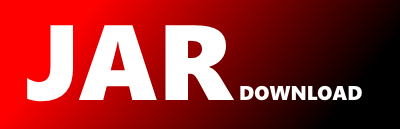
arjuna.client.guiauto.component.GuiAutoComponentFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arjuna-java Show documentation
Show all versions of arjuna-java Show documentation
Arjuna-Java is the client implementation in Java for development of test automation using Arjuna. It uses TestNG as the test engine. With minor tweaks, it can be used with any other test engine or custom test automation implementations. Arjuna is a Python based test automation framework developed by Rahul Verma (www.rahulverma.net)
The newest version!
/*******************************************************************************
* Copyright 2015-19 Test Mile Software Testing Pvt Ltd
*
* Website: www.TestMile.com
* Email: support [at] testmile.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package arjuna.client.guiauto.component;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import arjuna.client.core.action.GuiAutoActionType;
import arjuna.client.core.config.ArjunaComponent;
import arjuna.client.core.connector.BaseSetuObject;
import arjuna.client.core.connector.SetuArg;
import arjuna.client.core.connector.SetuResponse;
import arjuna.client.guiauto.automator.AppAutomator;
import arjuna.client.testsession.TestSession;
import arjuna.tpi.guiauto.GuiSource;
import arjuna.tpi.guiauto.With;
import arjuna.tpi.guiauto.component.*;
public class GuiAutoComponentFactory {
public static GuiElement Element(TestSession session, AppAutomator automator, String setuId) {
return new DefaultGuiElement(session, automator, setuId);
}
public static GuiMultiElement MultiElement(TestSession session, AppAutomator automator, String setuId) {
return new DefaultGuiMultiElement(session, automator, setuId);
}
public static DropDown DropDown(TestSession session, AppAutomator automator, String setuId) {
return new DefaultDropDown(session, automator, setuId);
}
public static RadioGroup RadioGroup(TestSession session, AppAutomator automator, String setuId) {
return new DefaultRadioGroup(session, automator, setuId);
}
public static Frame Frame(TestSession session, AppAutomator automator, String setuId) {
return new DefaultFrame(session, automator, setuId);
}
public static Alert Alert(TestSession session, AppAutomator automator, String setuId) {
return new DefaultAlert(session, automator, setuId);
}
public static MainWindow MainWindow(TestSession session, AppAutomator automator, String setuId) {
return new DefaultMainWindow(session, automator, setuId);
}
public static DomRoot DomRoot(TestSession session, AppAutomator automator) {
return new DefaultDomRoot(session, automator);
}
public static Browser Browser(TestSession session, AppAutomator automator) {
return new DefaultBrowser(session, automator);
}
private static class BaseComponent extends BaseSetuObject{
private AppAutomator automator;
private TestSession testSession;
private GuiComponentType compType;
public BaseComponent(TestSession session, AppAutomator automator, GuiComponentType compType) {
this.testSession = session;
this.automator = automator;
if (automator.isGui()) {
this.setGuiSetuIdArg(automator.getSetuId());
} else {
this.setAutomatorSetuIdArg(automator.getSetuId());
}
this.compType = compType;
this.setTestSessionSetuIdArg(testSession.getSetuId());
}
private SetuArg[] getTypeArgArray() {
return new SetuArg[] {SetuArg.arg("origGuiComponentType", this.compType)};
}
protected AppAutomator getAutomator() {
return this.automator;
}
protected TestSession getTestSession() {
return this.testSession;
}
protected SetuResponse sendSetuRequest(GuiAutoActionType actionType, SetuArg... args) throws Exception {
return this.sendRequest(ArjunaComponent.GUI_AUTOMATOR, actionType, SetuArg.combineArrays(getTypeArgArray(), args));
}
public GuiSource Source() throws Exception {
return new DefaultGuiSource(this.getAutomator(), this.sendSetuRequest(GuiAutoActionType.GET_SOURCE).getValueForKey("textBlobSetuId").asString());
}
protected GuiComponentType getComponentType() {
return this.compType;
}
}
private static class BaseElement extends BaseComponent{
public BaseElement(TestSession session, AppAutomator automator, GuiComponentType compType) {
super(session, automator, compType);
}
public BaseElement(TestSession session, AppAutomator automator, GuiComponentType compType, String elemSetuId) {
super(session, automator, compType);
this.setSetuId(elemSetuId);
this.setSelfSetuIdArg("elementSetuId");
}
public BaseElement(TestSession testSession, AppAutomator automator, GuiComponentType compType, String elemSetuId, int index) {
this(testSession, automator, compType, elemSetuId);
this.addArgs(
SetuArg.arg("isInstanceAction", true),
SetuArg.arg("instanceIndex", index)
);
}
protected void updateConfig(GuiActionConfig config) throws Exception {
this.sendSetuRequest(GuiAutoActionType.CONFIGURE, SetuArg.arg("elementConfig", config.getSettings()));
}
}
private static class DefaultGuiElement extends BaseElement implements GuiElement {
public DefaultGuiElement(TestSession session, AppAutomator automator, String setuId) {
super(session, automator, GuiComponentType.ELEMENT, setuId);
}
public DefaultGuiElement(TestSession session, AppAutomator automator, String setuId, int index) {
super(session, automator, GuiComponentType.ELEMENT, setuId, index);
}
@Override
public void enterText(String text) throws Exception {
this.sendSetuRequest(GuiAutoActionType.ENTER_TEXT, SetuArg.textArg(text));
}
@Override
public void setText(String text) throws Exception {
this.sendSetuRequest(GuiAutoActionType.SET_TEXT, SetuArg.textArg(text));
}
@Override
public void click() throws Exception {
this.sendSetuRequest(GuiAutoActionType.CLICK);
}
@Override
public void waitUntilPresent() throws Exception {
this.sendSetuRequest(GuiAutoActionType.WAIT_UNTIL_PRESENT);
}
@Override
public void waitUntilVisible() throws Exception {
this.sendSetuRequest(GuiAutoActionType.WAIT_UNTIL_VISIBLE);
}
@Override
public void waitUntilClickable() throws Exception {
this.sendSetuRequest(GuiAutoActionType.WAIT_UNTIL_CLICKABLE);
}
@Override
public void check() throws Exception {
this.sendSetuRequest(GuiAutoActionType.CHECK);
}
@Override
public void uncheck() throws Exception {
this.sendSetuRequest(GuiAutoActionType.UNCHECK);
}
@Override
public void identify() throws Exception {
this.sendSetuRequest(GuiAutoActionType.IDENTIFY);
}
@Override
public GuiElement configure(GuiActionConfig config) throws Exception {
super.updateConfig(config);
return this;
}
}
private static class DefaultGuiMultiElement extends BaseElement implements GuiMultiElement {
public DefaultGuiMultiElement(TestSession session, AppAutomator automator, String setuId) {
super(session, automator, GuiComponentType.MULTI_ELEMENT, setuId);
}
@Override
public GuiElement atIndex(int index) {
return new DefaultGuiElement(this.getTestSession(), this.getAutomator(), this.getSetuId(), index);
}
@Override
public GuiElement first() throws Exception {
return atIndex(0);
}
@Override
public GuiElement last() throws Exception {
return atIndex(this.length() - 1);
}
@Override
public GuiElement random() throws Exception {
SetuResponse response = this.sendSetuRequest(GuiAutoActionType.GET_RANDOM_INDEX);
return atIndex(response.getValue().asInt());
}
@Override
public int length() throws Exception {
SetuResponse response = this.sendSetuRequest(GuiAutoActionType.GET_INSTANCE_COUNT);
return response.getValue().asInt();
}
}
private static class MultiElementSelectable extends BaseElement {
protected MultiElementSelectable(TestSession session, AppAutomator automator, GuiComponentType compType, String setuId) {
super(session, automator, compType, setuId);
}
protected List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy