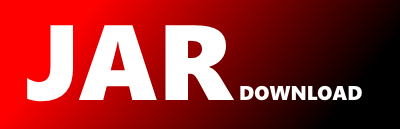
com.tersesystems.echopraxia.Logger Maven / Gradle / Ivy
package com.tersesystems.echopraxia;
import com.tersesystems.echopraxia.api.*;
import com.tersesystems.echopraxia.spi.AbstractLoggerSupport;
import com.tersesystems.echopraxia.spi.CoreLogger;
import java.util.function.Function;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
/**
* An echopraxia logger built around a field builder.
*
* @param the field builder type.
*/
public class Logger extends AbstractLoggerSupport, FB>
implements DefaultLoggerMethods {
/**
* This is where the logging methods are called, so the stacktrace element shows
* DefaultLoggerMethods as the caller.
*/
public static final String FQCN = DefaultLoggerMethods.class.getName();
/**
* @param core the coree logger
* @param fieldBuilder the field builder
*/
protected Logger(@NotNull CoreLogger core, @NotNull FB fieldBuilder) {
super(core, fieldBuilder, Logger.class);
}
/**
* Creates a new logger with the given field builder.
*
* @param newBuilder the given field builder.
* @param the type of the field builder.
* @return a new logger using the given field builder.
*/
@NotNull
public Logger withFieldBuilder(@NotNull T newBuilder) {
return newLogger(newBuilder);
}
// This is not part of the AbstractLoggerSupport
protected Logger newLogger(T newBuilder) {
if (this.fieldBuilder == newBuilder) {
//noinspection unchecked
return (Logger) this;
}
return new Logger<>(core(), newBuilder);
}
@Override
protected @NotNull Logger newLogger(CoreLogger core) {
return new Logger<>(core, fieldBuilder);
}
@Override
protected @NotNull Logger neverLogger() {
return new NeverLogger<>(core.withCondition(Condition.never()), fieldBuilder);
}
/**
* An optimized logger for use with {@code Condition.never()}.
*
* @param the field builder type.
*/
public static class NeverLogger extends Logger {
protected NeverLogger(@NotNull CoreLogger core, @NotNull FB fieldBuilder) {
super(core, fieldBuilder);
}
@Override
public @NotNull Logger withThreadContext() {
return this;
}
protected @NotNull Logger newLogger(CoreLogger core) {
return this;
}
@Override
public @NotNull Logger withFieldBuilder(@NotNull T newBuilder) {
return new NeverLogger(core, newBuilder);
}
@Override
public @NotNull Logger withFields(@NotNull Function f) {
return this;
}
@Override
public @NotNull Logger withCondition(@NotNull Condition condition) {
return this;
}
/**
* @return true if the logger level is TRACE or higher.
*/
public boolean isTraceEnabled() {
return false;
}
/**
* @param condition the given condition.
* @return true if the logger level is TRACE or higher and the condition is met.
*/
public boolean isTraceEnabled(@NotNull Condition condition) {
return false;
}
@Override
public void trace(@Nullable String message) {
// do nothing
}
@Override
public void trace(@Nullable String message, @NotNull Function f) {
// do nothing
}
@Override
public void trace(@Nullable String message, @NotNull Throwable e) {
// do nothing
}
@Override
public void trace(@NotNull Condition condition, @Nullable String message) {
// do nothing
}
@Override
public void trace(
@NotNull Condition condition,
@Nullable String message,
@NotNull Function f) {
// do nothing
}
@Override
public void trace(
@NotNull Condition condition, @Nullable String message, @NotNull Throwable e) {
// do nothing
}
/**
* @return true if the logger level is DEBUG or higher.
*/
public boolean isDebugEnabled() {
return false;
}
/**
* @param condition the given condition.
* @return true if the logger level is DEBUG or higher and the condition is met.
*/
public boolean isDebugEnabled(@NotNull Condition condition) {
return false;
}
@Override
public void debug(@Nullable String message) {
// do nothing
}
@Override
public void debug(@Nullable String message, @NotNull Function f) {
// do nothing
}
@Override
public void debug(@Nullable String message, @NotNull Throwable e) {
// do nothing
}
@Override
public void debug(@NotNull Condition condition, @Nullable String message) {
// do nothing
}
@Override
public void debug(
@NotNull Condition condition,
@Nullable String message,
@NotNull Function f) {
// do nothing
}
@Override
public void debug(
@NotNull Condition condition, @Nullable String message, @NotNull Throwable e) {
// do nothing
}
/**
* @return true if the logger level is INFO or higher.
*/
public boolean isInfoEnabled() {
return false;
}
/**
* @param condition the given condition.
* @return true if the logger level is INFO or higher and the condition is met.
*/
public boolean isInfoEnabled(@NotNull Condition condition) {
return false;
}
@Override
public void info(@Nullable String message) {
// do nothing
}
@Override
public void info(@Nullable String message, @NotNull Function f) {
// do nothing
}
@Override
public void info(@Nullable String message, @NotNull Throwable e) {
// do nothing
}
@Override
public void info(@NotNull Condition condition, @Nullable String message) {
// do nothing
}
@Override
public void info(
@NotNull Condition condition,
@Nullable String message,
@NotNull Function f) {
// do nothing
}
@Override
public void info(@NotNull Condition condition, @Nullable String message, @NotNull Throwable e) {
// do nothing
}
/**
* @return true if the logger level is WARN or higher.
*/
public boolean isWarnEnabled() {
return false;
}
/**
* @param condition the given condition.
* @return true if the logger level is WARN or higher and the condition is met.
*/
public boolean isWarnEnabled(@NotNull Condition condition) {
return false;
}
@Override
public void warn(@Nullable String message, @NotNull Function f) {
// do nothing
}
@Override
public void warn(@Nullable String message, @NotNull Throwable e) {
// do nothing
}
@Override
public void warn(@NotNull Condition condition, @Nullable String message) {
// do nothing
}
@Override
public void warn(
@NotNull Condition condition,
@Nullable String message,
@NotNull Function f) {
// do nothing
}
@Override
public void warn(@NotNull Condition condition, @Nullable String message, @NotNull Throwable e) {
// do nothing
}
/**
* @return true if the logger level is ERROR or higher.
*/
public boolean isErrorEnabled() {
return false;
}
/**
* @param condition the given condition.
* @return true if the logger level is ERROR or higher and the condition is met.
*/
public boolean isErrorEnabled(@NotNull Condition condition) {
return false;
}
@Override
public void error(@Nullable String message) {
// do nothing
}
@Override
public void error(@Nullable String message, @NotNull Function f) {
// do nothing
}
@Override
public void error(@Nullable String message, @NotNull Throwable e) {
// do nothing
}
@Override
public void error(@NotNull Condition condition, @Nullable String message) {
// do nothing
}
@Override
public void error(
@NotNull Condition condition,
@Nullable String message,
@NotNull Function f) {
// do nothing
}
@Override
public void error(
@NotNull Condition condition, @Nullable String message, @NotNull Throwable e) {
// do nothing
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy