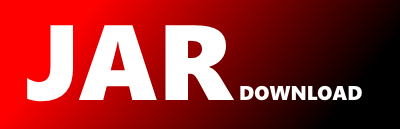
arjuna.lib.value.AbstractValueList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arjuna-java Show documentation
Show all versions of arjuna-java Show documentation
Arjuna-Java is the client implementation in Java for development of test automation using Arjuna. It uses TestNG as the test engine. With minor tweaks, it can be used with any other test engine or custom test automation implementations. Arjuna is a Python based test automation framework developed by Rahul Verma (www.rahulverma.net)
The newest version!
package arjuna.lib.value;
import java.util.ArrayList;
import java.util.List;
import arjuna.tpi.value.Value;
public class AbstractValueList implements RWValueList{
private List values = null;
public AbstractValueList() {
this.values = new ArrayList();
}
public AbstractValueList(Object[] values) {
this();
this.addAllObjects(values);
}
public AbstractValueList(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy