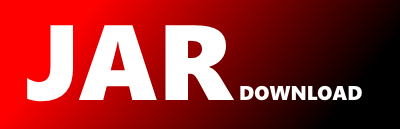
arjuna.lib.console.Console Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arjuna-java Show documentation
Show all versions of arjuna-java Show documentation
Arjuna-Java is the client implementation in Java for development of test automation using Arjuna. It uses TestNG as the test engine. With minor tweaks, it can be used with any other test engine or custom test automation implementations. Arjuna is a Python based test automation framework developed by Rahul Verma (www.rahulverma.net)
The newest version!
package arjuna.lib.console;
import org.apache.log4j.Logger;
import arjuna.lib.utils.ExceptionBatteries;
public class Console {
private static Logger logger = null;
private static boolean done = false;
private static String separator = null;
public synchronized static void init() {
if (done)
return;
//logger = Arjuna.getLogger();
separator = System.getProperty("line.separator");
done = true;
}
private synchronized static boolean logToCentralLog(String message) {
logger.debug(message);
return true;
}
public synchronized static void display(String message) {
boolean shouldPrint = logToCentralLog(message);
if (shouldPrint) {
System.out.println(message);
}
}
public synchronized static void displayError(String message) {
boolean shouldPrint = logToCentralLog(message);
if (shouldPrint) {
System.err.println(message);
}
}
private synchronized static void errorForConsole(String message) {
System.err.println(message);
}
public synchronized static void displayOnSameLine(String message) {
boolean shouldPrint = logToCentralLog(message);
if (shouldPrint) {
System.out.print(message);
}
}
public synchronized static void marker(int length) {
String marker = new String(new char[length]).replace('\0', '-');
display(marker);
}
public synchronized static void markerError(int length) {
String marker = new String(new char[length]).replace('\0', '-');
displayError(marker);
}
public synchronized static void markerOnSameLine(int length) {
String marker = new String(new char[length]).replace('\0', '-');
displayOnSameLine(marker);
}
public synchronized static void marker(int length, char symbol) {
String marker = new String(new char[length]).replace('\0', symbol);
display(marker);
}
public synchronized static void markerError(int length, char symbol) {
String marker = new String(new char[length]).replace('\0', symbol);
displayError(marker);
}
public synchronized static void displayKeyValue(String key, String value) {
String message = String.format("%s %s", key, value);
display(message);
}
public synchronized static void displayPaddedKeyValue(String key, String value) {
String message = String.format("| %-20s| %s", key, value);
display(message);
}
public synchronized static void displayPaddedKeyValue(String key, String value, int leftPadding) {
String rightPad = String.format("%%%ds", leftPadding + 2);
String cleanedValue = value.replace(separator, separator + "|" + String.format(rightPad, "|")); // .replace("\t",
// String.format(rightPad,
// ""));
String fmt = String.format("| %%-%ds| %%s", leftPadding);
String message = String.format(fmt, key, cleanedValue);
display(message);
}
public synchronized static void displayPaddedKeyValueError(String key, String value, int leftPadding) {
String rightPad = String.format("%%%ds", leftPadding + 2);
String cleanedValue = value.replace(separator, separator + "|" + String.format(rightPad, "|")); // .replace("\t",
// String.format(rightPad,
// ""));
String fmt = String.format("| %%-%ds| %%s", leftPadding);
String message = String.format(fmt, key, cleanedValue);
displayError(message);
}
public synchronized static void displayExceptionBlock(Throwable e) {
Console.markerError(80);
Console.displayPaddedKeyValueError("Exception Type", e.getClass().getSimpleName(), 30);
if (e.getMessage() != null){
Console.displayPaddedKeyValueError("Exception Message", e.getMessage(), 30);
}
Console.displayPaddedKeyValueExceptionTrace("Exception Trace", ExceptionBatteries.getStackTraceAsString(e), 30);
Console.markerError(80);
}
public synchronized static void displayPaddedKeyValueExceptionTrace(String key, String value, int leftPadding) {
String rightPad = String.format("%%%ds", leftPadding + 2);
String cleanedValue = value.replace(separator, "|").replace("\t", " "); // .replace("\t",
// String.format(rightPad,
// ""));
String fmt = String.format("| %%-%ds| %%s", leftPadding);
String message = String.format(fmt, key, cleanedValue);
boolean shouldPrint = logToCentralLog(message);
if (shouldPrint) {
cleanedValue = value.replace(separator, separator + "|" + String.format(rightPad, "|")); // .replace("\t",
// String.format(rightPad,
// ""));
message = String.format(fmt, key, cleanedValue);
errorForConsole(message);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy