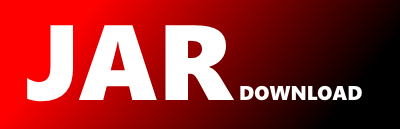
com.thesett.aima.logic.fol.compiler.BasicTraverser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aima Show documentation
Show all versions of aima Show documentation
Library of code developed from 'Artificial Intelligence a Modern Approach', Prentice Hall.
/*
* Copyright The Sett Ltd, 2005 to 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.thesett.aima.logic.fol.compiler;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.Queue;
import com.thesett.aima.logic.fol.Clause;
import com.thesett.aima.logic.fol.ClauseTraverser;
import com.thesett.aima.logic.fol.ClauseVisitor;
import com.thesett.aima.logic.fol.Functor;
import com.thesett.aima.logic.fol.FunctorTraverser;
import com.thesett.aima.logic.fol.Predicate;
import com.thesett.aima.logic.fol.PredicateTraverser;
import com.thesett.aima.logic.fol.PredicateVisitor;
import com.thesett.aima.logic.fol.Term;
import com.thesett.aima.search.Operator;
import com.thesett.aima.search.util.backtracking.Reversable;
import com.thesett.common.util.StackQueue;
/**
* BasicTraverser provides methods to produce reversible {@link Operator}s to transition over the structure of a
* {@link Term}. Structurally terms are built up as clauses, and functors, and default methods are provided to iterate
* over these constructions, with flags to set to determines whether heads or bodies of clauses are explored first, and
* whether the arguments of functors are explored left-to-right or right-to-left.
*
* A BasicTraverser can be extended to form a concrete traverser over terms by providing implementations of the
* methods to create operators for traversing into clause heads or bodies, and the arguments of functors. Any optional
* visit method to visit the root of a clause being traversed may be also be implemented, usually to initialize the root
* context of the traversal.
*
*
CRC Card
* Responsibilities Collaborations
* Establish an initial positional context upon visiting a clause.
* Provide traversal operators over clauses.
* Provide traversal operators over functors.
*
*
* @author Rupert Smith
*/
public abstract class BasicTraverser implements PredicateTraverser, ClauseTraverser, FunctorTraverser, ClauseVisitor,
PredicateVisitor
{
/** Used for debugging purposes. */
/* private static final Logger log = Logger.getLogger(BasicTraverser.class.getName()); */
/** Flag used to indicate that clause heads should come before bodies in the left-to-right ordering of clauses. */
protected boolean clauseHeadFirst;
/** Flag used to indicate that clause bodies should be taken in the intuitive left-to-right ordering. */
protected boolean leftToRightClauseBodies;
/** Flag used to indicate that predicate bodies should be taken in the intuitive left-to-right ordering. */
protected boolean leftToRightPredicateBodies;
/** Flag used to indicate that functor arguments should be taken in the intuitive left-to-right ordering. */
protected boolean leftToRightFunctorArgs;
/**
* Creates a traverser that uses the normal intuitive left-to-right traversal orderings for clauses and functors.
*/
public BasicTraverser()
{
clauseHeadFirst = true;
leftToRightClauseBodies = true;
leftToRightFunctorArgs = true;
leftToRightPredicateBodies = true;
}
/**
* Creates a traverser that uses the defined left-to-right traversal orderings for clauses and functors.
*
* @param clauseHeadFirst true to use the normal ordering, false for the reverse.
* @param leftToRightClauseBodies true to use the normal ordering, false for the reverse.
* @param leftToRightFunctorArgs true to use the normal ordering, false for the reverse.
*/
public BasicTraverser(boolean clauseHeadFirst, boolean leftToRightClauseBodies, boolean leftToRightFunctorArgs)
{
this.clauseHeadFirst = clauseHeadFirst;
this.leftToRightClauseBodies = leftToRightClauseBodies;
this.leftToRightFunctorArgs = leftToRightFunctorArgs;
}
/**
* {@inheritDoc}
*
* Can be used to visit a predicate, to set up an initial context for predicate traversals.
*/
public abstract void visit(Predicate predicate);
/**
* {@inheritDoc}
*
* Can be used to visit a clause, to set up an initial context for clause traversals.
*/
public abstract void visit(Clause clause);
/** {@inheritDoc} */
public IteratorResponsibilities | Collaborations * |
---|---|
Delegate to a stackable operation. * |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy