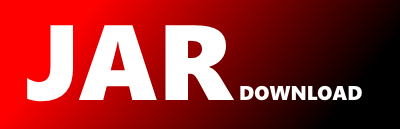
com.tencentcloudapi.cbs.v20170312.models.CreateDisksRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.cbs.v20170312.models;
import com.tencentcloudapi.common.AbstractModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class CreateDisksRequest extends AbstractModel{
/**
* Cloud disk media type. Valid values:
CLOUD_BASIC: HDD cloud disk
CLOUD_PREMIUM: Premium Cloud Storage
CLOUD_SSD: SSD
CLOUD_HSSD: Enhanced SSD
CLOUD_TSSD: Tremendous SSD
*/
@SerializedName("DiskType")
@Expose
private String DiskType;
/**
* Cloud disk billing method. POSTPAID_BY_HOUR: pay as you go by hour
CDCPAID: Billed together with the bound dedicated cluster
For information about the pricing of each method, see the cloud disk [Pricing Overview](https://intl.cloud.tencent.com/document/product/362/2413?from_cn_redirect=1).
*/
@SerializedName("DiskChargeType")
@Expose
private String DiskChargeType;
/**
* The location of the instance. The availability zone and the project that the instance belongs to can be specified using this parameter. If the project is not specified, it will be created under the default project.
*/
@SerializedName("Placement")
@Expose
private Placement Placement;
/**
* The displayed name of the cloud disk. If it is left empty, the default is 'Not named'. The maximum length cannot exceed 60 bytes.
*/
@SerializedName("DiskName")
@Expose
private String DiskName;
/**
* If the number of cloud disks to be created is left empty, the default is 1. There is a limit to the maximum number of cloud disks that can be created for a single request. For more information, please see [CBS Use Limits](https://intl.cloud.tencent.com/doc/product/362/5145?from_cn_redirect=1).
*/
@SerializedName("DiskCount")
@Expose
private Long DiskCount;
/**
* Relevant parameter settings for the prepaid mode (i.e., monthly subscription). The monthly subscription cloud disk purchase attributes such as usage period and whether or not auto-renewal is set up can be specified using this parameter.
This parameter is required when creating a prepaid cloud disk. This parameter is not required when creating an hourly postpaid cloud disk.
*/
@SerializedName("DiskChargePrepaid")
@Expose
private DiskChargePrepaid DiskChargePrepaid;
/**
* Cloud hard disk size (in GB).
If `SnapshotId` is passed, `DiskSize` cannot be passed. In this case, the size of the cloud disk is the size of the snapshot.
To pass `SnapshotId` and `DiskSize` at the same time, the size of the disk must be larger than or equal to the size of the snapshot.
For information about the size range of cloud disks, see cloud disk [Product Types](https://intl.cloud.tencent.com/document/product/362/2353?from_cn_redirect=1).
*/
@SerializedName("DiskSize")
@Expose
private Long DiskSize;
/**
* Snapshot ID. If this parameter is specified, the cloud disk is created based on the snapshot. The snapshot type must be a data disk snapshot. The snapshot can be queried in the DiskUsage field in the output parameter through the API [DescribeSnapshots](https://intl.cloud.tencent.com/document/product/362/15647?from_cn_redirect=1).
*/
@SerializedName("SnapshotId")
@Expose
private String SnapshotId;
/**
* A string to ensure the idempotency of the request, which is generated by the client. Each request shall have a unique string with a maximum of 64 ASCII characters. If this parameter is not specified, the idempotency of the request cannot be ensured.
*/
@SerializedName("ClientToken")
@Expose
private String ClientToken;
/**
* This parameter is used to create an encrypted cloud disk. Its value is always ENCRYPT.
*/
@SerializedName("Encrypt")
@Expose
private String Encrypt;
/**
* Cloud disk binding tag.
*/
@SerializedName("Tags")
@Expose
private Tag [] Tags;
/**
* The default of optional parameter is False. When True is selected, the cloud disk will be created as a shareable cloud disk.
*/
@SerializedName("Shareable")
@Expose
private Boolean Shareable;
/**
* Extra performance purchased for a cloud disk.
This optional parameter is only valid for Tremendous SSD (CLOUD_TSSD) and Enhanced SSD (CLOUD_HSSD).
*/
@SerializedName("ThroughputPerformance")
@Expose
private Long ThroughputPerformance;
/**
* Get Cloud disk media type. Valid values:
CLOUD_BASIC: HDD cloud disk
CLOUD_PREMIUM: Premium Cloud Storage
CLOUD_SSD: SSD
CLOUD_HSSD: Enhanced SSD
CLOUD_TSSD: Tremendous SSD
* @return DiskType Cloud disk media type. Valid values:
CLOUD_BASIC: HDD cloud disk
CLOUD_PREMIUM: Premium Cloud Storage
CLOUD_SSD: SSD
CLOUD_HSSD: Enhanced SSD
CLOUD_TSSD: Tremendous SSD
*/
public String getDiskType() {
return this.DiskType;
}
/**
* Set Cloud disk media type. Valid values:
CLOUD_BASIC: HDD cloud disk
CLOUD_PREMIUM: Premium Cloud Storage
CLOUD_SSD: SSD
CLOUD_HSSD: Enhanced SSD
CLOUD_TSSD: Tremendous SSD
* @param DiskType Cloud disk media type. Valid values:
CLOUD_BASIC: HDD cloud disk
CLOUD_PREMIUM: Premium Cloud Storage
CLOUD_SSD: SSD
CLOUD_HSSD: Enhanced SSD
CLOUD_TSSD: Tremendous SSD
*/
public void setDiskType(String DiskType) {
this.DiskType = DiskType;
}
/**
* Get Cloud disk billing method. POSTPAID_BY_HOUR: pay as you go by hour
CDCPAID: Billed together with the bound dedicated cluster
For information about the pricing of each method, see the cloud disk [Pricing Overview](https://intl.cloud.tencent.com/document/product/362/2413?from_cn_redirect=1).
* @return DiskChargeType Cloud disk billing method. POSTPAID_BY_HOUR: pay as you go by hour
CDCPAID: Billed together with the bound dedicated cluster
For information about the pricing of each method, see the cloud disk [Pricing Overview](https://intl.cloud.tencent.com/document/product/362/2413?from_cn_redirect=1).
*/
public String getDiskChargeType() {
return this.DiskChargeType;
}
/**
* Set Cloud disk billing method. POSTPAID_BY_HOUR: pay as you go by hour
CDCPAID: Billed together with the bound dedicated cluster
For information about the pricing of each method, see the cloud disk [Pricing Overview](https://intl.cloud.tencent.com/document/product/362/2413?from_cn_redirect=1).
* @param DiskChargeType Cloud disk billing method. POSTPAID_BY_HOUR: pay as you go by hour
CDCPAID: Billed together with the bound dedicated cluster
For information about the pricing of each method, see the cloud disk [Pricing Overview](https://intl.cloud.tencent.com/document/product/362/2413?from_cn_redirect=1).
*/
public void setDiskChargeType(String DiskChargeType) {
this.DiskChargeType = DiskChargeType;
}
/**
* Get The location of the instance. The availability zone and the project that the instance belongs to can be specified using this parameter. If the project is not specified, it will be created under the default project.
* @return Placement The location of the instance. The availability zone and the project that the instance belongs to can be specified using this parameter. If the project is not specified, it will be created under the default project.
*/
public Placement getPlacement() {
return this.Placement;
}
/**
* Set The location of the instance. The availability zone and the project that the instance belongs to can be specified using this parameter. If the project is not specified, it will be created under the default project.
* @param Placement The location of the instance. The availability zone and the project that the instance belongs to can be specified using this parameter. If the project is not specified, it will be created under the default project.
*/
public void setPlacement(Placement Placement) {
this.Placement = Placement;
}
/**
* Get The displayed name of the cloud disk. If it is left empty, the default is 'Not named'. The maximum length cannot exceed 60 bytes.
* @return DiskName The displayed name of the cloud disk. If it is left empty, the default is 'Not named'. The maximum length cannot exceed 60 bytes.
*/
public String getDiskName() {
return this.DiskName;
}
/**
* Set The displayed name of the cloud disk. If it is left empty, the default is 'Not named'. The maximum length cannot exceed 60 bytes.
* @param DiskName The displayed name of the cloud disk. If it is left empty, the default is 'Not named'. The maximum length cannot exceed 60 bytes.
*/
public void setDiskName(String DiskName) {
this.DiskName = DiskName;
}
/**
* Get If the number of cloud disks to be created is left empty, the default is 1. There is a limit to the maximum number of cloud disks that can be created for a single request. For more information, please see [CBS Use Limits](https://intl.cloud.tencent.com/doc/product/362/5145?from_cn_redirect=1).
* @return DiskCount If the number of cloud disks to be created is left empty, the default is 1. There is a limit to the maximum number of cloud disks that can be created for a single request. For more information, please see [CBS Use Limits](https://intl.cloud.tencent.com/doc/product/362/5145?from_cn_redirect=1).
*/
public Long getDiskCount() {
return this.DiskCount;
}
/**
* Set If the number of cloud disks to be created is left empty, the default is 1. There is a limit to the maximum number of cloud disks that can be created for a single request. For more information, please see [CBS Use Limits](https://intl.cloud.tencent.com/doc/product/362/5145?from_cn_redirect=1).
* @param DiskCount If the number of cloud disks to be created is left empty, the default is 1. There is a limit to the maximum number of cloud disks that can be created for a single request. For more information, please see [CBS Use Limits](https://intl.cloud.tencent.com/doc/product/362/5145?from_cn_redirect=1).
*/
public void setDiskCount(Long DiskCount) {
this.DiskCount = DiskCount;
}
/**
* Get Relevant parameter settings for the prepaid mode (i.e., monthly subscription). The monthly subscription cloud disk purchase attributes such as usage period and whether or not auto-renewal is set up can be specified using this parameter.
This parameter is required when creating a prepaid cloud disk. This parameter is not required when creating an hourly postpaid cloud disk.
* @return DiskChargePrepaid Relevant parameter settings for the prepaid mode (i.e., monthly subscription). The monthly subscription cloud disk purchase attributes such as usage period and whether or not auto-renewal is set up can be specified using this parameter.
This parameter is required when creating a prepaid cloud disk. This parameter is not required when creating an hourly postpaid cloud disk.
*/
public DiskChargePrepaid getDiskChargePrepaid() {
return this.DiskChargePrepaid;
}
/**
* Set Relevant parameter settings for the prepaid mode (i.e., monthly subscription). The monthly subscription cloud disk purchase attributes such as usage period and whether or not auto-renewal is set up can be specified using this parameter.
This parameter is required when creating a prepaid cloud disk. This parameter is not required when creating an hourly postpaid cloud disk.
* @param DiskChargePrepaid Relevant parameter settings for the prepaid mode (i.e., monthly subscription). The monthly subscription cloud disk purchase attributes such as usage period and whether or not auto-renewal is set up can be specified using this parameter.
This parameter is required when creating a prepaid cloud disk. This parameter is not required when creating an hourly postpaid cloud disk.
*/
public void setDiskChargePrepaid(DiskChargePrepaid DiskChargePrepaid) {
this.DiskChargePrepaid = DiskChargePrepaid;
}
/**
* Get Cloud hard disk size (in GB).
If `SnapshotId` is passed, `DiskSize` cannot be passed. In this case, the size of the cloud disk is the size of the snapshot.
To pass `SnapshotId` and `DiskSize` at the same time, the size of the disk must be larger than or equal to the size of the snapshot.
For information about the size range of cloud disks, see cloud disk [Product Types](https://intl.cloud.tencent.com/document/product/362/2353?from_cn_redirect=1).
* @return DiskSize Cloud hard disk size (in GB).
If `SnapshotId` is passed, `DiskSize` cannot be passed. In this case, the size of the cloud disk is the size of the snapshot.
To pass `SnapshotId` and `DiskSize` at the same time, the size of the disk must be larger than or equal to the size of the snapshot.
For information about the size range of cloud disks, see cloud disk [Product Types](https://intl.cloud.tencent.com/document/product/362/2353?from_cn_redirect=1).
*/
public Long getDiskSize() {
return this.DiskSize;
}
/**
* Set Cloud hard disk size (in GB).
If `SnapshotId` is passed, `DiskSize` cannot be passed. In this case, the size of the cloud disk is the size of the snapshot.
To pass `SnapshotId` and `DiskSize` at the same time, the size of the disk must be larger than or equal to the size of the snapshot.
For information about the size range of cloud disks, see cloud disk [Product Types](https://intl.cloud.tencent.com/document/product/362/2353?from_cn_redirect=1).
* @param DiskSize Cloud hard disk size (in GB).
If `SnapshotId` is passed, `DiskSize` cannot be passed. In this case, the size of the cloud disk is the size of the snapshot.
To pass `SnapshotId` and `DiskSize` at the same time, the size of the disk must be larger than or equal to the size of the snapshot.
For information about the size range of cloud disks, see cloud disk [Product Types](https://intl.cloud.tencent.com/document/product/362/2353?from_cn_redirect=1).
*/
public void setDiskSize(Long DiskSize) {
this.DiskSize = DiskSize;
}
/**
* Get Snapshot ID. If this parameter is specified, the cloud disk is created based on the snapshot. The snapshot type must be a data disk snapshot. The snapshot can be queried in the DiskUsage field in the output parameter through the API [DescribeSnapshots](https://intl.cloud.tencent.com/document/product/362/15647?from_cn_redirect=1).
* @return SnapshotId Snapshot ID. If this parameter is specified, the cloud disk is created based on the snapshot. The snapshot type must be a data disk snapshot. The snapshot can be queried in the DiskUsage field in the output parameter through the API [DescribeSnapshots](https://intl.cloud.tencent.com/document/product/362/15647?from_cn_redirect=1).
*/
public String getSnapshotId() {
return this.SnapshotId;
}
/**
* Set Snapshot ID. If this parameter is specified, the cloud disk is created based on the snapshot. The snapshot type must be a data disk snapshot. The snapshot can be queried in the DiskUsage field in the output parameter through the API [DescribeSnapshots](https://intl.cloud.tencent.com/document/product/362/15647?from_cn_redirect=1).
* @param SnapshotId Snapshot ID. If this parameter is specified, the cloud disk is created based on the snapshot. The snapshot type must be a data disk snapshot. The snapshot can be queried in the DiskUsage field in the output parameter through the API [DescribeSnapshots](https://intl.cloud.tencent.com/document/product/362/15647?from_cn_redirect=1).
*/
public void setSnapshotId(String SnapshotId) {
this.SnapshotId = SnapshotId;
}
/**
* Get A string to ensure the idempotency of the request, which is generated by the client. Each request shall have a unique string with a maximum of 64 ASCII characters. If this parameter is not specified, the idempotency of the request cannot be ensured.
* @return ClientToken A string to ensure the idempotency of the request, which is generated by the client. Each request shall have a unique string with a maximum of 64 ASCII characters. If this parameter is not specified, the idempotency of the request cannot be ensured.
*/
public String getClientToken() {
return this.ClientToken;
}
/**
* Set A string to ensure the idempotency of the request, which is generated by the client. Each request shall have a unique string with a maximum of 64 ASCII characters. If this parameter is not specified, the idempotency of the request cannot be ensured.
* @param ClientToken A string to ensure the idempotency of the request, which is generated by the client. Each request shall have a unique string with a maximum of 64 ASCII characters. If this parameter is not specified, the idempotency of the request cannot be ensured.
*/
public void setClientToken(String ClientToken) {
this.ClientToken = ClientToken;
}
/**
* Get This parameter is used to create an encrypted cloud disk. Its value is always ENCRYPT.
* @return Encrypt This parameter is used to create an encrypted cloud disk. Its value is always ENCRYPT.
*/
public String getEncrypt() {
return this.Encrypt;
}
/**
* Set This parameter is used to create an encrypted cloud disk. Its value is always ENCRYPT.
* @param Encrypt This parameter is used to create an encrypted cloud disk. Its value is always ENCRYPT.
*/
public void setEncrypt(String Encrypt) {
this.Encrypt = Encrypt;
}
/**
* Get Cloud disk binding tag.
* @return Tags Cloud disk binding tag.
*/
public Tag [] getTags() {
return this.Tags;
}
/**
* Set Cloud disk binding tag.
* @param Tags Cloud disk binding tag.
*/
public void setTags(Tag [] Tags) {
this.Tags = Tags;
}
/**
* Get The default of optional parameter is False. When True is selected, the cloud disk will be created as a shareable cloud disk.
* @return Shareable The default of optional parameter is False. When True is selected, the cloud disk will be created as a shareable cloud disk.
*/
public Boolean getShareable() {
return this.Shareable;
}
/**
* Set The default of optional parameter is False. When True is selected, the cloud disk will be created as a shareable cloud disk.
* @param Shareable The default of optional parameter is False. When True is selected, the cloud disk will be created as a shareable cloud disk.
*/
public void setShareable(Boolean Shareable) {
this.Shareable = Shareable;
}
/**
* Get Extra performance purchased for a cloud disk.
This optional parameter is only valid for Tremendous SSD (CLOUD_TSSD) and Enhanced SSD (CLOUD_HSSD).
* @return ThroughputPerformance Extra performance purchased for a cloud disk.
This optional parameter is only valid for Tremendous SSD (CLOUD_TSSD) and Enhanced SSD (CLOUD_HSSD).
*/
public Long getThroughputPerformance() {
return this.ThroughputPerformance;
}
/**
* Set Extra performance purchased for a cloud disk.
This optional parameter is only valid for Tremendous SSD (CLOUD_TSSD) and Enhanced SSD (CLOUD_HSSD).
* @param ThroughputPerformance Extra performance purchased for a cloud disk.
This optional parameter is only valid for Tremendous SSD (CLOUD_TSSD) and Enhanced SSD (CLOUD_HSSD).
*/
public void setThroughputPerformance(Long ThroughputPerformance) {
this.ThroughputPerformance = ThroughputPerformance;
}
public CreateDisksRequest() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public CreateDisksRequest(CreateDisksRequest source) {
if (source.DiskType != null) {
this.DiskType = new String(source.DiskType);
}
if (source.DiskChargeType != null) {
this.DiskChargeType = new String(source.DiskChargeType);
}
if (source.Placement != null) {
this.Placement = new Placement(source.Placement);
}
if (source.DiskName != null) {
this.DiskName = new String(source.DiskName);
}
if (source.DiskCount != null) {
this.DiskCount = new Long(source.DiskCount);
}
if (source.DiskChargePrepaid != null) {
this.DiskChargePrepaid = new DiskChargePrepaid(source.DiskChargePrepaid);
}
if (source.DiskSize != null) {
this.DiskSize = new Long(source.DiskSize);
}
if (source.SnapshotId != null) {
this.SnapshotId = new String(source.SnapshotId);
}
if (source.ClientToken != null) {
this.ClientToken = new String(source.ClientToken);
}
if (source.Encrypt != null) {
this.Encrypt = new String(source.Encrypt);
}
if (source.Tags != null) {
this.Tags = new Tag[source.Tags.length];
for (int i = 0; i < source.Tags.length; i++) {
this.Tags[i] = new Tag(source.Tags[i]);
}
}
if (source.Shareable != null) {
this.Shareable = new Boolean(source.Shareable);
}
if (source.ThroughputPerformance != null) {
this.ThroughputPerformance = new Long(source.ThroughputPerformance);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "DiskType", this.DiskType);
this.setParamSimple(map, prefix + "DiskChargeType", this.DiskChargeType);
this.setParamObj(map, prefix + "Placement.", this.Placement);
this.setParamSimple(map, prefix + "DiskName", this.DiskName);
this.setParamSimple(map, prefix + "DiskCount", this.DiskCount);
this.setParamObj(map, prefix + "DiskChargePrepaid.", this.DiskChargePrepaid);
this.setParamSimple(map, prefix + "DiskSize", this.DiskSize);
this.setParamSimple(map, prefix + "SnapshotId", this.SnapshotId);
this.setParamSimple(map, prefix + "ClientToken", this.ClientToken);
this.setParamSimple(map, prefix + "Encrypt", this.Encrypt);
this.setParamArrayObj(map, prefix + "Tags.", this.Tags);
this.setParamSimple(map, prefix + "Shareable", this.Shareable);
this.setParamSimple(map, prefix + "ThroughputPerformance", this.ThroughputPerformance);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy