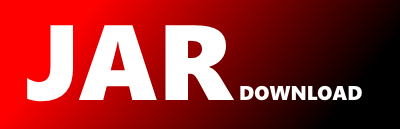
com.theoryinpractise.halbuilder.guava.Representations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of halbuilder-guava Show documentation
Show all versions of halbuilder-guava Show documentation
Google Guava Extensions to the HalBuilder Library
package com.theoryinpractise.halbuilder.guava;
import com.google.common.base.Function;
import com.google.common.base.Optional;
import com.google.common.base.Predicate;
import com.google.common.base.Predicates;
import com.theoryinpractise.halbuilder.api.Link;
import com.theoryinpractise.halbuilder.api.ReadableRepresentation;
import com.theoryinpractise.halbuilder.api.Representation;
import com.theoryinpractise.halbuilder.api.RepresentationException;
import com.theoryinpractise.halbuilder.impl.bytecode.InterfaceContract;
import com.theoryinpractise.halbuilder.impl.bytecode.InterfaceRenderer;
import java.net.URI;
import java.util.List;
public class Representations {
/**
* Add a link to this resource
*
* @param rel
* @param href The target href for the link, relative to the href of this resource.
*/
public static void withLink(Representation representation, String rel, String href, Predicate predicate) {
withLink(representation, rel, href,
Optional.of(predicate),
Optional.absent(),
Optional.absent(),
Optional.absent(),
Optional.absent());
}
/**
* Add a link to this resource
*
* @param rel
* @param uri The target URI for the link, possibly relative to the href of this resource.
*/
public static void withLink(Representation representation, String rel, URI uri, Predicate predicate) {
withLink(representation, rel, uri.toASCIIString(), predicate);
}
/**
* Add a link to this resource
*
* @param rel
* @param href The target href for the link, relative to the href of this resource.
*/
public static void withLink(Representation representation, String rel, String href, Optional> predicate, Optional name, Optional title, Optional hreflang, Optional profile) {
if (predicate.or(Predicates.alwaysTrue()).apply(representation)) {
representation.withLink(rel, href, name.orNull(), title.orNull(), hreflang.orNull(), profile.orNull());
}
}
/**
* Add a link to this resource
*
* @param rel
* @param uri The target URI for the link, possibly relative to the href of this resource.
*/
public static void withLink(Representation representation, String rel, URI uri, Optional> predicate, Optional name, Optional title, Optional hreflang, Optional profile) {
withLink(representation, rel, uri.toASCIIString(), predicate, name, title, hreflang, profile);
}
public static Optional tryResourceLink(ReadableRepresentation representation) {
return Optional.fromNullable(representation.getResourceLink());
}
public static Optional tryLinkByRel(ReadableRepresentation representation, String rel) {
return Optional.fromNullable(representation.getLinkByRel(rel));
}
/**
* Returns all embedded resources from the Representation that match the predicate
*
* @param predicate The predicate to check against in the embedded resources
* @return A List of matching objects (properties, links, resource)
*/
List extends ReadableRepresentation> getResources(ReadableRepresentation representation,
Predicate predicate) {
throw new UnsupportedOperationException("not implemented yet");
}
/**
* Returns a property from the Representation.
*
* @param name The property to return
* @return A Guava Optional for the property
*/
public static Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy