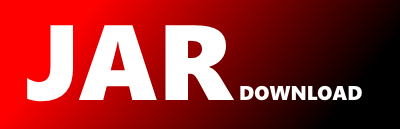
com.tencentcloudapi.gaap.v20180529.models.DestroyProxiesRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.gaap.v20180529.models;
import com.tencentcloudapi.common.AbstractModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class DestroyProxiesRequest extends AbstractModel{
/**
* The identifier for forced deletion
1: this connection list is deleted forcibly regardless of whether the origin server has been bound.
0: this connection list cannot be deleted if the origin server has been bound.
If this identifier is 0, the deletion can be performed only when all the connections have not been bound to any origin servers.
*/
@SerializedName("Force")
@Expose
private Long Force;
/**
* List of connection instance IDs; It's an old parameter, please switch to ProxyIds.
*/
@SerializedName("InstanceIds")
@Expose
private String [] InstanceIds;
/**
* A string used to ensure the idempotency of the request, which is generated by the user and must be unique to each request. The maximum length is 64 ASCII characters. If this parameter is not specified, the idempotency of the request cannot be guaranteed.
For more information, please see How to Ensure Idempotence.
*/
@SerializedName("ClientToken")
@Expose
private String ClientToken;
/**
* List of connection instance IDs; It's a new parameter.
*/
@SerializedName("ProxyIds")
@Expose
private String [] ProxyIds;
/**
* Get The identifier for forced deletion
1: this connection list is deleted forcibly regardless of whether the origin server has been bound.
0: this connection list cannot be deleted if the origin server has been bound.
If this identifier is 0, the deletion can be performed only when all the connections have not been bound to any origin servers.
* @return Force The identifier for forced deletion
1: this connection list is deleted forcibly regardless of whether the origin server has been bound.
0: this connection list cannot be deleted if the origin server has been bound.
If this identifier is 0, the deletion can be performed only when all the connections have not been bound to any origin servers.
*/
public Long getForce() {
return this.Force;
}
/**
* Set The identifier for forced deletion
1: this connection list is deleted forcibly regardless of whether the origin server has been bound.
0: this connection list cannot be deleted if the origin server has been bound.
If this identifier is 0, the deletion can be performed only when all the connections have not been bound to any origin servers.
* @param Force The identifier for forced deletion
1: this connection list is deleted forcibly regardless of whether the origin server has been bound.
0: this connection list cannot be deleted if the origin server has been bound.
If this identifier is 0, the deletion can be performed only when all the connections have not been bound to any origin servers.
*/
public void setForce(Long Force) {
this.Force = Force;
}
/**
* Get List of connection instance IDs; It's an old parameter, please switch to ProxyIds.
* @return InstanceIds List of connection instance IDs; It's an old parameter, please switch to ProxyIds.
*/
public String [] getInstanceIds() {
return this.InstanceIds;
}
/**
* Set List of connection instance IDs; It's an old parameter, please switch to ProxyIds.
* @param InstanceIds List of connection instance IDs; It's an old parameter, please switch to ProxyIds.
*/
public void setInstanceIds(String [] InstanceIds) {
this.InstanceIds = InstanceIds;
}
/**
* Get A string used to ensure the idempotency of the request, which is generated by the user and must be unique to each request. The maximum length is 64 ASCII characters. If this parameter is not specified, the idempotency of the request cannot be guaranteed.
For more information, please see How to Ensure Idempotence.
* @return ClientToken A string used to ensure the idempotency of the request, which is generated by the user and must be unique to each request. The maximum length is 64 ASCII characters. If this parameter is not specified, the idempotency of the request cannot be guaranteed.
For more information, please see How to Ensure Idempotence.
*/
public String getClientToken() {
return this.ClientToken;
}
/**
* Set A string used to ensure the idempotency of the request, which is generated by the user and must be unique to each request. The maximum length is 64 ASCII characters. If this parameter is not specified, the idempotency of the request cannot be guaranteed.
For more information, please see How to Ensure Idempotence.
* @param ClientToken A string used to ensure the idempotency of the request, which is generated by the user and must be unique to each request. The maximum length is 64 ASCII characters. If this parameter is not specified, the idempotency of the request cannot be guaranteed.
For more information, please see How to Ensure Idempotence.
*/
public void setClientToken(String ClientToken) {
this.ClientToken = ClientToken;
}
/**
* Get List of connection instance IDs; It's a new parameter.
* @return ProxyIds List of connection instance IDs; It's a new parameter.
*/
public String [] getProxyIds() {
return this.ProxyIds;
}
/**
* Set List of connection instance IDs; It's a new parameter.
* @param ProxyIds List of connection instance IDs; It's a new parameter.
*/
public void setProxyIds(String [] ProxyIds) {
this.ProxyIds = ProxyIds;
}
public DestroyProxiesRequest() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public DestroyProxiesRequest(DestroyProxiesRequest source) {
if (source.Force != null) {
this.Force = new Long(source.Force);
}
if (source.InstanceIds != null) {
this.InstanceIds = new String[source.InstanceIds.length];
for (int i = 0; i < source.InstanceIds.length; i++) {
this.InstanceIds[i] = new String(source.InstanceIds[i]);
}
}
if (source.ClientToken != null) {
this.ClientToken = new String(source.ClientToken);
}
if (source.ProxyIds != null) {
this.ProxyIds = new String[source.ProxyIds.length];
for (int i = 0; i < source.ProxyIds.length; i++) {
this.ProxyIds[i] = new String(source.ProxyIds[i]);
}
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "Force", this.Force);
this.setParamArraySimple(map, prefix + "InstanceIds.", this.InstanceIds);
this.setParamSimple(map, prefix + "ClientToken", this.ClientToken);
this.setParamArraySimple(map, prefix + "ProxyIds.", this.ProxyIds);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy