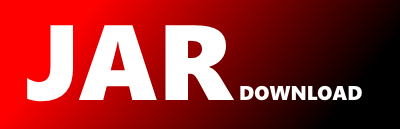
com.tencentcloudapi.vod.v20180717.models.ProcedureTask Maven / Gradle / Ivy
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.vod.v20180717.models;
import com.tencentcloudapi.common.AbstractModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class ProcedureTask extends AbstractModel{
/**
* Video processing task ID.
*/
@SerializedName("TaskId")
@Expose
private String TaskId;
/**
* Task flow status. Valid values:
PROCESSING: processing;
FINISH: completed.
*/
@SerializedName("Status")
@Expose
private String Status;
/**
* Disused. Please use `ErrCode` of each specific task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("ErrCode")
@Expose
private Long ErrCode;
/**
* Disused. Please use `Message` of each specific task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("Message")
@Expose
private String Message;
/**
* Media file ID.
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `FileId` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Id` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("FileId")
@Expose
private String FileId;
/**
* Media filename
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `BasicInfo.Name` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Name` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("FileName")
@Expose
private String FileName;
/**
* Media file address
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `BasicInfo.MediaUrl` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Url` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
*/
@SerializedName("FileUrl")
@Expose
private String FileUrl;
/**
* Source video metadata.
*/
@SerializedName("MetaData")
@Expose
private MediaMetaData MetaData;
/**
* Execution status and result of video processing task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("MediaProcessResultSet")
@Expose
private MediaProcessTaskResult [] MediaProcessResultSet;
/**
* Execution status and result of video content audit task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("AiContentReviewResultSet")
@Expose
private AiContentReviewResult [] AiContentReviewResultSet;
/**
* Execution status and result of video content analysis task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("AiAnalysisResultSet")
@Expose
private AiAnalysisResult [] AiAnalysisResultSet;
/**
* Execution status and result of video content recognition task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("AiRecognitionResultSet")
@Expose
private AiRecognitionResult [] AiRecognitionResultSet;
/**
* Task flow priority. Value range: [-10, 10].
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("TasksPriority")
@Expose
private Long TasksPriority;
/**
* Notification mode for change in task flow status.
Finish: an event notification will be initiated only after the task flow is completely executed;
Change: an event notification will be initiated as soon as the status of a subtask in the task flow changes;
None: no callback for the task flow will be accepted.
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("TasksNotifyMode")
@Expose
private String TasksNotifyMode;
/**
* The source context which is used to pass through the user request information. The task flow status change callback will return the value of this field. It can contain up to 1,000 characters.
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("SessionContext")
@Expose
private String SessionContext;
/**
* The ID used for deduplication. If there was a request with the same ID in the last seven days, the current request will return an error. The ID can contain up to 50 characters. If this parameter is left empty or a blank string is entered, no deduplication will be performed.
Note: this field may return null, indicating that no valid values can be obtained.
*/
@SerializedName("SessionId")
@Expose
private String SessionId;
/**
* Get Video processing task ID.
* @return TaskId Video processing task ID.
*/
public String getTaskId() {
return this.TaskId;
}
/**
* Set Video processing task ID.
* @param TaskId Video processing task ID.
*/
public void setTaskId(String TaskId) {
this.TaskId = TaskId;
}
/**
* Get Task flow status. Valid values:
PROCESSING: processing;
FINISH: completed.
* @return Status Task flow status. Valid values:
PROCESSING: processing;
FINISH: completed.
*/
public String getStatus() {
return this.Status;
}
/**
* Set Task flow status. Valid values:
PROCESSING: processing;
FINISH: completed.
* @param Status Task flow status. Valid values:
PROCESSING: processing;
FINISH: completed.
*/
public void setStatus(String Status) {
this.Status = Status;
}
/**
* Get Disused. Please use `ErrCode` of each specific task.
Note: this field may return null, indicating that no valid values can be obtained.
* @return ErrCode Disused. Please use `ErrCode` of each specific task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public Long getErrCode() {
return this.ErrCode;
}
/**
* Set Disused. Please use `ErrCode` of each specific task.
Note: this field may return null, indicating that no valid values can be obtained.
* @param ErrCode Disused. Please use `ErrCode` of each specific task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setErrCode(Long ErrCode) {
this.ErrCode = ErrCode;
}
/**
* Get Disused. Please use `Message` of each specific task.
Note: this field may return null, indicating that no valid values can be obtained.
* @return Message Disused. Please use `Message` of each specific task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public String getMessage() {
return this.Message;
}
/**
* Set Disused. Please use `Message` of each specific task.
Note: this field may return null, indicating that no valid values can be obtained.
* @param Message Disused. Please use `Message` of each specific task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setMessage(String Message) {
this.Message = Message;
}
/**
* Get Media file ID.
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `FileId` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Id` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
Note: this field may return null, indicating that no valid values can be obtained.
* @return FileId Media file ID.
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `FileId` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Id` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
Note: this field may return null, indicating that no valid values can be obtained.
*/
public String getFileId() {
return this.FileId;
}
/**
* Set Media file ID.
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `FileId` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Id` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
Note: this field may return null, indicating that no valid values can be obtained.
* @param FileId Media file ID.
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `FileId` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Id` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setFileId(String FileId) {
this.FileId = FileId;
}
/**
* Get Media filename
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `BasicInfo.Name` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Name` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
Note: this field may return null, indicating that no valid values can be obtained.
* @return FileName Media filename
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `BasicInfo.Name` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Name` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
Note: this field may return null, indicating that no valid values can be obtained.
*/
public String getFileName() {
return this.FileName;
}
/**
* Set Media filename
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `BasicInfo.Name` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Name` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
Note: this field may return null, indicating that no valid values can be obtained.
* @param FileName Media filename
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `BasicInfo.Name` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Name` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setFileName(String FileName) {
this.FileName = FileName;
}
/**
* Get Media file address
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `BasicInfo.MediaUrl` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Url` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
* @return FileUrl Media file address
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `BasicInfo.MediaUrl` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Url` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
*/
public String getFileUrl() {
return this.FileUrl;
}
/**
* Set Media file address
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `BasicInfo.MediaUrl` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Url` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
* @param FileUrl Media file address
If the task flow is initiated by [ProcessMedia](https://cloud.tencent.com/document/product/266/33427), this field means the `BasicInfo.MediaUrl` in [MediaInfo](https://cloud.tencent.com/document/product/266/31773#MediaInfo);
If the task flow is initiated by [ProcessMediaByUrl](https://cloud.tencent.com/document/product/266/33426), this field means the `Url` in [MediaInputInfo](https://cloud.tencent.com/document/product/266/31773#MediaInputInfo).
*/
public void setFileUrl(String FileUrl) {
this.FileUrl = FileUrl;
}
/**
* Get Source video metadata.
* @return MetaData Source video metadata.
*/
public MediaMetaData getMetaData() {
return this.MetaData;
}
/**
* Set Source video metadata.
* @param MetaData Source video metadata.
*/
public void setMetaData(MediaMetaData MetaData) {
this.MetaData = MetaData;
}
/**
* Get Execution status and result of video processing task.
Note: this field may return null, indicating that no valid values can be obtained.
* @return MediaProcessResultSet Execution status and result of video processing task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public MediaProcessTaskResult [] getMediaProcessResultSet() {
return this.MediaProcessResultSet;
}
/**
* Set Execution status and result of video processing task.
Note: this field may return null, indicating that no valid values can be obtained.
* @param MediaProcessResultSet Execution status and result of video processing task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setMediaProcessResultSet(MediaProcessTaskResult [] MediaProcessResultSet) {
this.MediaProcessResultSet = MediaProcessResultSet;
}
/**
* Get Execution status and result of video content audit task.
Note: this field may return null, indicating that no valid values can be obtained.
* @return AiContentReviewResultSet Execution status and result of video content audit task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public AiContentReviewResult [] getAiContentReviewResultSet() {
return this.AiContentReviewResultSet;
}
/**
* Set Execution status and result of video content audit task.
Note: this field may return null, indicating that no valid values can be obtained.
* @param AiContentReviewResultSet Execution status and result of video content audit task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setAiContentReviewResultSet(AiContentReviewResult [] AiContentReviewResultSet) {
this.AiContentReviewResultSet = AiContentReviewResultSet;
}
/**
* Get Execution status and result of video content analysis task.
Note: this field may return null, indicating that no valid values can be obtained.
* @return AiAnalysisResultSet Execution status and result of video content analysis task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public AiAnalysisResult [] getAiAnalysisResultSet() {
return this.AiAnalysisResultSet;
}
/**
* Set Execution status and result of video content analysis task.
Note: this field may return null, indicating that no valid values can be obtained.
* @param AiAnalysisResultSet Execution status and result of video content analysis task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setAiAnalysisResultSet(AiAnalysisResult [] AiAnalysisResultSet) {
this.AiAnalysisResultSet = AiAnalysisResultSet;
}
/**
* Get Execution status and result of video content recognition task.
Note: this field may return null, indicating that no valid values can be obtained.
* @return AiRecognitionResultSet Execution status and result of video content recognition task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public AiRecognitionResult [] getAiRecognitionResultSet() {
return this.AiRecognitionResultSet;
}
/**
* Set Execution status and result of video content recognition task.
Note: this field may return null, indicating that no valid values can be obtained.
* @param AiRecognitionResultSet Execution status and result of video content recognition task.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setAiRecognitionResultSet(AiRecognitionResult [] AiRecognitionResultSet) {
this.AiRecognitionResultSet = AiRecognitionResultSet;
}
/**
* Get Task flow priority. Value range: [-10, 10].
Note: this field may return null, indicating that no valid values can be obtained.
* @return TasksPriority Task flow priority. Value range: [-10, 10].
Note: this field may return null, indicating that no valid values can be obtained.
*/
public Long getTasksPriority() {
return this.TasksPriority;
}
/**
* Set Task flow priority. Value range: [-10, 10].
Note: this field may return null, indicating that no valid values can be obtained.
* @param TasksPriority Task flow priority. Value range: [-10, 10].
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setTasksPriority(Long TasksPriority) {
this.TasksPriority = TasksPriority;
}
/**
* Get Notification mode for change in task flow status.
Finish: an event notification will be initiated only after the task flow is completely executed;
Change: an event notification will be initiated as soon as the status of a subtask in the task flow changes;
None: no callback for the task flow will be accepted.
Note: this field may return null, indicating that no valid values can be obtained.
* @return TasksNotifyMode Notification mode for change in task flow status.
Finish: an event notification will be initiated only after the task flow is completely executed;
Change: an event notification will be initiated as soon as the status of a subtask in the task flow changes;
None: no callback for the task flow will be accepted.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public String getTasksNotifyMode() {
return this.TasksNotifyMode;
}
/**
* Set Notification mode for change in task flow status.
Finish: an event notification will be initiated only after the task flow is completely executed;
Change: an event notification will be initiated as soon as the status of a subtask in the task flow changes;
None: no callback for the task flow will be accepted.
Note: this field may return null, indicating that no valid values can be obtained.
* @param TasksNotifyMode Notification mode for change in task flow status.
Finish: an event notification will be initiated only after the task flow is completely executed;
Change: an event notification will be initiated as soon as the status of a subtask in the task flow changes;
None: no callback for the task flow will be accepted.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setTasksNotifyMode(String TasksNotifyMode) {
this.TasksNotifyMode = TasksNotifyMode;
}
/**
* Get The source context which is used to pass through the user request information. The task flow status change callback will return the value of this field. It can contain up to 1,000 characters.
Note: this field may return null, indicating that no valid values can be obtained.
* @return SessionContext The source context which is used to pass through the user request information. The task flow status change callback will return the value of this field. It can contain up to 1,000 characters.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public String getSessionContext() {
return this.SessionContext;
}
/**
* Set The source context which is used to pass through the user request information. The task flow status change callback will return the value of this field. It can contain up to 1,000 characters.
Note: this field may return null, indicating that no valid values can be obtained.
* @param SessionContext The source context which is used to pass through the user request information. The task flow status change callback will return the value of this field. It can contain up to 1,000 characters.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setSessionContext(String SessionContext) {
this.SessionContext = SessionContext;
}
/**
* Get The ID used for deduplication. If there was a request with the same ID in the last seven days, the current request will return an error. The ID can contain up to 50 characters. If this parameter is left empty or a blank string is entered, no deduplication will be performed.
Note: this field may return null, indicating that no valid values can be obtained.
* @return SessionId The ID used for deduplication. If there was a request with the same ID in the last seven days, the current request will return an error. The ID can contain up to 50 characters. If this parameter is left empty or a blank string is entered, no deduplication will be performed.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public String getSessionId() {
return this.SessionId;
}
/**
* Set The ID used for deduplication. If there was a request with the same ID in the last seven days, the current request will return an error. The ID can contain up to 50 characters. If this parameter is left empty or a blank string is entered, no deduplication will be performed.
Note: this field may return null, indicating that no valid values can be obtained.
* @param SessionId The ID used for deduplication. If there was a request with the same ID in the last seven days, the current request will return an error. The ID can contain up to 50 characters. If this parameter is left empty or a blank string is entered, no deduplication will be performed.
Note: this field may return null, indicating that no valid values can be obtained.
*/
public void setSessionId(String SessionId) {
this.SessionId = SessionId;
}
public ProcedureTask() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public ProcedureTask(ProcedureTask source) {
if (source.TaskId != null) {
this.TaskId = new String(source.TaskId);
}
if (source.Status != null) {
this.Status = new String(source.Status);
}
if (source.ErrCode != null) {
this.ErrCode = new Long(source.ErrCode);
}
if (source.Message != null) {
this.Message = new String(source.Message);
}
if (source.FileId != null) {
this.FileId = new String(source.FileId);
}
if (source.FileName != null) {
this.FileName = new String(source.FileName);
}
if (source.FileUrl != null) {
this.FileUrl = new String(source.FileUrl);
}
if (source.MetaData != null) {
this.MetaData = new MediaMetaData(source.MetaData);
}
if (source.MediaProcessResultSet != null) {
this.MediaProcessResultSet = new MediaProcessTaskResult[source.MediaProcessResultSet.length];
for (int i = 0; i < source.MediaProcessResultSet.length; i++) {
this.MediaProcessResultSet[i] = new MediaProcessTaskResult(source.MediaProcessResultSet[i]);
}
}
if (source.AiContentReviewResultSet != null) {
this.AiContentReviewResultSet = new AiContentReviewResult[source.AiContentReviewResultSet.length];
for (int i = 0; i < source.AiContentReviewResultSet.length; i++) {
this.AiContentReviewResultSet[i] = new AiContentReviewResult(source.AiContentReviewResultSet[i]);
}
}
if (source.AiAnalysisResultSet != null) {
this.AiAnalysisResultSet = new AiAnalysisResult[source.AiAnalysisResultSet.length];
for (int i = 0; i < source.AiAnalysisResultSet.length; i++) {
this.AiAnalysisResultSet[i] = new AiAnalysisResult(source.AiAnalysisResultSet[i]);
}
}
if (source.AiRecognitionResultSet != null) {
this.AiRecognitionResultSet = new AiRecognitionResult[source.AiRecognitionResultSet.length];
for (int i = 0; i < source.AiRecognitionResultSet.length; i++) {
this.AiRecognitionResultSet[i] = new AiRecognitionResult(source.AiRecognitionResultSet[i]);
}
}
if (source.TasksPriority != null) {
this.TasksPriority = new Long(source.TasksPriority);
}
if (source.TasksNotifyMode != null) {
this.TasksNotifyMode = new String(source.TasksNotifyMode);
}
if (source.SessionContext != null) {
this.SessionContext = new String(source.SessionContext);
}
if (source.SessionId != null) {
this.SessionId = new String(source.SessionId);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "TaskId", this.TaskId);
this.setParamSimple(map, prefix + "Status", this.Status);
this.setParamSimple(map, prefix + "ErrCode", this.ErrCode);
this.setParamSimple(map, prefix + "Message", this.Message);
this.setParamSimple(map, prefix + "FileId", this.FileId);
this.setParamSimple(map, prefix + "FileName", this.FileName);
this.setParamSimple(map, prefix + "FileUrl", this.FileUrl);
this.setParamObj(map, prefix + "MetaData.", this.MetaData);
this.setParamArrayObj(map, prefix + "MediaProcessResultSet.", this.MediaProcessResultSet);
this.setParamArrayObj(map, prefix + "AiContentReviewResultSet.", this.AiContentReviewResultSet);
this.setParamArrayObj(map, prefix + "AiAnalysisResultSet.", this.AiAnalysisResultSet);
this.setParamArrayObj(map, prefix + "AiRecognitionResultSet.", this.AiRecognitionResultSet);
this.setParamSimple(map, prefix + "TasksPriority", this.TasksPriority);
this.setParamSimple(map, prefix + "TasksNotifyMode", this.TasksNotifyMode);
this.setParamSimple(map, prefix + "SessionContext", this.SessionContext);
this.setParamSimple(map, prefix + "SessionId", this.SessionId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy