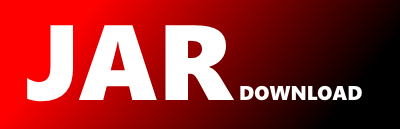
com.teradata.tempto.query.QueryResult Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.teradata.tempto.query;
import com.google.common.base.Preconditions;
import com.google.common.collect.BiMap;
import com.google.common.collect.HashBiMap;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
import java.sql.JDBCType;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import static com.google.common.base.Preconditions.checkState;
import static com.google.common.collect.Lists.newArrayList;
import static java.sql.JDBCType.INTEGER;
import static java.util.Optional.ofNullable;
import static java.util.stream.Collectors.toList;
/**
* Result of a query.
*
* It stores all returned values, column names and their types as {@link java.sql.JDBCType}.
*/
public class QueryResult
{
private final List columnTypes;
private final BiMap columnNamesIndexes;
private final List> values;
private final Optional jdbcResultSet;
private QueryResult(List columnTypes, BiMap columnNamesIndexes, List> values, Optional jdbcResultSet)
{
this.columnTypes = columnTypes;
this.values = values;
this.columnNamesIndexes = columnNamesIndexes;
this.jdbcResultSet = jdbcResultSet;
}
public int getRowsCount()
{
return values.size();
}
public int getColumnsCount()
{
return columnTypes.size();
}
public List getColumnTypes()
{
return columnTypes;
}
public JDBCType getColumnType(int columnIndex)
{
return columnTypes.get(fromSqlIndex(columnIndex));
}
public Optional tryFindColumnIndex(String columnName)
{
return ofNullable(columnNamesIndexes.get(columnName));
}
public List