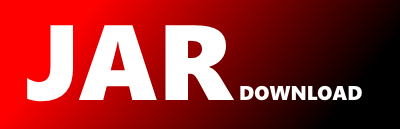
arjuna.lib.sysauto.process.ProcessOutput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arjuna-java Show documentation
Show all versions of arjuna-java Show documentation
Arjuna-Java is the client implementation in Java for development of test automation using Arjuna. It uses TestNG as the test engine. With minor tweaks, it can be used with any other test engine or custom test automation implementations. Arjuna is a Python based test automation framework developed by Rahul Verma (www.rahulverma.net)
The newest version!
package arjuna.lib.sysauto.process;
import java.io.IOException;
public class ProcessOutput {
private Process proc = null;
private String stdout = null;
private String stderr = null;
private int returnCode = -1000;
private ThreadSafeStreamReader errorGobbler;
private ThreadSafeStreamReader outputGobbler;
public ProcessOutput(Process proc) throws Exception {
this.proc = proc;
// any error message?
errorGobbler = new ThreadSafeStreamReader(proc.getErrorStream(), "ERROR");
errorGobbler.setPriority(Thread.MAX_PRIORITY);
// any output?
outputGobbler = new ThreadSafeStreamReader(proc.getInputStream(), "OUTPUT");
outputGobbler.setPriority(Thread.MAX_PRIORITY);
// kick them off
errorGobbler.start();
outputGobbler.start();
}
public void waitUntilComplete() throws Exception{
int exitVal = proc.waitFor();
this.stderr = errorGobbler.getOutput();
this.stdout = outputGobbler.getOutput();
this.returnCode = exitVal;
}
public void waitForLaunch() throws Exception {
Thread.sleep(2000);
proc.getOutputStream().close();
this.stderr = errorGobbler.getOutput();
this.stdout = outputGobbler.getOutput();
// this.returnCode = exitVal;
}
public boolean isProcessAlive() {
return this.proc.isAlive();
}
public String getStdoutText() {
return this.stdout;
}
public String getStderrText() {
return this.stderr;
}
public int getReturnCode() {
return this.returnCode;
}
public void exitProcess() throws Exception{
proc.destroy();
Thread.sleep(1000);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy