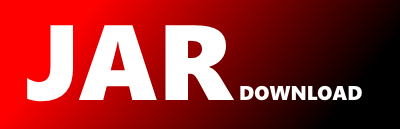
com.tencentcloudapi.billing.v20180709.BillingClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.billing.v20180709;
import java.lang.reflect.Type;
import com.google.gson.JsonSyntaxException;
import com.google.gson.reflect.TypeToken;
import com.tencentcloudapi.common.exception.TencentCloudSDKException;
import com.tencentcloudapi.common.AbstractClient;
import com.tencentcloudapi.common.profile.ClientProfile;
import com.tencentcloudapi.common.JsonResponseModel;
import com.tencentcloudapi.common.Credential;
import com.tencentcloudapi.billing.v20180709.models.*;
public class BillingClient extends AbstractClient{
private static String endpoint = "billing.tencentcloudapi.com";
private static String service = "billing";
private static String version = "2018-07-09";
public BillingClient(Credential credential, String region) {
this(credential, region, new ClientProfile());
}
public BillingClient(Credential credential, String region, ClientProfile profile) {
super(BillingClient.endpoint, BillingClient.version, credential, region, profile);
}
/**
*This API is used to check the Tencent Cloud account balance.
* @param req DescribeAccountBalanceRequest
* @return DescribeAccountBalanceResponse
* @throws TencentCloudSDKException
*/
public DescribeAccountBalanceResponse DescribeAccountBalance(DescribeAccountBalanceRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeAccountBalance");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query bill details.
* @param req DescribeBillDetailRequest
* @return DescribeBillDetailResponse
* @throws TencentCloudSDKException
*/
public DescribeBillDetailResponse DescribeBillDetail(DescribeBillDetailRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeBillDetail");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query bill resources summary.
* @param req DescribeBillResourceSummaryRequest
* @return DescribeBillResourceSummaryResponse
* @throws TencentCloudSDKException
*/
public DescribeBillResourceSummaryResponse DescribeBillResourceSummary(DescribeBillResourceSummaryRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeBillResourceSummary");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*Gets the bill summarized according to billing mode
* @param req DescribeBillSummaryByPayModeRequest
* @return DescribeBillSummaryByPayModeResponse
* @throws TencentCloudSDKException
*/
public DescribeBillSummaryByPayModeResponse DescribeBillSummaryByPayMode(DescribeBillSummaryByPayModeRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeBillSummaryByPayMode");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*Gets the bill summarized according to product
* @param req DescribeBillSummaryByProductRequest
* @return DescribeBillSummaryByProductResponse
* @throws TencentCloudSDKException
*/
public DescribeBillSummaryByProductResponse DescribeBillSummaryByProduct(DescribeBillSummaryByProductRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeBillSummaryByProduct");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*Gets the bill summarized according to project
* @param req DescribeBillSummaryByProjectRequest
* @return DescribeBillSummaryByProjectResponse
* @throws TencentCloudSDKException
*/
public DescribeBillSummaryByProjectResponse DescribeBillSummaryByProject(DescribeBillSummaryByProjectRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeBillSummaryByProject");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*Gets the bill summarized according to region
* @param req DescribeBillSummaryByRegionRequest
* @return DescribeBillSummaryByRegionResponse
* @throws TencentCloudSDKException
*/
public DescribeBillSummaryByRegionResponse DescribeBillSummaryByRegion(DescribeBillSummaryByRegionRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeBillSummaryByRegion");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to get the cost distribution over different tags.
* @param req DescribeBillSummaryByTagRequest
* @return DescribeBillSummaryByTagResponse
* @throws TencentCloudSDKException
*/
public DescribeBillSummaryByTagResponse DescribeBillSummaryByTag(DescribeBillSummaryByTagRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeBillSummaryByTag");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query COS usage details.
* @param req DescribeDosageCosDetailByDateRequest
* @return DescribeDosageCosDetailByDateResponse
* @throws TencentCloudSDKException
*/
public DescribeDosageCosDetailByDateResponse DescribeDosageCosDetailByDate(DescribeDosageCosDetailByDateRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeDosageCosDetailByDate");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query vouchers.
* @param req DescribeVoucherInfoRequest
* @return DescribeVoucherInfoResponse
* @throws TencentCloudSDKException
*/
public DescribeVoucherInfoResponse DescribeVoucherInfo(DescribeVoucherInfoRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeVoucherInfo");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*This API is used to query voucher usage details.
* @param req DescribeVoucherUsageDetailsRequest
* @return DescribeVoucherUsageDetailsResponse
* @throws TencentCloudSDKException
*/
public DescribeVoucherUsageDetailsResponse DescribeVoucherUsageDetails(DescribeVoucherUsageDetailsRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeVoucherUsageDetails");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy