIC (`QUIC`).
WebSocket (`WebSocket`).
Video dragging (`VideoSeek`).
Token authentication (`Authentication`).
`CacheKey`: Custom cache key.
`Cache`: Node cache TTL.
`MaxAge`: Browser cache TTL.
`OfflineCache`: Offline cache.
`SmartRouting`: Smart acceleration.
`RangeOriginPull`: Range GETs.
`UpstreamHttp2`: HTTP/2 forwarding.
`HostHeader`: Host header rewrite.
`ForceRedirect`: Force HTTPS.
`OriginPullProtocol`: Origin-pull HTTPS.
`CachePrefresh`: Cache prefresh.
`Compression`: Smart compression.
`RequestHeader`: HTTP request header modification.
HTTP response header modification (`ResponseHeader`).
Status code cache TTL (`StatusCodeCache`).
`Hsts`.
`ClientIpHeader`.
`TlsVersion`.
`OcspStapling`.
*/
public void setAction(String Action) {
this.Action = Action;
}
/**
* Get Parameter information
* @return Properties Parameter information
*/
public RulesProperties [] getProperties() {
return this.Properties;
}
/**
* Set Parameter information
* @param Properties Parameter information
*/
public void setProperties(RulesProperties [] Properties) {
this.Properties = Properties;
}
public RulesSettingAction() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public RulesSettingAction(RulesSettingAction source) {
if (source.Action != null) {
this.Action = new String(source.Action);
}
if (source.Properties != null) {
this.Properties = new RulesProperties[source.Properties.length];
for (int i = 0; i < source.Properties.length; i++) {
this.Properties[i] = new RulesProperties(source.Properties[i]);
}
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "Action", this.Action);
this.setParamArrayObj(map, prefix + "Properties.", this.Properties);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy