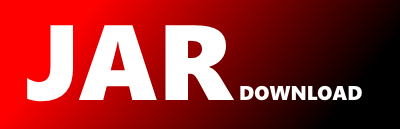
com.theicenet.cryptography.signature.SignatureService Maven / Gradle / Ivy
/*
* Copyright 2019-2020 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.theicenet.cryptography.signature;
import java.io.InputStream;
import java.security.PrivateKey;
import java.security.PublicKey;
/**
* A SignatureService instance is a component which implements a mechanism to work with
* public key cryptography (asymmetric cryptography) digital signature.
*
* @see Digital signature
* @see Public-key cryptography
*
* @apiNote Any implementation of this interface must be unconditionally thread-safe.
*
* @author Juan Fidalgo
* @since 1.0.0
*/
public interface SignatureService {
/**
* Calculates the signature of content using privateKey.
*
* @param privateKey private key to use to produce signature of content
* @param content content to produce signature with privateKey
* @return signature which is the result of signing content with the privateKey
*/
byte[] sign(PrivateKey privateKey, byte[] content);
/**
* Verifies if signature is a valid signature for content produced signing
* content with the private key pair of publicKey.
*
* @param publicKey public key which is the pair of the private key which was used to
* sign content to produce signature
* @param content content to verify that signature is a valid a signature produced signing
* content with the private key pair of publicKey
* @param signature signature to verify that it's a valid signature for content produced
* signing content with the private key pair of publicKey
* @return true if signature is a valid signature of content when signing using
* private key pair of publicKey. false otherwise.
*/
boolean verify(PublicKey publicKey, byte[] content, byte[] signature);
/**
* Calculates the signature of contentInputStream using privateKey.
*
* @apiNote Once this method returns the input stream must have been closed so it can't be mutated.
*
* @param privateKey private key to use to produce signature of contentInputStream
* @param contentInputStream input stream with content to produce signature
* with privateKey
* @return signature which is the result of signing contentInputStream with the
* privateKey
*/
byte[] sign(PrivateKey privateKey, InputStream contentInputStream);
/**
* Verifies if signature is a valid signature for contentInputStream produced
* signing contentInputStream with the private key pair of publicKey.
*
* @apiNote Once this method returns the input stream must have been closed so it can't be mutated.
*
* @param publicKey public key which is the pair of the private key which was used to
* sign contentInputStream to produce signature
* @param contentInputStream input stream with content to verify that signature is a valid
* signature produced signing contentInputStream with the private
* key pair of publicKey
* @param signature signature to verify that it's a valid signature for contentInputStream
* produced signing contentInputStream with the private key pair of
* publicKey
* @return true if signature is a valid signature of contentInputStream when signing
* using private key pair of publicKey. false otherwise.
*/
boolean verify(PublicKey publicKey, InputStream contentInputStream, byte[] signature);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy