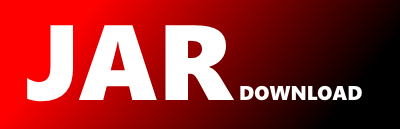
arjuna.lib.config.DefaultTestContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arjuna-java Show documentation
Show all versions of arjuna-java Show documentation
Arjuna-Java is the client implementation in Java for development of test automation using Arjuna. It uses TestNG as the test engine. With minor tweaks, it can be used with any other test engine or custom test automation implementations. Arjuna is a Python based test automation framework developed by Rahul Verma (www.rahulverma.net)
The newest version!
package arjuna.lib.config;
import java.util.HashMap;
import java.util.Map;
import arjuna.client.testsession.TestSession;
import arjuna.tpi.Arjuna;
import arjuna.tpi.test.TestConfig;
import arjuna.tpi.test.TestContext;
public class DefaultTestContext implements TestContext {
private TestSession testSession;
private String name;
private Map configMap = new HashMap();
public DefaultTestContext(TestSession session, String name) throws Exception {
this.testSession = session;
this.name = name;
ConfigBuilder builder = this.ConfigBuilder();
builder.sourceConfig(Arjuna.getCentralConfig());
builder.build(ConfigBuilder.DEFAULT_CONF_NAME);
}
public DefaultTestContext(TestSession session, String name, TestContext parentContext) {
this.testSession = session;
this.name = name;
this.configMap = parentContext.cloneConfigMap();
}
@Override
public ConfigBuilder ConfigBuilder() {
return new ConfigBuilder(this.testSession, this.configMap);
}
@Override
public TestConfig getConfig() {
return this.configMap.get(ConfigBuilder.DEFAULT_CONF_NAME);
}
@Override
public TestConfig getConfig(String name) throws Exception {
if (name == null) {
throw new Exception("Config name was passed as null.");
} else {
try {
return this.configMap.get(name.toLowerCase());
} catch (Exception e) {
throw new Exception("No context config found with name: " + name);
}
}
}
@Override
public String getName() {
return this.name;
}
protected void updateOptions(Map optionMap) throws Exception {
for (String confName: this.configMap.keySet()) {
ConfigBuilder builder = this.ConfigBuilder();
builder.sourceConfig(this.configMap.get(confName));
builder.options(optionMap);
builder.build(confName);
}
}
@Override
public Map cloneConfigMap() {
Map outMap = new HashMap();
outMap.putAll(this.configMap);
return outMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy