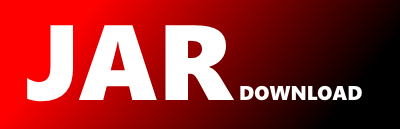
com.tencentcloudapi.lcic.v20220817.LcicClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tencentcloud-sdk-java-lcic Show documentation
Show all versions of tencentcloud-sdk-java-lcic Show documentation
Tencent Cloud Open API SDK for Java
/*
* Copyright (c) 2017-2018 THL A29 Limited, a Tencent company. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tencentcloudapi.lcic.v20220817;
import java.lang.reflect.Type;
import com.google.gson.JsonSyntaxException;
import com.google.gson.reflect.TypeToken;
import com.tencentcloudapi.common.exception.TencentCloudSDKException;
import com.tencentcloudapi.common.AbstractClient;
import com.tencentcloudapi.common.profile.ClientProfile;
import com.tencentcloudapi.common.JsonResponseModel;
import com.tencentcloudapi.common.Credential;
import com.tencentcloudapi.lcic.v20220817.models.*;
public class LcicClient extends AbstractClient{
private static String endpoint = "lcic.tencentcloudapi.com";
private static String service = "lcic";
private static String version = "2022-08-17";
public LcicClient(Credential credential, String region) {
this(credential, region, new ClientProfile());
}
public LcicClient(Credential credential, String region, ClientProfile profile) {
super(LcicClient.endpoint, LcicClient.version, credential, region, profile);
}
/**
*绑定文档到房间
* @param req BindDocumentToRoomRequest
* @return BindDocumentToRoomResponse
* @throws TencentCloudSDKException
*/
public BindDocumentToRoomResponse BindDocumentToRoom(BindDocumentToRoomRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "BindDocumentToRoom");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*创建房间内可以使用的文档。
* @param req CreateDocumentRequest
* @return CreateDocumentResponse
* @throws TencentCloudSDKException
*/
public CreateDocumentResponse CreateDocument(CreateDocumentRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "CreateDocument");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*创建房间
* @param req CreateRoomRequest
* @return CreateRoomResponse
* @throws TencentCloudSDKException
*/
public CreateRoomResponse CreateRoom(CreateRoomRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "CreateRoom");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*创建巡课
* @param req CreateSupervisorRequest
* @return CreateSupervisorResponse
* @throws TencentCloudSDKException
*/
public CreateSupervisorResponse CreateSupervisor(CreateSupervisorRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "CreateSupervisor");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*删除房间
* @param req DeleteRoomRequest
* @return DeleteRoomResponse
* @throws TencentCloudSDKException
*/
public DeleteRoomResponse DeleteRoom(DeleteRoomRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DeleteRoom");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*获取房间信息
* @param req DescribeRoomRequest
* @return DescribeRoomResponse
* @throws TencentCloudSDKException
*/
public DescribeRoomResponse DescribeRoom(DescribeRoomRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeRoom");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*获取房间统计信息,仅可在房间结束后调用。
* @param req DescribeRoomStatisticsRequest
* @return DescribeRoomStatisticsResponse
* @throws TencentCloudSDKException
*/
public DescribeRoomStatisticsResponse DescribeRoomStatistics(DescribeRoomStatisticsRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeRoomStatistics");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*获取用户信息
* @param req DescribeUserRequest
* @return DescribeUserResponse
* @throws TencentCloudSDKException
*/
public DescribeUserResponse DescribeUser(DescribeUserRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "DescribeUser");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*使用源账号登录,源账号为注册时填入的originId
* @param req LoginOriginIdRequest
* @return LoginOriginIdResponse
* @throws TencentCloudSDKException
*/
public LoginOriginIdResponse LoginOriginId(LoginOriginIdRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "LoginOriginId");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*登录
* @param req LoginUserRequest
* @return LoginUserResponse
* @throws TencentCloudSDKException
*/
public LoginUserResponse LoginUser(LoginUserRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "LoginUser");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*修改应用
* @param req ModifyAppRequest
* @return ModifyAppResponse
* @throws TencentCloudSDKException
*/
public ModifyAppResponse ModifyApp(ModifyAppRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "ModifyApp");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*注册用户
* @param req RegisterUserRequest
* @return RegisterUserResponse
* @throws TencentCloudSDKException
*/
public RegisterUserResponse RegisterUser(RegisterUserRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "RegisterUser");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*设置应用的自定义内容,包括应用图标,自定义的代码等。如果已存在,则为更新。更新js、css内容后,要生效也需要调用该接口
* @param req SetAppCustomContentRequest
* @return SetAppCustomContentResponse
* @throws TencentCloudSDKException
*/
public SetAppCustomContentResponse SetAppCustomContent(SetAppCustomContentRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "SetAppCustomContent");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
/**
*文档从房间解绑
* @param req UnbindDocumentFromRoomRequest
* @return UnbindDocumentFromRoomResponse
* @throws TencentCloudSDKException
*/
public UnbindDocumentFromRoomResponse UnbindDocumentFromRoom(UnbindDocumentFromRoomRequest req) throws TencentCloudSDKException{
JsonResponseModel rsp = null;
String rspStr = "";
try {
Type type = new TypeToken>() {
}.getType();
rspStr = this.internalRequest(req, "UnbindDocumentFromRoom");
rsp = gson.fromJson(rspStr, type);
} catch (JsonSyntaxException e) {
throw new TencentCloudSDKException("response message: " + rspStr + ".\n Error message: " + e.getMessage());
}
return rsp.response;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy