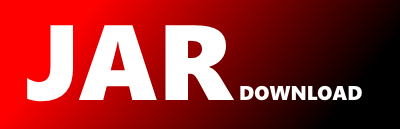
org.apache.hadoop.security.proto.SecurityProtos Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hadoop-apache2 Show documentation
Show all versions of hadoop-apache2 Show documentation
Shaded version of Apache Hadoop 2.x for Presto
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: Security.proto
package org.apache.hadoop.security.proto;
public final class SecurityProtos {
private SecurityProtos() {}
public static void registerAllExtensions(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistry registry) {
}
public interface TokenProtoOrBuilder
extends com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.MessageOrBuilder {
// required bytes identifier = 1;
/**
* required bytes identifier = 1;
*/
boolean hasIdentifier();
/**
* required bytes identifier = 1;
*/
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString getIdentifier();
// required bytes password = 2;
/**
* required bytes password = 2;
*/
boolean hasPassword();
/**
* required bytes password = 2;
*/
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString getPassword();
// required string kind = 3;
/**
* required string kind = 3;
*/
boolean hasKind();
/**
* required string kind = 3;
*/
java.lang.String getKind();
/**
* required string kind = 3;
*/
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString
getKindBytes();
// required string service = 4;
/**
* required string service = 4;
*/
boolean hasService();
/**
* required string service = 4;
*/
java.lang.String getService();
/**
* required string service = 4;
*/
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString
getServiceBytes();
}
/**
* Protobuf type {@code hadoop.common.TokenProto}
*
*
**
* Security token identifier
*
*/
public static final class TokenProto extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage
implements TokenProtoOrBuilder {
// Use TokenProto.newBuilder() to construct.
private TokenProto(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TokenProto(boolean noInit) { this.unknownFields = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TokenProto defaultInstance;
public static TokenProto getDefaultInstance() {
return defaultInstance;
}
public TokenProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TokenProto(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
identifier_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
password_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
kind_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
service_ = input.readBytes();
break;
}
}
}
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_TokenProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_TokenProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.TokenProto.class, org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder.class);
}
public static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser PARSER =
new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.AbstractParser() {
public TokenProto parsePartialFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return new TokenProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required bytes identifier = 1;
public static final int IDENTIFIER_FIELD_NUMBER = 1;
private com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString identifier_;
/**
* required bytes identifier = 1;
*/
public boolean hasIdentifier() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes identifier = 1;
*/
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString getIdentifier() {
return identifier_;
}
// required bytes password = 2;
public static final int PASSWORD_FIELD_NUMBER = 2;
private com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString password_;
/**
* required bytes password = 2;
*/
public boolean hasPassword() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes password = 2;
*/
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString getPassword() {
return password_;
}
// required string kind = 3;
public static final int KIND_FIELD_NUMBER = 3;
private java.lang.Object kind_;
/**
* required string kind = 3;
*/
public boolean hasKind() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required string kind = 3;
*/
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString bs =
(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
kind_ = s;
}
return s;
}
}
/**
* required string kind = 3;
*/
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString
getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString b =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref;
}
}
// required string service = 4;
public static final int SERVICE_FIELD_NUMBER = 4;
private java.lang.Object service_;
/**
* required string service = 4;
*/
public boolean hasService() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required string service = 4;
*/
public java.lang.String getService() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString bs =
(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
service_ = s;
}
return s;
}
}
/**
* required string service = 4;
*/
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString b =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
identifier_ = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.EMPTY;
password_ = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.EMPTY;
kind_ = "";
service_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasIdentifier()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPassword()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasKind()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasService()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, identifier_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, password_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getKindBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getServiceBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream
.computeBytesSize(1, identifier_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream
.computeBytesSize(2, password_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getKindBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getServiceBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.hadoop.security.proto.SecurityProtos.TokenProto)) {
return super.equals(obj);
}
org.apache.hadoop.security.proto.SecurityProtos.TokenProto other = (org.apache.hadoop.security.proto.SecurityProtos.TokenProto) obj;
boolean result = true;
result = result && (hasIdentifier() == other.hasIdentifier());
if (hasIdentifier()) {
result = result && getIdentifier()
.equals(other.getIdentifier());
}
result = result && (hasPassword() == other.hasPassword());
if (hasPassword()) {
result = result && getPassword()
.equals(other.getPassword());
}
result = result && (hasKind() == other.hasKind());
if (hasKind()) {
result = result && getKind()
.equals(other.getKind());
}
result = result && (hasService() == other.hasService());
if (hasService()) {
result = result && getService()
.equals(other.getService());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasIdentifier()) {
hash = (37 * hash) + IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getIdentifier().hashCode();
}
if (hasPassword()) {
hash = (37 * hash) + PASSWORD_FIELD_NUMBER;
hash = (53 * hash) + getPassword().hashCode();
}
if (hasKind()) {
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + getKind().hashCode();
}
if (hasService()) {
hash = (37 * hash) + SERVICE_FIELD_NUMBER;
hash = (53 * hash) + getService().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.hadoop.security.proto.SecurityProtos.TokenProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.TokenProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.TokenProto parseFrom(byte[] data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.TokenProto parseFrom(
byte[] data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.TokenProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.TokenProto parseFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.TokenProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.TokenProto parseDelimitedFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.TokenProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.TokenProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.hadoop.security.proto.SecurityProtos.TokenProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code hadoop.common.TokenProto}
*
*
**
* Security token identifier
*
*/
public static final class Builder extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder
implements org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder {
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_TokenProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_TokenProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.TokenProto.class, org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder.class);
}
// Construct using org.apache.hadoop.security.proto.SecurityProtos.TokenProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
identifier_ = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
password_ = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
kind_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
service_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_TokenProto_descriptor;
}
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto getDefaultInstanceForType() {
return org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
}
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto build() {
org.apache.hadoop.security.proto.SecurityProtos.TokenProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto buildPartial() {
org.apache.hadoop.security.proto.SecurityProtos.TokenProto result = new org.apache.hadoop.security.proto.SecurityProtos.TokenProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.identifier_ = identifier_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.password_ = password_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.kind_ = kind_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.service_ = service_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Message other) {
if (other instanceof org.apache.hadoop.security.proto.SecurityProtos.TokenProto) {
return mergeFrom((org.apache.hadoop.security.proto.SecurityProtos.TokenProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.hadoop.security.proto.SecurityProtos.TokenProto other) {
if (other == org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance()) return this;
if (other.hasIdentifier()) {
setIdentifier(other.getIdentifier());
}
if (other.hasPassword()) {
setPassword(other.getPassword());
}
if (other.hasKind()) {
bitField0_ |= 0x00000004;
kind_ = other.kind_;
onChanged();
}
if (other.hasService()) {
bitField0_ |= 0x00000008;
service_ = other.service_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasIdentifier()) {
return false;
}
if (!hasPassword()) {
return false;
}
if (!hasKind()) {
return false;
}
if (!hasService()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.hadoop.security.proto.SecurityProtos.TokenProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.hadoop.security.proto.SecurityProtos.TokenProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required bytes identifier = 1;
private com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString identifier_ = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.EMPTY;
/**
* required bytes identifier = 1;
*/
public boolean hasIdentifier() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes identifier = 1;
*/
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString getIdentifier() {
return identifier_;
}
/**
* required bytes identifier = 1;
*/
public Builder setIdentifier(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
identifier_ = value;
onChanged();
return this;
}
/**
* required bytes identifier = 1;
*/
public Builder clearIdentifier() {
bitField0_ = (bitField0_ & ~0x00000001);
identifier_ = getDefaultInstance().getIdentifier();
onChanged();
return this;
}
// required bytes password = 2;
private com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString password_ = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.EMPTY;
/**
* required bytes password = 2;
*/
public boolean hasPassword() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes password = 2;
*/
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString getPassword() {
return password_;
}
/**
* required bytes password = 2;
*/
public Builder setPassword(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
password_ = value;
onChanged();
return this;
}
/**
* required bytes password = 2;
*/
public Builder clearPassword() {
bitField0_ = (bitField0_ & ~0x00000002);
password_ = getDefaultInstance().getPassword();
onChanged();
return this;
}
// required string kind = 3;
private java.lang.Object kind_ = "";
/**
* required string kind = 3;
*/
public boolean hasKind() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required string kind = 3;
*/
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref)
.toStringUtf8();
kind_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string kind = 3;
*/
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString
getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof String) {
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString b =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref;
}
}
/**
* required string kind = 3;
*/
public Builder setKind(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
kind_ = value;
onChanged();
return this;
}
/**
* required string kind = 3;
*/
public Builder clearKind() {
bitField0_ = (bitField0_ & ~0x00000004);
kind_ = getDefaultInstance().getKind();
onChanged();
return this;
}
/**
* required string kind = 3;
*/
public Builder setKindBytes(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
kind_ = value;
onChanged();
return this;
}
// required string service = 4;
private java.lang.Object service_ = "";
/**
* required string service = 4;
*/
public boolean hasService() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required string service = 4;
*/
public java.lang.String getService() {
java.lang.Object ref = service_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref)
.toStringUtf8();
service_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string service = 4;
*/
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof String) {
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString b =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref;
}
}
/**
* required string service = 4;
*/
public Builder setService(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
service_ = value;
onChanged();
return this;
}
/**
* required string service = 4;
*/
public Builder clearService() {
bitField0_ = (bitField0_ & ~0x00000008);
service_ = getDefaultInstance().getService();
onChanged();
return this;
}
/**
* required string service = 4;
*/
public Builder setServiceBytes(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
service_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:hadoop.common.TokenProto)
}
static {
defaultInstance = new TokenProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:hadoop.common.TokenProto)
}
public interface GetDelegationTokenRequestProtoOrBuilder
extends com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.MessageOrBuilder {
// required string renewer = 1;
/**
* required string renewer = 1;
*/
boolean hasRenewer();
/**
* required string renewer = 1;
*/
java.lang.String getRenewer();
/**
* required string renewer = 1;
*/
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString
getRenewerBytes();
}
/**
* Protobuf type {@code hadoop.common.GetDelegationTokenRequestProto}
*/
public static final class GetDelegationTokenRequestProto extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage
implements GetDelegationTokenRequestProtoOrBuilder {
// Use GetDelegationTokenRequestProto.newBuilder() to construct.
private GetDelegationTokenRequestProto(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private GetDelegationTokenRequestProto(boolean noInit) { this.unknownFields = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final GetDelegationTokenRequestProto defaultInstance;
public static GetDelegationTokenRequestProto getDefaultInstance() {
return defaultInstance;
}
public GetDelegationTokenRequestProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetDelegationTokenRequestProto(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
renewer_ = input.readBytes();
break;
}
}
}
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_GetDelegationTokenRequestProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_GetDelegationTokenRequestProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto.class, org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto.Builder.class);
}
public static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser PARSER =
new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.AbstractParser() {
public GetDelegationTokenRequestProto parsePartialFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return new GetDelegationTokenRequestProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required string renewer = 1;
public static final int RENEWER_FIELD_NUMBER = 1;
private java.lang.Object renewer_;
/**
* required string renewer = 1;
*/
public boolean hasRenewer() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string renewer = 1;
*/
public java.lang.String getRenewer() {
java.lang.Object ref = renewer_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString bs =
(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
renewer_ = s;
}
return s;
}
}
/**
* required string renewer = 1;
*/
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString
getRenewerBytes() {
java.lang.Object ref = renewer_;
if (ref instanceof java.lang.String) {
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString b =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
renewer_ = b;
return b;
} else {
return (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
renewer_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasRenewer()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getRenewerBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getRenewerBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto)) {
return super.equals(obj);
}
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto other = (org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto) obj;
boolean result = true;
result = result && (hasRenewer() == other.hasRenewer());
if (hasRenewer()) {
result = result && getRenewer()
.equals(other.getRenewer());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasRenewer()) {
hash = (37 * hash) + RENEWER_FIELD_NUMBER;
hash = (53 * hash) + getRenewer().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto parseFrom(byte[] data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto parseFrom(
byte[] data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto parseFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto parseDelimitedFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code hadoop.common.GetDelegationTokenRequestProto}
*/
public static final class Builder extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder
implements org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProtoOrBuilder {
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_GetDelegationTokenRequestProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_GetDelegationTokenRequestProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto.class, org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto.Builder.class);
}
// Construct using org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
renewer_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_GetDelegationTokenRequestProto_descriptor;
}
public org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto getDefaultInstanceForType() {
return org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto.getDefaultInstance();
}
public org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto build() {
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto buildPartial() {
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto result = new org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.renewer_ = renewer_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Message other) {
if (other instanceof org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto) {
return mergeFrom((org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto other) {
if (other == org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto.getDefaultInstance()) return this;
if (other.hasRenewer()) {
bitField0_ |= 0x00000001;
renewer_ = other.renewer_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasRenewer()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenRequestProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required string renewer = 1;
private java.lang.Object renewer_ = "";
/**
* required string renewer = 1;
*/
public boolean hasRenewer() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string renewer = 1;
*/
public java.lang.String getRenewer() {
java.lang.Object ref = renewer_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref)
.toStringUtf8();
renewer_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string renewer = 1;
*/
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString
getRenewerBytes() {
java.lang.Object ref = renewer_;
if (ref instanceof String) {
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString b =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
renewer_ = b;
return b;
} else {
return (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString) ref;
}
}
/**
* required string renewer = 1;
*/
public Builder setRenewer(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
renewer_ = value;
onChanged();
return this;
}
/**
* required string renewer = 1;
*/
public Builder clearRenewer() {
bitField0_ = (bitField0_ & ~0x00000001);
renewer_ = getDefaultInstance().getRenewer();
onChanged();
return this;
}
/**
* required string renewer = 1;
*/
public Builder setRenewerBytes(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
renewer_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:hadoop.common.GetDelegationTokenRequestProto)
}
static {
defaultInstance = new GetDelegationTokenRequestProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:hadoop.common.GetDelegationTokenRequestProto)
}
public interface GetDelegationTokenResponseProtoOrBuilder
extends com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.MessageOrBuilder {
// optional .hadoop.common.TokenProto token = 1;
/**
* optional .hadoop.common.TokenProto token = 1;
*/
boolean hasToken();
/**
* optional .hadoop.common.TokenProto token = 1;
*/
org.apache.hadoop.security.proto.SecurityProtos.TokenProto getToken();
/**
* optional .hadoop.common.TokenProto token = 1;
*/
org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder getTokenOrBuilder();
}
/**
* Protobuf type {@code hadoop.common.GetDelegationTokenResponseProto}
*/
public static final class GetDelegationTokenResponseProto extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage
implements GetDelegationTokenResponseProtoOrBuilder {
// Use GetDelegationTokenResponseProto.newBuilder() to construct.
private GetDelegationTokenResponseProto(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private GetDelegationTokenResponseProto(boolean noInit) { this.unknownFields = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final GetDelegationTokenResponseProto defaultInstance;
public static GetDelegationTokenResponseProto getDefaultInstance() {
return defaultInstance;
}
public GetDelegationTokenResponseProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetDelegationTokenResponseProto(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = token_.toBuilder();
}
token_ = input.readMessage(org.apache.hadoop.security.proto.SecurityProtos.TokenProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(token_);
token_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_GetDelegationTokenResponseProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_GetDelegationTokenResponseProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto.class, org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto.Builder.class);
}
public static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser PARSER =
new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.AbstractParser() {
public GetDelegationTokenResponseProto parsePartialFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return new GetDelegationTokenResponseProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional .hadoop.common.TokenProto token = 1;
public static final int TOKEN_FIELD_NUMBER = 1;
private org.apache.hadoop.security.proto.SecurityProtos.TokenProto token_;
/**
* optional .hadoop.common.TokenProto token = 1;
*/
public boolean hasToken() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto getToken() {
return token_;
}
/**
* optional .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder getTokenOrBuilder() {
return token_;
}
private void initFields() {
token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (hasToken()) {
if (!getToken().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, token_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream
.computeMessageSize(1, token_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto)) {
return super.equals(obj);
}
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto other = (org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto) obj;
boolean result = true;
result = result && (hasToken() == other.hasToken());
if (hasToken()) {
result = result && getToken()
.equals(other.getToken());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasToken()) {
hash = (37 * hash) + TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getToken().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto parseFrom(byte[] data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto parseFrom(
byte[] data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto parseFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto parseDelimitedFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code hadoop.common.GetDelegationTokenResponseProto}
*/
public static final class Builder extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder
implements org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProtoOrBuilder {
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_GetDelegationTokenResponseProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_GetDelegationTokenResponseProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto.class, org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto.Builder.class);
}
// Construct using org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getTokenFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (tokenBuilder_ == null) {
token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
} else {
tokenBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_GetDelegationTokenResponseProto_descriptor;
}
public org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto getDefaultInstanceForType() {
return org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto.getDefaultInstance();
}
public org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto build() {
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto buildPartial() {
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto result = new org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (tokenBuilder_ == null) {
result.token_ = token_;
} else {
result.token_ = tokenBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Message other) {
if (other instanceof org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto) {
return mergeFrom((org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto other) {
if (other == org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto.getDefaultInstance()) return this;
if (other.hasToken()) {
mergeToken(other.getToken());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (hasToken()) {
if (!getToken().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.hadoop.security.proto.SecurityProtos.GetDelegationTokenResponseProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional .hadoop.common.TokenProto token = 1;
private org.apache.hadoop.security.proto.SecurityProtos.TokenProto token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
private com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.SingleFieldBuilder<
org.apache.hadoop.security.proto.SecurityProtos.TokenProto, org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder, org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder> tokenBuilder_;
/**
* optional .hadoop.common.TokenProto token = 1;
*/
public boolean hasToken() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto getToken() {
if (tokenBuilder_ == null) {
return token_;
} else {
return tokenBuilder_.getMessage();
}
}
/**
* optional .hadoop.common.TokenProto token = 1;
*/
public Builder setToken(org.apache.hadoop.security.proto.SecurityProtos.TokenProto value) {
if (tokenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
token_ = value;
onChanged();
} else {
tokenBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .hadoop.common.TokenProto token = 1;
*/
public Builder setToken(
org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder builderForValue) {
if (tokenBuilder_ == null) {
token_ = builderForValue.build();
onChanged();
} else {
tokenBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .hadoop.common.TokenProto token = 1;
*/
public Builder mergeToken(org.apache.hadoop.security.proto.SecurityProtos.TokenProto value) {
if (tokenBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
token_ != org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance()) {
token_ =
org.apache.hadoop.security.proto.SecurityProtos.TokenProto.newBuilder(token_).mergeFrom(value).buildPartial();
} else {
token_ = value;
}
onChanged();
} else {
tokenBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .hadoop.common.TokenProto token = 1;
*/
public Builder clearToken() {
if (tokenBuilder_ == null) {
token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
onChanged();
} else {
tokenBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder getTokenBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getTokenFieldBuilder().getBuilder();
}
/**
* optional .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder getTokenOrBuilder() {
if (tokenBuilder_ != null) {
return tokenBuilder_.getMessageOrBuilder();
} else {
return token_;
}
}
/**
* optional .hadoop.common.TokenProto token = 1;
*/
private com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.SingleFieldBuilder<
org.apache.hadoop.security.proto.SecurityProtos.TokenProto, org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder, org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder>
getTokenFieldBuilder() {
if (tokenBuilder_ == null) {
tokenBuilder_ = new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.SingleFieldBuilder<
org.apache.hadoop.security.proto.SecurityProtos.TokenProto, org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder, org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder>(
token_,
getParentForChildren(),
isClean());
token_ = null;
}
return tokenBuilder_;
}
// @@protoc_insertion_point(builder_scope:hadoop.common.GetDelegationTokenResponseProto)
}
static {
defaultInstance = new GetDelegationTokenResponseProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:hadoop.common.GetDelegationTokenResponseProto)
}
public interface RenewDelegationTokenRequestProtoOrBuilder
extends com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.MessageOrBuilder {
// required .hadoop.common.TokenProto token = 1;
/**
* required .hadoop.common.TokenProto token = 1;
*/
boolean hasToken();
/**
* required .hadoop.common.TokenProto token = 1;
*/
org.apache.hadoop.security.proto.SecurityProtos.TokenProto getToken();
/**
* required .hadoop.common.TokenProto token = 1;
*/
org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder getTokenOrBuilder();
}
/**
* Protobuf type {@code hadoop.common.RenewDelegationTokenRequestProto}
*/
public static final class RenewDelegationTokenRequestProto extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage
implements RenewDelegationTokenRequestProtoOrBuilder {
// Use RenewDelegationTokenRequestProto.newBuilder() to construct.
private RenewDelegationTokenRequestProto(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RenewDelegationTokenRequestProto(boolean noInit) { this.unknownFields = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RenewDelegationTokenRequestProto defaultInstance;
public static RenewDelegationTokenRequestProto getDefaultInstance() {
return defaultInstance;
}
public RenewDelegationTokenRequestProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RenewDelegationTokenRequestProto(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = token_.toBuilder();
}
token_ = input.readMessage(org.apache.hadoop.security.proto.SecurityProtos.TokenProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(token_);
token_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_RenewDelegationTokenRequestProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_RenewDelegationTokenRequestProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto.class, org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto.Builder.class);
}
public static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser PARSER =
new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.AbstractParser() {
public RenewDelegationTokenRequestProto parsePartialFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return new RenewDelegationTokenRequestProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .hadoop.common.TokenProto token = 1;
public static final int TOKEN_FIELD_NUMBER = 1;
private org.apache.hadoop.security.proto.SecurityProtos.TokenProto token_;
/**
* required .hadoop.common.TokenProto token = 1;
*/
public boolean hasToken() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto getToken() {
return token_;
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder getTokenOrBuilder() {
return token_;
}
private void initFields() {
token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasToken()) {
memoizedIsInitialized = 0;
return false;
}
if (!getToken().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, token_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream
.computeMessageSize(1, token_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto)) {
return super.equals(obj);
}
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto other = (org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto) obj;
boolean result = true;
result = result && (hasToken() == other.hasToken());
if (hasToken()) {
result = result && getToken()
.equals(other.getToken());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasToken()) {
hash = (37 * hash) + TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getToken().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto parseFrom(byte[] data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto parseFrom(
byte[] data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto parseFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto parseDelimitedFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code hadoop.common.RenewDelegationTokenRequestProto}
*/
public static final class Builder extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder
implements org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProtoOrBuilder {
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_RenewDelegationTokenRequestProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_RenewDelegationTokenRequestProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto.class, org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto.Builder.class);
}
// Construct using org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getTokenFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (tokenBuilder_ == null) {
token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
} else {
tokenBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_RenewDelegationTokenRequestProto_descriptor;
}
public org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto getDefaultInstanceForType() {
return org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto.getDefaultInstance();
}
public org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto build() {
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto buildPartial() {
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto result = new org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (tokenBuilder_ == null) {
result.token_ = token_;
} else {
result.token_ = tokenBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Message other) {
if (other instanceof org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto) {
return mergeFrom((org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto other) {
if (other == org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto.getDefaultInstance()) return this;
if (other.hasToken()) {
mergeToken(other.getToken());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasToken()) {
return false;
}
if (!getToken().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenRequestProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .hadoop.common.TokenProto token = 1;
private org.apache.hadoop.security.proto.SecurityProtos.TokenProto token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
private com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.SingleFieldBuilder<
org.apache.hadoop.security.proto.SecurityProtos.TokenProto, org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder, org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder> tokenBuilder_;
/**
* required .hadoop.common.TokenProto token = 1;
*/
public boolean hasToken() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto getToken() {
if (tokenBuilder_ == null) {
return token_;
} else {
return tokenBuilder_.getMessage();
}
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public Builder setToken(org.apache.hadoop.security.proto.SecurityProtos.TokenProto value) {
if (tokenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
token_ = value;
onChanged();
} else {
tokenBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public Builder setToken(
org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder builderForValue) {
if (tokenBuilder_ == null) {
token_ = builderForValue.build();
onChanged();
} else {
tokenBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public Builder mergeToken(org.apache.hadoop.security.proto.SecurityProtos.TokenProto value) {
if (tokenBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
token_ != org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance()) {
token_ =
org.apache.hadoop.security.proto.SecurityProtos.TokenProto.newBuilder(token_).mergeFrom(value).buildPartial();
} else {
token_ = value;
}
onChanged();
} else {
tokenBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public Builder clearToken() {
if (tokenBuilder_ == null) {
token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
onChanged();
} else {
tokenBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder getTokenBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getTokenFieldBuilder().getBuilder();
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder getTokenOrBuilder() {
if (tokenBuilder_ != null) {
return tokenBuilder_.getMessageOrBuilder();
} else {
return token_;
}
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
private com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.SingleFieldBuilder<
org.apache.hadoop.security.proto.SecurityProtos.TokenProto, org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder, org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder>
getTokenFieldBuilder() {
if (tokenBuilder_ == null) {
tokenBuilder_ = new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.SingleFieldBuilder<
org.apache.hadoop.security.proto.SecurityProtos.TokenProto, org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder, org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder>(
token_,
getParentForChildren(),
isClean());
token_ = null;
}
return tokenBuilder_;
}
// @@protoc_insertion_point(builder_scope:hadoop.common.RenewDelegationTokenRequestProto)
}
static {
defaultInstance = new RenewDelegationTokenRequestProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:hadoop.common.RenewDelegationTokenRequestProto)
}
public interface RenewDelegationTokenResponseProtoOrBuilder
extends com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.MessageOrBuilder {
// required uint64 newExpiryTime = 1;
/**
* required uint64 newExpiryTime = 1;
*/
boolean hasNewExpiryTime();
/**
* required uint64 newExpiryTime = 1;
*/
long getNewExpiryTime();
}
/**
* Protobuf type {@code hadoop.common.RenewDelegationTokenResponseProto}
*/
public static final class RenewDelegationTokenResponseProto extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage
implements RenewDelegationTokenResponseProtoOrBuilder {
// Use RenewDelegationTokenResponseProto.newBuilder() to construct.
private RenewDelegationTokenResponseProto(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RenewDelegationTokenResponseProto(boolean noInit) { this.unknownFields = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RenewDelegationTokenResponseProto defaultInstance;
public static RenewDelegationTokenResponseProto getDefaultInstance() {
return defaultInstance;
}
public RenewDelegationTokenResponseProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RenewDelegationTokenResponseProto(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
newExpiryTime_ = input.readUInt64();
break;
}
}
}
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_RenewDelegationTokenResponseProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_RenewDelegationTokenResponseProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto.class, org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto.Builder.class);
}
public static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser PARSER =
new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.AbstractParser() {
public RenewDelegationTokenResponseProto parsePartialFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return new RenewDelegationTokenResponseProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required uint64 newExpiryTime = 1;
public static final int NEWEXPIRYTIME_FIELD_NUMBER = 1;
private long newExpiryTime_;
/**
* required uint64 newExpiryTime = 1;
*/
public boolean hasNewExpiryTime() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required uint64 newExpiryTime = 1;
*/
public long getNewExpiryTime() {
return newExpiryTime_;
}
private void initFields() {
newExpiryTime_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasNewExpiryTime()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt64(1, newExpiryTime_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, newExpiryTime_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto)) {
return super.equals(obj);
}
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto other = (org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto) obj;
boolean result = true;
result = result && (hasNewExpiryTime() == other.hasNewExpiryTime());
if (hasNewExpiryTime()) {
result = result && (getNewExpiryTime()
== other.getNewExpiryTime());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasNewExpiryTime()) {
hash = (37 * hash) + NEWEXPIRYTIME_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getNewExpiryTime());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto parseFrom(byte[] data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto parseFrom(
byte[] data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto parseFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto parseDelimitedFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code hadoop.common.RenewDelegationTokenResponseProto}
*/
public static final class Builder extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder
implements org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProtoOrBuilder {
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_RenewDelegationTokenResponseProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_RenewDelegationTokenResponseProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto.class, org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto.Builder.class);
}
// Construct using org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
newExpiryTime_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_RenewDelegationTokenResponseProto_descriptor;
}
public org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto getDefaultInstanceForType() {
return org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto.getDefaultInstance();
}
public org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto build() {
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto buildPartial() {
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto result = new org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.newExpiryTime_ = newExpiryTime_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Message other) {
if (other instanceof org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto) {
return mergeFrom((org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto other) {
if (other == org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto.getDefaultInstance()) return this;
if (other.hasNewExpiryTime()) {
setNewExpiryTime(other.getNewExpiryTime());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasNewExpiryTime()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.hadoop.security.proto.SecurityProtos.RenewDelegationTokenResponseProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required uint64 newExpiryTime = 1;
private long newExpiryTime_ ;
/**
* required uint64 newExpiryTime = 1;
*/
public boolean hasNewExpiryTime() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required uint64 newExpiryTime = 1;
*/
public long getNewExpiryTime() {
return newExpiryTime_;
}
/**
* required uint64 newExpiryTime = 1;
*/
public Builder setNewExpiryTime(long value) {
bitField0_ |= 0x00000001;
newExpiryTime_ = value;
onChanged();
return this;
}
/**
* required uint64 newExpiryTime = 1;
*/
public Builder clearNewExpiryTime() {
bitField0_ = (bitField0_ & ~0x00000001);
newExpiryTime_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:hadoop.common.RenewDelegationTokenResponseProto)
}
static {
defaultInstance = new RenewDelegationTokenResponseProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:hadoop.common.RenewDelegationTokenResponseProto)
}
public interface CancelDelegationTokenRequestProtoOrBuilder
extends com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.MessageOrBuilder {
// required .hadoop.common.TokenProto token = 1;
/**
* required .hadoop.common.TokenProto token = 1;
*/
boolean hasToken();
/**
* required .hadoop.common.TokenProto token = 1;
*/
org.apache.hadoop.security.proto.SecurityProtos.TokenProto getToken();
/**
* required .hadoop.common.TokenProto token = 1;
*/
org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder getTokenOrBuilder();
}
/**
* Protobuf type {@code hadoop.common.CancelDelegationTokenRequestProto}
*/
public static final class CancelDelegationTokenRequestProto extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage
implements CancelDelegationTokenRequestProtoOrBuilder {
// Use CancelDelegationTokenRequestProto.newBuilder() to construct.
private CancelDelegationTokenRequestProto(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private CancelDelegationTokenRequestProto(boolean noInit) { this.unknownFields = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final CancelDelegationTokenRequestProto defaultInstance;
public static CancelDelegationTokenRequestProto getDefaultInstance() {
return defaultInstance;
}
public CancelDelegationTokenRequestProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CancelDelegationTokenRequestProto(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = token_.toBuilder();
}
token_ = input.readMessage(org.apache.hadoop.security.proto.SecurityProtos.TokenProto.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(token_);
token_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_CancelDelegationTokenRequestProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_CancelDelegationTokenRequestProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto.class, org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto.Builder.class);
}
public static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser PARSER =
new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.AbstractParser() {
public CancelDelegationTokenRequestProto parsePartialFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return new CancelDelegationTokenRequestProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// required .hadoop.common.TokenProto token = 1;
public static final int TOKEN_FIELD_NUMBER = 1;
private org.apache.hadoop.security.proto.SecurityProtos.TokenProto token_;
/**
* required .hadoop.common.TokenProto token = 1;
*/
public boolean hasToken() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto getToken() {
return token_;
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder getTokenOrBuilder() {
return token_;
}
private void initFields() {
token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
if (!hasToken()) {
memoizedIsInitialized = 0;
return false;
}
if (!getToken().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, token_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream
.computeMessageSize(1, token_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto)) {
return super.equals(obj);
}
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto other = (org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto) obj;
boolean result = true;
result = result && (hasToken() == other.hasToken());
if (hasToken()) {
result = result && getToken()
.equals(other.getToken());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasToken()) {
hash = (37 * hash) + TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getToken().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto parseFrom(byte[] data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto parseFrom(
byte[] data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto parseFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto parseDelimitedFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code hadoop.common.CancelDelegationTokenRequestProto}
*/
public static final class Builder extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder
implements org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProtoOrBuilder {
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_CancelDelegationTokenRequestProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_CancelDelegationTokenRequestProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto.class, org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto.Builder.class);
}
// Construct using org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getTokenFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (tokenBuilder_ == null) {
token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
} else {
tokenBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_CancelDelegationTokenRequestProto_descriptor;
}
public org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto getDefaultInstanceForType() {
return org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto.getDefaultInstance();
}
public org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto build() {
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto buildPartial() {
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto result = new org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (tokenBuilder_ == null) {
result.token_ = token_;
} else {
result.token_ = tokenBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Message other) {
if (other instanceof org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto) {
return mergeFrom((org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto other) {
if (other == org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto.getDefaultInstance()) return this;
if (other.hasToken()) {
mergeToken(other.getToken());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasToken()) {
return false;
}
if (!getToken().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenRequestProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// required .hadoop.common.TokenProto token = 1;
private org.apache.hadoop.security.proto.SecurityProtos.TokenProto token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
private com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.SingleFieldBuilder<
org.apache.hadoop.security.proto.SecurityProtos.TokenProto, org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder, org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder> tokenBuilder_;
/**
* required .hadoop.common.TokenProto token = 1;
*/
public boolean hasToken() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto getToken() {
if (tokenBuilder_ == null) {
return token_;
} else {
return tokenBuilder_.getMessage();
}
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public Builder setToken(org.apache.hadoop.security.proto.SecurityProtos.TokenProto value) {
if (tokenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
token_ = value;
onChanged();
} else {
tokenBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public Builder setToken(
org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder builderForValue) {
if (tokenBuilder_ == null) {
token_ = builderForValue.build();
onChanged();
} else {
tokenBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public Builder mergeToken(org.apache.hadoop.security.proto.SecurityProtos.TokenProto value) {
if (tokenBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
token_ != org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance()) {
token_ =
org.apache.hadoop.security.proto.SecurityProtos.TokenProto.newBuilder(token_).mergeFrom(value).buildPartial();
} else {
token_ = value;
}
onChanged();
} else {
tokenBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public Builder clearToken() {
if (tokenBuilder_ == null) {
token_ = org.apache.hadoop.security.proto.SecurityProtos.TokenProto.getDefaultInstance();
onChanged();
} else {
tokenBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder getTokenBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getTokenFieldBuilder().getBuilder();
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
public org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder getTokenOrBuilder() {
if (tokenBuilder_ != null) {
return tokenBuilder_.getMessageOrBuilder();
} else {
return token_;
}
}
/**
* required .hadoop.common.TokenProto token = 1;
*/
private com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.SingleFieldBuilder<
org.apache.hadoop.security.proto.SecurityProtos.TokenProto, org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder, org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder>
getTokenFieldBuilder() {
if (tokenBuilder_ == null) {
tokenBuilder_ = new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.SingleFieldBuilder<
org.apache.hadoop.security.proto.SecurityProtos.TokenProto, org.apache.hadoop.security.proto.SecurityProtos.TokenProto.Builder, org.apache.hadoop.security.proto.SecurityProtos.TokenProtoOrBuilder>(
token_,
getParentForChildren(),
isClean());
token_ = null;
}
return tokenBuilder_;
}
// @@protoc_insertion_point(builder_scope:hadoop.common.CancelDelegationTokenRequestProto)
}
static {
defaultInstance = new CancelDelegationTokenRequestProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:hadoop.common.CancelDelegationTokenRequestProto)
}
public interface CancelDelegationTokenResponseProtoOrBuilder
extends com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code hadoop.common.CancelDelegationTokenResponseProto}
*
*
* void response
*
*/
public static final class CancelDelegationTokenResponseProto extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage
implements CancelDelegationTokenResponseProtoOrBuilder {
// Use CancelDelegationTokenResponseProto.newBuilder() to construct.
private CancelDelegationTokenResponseProto(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private CancelDelegationTokenResponseProto(boolean noInit) { this.unknownFields = com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final CancelDelegationTokenResponseProto defaultInstance;
public static CancelDelegationTokenResponseProto getDefaultInstance() {
return defaultInstance;
}
public CancelDelegationTokenResponseProto getDefaultInstanceForType() {
return defaultInstance;
}
private final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CancelDelegationTokenResponseProto(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
initFields();
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_CancelDelegationTokenResponseProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_CancelDelegationTokenResponseProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto.class, org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto.Builder.class);
}
public static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser PARSER =
new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.AbstractParser() {
public CancelDelegationTokenResponseProto parsePartialFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return new CancelDelegationTokenResponseProto(input, extensionRegistry);
}
};
@java.lang.Override
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private void initFields() {
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto)) {
return super.equals(obj);
}
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto other = (org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto) obj;
boolean result = true;
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ByteString data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto parseFrom(byte[] data)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto parseFrom(
byte[] data,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto parseFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto parseDelimitedFrom(
java.io.InputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto parseFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code hadoop.common.CancelDelegationTokenResponseProto}
*
*
* void response
*
*/
public static final class Builder extends
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.Builder
implements org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProtoOrBuilder {
public static final com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_CancelDelegationTokenResponseProto_descriptor;
}
protected com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_CancelDelegationTokenResponseProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto.class, org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto.Builder.class);
}
// Construct using org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.hadoop.security.proto.SecurityProtos.internal_static_hadoop_common_CancelDelegationTokenResponseProto_descriptor;
}
public org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto getDefaultInstanceForType() {
return org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto.getDefaultInstance();
}
public org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto build() {
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto buildPartial() {
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto result = new org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto(this);
onBuilt();
return result;
}
public Builder mergeFrom(com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Message other) {
if (other instanceof org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto) {
return mergeFrom((org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto other) {
if (other == org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.CodedInputStream input,
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.hadoop.security.proto.SecurityProtos.CancelDelegationTokenResponseProto) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
// @@protoc_insertion_point(builder_scope:hadoop.common.CancelDelegationTokenResponseProto)
}
static {
defaultInstance = new CancelDelegationTokenResponseProto(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:hadoop.common.CancelDelegationTokenResponseProto)
}
private static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
internal_static_hadoop_common_TokenProto_descriptor;
private static
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_hadoop_common_TokenProto_fieldAccessorTable;
private static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
internal_static_hadoop_common_GetDelegationTokenRequestProto_descriptor;
private static
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_hadoop_common_GetDelegationTokenRequestProto_fieldAccessorTable;
private static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
internal_static_hadoop_common_GetDelegationTokenResponseProto_descriptor;
private static
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_hadoop_common_GetDelegationTokenResponseProto_fieldAccessorTable;
private static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
internal_static_hadoop_common_RenewDelegationTokenRequestProto_descriptor;
private static
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_hadoop_common_RenewDelegationTokenRequestProto_fieldAccessorTable;
private static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
internal_static_hadoop_common_RenewDelegationTokenResponseProto_descriptor;
private static
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_hadoop_common_RenewDelegationTokenResponseProto_fieldAccessorTable;
private static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
internal_static_hadoop_common_CancelDelegationTokenRequestProto_descriptor;
private static
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_hadoop_common_CancelDelegationTokenRequestProto_fieldAccessorTable;
private static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.Descriptor
internal_static_hadoop_common_CancelDelegationTokenResponseProto_descriptor;
private static
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_hadoop_common_CancelDelegationTokenResponseProto_fieldAccessorTable;
public static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\016Security.proto\022\rhadoop.common\"Q\n\nToken" +
"Proto\022\022\n\nidentifier\030\001 \002(\014\022\020\n\010password\030\002 " +
"\002(\014\022\014\n\004kind\030\003 \002(\t\022\017\n\007service\030\004 \002(\t\"1\n\036Ge" +
"tDelegationTokenRequestProto\022\017\n\007renewer\030" +
"\001 \002(\t\"K\n\037GetDelegationTokenResponseProto" +
"\022(\n\005token\030\001 \001(\0132\031.hadoop.common.TokenPro" +
"to\"L\n RenewDelegationTokenRequestProto\022(" +
"\n\005token\030\001 \002(\0132\031.hadoop.common.TokenProto" +
"\":\n!RenewDelegationTokenResponseProto\022\025\n" +
"\rnewExpiryTime\030\001 \002(\004\"M\n!CancelDelegation",
"TokenRequestProto\022(\n\005token\030\001 \002(\0132\031.hadoo" +
"p.common.TokenProto\"$\n\"CancelDelegationT" +
"okenResponseProtoB8\n org.apache.hadoop.s" +
"ecurity.protoB\016SecurityProtos\210\001\001\240\001\001"
};
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.ExtensionRegistry assignDescriptors(
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_hadoop_common_TokenProto_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_hadoop_common_TokenProto_fieldAccessorTable = new
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_hadoop_common_TokenProto_descriptor,
new java.lang.String[] { "Identifier", "Password", "Kind", "Service", });
internal_static_hadoop_common_GetDelegationTokenRequestProto_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_hadoop_common_GetDelegationTokenRequestProto_fieldAccessorTable = new
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_hadoop_common_GetDelegationTokenRequestProto_descriptor,
new java.lang.String[] { "Renewer", });
internal_static_hadoop_common_GetDelegationTokenResponseProto_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_hadoop_common_GetDelegationTokenResponseProto_fieldAccessorTable = new
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_hadoop_common_GetDelegationTokenResponseProto_descriptor,
new java.lang.String[] { "Token", });
internal_static_hadoop_common_RenewDelegationTokenRequestProto_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_hadoop_common_RenewDelegationTokenRequestProto_fieldAccessorTable = new
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_hadoop_common_RenewDelegationTokenRequestProto_descriptor,
new java.lang.String[] { "Token", });
internal_static_hadoop_common_RenewDelegationTokenResponseProto_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_hadoop_common_RenewDelegationTokenResponseProto_fieldAccessorTable = new
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_hadoop_common_RenewDelegationTokenResponseProto_descriptor,
new java.lang.String[] { "NewExpiryTime", });
internal_static_hadoop_common_CancelDelegationTokenRequestProto_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_hadoop_common_CancelDelegationTokenRequestProto_fieldAccessorTable = new
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_hadoop_common_CancelDelegationTokenRequestProto_descriptor,
new java.lang.String[] { "Token", });
internal_static_hadoop_common_CancelDelegationTokenResponseProto_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_hadoop_common_CancelDelegationTokenResponseProto_fieldAccessorTable = new
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_hadoop_common_CancelDelegationTokenResponseProto_descriptor,
new java.lang.String[] { });
return null;
}
};
com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.facebook.presto.hadoop.$internal.com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy