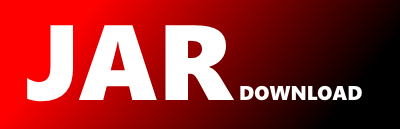
com.jfreeman.lazy.QuadThunk Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lazy Show documentation
Show all versions of lazy Show documentation
A library for type-safe, tractable lazy evaluation and late binding in Java.
package com.jfreeman.lazy;
import com.google.common.collect.ImmutableList;
import com.jfreeman.function.QuadFunction;
/**
* A lazy value with four dependencies.
*
* @param the type of the value
* @param the type of the first dependency
* @param the type of the second dependency
* @param the type of the third dependency
* @param the type of the fourth dependency
* @author jfreeman
*/
public final class QuadThunk
extends AbstractThunk
{
private Lazy _depA;
private Lazy _depB;
private Lazy _depC;
private Lazy _depD;
private QuadFunction _func;
private QuadThunk(
Lazy a, Lazy b, Lazy c, Lazy d,
QuadFunction func)
{
_depA = a;
_depB = b;
_depC = c;
_depD = d;
_func = func;
}
public static QuadThunk of(
Lazy a, Lazy b, Lazy c, Lazy d,
QuadFunction func)
{
return new QuadThunk<>(a, b, c, d, func);
}
@Override
public boolean isForced() {
return _func == null;
}
@Override
public Iterable extends Lazy>> getDependencies()
throws IllegalStateException
{
if (isForced()) {
throw new IllegalStateException("already forced");
}
return ImmutableList.of(_depA, _depB, _depC, _depD);
}
@Override
public T force()
throws IllegalStateException
{
if (isForced()) {
throw new IllegalStateException("already forced");
}
final A a = _depA.getValue();
final B b = _depB.getValue();
final C c = _depC.getValue();
final D d = _depD.getValue();
_value = _func.apply(a, b, c, d);
_func = null;
_depA = null;
_depB = null;
_depC = null;
_depD = null;
return _value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy