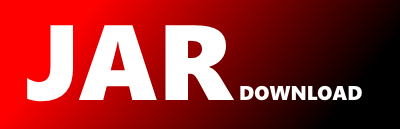
com.thesett.aima.logic.fol.Cons Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aima Show documentation
Show all versions of aima Show documentation
Library of code developed from 'Artificial Intelligence a Modern Approach', Prentice Hall.
/*
* Copyright The Sett Ltd, 2005 to 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.thesett.aima.logic.fol;
import java.util.Iterator;
import com.thesett.common.util.SequenceIterator;
/**
* Cons is a list conjoining functor. It always takes two argument, the next list element and the remainder of this
* list. This implementation exists purely for the sake of providing a different pretty printing method than standard
* functors.
*
*
CRC Card
* Responsibilities Collaborations
* Pretty print a list. {@link Term}.
* Provide a standard Java iterator over the recursive list.
*
*
* @author Rupert Smith
*/
public class Cons extends RecursiveList
{
/**
* Creates a cons functor. Two arguments must be specified.
*
* @param name The interned name of the cons functor.
* @param arguments The arguments; there must be two.
*/
public Cons(int name, Term[] arguments)
{
super(name, arguments);
if (arguments.length != 2)
{
throw new IllegalArgumentException("Cons must always take 2 arguments.");
}
}
/**
* Reports whether this list is the empty list 'nil'.
*
* @return true if this is the empty list 'nil'.
*/
public boolean isNil()
{
return false;
}
/**
* Provides a Java iterator over this recursively defined list.
*
* @return A Java iterator over this recursively defined list.
*/
public IteratorResponsibilities | Collaborations * |
---|---|
Provide a standard Java iterator over the recursive list. | {@link Cons}. * |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy