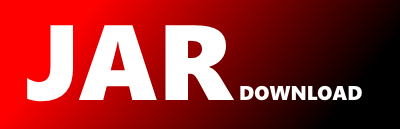
com.tencentcloudapi.as.v20180419.models.ServiceSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.as.v20180419.models;
import com.tencentcloudapi.common.AbstractModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class ServiceSettings extends AbstractModel{
/**
* Enables unhealthy instance replacement. If this feature is enabled, AS will replace instances that are flagged as unhealthy by Cloud Monitor. If this parameter is not specified, the value will be False by default.
*/
@SerializedName("ReplaceMonitorUnhealthy")
@Expose
private Boolean ReplaceMonitorUnhealthy;
/**
* Valid values:
CLASSIC_SCALING: this is the typical scaling method, which creates and terminates instances to perform scaling operations.
WAKE_UP_STOPPED_SCALING: this scaling method first tries to start stopped instances. If the number of instances woken up is insufficient, the system creates new instances for scale-out. For scale-in, instances are terminated as in the typical method. You can use the StopAutoScalingInstances API to stop instances in the scaling group. Scale-out operations triggered by alarms will still create new instances.
Default value: CLASSIC_SCALING
*/
@SerializedName("ScalingMode")
@Expose
private String ScalingMode;
/**
* Get Enables unhealthy instance replacement. If this feature is enabled, AS will replace instances that are flagged as unhealthy by Cloud Monitor. If this parameter is not specified, the value will be False by default.
* @return ReplaceMonitorUnhealthy Enables unhealthy instance replacement. If this feature is enabled, AS will replace instances that are flagged as unhealthy by Cloud Monitor. If this parameter is not specified, the value will be False by default.
*/
public Boolean getReplaceMonitorUnhealthy() {
return this.ReplaceMonitorUnhealthy;
}
/**
* Set Enables unhealthy instance replacement. If this feature is enabled, AS will replace instances that are flagged as unhealthy by Cloud Monitor. If this parameter is not specified, the value will be False by default.
* @param ReplaceMonitorUnhealthy Enables unhealthy instance replacement. If this feature is enabled, AS will replace instances that are flagged as unhealthy by Cloud Monitor. If this parameter is not specified, the value will be False by default.
*/
public void setReplaceMonitorUnhealthy(Boolean ReplaceMonitorUnhealthy) {
this.ReplaceMonitorUnhealthy = ReplaceMonitorUnhealthy;
}
/**
* Get Valid values:
CLASSIC_SCALING: this is the typical scaling method, which creates and terminates instances to perform scaling operations.
WAKE_UP_STOPPED_SCALING: this scaling method first tries to start stopped instances. If the number of instances woken up is insufficient, the system creates new instances for scale-out. For scale-in, instances are terminated as in the typical method. You can use the StopAutoScalingInstances API to stop instances in the scaling group. Scale-out operations triggered by alarms will still create new instances.
Default value: CLASSIC_SCALING
* @return ScalingMode Valid values:
CLASSIC_SCALING: this is the typical scaling method, which creates and terminates instances to perform scaling operations.
WAKE_UP_STOPPED_SCALING: this scaling method first tries to start stopped instances. If the number of instances woken up is insufficient, the system creates new instances for scale-out. For scale-in, instances are terminated as in the typical method. You can use the StopAutoScalingInstances API to stop instances in the scaling group. Scale-out operations triggered by alarms will still create new instances.
Default value: CLASSIC_SCALING
*/
public String getScalingMode() {
return this.ScalingMode;
}
/**
* Set Valid values:
CLASSIC_SCALING: this is the typical scaling method, which creates and terminates instances to perform scaling operations.
WAKE_UP_STOPPED_SCALING: this scaling method first tries to start stopped instances. If the number of instances woken up is insufficient, the system creates new instances for scale-out. For scale-in, instances are terminated as in the typical method. You can use the StopAutoScalingInstances API to stop instances in the scaling group. Scale-out operations triggered by alarms will still create new instances.
Default value: CLASSIC_SCALING
* @param ScalingMode Valid values:
CLASSIC_SCALING: this is the typical scaling method, which creates and terminates instances to perform scaling operations.
WAKE_UP_STOPPED_SCALING: this scaling method first tries to start stopped instances. If the number of instances woken up is insufficient, the system creates new instances for scale-out. For scale-in, instances are terminated as in the typical method. You can use the StopAutoScalingInstances API to stop instances in the scaling group. Scale-out operations triggered by alarms will still create new instances.
Default value: CLASSIC_SCALING
*/
public void setScalingMode(String ScalingMode) {
this.ScalingMode = ScalingMode;
}
public ServiceSettings() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public ServiceSettings(ServiceSettings source) {
if (source.ReplaceMonitorUnhealthy != null) {
this.ReplaceMonitorUnhealthy = new Boolean(source.ReplaceMonitorUnhealthy);
}
if (source.ScalingMode != null) {
this.ScalingMode = new String(source.ScalingMode);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "ReplaceMonitorUnhealthy", this.ReplaceMonitorUnhealthy);
this.setParamSimple(map, prefix + "ScalingMode", this.ScalingMode);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy