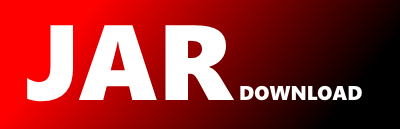
com.tencentcloudapi.teo.v20220901.models.CreateLogTopicTaskRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Show all versions of tencentcloud-sdk-java-intl-en Show documentation
Tencent Cloud API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.teo.v20220901.models;
import com.tencentcloudapi.common.AbstractModel;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.Expose;
import java.util.HashMap;
public class CreateLogTopicTaskRequest extends AbstractModel{
/**
* ID of the logset.
*/
@SerializedName("LogSetId")
@Expose
private String LogSetId;
/**
* Region of the logset.
*/
@SerializedName("LogSetRegion")
@Expose
private String LogSetRegion;
/**
* Topic name of the logset.
*/
@SerializedName("TopicName")
@Expose
private String TopicName;
/**
* Name of the shipping task.
*/
@SerializedName("TaskName")
@Expose
private String TaskName;
/**
* ID of the site.
*/
@SerializedName("ZoneId")
@Expose
private String ZoneId;
/**
* Name of the site.
*/
@SerializedName("ZoneName")
@Expose
private String ZoneName;
/**
* Type of the shipping entity. Values:
`domain`: L7 acceleration logs;
`application`: L4 acceleration logs;
`web-rateLiming`: Rate limiting logs;
`web-attack`: Web security logs;
`web-rule`: Custom rule logs;
`web-bot`: Bot management logs.
*/
@SerializedName("EntityType")
@Expose
private String EntityType;
/**
* Retention period of the log topic. Value range: 1–366 (in days).
*/
@SerializedName("Period")
@Expose
private Long Period;
/**
* List of shipping entities.
*/
@SerializedName("EntityList")
@Expose
private String [] EntityList;
/**
* Acceleration region. Values:
`mainland`: Chinese mainland.
`overseas`: Global (outside the Chinese mainland). If this field is not specified, the acceleration region will be determined based on the user’s region.
*/
@SerializedName("Area")
@Expose
private String Area;
/**
* Get ID of the logset.
* @return LogSetId ID of the logset.
*/
public String getLogSetId() {
return this.LogSetId;
}
/**
* Set ID of the logset.
* @param LogSetId ID of the logset.
*/
public void setLogSetId(String LogSetId) {
this.LogSetId = LogSetId;
}
/**
* Get Region of the logset.
* @return LogSetRegion Region of the logset.
*/
public String getLogSetRegion() {
return this.LogSetRegion;
}
/**
* Set Region of the logset.
* @param LogSetRegion Region of the logset.
*/
public void setLogSetRegion(String LogSetRegion) {
this.LogSetRegion = LogSetRegion;
}
/**
* Get Topic name of the logset.
* @return TopicName Topic name of the logset.
*/
public String getTopicName() {
return this.TopicName;
}
/**
* Set Topic name of the logset.
* @param TopicName Topic name of the logset.
*/
public void setTopicName(String TopicName) {
this.TopicName = TopicName;
}
/**
* Get Name of the shipping task.
* @return TaskName Name of the shipping task.
*/
public String getTaskName() {
return this.TaskName;
}
/**
* Set Name of the shipping task.
* @param TaskName Name of the shipping task.
*/
public void setTaskName(String TaskName) {
this.TaskName = TaskName;
}
/**
* Get ID of the site.
* @return ZoneId ID of the site.
*/
public String getZoneId() {
return this.ZoneId;
}
/**
* Set ID of the site.
* @param ZoneId ID of the site.
*/
public void setZoneId(String ZoneId) {
this.ZoneId = ZoneId;
}
/**
* Get Name of the site.
* @return ZoneName Name of the site.
*/
public String getZoneName() {
return this.ZoneName;
}
/**
* Set Name of the site.
* @param ZoneName Name of the site.
*/
public void setZoneName(String ZoneName) {
this.ZoneName = ZoneName;
}
/**
* Get Type of the shipping entity. Values:
`domain`: L7 acceleration logs;
`application`: L4 acceleration logs;
`web-rateLiming`: Rate limiting logs;
`web-attack`: Web security logs;
`web-rule`: Custom rule logs;
`web-bot`: Bot management logs.
* @return EntityType Type of the shipping entity. Values:
`domain`: L7 acceleration logs;
`application`: L4 acceleration logs;
`web-rateLiming`: Rate limiting logs;
`web-attack`: Web security logs;
`web-rule`: Custom rule logs;
`web-bot`: Bot management logs.
*/
public String getEntityType() {
return this.EntityType;
}
/**
* Set Type of the shipping entity. Values:
`domain`: L7 acceleration logs;
`application`: L4 acceleration logs;
`web-rateLiming`: Rate limiting logs;
`web-attack`: Web security logs;
`web-rule`: Custom rule logs;
`web-bot`: Bot management logs.
* @param EntityType Type of the shipping entity. Values:
`domain`: L7 acceleration logs;
`application`: L4 acceleration logs;
`web-rateLiming`: Rate limiting logs;
`web-attack`: Web security logs;
`web-rule`: Custom rule logs;
`web-bot`: Bot management logs.
*/
public void setEntityType(String EntityType) {
this.EntityType = EntityType;
}
/**
* Get Retention period of the log topic. Value range: 1–366 (in days).
* @return Period Retention period of the log topic. Value range: 1–366 (in days).
*/
public Long getPeriod() {
return this.Period;
}
/**
* Set Retention period of the log topic. Value range: 1–366 (in days).
* @param Period Retention period of the log topic. Value range: 1–366 (in days).
*/
public void setPeriod(Long Period) {
this.Period = Period;
}
/**
* Get List of shipping entities.
* @return EntityList List of shipping entities.
*/
public String [] getEntityList() {
return this.EntityList;
}
/**
* Set List of shipping entities.
* @param EntityList List of shipping entities.
*/
public void setEntityList(String [] EntityList) {
this.EntityList = EntityList;
}
/**
* Get Acceleration region. Values:
`mainland`: Chinese mainland.
`overseas`: Global (outside the Chinese mainland). If this field is not specified, the acceleration region will be determined based on the user’s region.
* @return Area Acceleration region. Values:
`mainland`: Chinese mainland.
`overseas`: Global (outside the Chinese mainland). If this field is not specified, the acceleration region will be determined based on the user’s region.
*/
public String getArea() {
return this.Area;
}
/**
* Set Acceleration region. Values:
`mainland`: Chinese mainland.
`overseas`: Global (outside the Chinese mainland). If this field is not specified, the acceleration region will be determined based on the user’s region.
* @param Area Acceleration region. Values:
`mainland`: Chinese mainland.
`overseas`: Global (outside the Chinese mainland). If this field is not specified, the acceleration region will be determined based on the user’s region.
*/
public void setArea(String Area) {
this.Area = Area;
}
public CreateLogTopicTaskRequest() {
}
/**
* NOTE: Any ambiguous key set via .set("AnyKey", "value") will be a shallow copy,
* and any explicit key, i.e Foo, set via .setFoo("value") will be a deep copy.
*/
public CreateLogTopicTaskRequest(CreateLogTopicTaskRequest source) {
if (source.LogSetId != null) {
this.LogSetId = new String(source.LogSetId);
}
if (source.LogSetRegion != null) {
this.LogSetRegion = new String(source.LogSetRegion);
}
if (source.TopicName != null) {
this.TopicName = new String(source.TopicName);
}
if (source.TaskName != null) {
this.TaskName = new String(source.TaskName);
}
if (source.ZoneId != null) {
this.ZoneId = new String(source.ZoneId);
}
if (source.ZoneName != null) {
this.ZoneName = new String(source.ZoneName);
}
if (source.EntityType != null) {
this.EntityType = new String(source.EntityType);
}
if (source.Period != null) {
this.Period = new Long(source.Period);
}
if (source.EntityList != null) {
this.EntityList = new String[source.EntityList.length];
for (int i = 0; i < source.EntityList.length; i++) {
this.EntityList[i] = new String(source.EntityList[i]);
}
}
if (source.Area != null) {
this.Area = new String(source.Area);
}
}
/**
* Internal implementation, normal users should not use it.
*/
public void toMap(HashMap map, String prefix) {
this.setParamSimple(map, prefix + "LogSetId", this.LogSetId);
this.setParamSimple(map, prefix + "LogSetRegion", this.LogSetRegion);
this.setParamSimple(map, prefix + "TopicName", this.TopicName);
this.setParamSimple(map, prefix + "TaskName", this.TaskName);
this.setParamSimple(map, prefix + "ZoneId", this.ZoneId);
this.setParamSimple(map, prefix + "ZoneName", this.ZoneName);
this.setParamSimple(map, prefix + "EntityType", this.EntityType);
this.setParamSimple(map, prefix + "Period", this.Period);
this.setParamArraySimple(map, prefix + "EntityList.", this.EntityList);
this.setParamSimple(map, prefix + "Area", this.Area);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy