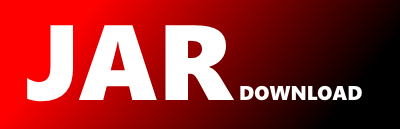
com.theicenet.cryptography.cipher.symmetric.SymmetricAEADCipherService Maven / Gradle / Ivy
/*
* Copyright 2019-2020 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.theicenet.cryptography.cipher.symmetric;
import java.io.InputStream;
import java.io.OutputStream;
import javax.crypto.SecretKey;
/**
* A SymmetricAEADCipherService instance is a component which encrypts and decrypts content
* using a secret key cryptography (symmetric cryptography) algorithm with a block cipher
* and a mode of operation which does require an initialisation vector (IV) and supports
* Authenticated Encryption with Associated Data (AEAD).
*
* @see Symmetric-key algorithm
* @see Block cipher
* @see Block cipher mode of operation
* @see Initialization vector (IV)
* @see Authenticated Encryption with Associated Data (AEAD)
*
* @apiNote Any implementation of this interface must be unconditionally thread-safe.
*
* @author Juan Fidalgo
* @since 1.2.0
*/
public interface SymmetricAEADCipherService {
/**
* Encrypts what is passed in clearContent using the secret key secretKey and the
* iv.
*
* @param secretKey secret key to use to encrypt the passed clearContent
* @param iv initialization vector to use to encrypt the passed clearContent
* @param clearContent clear content to encrypt using secretKey and the iv
* @param associatedData associated data (cleartext) to be included to produce the authentication tag
* @return result of encrypting clearContent with the secretKey and the iv
* by using a secret key cryptography algorithm
*/
byte[] encrypt(SecretKey secretKey, byte[] iv, byte[] clearContent, byte[]... associatedData);
/**
* Decrypts what is passed in encryptedContent using the secret key privateKey and
* the iv.
*
* @param secretKey secret key to use to decrypt the passed encryptedContent
* @param iv initialization vector to use to decrypt the passed encryptedContent
* @param encryptedContent encrypted content to decrypt using secretKey and the iv
* @param associatedData associated data (cleartext) to be included to validate the authentication tag
* @return the clear content, which is the result of decrypting encryptedContent
* with the secretKey and the iv by using a secret key cryptography
* algorithm
*/
byte[] decrypt(SecretKey secretKey, byte[] iv, byte[] encryptedContent, byte[]... associatedData);
/**
*
* Encrypts what is passed in clearContentInputStream using the secret key secretKey
* and the iv and sends the encrypted result to encryptedContentOutputStream.
*
* @apiNote Once this method returns the input and output streams must have been closed
* so they can't be mutated.
*
* @param secretKey secretKey secret key to use to encrypt the input clearContentInputStream
* @param iv initialization vector to use to encrypt the passed clearContentInputStream
* @param associatedData associated data (cleartext) to be included to produce the authentication tag
* @param clearContentInputStream input stream with clear content to encrypt using secretKey
* and the iv
* @param encryptedContentOutputStream output stream where is sent the result of encrypting
* clearContentInputStream with the secretKey
* and the iv by using a secret key cryptography
* algorithm
*/
void encrypt(
SecretKey secretKey,
byte[] iv,
InputStream clearContentInputStream,
OutputStream encryptedContentOutputStream,
byte[]... associatedData);
/**
* Decrypts what is passed in encryptedContentInputStream using the secret key
* secretKey and the iv and sends the decrypted result
* to clearContentOutputStream.
*
* @apiNote Once this method returns the input and output streams must have been closed
* so they can't be mutated.
*
* @param secretKey secret key to use to decrypt the input encryptedContentInputStream
* @param iv initialization vector to use to decrypt the passed encryptedContentInputStream
* @param associatedData associated data (cleartext) to be included to validate the authentication tag
* @param encryptedContentInputStream input stream with encrypted content to decrypt using
* secretKey and the iv
* @param clearContentOutputStream output stream where is sent the result of decrypting
* encryptedContentInputStream with the secretKey
* and the iv by using a secret key cryptography
* algorithm
*/
void decrypt(
SecretKey secretKey,
byte[] iv,
InputStream encryptedContentInputStream,
OutputStream clearContentOutputStream,
byte[]... associatedData);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy