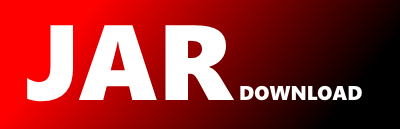
com.teradata.tempto.assertions.QueryAssert Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.teradata.tempto.assertions;
import com.teradata.tempto.configuration.Configuration;
import com.teradata.tempto.internal.convention.SqlResultDescriptor;
import com.teradata.tempto.internal.query.QueryResultValueComparator;
import com.teradata.tempto.query.QueryExecutionException;
import com.teradata.tempto.query.QueryExecutor;
import com.teradata.tempto.query.QueryResult;
import org.assertj.core.api.AbstractAssert;
import org.assertj.core.api.Assertions;
import org.slf4j.Logger;
import java.sql.JDBCType;
import java.text.DecimalFormat;
import java.text.NumberFormat;
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import static com.google.common.collect.Lists.newArrayList;
import static com.teradata.tempto.assertions.QueryAssert.Row.row;
import static com.teradata.tempto.internal.configuration.TestConfigurationFactory.createTestConfiguration;
import static com.teradata.tempto.query.QueryResult.fromSqlIndex;
import static com.teradata.tempto.query.QueryResult.toSqlIndex;
import static java.lang.String.format;
import static java.sql.JDBCType.INTEGER;
import static java.util.Optional.ofNullable;
import static org.slf4j.LoggerFactory.getLogger;
public class QueryAssert
extends AbstractAssert
{
private static final Logger LOGGER = getLogger(QueryExecutor.class);
private static final NumberFormat DECIMAL_FORMAT = new DecimalFormat("#0.00000000000");
private final List> columnComparators;
private final List columnTypes;
private QueryAssert(QueryResult actual)
{
super(actual, QueryAssert.class);
this.columnComparators = getComparators(actual);
this.columnTypes = actual.getColumnTypes();
}
public static QueryAssert assertThat(QueryResult queryResult)
{
return new QueryAssert(queryResult);
}
public static QueryExecutionAssert assertThat(QueryCallback queryCallback)
{
QueryExecutionException executionException = null;
try {
queryCallback.executeQuery();
}
catch (QueryExecutionException e) {
executionException = e;
}
return new QueryExecutionAssert(ofNullable(executionException));
}
public QueryAssert matches(SqlResultDescriptor sqlResultDescriptor)
{
if (sqlResultDescriptor.getExpectedTypes().isPresent()) {
hasColumns(sqlResultDescriptor.getExpectedTypes().get());
}
List rows = null;
try {
rows = sqlResultDescriptor.getRows(columnTypes);
}
catch (Exception e) {
failWithMessage("Could not map expected file content to query column types; types=%s; content=<%s>; error=<%s>",
columnTypes, sqlResultDescriptor.getOriginalContent(), e.getMessage());
}
if (sqlResultDescriptor.isIgnoreOrder()) {
contains(rows);
}
else {
containsExactly(rows);
}
if (!sqlResultDescriptor.isIgnoreExcessRows()) {
hasRowsCount(rows.size());
}
return this;
}
public QueryAssert hasRowsCount(int resultCount)
{
if (actual.getRowsCount() != resultCount) {
failWithMessage("Expected row count to be <%s>, but was <%s>; rows=%s", resultCount, actual.getRowsCount(), actual.rows());
}
return this;
}
public QueryAssert hasNoRows()
{
return hasRowsCount(0);
}
public QueryAssert hasAnyRows()
{
if (actual.getRowsCount() == 0) {
failWithMessage("Expected some rows to be returned from query");
}
return this;
}
public QueryAssert hasColumnsCount(int columnCount)
{
if (actual.getColumnsCount() != columnCount) {
failWithMessage("Expected column count to be <%s>, but was <%s> - columns <%s>", columnCount, actual.getColumnsCount(), actual.getColumnTypes());
}
return this;
}
public QueryAssert hasColumns(List expectedTypes)
{
hasColumnsCount(expectedTypes.size());
for (int i = 0; i < expectedTypes.size(); i++) {
JDBCType expectedType = expectedTypes.get(i);
JDBCType actualType = actual.getColumnType(toSqlIndex(i));
if (!actualType.equals(expectedType)) {
failWithMessage("Expected <%s> column of type <%s>, but was <%s>, actual columns: %s", i, expectedType, actualType, actual.getColumnTypes());
}
}
return this;
}
public QueryAssert hasColumns(JDBCType... expectedTypes)
{
return hasColumns(Arrays.asList(expectedTypes));
}
/**
* Verifies that the actual result set contains all the given {@code rows}
*
* @param rows Rows to be matched
* @return this
*/
public QueryAssert contains(List rows)
{
List> missingRows = newArrayList();
for (Row row : rows) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy