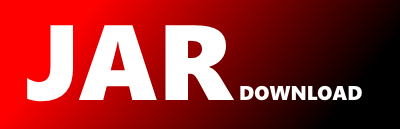
com.tencentcloudapi.apigateway.v20180808.ApigatewayClient Maven / Gradle / Ivy
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.apigateway.v20180808;
import java.lang.reflect.Type;
import com.google.gson.JsonSyntaxException;
import com.google.gson.reflect.TypeToken;
import com.tencentcloudapi.common.exception.TencentCloudSDKException;
import com.tencentcloudapi.common.AbstractClient;
import com.tencentcloudapi.common.profile.ClientProfile;
import com.tencentcloudapi.common.JsonResponseModel;
import com.tencentcloudapi.common.Credential;
import com.tencentcloudapi.apigateway.v20180808.models.*;
public class ApigatewayClient extends AbstractClient{
private static String endpoint = "apigateway.tencentcloudapi.com";
private static String service = "apigateway";
private static String version = "2018-08-08";
public ApigatewayClient(Credential credential, String region) {
this(credential, region, new ClientProfile());
}
public ApigatewayClient(Credential credential, String region, ClientProfile profile) {
super(ApigatewayClient.endpoint, ApigatewayClient.version, credential, region, profile);
}
/**
*This API is used to bind a usage plan to a service or API.
After you publish a service to an environment, if the API requires authentication and can be called only when it is bound to a usage plan, you can use this API to bind a usage plan to the specified environment.
Currently, a usage plan can be bound to an API; however, under the same service, usage plans bound to a service and usage plans bound to an API cannot coexist. Therefore, in an environment to which a service-level usage plan has already been bound, please use the `DemoteServiceUsagePlan` API to degrade it.
* @param req BindEnvironmentRequest
* @return BindEnvironmentResponse
* @throws TencentCloudSDKException
*/
public BindEnvironmentResponse BindEnvironment(BindEnvironmentRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "BindEnvironment");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to bind an IP policy to an API.
* @param req BindIPStrategyRequest
* @return BindIPStrategyResponse
* @throws TencentCloudSDKException
*/
public BindIPStrategyResponse BindIPStrategy(BindIPStrategyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "BindIPStrategy");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to bind a key to a usage plan.
You can bind a key to a usage plan and bind the usage plan to an environment where a service is published, so that callers can use the key to call APIs in the service. You can use this API to bind a key to a usage plan.
* @param req BindSecretIdsRequest
* @return BindSecretIdsResponse
* @throws TencentCloudSDKException
*/
public BindSecretIdsResponse BindSecretIds(BindSecretIdsRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "BindSecretIds");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to bind a custom domain name to a service.
Each service in API Gateway provides a default domain name for users to call. If you want to use your own domain name, you can bind a custom domain name to the target service. After getting the ICP filing and configuring the CNAME record between the custom and default domain names, you can directly call the custom domain name.
* @param req BindSubDomainRequest
* @return BindSubDomainResponse
* @throws TencentCloudSDKException
*/
public BindSubDomainResponse BindSubDomain(BindSubDomainRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "BindSubDomain");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to build an API document.
* @param req BuildAPIDocRequest
* @return BuildAPIDocResponse
* @throws TencentCloudSDKException
*/
public BuildAPIDocResponse BuildAPIDoc(BuildAPIDocRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "BuildAPIDoc");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to create an API document.
* @param req CreateAPIDocRequest
* @return CreateAPIDocResponse
* @throws TencentCloudSDKException
*/
public CreateAPIDocResponse CreateAPIDoc(CreateAPIDocRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "CreateAPIDoc");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to create an API. Before creating an API, you need to create a service, as each API belongs to a certain service.
* @param req CreateApiRequest
* @return CreateApiResponse
* @throws TencentCloudSDKException
*/
public CreateApiResponse CreateApi(CreateApiRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "CreateApi");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to create an API key pair.
* @param req CreateApiKeyRequest
* @return CreateApiKeyResponse
* @throws TencentCloudSDKException
*/
public CreateApiKeyResponse CreateApiKey(CreateApiKeyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "CreateApiKey");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to create a service IP policy.
* @param req CreateIPStrategyRequest
* @return CreateIPStrategyResponse
* @throws TencentCloudSDKException
*/
public CreateIPStrategyResponse CreateIPStrategy(CreateIPStrategyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "CreateIPStrategy");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to create a service.
The maximum unit in API Gateway is service. Multiple APIs can be created in one service, and each service has a default domain name for users to call. You can also bind your own custom domain name to a service.
* @param req CreateServiceRequest
* @return CreateServiceResponse
* @throws TencentCloudSDKException
*/
public CreateServiceResponse CreateService(CreateServiceRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "CreateService");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to create a usage plan.
To use API Gateway, you need to create a usage plan and bind it to a service environment.
* @param req CreateUsagePlanRequest
* @return CreateUsagePlanResponse
* @throws TencentCloudSDKException
*/
public CreateUsagePlanResponse CreateUsagePlan(CreateUsagePlanRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "CreateUsagePlan");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to delete an API document.
* @param req DeleteAPIDocRequest
* @return DeleteAPIDocResponse
* @throws TencentCloudSDKException
*/
public DeleteAPIDocResponse DeleteAPIDoc(DeleteAPIDocRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DeleteAPIDoc");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to delete a created API.
* @param req DeleteApiRequest
* @return DeleteApiResponse
* @throws TencentCloudSDKException
*/
public DeleteApiResponse DeleteApi(DeleteApiRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DeleteApi");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to delete an API key pair.
* @param req DeleteApiKeyRequest
* @return DeleteApiKeyResponse
* @throws TencentCloudSDKException
*/
public DeleteApiKeyResponse DeleteApiKey(DeleteApiKeyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DeleteApiKey");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to delete a service IP policy.
* @param req DeleteIPStrategyRequest
* @return DeleteIPStrategyResponse
* @throws TencentCloudSDKException
*/
public DeleteIPStrategyResponse DeleteIPStrategy(DeleteIPStrategyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DeleteIPStrategy");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to delete a service in API Gateway.
* @param req DeleteServiceRequest
* @return DeleteServiceResponse
* @throws TencentCloudSDKException
*/
public DeleteServiceResponse DeleteService(DeleteServiceRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DeleteService");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to delete a custom domain name mapping in a service environment.
You can use this API if you use a custom domain name and custom mapping. Please note that if you delete all mappings in all environments, a failure will be returned when this API is called.
* @param req DeleteServiceSubDomainMappingRequest
* @return DeleteServiceSubDomainMappingResponse
* @throws TencentCloudSDKException
*/
public DeleteServiceSubDomainMappingResponse DeleteServiceSubDomainMapping(DeleteServiceSubDomainMappingRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DeleteServiceSubDomainMapping");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to delete a usage plan.
* @param req DeleteUsagePlanRequest
* @return DeleteUsagePlanResponse
* @throws TencentCloudSDKException
*/
public DeleteUsagePlanResponse DeleteUsagePlan(DeleteUsagePlanRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DeleteUsagePlan");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to degrade a usage plan of a service in an environment to the API level.
This operation will be denied if there are no APIs under the service.
This operation will also be denied if the current environment has not been published.
* @param req DemoteServiceUsagePlanRequest
* @return DemoteServiceUsagePlanResponse
* @throws TencentCloudSDKException
*/
public DemoteServiceUsagePlanResponse DemoteServiceUsagePlan(DemoteServiceUsagePlanRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DemoteServiceUsagePlan");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the details of an API document.
* @param req DescribeAPIDocDetailRequest
* @return DescribeAPIDocDetailResponse
* @throws TencentCloudSDKException
*/
public DescribeAPIDocDetailResponse DescribeAPIDocDetail(DescribeAPIDocDetailRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeAPIDocDetail");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the list of API documents.
* @param req DescribeAPIDocsRequest
* @return DescribeAPIDocsResponse
* @throws TencentCloudSDKException
*/
public DescribeAPIDocsResponse DescribeAPIDocs(DescribeAPIDocsRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeAPIDocs");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API (`DescribeApi`) is used to query the details of the APIs users manage via Tencent Cloud API Gateway.
* @param req DescribeApiRequest
* @return DescribeApiResponse
* @throws TencentCloudSDKException
*/
public DescribeApiResponse DescribeApi(DescribeApiRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeApi");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to display the throttling policies bound to an API.
* @param req DescribeApiEnvironmentStrategyRequest
* @return DescribeApiEnvironmentStrategyResponse
* @throws TencentCloudSDKException
*/
public DescribeApiEnvironmentStrategyResponse DescribeApiEnvironmentStrategy(DescribeApiEnvironmentStrategyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeApiEnvironmentStrategy");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the details of a key.
After creating an API key, you can query its details by using this API.
* @param req DescribeApiKeyRequest
* @return DescribeApiKeyResponse
* @throws TencentCloudSDKException
*/
public DescribeApiKeyResponse DescribeApiKey(DescribeApiKeyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeApiKey");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the list of keys.
If you have created multiple API key pairs, you can use this API to query the information of one or more keys. This API does not display the `secretKey`.
* @param req DescribeApiKeysStatusRequest
* @return DescribeApiKeysStatusResponse
* @throws TencentCloudSDKException
*/
public DescribeApiKeysStatusResponse DescribeApiKeysStatus(DescribeApiKeysStatusRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeApiKeysStatus");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the details of API usage plans in a service.
To make authentication and throttling for a service take effect, you need to bind a usage plan to it. This API is used to query all usage plans bound to a service and APIs under it.
* @param req DescribeApiUsagePlanRequest
* @return DescribeApiUsagePlanResponse
* @throws TencentCloudSDKException
*/
public DescribeApiUsagePlanResponse DescribeApiUsagePlan(DescribeApiUsagePlanRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeApiUsagePlan");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to view a certain API or the list of all APIs under a service and relevant information.
* @param req DescribeApisStatusRequest
* @return DescribeApisStatusResponse
* @throws TencentCloudSDKException
*/
public DescribeApisStatusResponse DescribeApisStatus(DescribeApisStatusRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeApisStatus");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query IP policy details.
* @param req DescribeIPStrategyRequest
* @return DescribeIPStrategyResponse
* @throws TencentCloudSDKException
*/
public DescribeIPStrategyResponse DescribeIPStrategy(DescribeIPStrategyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeIPStrategy");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the list of APIs to which an IP policy can be bound, i.e., the difference set between all APIs under the service and the APIs already bound to the policy.
* @param req DescribeIPStrategyApisStatusRequest
* @return DescribeIPStrategyApisStatusResponse
* @throws TencentCloudSDKException
*/
public DescribeIPStrategyApisStatusResponse DescribeIPStrategyApisStatus(DescribeIPStrategyApisStatusRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeIPStrategyApisStatus");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the list of service IP policies.
* @param req DescribeIPStrategysStatusRequest
* @return DescribeIPStrategysStatusResponse
* @throws TencentCloudSDKException
*/
public DescribeIPStrategysStatusResponse DescribeIPStrategysStatus(DescribeIPStrategysStatusRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeIPStrategysStatus");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to search for logs.
* @param req DescribeLogSearchRequest
* @return DescribeLogSearchResponse
* @throws TencentCloudSDKException
*/
public DescribeLogSearchResponse DescribeLogSearch(DescribeLogSearchRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeLogSearch");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the details of a service, such as its description, domain name, protocol, creation time, and releases.
* @param req DescribeServiceRequest
* @return DescribeServiceResponse
* @throws TencentCloudSDKException
*/
public DescribeServiceResponse DescribeService(DescribeServiceRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeService");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the list of environments under a service. All environments and their statuses under the queried service will be returned.
* @param req DescribeServiceEnvironmentListRequest
* @return DescribeServiceEnvironmentListResponse
* @throws TencentCloudSDKException
*/
public DescribeServiceEnvironmentListResponse DescribeServiceEnvironmentList(DescribeServiceEnvironmentListRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeServiceEnvironmentList");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the release history in a service environment.
A service can only be used when it is published to an environment after creation. This API is used to query the release history in an environment under a service.
* @param req DescribeServiceEnvironmentReleaseHistoryRequest
* @return DescribeServiceEnvironmentReleaseHistoryResponse
* @throws TencentCloudSDKException
*/
public DescribeServiceEnvironmentReleaseHistoryResponse DescribeServiceEnvironmentReleaseHistory(DescribeServiceEnvironmentReleaseHistoryRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeServiceEnvironmentReleaseHistory");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to display a service throttling policy.
* @param req DescribeServiceEnvironmentStrategyRequest
* @return DescribeServiceEnvironmentStrategyResponse
* @throws TencentCloudSDKException
*/
public DescribeServiceEnvironmentStrategyResponse DescribeServiceEnvironmentStrategy(DescribeServiceEnvironmentStrategyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeServiceEnvironmentStrategy");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the list of all published versions under a service.
A service is generally published on several versions. This API can be used to query the published versions.
* @param req DescribeServiceReleaseVersionRequest
* @return DescribeServiceReleaseVersionResponse
* @throws TencentCloudSDKException
*/
public DescribeServiceReleaseVersionResponse DescribeServiceReleaseVersion(DescribeServiceReleaseVersionRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeServiceReleaseVersion");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the path mappings of a custom domain name.
In API Gateway, you can bind a custom domain name to a service and map its paths. You can customize different path mappings to up to 3 environments under the service. This API is used to query the list of path mappings of a custom domain name bound to a service.
* @param req DescribeServiceSubDomainMappingsRequest
* @return DescribeServiceSubDomainMappingsResponse
* @throws TencentCloudSDKException
*/
public DescribeServiceSubDomainMappingsResponse DescribeServiceSubDomainMappings(DescribeServiceSubDomainMappingsRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeServiceSubDomainMappings");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the list of custom domain names.
In API Gateway, you can bind custom domain names to a service for service call. This API is used to query the list of custom domain names bound to a service.
* @param req DescribeServiceSubDomainsRequest
* @return DescribeServiceSubDomainsResponse
* @throws TencentCloudSDKException
*/
public DescribeServiceSubDomainsResponse DescribeServiceSubDomains(DescribeServiceSubDomainsRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeServiceSubDomains");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the details of usage plans in a service.
To make authentication and throttling for a service take effect, you need to bind a usage plan to it. This API is used to query all usage plans bound to a service.
* @param req DescribeServiceUsagePlanRequest
* @return DescribeServiceUsagePlanResponse
* @throws TencentCloudSDKException
*/
public DescribeServiceUsagePlanResponse DescribeServiceUsagePlan(DescribeServiceUsagePlanRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeServiceUsagePlan");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the list of one or more services and return relevant domain name, time, and other information.
* @param req DescribeServicesStatusRequest
* @return DescribeServicesStatusResponse
* @throws TencentCloudSDKException
*/
public DescribeServicesStatusResponse DescribeServicesStatus(DescribeServicesStatusRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeServicesStatus");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the details of a usage plan, such as its name, QPS, creation time, and bound environment.
* @param req DescribeUsagePlanRequest
* @return DescribeUsagePlanResponse
* @throws TencentCloudSDKException
*/
public DescribeUsagePlanResponse DescribeUsagePlan(DescribeUsagePlanRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeUsagePlan");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the list of environments bound to a usage plan.
After binding a usage plan to environments, you can use this API to query all service environments bound to the usage plan.
* @param req DescribeUsagePlanEnvironmentsRequest
* @return DescribeUsagePlanEnvironmentsResponse
* @throws TencentCloudSDKException
*/
public DescribeUsagePlanEnvironmentsResponse DescribeUsagePlanEnvironments(DescribeUsagePlanEnvironmentsRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeUsagePlanEnvironments");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the list of keys bound to a usage plan.
In API Gateway, a usage plan can be bound to multiple key pairs. You can use this API to query the list of keys bound to it.
* @param req DescribeUsagePlanSecretIdsRequest
* @return DescribeUsagePlanSecretIdsResponse
* @throws TencentCloudSDKException
*/
public DescribeUsagePlanSecretIdsResponse DescribeUsagePlanSecretIds(DescribeUsagePlanSecretIdsRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeUsagePlanSecretIds");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query the list of usage plans.
* @param req DescribeUsagePlansStatusRequest
* @return DescribeUsagePlansStatusResponse
* @throws TencentCloudSDKException
*/
public DescribeUsagePlansStatusResponse DescribeUsagePlansStatus(DescribeUsagePlansStatusRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeUsagePlansStatus");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to disable an API key.
* @param req DisableApiKeyRequest
* @return DisableApiKeyResponse
* @throws TencentCloudSDKException
*/
public DisableApiKeyResponse DisableApiKey(DisableApiKeyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DisableApiKey");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to enable a disabled API key.
* @param req EnableApiKeyRequest
* @return EnableApiKeyResponse
* @throws TencentCloudSDKException
*/
public EnableApiKeyResponse EnableApiKey(EnableApiKeyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "EnableApiKey");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to automatically generate API documents and SDKs. One document and one SDK will be generated for each environment under each service, respectively.
* @param req GenerateApiDocumentRequest
* @return GenerateApiDocumentResponse
* @throws TencentCloudSDKException
*/
public GenerateApiDocumentResponse GenerateApiDocument(GenerateApiDocumentRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "GenerateApiDocument");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to modify an API document.
* @param req ModifyAPIDocRequest
* @return ModifyAPIDocResponse
* @throws TencentCloudSDKException
*/
public ModifyAPIDocResponse ModifyAPIDoc(ModifyAPIDocRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ModifyAPIDoc");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to modify an API. You can call this API to edit/modify a configured API. The modified API takes effect only after its service is published to the corresponding environment again.
* @param req ModifyApiRequest
* @return ModifyApiResponse
* @throws TencentCloudSDKException
*/
public ModifyApiResponse ModifyApi(ModifyApiRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ModifyApi");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to modify an API throttling policy.
* @param req ModifyApiEnvironmentStrategyRequest
* @return ModifyApiEnvironmentStrategyResponse
* @throws TencentCloudSDKException
*/
public ModifyApiEnvironmentStrategyResponse ModifyApiEnvironmentStrategy(ModifyApiEnvironmentStrategyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ModifyApiEnvironmentStrategy");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to incrementally update an API and mainly called by programs (different from `ModifyApi`, which requires that full API parameters be passed in and is suitable for use in the console).
* @param req ModifyApiIncrementRequest
* @return ModifyApiIncrementResponse
* @throws TencentCloudSDKException
*/
public ModifyApiIncrementResponse ModifyApiIncrement(ModifyApiIncrementRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ModifyApiIncrement");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to modify a service IP policy.
* @param req ModifyIPStrategyRequest
* @return ModifyIPStrategyResponse
* @throws TencentCloudSDKException
*/
public ModifyIPStrategyResponse ModifyIPStrategy(ModifyIPStrategyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ModifyIPStrategy");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to modify the relevant information of a service. After a service is created, its name, description, and service type can be modified.
* @param req ModifyServiceRequest
* @return ModifyServiceResponse
* @throws TencentCloudSDKException
*/
public ModifyServiceResponse ModifyService(ModifyServiceRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ModifyService");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to modify a service throttling policy.
* @param req ModifyServiceEnvironmentStrategyRequest
* @return ModifyServiceEnvironmentStrategyResponse
* @throws TencentCloudSDKException
*/
public ModifyServiceEnvironmentStrategyResponse ModifyServiceEnvironmentStrategy(ModifyServiceEnvironmentStrategyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ModifyServiceEnvironmentStrategy");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to modify the path mapping in the custom domain name settings of a service. The path mapping rule can be modified before it is bound to the custom domain name.
* @param req ModifySubDomainRequest
* @return ModifySubDomainResponse
* @throws TencentCloudSDKException
*/
public ModifySubDomainResponse ModifySubDomain(ModifySubDomainRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ModifySubDomain");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to modify the name, description, and QPS of a usage plan.
* @param req ModifyUsagePlanRequest
* @return ModifyUsagePlanResponse
* @throws TencentCloudSDKException
*/
public ModifyUsagePlanResponse ModifyUsagePlan(ModifyUsagePlanRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ModifyUsagePlan");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to publish a service.
An API Gateway service can only be called when it is published to an environment and takes effect after creation. This API is used to publish a service to an environment such as the `release` environment.
* @param req ReleaseServiceRequest
* @return ReleaseServiceResponse
* @throws TencentCloudSDKException
*/
public ReleaseServiceResponse ReleaseService(ReleaseServiceRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ReleaseService");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to reset the password of an API document.
* @param req ResetAPIDocPasswordRequest
* @return ResetAPIDocPasswordResponse
* @throws TencentCloudSDKException
*/
public ResetAPIDocPasswordResponse ResetAPIDocPassword(ResetAPIDocPasswordRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ResetAPIDocPassword");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to unbind a usage plan from a specified environment.
* @param req UnBindEnvironmentRequest
* @return UnBindEnvironmentResponse
* @throws TencentCloudSDKException
*/
public UnBindEnvironmentResponse UnBindEnvironment(UnBindEnvironmentRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "UnBindEnvironment");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to unbind an IP policy from a service.
* @param req UnBindIPStrategyRequest
* @return UnBindIPStrategyResponse
* @throws TencentCloudSDKException
*/
public UnBindIPStrategyResponse UnBindIPStrategy(UnBindIPStrategyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "UnBindIPStrategy");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to unbind a key from a usage plan.
* @param req UnBindSecretIdsRequest
* @return UnBindSecretIdsResponse
* @throws TencentCloudSDKException
*/
public UnBindSecretIdsResponse UnBindSecretIds(UnBindSecretIdsRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "UnBindSecretIds");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to unbind a custom domain name.
After binding a custom domain name to a service by using API Gateway, you can use this API to unbind it.
* @param req UnBindSubDomainRequest
* @return UnBindSubDomainResponse
* @throws TencentCloudSDKException
*/
public UnBindSubDomainResponse UnBindSubDomain(UnBindSubDomainRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "UnBindSubDomain");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to deactivate a service.
Only after a service is published to an environment can its APIs be called. You can call this API to deactivate a service in the release environment. Once deactivated, the service cannot be called.
* @param req UnReleaseServiceRequest
* @return UnReleaseServiceResponse
* @throws TencentCloudSDKException
*/
public UnReleaseServiceResponse UnReleaseService(UnReleaseServiceRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "UnReleaseService");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to update a created API key pair.
* @param req UpdateApiKeyRequest
* @return UpdateApiKeyResponse
* @throws TencentCloudSDKException
*/
public UpdateApiKeyResponse UpdateApiKey(UpdateApiKeyRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "UpdateApiKey");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to switch the running version of a service published in an environment to a specified version. After you create a service by using API Gateway and publish it to an environment, multiple versions will be generated during development. In this case, you can call this API to switch versions.
* @param req UpdateServiceRequest
* @return UpdateServiceResponse
* @throws TencentCloudSDKException
*/
public UpdateServiceResponse UpdateService(UpdateServiceRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "UpdateService");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy