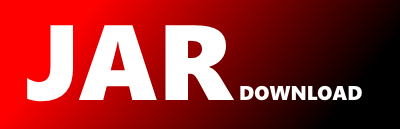
com.testvagrant.monitor.helpers.TestCaseHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of optimus-monitor Show documentation
Show all versions of optimus-monitor Show documentation
The Java Client provides access to Optimus cloud for all java based projects.
The newest version!
/*
* Copyright (c) 2017. TestVagrant Technologies
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.testvagrant.monitor.helpers;
import com.testvagrant.monitor.entities.TestCase;
import org.apache.commons.io.FilenameUtils;
import org.apache.commons.lang3.SystemUtils;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import static com.google.common.base.Preconditions.checkNotNull;
public class TestCaseHelper {
private TestCase testCase;
private Matcher matcher;
public TestCaseHelper(TestCase testCase) {
this.testCase = testCase;
}
public String getUniqueScenarioName() {
checkNotNull(testCase);
String scenarioNameString = getScenarioName() + "-" + getLineNumber();
return scenarioNameString;
}
@Deprecated
private Matcher getMatcher() {
Pattern p = Pattern.compile("((.*?);)(.*)(;;[0-9+])?");
String id = testCase.getId();
String[] split = id.split(":");
String featureName = FilenameUtils.getBaseName(split[0]);
String lineNumber = split[1];
String name = testCase.getName();
String input = featureName + ";" + name + ";;" + lineNumber;
Matcher matcher = p.matcher(input);
System.out.println("unique scenario name -- " + input);
matcher.find();
return matcher;
}
//Getting line number from the uri string with changes according to cucumber 2.0.1
public String getLineNumber() {
String id = testCase.getId();
if (id.contains(":")) {
if (SystemUtils.IS_OS_WINDOWS) {
int colonIndex = id.lastIndexOf(":");
return id.substring(colonIndex + 1, id.length());
} else {
String[] uriArray = id.split(":");
if (uriArray.length > 1)
return uriArray[1];
}
}
return "0";
}
private String getScenarioName() {
return testCase.getName().replaceAll(" ", "-");
}
public String getParentFeatureName() {
return matcher.group(2);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy