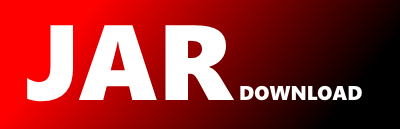
com.theagilemonkeys.meets.magento.models.MeetsMageProduct Maven / Gradle / Ivy
package com.theagilemonkeys.meets.magento.models;
import com.theagilemonkeys.meets.apimethod.ApiMethodModelHelper;
import com.theagilemonkeys.meets.magento.methods.CatalogProductGetSuperAttributes;
import com.theagilemonkeys.meets.magento.methods.Products;
import com.theagilemonkeys.meets.magento.models.base.MeetsMageModel;
import com.theagilemonkeys.meets.models.MeetsProduct;
import com.theagilemonkeys.meets.utils.StringUtils;
import org.jdeferred.DoneCallback;
import java.util.*;
/**
* Android Meets SDK
* Original work Copyright (c) 2014 [TheAgileMonkeys]
*
* @author Álvaro López Espinosa
*/
@SuppressWarnings("unchecked")
public class MeetsMageProduct extends MeetsMageModel implements MeetsProduct {
private int entity_id;
private String product_id; //This is the same as entity_id, but some Soap calls returns it with different names
private String sku;
private String type_id;
private String name;
private String description;
private String short_description;
private Object image_url;
private Double final_price_with_tax;
private String price;
private transient List associatedProducs;
private transient List images;
private transient List attributes;
private transient ConfigurationsData configurationData;
private transient Configuration configuration;
@Override
public MeetsProduct fetch() {
ApiMethodModelHelper.DelayedParams params = new ApiMethodModelHelper.DelayedParams() {
@Override
public List buildUrlExtraSegments() {
return Arrays.asList(String.valueOf(getId()));
}
};
pushMethod(new Products(), params)
.done(updateFromResult)
.always(triggerListeners);
return this;
}
////////////////////// Property getters and setters////////////////////////
@Override
public int getId() {
return entity_id > 0 ? entity_id : Integer.parseInt(product_id);
}
@Override
public MeetsProduct setId(int id) {
entity_id = id;
product_id = String.valueOf(id);
return this;
}
@Override
public String getSku() {
return sku;
}
@Override
public String getType() {
return type_id;
}
@Override
public String getName() {
return name;
}
@Override
public String getDescription() {
String res = StringUtils.safeValueOf(description);
if ( res.length() <= 0 )
res = StringUtils.safeValueOf(short_description);
return res;
}
@Override
public Object getImageUrl() {
return image_url;
}
@Override
public List getImages() {
return images;
}
// @Override
// public List getAttributes() {
// return attributes;
// }
@Override
public double getPrice() {
return final_price_with_tax != null ? final_price_with_tax
: Double.parseDouble(price);
}
@Override
public double getPriceOfConfiguration(Configuration config) {
double totalPrice = getPrice();
if(configurationData != null && config != null) {
totalPrice = configurationData.calculateTotalPrice(totalPrice, config);
}
return totalPrice;
}
@Override
public double getPriceOfSelectedConfiguration() {
return getPriceOfConfiguration(configuration);
}
@Override
public List getAssociatedProducts() {
return associatedProducs;
}
@Override
public MeetsProduct fetchImages() {
ApiMethodModelHelper.DelayedParams params = new ApiMethodModelHelper.DelayedParams() {
@Override
public List buildUrlExtraSegments() {
return Arrays.asList(String.valueOf(getId()), "images");
}
};
pushMethod(new Products().setResponseClass(null), params)
.done(new DoneCallback() {
@Override
public void onDone(Object result) {
images = new ArrayList();
for(Map imageData : (List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy