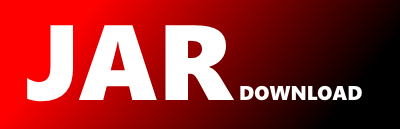
com.tencentcloudapi.vod.v20180717.models.CreateHeadTailTemplateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.vod.v20180717.models;
import com.tencentcloudapi.common.AbstractModel;
import com.tencentcloudapi.common.SSEResponseModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class CreateHeadTailTemplateRequest extends AbstractModel {
/**
* Template name, length limit is 64 characters.
*/
@SerializedName("Name")
@Expose
private String Name;
/**
* The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
*/
@SerializedName("SubAppId")
@Expose
private Long SubAppId;
/**
* Template description information, length limit is 256 characters.
*/
@SerializedName("Comment")
@Expose
private String Comment;
/**
* Title candidate list, fill in the FileId of the video. When transcoding, the title closest to the aspect ratio of the feature film will be automatically selected (when the aspect ratio is the same, the front candidate will take precedence). Supports up to 5 candidate titles.
*/
@SerializedName("HeadCandidateSet")
@Expose
private String [] HeadCandidateSet;
/**
* Ending candidate list, fill in the FileId of the video. When transcoding, the ending with the aspect ratio closest to the feature film will be automatically selected (when the aspect ratio is the same, the front candidate will take precedence). Supports up to 5 candidate endings.
*/
@SerializedName("TailCandidateSet")
@Expose
private String [] TailCandidateSet;
/**
* Padding method. When the video stream configuration width and height parameters are inconsistent with the aspect ratio of the original video, the transcoding processing method is "padding". Optional filling method:
stretch: stretch, stretch each frame to fill the entire screen, which may cause the transcoded video to be "squashed" or "stretched";
gauss: Gaussian blur, keep the video aspect ratio unchanged, use Gaussian blur for the remaining edges;
white: leave blank, keep the video aspect ratio unchanged, use the remaining edges for the edge Use white filling;
black: Leave black, keep the video aspect ratio unchanged, and fill the remaining edges with black.
Default value: stretch.
*/
@SerializedName("FillType")
@Expose
private String FillType;
/**
* Get Template name, length limit is 64 characters.
* @return Name Template name, length limit is 64 characters.
*/
public String getName() {
return this.Name;
}
/**
* Set Template name, length limit is 64 characters.
* @param Name Template name, length limit is 64 characters.
*/
public void setName(String Name) {
this.Name = Name;
}
/**
* Get The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
* @return SubAppId The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
*/
public Long getSubAppId() {
return this.SubAppId;
}
/**
* Set The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
* @param SubAppId The VOD [application](https://intl.cloud.tencent.com/document/product/266/14574) ID. For customers who activate VOD service from December 25, 2023, if they want to access resources in a VOD application (whether it's the default application or a newly created one), they must fill in this field with the application ID.
*/
public void setSubAppId(Long SubAppId) {
this.SubAppId = SubAppId;
}
/**
* Get Template description information, length limit is 256 characters.
* @return Comment Template description information, length limit is 256 characters.
*/
public String getComment() {
return this.Comment;
}
/**
* Set Template description information, length limit is 256 characters.
* @param Comment Template description information, length limit is 256 characters.
*/
public void setComment(String Comment) {
this.Comment = Comment;
}
/**
* Get Title candidate list, fill in the FileId of the video. When transcoding, the title closest to the aspect ratio of the feature film will be automatically selected (when the aspect ratio is the same, the front candidate will take precedence). Supports up to 5 candidate titles.
* @return HeadCandidateSet Title candidate list, fill in the FileId of the video. When transcoding, the title closest to the aspect ratio of the feature film will be automatically selected (when the aspect ratio is the same, the front candidate will take precedence). Supports up to 5 candidate titles.
*/
public String [] getHeadCandidateSet() {
return this.HeadCandidateSet;
}
/**
* Set Title candidate list, fill in the FileId of the video. When transcoding, the title closest to the aspect ratio of the feature film will be automatically selected (when the aspect ratio is the same, the front candidate will take precedence). Supports up to 5 candidate titles.
* @param HeadCandidateSet Title candidate list, fill in the FileId of the video. When transcoding, the title closest to the aspect ratio of the feature film will be automatically selected (when the aspect ratio is the same, the front candidate will take precedence). Supports up to 5 candidate titles.
*/
public void setHeadCandidateSet(String [] HeadCandidateSet) {
this.HeadCandidateSet = HeadCandidateSet;
}
/**
* Get Ending candidate list, fill in the FileId of the video. When transcoding, the ending with the aspect ratio closest to the feature film will be automatically selected (when the aspect ratio is the same, the front candidate will take precedence). Supports up to 5 candidate endings.
* @return TailCandidateSet Ending candidate list, fill in the FileId of the video. When transcoding, the ending with the aspect ratio closest to the feature film will be automatically selected (when the aspect ratio is the same, the front candidate will take precedence). Supports up to 5 candidate endings.
*/
public String [] getTailCandidateSet() {
return this.TailCandidateSet;
}
/**
* Set Ending candidate list, fill in the FileId of the video. When transcoding, the ending with the aspect ratio closest to the feature film will be automatically selected (when the aspect ratio is the same, the front candidate will take precedence). Supports up to 5 candidate endings.
* @param TailCandidateSet Ending candidate list, fill in the FileId of the video. When transcoding, the ending with the aspect ratio closest to the feature film will be automatically selected (when the aspect ratio is the same, the front candidate will take precedence). Supports up to 5 candidate endings.
*/
public void setTailCandidateSet(String [] TailCandidateSet) {
this.TailCandidateSet = TailCandidateSet;
}
/**
* Get Padding method. When the video stream configuration width and height parameters are inconsistent with the aspect ratio of the original video, the transcoding processing method is "padding". Optional filling method:
stretch: stretch, stretch each frame to fill the entire screen, which may cause the transcoded video to be "squashed" or "stretched";
gauss: Gaussian blur, keep the video aspect ratio unchanged, use Gaussian blur for the remaining edges;
white: leave blank, keep the video aspect ratio unchanged, use the remaining edges for the edge Use white filling;
black: Leave black, keep the video aspect ratio unchanged, and fill the remaining edges with black.
Default value: stretch.
* @return FillType Padding method. When the video stream configuration width and height parameters are inconsistent with the aspect ratio of the original video, the transcoding processing method is "padding". Optional filling method:
stretch: stretch, stretch each frame to fill the entire screen, which may cause the transcoded video to be "squashed" or "stretched";
gauss: Gaussian blur, keep the video aspect ratio unchanged, use Gaussian blur for the remaining edges;
white: leave blank, keep the video aspect ratio unchanged, use the remaining edges for the edge Use white filling;
black: Leave black, keep the video aspect ratio unchanged, and fill the remaining edges with black.
Default value: stretch.
*/
public String getFillType() {
return this.FillType;
}
/**
* Set Padding method. When the video stream configuration width and height parameters are inconsistent with the aspect ratio of the original video, the transcoding processing method is "padding". Optional filling method:
stretch: stretch, stretch each frame to fill the entire screen, which may cause the transcoded video to be "squashed" or "stretched";
gauss: Gaussian blur, keep the video aspect ratio unchanged, use Gaussian blur for the remaining edges;
white: leave blank, keep the video aspect ratio unchanged, use the remaining edges for the edge Use white filling;
black: Leave black, keep the video aspect ratio unchanged, and fill the remaining edges with black.
Default value: stretch.
* @param FillType Padding method. When the video stream configuration width and height parameters are inconsistent with the aspect ratio of the original video, the transcoding processing method is "padding". Optional filling method:
stretch: stretch, stretch each frame to fill the entire screen, which may cause the transcoded video to be "squashed" or "stretched";
gauss: Gaussian blur, keep the video aspect ratio unchanged, use Gaussian blur for the remaining edges;
white: leave blank, keep the video aspect ratio unchanged, use the remaining edges for the edge Use white filling;
black: Leave black, keep the video aspect ratio unchanged, and fill the remaining edges with black.
Default value: stretch.
*/
public void setFillType(String FillType) {
this.FillType = FillType;
}
public CreateHeadTailTemplateRequest() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public CreateHeadTailTemplateRequest(CreateHeadTailTemplateRequest source) {
if (source.Name != null) {
this.Name = new String(source.Name);
}
if (source.SubAppId != null) {
this.SubAppId = new Long(source.SubAppId);
}
if (source.Comment != null) {
this.Comment = new String(source.Comment);
}
if (source.HeadCandidateSet != null) {
this.HeadCandidateSet = new String[source.HeadCandidateSet.length];
for (int i = 0; i < source.HeadCandidateSet.length; i++) {
this.HeadCandidateSet[i] = new String(source.HeadCandidateSet[i]);
}
}
if (source.TailCandidateSet != null) {
this.TailCandidateSet = new String[source.TailCandidateSet.length];
for (int i = 0; i < source.TailCandidateSet.length; i++) {
this.TailCandidateSet[i] = new String(source.TailCandidateSet[i]);
}
}
if (source.FillType != null) {
this.FillType = new String(source.FillType);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "Name", this.Name);
this.setParamSimple(map, prefix + "SubAppId", this.SubAppId);
this.setParamSimple(map, prefix + "Comment", this.Comment);
this.setParamArraySimple(map, prefix + "HeadCandidateSet.", this.HeadCandidateSet);
this.setParamArraySimple(map, prefix + "TailCandidateSet.", this.TailCandidateSet);
this.setParamSimple(map, prefix + "FillType", this.FillType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy